Mastering PHP Form File Upload: A Comprehensive Guide for Effective Implementation
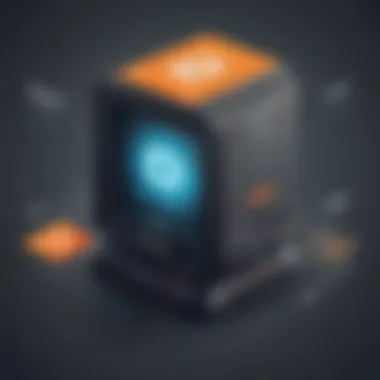
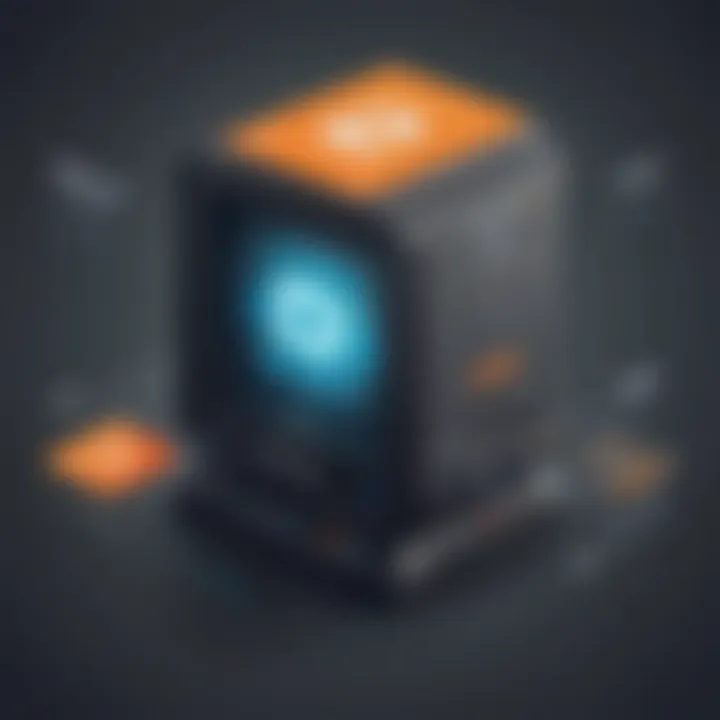
Introduction to PHP
PHP, which stands for Hypertext Preprocessor, is a widely used server-side scripting language, particularly well-known for web development. Launched in 1994 by Rasmus Lerdorf, PHP has evolved over the years to become a versatile language offering a myriad of functionalities. Its popularity stems from its open-source nature, ease of integration with other technologies, and vast community support. Developers primarily use PHP for generating dynamic web pages, handling form data, managing databases, and creating secure login systems.
Basic Syntax and Concepts
When delving into PHP form file upload, understanding the basic syntax and concepts is crucial. PHP is a loosely typed language, meaning variables do not need to be declared with a specific data type. Variables in PHP start with the $ sign, followed by the variable name. Data types in PHP include strings, integers, floats, booleans, arrays, and objects. Operators in PHP are symbols that perform specific operations on variables and values. Expressions, on the other hand, are combinations of values, variables, and operators that, when evaluated, result in a single value. Control structures like loops and conditional statements allow developers to dictate the flow of the program based on certain conditions.
Advanced Topics
In mastering PHP form file uploads, delving into advanced topics such as functions and methods, object-oriented programming (OOP), and exception handling can enhance the code's functionality and maintainability. Functions in PHP are blocks of code that can be repeatedly executed, enhancing code reusability and organization. Object-oriented programming emphasizes creating objects that encapsulate data and functions, promoting code modularity and flexibility. Exception handling in PHP allows developers to gracefully manage errors or unexpected events that may occur during the execution of a program.
Hands-On Examples
One of the most effective ways to master PHP form file uploads is through hands-on examples. Starting with simple programs that illustrate basic file upload functionalities, developers can gradually progress to intermediate projects that involve manipulating and controlling uploaded files. Code snippets are invaluable resources that offer concise and practical solutions to common challenges encountered during PHP form file uploads, serving as building blocks for more complex applications.
Resources and Further Learning
To continue honing PHP form file upload skills, exploring recommended books and tutorials can provide in-depth insights and advanced techniques. Online courses and platforms offer structured learning paths, interactive exercises, and real-world projects to enhance practical proficiency. Engaging with community forums and groups allows developers to seek advice, share experiences, and stay updated on the latest trends and best practices in PHP form file upload development.
