Mastering Swing in Java: A Deep Dive Guide
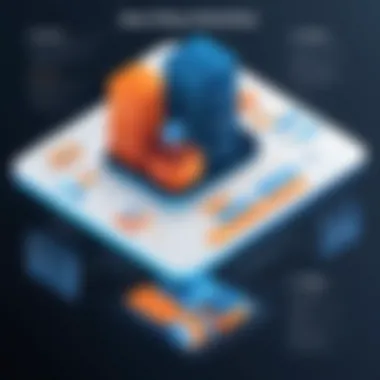
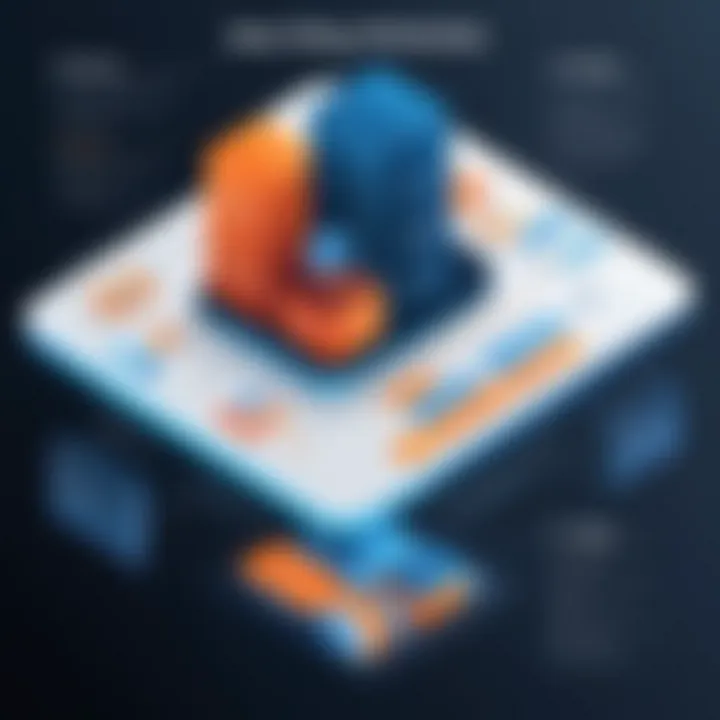
Intro
Swing is an important toolkit for developing graphical user interfaces (GUIs) in Java. It allows programmers to create rich, interactive applications that can run on various platforms without needing modification. This versatility makes Swing a favored choice among Java developers.
To properly understand Swing, it is useful to reflect on the fundamental concepts of Java as a programming language. Before we dive into the specifics of Swing, let’s first explore what Java entails and how it facilitates GUI development.
Preamble to Programming Language
History and Background
Java originated at Sun Microsystems in the mid-1990s. It was designed to be a simple, object-oriented programming language. Java gained popularity for its portability, allowing developers to write code once and run it on any platform that supports Java. The slogan "Write Once, Run Anywhere" has become synonymous with Java's appeal.
Features and Uses
Java supports various programming paradigms, notably object-oriented programming. Its key features include:
- Platform Independence: Java programs can run across different operating systems.
- Automatic Memory Management: Java handles memory allocation and deallocation, reducing memory leaks.
- Rich API: A comprehensive standard library that simplifies tasks in GUI development, network operations, and data manipulation.
The uses of Java are extensive, spanning from web applications to mobile apps and server-side applications. Its robustness and scalability make it suitable for enterprise-level solutions.
Popularity and Scope
Java holds a significant share in the programming realm. Its community is enormous, with numerous resources available for learners and professionals alike. According to various surveys, Java consistently ranks among the top programming languages. Its application in Android development, large systems, and cloud computing underlines its continuous relevance.
Overview of Swing
Swing is a part of the Java Foundation Classes (JFC) and provides a rich set of GUI components. It enables developers to build sophisticated UIs more easily than with the original Abstract Window Toolkit (AWT). Unlike AWT, Swing components are lightweight, meaning their functionalities are not tied to the underlying system. This independence enhances the appearance and behavior of applications.
