Mastering Programming Languages: A Comprehensive Guide for Beginners and Intermediates
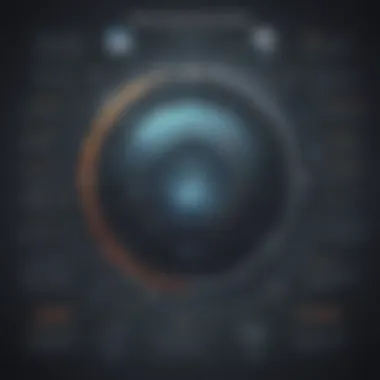
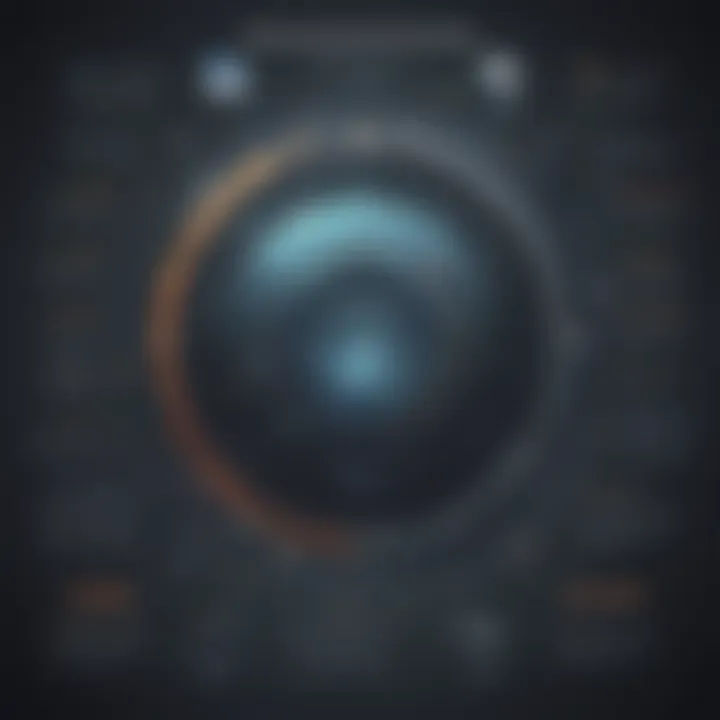
Introduction to Programming Language
When delving into the realm of programming languages, it is crucial to understand the historical evolution that paved the way for the diverse landscape we see today. From the birth of early programming languages to the advancement of modern coding paradigms, each iteration has contributed to the rich tapestry of syntax and structures that programmers navigate. Exploring the lineage of programming languages provides invaluable insights into how computational thinking has evolved over time.
Diving deeper into the features and uses of programming languages unravels the intricate tapestry of technological possibilities they offer. Whether it's the robust data handling capabilities of Python or the real-time processing prowess of C++, each language brings its unique strengths to the table. Understanding the applications and scopes of different programming languages equips learners with the knowledge needed to choose the right tool for a given coding task.
Basic Syntax and Concepts
As beginners embark on their coding journey, grasping the fundamental building blocks of programming is paramount. Variables and data types lay the foundation for storing and manipulating information within a program, acting as the core elements that drive computation forward. Moreover, mastering operators and expressions empowers programmers to perform mathematical calculations and logical operations essential for algorithmic problem-solving. Control structures, including loops and conditional statements, dictate the flow of a program, enabling it to make decisions dynamically based on varying input.
Advanced Topics
Transitioning from basic syntax to more complex concepts involves delving into functions and methods, the building blocks of reusable code segments that streamline program logic. Object-oriented programming (OOP) introduces a paradigm shift by encapsulating data and behavior within objects, fostering code modularity and reusability. Additionally, robust software design entails implementing exception handling mechanisms to gracefully manage and recover from runtime errors, enhancing the stability and resilience of an application.
Hands-On Examples
Practical application reinforces theoretical knowledge, making hands-on examples a pivotal component of programming education. Simple programs, such as basic algorithms and data manipulation scripts, elucidate core concepts through executable code snippets. Intermediate projects further challenge learners by integrating multiple concepts into cohesive applications, fostering problem-solving skills and coding fluency. Additionally, sharing code snippets allows for exploration and experimentation, nurturing creativity and innovation in coding endeavors.
