Mastering Programming Languages: A Complete Guide
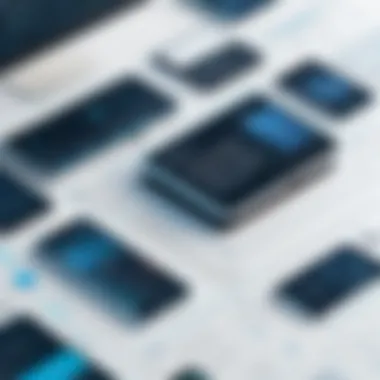
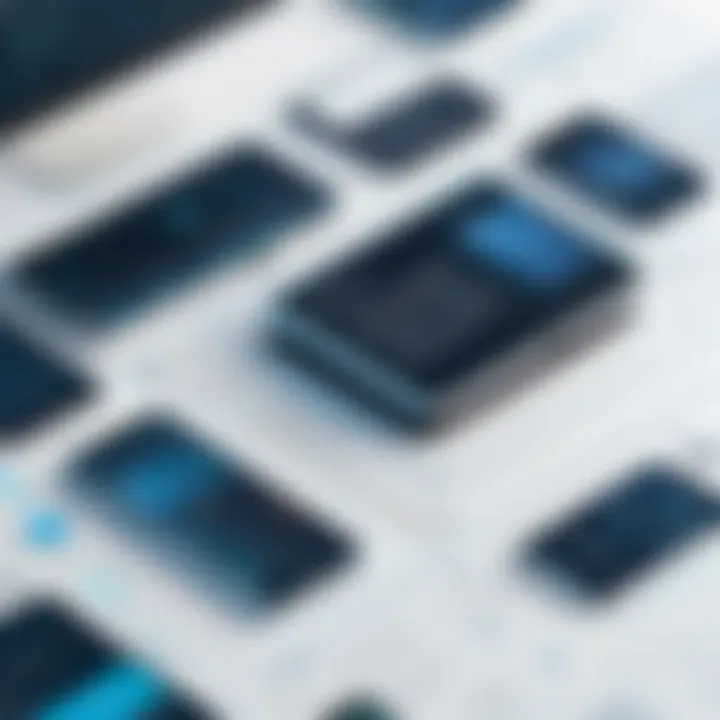
Foreword to Programming Language
Programming languages sit at the heart of technology, acting as the bridge between human intentions and machine actions. They allow programmers to convey commands to computers, initiating an array of functions that result in modern computing applications. Grasping programming languages is not merely about learning syntax but requires an understanding of their underlying principles, history, and the contexts in which they thrive.
History and Background
The evolution of programming languages can be likened to the chapters of a book, each page revealing a new stride toward greater complexity and capability. The earliest forms of programming were mere machine codes, reliant on the specific architecture of the hardware. As needs multiplied, assembly language emerged, allowing for more human-readable code.
By the late 1950s, high-level languages such as Fortran and Lisp provided abstractions that simplified the coding process, emphasizing problem-solving rather than the mechanics of hardware. This shift marked a significant leap forward, facilitating rapid advancements in software engineering and paving the way for today's popular languages like Java, C, and C++. Knowing where these languages come from helps learners appreciate their design philosophies and applications in todayās tech landscape.
Features and Uses
When diving into a programming language, understanding its features is crucial. Each language brings unique characteristics tailored to different tasks:
- Java: Known for its portability, Java underpins many enterprise-level applications and Android development. Its 'write once, run anywhere' promise is a cornerstone of its popularity.
- C: Esteemed for its performance and close-to-hardware capabilities, C is often the preferred choice for system programming and scenarios needing direct manipulation of hardware resources.
- C++: Building upon the foundations of C, C++ integrates object-oriented programming (OOP), making it suitable for software requiring a strong structure and scalability, like game development or complex simulation programs.
The application of these languages speaks to the diverse challenges developers face daily. Each choice reflects both the nature of the task and the developerās personal comfort with the language's constructs.
Popularity and Scope
The landscape of programming languages is ever-changing, influenced by emerging technologies and industry demands. According to various indices, languages such as Python have surged in useāespecially in data science and AIāwhile historical giants like Java remain staples for many large systems. Understanding this dynamic aids learners in aligning their educational paths with industry trends, ensuring their skillsets remain valuable.
"Programming isn't about what you know; it's about what you can figure out."
This concept emphasizes the importance of adaptability in learning new languages as technology evolves.
In summary, a programming language isnāt merely a tool; itās a living entity that shapes our interaction with technology. Analyzing its history, features, and current landscape prepares aspiring programmers for the journey ahead. As we turn the page to the next sections, we will explore the fundamental concepts that form the bedrock of programming, bridging theory with hands-on examples.
Understanding Programming Languages
Programming languages serve as the backbone of software development. They enable developers to communicate with computers, crafting the invisible machinery that powers our digital world. This section unpacks the core concepts surrounding programming languages, recognizing their significance and various facets that shape the landscape of coding today.
Definition and Importance
At its core, a programming language is a formal set of instructions that can be understood and processed by computers. It allows developers to write code that dictates how a computer should perform specific tasks. The importance of these languages cannot be overstated, as they are the primary medium through which we instruct machines.
The reality is that in our tech-savvy age, programming languages offer myriad benefits:
- Problem-Solving: They enable individuals to create solutions for real-world issues.
