Mastering Software Architecture: A Tactical Guide
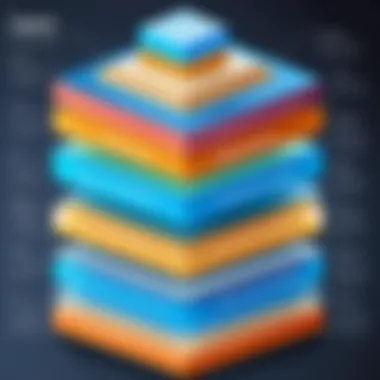
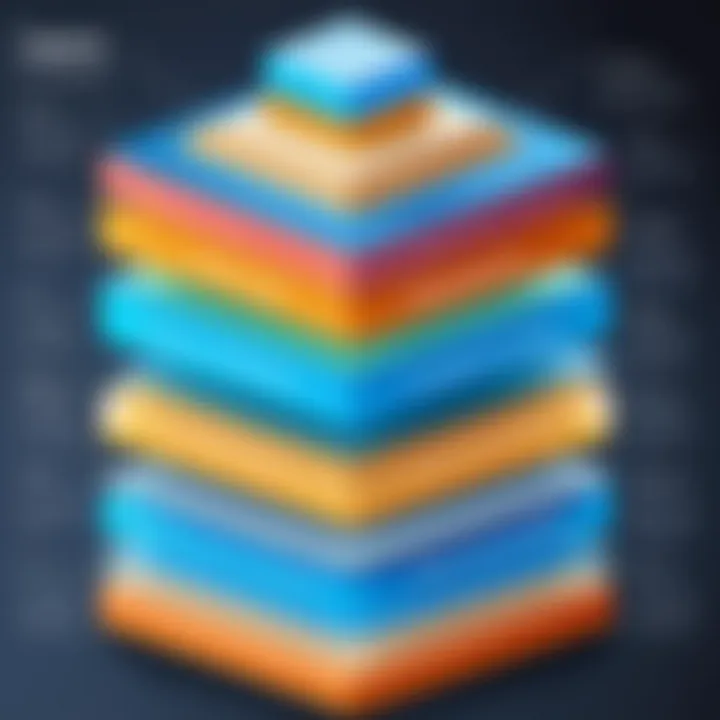
Intro
In the ever-evolving landscape of modern software development, understanding software architecture is akin to holding the blueprint to a city. Just as a city needs careful planning and structure to thrive, software systems require a robust architectural framework to function smoothly. This article aims to unravel the fundamental principles of software architecture, offering a blend of theoretical concepts and practical examples that will resonate with both beginners and those looking to deepen their expertise.
To embark on this journey, we will explore the landscape of programming languages, delve into their underlying concepts, and address how the choice of architecture influences the development process. With a comprehensive approach in mind, we aim to equip you with the knowledge that will help you navigate this intricate field with confidence.
Foreword to Programming Language
History and Background
Programming languages have evolved significantly since the first machine code was designed. In the mid-twentieth century, assembly languages emerged, which gave programmers more control over the hardware. As time marched on, higher-level languages like FORTRAN and COBOL broke grounds by providing more abstraction and ease of use.
Fast forward to today, we stand amidst a plethora of languages, each tailored for particular tasks and applications. Languages like Python, Java, and JavaScript dominate the landscape, wielding the art of versatility and community support.
Features and Uses
Each programming language comes with its quirks and capabilities. For example, Python delights many developers with its clear syntax and extensive libraries ideal for rapid development. Java, on the other hand, boasts robustness and portability, making it a staple in large-scale enterprise applications. JavaScript reigns supreme in web development, providing interactive elements that breathe life into static pages.
In practical terms, the functional necessity of a programming language often dictates its adoption. New developers should assess project needs and explore languages that align well with those goals.
Popularity and Scope
As of today, the landscape is characterized by a few heavyweights. According to various industry reports, Python continues to climb the charts, especially favored in data science and machine learning realms.
Java holds its ground primarily in large-scale systems, while JavaScript's ever-expanding domain of frontend, backend, and even mobile app development showcases its adaptability.
The popularity of a programming language often mirrors its community—a vibrant, supportive group can significantly enhance one's learning experience, steering newcomers toward robust resources and practical advice.
Basic Syntax and Concepts
Understanding the syntax is akin to learning the grammatical rules of a new language. It lays the groundwork for any coding endeavor.
Variables and Data Types
Variables are essentially containers that hold data values. In most programming languages, the data types dictate what kind of data can be stored, be it integers, strings, or floating-point numbers.
Consider this code snippet in Python:
Here, we have variables representing a person’s name, age, and height, showcasing the simplicity and readability of Python.
Operators and Expressions
Operators are the bread and butter of programming; they manipulate data and variables. From arithmetic operations, like addition and subtraction, to logical operations, such as and, or, not, they form the backbone of expressions used to evaluate conditions.