Resources and Further Learning
Amidst the vast expanse of programming resources, curated guides and recommendations can significantly accelerate the learning journey. Browse through recommended books and tutorials to gain in-depth knowledge and practical insights from experienced developers. Enroll in online courses and platforms offering interactive lessons and coding challenges to enhance your skills iteratively. Engage with community forums and groups to seek advice, share experiences, and collaborate on coding projects, fostering a supportive network of peers and mentors in the ever-evolving landscape of programming.
Introduction to Programming Languages
Programming languages are the foundational building blocks essential for all aspiring programmers to comprehend. Understandably, the journey of mastering a programming language can be daunting but rewarding. This section aims to provide a thorough overview of the key concepts, assisting beginners and intermediate learners in grasping the fundamental principles necessary to navigate the intricate world of coding and software development.
Understanding the Basics of Programming
Key Concepts in Programming
Exploring the essence of programming, key concepts serve as the cornerstone for creating logical and efficient solutions to complex problems. Understanding variables, data types, and control structures lays the groundwork for writing functional code. These fundamental concepts allow programmers to execute algorithms efficiently, optimize performance, and enhance code readability.
Fundamentals of Algorithms
Fundamentals of algorithms delve into the realm of problem-solving strategies, emphasizing the importance of algorithmic efficiency and scalability. By mastering the fundamentals, programmers can develop algorithmic thinking, critical for designing robust solutions to diverse computing challenges. Algorithms form the core of programming logic, enhancing logical reasoning and promoting innovation in software development.
Syntax and Logic
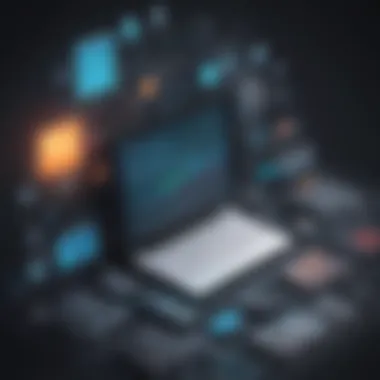
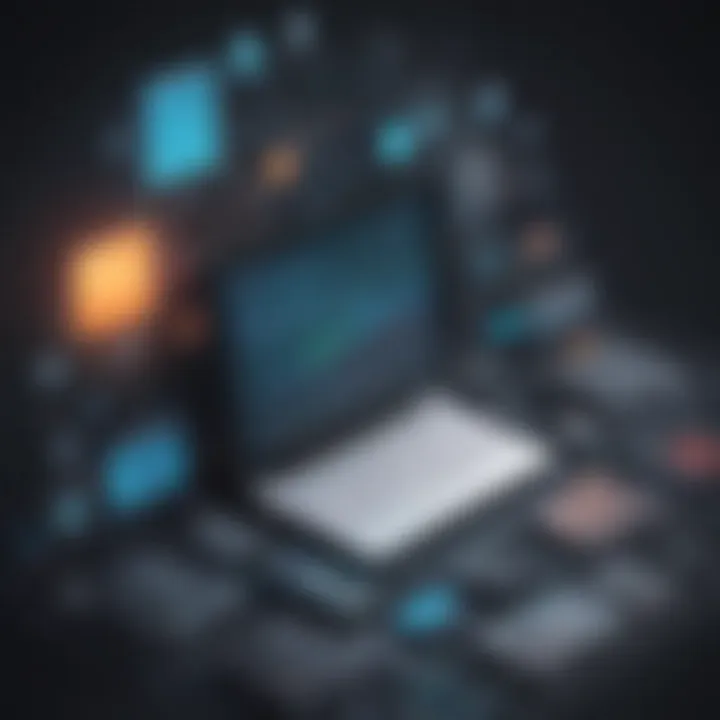
Syntax and logic dictate the structure and flow of code execution, ensuring the accuracy and precision of programming languages. Proficiency in syntax enables programmers to express solutions effectively, while logical reasoning facilitates the creation of error-free code. Understanding syntax intricacies and logical operations is paramount for developing reliable and efficient software applications.
Choosing the Right Language
Factors to Consider
When selecting a programming language, various factors like project requirements, learning curve, and industry demand play a crucial role. Considering factors such as language popularity, community support, and versatility helps programmers make informed decisions. By evaluating these aspects, individuals can choose a language aligned with their goals, ensuring a fulfilling coding experience.