- Innovation: With the help of programming languages, new applications and systems are continuously developed, energizing industries.
- Career Opportunities: Proficiency in programming languages opens doors to a wide range of jobs across different sectors, transforming career trajectories.
History of Programming Languages
Programming languages have evolved significantly since their inception. The journey begins with machine language, a low-level language that is nearly incomprehensible to humans. Over time, assembly languages emerged, simplifying coding significantly.
Then came high-level languages, pioneered by Fortran in the 1950s, which made programming more accessible. Following Fortran, a slew of languages appeared, including COBOL and C, bringing about a paradigm shift in software development. Modern languages like Python and JavaScript have since redefined programming, emphasizing readability and ease of use. This evolution is a testament to how programming languages strive to meet the needs of developers while adapting to an ever-changing technological landscape.
Types of Programming Languages
Programming languages can be categorized based on various criteria. Understanding these categories helps in selecting the right language for a given project.
Compiled vs. Interpreted Languages
When categorizing programming languages, one key aspect to consider is whether they are compiled or interpreted. Compiled languages, such as C and C++, utilize a compiler to translate the entire program into machine code before execution. This process often results in fast performance and efficient resource management.
On the flip side, interpreted languages like Python and Ruby are executed line-by-line by an interpreter at runtime. This characteristic enhances flexibility and ease of debugging, making them a popular choice for many developers, especially beginners. However, it can lead to slower performance compared to their compiled counterparts.
High-Level vs. Low-Level Languages
Another important distinction in programming languages lies in their abstraction level. High-level languages such as Java and Python allow developers to write code that is closer to human language, making it easier to read and maintain. This abstraction facilitates the development of complex software with less effort and enables a broader range of developers to participate in the programming process.
Conversely, low-level languages like Assembly provide little abstraction from a computerās instruction set. They are often crucial for system-level programming due to their efficiency but can be less user-friendly and more challenging to work with for most developers.
Scripting Languages
Scripting languages, including JavaScript, Perl, and Shell, play a vital role in programming environments. They are typically interpreted and are often embedded within other applications to automate tasks. One of the remarkable aspects of scripting languages is their versatility.
They are well-suited for quick development cycles and are frequently used in web development to create interactive features. However, they may lack the robustness and performance efficiency of compiled languages, which can affect their applicability in more intensive operations. Thus, while scripting languages offer many advantages, they're best utilized alongside traditional programming paradigms in a layered architecture.
The choice of programming language impacts how efficiently and effectively developers can solve problems and address user needs.
In summary, understanding the various types of programming languages equips learners with the perspective necessary for informed decision-making, allowing them to tailor their approach based on project requirements and personal preference.
Java: An Preface
Java plays a pivotal role in the world of programming languages, being not just a popular choice but often a go-to for many developers. Its adaptability and portability make it a strong candidate for a variety of applications. With a syntax that is both straightforward and robust, Java allows new programmers to grasp core concepts without getting entangled in complex rules. Understanding Java's significance in today's tech landscape opens doors to numerous careers in software engineering, mobile app development, and web applications.
Java Syntax and Structure
At its core, Java is designed to be easy to read and write. The basic syntax employs familiar constructs from C and C++, making it easier for those with a background in those languages to transition. Code in Java is structured into classes and methods, establishing a clear framework for developers to follow.
For instance, a simple Java program to print "Hello, World!" is structured as follows:
This clarity and organization not only help in better understanding but also enhance code maintainability, a trait that is highly coveted in software development.
Object-Oriented Programming Concepts
Java is fundamentally an object-oriented language, which means it revolves around the concept of objects. This approach is essential in encapsulating data and behavior, making it a flexible and powerful paradigm.
Classes and Objects
Classes are blueprints for creating objects. Each class can contain fields and methods, which defines the structure and behaviors of the objects created from it. The key characteristic of classes and objects is reusability, allowing developers to create applications that can be modified easily without extensive rewrites.
For example, defining a class for a car might look like this:
The unique feature here is that objects can be instantiated from the class, each with its individual state. This encapsulation of attributes simplifies the complexity of the programs.
Inheritance
Inheritance is a mechanism wherein one class can inherit fields and methods from another. This is a great way to promote code reusability, allowing new classes to extend existing ones without rewriting common functionality. For instance, if a new class called inherits from the class, it can leverage the predefined attributes and methods, while also introducing any new features specific to electric vehicles.
This hierarchical structure helps in organizing codebases effectively, leading to better maintenance and scalability.
Polymorphism
Polymorphism allows one interface to be used for different underlying forms. In Java, it enables methods to perform different functions based on the object that itās acting upon. This aspect is particularly useful when designing systems that require components to interact flexibly.
Consider a method that accepts an object of type . Depending on whether it's called with an instance of or , the corresponding method executes the appropriate behavior designated for each.
Encapsulation
Encapsulation promotes restricted access to certain components, which means an object's internal state can only be changed by its own methods. This leads to reducing unintended interference and misuse of the members, hence improving security within the code.
Default access modifiers can control the visibility of classes, methods, and variables, ensuring that encapsulation principles are met effectively.