Key Characteristics of Swing
- Lightweight Components: Swing's components are entirely written in Java, making them adaptable and consistent across platforms.
- Pluggable Look and Feel: Developers can customize the appearance of their applications using various styles.
- Event-Driven Programming: Swing operates on events, allowing better control over user interactions through listeners.
Basic Swing Components
Swing includes many components such as buttons, labels, text fields, and panels. Understanding these basic components forms the foundation for building complex interfaces.
Prolusion to Swing
Swing is essential for developing graphical user interfaces (GUIs) in Java. The framework builds on the Abstract Window Toolkit (AWT) but offers a more flexible and sophisticated approach to creating UIs. It allows developers to craft visually appealing and highly functional interfaces. This introduction serves as a foundation for understanding how Swing operates and its significance in Java application development.
What is Swing?
Swing is a part of the Java Foundation Classes (JFC) and provides a set of 'lighter' components that work on top of AWT. Unlike AWT, which relies on native platform components, Swing is fully written in Java. This characteristic enables Swing components to be rendered consistently across various operating systems. The flexibility comes from the decoupling of interface representation from the underlying OS, making it easier to maintain and update applications.
The core philosophy behind Swing revolves around the creation of rich client-side applications. With Swing, developers can use various UI components such as buttons, text fields, tables, and trees that are customizable and easily integrated into applications. Moreover, features like pluggable look-and-feel enable developers to change the appearance of applications without altering their code. This adaptability is crucial for creating modern applications that respond to user preferences.
History and Evolution of Swing
Swing’s journey began in the late 1990s when the need for a robust GUI toolkit became apparent. The first version of Swing was introduced with Java 1.2, dubbed the Java Foundation Classes. It was developed to address the limitations of AWT and to provide developers with advanced tools for GUI development. The evolution of Swing is closely tied to Java's growth as a programming language, emerging as a preferred choice for building enterprise-level applications.
Over the years, Swing received numerous updates, continually enhancing its capabilities. Each update incorporated user feedback and technological advances. The fundamental design of Swing remains largely unchanged, showcasing its stability and efficiency in application development. The integration of MVC (Model-View-Controller) architecture further allows for the separation of data, presentation, and user input, making Swing a powerful toolkit for complex applications.
"Swing remains a pivotal tool in Java's ecosystem, providing a foundation for building user-friendly interfaces that are scalable and maintainable."
Understanding Swing is vital for anyone wishing to dive deeper into Java application development. Its components and design principles form the backbone of effective user interaction. As programmers familiarize themselves with Swing, they gain the tools and methodologies to create more immersive user experiences.
The significance of learning Swing extends beyond its historical context; it is a stepping stone for future programming endeavors, especially for those aiming for a career in software development or UI design.
Swing vs. AWT
The relationship between Swing and AWT is crucial for understanding the graphical user interface capabilities in Java. AWT, or Abstract Window Toolkit, was the original API for creating GUI applications in Java. It provides a set of components that interact directly with the native operating system's graphical abilities. While AWT laid the foundation for GUI programming, Swing was introduced as an extension to provide more advanced and flexible features. Swing is built on top of AWT, which allows it to use its fundamental components, but it also adds its own lightweight components to ensure cross-platform compatibility.
Knowing how Swing relates to AWT helps in grasping the evolution of Java GUI development. Swing not only enhances user interface capabilities but also resolves many limitations found in AWT. This section will explore AWT in detail before diving into the significant differences between these two toolkits.
Understanding AWT
AWT is an open-source windowing toolkit that Java developers have used since its inception. It contains a set of graphical components such as buttons, scroll bars, and text fields. These components are considered heavyweight components, meaning they rely on the native systems for rendering. This characteristic can lead to differences in behavior and appearance across different operating systems.