Popular Programming Languages
Popular programming languages such as Python, Java, and JavaScript offer unique features and cater to diverse development needs. Whether focusing on web development, data science, or mobile applications, popular languages provide extensive libraries and frameworks, simplifying project implementation. Understanding the strengths and weaknesses of each language facilitates strategic language selection based on project specifications.
Language Applications
Programming languages find applications across various domains, from artificial intelligence to cybersecurity. Each language excels in specific areas, influencing its usability and relevance in different industries. Knowing the practical applications of languages like C++, SQL, or Ruby empowers programmers to choose the most suitable language for their intended use case, optimizing development efficiency.
Setting Up Your Development Environment
Selecting IDEs and Tools
The selection of Integrated Development Environments (IDEs) and tools significantly impacts a programmer's workflow and productivity. Choosing IDEs equipped with debugging features, code completion, and version control enhances code quality and streamlines development processes. Intuitive tools and plugins aid in code navigation, refactoring, and testing, fostering a conducive programming environment.
Installing Compilers
Installing compilers is vital for converting human-readable code into machine-executable instructions. Efficient compilers ensure code compilation accuracy and performance optimization, enabling seamless execution across different platforms. By installing reliable compilers compatible with chosen languages, programmers can compile and run code effortlessly, expediting the development lifecycle.
Configuring Build Environments
Configuring build environments involves setting up dependencies, libraries, and build tools essential for project compilation and execution. Customizing build configurations and environment variables optimizes the build process, eliminating errors and enhancing project scalability. Streamlining build environment setup enhances code consistency and promotes collaboration among development teams, elevating overall project efficiency.
Mastering the Fundamentals
Mastering the fundamentals of programming is a pivotal aspect of this comprehensive guide for beginners and intermediates. Understanding variables, data types, control structures, and loops lays the groundwork for developing robust and efficient code. By focusing on these core concepts, learners can grasp the essential building blocks of coding, enabling them to tackle more complex programming challenges with confidence and proficiency.
Variables and Data Types
Understanding Data Storage
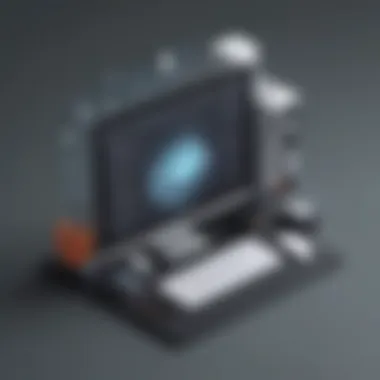
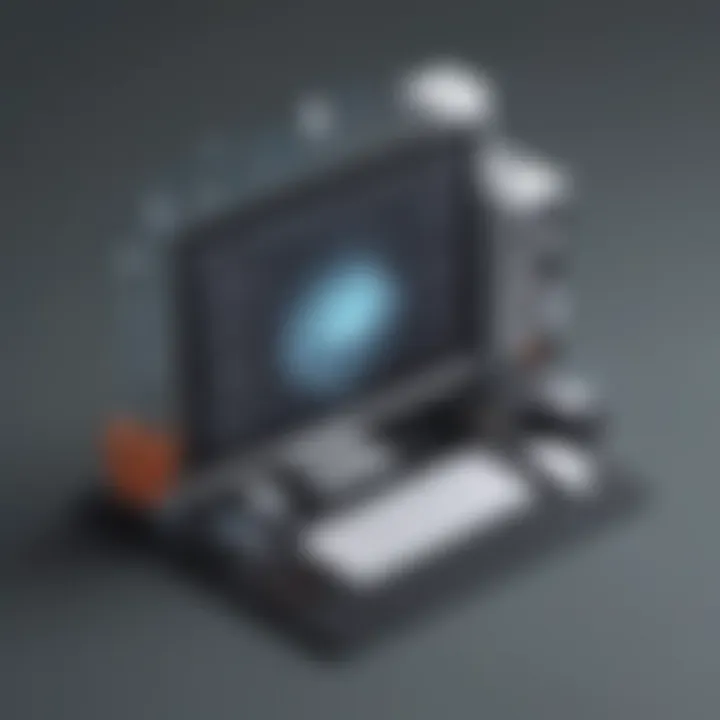
Illustrating the essence of data storage is critical in programming. Data storage refers to the techniques used to store and retrieve data effectively within a program. By mastering data storage, developers can optimize memory usage, enhance efficiency, and ensure data integrity. Understanding how data is stored and accessed in programming languages is fundamental to creating reliable and scalable applications.