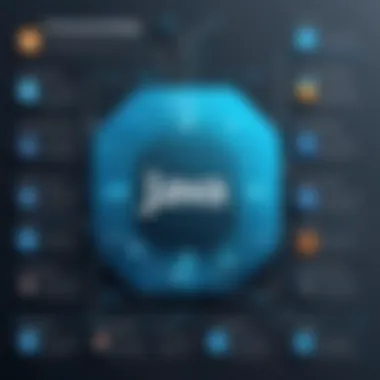
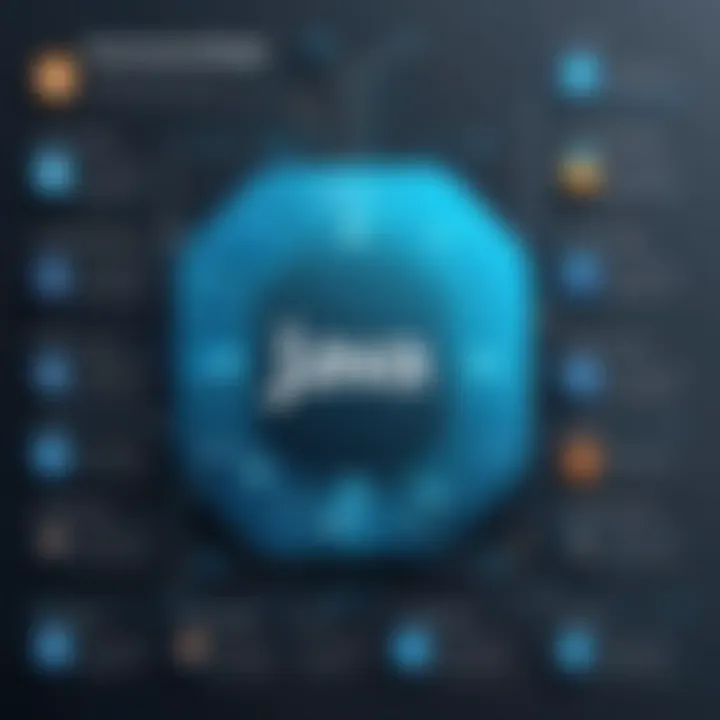
Practical Applications of Java
Java's utility extends far beyond basic programming tasks. Its versatility has allowed it to dominate various sectors, making it an integral part of the technology stack for many enterprises.
Enterprise Applications
Java is widely used for building large-scale enterprise applications, thanks to frameworks like Spring and Hibernate. These tools provide robust security, performance, and scalability features. Enterprises favor Java for its ability to handle vast amounts of transactions with surprising efficiency. Thus, it's no wonder Java has become synonymous with enterprise solutions.
Mobile Development
In the realm of mobile application development, Java is fundamental to the Android ecosystem. The Android SDK relies heavily on Java, offering spacious opportunities for developers to build applications that run on millions of devices globally. The performance efficiency and strong community support for Java further solidify its choice in this domain.
Web Services
Java serves as a backbone for many web services, using technologies like Java EE and Spring Boot. It facilitates building scalable, flexible, and secure web applications. The unique feature is its platform independence, meaning applications built in Java can run on any device that supports Java without modification. This flexibility and the ability to integrate with numerous protocols make Java a strong player in web development.
Overall, Java's structure, combined with its wide-ranging practical applications, presents it as a programming language not only relevant in todayās landscape but crucial for aspiring programmers aiming to make their mark.
Language Fundamentals
C language stands as one of the most significant programming languages in the world of software development. It's hailed for its efficiency and is considered a foundational technology in the programming landscape. Understanding C language fundamentals is crucial not just for budding programmers, but also for seasoned developers who wish to deepen their grasp of how languages work at a lower level. C often acts as a stepping stone to more complex languages, allowing learners to appreciate the underlying mechanics of programming.
Basic Syntax and Functions
At the heart of learning any programming language is its syntax. In C, the syntax is relatively straightforward, making it an ideal starting point for newcomers. C relies on a combination of keywords, identifiers, and symbols to create statements. Here's a basic outline of how functions are defined in C:
In this example, directs the preprocessor to include the standard input-output library. The function acts as the entry point of the program. This simplicity in structure allows learners to focus more on functionality rather than syntax complexity.
Control Structures in
Control structures are fundamental in steering the flow of a program. They dictate how the program responds under different conditions.
Conditional Statements
Conditional statements, like , , and , are essential for decision-making in C programs. These constructs allow the software to execute different code paths based on specific conditions. For instance, consider the following code:
The key characteristic of conditional statements is their power to introduce logic into coding. They help streamline the thought process in coding by mimicking real-life decision-making. Such statements are a popular choice due to their effectiveness in writing clean, logical structures. However, they can become unwieldy with excessive nesting, leading to less readable code.
Loops
Loops, such as , , and , are used to execute a block of code multiple times until a certain condition is met. This feature is vital for tasks where repetition is required. For example:
The versatility of loops allows programmers to efficiently handle large data sets and repetitive tasks. They are celebrated choices among programmers for these very reasons. However, improperly defined loop conditions can lead to infinite loops, rendering the program non-responsive.
Switch Cases
Switch cases provide a structured way to handle multiple conditions based on the value of a variable. This is akin to having several statements, but in a more organized manner.
One of the key features of switch cases is their ability to enhance code clarity and manageability when dealing with many conditions. This makes them a beneficial option for scenarios where numerous outcomes are possible. However, they lack flexibility compared to conditional statements, as the cases cannot be evaluated with complex expressions.
