Mastering the Python Print Function: A Detailed Guide
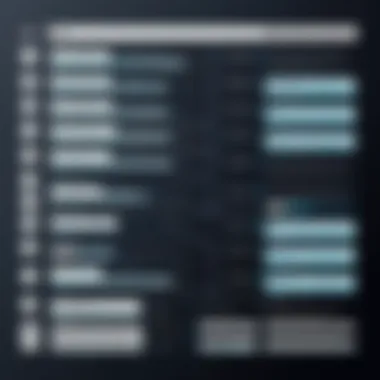
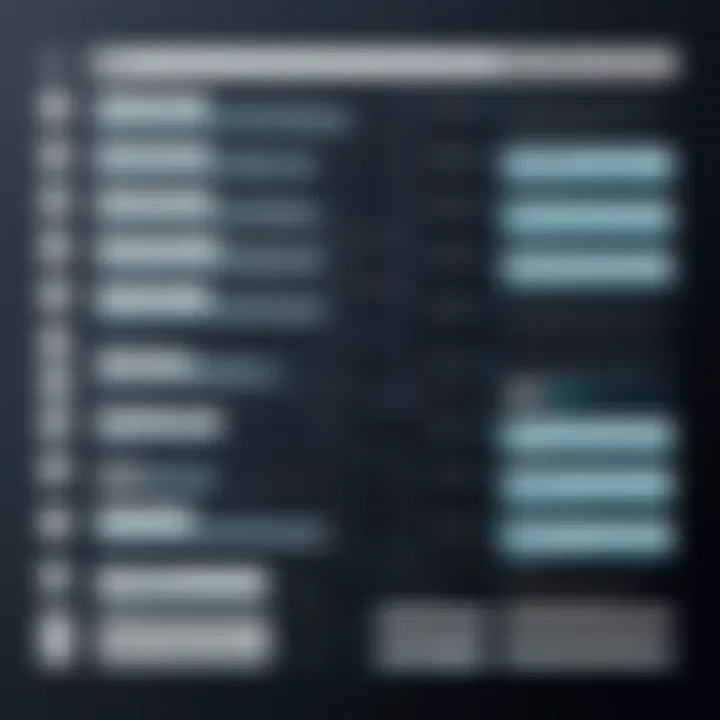
Intro
When venturing into the realm of programming, understanding how to output data effectively is crucial. The way we use the print function in Python serves as one of many foundational building blocks for any programmer. This guide is your trusty companion, laying out everything you need to know to master this fundamental tool.
Importance of the Print Function
The print function is how we display information to users, debug our code, and demonstrate the results of our algorithms. Without it, we’re left in the dark, unsure of what our code is doing.
Woven throughout this guide are practical examples that not only clarify concepts but also expand your understanding of Python's capabilities. Let’s take a deep dive and unravel the power of printing in Python.
Foreword to Programming Language
History and Background
Python burst onto the scene back in the late 1980s, thanks to Guido van Rossum. The goal was to create a language that was easy to read and write yet powerful enough to handle complex tasks. While other languages can make coding feel like deciphering hieroglyphics, Python has maintained a strong emphasis on simplicity and readability. This focus has allowed it to grow in popularity and scope, making it a favorite among both beginners and seasoned developers.
Features and Uses
Python’s versatility is remarkable. It’s used everywhere, from web development to data analysis— even in artificial intelligence. The syntax is clear, which lowers the barrier for entry. To highlight a few features that cater to its diverse applications:
- Simple Syntax: Easy to read, easy to write.
- Rich Libraries: Extensive libraries support diverse functionalities.
- Multi-Purpose: Great for both scripting and larger applications.
Popularity and Scope
It’s no surprise Python ranks among the top programming languages in various surveys. Its robust community means ample resources for learning and troubleshooting. If you’re starting out or hoping to expand your skillset, Python’s relevance in today’s tech landscape makes it an excellent choice.
Basic Syntax and Concepts
Variables and Data Types
At the heart of any programming language lies variables. In Python, these can hold various data types. Here are the basics:
- Strings: Textual data, defined using single or double quotes.
- Integers: Whole numbers without decimal points.