Some key features of AWT include:
- Direct Connection to Native OS: Components are tied closely to the underlying operating system, meaning their rendering can vary by platform.
- Heavyweight Components: Each AWT component has a corresponding native system component, which can lead to resource overhead.
- Event Handling Model: AWT employs a simple event handling model that relies on the delegation event model.
AWT serves as the building blocks for Swing components. Understanding AWT is essential for grasping how Swing enhances those capabilities.
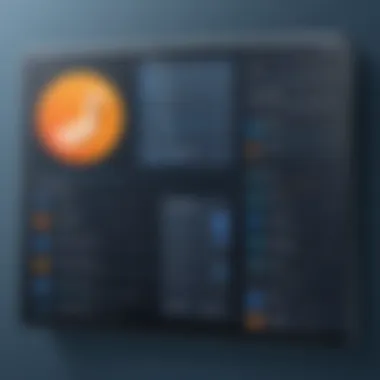
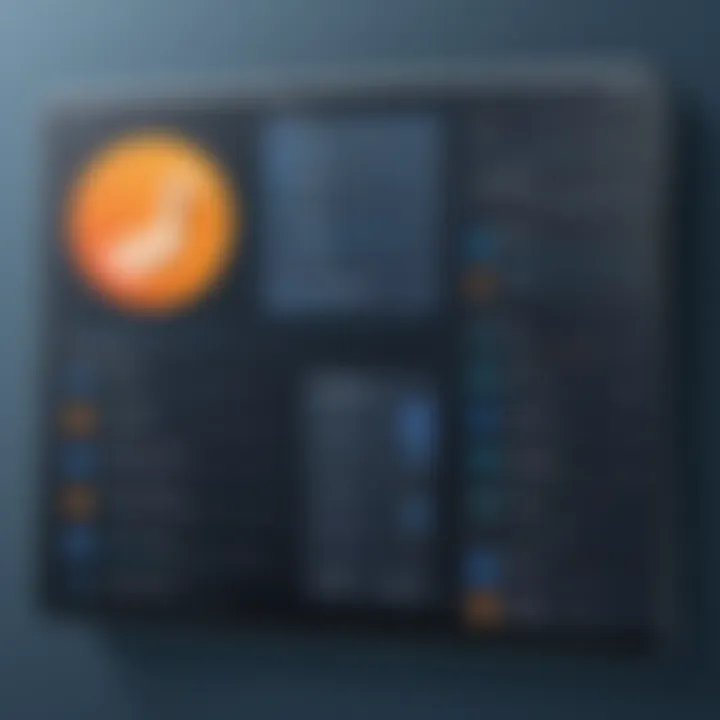
Key Differences between AWT and Swing
When comparing Swing and AWT, the distinctions in design, capabilities, and performance become clear. The following points summarize critical differences:
- Lightweight vs. Heavyweight: Swing uses lightweight components that do not rely on the native system for rendering, resulting in a more consistent UI across platforms.
- Rich Set of Components: Swing offers a more extensive set of components than AWT, including advanced elements like trees, tables, and trees that are not available in AWT.
- Look-and-Feel: Swing allows developers to easily change the look and feel of applications with several pre-defined themes. AWT lacks this flexibility and is generally limited to the native look.
- Performance: Because Swing manages its rendering, applications can be optimized for performance better than AWT applications.
- Threading Model: Swing uses a single-threaded rendering model while AWT might suffer from performance issues due to its reliance on heavyweight components.
In summary, while AWT set the foundation of Java GUI development, Swing has transformed it into a more powerful and flexible toolkit. Understanding these differences is crucial for developers seeking to create effective user interfaces.
Core Components of Swing
Understanding the core components of Swing is essential when working within the Java framework for building graphical user interfaces. The importance of these core components lies in their role as the building blocks for user interaction. Swing offers a variety of components that are flexible and adaptable to different design needs. Knowing how to effectively utilize these components is crucial for developers aiming to create intuitive and functional applications.