Memory Management Techniques
Memory management is crucial in C, given that it allows programmers to directly control memory allocation and deallocation. Understanding how to manage memory effectively can prevent resource wastage and improve performance considerably.
Pointers
Pointers in C are variables that store memory addresses. They provide a robust way to handle memory and enable dynamic data structures. For example:
The strength of pointers lies in their ability to give direct access to memory locations. However, they can be tricky for beginners, potentially leading to errors like dereferencing null or uninitialized pointers.
Dynamic Memory Allocation
Dynamic memory allocation allows programs to request memory during runtime, which is essential for structures like linked lists or dynamic arrays. Functions like and are commonly used for this purpose. Here's a basic example:
The primary advantage of dynamic memory allocation is flexibility; you can allocate memory as needed. Yet, it requires careful management, as forgetting to free memory can lead to memory leaks, which are detrimental to resource usage.
Memory Leak Prevention
Memory leaks occur when memory is allocated but not properly deallocated. This can accumulate over time, eventually leading to significant performance issues. To prevent memory leaks, itās essential to implement rigorous memory management techniques, such as pairing every with a .
The key characteristic of memory leak prevention is ensuring system efficiency. Addressing leaks can significantly enhance the stability of applications, yet it demands discipline and continuous vigilance in coding practices. By emphasizing responsible memory management techniques, programmers can substantially improve program reliability and performance.
++: An Advanced Perspective
C++ stands out as a powerful and versatile programming language used widely for various applications. Delving into this language provides insights into why it has maintained its popularity and relevance over the decades. C++ combines the efficiency of low-level languages with the flexibility of high-level programming paradigms, making it ideal for systems programming, game development, and real-time applications. Understanding advanced concepts in C++ lays a foundation for mastering its intricacies and optimizing code performance. Furthermore, it teaches programmers to think about how software can be structured and implemented across different systems.
Advanced OOP Concepts
Abstraction
Abstraction in C++ serves as a cornerstone of object-oriented programming (OOP). In essence, abstraction allows programmers to manage complexity by hiding unnecessary details and exposing only the essential features of an object. It's like using a car; you donāt need to understand how the engine works to drive it. This characteristic makes abstraction a favored approach within this article, as it simplifies problem-solving and enhances code clarity.
Unique features of abstraction in C++ include abstract classes and interfaces, which facilitate a design pattern that promotes loose coupling. However, one of the disadvantages might be that overlying too much on abstraction can sometimes lead to performance overhead due to the extra layer of abstraction involved.
Friend Functions
Friend functions are another notable feature of C++. They offer the ability for functions to access the private and protected members of a class. This aspect is helpful for operator overloading and other scenarios where functions need to work closely with the underlying implementation of a class without becoming part of its interface.
The key benefit of friend functions is their flexibility; they allow for clean, efficient code while still maintaining encapsulation. However, the downside is that introducing friend functions can blur the lines of encapsulation, leading to tightly coupled code if not used judiciously.
Operator Overloading
Operator overloading reflects C++'s ability to extend the usability of operators and enhance code readability. By defining how operators work with user-defined types, it allows programmers to use natural syntax while performing operations. For instance, if you create a class for complex numbers, you can make the operator work seamlessly with them just like it would for regular integers.
This unique characteristic enhances the expressiveness of the code, making it easier to read and maintain. However, overusing operator overloading can potentially lead to confusion, especially if it deviates from standard expectations, which might surprise users of the code.
Templates and Standard Template Library
C++ supports templates, an advanced feature that enables programmers to write generic and reusable code. Templates allow functions and classes to operate with any data type without sacrificing performance. The Standard Template Library (STL), a powerful feature of C++, provides a collection of algorithms and data structures, promoting code reusability and efficiency. An example of STL's efficiency is how it simplifies complex operations, such as sorts or searches, without needing to rewrite the logic each time.
Real-World Applications of ++
Game Development
Game development is one of the leading industries relying heavily on C++. Its ability to manage resources and provide real-time performance makes it favorable for developing high-quality gaming experiences. The object-oriented features support complex graphics and physics engines. A unique feature in game development is the utilization of C++ for creating cross-platform games, which can be run on multiple systems with minimal adjustments. The downside is that the learning curve can be steep for beginners, given the complexities involved.