For instance:
The above expressions show how operators work to derive new values and make conditional evaluations.
Control Structures
Control structures guide the flow of execution in programs. They include conditionals like if-statements and loops such as for and while. A simple check on age might look like this:
This basic structure allows programs to make decisions based on conditions set by the developer.
Advanced Topics
Once you grasp the basics, diving deeper into advanced topics reveals the power and flexibility of programming.
Functions and Methods
Functions are reusable blocks of code that perform specific tasks. They help keep code organized and manageable. For example:
Reusability makes you a more efficient coder; write once, use anywhere.
Object-Oriented Programming
Object-oriented programming (OOP) introduces concepts like classes and objects, encapsulating data and behavior. It promotes code reusability and organization, enhancing long-term maintenance.
Exception Handling
Life’s unpredictable, and so is coding. Exception handling allows developers to gracefully manage errors without crashing the entire application, using constructs like try and except in Python.
Hands-On Examples
Theory, while essential, gains life through hands-on experience. Consider starting with simple programs, and progressively tackle more complex projects.
Simple Programs
Lightweight programs like a basic calculator can sharpen your skills.
Intermediate Projects
Once comfortable, consider creating a task manager app, integrating file handling to solidify your knowledge.
Code Snippets
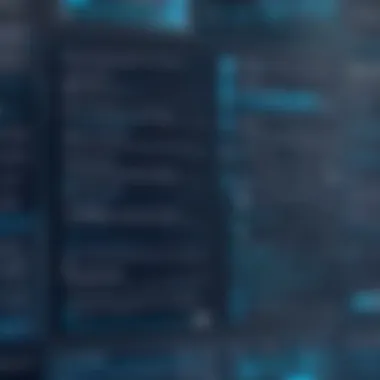
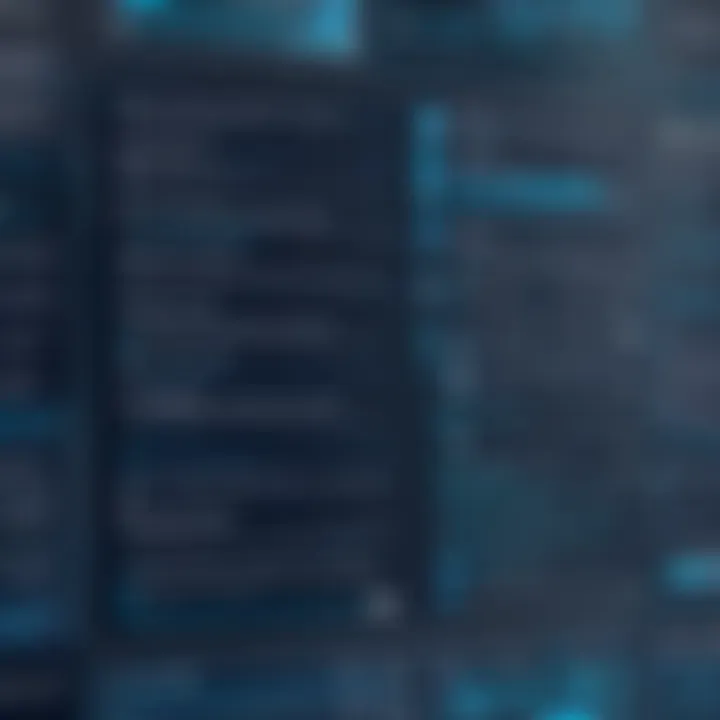
Keep an eye on community platforms like Reddit's /r/learnprogramming, where you can share code snippets and seek feedback.
Resources and Further Learning
As your understanding deepens, continue to seek knowledge and support from various resources.
Recommended Books and Tutorials
Books like "Automate the Boring Stuff with Python" can guide novice programmers.
Online Courses and Platforms
Platforms like Coursera and Udemy offer structured learning that can feed your curiosity.
Community Forums and Groups
Engagement in forums, such as Reddit or Facebook groups, allows for real-time feedback and sharing experiences with peers.
Frequent interactions with a community help polish your skills and understanding, making coding less of a lonely journey.
Prelims to Software Architecture
Software architecture is more than just a blueprint for development; it’s the very backbone of software design and implementation. This subject is critical in the realm of programming for various reasons, most notably because it lays the groundwork for understanding how different components of a system interact, scale, and evolve over time. Without a solid grasp of software architecture, developers risk creating applications that may solve immediate problems but falter under long-term demands.
Definition and Importance
Software architecture is defined as the structure of a software system, which includes the software components, their relationships, and principles guiding its design and evolution. It serves as a high-level framework that informs both business and technical decisions. One cannot overstate its importance: effective architecture supports adaptability, quality, and sustainability, reducing costs while improving efficiency in the long run.