- Floats: Numbers with decimals.
- Booleans: True or False values.
Operators and Expressions
Python supports a variety of operators for performing calculations and comparisons:
- Arithmetic Operators ( +, -, *, / ) for basic calculations.
- Comparison Operators ( ==, !=, >, ) for comparing values.
When doing anything with variables, be mindful of operator precedence to avoid unexpected results.
Control Structures
Control structures like loops and conditional statements grant your program decision-making abilities. You can use the statement to evaluate conditions, or use and loops to iterate through data. These structures bring life to your code, guiding how it responds to inputs or varying situations.
Advanced Topics
Functions and Methods
Understanding how to define your functions is crucial. Functions allow you to encapsulate code that performs specific tasks. Here’s a simple example:
When defining methods in classes, it’s essential to know how they differ from functions, especially concerning instance variables.
Object-Oriented Programming
With object-oriented programming, the focus shifts from functions to objects. Classes serve as templates, allowing you to create objects that bundle data and functionality. Mastering OOP will enhance your Python skills significantly, enabling the design of complex systems that mirror real-world scenarios.
Exception Handling
Error handling is a vital skill for any programmer. Python uses and blocks to manage errors gracefully. A simple example looks like this:
This ensures your program doesn’t crash unexpectedly, and instead, it offers informative feedback.
Hands-On Examples
Simple Programs
Getting your feet wet with simple programs can solidify key concepts. Consider a basic calculator that can add two numbers. You’ll learn how to handle user input and implement print functionalities to show results clearly.
Intermediate Projects
As you grow more comfortable, delve into projects like a to-do list application. This involves using lists and functions to manage tasks effectively while employing the print function to showcase tasks to users.
Code Snippets
Utilizing snippets alongside full programs allows for quick testing of specific functions or ideas. For instance, testing how handles various data types can be enlightening.
Each of these illustrates how the print function can handle different inputs seamlessly.
Resources and Further Learning
Recommended Books and Tutorials
To truly grasp Python, consider diving into books like 'Automate the Boring Stuff with Python' or 'Python Crash Course'. They offer foundational knowledge and practical applications.
Online Courses and Platforms
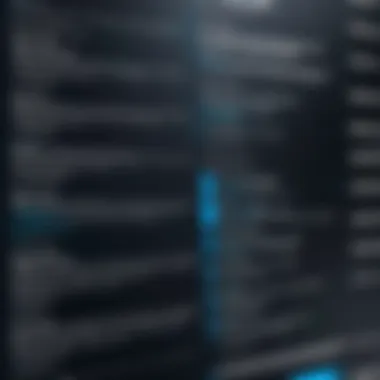
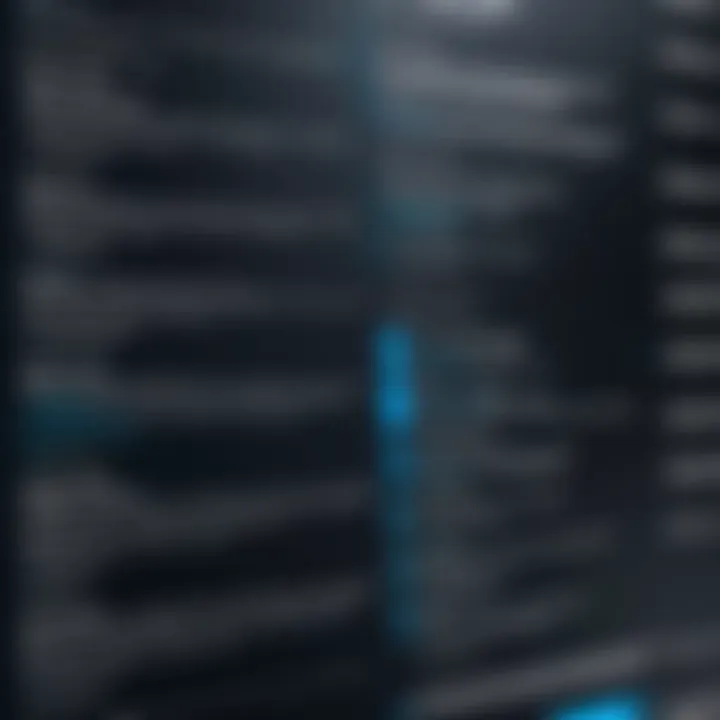
Platforms like Coursera and edX offer structured online courses that can guide you through from basics to advanced topics while engaging with a community.