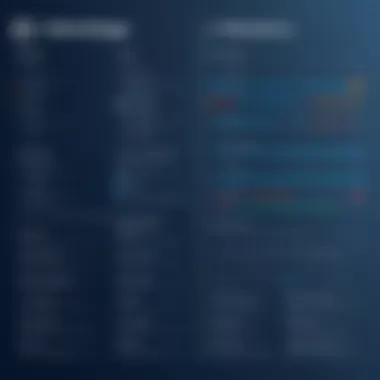
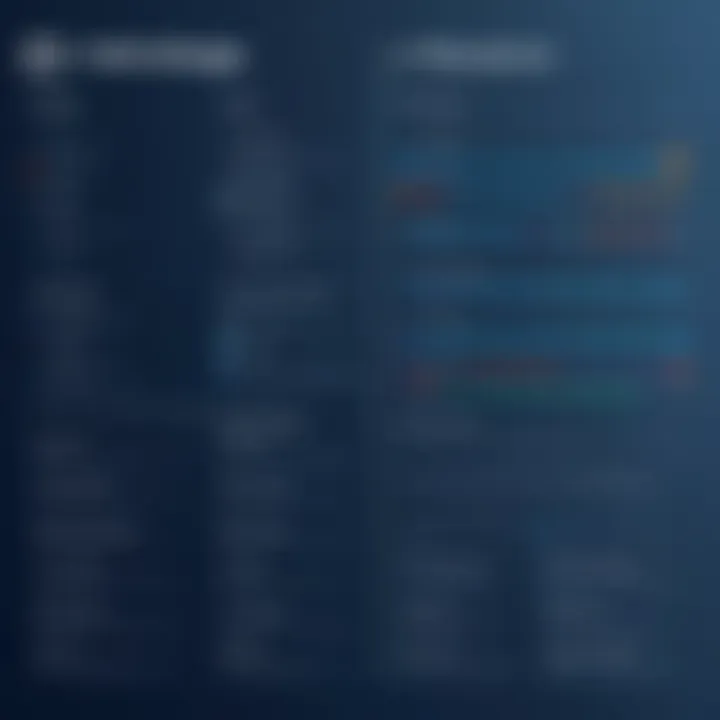
System/Software Development
System and software development leverages C++ for its low-level manipulation capabilities. The language provides programmers direct access to hardware, crucial for developing operating systems and device drivers. Being able to control memory and processes gives C++ a distinct advantage over higher-level languages in terms of performance and efficiency. However, developing systems in C++ requires a careful approach to memory management, as improper handling can lead to issues such as memory leaks.
Graphics Applications
Graphics applications owe much to C++ due to its ability to handle intensive computations with ease. The language is often used in rendering engines for real-time graphics and high-end visual effects, which require performance and precision. The performance of graphics applications in C++ can torque around with frameworks such as OpenGL or DirectX, providing direct access to graphics hardware. Nevertheless, C++ can sometimes lead to more complex code compared to higher-level languages, raising the barrier for newcomers in the graphics domain.
In summary, C++ reveals a multitude of advanced features making it a top-tier choice for applications demanding high performance, scalability, and effective resource management. Understanding its rich paradigms can elevate oneās programming capabilities, bridging the gap between fundamentals and sophisticated technical applications.
Best Practices in Programming
Best practices in programming are pivotal for transforming raw code into maintainable and efficient structures. They act as the backbone of professional coding, guiding developers through challenges like readability, team collaboration, and project longevity. Adopting these practices can save time, reduce errors, and make the code easier to understand, not only for the original creator but also for future generations of programmers. In the fast-evolving tech landscape, honing oneās skills in following best practices is essential.
Code Readability and Documentation
Code readability might sound like a buzzword, but it's more like the golden rule for any programmer. The idea is simpleāif your code appears as if it were scribbled in a foreign language by a sleepy toddler, nobody, including you, will want to work on it later. Clear and self-explanatory code allows both the creator and other developers to grasp what's happening at a glance.
Effective documentation complements readable code. Think of it as the unwritten laws of your coding universe. It provides insights about function purposes, expected inputs, and potential outputs. Tools like Javadoc for Java or Doxygen for C/C++ help automate documentation, making the process a little less tedious. Therefore, well-documented and readable code can significantly ease future updates and debugging efforts.
Version Control Systems
Version control systems are like safety nets for developers. They track and manage changes to code, offering ways to revert back to previous states in case things go awry. Among them, Git stands out due to its flexibility and robustness.
Git Basics
Git operates on the principle of snapshots. Each time you save your work, Git captures the current state. This feature allows you to revisit earlier stages easily. Gitās branching capability is another key aspectāit lets developers create separate paths for new features or fixes, without jeopardizing the main codebase. This modular approach ensures that experimental features can be tested without affecting the overall project.
Branching Strategies
Branching strategies are critical for effective teamwork. A well-thought plan helps organize features and fixes. Common strategies include the Git Flow, which uses multiple branches for features, releases, and hotfixes, providing clarity. This method promotes better collaboration among team members, enabling them to work asynchronously. However, if not managed carefully, branching can lead to a tangled web of disconnected code.
Pull Requests
Pull requests serve as an essential checkpoint before merging code changes. They invite team discussions on new features or fixes, fostering collaboration and improvements before they become permanent. This process reduces misunderstandings and enhances code quality through peer reviews. However, over-using pull requests can bog down the development process, so finding a balance is key.
Testing and Debugging Techniques
Testing and debugging can often feel like a chore. Yet, they are indispensable for ensuring that code runs smoothly and fulfills its intended purpose. Various techniques exist to address possible pitfalls in coding.
Unit Testing
Unit testing isolates individual components of a program to verify their correctness. By providing a safety net during development, unit tests can catch errors early on. Furthermore, having a robust set of unit tests allows for safer code refactoring, enhancing the overall integrity of the software. However, writing comprehensive unit tests can require extra time and effort up front, which might be seen as a drawback.
Integration Testing
Integration testing builds on unit testing by examining how different modules of an application interact. By pinpointing issues that can arise when various pieces work together, integration testing can reveal bugs that might go unnoticed in unit tests alone. Although it adds another layer of complexity, itās crucial for quality assurance before deploying code.
Common Debugging Tools
Debugging tools, like GDB for C/C++ or Visual Studio's debugger for C#, play a vital role in diagnosing and solving issues in code. These tools allow developers to step through code, inspect variable states, and modify code on the fly. The catch? Learning to effectively use these tools requires practice and patience, but the payoff is considerable when it comes to time saved in troubleshooting.