Swing components are lightweight and platform-independent. This means they can be used across different operating systems without altering the look and feel. Additionally, they provide a more advanced feature set than AWT components, which makes them preferable for modern applications. Each component serves a specific purpose and allows developers to create complex interfaces while maintaining user-friendliness.
JFrame: The Window
The JFrame component is the primary window for any Swing application. It acts as a container that holds various other components, providing the main visual environment for the program. When you create your first Swing application, JFrame is typically the first component you will instantiate.
JFrame allows for customization through methods to set title, size, and close operation. Here are some key points regarding JFrame:
- Title: The title is set when creating a JFrame instance. This title appears in the title bar of the window.
- Size: You can define the width and height of the JFrame. Setting the right size enhances user experience.
- Close Operation: It's important to define how the application behaves when the window is closed. For example, setting the default close operation to EXIT_ON_CLOSE ensures the application terminates when the window closes.
JButton: The Basic Button
JButton is one of the simplest yet most used components in Swing. It represents a clickable button, which can trigger actions when pressed. Developers use JButton for various purposes, such as submitting forms or executing tasks in response to user input.
Some notable features of JButton include:
- Labels: You can set text or icons as labels on a JButton, making it more informative.
- Event Handling: JButton can listen for action events. By adding action listeners, you can create interactive applications that respond to user commands.
- Custom Look and Feel: JButton can be styled differently based on the application’s theme, which enhances visual appeal.
JLabel: Displaying Text and Images
JLabel is a versatile component mainly used for displaying text or images on the interface. It does not respond to user input; rather it serves an informational purpose. This component is essential for guiding users by providing labels and information related to input fields.
Key features of JLabel include:
- Text Display: You can set text directly. For additional clarity, HTML formatting is also supported.
- Image Display: JLabel can show images alongside or instead of text. This capability allows for effective visual communication in applications.
- Alignment: You can align the text or images horizontally and vertically, improving layout design.
JTextField and JTextArea: Text Input
Input from users is fundamental in many applications. JTextField and JTextArea are Swing components designed for this purpose.
- JTextField: This is a single-line text input field. It's ideal for short entries like usernames or passwords. Key points include:
- JTextArea: Unlike JTextField, JTextArea allows for multi-line input. This is suitable for larger text, such as comments or descriptions. Important aspects include:
- Editable: Users can enter and edit their input.
- Placeholder Text: To guide users, you might implement placeholder text within the field.
- Line Wrap: You can enable line wrapping, which is helpful for readability.
- Scrolling: JTextArea supports scrolling, allowing users to scroll through long entries easily.
Swing Layout Managers
Swing Layout Managers play a crucial role in creating user interfaces in Java applications. They determine how components are arranged within a container, ensuring a visually appealing and organized layout. Without effective layout management, GUIs can become confusing and cumbersome for users. Understanding and utilizing various layout managers greatly enhances the user experience and the overall aesthetics of an application.
Preamble to Layout Management
Layout management is essential for developing responsive GUIs. In Swing, there are multiple layout managers, each designed for specific layout requirements. They automatically adjust the size and position of components based on the container's size and the components themselves. This adaptive nature is vital for cross-platform applications, as it allows the interface to function consistently across different devices and resolutions.
When managing layouts, developers must consider factors such as:
- Component alignment: Ensures that components are placed logically and intuitively.
- Dynamic resizing: Adapts to changes in the container size, providing flexibility and responsiveness.
- Component visibility: Important for prioritizing user interaction and experience.
FlowLayout
FlowLayout is a simple layout manager that arranges components in a left-to-right flow, similar to text in a paragraph. When the container is resized, components wrap to the next line as necessary. This layout manager is best suited for components of varying sizes, but it lacks versatility for more complex layouts. FlowLayout is default for JPanel, making it a common starting point for many Swing applications.
Features of FlowLayout include:
- Horizontal and vertical alignment options, allowing for customization.
