Mastering Python's Find Function: A Comprehensive Guide
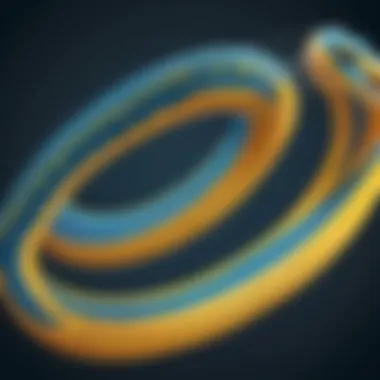
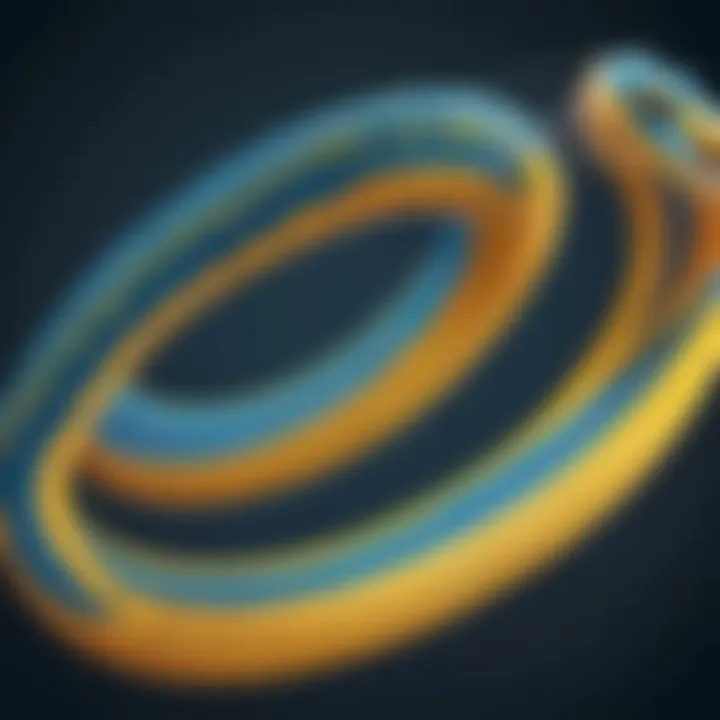
Intro
In the vast world of programming, Python stands out for its simplicity and versatility. At its core, one of Python's quintessential features is its ability to search and manipulate data, which brings us to the find functionality. Understood in multiple contexts, from strings to more complex data structures, the find function plays a critical role in programming tasks.
A Historical Perspective on Python
Before we dive into the nitty-gritty of the find function, it might be worth taking a step back to understand where it all began. Since its inception in the late 1980s by Guido van Rossum, Python has evolved, thanks to its clear syntax and ease of use. The language was designed to be read like plain English, making it accessible to both newbies and seasoned programmers alike.
Key Features of Python
Many features contribute to Python's popularity:
- Readable Syntax: The plain-language design allows for easier comprehension.
- Comprehensive Libraries: A plethora of libraries simplifies complex tasks like data analysis, web development, and more.
- Cross-Platform Compatibility: Whether on Linux, Windows, or macOS, Python remains consistent.
Additionally, Python is heavily utilized in diverse fields, whether itâs web development, data science, or machine learning.
The Relevance of the 'Find' Function
The find functionality is not merely a toolâit's a cornerstone for data navigation. Functionality can extend to searching characters in strings or even locating elements in lists. This versatility finds applicability in real-world scenarios, such as parsing user inputs or configuring settings in apps.
"Mastering the use of the find function allows developers to handle data more effectively, leading to cleaner and more efficient code."
Basic Concepts of the Find Function
Now, itâs time to peel back the layers of this functionality. In Python, the find method primarily exists to determine the position of a substring within a string. If the substring exists, it returns the starting index; otherwise, it yields -1. Letâs explore the syntax:
This code locates the word "World" within the string, showcasing the usefulness of the find method in determining the position of desired text within a larger body.