Introduction
In the realm of web development, mastering the intricacies of PHP form file upload holds immense significance. Through this comprehensive guide, we delve into the essence of understanding PHP form file upload, exploring its fundamental basics, as well as the critical aspects of security and efficient functionality. This article serves as a beacon for both beginners and intermediate learners, providing them with the tools and insights required to navigate the complexities of PHP form file uploads successfully. By shedding light on practical examples and detailed step-by-step instructions, we aim to equip individuals with the expertise needed to excel in implementing file upload functionalities within PHP forms.
From the very foundational elements of file upload in web development to the advanced nuances of PHP functions tailored for this purpose, this guide illuminates every aspect of PHP form file upload, ensuring a robust understanding and execution of this vital functionality. By focusing on best practices for secure file uploads, the article underscores the importance of file upload security and elucidates common security risks that developers must be cognizant of. By dissecting PHP functions such as , , , and , readers will gain an in-depth comprehension of the mechanisms at play during file uploads.
The subsequent sections of the article delve into the practical implementation of file uploads in PHP forms, elucidating the HTML form setup, the significance of handling file uploads within PHP using the superglobal, and the necessary validation and error handling procedures essential for a seamless user experience. Moreover, the guide offers insights into enhancing user experience through progress bar implementation, feedback mechanisms for successful uploads, and optimization tips to improve file upload performance.
Additionally, the guide delves into the critical realm of securing file upload functionality, addressing the need for sanitizing user input, implementing CSRF protection, and preventing file execution vulnerabilities to bolster the overall security of PHP form file uploads. By synthesizing these multifaceted elements into a cohesive narrative, this article aims to empower readers with a deep understanding of mastering PHP form file upload, equipping them to navigate the complexities of this crucial aspect of web development with confidence and expertise.
Understanding PHP Form File Upload
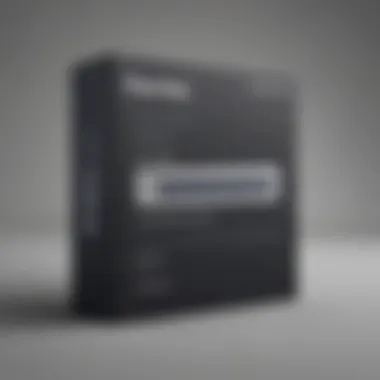
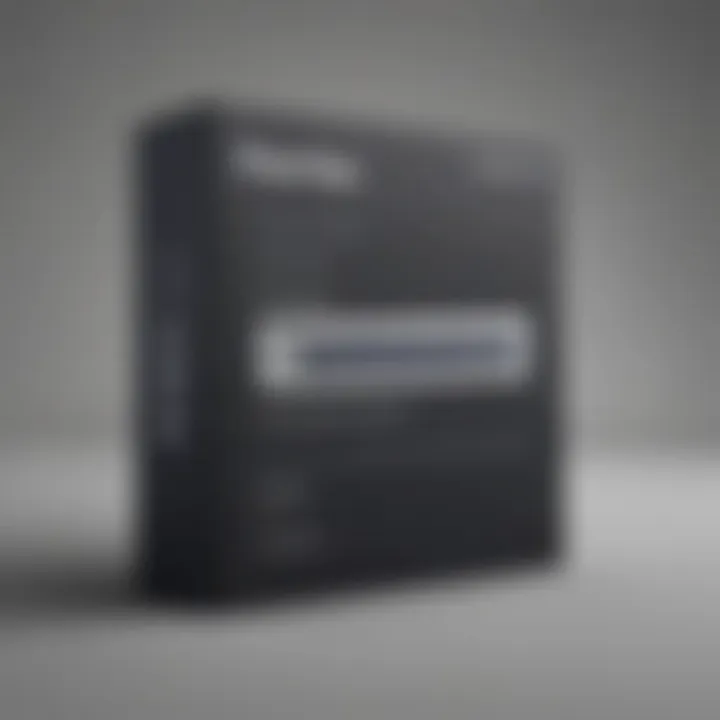
In this comprehensive guide focusing on mastering PHP form file uploads, delving into the core of file upload functionalities in PHP forms becomes paramount. Understanding PHP Form File Upload is vital as it forms the foundation for implementing successful file uploads with PHP. By grasping the intricacies of this topic, beginners and intermediate learners can gain the necessary skills to navigate the complexities of file uploads seamlessly. This section will illuminate the key concepts and best practices essential for executing efficient PHP form file uploads.