In practical terms, the implications of poor architectural choices can be dire. For instance, if a company neglects to architect a system with scalability in mind, they could soon find themselves facing enormous challenges meeting user demands, leading to slower response times and disappointed customers.
Historical Evolution
The evolution of software architecture reflects the broader changes within the tech industry itself. In the early days of computing, architectures were often monolithic. The systems were tightly coupled, and any modification typically required considerable effort. As programmers encountered limitations, diverse architectural approaches began to emerge, including client-server models in the 1980s and evolving into n-tier architecture in the 1990s.
Fast forward to today, and we see a shift toward microservices and cloud-native architectures, which allow for tremendous flexibility and scaling. These transformations in software architecture are directly tied to the increasing demands for rapid development cycles and the need for robust, resilient systems capable of handling the modern Internet of Things (IoT) landscape.
Key Components of Software Architecture
When discussing software architecture, it’s essential to highlight its core components. Here are a few that are fundamental:
- Components: These are the building blocks of the architecture, including modules, classes, services, and more.
- Relationships: This highlights how components interact. A good architecture defines what these interactions look like, ensuring reliable connections and data flow.
- Principles: Fundamental guidelines that shape the decisions made during the design process. Key principles may touch upon concepts like reusability, scalability, and maintainability.
- Patterns: Reusable solutions that can be applied to common problems, acting as prescribed guidelines for addressing specific challenges in software design.
"Software architecture isn’t just a technical issue; it represents a crucial intersection where business goals and technological capabilities meet."
Understanding these components not only equips you to design better systems but also enables you to communicate effectively with stakeholders, ensuring that the architecture aligns with both technical and business needs. The overarching aim is to create an architecture that is resilient, adaptable, and capable of providing real value to users.
Fundamental Principles of Software Architecture
In the landscape of software development, the importance of foundational principles can't be overstated. The Framework for software architecture serves as the bedrock on which robust, maintainable, and scalable systems stand tall. As we peel back the layers of software architecture, we discover that these principles—namely Separation of Concerns, Modularity, and Abstraction—are essential for creating software that not only meets user expectations but also copes with future demands and changes. By adopting these principles, architects can navigate the complexities of modern software systems, leading to more efficient design and development processes.
Separation of Concerns
Separation of Concerns (SoC) emphasizes dividing a program into distinct sections, each addressing a different concern. This principle is crucial because it allows developers to isolate various aspects of a system, making it easier to understand, manage, and modify. For instance, take a simplistic blog application; one section can focus solely on the user interface, while another can handle data storage and retrieval. This distinction helps developers zero in on specific areas without being bogged down by unrelated functionality.
By implementing SoC, teams can also enhance collaboration. It enables parallel development, where multiple teams can work on different modules simultaneously, never stepping on each other’s toes. Furthermore, systems built with this principle are often easier to test. Testing can focus on one concern at a time, leading to more reliable outcomes. Overall, SoC one might even say, is the glue that holds the chaotic world of software development together.
Modularity
Modularity refers to breaking a system into smaller, manageable parts—modules—that can be developed, tested, and maintained independently. It's akin to putting together a jigsaw puzzle; each piece contributes to the big picture yet can be worked on separately. This means if a module needs a tweak or overhaul, developers can make those changes without disturbing the entire system.
One of the biggest benefits of modularity is reusability. Once a module is developed, it can be utilized in different projects without starting from scratch. For example, a payment processing module created for an e-commerce site can easily be adapted for use in a fundraising platform.
Modular systems also pave the way for scalability. If a particular feature of a software application grows, additional modules can be added to enhance functionality without major overhauls. Thus, modularity not only brings agility but also sets the stage for future growth and innovation.
Abstraction
Abstraction is the process of simplifying complex reality by modeling classes based on the essential properties and behaviors an object should have. This principle allows developers to focus on the high-level functionality without getting lost in the weeds of implementation details. For instance, when creating a user authentication system, developers can abstract away the intricate details of password handling and just interact with a simplified interface.
The merit of abstraction lies in risk mitigation. By hiding complexity, developers reduce the potential for errors, as users of the abstraction don't need to navigate all of its underlying complexities. Moreover, this leads to better maintainability. Suppose there’s a need to modify how authentication works—thanks to abstraction, developers can change the underlying code without affecting the systems that rely on this functionality. Ultimately, abstraction encourages cleaner code, which makes it easier for teams to onboard new members and maintain the system over time.
"Separation of Concerns, Modularity, and Abstraction form the triad of principles that underpin effective software architecture, guiding developers toward better design and implementation practices."
By grounding your software projects in these fundamental principles, you're not just improving individual projects; you're laying a sturdy foundation for your entire approach to software development, resulting in more sustainable and scalable solutions.