- Automatic wrapping of components, ensuring adaptability.
- Simple implementation and easy usage.
Example of using FlowLayout:
BorderLayout
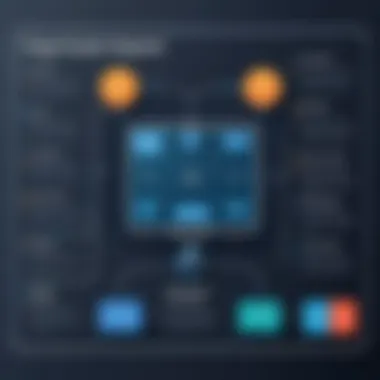
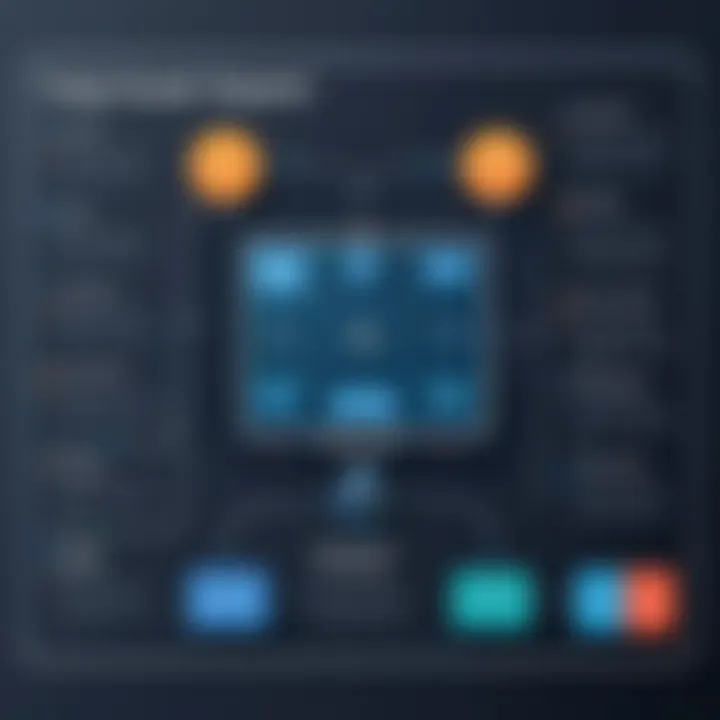
BorderLayout is a more structured layout manager that divides the container into five distinct areas: North, South, East, West, and Center. Each area can hold only one component, which makes it ideal for applications needing a clear hierarchy. This layout helps maintain a balance between the components, especially as window sizes change.
Key characteristics of BorderLayout:
- Enables efficient use of space by positioning components in a specific order.
- Facilitates easy addition and removal of components without disrupting the layout.
- Promotes a clean, organized appearance.
GridLayout
GridLayout divides the container into a grid of equally sized cells. Each cell can hold one component, and the user can specify both the number of rows and columns. This layout is particularly useful for applications with uniform components, such as buttons in a calculator or a grid of images.
Advantages of GridLayout include:
- Consistent component sizes and positioning, enhancing the visual balance.
- Easy management of multiple components in a systematic manner.
- Flexibility in adjusting the number of rows and columns by modifying the constructor parameters.
Overall, understanding Swing Layout Managers is vital for developers aiming to create responsive and adaptable user interfaces. Each layout manager has its strengths and applicability, catering to different design requirements.
Event Handling in Swing
Event handling is a crucial aspect of Swing programming. It forms the backbone of user interaction within Java GUI applications. Without effective event handling, a graphical user interface lacks responsiveness and functionality. Developers must carefully manage user actions and application responses to create a seamless experience. In this section, we will explore various elements of event handling, the benefits it provides, and key considerations that every developer should keep in mind.
Understanding Events
In the realm of Swing, an event is an action triggered by user interaction or by the program itself. Events can include mouse movements, button clicks, key presses, or window adjustments. Understanding how these events work is essential for building interactive applications.