Numeric and String Types
Numeric and string types are fundamental data types in programming languages. Numeric types like integers and floats handle numerical values, while string types manage textual data. Mastering numeric and string types equips programmers with the ability to perform mathematical operations, manipulate strings, and format text. These data types are extensively used in various applications and are indispensable for performing basic and advanced programming tasks.
Type Conversion
Type conversion involves converting data from one type to another in programming. It allows for interoperability between different data types, facilitating data manipulation and processing. Understanding type conversion enables programmers to ensure compatibility between variables, handle mixed-type data efficiently, and prevent errors during operations. Mastering type conversion is essential for writing versatile and error-free code.
Control Structures and Loops
If-Else Statements
The if-else statement is a fundamental control structure in programming that allows for conditional execution of code. By evaluating expressions and making decisions based on specific conditions, if-else statements control the flow of a program. Mastering if-else statements enables programmers to implement logic, handle different scenarios, and create dynamic application behaviors. Understanding this construct is key to writing logical and structured code.
Switch Cases
Switch cases provide a streamlined way to implement multi-way branches in code. This control structure evaluates an expression and executes the corresponding block of code based on the value of the expression. Mastering switch cases enhances code readability, simplifies complex decision-making processes, and improves code efficiency. This construct is ideal for scenarios involving multiple branching conditions and offers a concise alternative to nested if-else statements.
Looping Constructs
Loops are essential for repeating a block of code multiple times until a specified condition is met. By mastering looping constructs like for, while, and do-while loops, programmers can automate repetitive tasks, iterate over data structures, and implement efficient algorithms. Understanding looping mechanisms is crucial for managing iterations, optimizing code execution, and enhancing program performance. Looping constructs are indispensable for creating dynamic and interactive applications.
Functions and Modular Programming
Defining Functions
Functions are modular blocks of code that perform specific tasks and promote code reusability. By defining functions, programmers can segregate functionality, enhance code organization, and promote abstraction. Mastering the creation and utilization of functions enables developers to design modular applications, simplify complex logic, and improve code maintainability. Functions play a vital role in structuring programs and facilitating collaborative development efforts.
Parameter Passing
Parameter passing allows values to be transferred between functions in programming. By understanding parameter passing mechanisms like pass by value and pass by reference, programmers can manipulate data effectively within functions. Mastering parameter passing ensures smooth data flow, facilitates communication between different parts of a program, and enhances code flexibility. This concept is essential for building scalable and interactive applications.
Recursion
Recursion is a programming technique where a function calls itself to solve smaller instances of a problem. Mastering recursion enables programmers to solve complex tasks efficiently, implement elegant solutions, and simplify algorithmic designs. Understanding recursion promotes creativity in problem-solving, optimizes memory usage, and fosters elegant code implementation. Recursion is a powerful concept that expands programming horizons and encourages innovative thinking in software development.