Architectural Styles and Patterns
Understanding architectural styles and patterns is essential for anyone involved in software engineering. These frameworks serve as blueprints for designing software systems and can influence various aspects such as maintainability, scalability, and performance. Designers need to carefully consider which style or pattern best fits the needs of their project as each has its unique traits and may cater to specific requirements or objectives.
Layered Architecture
Layered architecture stands as one of the most common designs in software development. This approach organizes code into layers, each with a distinct role, promoting separation of concerns. Generally, a typical layered architecture might have the following structure:
- Presentation Layer: This is the topmost layer, interfacing with users directly through graphical user interfaces or APIs. It translates user commands into tasks for the lower layers.
- Business Logic Layer: Here, business rules and operations reside. This layer executes the necessary actions dictated by the user input received from the presentation layer.
- Data Access Layer: This bottom layer manages the interactions with data storage, be it databases or other forms of persistent storage.
Choosing a layered approach simplifies team collaboration, as developers can specialize in a particular layer, leading to increased productivity. However, it can also introduce challenges like increased complexity and often a slower system performance due to inter-layer communication. It's a bit of a double-edged sword, requiring careful consideration during the design phase.
Microservices Architecture
Microservices architecture represents a more contemporary approach, where applications are broken down into small, independent services. Each component can be developed, deployed, and maintained separately, communicating via lightweight protocols.
Some key benefits of microservices include:
- Scalability: Because each service operates independently, it’s easier to scale parts of an application without needing to scale the entire system. This can lead to more efficient resource usage.
- Flexibility in Technology Stack: Teams can use different technologies and programming languages for different services, allowing for optimal solutions based on specific needs.
- Improved Fault Isolation: If one service fails, it has a reduced impact on the overall system. This is crucial for maintaining application reliability.
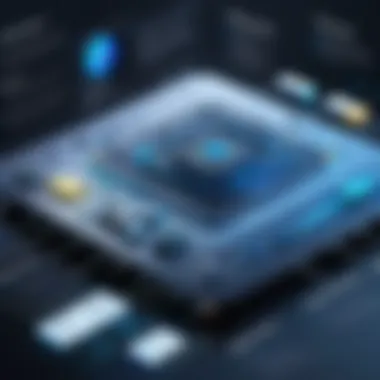
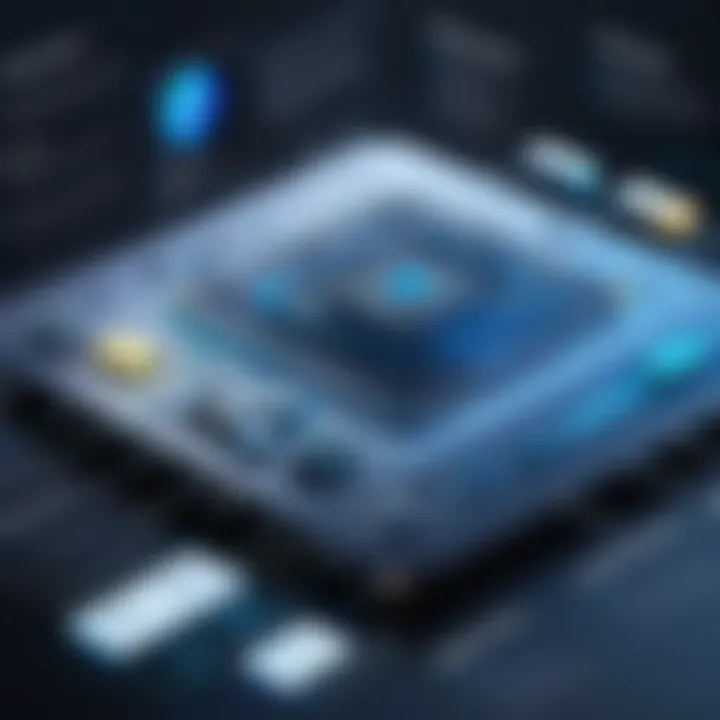
However, this architecture comes with its own set of challenges. For instance, managing multiple services can complicate deployment and monitoring efforts. Developers might encounter various difficulties related to service coordination and data consistency, which can be especially tricky.
Event-Driven Architecture
Event-driven architecture (EDA) revolves around the production, detection, consumption, and reaction to events. This style enables systems to be more reactive and adaptable to various input changes, which is a significant benefit in today's fast-paced environments.
The core components of EDA generally include:
- Event Producers: These generate events based on specific criteria or triggers, such as user actions or changes in data.
- Event Channels: Through which events are transmitted. This can be accomplished via message queues or event streams.
- Event Consumers: These trigger responses or actions as a result of the events they receive.
Implementing an event-driven architecture can enhance responsiveness and enable better resource optimization. Organizations often find it easier to scale components based on the event traffic they receive. However, decision-makers should remain cautious of complexities this architecture might introduce. For instance, debugging can become challenging because the flow of data isn't as linear compared to more traditional approaches.