Community Forums and Groups
Participating in forums like Reddit's r/learnpython can be incredibly beneficial. Engaging with a community aids in knowledge exchange and offers support during tricky coding jams.
Intro to the Print Function
The print function is one of the foundational blocks in Python programming. It's like the bread and butter for anyone stepping into the world of coding. When you launch a new Python script, the first command often included is . This humble function serves as a direct means to communicate with users, showcasing outputs, debugging information, and results of computations in a readable format. Understanding how to leverage the print function effectively can enhance both your coding efficiency and clarity.
What is the Print Function?
At its core, the print function in Python is designed to output data to the console. The syntax is straightforward: you typically call followed by the data you want to display within parentheses. For example, sends that greeting to the screen for anyone watching. It can handle various data types including strings, integers, and even lists. It’s kind of like a Swiss Army knife; capable of doing a lot, but at the same time, it requires some understanding to utilize all its features effectively.
Even though it appears simple, there are nuances to explore. The function can concatenate multiple items and format them before output, which can become crucial when dealing with more substantial datasets or user inputs. Think of it as dressing up a dish before serving it to guests—presentation is half the feast.
Importance in Python Programming
In the realm of programming, clarity plays a key role. The print function acts as a bridge between your code and the output it generates. It offers a clear view of what’s happening in your application at any point in time. When debugging, you can sprinkle commands throughout your code to monitor variable values or track the flow of operations. This practice is akin to leaving breadcrumbs so you can navigate back if something goes awry.
Moreover, the print function promotes user engagement by displaying results in a user-friendly format. Whether you’re writing a simple script or developing a complex application, ensuring that your output is understandable can often make or break the user experience. Printing with intention lets you create outputs that attract attention and deliver necessary information clearly.
Understanding the print function is not just beneficial; it is essential for anyone looking to master Python programming.
In summation, the print function may seem elementary, but its importance is profound. It's the key to communication in programming, offering insights that can help both the programmer and the end-user grasp what’s happening behind the scenes. This guide will unravel this essential function, equipping you to make the most out of your coding adventures.
Basic Syntax of the Print Function
Understanding the basic syntax of the function is a cornerstone for anyone eager to delve into the world of Python programming. This foundational aspect not only lays the groundwork for effective output but also enhances your overall coding experience. Grasping the syntax can help prevent common errors and misunderstandings, allowing for cleaner code and improved communication through your programs. The straightforward design of the function makes it accessible for beginners and enables more seasoned programmers to utilize it with versatility.
Understanding the Syntax
To get started with the function, it’s vital to know its structure. The most basic format is simply:
In this example, can refer to a string, number, or even a complex data structure. It’s worth noting that you can pass multiple values to , separating them with commas. This function then takes care of outputting them to the console:
The flexibility in the syntax makes the function a swiss army knife in your coding journey, suitable for both simple outputs and more complex data handling.
Single Arguments vs. Multiple Arguments
When using the function, distinguishing between single and multiple arguments is crucial.
- Single Argument: When you pass just one item:
- Multiple Arguments: When you have more than one item, the function can concatenate them, separating each by a space by default:
- The function processes this single value and prints it directly. For instance:This outputs: Learning Python is fun!
- For example:This outputs: Python is powerful!
Being aware that you can mix data types as well – strings, integers, floats – is also important. The function will convert them to strings for display. However, be cautious about the data types you pass; otherwise, you might find your output goes awry.
Additionally, Python allows customization of the separator and end character using the and parameters respectively. This means you can shape how your output looks at the terminal:
The above code produces: Hello - World!
Such features can be a game changer, especially when dealing with multiple data streams or in applications requiring specific formatting.
The understanding of this basic syntax empowers you to craft outputs that not only convey the data but do so in a manner that is coherent and easy to digest.