Application in Lists and Libraries
Beyond strings, the concept of finding elements extends into lists. While Python doesn't have a direct find method for lists, one can rely on the index method or employ list comprehensions to achieve similar results. For example:
Likewise, when it comes to external libraries, functions such as find can be integrated into larger frameworks or used with databases for more extensive tasks, particularly in data handling and search functionalities.
Limitations to Consider
Not everything about the find function is sunshine and rainbows. Though a useful tool, it has limitations:
- It only returns the first occurrence of the substring.
- It does not support case-insensitive searches directly.
- If the substring is not found, one has to check for -1, which could be easily overlooked.
Resources for Mastery
If you're keen to delve deeper into Pythonâs capabilities beyond the find functionality, here are some worthwhile resources:
- Books: "Automate the Boring Stuff with Python" by Al Sweigart.
- Online Courses: Platforms like Coursera and edX offer tailored Python courses for all levels.
- Forums: Engage with communities on Reddit or Facebook for discussions and insights.
In summary, understanding the find function is fundamental in mastering Python. Its utility in string manipulation and data searching gives programmers a powerful tool to enhance their coding workflows.
Preface to Finding Elements in Python
Finding elements within a data structure is a fundamental skill in any programming language, and Python is no exception. The capacity to identify and locate specific data can lead to efficient code and better problem-solving strategies. In this introductory section, we'll uncover the essentials of finding elements in Python, specifically its significance, benefits, and some key considerations that can shape how you approach searching within your code.
Understanding the Concept of 'Finding'
At its core, finding refers to the action of locating an element within a collection, be it a string, list, or any other data type. Python provides various built-in methods to facilitate this process.
Imagine looking for a needle in a haystack. Without a tool, your search could become cumbersome and time-consuming. Likewise, in Python, without the right function, finding specific information can take longer than necessary. The method in strings and the method in lists play crucial roles here. These functions let you effortlessly pinpoint elements and handle data more effectively.
So, why should a programmer, whether a novice or seasoned, care about this? Primarily, it enhances the capability to manipulate and manage data more efficiently. Whether youâre pulling usernames from a list or searching for a substring within a larger string, having a thorough grasp on how to utilize finding methods empowers your data-handling skills. This can ultimately translate to a more streamlined development process and a reduction in debugging time.
The Importance of Search Operations
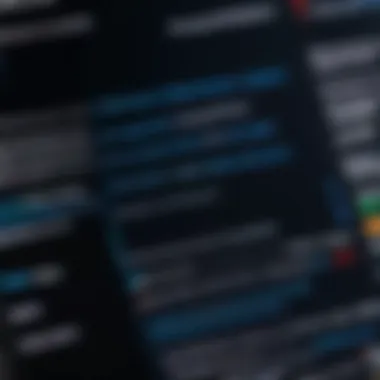
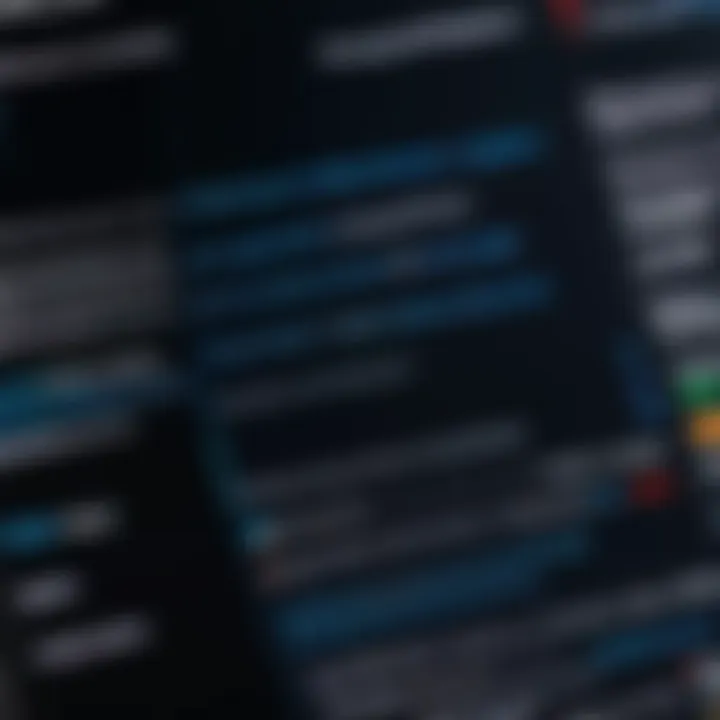
Search operations are not merely handy conveniences but essential tools in the programmer's repertoire. Conducting searches effectively can greatly influence the performance and scalability of applications. Here are several reasons why mastering search operations is vital:
- Efficiency: Quick and precise searches can drastically reduce the time complexity of your program, especially when dealing with large datasets.