"Choosing the right architectural style can be the difference between a well-functioning application and a convoluted mess that's hard to maintain."
In summary, architectural styles and patterns are fundamental when designing software systems. With the right strategy, teams can enhance not only the efficiency of their processes but also the quality of the final products. Understanding the nuances among various styles enables better decision-making tailored to project needs.
Designing Software Architecture
Designing software architecture is a crucial stage in the software development process. It sets the groundwork for everything that follows—from the initial coding to the final deployment. A well-thought-out architecture isn't just a technical necessity; it's a strategic framework that supports the entire project lifecycle. Key aspects such as scalability, maintainability, and performance hinge significantly on how the architecture is designed. When architects carefully consider their design choices, they not only solve immediate problems but also future-proof their systems for years to come.
Requirements Analysis
Understanding what the software needs to accomplish is where the rubber meets the road. Requirements analysis delves into capturing functional and non-functional requirements representing the expectations of stakeholders. This is not just about gathering a list of features but diving deep into user needs and business goals.
- Conduct interviews with stakeholders.
- Utilize use cases and user stories.
- Define performance metrics and constraints.
The aim is to build a clear and shared understanding of what the software should do, which ultimately shapes the architecture design. A common pitfall here is sparse or vague requirements, leading to costly revisions later on. As the saying goes, “A stitch in time saves nine.” Investing effort early pays dividends down the line.
System Modeling
System modeling translates requirements into visual representations of the architecture. This helps to clarify the relationships between components and how they interact. Various modeling tools, such as UML diagrams, can be used to depict the system's structure and behavior.
The primary models include:
- Class Diagrams: Show the system's classes and relationships.
- Sequence Diagrams: Illustrate how processes operate across different parts of the system.
- Component Diagrams: Detail how various components interact within the system.
By employing these diagrams, architects can detect potential issues early, much like a pilot using instruments to navigate through turbulence. Painstaking attention to detail in this phase can catch misalignments that could derail the software’s success in the actual implementation phase.
Documentation Practices
Documenting each decision and each element of the architecture is a practice that can’t be overlooked. Documentation practices serve as a critical reference point throughout the development lifecycle. It not only helps current teams but is also vital for new team members who may join later. A well-documented architecture can bridge gaps in understanding and streamline future development efforts.
Good documentation might include:
- Architectural decision records.
- Design specifications.
- Reasoning behind design choices.
To put simply, good documentation is like a map leading you through a maze of decisions. Without it, teams may easily stray from the original intent and face challenges that could have been avoided. An architect who understands the importance of writing down their thought processes is not just building software—they’re building a resilient foundation for future innovation.
"The art of architecture lies primarily in the understanding and representation of the interactions between the parts of a whole."
Tools and Technologies in Software Architecture
The world of software architecture continues to evolve, presenting a landscape replete with myriad tools and technologies that facilitate the design and implementation processes. The significance of embracing these tools cannot be overstated; they empower developers and architects to architect better systems with greater efficiency and flexibility. Adopting the right tools can impact the overall development lifecycle, influence team dynamics, and enhance project outcomes. This section dives into the nuances of various aspects, including architecture modeling tools, development frameworks, and testing and evaluation tools.
Architecture Modeling Tools
Architecture modeling tools serve as the backbone when it comes to designing systems. These tools provide a visual representation of the architecture, which can simplify complex concepts and enhance communication among team members. By offering diagrams and models, architects can better illustrate their vision, making it easier for stakeholders to grasp the project requirements and goals.
Some popular architecture modeling tools include Lucidchart, Visual Paradigm, and Sparx Systems Enterprise Architect. Each of these tools bring unique features to the table:
- Lucidchart is known for its intuitive drag-and-drop interface, allowing users to create flowcharts and diagrams easily.
- Visual Paradigm emphasizes collaboration, offering real-time editing features which can foster teamwork.
- Sparx Systems Enterprise Architect caters to more complex projects with robust support for UML modeling and enterprise-level architecture tools.
Utilizing these tools not only helps create detailed architectural models but also aids in documenting decisions that have been made throughout the development process. This documentation becomes an invaluable resource for future developers or team members who might work on the project later.
Development Frameworks
In the realm of software architecture, development frameworks serve as foundational building blocks that enable developers to streamline their coding efforts. These frameworks introduce a structure that allows for the rapid development of applications while encouraging best practices. Combining pre-built components and libraries, frameworks like Spring, Django, and Ruby on Rails allow developers to focus more on solving business challenges rather than getting bogged down in boilerplate code.
For instance, Spring is a comprehensive framework for Java that promotes best practices like dependency injection and aspect-oriented programming. With Django, developers can rapidly build web applications in Python, leveraging its robust security features and built-in admin panel. Meanwhile, Ruby on Rails emphasizes convention over configuration, helping developers to get applications up and running quickly.