Basics of File Upload in Web Development
File upload functionalities play a pivotal role in web development, enabling users to upload various file types such as images, videos, and documents to web servers. Understanding the basics of file upload in web development involves comprehending how files are transferred from client browsers to web servers. It also involves understanding the HTTP protocol's multipart form-data, which facilitates file uploads seamlessly. Through a detailed examination of the fundamentals of file upload, developers can streamline the process and enhance user experience by implementing efficient file upload mechanisms.
Importance of File Upload Security
Common Security Risks
When it comes to file upload security, common risks such as malicious file uploads, CSRF attacks, and file execution vulnerabilities pose significant threats to web applications. Understanding these risks is crucial for implementing robust security measures that safeguard against unauthorized file uploads and potential exploits. By addressing common security risks proactively, developers can fortify their applications and protect user data from potential breaches.
Best Practices for Secure File Uploads
To mitigate security vulnerabilities associated with file uploads, adhering to best practices is imperative. Implementing measures such as validating file types, setting file size limits, and employing secure file storage mechanisms can bolster the security posture of web applications. By following best practices for secure file uploads, developers can create a safer environment for users to upload files without compromising data integrity.
PHP Functions for File Uploads
move_uploaded_file()
The move_uploaded_file() function in PHP facilitates the transfer of uploaded files to a specified destination directory on the server. This function ensures that files are securely moved to the designated location, allowing developers to manage file uploads efficiently.
is_uploaded_file()
The is_uploaded_file() function serves as a fundamental check to verify whether a file was uploaded via an HTTP POST request. By confirming the status of uploaded files, developers can validate the integrity of the uploaded content and prevent unauthorized file uploads.
basename()
The basename() function in PHP extracts the filename from a given path, simplifying file manipulation tasks. By utilizing this function, developers can retrieve the filename associated with uploaded files and perform further operations based on the extracted information.
file_exists()
The file_exists() function verifies the existence of a file in a specified directory, enabling developers to validate file paths before processing uploads. This function plays a crucial role in file upload validation, ensuring that files are correctly accessed and managed within the server's filesystem.
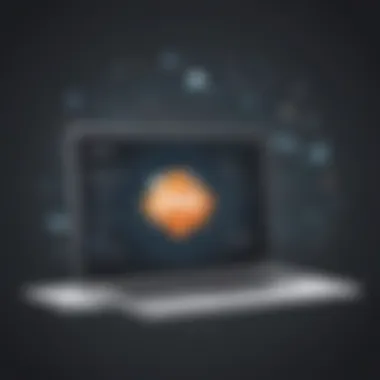
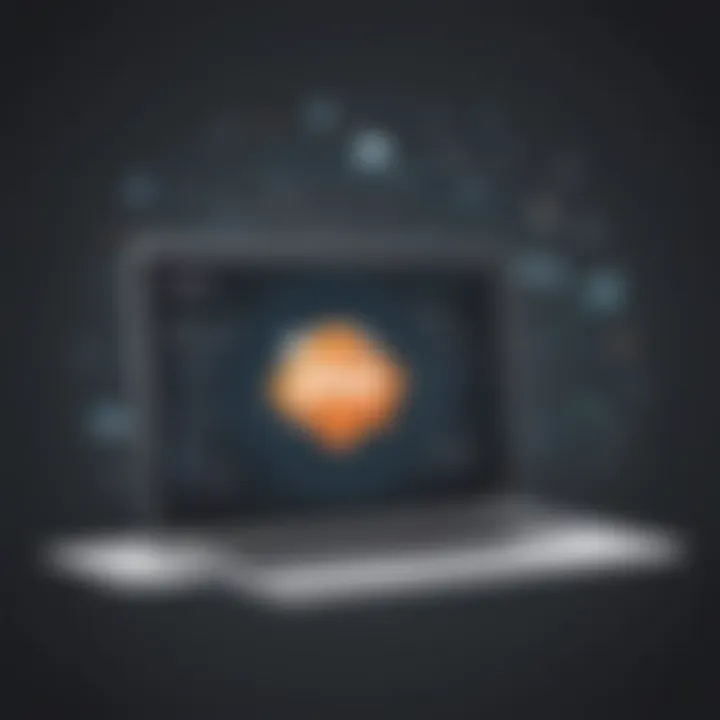
Implementing File Upload in PHP Forms
Implementing File Upload in PHP Forms holds significant importance within the realm of PHP development. It serves as a pivotal aspect in web programming, enabling users to upload files seamlessly through web interfaces. This section delves into the intricacies of integrating file upload functionalities within PHP forms, emphasizing the seamless transfer of data from the client side to the server. By understanding the process of implementing file uploads in PHP forms, developers can enhance user interaction, data collection, and overall website functionality. Through a structured approach to form design and file handling, developers can ensure a robust and secure file upload mechanism that aligns with industry standards and best practices.