- Debugging: When it comes to rooting out bugs, knowing how to find elements can assist in identifying issues in your logic or data structures.
- Data Integrity: Ensuring that the right data is being accessed and modified helps maintain the integrity of your application, preventing unwanted alterations.
- User Experience: Applications that feature fast, responsive searches often lead to better user satisfaction, as users expect their queries to yield immediate results.
"The faster you can find what you need, the more time you can spend building what comes next."
In summary, understanding how to effectively find elements in Python is not simply an academic exercise; it has real-world implications that can affect the performance and usability of your applications. As we delve deeper into the various methods and techniques for finding elements in Python, keep these concepts in mind, as they will lay the groundwork for more advanced topics later in this article.
Using String Find Methods in Python
When working with Python, string manipulation is often just part of the job. Youâre going to want an efficient way to identify and retrieve specific segments within text. That's where the string find methods come in; they provide a solid set of tools for this purpose. Whether you're trying to extract keywords for data analysis or simply checking for the presence of certain substrings in user inputs, these methods are invaluable.
By mastering the find functionality, programmers not only increase their efficiency but also enhance their overall coding proficiency. This section will dive into the intricacies of using string find methods, exploring their practical applications, limitations, and how they fit into broader programming tasks.
Foreword to String Methods
In the realm of Python, strings are more than just sequences of characters. They form the backbone of text processing. String methods, specifically, offer various actions one can perform with these sequences.
For instance, you can search, replace, split, or modify strings in numerous ways to suit your needs. Among these, the find methods stand out due to their simplicity and effectiveness. Understanding these methods involves getting comfortable with searching for substrings within larger stringsâa skill that seems trivial, yet is essential in real-world applications.
The Find Method: A Detailed Overview
At its core, the method does one thing: it locates a substring within a string and provides its index. If the substring isn't found, it gives back -1, signifying that the search was unsuccessful. Here's how you can use it:
This code snippet will print , as thatâs where the substring "world" begins in the text. The beauty of the find method is its versatility; you can also specify start and end indices to narrow down your search:
This version of the method searches for the letter 'o' starting from the 6th character.
Comparing Find with Rfind
While the method finds the first occurrence of a substring, does the oppositeâit finds the last. This distinction can be crucial depending on what you need.
For example, let's examine:
This demonstrates clearly how both methods serve different needs. Knowing when to use which can elevate your string manipulation skills considerably.
Handling Substring Searches
Searching for substrings isnât a one-size-fits-all scenario. You will most likely face situations where you need to adapt your searching technique based on context. Using methods such as and , can assist in these cases. These methods check whether a string begins or ends with a specified substring, returning a boolean value:
Additionally, using a combination of these methods can help create robust conditions for text validations or filtering.
Common Pitfalls When Using Find
While is handy, it does have its quirks that can trip up the unwary.