Practical Examples
The inclusion of practical examples is crucial in this guide, as they serve as the bridge between theory and application. In any programming journey, understanding the print function is foundational. By seeing how this function operates in real-world contexts, learners can grasp its importance more fully. Examples not only illustrate the use of syntactical rules but also showcase the nuances of how outputs can affect readability and debugging.
Practical examples empower students to implement what they learn, allowing for a hands-on experience that deepens comprehension. They can compare multiple scenarios and relate to them in various projects, thanks to the versatility of the examples provided.
Basic Example of Print
Printing Strings
Printing strings is often one of the first things a Python learner encounters. It's as straightforward as pie, but it serves tremendous importance in programming. The key characteristic here is simplicity; using the print function with strings allows for immediate feedback and visibility of results. When you write , you instantly confirm that your environment is working and that you can convey textual information effectively.
The unique feature of printing strings is that you can visualize concepts, allowing for easier troubleshooting and clearer demonstrations within tutorials. For new learners, being able to see their text output without extra complexity provides a confident stepping stone into more advanced topics.
However, a disadvantage might arise when learners use excessive string printing for debugging purposes; it can clutter output and make it less readable. Keeping outputs concise can help mitigate this issue.
Printing Variables
When you transition to variables, printing becomes even more powerful. The aspect of printing variables revolves around dynamically displaying values stored in memory, which is essential for any meaningful programming task. Imagine having a variable called that holds your name. By executing , you will show the current value of that variable.
This approach provides flexibility, allowing the programmer to maintain a clean codebase without hardcoding text. The key characteristic here is adaptability. It opens the door to changeable data, making your output reflective of various states within your program.
The unique feature of variable printing lies in its ability to showcase user interactions—say, capturing user input and immediately displaying it. While it's a beneficial method, one downside is that if the variables hold complex data types or multiple data points, the printed output might become difficult to interpret. Nevertheless, with thoughtful management strategies, this can become a non-issue.
Formatted Output
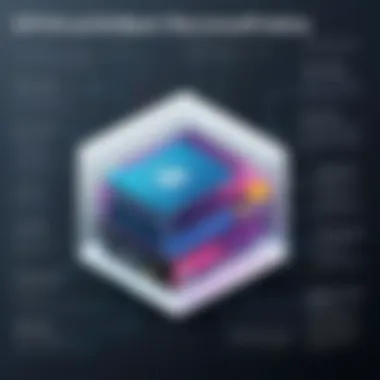
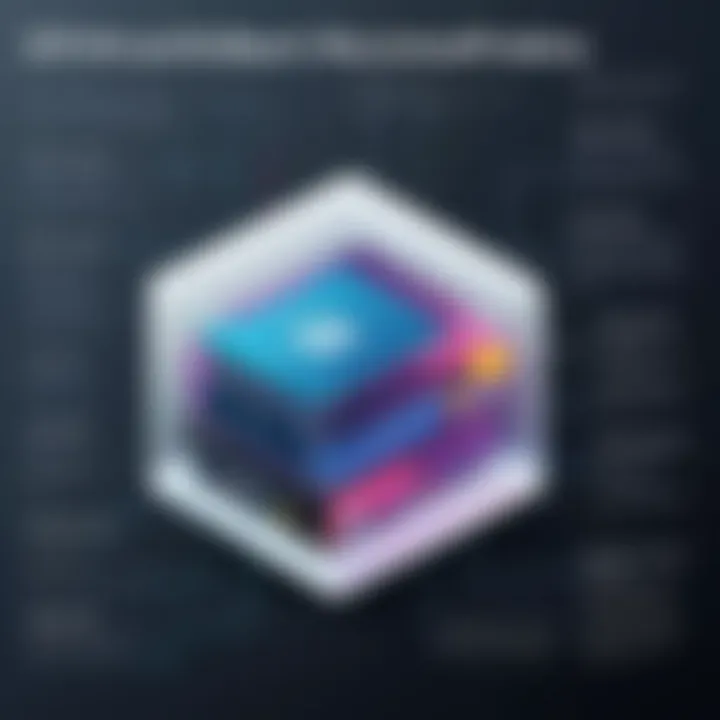
Using f-Strings
Now, we come to formatted output, which allows for more control over how data is displayed. Using f-strings marks a significant improvement for Python programmers. This feature, introduced in Python 3.6, combines the ease of variable embedding with string printing. So, if you write followed by , you can directly include variables in the string, and the output will be both dynamic and precise.