When an event occurs, it generates an object that encapsulates details about the action performed. For example, if a user clicks a button, a is created, providing context such as coordinates of the click and which mouse button was pressed.
These events are the fuel for dynamic applications, allowing developers to create responsive interfaces that react to user actions. Without events, the applications would feel static and unengaging.
Event Listeners
Event listeners in Swing are interfaces that wait for a specific event to occur. They act as a bridge between the event generator and the event handler in the application. To handle an event, a developer must implement the listener interface appropriate for the event type. Some of the most common listener interfaces in Swing include:
- : Used for handling button clicks and menu selections.
- : Monitors mouse actions like clicks and entering/leaving components.
- : Listens for keyboard events, like key presses and releases.
Each listener interface includes methods that are invoked when an event occurs. For instance, the method in responds to action events. Here is an example snippet:
This code adds a listener to a button. When the button is clicked, "Button Clicked!" is printed to the console.
Understanding how to register listeners, handle events, and process event objects is fundamental to Swing programming.
Action Events and Key Events
Action events and key events are two primary categories of user-generated events in Swing applications.
Action Events occur when a user interacts with components like buttons or menu items. These events are typically used to trigger actions such as form submissions or switching views.
Key Events involve keyboard actions, allowing for text input and navigation within the application. Developers use key listeners to enhance user experience by providing functionalities like shortcuts or handling specific key combinations.
Here’s how action events works in a basic example:
In summary, effective handling of action and key events is necessary for producing applications that feel intuitive and responsive. This understanding leads to the creation of efficient, user-friendly interfaces that meet the needs of end-users.
"Event handling is not just about response; it’s about enhancing the user experience through thoughtful design and execution."
As Swing continues to evolve, mastering event handling remains essential for any developer seeking to create robust applications.
Creating a Simple Swing Application
Creating a simple Swing application serves as a foundational experience for anyone looking to understand GUI development in Java. It combines theoretical knowledge with practical application, allowing programmers to see how components interact within an environment. This process not only highlights the strengths of Swing, such as its flexibility and ease of use, but also lays the groundwork for more advanced topics. When you embark on designing a Swing application, you learn to manage both the graphical interface and the logical flows of user interaction.
Setting Up Your Environment
Before you start coding, setting up your development environment is crucial. You need to have the Java Development Kit (JDK) installed on your machine. The JDK allows you to compile and run Java applications.
- Download the JDK: You can visit the official Oracle website or OpenJDK for a safe download. Ensure you choose a version compatible with your operating system.
- Install an IDE: An Integrated Development Environment such as IntelliJ IDEA or Eclipse can significantly streamline your coding process. These tools provide useful features like code completion, debugging, and easy project management.
- Configure the IDE: After installation, configure your IDE to use the JDK you installed. This configuration allows the IDE to compile and run Java code smoothly.
Once your environment is ready, you can begin writing your Swing application.