- Case Sensitivity: It is important to remember that searches are case-sensitive. So, "hello" and "Hello" would not match.
- Non-existence Without Warning: Returning -1 can sometimes lead to unregistered errors in logic if not handled properly.
- Efficiency on Large Texts: Repeated calls to the find method on extensive data can lead to inefficiencies. Always consider your data size and the frequency of searches.
Finding Elements in Lists
Finding elements in lists is a fundamental skill every Python programmer should master. Lists are one of the most common data structures in Python, and they are used in a myriad of contexts. Whether you're dealing with simple data collection or complex datasets, knowing how to effectively search for elements is essential. This not only enhances your coding speed but also boosts efficiency in your programs.
The techniques weâll discuss here will arm you with the necessary tools to locate elements quickly, assess their significance, and manipulate lists with more confidence. In addition, every method has its own nuances ensuring that you can pick the right tool for the job based on your specific scenario.
Finding Indexes Using Index Method
The method is a straightforward way to find the position of an element in a list. When you know exactly what you're looking for, this method can save you a lot of time. For example:
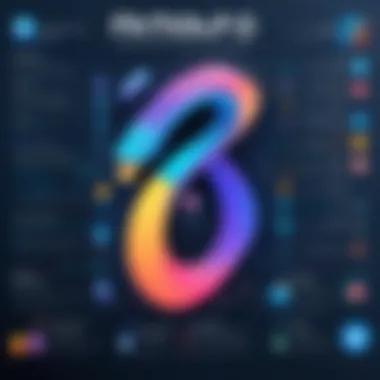
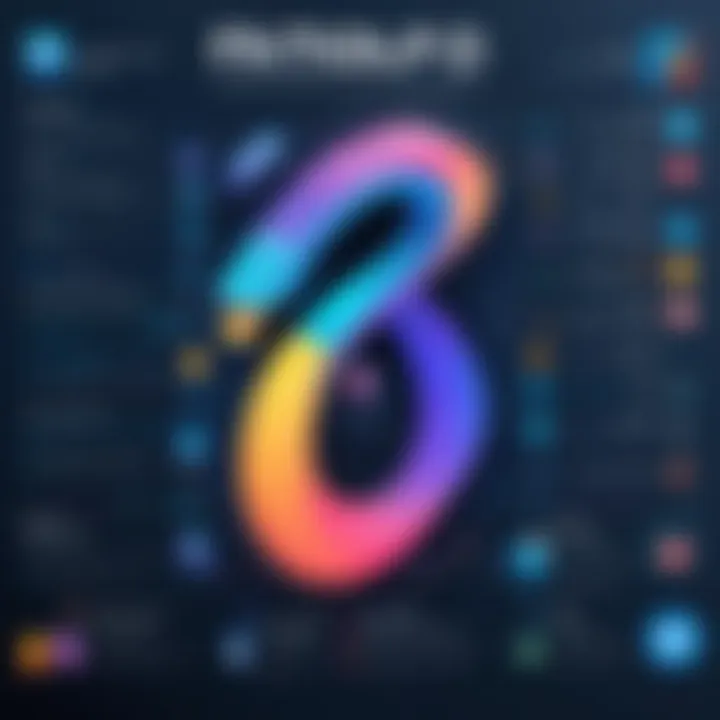
Here, using returns , meaning the number 15 is at the third position (indexes count starts from 0). However, there are a couple of things to keep in mind. If the element is not present in the list, Python raises a . You might want to handle this or check for existence beforehand.
Identifying Object Existence with 'in'
The keyword is a simple yet powerful feature for checking if an item exists within a list. Unlike the method, using does not throw errors, making it a safer option if you're uncertain about the presence of an element. An example would illustrate this better:
This is not just reserved for integers; you can find strings, float values, or even more complex objects. Pinpointing existence directly can be quite handy, especially when you're iterating through items or filtering data based on conditions.