The key characteristic of f-strings is their clarity and efficiency. They make it easy to see at a glance what variables you're printing and how they fit into the message. One unique advantage is that you can also include expressions within the curly braces, allowing for even more complex outputs like calculations or formatting directives directly.
Though f-strings are fantastic, one should be cautious about their usage when formatting non-string data types or complex objects—if not utilized correctly, this can lead to errors or confusing outputs.
Using Format Method
The unique feature of this method is its versatility, especially when dealing with strings composed of multiple components that require specific formatting. One benefit of using the format method is that it can also handle various data types without extensive modifications to your initial string.
However, with great power comes responsibility—if you aren't careful with your placeholders or fail to provide enough arguments, it can lead to index errors. To avoid confusion down the line, one must ensure that the number of placeholders matches the number of supplied values.
Every programmer has their own style, but choosing the right output method can significantly streamline your workflow and improve clarity in your code.
In summary, executing practical examples immensely enriches the learning experience when it comes to understanding the print function in Python. By stepping through the nuances of string and variable printing, along with the intricacies of f-strings and formatting methods, learners can cultivate skills that pave the way for future success in programming.
Handling Output
When it comes to programming in Python, handling output is a critical aspect that ties together user interaction and data presentation. Output can vary significantly, from displaying data on a screen to logging information for later analysis. Understanding how to effectively manage output allows developers to create more intuitive and user-friendly applications. This section dives deep into both traditional and advanced methods for directing output.
Output to Different Outputs
The ability to direct output to various destinations is crucial for a flexible programming experience. In Python, the function primarily sends text to the console, but it can also be adapted to other outputs. Let's explore two main forms of output—standard output and files or streams.
Standard Output
Standard output refers to the default destination for output data—usually the console or terminal. This form of output is fundamental for debugging and user interaction. One significant advantage of using standard output is its immediacy; it allows developers to quickly see results during development or testing phases.
- Key Characteristic: The use of standard output is straightforward and doesn't require any additional code or setup. It simply involves calling with the desired arguments.
- Benefits: This method of output is widely recognized and utilized. It facilitates rapid feedback, which is invaluable when trying to identify issues or better understand the flow of a program.
- Drawbacks: However, relying solely on standard output can become cumbersome in larger projects. The console can quickly fill up with messages, making it hard to differentiate between critical information and routine debug statements.
Files and Streams
Another valuable dimension of output handling is directing information to files and streams. This capability is vital for maintaining logs, saving configurations, or exporting data for further analysis. In many applications, particularly those that run in the background or as part of a larger system, writing output to files is imperative.
- Key Characteristic: Outputting to files or streams involves a bit more setup than standard output, requiring the specification of the file path and often the mode (read, write, etc.).
- Benefits: Using files for output allows developers to retain data across program executions, which is essential for reporting and analysis. Additionally, it helps manage clutter in the console, keeping it clean for immediate interactions.
- Drawbacks: On the flip side, writing to files can introduce complexity in code and potential errors like file-not-found situations or permission issues that may disrupt the flow of a program.
Controlling End Character
When outputting text in Python, having the ability to control what occurs at the end of each line is a noteworthy feature. By default, the function adds a newline character after the output, effectively moving to the next line. However, you can customize this behavior using the optional parameter.
Changing the end character can help in formatting your results neatly. For instance, if you wanted to display a series of items on the same line, you might use a space or comma as your end character instead of the default newline.
By controlling the end character, you can create a more polished and formatted output, catering specifically to your application's needs.
Advanced Usage
Understanding the advanced usage of the print function in Python opens up various opportunities to personalize and enhance output in a clearer and more efficient manner. When you become familiar with these sophisticated techniques, you not only improve how your programs convey information but also sharpen your coding skills overall. These features help in navigating challenges that come with formatting outputs, especially when dealing with more complex data sets or customizing prints for better readability.