Effective programming is not just about writing code; itās about writing good code. Following best practices ensures that programming becomes less of a headache and more of a craft.
Navigating the Development Environment
In today's programming landscape, the development environment serves as the foundation for crafting code effectively and efficiently. Understanding how to navigate this environment is vital for programmers at all levels. A well-set-up environment can significantly affect productivity while allowing for the seamless integration of various tools necessary for coding, testing, and deployment. As technology progresses, having a firm grip on this aspect can enhance your programming skills by providing you with the right suite of tools to accomplish your tasks.
When writers say, "Feeling comfortable in your space is key to success!" they might be hinting at the importance of personalization in your development setting. An optimized environment includes Integrated Development Environments (IDEs), command-line tools, and customization options that make coding smoother.
Integrated Development Environments (IDEs)
IDEs are comprehensive software applications that provide all the tools a developer needs to write, test, and debug code in one package. The convenience they offer is unbeatable, saving time and reducing the complexity associated with coding tasks. These environments are often user-friendly and come with powerful features that assist in maintaining code quality. Letās take a closer look at popular IDEs for Java, C, and C++ to understand their distinct characteristics.
Popular IDEs for Java
The world of Java programming has several standout IDEs, one being IntelliJ IDEA. This tool is well-known for its robust features and unmatched support for various languages, though its deep integration can take some time to learn. The key characteristic of IntelliJ is its intelligent code completion, which aids developers in writing efficient code faster.
Another highly regarded option is Eclipse, particularly loved for its versatility and extensibility through plugins. A notable unique feature of Eclipse is the ability to work with multiple programming languages seamlessly. However, its complexity can be intimidating, especially for newcomers.
Advantages of using these IDEs include:
- Extensive support for frameworks and libraries.
- Built-in version control capabilities.
- User-friendly interface that streamlines the coding process.
Disadvantages might involve:
- A steep learning curve for beginners.
- System resource demands, especially with larger projects.
IDEs
When it comes to C programming, Code::Blocks is a favorite among many developers. This IDE promotes a customizable interface, allowing for layouts that fit personal preference, which is particularly appealing for beginners. Its key characteristic is the ability to configure build options easily.
Other notable IDEs include Dev-C++, which promotes simplicity and ease of use, especially for those just starting with C code. One unique feature is its straightforward interface, making it less daunting for new coders, though it might lack some advanced functionalities found in other IDEs.
Key advantages include:
- Lightweight design leading to faster load times.
- Effective for both small-scale and larger projects without overwhelming the user.
Downsides include:
- Limited features compared to heavier IDEs.
- Some might find debugging not as comprehensive.
++ IDEs
For C++, Visual Studio is often deemed the gold standard. Its key characteristic is the deep integration with Microsoft's ecosystem, making it the go-to for Windows-based applications. This IDE shines when it comes to debugging, providing a fine-tuned experience.
Another popular option is CLion, which is particularly robust for larger projects. With its unique feature of refactoring assistance, programmers can enhance existing codebases with relative ease.
Benefits of using these C++ IDEs include:
- Comprehensive debugging tools.
- Strong support for larger team configurations.
However, potential restrictions might be:
- High resource consumption, making it sluggish on older machines.
- The cost associated with some features of Visual Studio could deter freelance developers.
Command Line Tools
Command line tools, while often overlooked, provide developers with a lightweight alternative to bulky IDEs. Getting a grip on compiling, running scripts, and using Makefiles is like learning to ride a bike - once you've got the hang of it, youāre set to go anywhere.
Compiling Programs
Compiling is an essential aspect when turning source code into executable programs. In this context, tools like GCC (GNU Compiler Collection) are invaluable. Key characteristics of this tool include its support for various programming languages and its active community behind it.
One unique feature is its modular approach, allowing developers to choose only what they need. Its ability to manage complex projects and dependencies stands out as an advantage.
However, hereās a caveat: In the wrong hands, GCC can lead to cryptic error messages that bewilder even seasoned programmers.