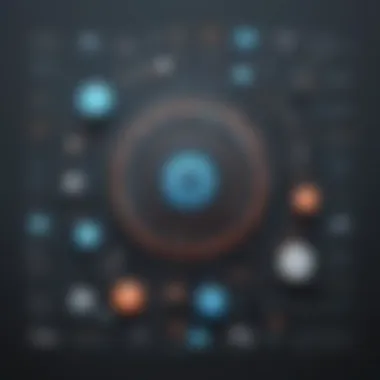
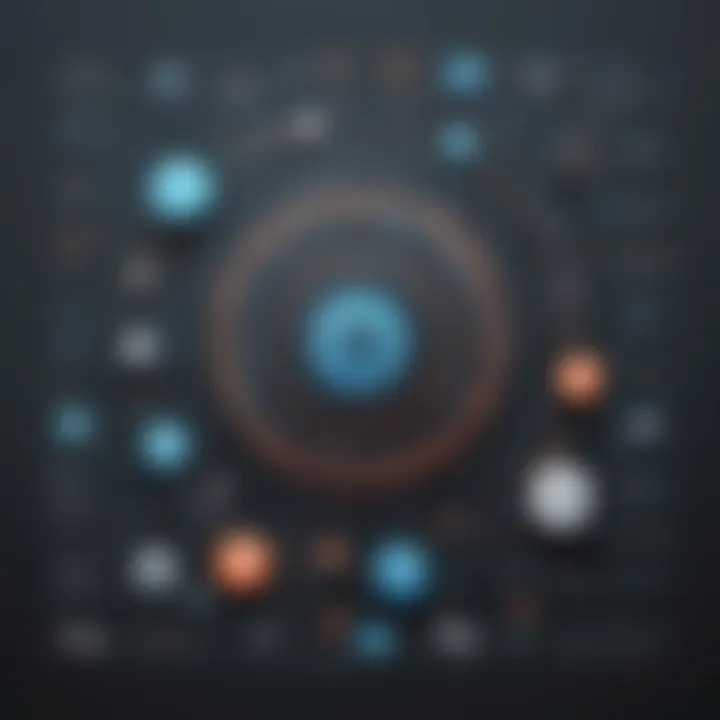
Advanced Concepts and Best Practices
In this section, we delve into the intricacies of advanced concepts and best practices in programming languages, shedding light on crucial elements that elevate programming skills. Understanding these advanced concepts not only enhances coding proficiency but also cultivates a mindset focused on efficiency and scalability. By grasping these concepts, beginners and intermediates can elevate their programming prowess and build robust, maintainable software solutions. Emphasizing best practices ensures code quality, readability, and maintainability, essential aspects of professional programming.
Object-Oriented Programming
Encapsulation and Inheritance
When we discuss encapsulation and inheritance in the context of object-oriented programming, we are delving into fundamental principles that underpin code organization and reusability. Encapsulation involves bundling data and methods within a class, shielding internal implementation details from external interference. On the other hand, inheritance allows classes to inherit attributes and methods from parent classes, fostering code reuse and promoting a hierarchical structure. The key characteristic of encapsulation and inheritance lies in promoting modularity and extensibility, enabling developers to manage complexity effectively. While encapsulation safeguards data integrity, inheritance facilitates code reuse and the establishment of relationships between objects, underscoring their pivotal roles in software design.
Polymorphism
Polymorphism, a cornerstone of object-oriented programming, empowers developers to write code that can operate on objects of multiple classes, fostering flexibility and extensibility. The key characteristic of polymorphism lies in the ability to invoke methods that exhibit different behaviors based on the object's type, promoting code reusability and adaptability. By leveraging polymorphism, programmers can write generic code that can accommodate varying object types, enhancing the scalability and maintainability of their applications. However, careful consideration is necessary to ensure that polymorphic behavior is implemented judiciously, avoiding complexity and promoting code clarity and predictability.
Design Patterns
Design patterns elucidate proven solutions to recurring design problems in software development, offering blueprints for designing flexible and robust code structures. The key characteristic of design patterns is their ability to encapsulate best practices, architectural paradigms, and design principles into reusable templates. By following design patterns, developers can streamline the development process, enhance code modularity, and promote code maintainability. Employing design patterns fosters code consistency and readability while facilitating communication among developers working on the same codebase. However, it is essential to strike a balance between pattern adherence and adaptability to individual project requirements, ensuring that design patterns serve as aids rather than constraints in the software development process.
Practical Applications and Projects
In the realm of mastering programming languages, the section on Practical Applications and Projects stands as a cornerstone for learners of all levels. This segment delves into the real-world application of programming skills, emphasizing the crucial transition from theoretical knowledge to practical implementation. By engaging in hands-on projects, individuals consolidate their learning, reinforce key concepts, and hone their problem-solving abilities within a simulated development environment. The significance of Practical Applications and Projects lies in its capacity to bridge the gap between theoretical understanding and practical execution.
Building Your First Application
Project Planning: Project Planning serves as the compass that guides developers through the intricacies of initiating a new application. This phase involves outlining the project scope, defining objectives, establishing timelines, and allocating resources accordingly. The key characteristic of Project Planning lies in its ability to set a clear direction for the development process, ensuring structured progress and efficient utilization of resources. By meticulously outlining every aspect of the project, developers can mitigate risks, foresee challenges, and streamline the development lifecycle, making it a vital component in the realm of software development.