Iterating Through Lists to Find Values
When the list is long or you need to apply conditions, you might end up iterating through the list. A simple loop can do the trick. This not only allows identification but also makes decisions based on your criteria. For example:
In this case, the loop checks each score and prints those above 85. Such an approach is flexible, allowing plenty of room to customize your actions on each item.
Using the function can make this process even smoother, as it provides both the index and the value of each item simultaneously. Hereâs how:
In the above scenario, gives both the index and value directly, making it easy to report on the data's position when needed.
Remember, effectively finding elements in lists can change the way you interact with data, allowing for more efficient coding practices over time.
To summarize, mastering these methods for finding elements in lists enhance your coding toolbox. You'll find using , , and iteration collectively will cover various situations, making your programs both robust and efficient.
Advanced Finding Techniques
The realm of programming is expansive, and as such, finding elements efficiently is a paramount skill for any aspiring programmer. While basic methods of finding elements in strings and lists are essential, there is great value in advancing our techniques. Advanced Finding Techniques empower developers to handle more complex search scenarios and enables finer control over the search process. These techniques help optimize code performance and usability, crucial for those wanting to harness Python's capabilities fully in practical applications.
Using Regular Expressions for Pattern Matching
Regular expressions (regex) serve as a powerful tool for pattern matching and searching through strings. This feature is particularly noteworthy when it comes to filtering data or extracting specific information from text strings that follow a certain format. Python's module provides a host of functions that facilitate pattern searching, including , , and .
The importance of this can be illustrated by considering a real-world application, such as validating email addresses. Regular expressions can effectively define the structure of a valid email, making them indispensable in scenarios that require data validation.
A critical aspect to remember when using regular expressions is their complexity. Crafting the correct regex patterns requires careful thought; a minor mistake can lead to unexpected results or missed matches. However, the benefits far outweigh the occasional challenges. Regular expressions come in handy in various fields, whether youâre manipulating strings, analyzing data, or scraping content from the web.
Leveraging List Comprehensions for Finding
List comprehensions can make finding values in lists both succinct and readable. This feature allows programmers to write a more compact code snippet, which can be especially handy when filtering lists based on certain conditions. By constructing a new list based on existing list elements that satisfy specific criteria, you save time and enhance code clarity.
For instance, imagine needing a list of even numbers from a larger list of integers. Instead of using traditional looping methods which can be cumbersome, you might simply implement a list comprehension:
Beyond readability, list comprehensions also cater to performance. Running a single-line expression often exhibits better efficiency than a for-loop, especially for larger datasets. This technique not only streamlines your code but also enhances its overall performance.
As with all techniques, itâs still important to consider the implications of readability versus compactness. If using list comprehensions turns your code into a puzzle rather than a straightforward process, perhaps sticking to traditional methods may serve better. The ultimate choice should favour clarity and maintainability, especially when collaborating with others or returning to your work after a time away.
Integrating 'Find' with Libraries
Integrating the 'find' functionality with libraries like Pandas and NumPy is crucial for programmers who handle data-heavy applications. Libraries extend Pythonâs basic capabilities, making it easier to manage and manipulate large datasets efficiently. As we dive into this section, we will look at two significant libraries: Pandas and NumPy, to understand how they enhance the search functionalities that Python offers natively.
Using Pandas for Data Search
Pandas is a powerhouse when it comes to data analysis and manipulation. It provides versatile data structures like DataFrames and Series, which facilitate complex data handling effectively. When it comes to searching within these structures, the flexibility it offers is unmatched.