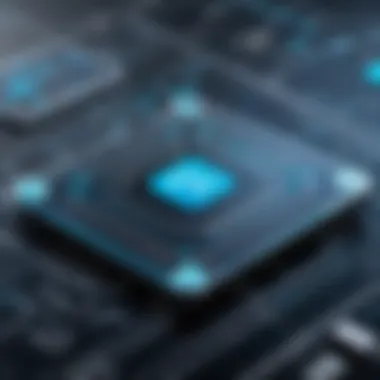
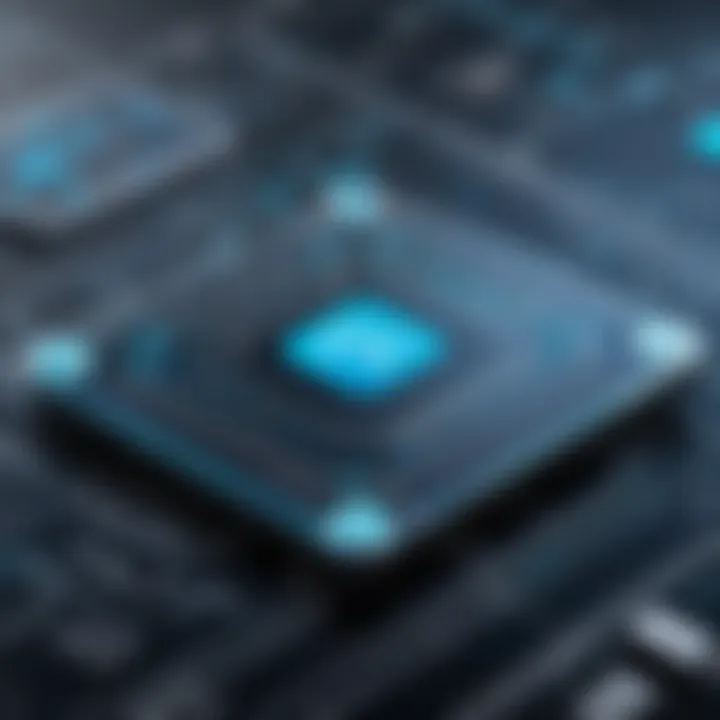
Running Scripts
Running scripts is all about execution. Tools like Bash provide an efficient way to execute scripts in Unix-like environments. The key characteristic here is the versatility offered in managing tasks through shell scripts. Itās particularly revered for its simplicity and powerful command execution.
One unique feature of Bash is its ability to automate repetitive tasks, which can save hours during tedious developments. Yet, it may also be overwhelming for new users unfamiliar with command-line syntax.
Using Makefiles
Makefiles are like the air traffic controllers of programming tasks. They help manage the build process seamlessly, especially in larger projects. The key characteristic of Makefiles is their ability to automate build commands.
One appealing unique feature is the capability to only rebuild files that have changed, making the process more efficient. This flexibility provides a clear advantage in managing complex projects but may pose a challenge for new users trying to grasp their structure.
Customization of IDEs
Customizing your IDE is akin to personalizing your workspace - it reflects your style and efficiency. Streamlining toolbars, adjusting themes, or setting up specific shortcuts can drastically enhance the coding experience. This customization not only helps in optimizing workflow but also minimizes distractions, allowing for deeper focus.
Each developer may prefer a different style, so finding the right balance between aesthetic and functionality is crucial.
By taking the time to personalize your IDE, you invest in a setting that supports your unique coding journey. Through smoother navigation and tailored features, the pathway to proficiency undoubtedly becomes less daunting.
Exploring Emerging Programming Languages
The digital world is constantly changing, and so are the languages we use to build it. Exploring emerging programming languages is a vital undertaking in todayās tech environment. It opens doors to new possibilities, enhances development efficiency, and caters to specific needs in the market. Here we have three up-and-coming players worth diving into: Rust, Go, and Swift. Each language brings unique strengths and emphasizes different aspects of programming. Understanding these languages can enhance a developer's toolkit significantly.
Rust: The New Frontier
Rust is making waves in the programming community for several good reasons. First and foremost, it focuses on memory safety without a garbage collector, which means it can do what languages like C or C++ do but with fewer risks related to memory corruption. This is a game changer, especially in system-level programming where errors can cause catastrophic failures.
Rust emphasizes concurrency, enabling developers to write code that efficiently runs across multiple threads. In practice, this means you can maximize performance, especially in high-load environments.
Furthermore, the growing popularity of Rust is often linked to its comprehensive tooling and documentation, making it easier for newcomers to adopt. The community around Rust is vibrant and supportive, ensuring learners and developers have the resources they need.
"Memory safety is not just a feature of Rust; itās its core philosophy."
Go Language and Its Applications
Go, also known as Golang, is yet another language that's carving its niche. Developed by Google, it's designed for simplicity and efficiency, making it easy to read and write. These qualities make Go a favorite among teams looking to build reliable and scalable web services quickly.
One of its standout features is the goroutine, which allows functions to run concurrently, making it excellent for handling multiple tasks at once. This is particularly beneficial in cloud computing environments. Organizations increasingly turn to Go for building microservices, as it simplifies communication between various components of an application.
Moreover, Go's strong community widespread support ensures a rich ecosystem of libraries and frameworks that can speed up development. Developers often find themselves producing cleaner code with fewer lines, which is a win-win.
Swift: Programming for Apple Ecosystem
Swift is Apple's answer to modern programming challenges. It was created to work seamlessly across their ecosystem, including macOS, iOS, watchOS, and tvOS. One of its primary goals is to make coding simple for new developers while being robust enough for seasoned programmers.
The language emphasizes performance and safety, encouraging best practices like type inference, optionals, and error handling. With these features, Swift helps developers write less error-prone code. Swift also has a strong focus on interoperability with Objective-C, which leverages legacy code while moving forward.
Furthermore, Swiftās widespread adoption within the Apple developer community showcases its efficacy. Apple constantly updates it with new features and improvements, ensuring it remains a competitive choice in mobile and desktop applications.
Resources for Continued Learning
In the ever-evolving field of programming, continual learning is not just a luxury; itās a necessity. Staying current with the latest trends, languages, and technologies greatly enhances one's programming journey and significantly boosts career prospects. Resources for continued learning provide learners with the tools to adapt to new challenges, refine their skills, and broaden their knowledge base. More than just textbooks or courses, these resources create an ongoing dialogue in the programmer community where exchange of ideas fuels innovation and development.