Using Separator Parameter
The separator parameter can be a game-changer when displaying multiple pieces of information in a single print statement. By default, Python separates items with a space, but you can customize this to fit your needs.
For instance, consider the following:
This default behavior outputs: , but sometimes you might prefer to have a comma, for example.
This change in the separator provides a clearer distinction between the printed values. Furthermore, using a unique separator can enhance clarity when the context requires it.
Here are some benefits of using the separator parameter:
- Improved Readability: You can make your output more organized and easier to read, particularly with lists of items.
- Flexibility: It helps maintain control over how outputs appear without needing to modify the data format.
- Presentation: Especially useful in displaying tabular data or groupings, creating a visually appealing layout.
Print Function with Custom Functions
Integrating the print function with custom functions in Python can significantly boost the potential of your code. By creating functions that return formatted strings, you expand the usefulness of print entirely.
Let’s say you have a function that generates a greeting based on the time of day. In such a case, you could utilize the print function like this:
This example demonstrates how the print function receives formatted output from the custom function. It's a minimalistic approach, but it highlights the elegance of combining both to achieve a desirable result.
Consider these points when utilizing print with custom functions:
- Modularity: Breaking down code into smaller parts is easier to manage and understand.
- Reusability: Functions can be reused, improving efficiency and keeping code cleaner.
- Testing: Easier to test individual components of your program, leading to fewer bugs.
"Combining the print function with custom functions not only enhances output formatting but also streamlines code organization and reusability."
Each of these advanced usages explores facets of the print function that elevate its utility beyond simple output. It is crucial for learners to embrace these concepts as they deepen their understanding of Python's capabilities.
Common Mistakes to Avoid
When embarking on the journey of learning Python—particularly, the print function—steering clear of common pitfalls can make all the difference. Mistakes in programming not only lead to errors but can also frustrate learners, leading them to doubt their understanding of the language. By identifying and discussing typical errors, this section aims to fortify your knowledge and enhance your efficiency when using Python's print capabilities.
Common Syntax Errors
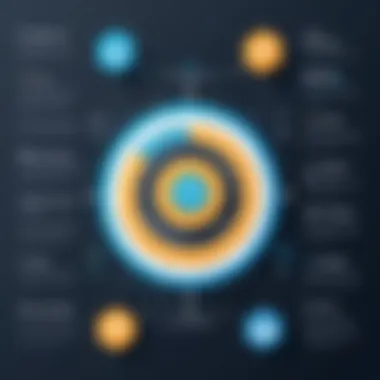
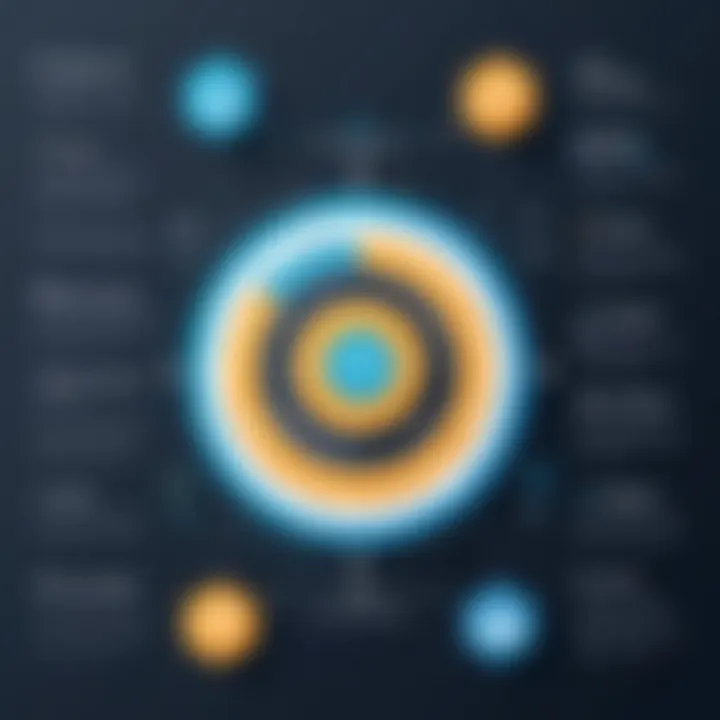
Syntax is the backbone of code; even minor glitches can derail a well-structured script. Here are some common syntax errors that beginners often stumble upon when using the print function:
- Missing Parentheses: One of the classic slip-ups is forgetting to close the parentheses after your print arguments. For instance, will raise a syntax error. Remember, closing those parentheses is crucial!