For instance, consider a DataFrame containing information about different students, including their names, ages, and grades. You might want to find a specific student's information or filter based on specific conditions. Here's an example:
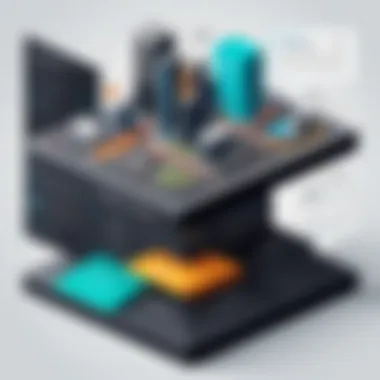
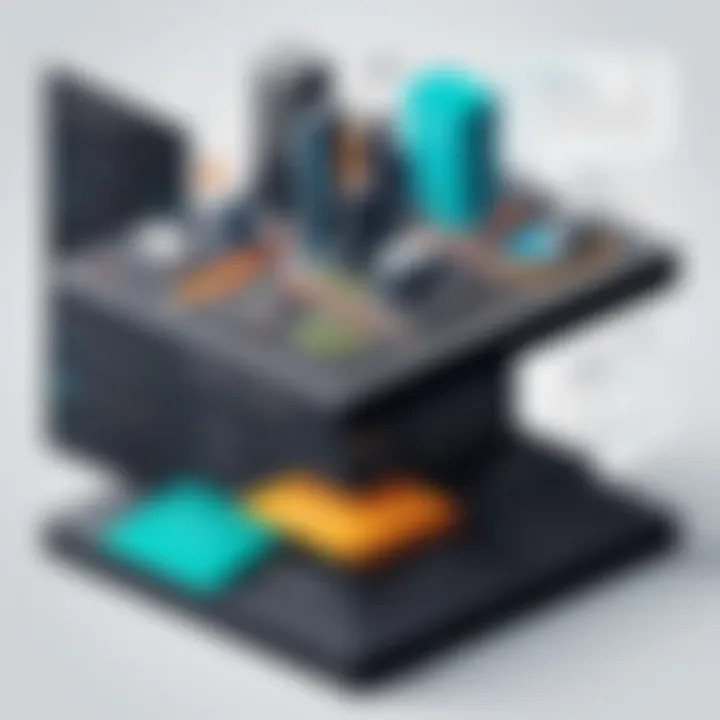
In this code, the search operation becomes straightforward with the syntax provided by Pandas. The operator allows you to filter rows efficiently based on conditions. Itâs worth noting that this approach is inspired by the ease of the 'find' operation and takes it to the next level by allowing for more complex queries.
Employing NumPy for Finding Values in Arrays
NumPy is known for its speed and efficiency in handling numerical data. It is particularly beneficial when searching for values within arrays or matrices. Want to know the index of a certain value in a NumPy array? No problem. The function can be your best friend in such scenarios.
Take this scenario where you have an array of temperatures recorded throughout a week:
With NumPyâs , you can quickly locate the exact position of a value in the array with minimal coding overhead. This functionality is particularly essential in data analysis, where pinpointing specific data points can drastically cut down analysis time.
Integrating 'find' functionality with libraries like Pandas and NumPy allows for more robust and complex data handling that builds on Python's native capabilities. This results in significant time savings and increased analytical power when working with large datasets.
Summary
Both Pandas and NumPy not only integrate seamlessly into Pythonâs ecosystem but also expand the capabilities of the 'find' functionality. This integration transforms how we approach data queries, especially within larger and more structured datasets. By leveraging these libraries, programmers can streamline their data search processes significantly, allowing for deeper insights and more efficient coding practices.
Case Studies and Practical Examples
Understanding the practical implications of Python's find methods is crucial for not just theory but for real-world applications too. By diving into case studies and practical examples, one can see how these functionalities solve actual problems. This brings the programming concepts to life, making them relevant to everyday coding challenges. The ability to find and manipulate data quickly can save time and enhance performance, particularly in data-driven environments.
Key benefits of focusing on practical examples include:
- Real-world relevance: Theory is often abstract, but case studies root concepts in tangible situations.
- Insights into best practices: Analyzing what works and what doesnât can guide learners to adopt better coding habits.
- Problem-solving skills: Exploring practical examples helps develop analytical thinking when encountering similar issues.