HTML Form Setup
Establishing the HTML form structure is the cornerstone of enabling file uploads in PHP. It involves defining the form attributes, input fields, and submit buttons necessary for receiving and processing user-uploaded files. The HTML form acts as the interface through which users interact with the file upload functionality, providing a seamless experience for selecting and transmitting files to the server. Structuring the HTML form correctly ensures compatibility with PHP scripts for handling file uploads, streamlining the process of data submission and processing.
Handling File Upload in PHP
$_FILES Superglobal
The $_FILES superglobal plays a fundamental role in processing file uploads within PHP scripts. It provides developers with access to vital information about the uploaded file, including the file name, type, size, and temporary storage location. By leveraging the $_FILES superglobal, developers can extract and manipulate uploaded file data, facilitating effective file handling and storage operations. Its versatility and ease of use make it a preferred choice for managing file uploads and enhancing the functionality of PHP forms.
File Upload Constraints
File upload constraints are essential parameters that govern the acceptable characteristics of uploaded files. These constraints encompass file size limitations, allowed file types, and additional validation rules that ensure the security and integrity of the uploaded files. By defining and enforcing file upload constraints, developers can prevent malicious file uploads, optimize server resources, and maintain data consistency within the application. Balancing user flexibility with security measures, file upload constraints contribute to a robust and user-friendly file upload experience within PHP forms.
Validation and Error Handling
Checking File Size and Type
Validating file size and type is a critical aspect of file upload functionality in PHP. By verifying the size and format of uploaded files, developers can prevent erroneous data uploads, server overloads, and security breaches. Implementing robust checks for file size and type ensures that only legitimate files meeting the specified criteria are processed, promoting data integrity and system reliability. Effective validation of file attributes enhances the user experience by providing meaningful feedback and instructions for successful file uploads.
Error Messages and Exceptions
Error messages and exceptions play a vital role in guiding users through the file upload process in PHP forms. By generating informative error messages and handling exceptions gracefully, developers can communicate upload failures, validation errors, and system issues effectively. Clear and concise error messages help users troubleshoot upload issues, rectify input errors, and comprehend the necessary steps for successful file submission. Implementing a comprehensive error handling system enhances the overall user experience and streamlines the file upload process in PHP applications.
Enhancing User Experience
In the world of PHP form file upload, enhancing user experience occupies a paramount position. The smooth function of a progress bar not only enhances the overall aesthetic appeal of the website but also provides crucial feedback to users, ensuring they are aware of the upload status. A progress bar acts as a visual indicator, offering transparency and reassurance during the uploading process. Additionally, it decreases user frustration by showcasing the completion status, thereby improving user satisfaction.
Progress Bar Implementation
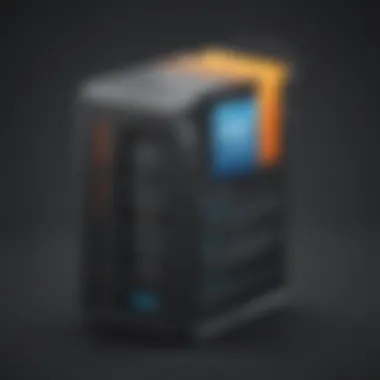
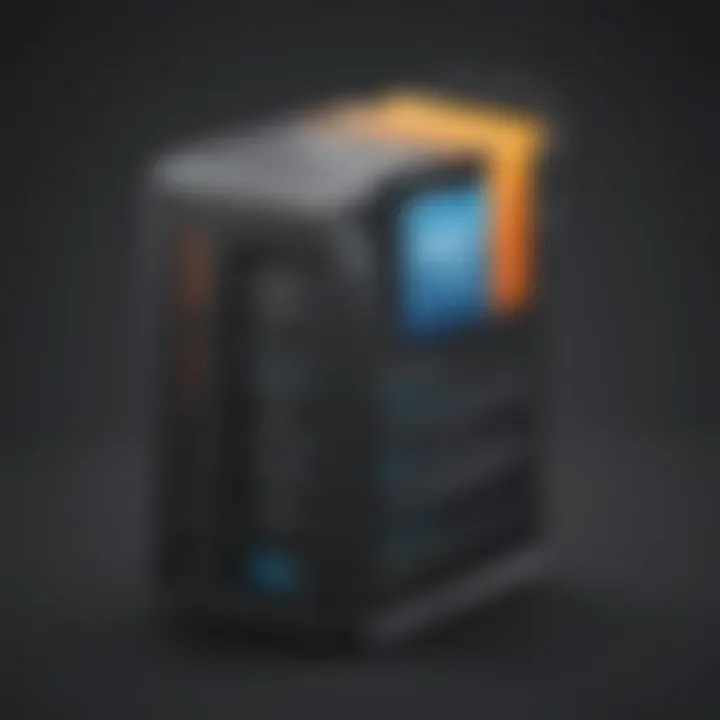
When delving into the realm of progress bar implementation, it is vital to consider various aspects that contribute to its effectiveness. A well-designed progress bar should accurately reflect the upload progress, providing real-time updates to users. By utilizing Java Script or AJAX, developers can create dynamic progress bars that provide a seamless experience. Implementing a progress bar introduces an element of interactivity, engaging users and making the upload process more user-friendly.