Benefits of Using Frameworks:
- Reduce development time through reusable components.
- Encourage standardized coding practices.
- Provide built-in security features that help protect software.
Incorporating the right development framework can lead to enhanced maintainability, scalability, and overall product quality. It's about aligning the right tools with the project’s unique needs to foster more efficient software development processes.
Testing and Evaluation Tools
Testing and evaluation tools play a crucial role in the software development process, focusing on ensuring that the architecture meets both functional and performance requirements. These tools enable developers to identify bugs, assess system performance, and validate that the architecture adheres to specified requirements. Notable testing tools such as JUnit, Selenium, and Postman provide diverse functionalities that cater to various testing needs.
- JUnit specializes in unit testing, allowing developers to write and run tests against their code.
- Selenium offers powerful capabilities for automating web browsers and is widely used for functional testing.
- Postman simplifies API testing, making it easier for developers to send requests and validate responses.
Beyond just identifying issues, these tools contribute to better decision-making, as they furnish data-driven insights that allow developers to refine the architecture iteratively. Moreover, robust testing practices engender greater confidence in the deployment process, as the software becomes less prone to failures in production.
"Tools and technologies form the lifeline of effective software architecture, influencing every stage from design to deployment."
Challenges in Software Architecture
In the intricate landscape of software architecture, certain challenges loom large. These hurdles not only impede the progress of development but, if not addressed, can lead to catastrophic failures down the road. Tackling these issues is essential for professionals and learners alike to foster a robust understanding of software design principles and facilitate better outcomes. It's not just about crafting code; it’s about building scalable and maintainable systems that withstand the test of time. Understanding these challenges provides insights into how to strategically navigate the complexities involved.
Technical Debt
Technical debt refers to the long-term consequences of poor decisions in design or coding practices. Much like financial debt, it can accumulate quickly and burden the project over time, often leading to further complications. Developers often feel the pinch when they must choose between quick fixes and more sustainable solutions due to time pressures or limited resources. However, while it may seem easier to cut corners now, the price tag later can be substantial.
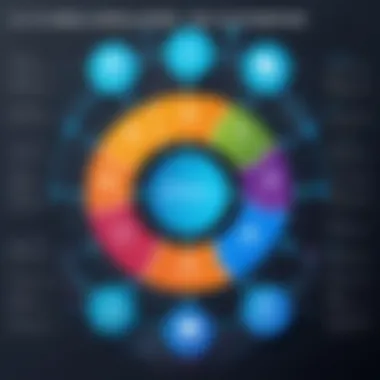
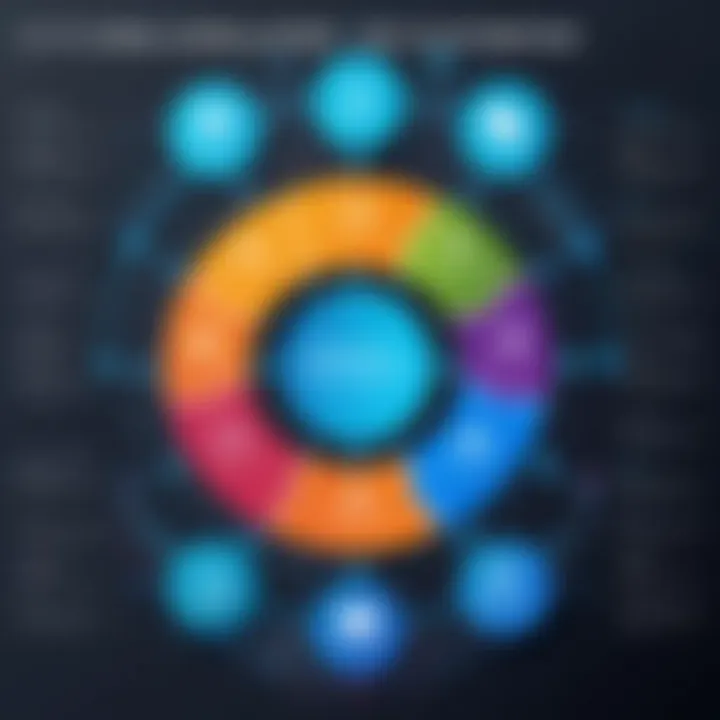
Key Aspects of Technical Debt
- Identification: Regular code audits and reviews can help in spotting instances of technical debt.
- Management: Establishing priorities for paying down debt can greatly benefit long-term project performance.
- Communication: Keeping all stakeholders informed helps maintain a clear understanding of trade-offs associated with technical debt.
"An ounce of prevention is worth a pound of cure." – Benjamin Franklin
Addressing technical debt proactively can lead to cleaner architectures and more robust systems. Developers should cultivate a mindset of cautious optimism, recognizing when quick solutions could lead to larger issues down the line.