When analyzing strings or lists, one can identify necessary functions like , which is often more efficient than brute force methods. Evaluating how experienced programmers use these functions can offer valuable perspectives and foster deeper understanding amongst learners who are just starting in their programming journey.
Example of String Search Implementation
To see Pythonâs string search capabilities in action, letâs consider a simple text processing scenario. Suppose we have a paragraph that needs to check the occurrence of a specific word. For instance, if you were to search for the word "Python" in a text, the function can pinpoint its location seamlessly. Here's an example:
In this code snippet, we use the method, which returns the index of the first occurrence of the substring. If the word isn't found, it returns , allowing for straightforward error handling. This shows how effective search operations can streamline text processing tasks.
List Manipulation with Finding Techniques
The art of manipulating lists is a cornerstone of Python programming. It's not just about searching; itâs about knowing how to optimize and utilize various methods in tandem with finding functionalities. Let's say we have a list of user ages and want to find if a certain age exists and its position.
Consider the following example:
Here, we combine for existence check, and for fetching the specific index right after confirming the existence. It's a clean, efficient way to handle lists while searching for values.
In the world of programming, leveraging these techniques can elevate oneâs ability to write cleaner code that's easier to maintain. By looking at these examples, learners can better appreciate how theoretical knowledge translates into practical skills that are essential in any programming endeavor.
End on Finding Elements in Python
The conclusion section serves as a capstone to this exploration of Python's find capabilities. It distills the most significant points into digestible insights, ensuring that readers walk away not just with knowledge, but with a clear understanding of how to practically apply it in their coding endeavors.
The various methods of finding substrings in strings, locating elements in lists, and utilizing libraries such as Pandas and NumPy enrich the agility of a programmerâs toolkit. The versatility of the find function allows developers to search and manipulate data efficiently. This isnât just about writing a line of code; itâs about fostering the ability to problem solve, analyze, and adapt in a constantly evolving tech landscape.
In a world overflowing with data, the ability to search effectively transforms raw information into actionable insights. When programmers understand the nuances and limitations of find operations, they can circumvent common pitfalls, optimizing their code and enhancing its readability. This ultimately leads to more maintainable and efficient applications.
"The efficiency with which one can find and organize elements in Python can make the difference between a struggling developer and a seasoned programmer."
Summarizing Key Takeaways
To wrap things up, here are a few essential points that should stick in your mind:
- Comprehensiveness of Search Options: From strings to lists, Python provides a robust framework for finding elements in various data structures.
- Method Specialization: Each methodâlike , , or using memberships checksâhas its unique purpose and ideal scenarios.
- Error Handling: Knowing how to handle exceptions or return values when elements are not found is critical in avoiding code crashes and ensuring a smoother user experience.
- Integration with Powerful Libraries: Leveraging libraries such as Pandas and NumPy boosts functionality, allowing for advanced searches and data manipulations.
- Practice & Application: Real-world scenarios and practice exercises solidify understanding, turning conceptual knowledge into practical skills.
Future Outlook on Search Functionalities
As technology evolves, so too do the tools and methods available for searching within data. Here are a few trends to keep an eye on:
- Increased Use of Machine Learning: As machine learning becomes more embedded in data science, search functionalities will likely include more predictive and intelligent search options.
- Enhanced User Experience: Future search methodologies will focus more on user experience, AI-driven suggestions, and customization. Being able to forecast what users need can make finding elements not just effective but intuitive.
- Cross-Platform Functionalities: As applications grow, having seamless search functionalities across different platforms and devices will deepen relevance.
- Ongoing Community Contributions: Open-source contributions will continue to enhance Python's capabilities, including search functions, as communities collaborate to identify gaps and innovate.
While finding elements in Python may seem a basic skill, its implications are profound. Continually honing this craft will not only make you a better programmer but also prepare you for future demands in the field. This ongoing journey through Python's capabilities will yield bountiful rewards, both theoretically and practically.