- Improper Quotation Marks: It's essential to ensure that the quotation marks used for strings are consistent. For example, using mixed quotation styles can lead to confusion: will also raise an error. Stick to one type—either single or double quotes—for clarity.
- Incorrect Spacing: In Python, extra spaces or misaligned indentations can be problematic. Make sure your code is neatly arranged—like stacking books on a shelf. For instance, writing is valid but could confuse readability; thus, keeping it is more organized.
Be aware of such syntax errors; avoiding them will save you from a significant headache and keep your coding process smooth.
Misunderstanding Arguments
The print function’s arguments can often be a source of confusion for learners. Understanding how to properly utilize these arguments will enhance your coding skills and output formatting. Here are a few common misunderstandings:
- Default Separator: When printing multiple items, many beginners overlook the default behavior of , which separates items with a space. For example, running outputs . If you meant for them to have a specific delimiter, like a comma, you need to specify it using the parameter: will give .
- Using End Parameter: Another frequent error involves not utilizing the parameter effectively. By default, ends each statement with a newline character. If you want to print several statements on the same line, you must explicitly define the : makes sure that the next print output keeps running on the same line.
- Omitting Required Arguments: While defined parameters can be optional or positional, some learners tend to forget about certain necessary arguments when defining custom functions. For clarity's sake, ensure you know which can or cannot be omitted based on the context of usage.
By keeping these common misconceptions in mind, you can navigate the complexities of the print function more adeptly, avoiding frustration and enhancing your coding experience.
Best Practices for Using Print
When working with Python, the print function may seem simple at first glance, but its effective use plays a significant role in the clarity and functionality of your code. Understanding best practices associated with print can greatly enhance not only the readability of the output but also the overall debugging process. This section delves deeper into the elements that make print usage optimal, ensuring that you write clean and effective code.
Maintaining Readability in Output
The readability of output is crucial in programming. When your print statements are clear and well-formatted, the information presented becomes accessible and understandable. Here are some key considerations:
- Use Descriptive Messages: Always accompany your output with descriptive texts to provide context. For instance, instead of simply printing a variable's value, you might want to say something like:This provides immediate clarity on what the number represents.
- Consistent Formatting: Adhering to a formatting style throughout your code improves understanding. This could involve making use of indentation, spacing, and consistent string templates.
- Limit Output Length: Avoid overwhelming the user with too much information at once. If there's a lot of data to display, consider displaying summaries or chunks instead of dumping everything at once.
- Utilize New Lines: Separating output with new lines can help break dense information, making it more digestible.
By following these suggestions, you create outputs that are formatted in a way that lets users grasp the information quickly and efficiently.
Printing Debug Information
Debugging is essential, especially when your code begins to grow in complexity. The print function can serve as a valuable debugging tool; however, to maximize its effectiveness, consider the following practices:
- Prefix Your Debug Statements: Differentiate debug outputs from regular information by prefixing them. This allows you to spot them quickly. For instance:
- Include Variable States at Key Points: It’s useful to display the state of important variables at critical junctures in your code. This can help trace the flow and logic effectively.
- Use Conditional Statements for Debug Outputs: You might not want to have debug prints in production code. Consider adding a flag that toggles debug printing on or off.
Given these practices, you can harness the print function to its fullest potential when troubleshooting your code. Print is more than just outputting to the console; it's a tool that, when wielded properly, can lead to clearer, more maintainable code.
Remember: Effective print statements can save you from pulling your hair out while debugging, allowing you to spot issues calmly and efficiently.
Transitioning from Print to Logging
When developing Python applications, one may find themselves gravitating towards the function for its simplicity in conveying messages to users or developers during code execution. However, as programs grow in complexity, the inadequacies of become glaringly apparent. This is where understanding the transition from the basic function to a more sophisticated logging mechanism becomes critical.