Scalability Issues
Scalability is another formidable challenge in the realm of software architecture. As demands on the system increase—whether from more users, data, or features—the architecture must adapt to accommodate growth without compromising performance. Inadequate planning for scalability can result in sluggish applications that frustrate users and undermine business goals.
Strategies for Addressing Scalability
- Load Balancing: Distributing workloads efficiently across multiple servers can enhance performance.
- Horizontal Scaling: Adding more machines to handle the increase in load often proves more effective than simply beefing up existing servers.
- Performance Testing: Regularly conducting stress tests can help identify bottlenecks before they become critical issues.
Software architects must anticipate user growth and design systems that can expand gracefully. This foresight ensures that applications remain responsive and reliable under varying loads.
Maintaining Legacy Systems
Legacy systems present another layer of complexity. These older software solutions often rely on outdated languages or frameworks, making them difficult to maintain and integrate with modern technologies. Yet they frequently still play a vital role in business processes.
Considerations for Legacy Maintenance
- Assessment: Evaluate which parts of the legacy systems are essential versus those that can be phased out.
- Refactoring: Gradually rewriting components can modernize the system without a complete overhaul.
- Documentation: Thorough documentation helps both new and existing team members understand the system more effectively.
Striking a balance between maintaining these legacy systems and pursuing new developments can be quite a juggling act. Professionals must navigate this careful dance, ensuring that the old does not hinder the new.
In summation, understanding the challenges associated with software architecture—such as technical debt, scalability issues, and maintaining legacy systems—empowers software developers and architects to design solutions that are not just effective but also sustainable. Treading this path requires careful consideration, constant vigilance, and a proactive approach to design and implementation.
Real-World Applications of Software Architecture
The significance of software architecture in real-world applications cannot be overstated. It acts as the blueprint for software systems, influencing not just the technical aspects but also determining how software will interact with business objectives. By employing a structured approach to software design, organizations can craft more efficient, scalable, and maintainable applications that align closely with user needs and market demands. The practical implications of well-designed software architecture span multiple industries, from healthcare to finance, showcasing its versatility and critical role in achieving success.
Case Studies from Industry
When considering the tangible impact of software architecture, case studies from various sectors reveal successful implementations that stand as benchmarks for others. For instance, consider how Spotify employs a microservices architecture enabling teams to innovate rapidly. Each development team has the autonomy to deploy changes independent of others, reducing bottlenecks in the software delivery process. This architecture promotes both agility and a robust service quality.
In another example, Amazon leverages a layered architecture that helps in isolating concerns. This structure allows developers to modify one layer without affecting others, which is crucial for scaling and enhancing their vast portfolio of services. The result is a system that can accommodate a dynamic number of transactions—vital during peak shopping seasons like Black Friday. These case studies underline how strategic architectural choices can lead to significant advancements in operational efficiency.
Impact on Software Quality and Performance
The relationship between software architecture and overall quality is profound. A well-thought-out architecture directly contributes to software performance, maintainability, and resilience. For instance, employing event-driven architecture can effectively handle asynchronous workloads, which is crucial for applications needing to process large streams of data in real-time.
Performance enhancements often stem from selecting the appropriate architectural style. For example, systems designed with microservices can optimize resource allocation, allowing individual services to be scaled up or down based on demand. This agility supports higher throughput and lower latency, enhancing the end-user experience. Moreover, the quality assurance phase becomes noticeably less cumbersome, as modular designs enable targeted testing and fault isolation.
Business Implications
The architectural decisions made in software development go hand in hand with business goals. A strategic approach can lead to reduced operational costs and increased agility in response to market changes. For instance, adopting a modular architecture can facilitate quicker integration of new functionalities, which can respond promptly to customer feedback. This ability to pivot swiftly is a significant advantage in today's competitive landscape.
Furthermore, the long-term cost benefits associated with good software architecture—such as lower maintenance expenditures and improved scalability—can have a meaningful impact on the bottom line. Companies that prioritize architecture often find themselves with a product that not only meets current requirements but can also evolve as new needs arise. This adaptability is increasingly vital in an era where technological advancements are seemingly relentless.
Future Trends in Software Architecture
In an era where technology advances at the speed of light, understanding future trends in software architecture becomes crucial for developers and organizations alike. This section delves into the pivotal aspects of upcoming trends, emphasizing why they matter and the potential impact on software development practices. The relevance of these trends can't be overstated—they shape the landscape in which we operate, push efficiency, and inspire innovation.
Emerging Technologies
As we gaze into the crystal ball of software architecture, it's hard not to notice the rising tide of emerging technologies that are reshaping our field. Technologies like artificial intelligence, blockchain, and cloud computing are now common parlance, yet their implications for software architecture are profound.