Online Courses and Certifications
Online courses have transformed the way we learn programming. Unlike traditional classroom settings that often feel constrained, online platforms offer a flexible approach. Popular sites like Coursera, Udemy, and edX provide a broad spectrum of programming courses tailored to all skill levelsāfrom complete novices to seasoned professionals. One significant advantage of these platforms is the availability of accredited certifications that can enhance a resume, giving job seekers an edge in a competitive market.
While enrolled, learners can enjoy interactive content, quizzes, and community discussions. These elements not only keep the material engaging but also ensure comprehension through practical application.
Considerations for choosing the right course include:
- Course Content: Make sure it aligns with your goals.
- Instructor Expertise: Look for instructors who have both practical experience and teaching credentials.
- Reviews and Ratings: Previous student feedback can provide insights into course quality.
"The beauty of online learning is its flexibility; you can upskill during your lunch break or while sipping coffee on the weekend."
Books and Literature on Programming
Books remain an excellent resource for diving deeper into programming concepts. Many readers find that the tactile experience of flipping pages fosters a better understanding than staring at a screen. Literature on programming covers everything from introductory topics to advanced theories. Titles such as "Clean Code" by Robert C. Martin or "You Donāt Know JS" by Kyle Simpson are indispensable for grasping core principles.
When selecting books, pay attention to:
- Relevance: Ensure the material is not outdated; technology moves fast!
- Author Credentials: Authors with industry experience offer practical insights that theory alone can't provide.
- Community Recommendations: Join forums or Reddit discussions to see what others are finding valuable.
Community Forums and Support
Finding a community can be just as crucial as formal education. Platforms like Reddit have subreddits dedicated to the discussion of various programming languages, where learners can ask questions or share experiences. Communities foster collaboration, where learners can find support as they tackle difficult concepts or projects. Moreover, discussions offer practical, real-world problem solving that textbooks may overlook.
Some popular online forums and community resources include:
Active participation in these forums can significantly enhance understanding, as questions often spark valuable discussions that widen perspectives. Engaging in coding challenges and group projects can also solidify skills while providing immediate feedback.
As you continue your programming journey, remember that resources are plentiful. The key is to leverage them wisely to create a tailored learning experience that suits your personal and professional ambitions.
Future Trends in Programming
As we advance into an increasingly tech-forward era, understanding the future trends in programming becomes paramount for both seasoned developers and newcomers alike. These trends arenāt just passing fads; they shape the landscape of software development, redefining how programmers approach problem-solving and project management. Grasping these emerging patterns can provide insights into the skills one needs to cultivate, the tools to adopt, and the sectors that will thrive in the coming years. Let's delve into some pivotal trends that are setting the stage for the future of programming.
Artificial Intelligence and Machine Learning Integration
Artificial intelligence (AI) and machine learning (ML) are not just buzzwords; theyāre revolutionizing the way we understand and interact with technology.
Incorporating AI and ML into software development can lead to more efficient algorithms, predictive analytics, and automation of repetitive tasks. For instance, developers can leverage ML algorithms to optimize code performance or to detect bugs that would have otherwise gone unnoticed. Tools like TensorFlow and PyTorch are making this integration easier, providing frameworks that allow even beginners to experiment with neural networks.
"The integration of AI in programming isn't just about smarter code; itās about enabling a new wave of creativity and capability in software solutions."
One of the clear benefits of this integration is enhanced user experiences. Applications equipped with AI can learn user behavior and adapt accordingly, dramatically improving functionality and satisfaction. Furthermore, industries such as finance, healthcare, and marketing are observing substantial changes, leading to more data-driven decisions.
The Rise of Low-Code/No-Code Platforms
Low-code and no-code platforms are taking the tech world by storm, catering to those who may not have extensive programming expertise but still seek to create functional applications. These platforms simplify development processes, allowing for rapid prototyping and deployment.
Tools like AppGyver and Bubble have gained traction, enabling users to build applications through intuitive visual interfaces rather than traditional coding.
Here are some benefits of low-code/no-code platforms:
- Accessibility: Empowering more people to contribute to software development regardless of technical background.
- Speed: Fast-tracking project timelines by reducing the need for extensive coding.
- Collaboration: Encouraging teamwork among techies and non-techies alike, bridging gaps in understanding.
While these platforms have their limitationsālike scalability and customizationāthey hold enormous potential in the democratization of programming. Learning to adapt to these tools could prove essential for developers aiming to stay relevant as the landscape evolves.
Adaptability of Programming Languages
As technology moves at breakneck speed, adaptability of programming languages becomes a non-negotiable trait for development. The programming languages that can easily morph to meet new requirements will thrive, while others may fade into obscurity.
Take Python, widely regarded for its versatility; it finds its niche in web development, data analysis, artificial intelligence, and even scripting. Programming languages today are not one-trick ponies, they are expected to handle a multitude of tasks efficiently.
Key considerations for adaptability include:
- Community Support: A robust community can lead to extensive libraries and frameworks, enhancing adaptability.
- Meeting New Challenges: The capacity to integrate with emerging technologies, like blockchain or IoT, is crucial for relevance.
- Learning Curve: Languages that ease the syntax barriers and reduce the steep learning curves tend to attract more developers.
In summary, keeping an eye on the adaptability trends of programming languages is vital for anyone serious about a career in tech. The capacity to evolve with changing environments is what often sets successful developers apart today.