The transition from to logging is not merely a step up in complexity; it's a fundamental shift in how we approach information output. Logging provides a structured way to record messages, errors, and diagnostic information about the runtime behavior of an application. This shift offers numerous advantages, such as:
- Controlled verbosity: With logging, you can adjust the level of detail shown without changing code. Levels like DEBUG, INFO, WARNING, ERROR, and CRITICAL allow you to filter messages efficiently.
- Persistent records: Logs can be saved to files, databases, or external monitoring systems, providing historical insights that cannot offer.
- Better context: Logging includes timestamps and severity levels, giving a richer context than a simple text output.
This shift is particularly important as Python applications move from simple scripts to larger, more intricate projects where efficient debugging and monitoring become crucial. Here, we’ll discuss when to use logging over , and how to set it up effectively.
When to Use Logging Instead of Print
Switching to logging is advisable in several scenarios. If you find yourself doing any of the following, it’s time to consider logging:
- You’re debugging an application with many components. Using makes it tough to track messages across files.
- You need to document issues beyond the current session. Logging can retain error traces even after code execution ends.
- Performance matters. Printing to the console can slow down execution, especially in loops or extensive data processing tasks. Logging can be configured to minimize performance impact.
"Print statements are quick and easy, but they lack the functionality to manage complexity beyond a certain point."
Basic Logging Setup
Setting up logging in a Python application is straightforward, thanks to the built-in module. Here’s how to get started with a basic configuration:
- Import the logging module:
- Set up basic configuration: You can define the log level and the format of the output messages:
- Use logging methods: Replace statements with logging calls. For instance, instead of , write:
This will automatically log the time, the log level, and the message you provide. By default, warnings and errors will still appear even if you set the level to INFO. Therefore, if you want to see only critical messages, you could adjust it with the method to .
In summary, the transition from to logging is more than just a change in toolset; it’s about embracing a methodology that respects the complexity of modern applications. Utilizing logging effectively can enhance your development process and lead to more maintainable and debuggable code.
Finale
As we wrap up our exploration of the print function in Python, it’s crucial to recognize the far-reaching implications this simple command can have in both coding endeavors and practical applications. The print function serves as the cornerstone for understanding how to present information clearly and effectively in your programs. Not only does it provide immediate feedback, which is vital for beginners, but it also plays a role in debugging and displaying the flow of data within more complex systems.
Recap of Key Points
In this guide, we covered several essential elements regarding the print function:
- Understanding the Basics: We discussed the fundamental syntax and various argument types that can be passed to the print function. Knowing how to print strings and variables sets the stage for any Python programmer.
- Formatted Output: By using techniques like f-Strings and the format method, we learned how to enhance the visual readability of outputs. This skill is indispensable, especially when displaying data for an audience.
- Advanced Functionalities: The inclusion of parameters like separator and end character broadens how information can be presented. Understanding these nuances can save you a lot of headaches later on.
- Common Pitfalls: We identified frequent mistakes that can trip up even seasoned programmers, such as syntax errors and misconceptions about arguments.
- Transitioning to Logging: Recognizing when to shift from using print to a logging framework is paramount for building scalable applications.
Next Steps in Python Programming
The journey doesn’t end here. Having grasped the print function, it’s time to think about the logical progression in your Python programming journey:
- Experiment with Projects: Create small projects where the print function is heavily utilized—such as a budgeting application or a personal diary program. These projects help cement your understanding while providing tangible outcomes.
- Dive Deeper into Data Structures: Begin to work with lists, dictionaries, and other data structures, applying print to visualize the contents. This will enhance your understanding of how data flows in Python.
- Learn About Python Libraries: Familiarize yourself with helpful libraries like NumPy and Pandas that also make extensive use of printing for debugging and data visualization.
- Consider Object-Oriented Programming: As you become more comfortable in Python, exploring object-oriented concepts will broaden your programming skills and context. The print function can have advanced applications in this paradigm as well.
By following these steps, you will not only enhance your proficiency but also build a robust foundation for mastering Python and its many capabilities.