- Artificial Intelligence: This technology is not just for data analysis anymore. It is becoming a key player in optimizing architectural decisions. With AI-enabled design tools, architects can simulate different configurations and predict outcomes. This means making informed decisions earlier in the design process, reducing the guesswork that often leads to expensive revisions later on.
- Blockchain: Traditionally a buzzword in the cryptocurrency world, blockchain's distributed ledger system has much broader applications. In software architecture, it paves the way for decentralization, enhancing security and transparency. Software systems built with blockchain can operate without a central authority, which could transform industries like finance and healthcare.
- Cloud Computing: Perhaps the most significant shift in recent years, cloud computing isn't just a storage solution; it’s an architectural paradigm. Utilizing cloud infrastructure enables scalability, flexibility, and accessibility. As microservices and serverless architectures rise, the ability to deploy systems on the cloud can significantly enhance performance while lowering costs.
In summary, being well-versed in these technologies equips developers with the tools to make more robust architectural choices.
Architectural Trends in Software Development
As software development evolves, so too do the architectural approaches that developers adopt. Several key trends are shaping the way software is designed, making it leaner, more responsive, and easier to maintain:
- Microservices: Moving away from monolithic systems, many organizations are embracing microservices architecture. This allows for the construction of applications as a suite of independently deployable services that can be developed, tested, and deployed separately. The benefit? Enhanced scalability and a reduction in dependencies—thereby allowing teams to innovate at their own pace.
- API-First Design: In today’s interconnected world, developing software that integrates seamlessly with other applications is vital. API-first design not only prioritizes the creation of APIs but also enables teams to visualize how components interact before diving into the code. This fosters a clear understanding among stakeholders and simplifies the integration of diverse systems.
- Containerization: Technologies like Docker and Kubernetes are revolutionizing the way software is deployed and managed. Containerization encapsulates an application and its dependencies together, ensuring it runs consistently across environments. This eliminates the classic “it works on my machine” scenario, pushing faster and more reliable deployments into the spotlight.
These trends push the envelope, nudging architects and developers to rethink how they approach problem-solving in software development.
Anticipated Challenges Ahead
With all advancements come challenges. As we discuss future trends, it’s essential to acknowledge the hurdles that might accompany them:
- Skill Gaps: As technology advances, so must the skills of the workforce. The rapid development of new tools often leaves teams scrambling to keep up, leading to a talent shortage in areas like AI and blockchain.
- Integration Issues: As applications become more modular and interconnected, ensuring that these diverse systems work together smoothly is crucial. Architects need to prioritize interoperability in their designs, which can often complicate integration processes.
- Security Concerns: With the rise of decentralized technologies like blockchain, new security challenges emerge. Architecting systems to safeguard data while maintaining user privacy will be at the forefront of development efforts. Constant vigilance is required to stay a step ahead of potential threats.
In summary, while the road ahead appears promising, it is fraught with challenges that require attention and proactive strategies. By acknowledging these potential pitfalls, developers can create more resilient architectures that stand the test of time.
"Embracing future trends in software architecture is not merely about adapting; it’s about strategically weaving innovation into the fabric of development processes."
The End
The conclusion of this article encapsulates the significance of understanding software architecture. In a world where software solutions dictate the pace of business, the architecture that underpins these solutions is crucial. Thoughtful architecture not only ensures that systems are robust and scalable, but also that they remain adaptable to a continually shifting technological landscape.
Summary of Key Concepts
Throughout the article, we covered a variety of essential topics. First, we identified software architecture as the bedrock of successful software design. Key principles like separation of concerns, modularity, and abstraction allow developers to manage complexity in their systems. Additionally, we explored various architectural styles – such as layered architecture and microservices – that offer diverse approaches to system design based on specific project needs.
The discussion on tools and technologies underscored their importance in both the planning and implementation phases of software development. From architecture modeling tools to testing frameworks, these resources enable developers to streamline their workflow and ensure high-quality outputs.
Moreover, the real-world applications highlighted the vital role architecture plays in enhancing software quality and business outcomes. Understanding the correlation between architectural choices and project success prepares developers to make informed decisions, ultimately leading to more efficient systems.
Emphasizing the Importance of Architecture
Software architecture holds a pivotal place in development for several reasons. Firstly, it influences product quality. A well-structured architecture can significantly reduce bugs and issues during both development and maintenance phases. If the underlying structure is flawed, it can lead to a cascade of problems that affect the entire project.
Secondly, architecture impacts team collaboration. With clear architectural guidelines, teamwork becomes more efficient. Developers can work independently on different parts of the system without stepping on each other’s toes. Furthermore, it enables new team members to understand the system quicker, making onboarding a smoother process.
Lastly, architecture supports scalability. As business requirements evolve, a flexible architecture allows for tweaks and enhancements without a complete overhaul of the existing system. The ability to integrate new functionalities without disrupting the entire service—this is what keeps businesses innovative and competitive.