Writing the Code
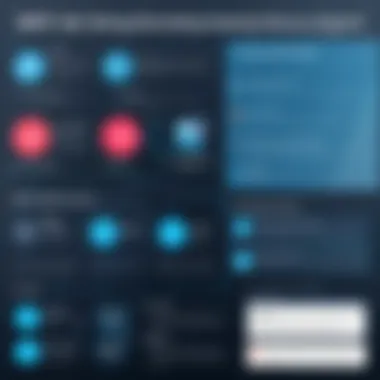
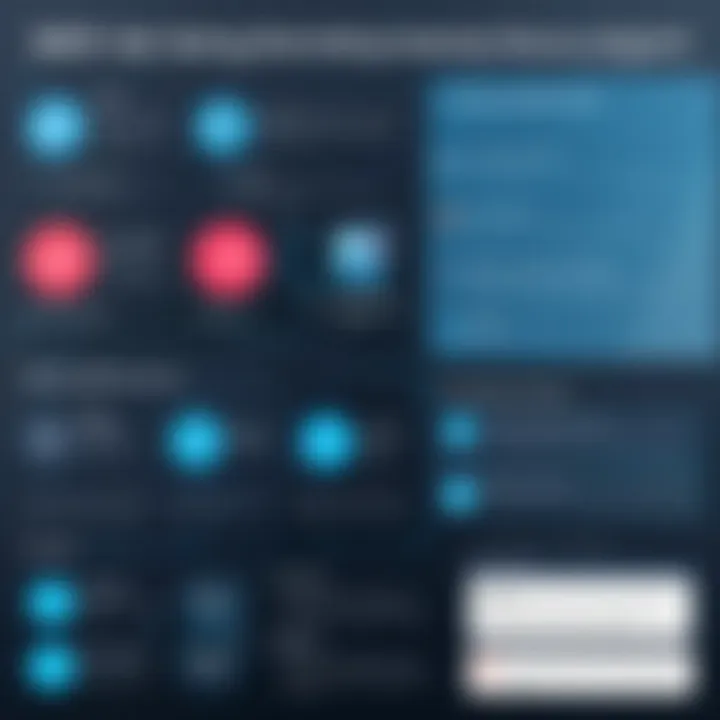
The actual coding process is where you will implement the components of your user interface. Start with a simple class that extends , which will serve as your main window.
Here’s a basic example to create a simplistic window:
In this code snippet, the constructor of the class sets up the window with a title and dimensions. The method makes the window appear. Running this code will display a simple window, demonstrating how easily one can create applications using Swing.
Running the Application
After writing the code, it’s time to run your application. Most IDEs provide a straightforward way to execute your code. Click the run button or use a shortcut key, usually Ctrl + R or Shift + F10, depending on your IDE.
- If you have set everything correctly, a window should pop up displaying your title and size.
- If you encounter any errors, check the console for error messages and troubleshoot according to the feedback.
Running your first Swing application is a pivotal moment in your learning journey. It gives you tangible results from the abstract concepts you have been studying.
"Programming is not just about writing code; it's about understanding the architecture behind it."
Best Practices for Swing Development
Developing applications using Swing requires an understanding of various best practices. These practices are essential not just for functionality but also for maintaining a favorable user experience. By following these guidelines, developers can create applications that are robust, efficient, and easy to navigate.
Consistent User Experience
A consistent user experience is vital in maintaining user satisfaction. When users interact with a Swing application, they expect similar behaviors and interfaces across different components. This predictability helps users feel comfortable navigating through the application. Here are a few strategies to ensure a consistent user experience:
- Use Common Layouts: Employ layout managers like FlowLayout or BorderLayout uniformly across the application. This ensures components align properly and fit well across various resolutions and screen sizes.
- Standardized Component Usage: Keep button styles, color schemes, and fonts consistent. Users can navigate and interact with the application intuitively if they know where to find elements with familiar appearances.
- Feedback Mechanisms: Provide immediate feedback for user actions, such as changing button colors when hovered over or displaying messages on successful actions. This helps to foster an understanding of what the application is doing.
- Accessibility Considerations: Ensure that the application is accessible to all users. This can include keyboard shortcuts and alternative text descriptions for graphical elements.
"User interface design is not just about aesthetics; it’s about creating functional and understandable interactions."
Efficient Resource Management
Resource management is another critical aspect of Swing development. Swing applications can be resource-intensive and must be optimized to avoid performance degradation. Efficient resource management involves:
- Minimizing Memory Consumption: Make use of lightweight components whenever possible. They consume fewer resources compared to heavy components, thus improving application responsiveness.
- Thread Management: Swing applications should use the Event Dispatch Thread (EDT) to ensure smooth interactions. Long-running tasks should be performed in separate threads to keep the EDT responsive. Consider using SwingWorker for this purpose.