Implementation Steps: Implementation Steps form the building blocks of turning a conceptual idea into a functional application. This process involves translating design specifications into executable code, integrating various modules, and conducting rigorous testing to ensure seamless functionality. The key characteristic of Implementation Steps is its transformative nature, where theoretical concepts materialize into tangible software solutions. By following a systematic approach to implementation, developers can ensure code quality, optimize performance, and deliver user-centric applications that meet industry standards.
Testing and Deployment: Testing and Deployment mark the final phase of the development cycle, where the application undergoes rigorous testing to identify bugs, errors, and performance issues before its release to end-users. This phase involves executing test cases, conducting user acceptance testing, and preparing the application for deployment across different platforms. The key characteristic of Testing and Deployment lies in its role of ensuring the reliability, efficiency, and user-friendliness of the application. By conducting comprehensive tests and deploying the application systematically, developers can deliver flawless software solutions that meet user expectations and industry benchmarks.
Exploring Real-World Case Studies
Software Development Lifecycle: The Software Development Lifecycle encapsulates the systematic approach to developing software applications, encompassing phases such as planning, development, testing, deployment, and maintenance. This structured framework ensures that software projects adhere to predefined milestones, quality standards, and stakeholder requirements. The key characteristic of the Software Development Lifecycle is its iterative nature, allowing developers to incorporate feedback, make necessary revisions, and adapt to evolving market trends. By following a well-defined lifecycle, developers can ensure project success, timely delivery, and stakeholder satisfaction.
Problem-Solving Approaches: Problem-Solving Approaches equip developers with strategies and methodologies to tackle complex challenges encountered during the development process. This segment emphasizes the importance of critical thinking, analytical skills, and creativity in devising innovative solutions to intricate problems. The key characteristic of Problem-Solving Approaches is their adaptive nature, empowering developers to think laterally, approach issues from different perspectives, and devise effective strategies to overcome obstacles. By adopting a problem-solving mindset, developers can enhance their decision-making abilities, foster innovation, and drive continuous improvement in software development.
Industry Best Practices: Industry Best Practices encapsulate the established guidelines, standards, and methodologies followed by industry professionals to ensure the delivery of high-quality software solutions. This segment emphasizes adherence to coding standards, documentation practices, version control, and collaboration strategies that optimize development workflows. The key characteristic of Industry Best Practices is their role in facilitating consistency, scalability, and efficiency in software development projects. By aligning with industry best practices, developers can enhance code quality, facilitate seamless collaboration, and adhere to industry benchmarks, thereby enhancing the overall success and market acceptance of their applications.
Continuous Learning and Growth
Online Resources: Online Resources serve as a vast reservoir of knowledge, tutorials, forums, and tools that aid developers in continuous learning and skill enhancement. This segment highlights the importance of leveraging online resources to stay updated on emerging technologies, industry trends, and best practices. The key characteristic of Online Resources lies in their accessibility, affordability, and convenience, providing developers with a plethora of educational materials at their fingertips. By tapping into online resources, developers can broaden their knowledge base, acquire new skills, and stay abreast of industry advancements.
Community Engagement: Community Engagement emphasizes the value of networking, collaborating, and sharing knowledge within the programming community. This segment encourages developers to participate in forums, workshops, conferences, and open-source projects to foster meaningful connections and knowledge exchange. The key characteristic of Community Engagement is its collaborative nature, where developers can seek mentorship, receive feedback, and contribute to the collective growth of the programming community. By engaging with peers and industry experts, developers can cultivate a supportive network, gain insights from diverse perspectives, and nurture a culture of continuous learning and innovation.
Career Paths: Career Paths outline the various trajectories, specializations, and growth opportunities available to individuals pursuing a career in programming and software development. This segment explores different roles such as software engineer, web developer, data analyst, and systems architect, providing insights into the skill sets, responsibilities, and career progression within each domain. The key characteristic of Career Paths is their informative nature, equipping individuals with the knowledge and resources to make informed career choices and strategic career moves. By understanding various career paths and industry trends, individuals can align their skill development efforts, pursue rewarding opportunities, and embark on a fulfilling career journey in the dynamic realm of programming and software development.