- Dispose Unused Resources: Always clean up resources that are no longer needed, such as removing listeners or disposing of components. This helps in preventing memory leaks, which can crash applications over time.
- Optimize Painting Performance: The repainting process can be resource-heavy. Minimize the frequency of repaint calls and update only the parts of the interface that need changes. This conserves CPU usage and enhances the overall performance of the application.
Following these best practices can greatly improve the quality of Swing applications. Not only does it foster user satisfaction, but it also ensures that the application can handle a range of use cases effectively. A well-designed Swing application is not just easy to use; it also meets the performance expectations of today’s users.
Common Challenges in Swing Development
The development of graphical user interfaces using Swing does not come without its hurdles. Acknowledging these challenges is crucial for both novice and experienced developers. Understanding the difficulties can help in crafting better applications. In this section, we highlight two significant issues: GUI Responsiveness and Cross-Platform Compatibility. Addressing these elements is essential for enhancing user experience and ensuring application efficiency.
GUI Responsiveness
A responsive GUI remains central to user satisfaction. In Swing, maintaining responsiveness implies that the interface reacts promptly to user actions. However, Swing is single-threaded by nature. This means that if a long running task is happening in the Event Dispatch Thread (EDT), the user interface may freeze. This delay can lead to a frustrating experience for users who expect efficient interaction.
To mitigate this issue, developers can use background threads for long operations. The class is beneficial in this regard. It allows developers to perform lengthy tasks without freezing the user interface. Here is a basic example of using :
Implementing this pattern will keep the GUI responsive while performing intensive background operations. It is crucial to prioritize smooth performance when developing applications.
Cross-Platform Compatibility
Swing is designed to be platform-independent, allowing applications to run on various operating systems without significant changes. Nevertheless, achieving true cross-platform compatibility can pose its own set of challenges. Differences in operating systems can affect the appearance of Swing components and their behavior.
For instance, Swing relies on the underlying windowing system to render GUI elements. It is common to notice discrepancies in fonts, colors, and component sizes across platforms. To reduce these disparities, developers should focus on UI consistency. This can be accomplished by:
- Using a standard look-and-feel, such as , which is more uniform across systems.
- Testing applications on multiple platforms to identify and rectify inconsistencies before deployment.
- Avoiding extensive reliance on platform-specific features that can limit functionality on other systems.
By focusing on these considerations, developers can produce applications that maintain their intended functionality and visual integrity across different environments. In summary, confronting these challenges head-on is vital for ensuring success when developing with Swing. By enhancing GUI responsiveness and striving for cross-platform compatibility, programmers can create applications that not only function but also delight users.
Finale
In this section, we reflect on the significance of Swing as a GUI toolkit within the Java programming landscape. Understanding the conclusions drawn from our exploration of Swing helps to frame its value in software development. Swing is not just a collection of components; it is a framework that embodies principles which facilitate the creation of complex user interfaces.
Summarizing Key Points
Throughout the article, we discussed several key points that define Swing's role in Java application development. First, its robust architecture supports a wide range of GUI components, enabling developers to build intuitive interfaces. Second, the flexibility offered by Swing's layout managers aids in designing responsive applications that can adapt to various screen sizes.
Moreover, we examined the event handling mechanism which allows for effective interaction between users and the application, a cornerstone of any modern GUI. We also highlighted best practices for resource management and user experience consistency, which should be adhered to in any serious development endeavor.
In summary, understanding these fundamental aspects of Swing lays the foundation for effective programming and successful software application creation.
Future of Swing in Modern Development
Despite the emergence of newer frameworks and libraries, Swing continues to hold relevance in modern development. It is particularly notable in legacy systems where significant investments have been made. The knowledge of Swing is often a necessary skill for maintaining and enhancing these applications.
Additionally, with the rise of integrated development environments, the handling of Swing components has become more streamlined. This makes it accessible for new programmers. As Java evolves, the community may integrate Swing with more modern practices, such as making it compatible with JavaFX or using it within cloud-based applications.