Feedback Mechanisms for Successful Uploads
Feedback mechanisms play a crucial role in ensuring successful uploads in PHP forms. Displaying clear and concise messages upon completion of the upload process reassures users that their files have been successfully submitted. Error handling is equally important, as it allows users to rectify any issues promptly. Providing informative feedback, such as file size limitations or accepted file types, assists users in understanding the upload requirements, reducing errors and minimizing confusion.
Tips for Optimizing File Upload Performance
Optimizing file upload performance is a multifaceted process that involves various strategies. To enhance efficiency, developers can implement techniques such as file compression, which reduces upload times and minimizes bandwidth usage. Moreover, optimizing server configurations and utilizing caching mechanisms can significantly improve upload speed. Validating file inputs on the client-side before submission helps in preventing unnecessary uploads, thereby streamlining the process. By incorporating these optimization tips, developers can ensure a swift and seamless file upload experience for users.
Securing File Upload Functionality
In the realm of PHP form file uploads, securing file upload functionality is paramount. The importance of this topic within the scope of this comprehensive guide cannot be overstated. Security measures are crucial to prevent potential vulnerabilities and attacks that can compromise the integrity of the uploaded files and the entire application. By focusing on securing file upload functionality, users can safeguard their systems against malicious intent and ensure the robustness of their PHP forms.
Sanitizing User Input
The process of sanitizing user input plays a pivotal role in fortifying the security of file uploads in PHP forms. Sanitization involves validating and cleansing user-generated data before further processing it within the application. By implementing strict sanitization techniques, such as filtering out unwanted characters, checking input lengths, and verifying file types, developers can mitigate the risks associated with malicious content and injection attacks. By emphasizing the importance of sanitizing user input, developers can enhance the resilience of their PHP forms and protect them from potential vulnerabilities.
Implementing CSRF Protection
Cross-Site Request Forgery (CSRF) protection is a critical aspect of securing file upload functionality in PHP forms. CSRF attacks involve malicious entities exploiting the trust a website has in a user's browser to execute unauthorized actions on behalf of the user. By implementing CSRF protection mechanisms, such as unique tokens for each request and validating the origin of requests, developers can thwart CSRF attacks and prevent unauthorized file uploads. Emphasizing the implementation of CSRF protection underscores the commitment to maintaining a secure and reliable file upload system in PHP forms.
Preventing File Execution Vulnerabilities
Preventing file execution vulnerabilities is essential for maintaining the integrity of PHP form file uploads. File execution vulnerabilities can arise when uploaded files contain malicious code or scripts that can be executed within the application environment. By incorporating stringent file type restrictions, validating file content, and preventing direct access to uploaded files, developers can mitigate the risks associated with file execution vulnerabilities. Addressing the importance of preventing file execution vulnerabilities demonstrates a proactive approach to enhancing the security posture of PHP forms and safeguarding against potential exploitation.
Conclusion
In the realm of mastering PHP form file upload, the Conclusion section encapsulates the significance of ensuring a robust understanding and implementation of the discussed concepts. It serves as the culmination of the entire guide, offering a reflection on the key aspects and guiding principles elucidated throughout the article.
Understanding the Conclusion section is crucial for reinforcing the core learnings acquired in the preceding sections. By emphasizing the practical examples and step-by-step guidance provided, readers can grasp the essence of effective PHP form file uploads and how to navigate potential challenges that may arise during implementation.
A pivotal element of the Conclusion is highlighting the importance of proper validation and error handling in file uploads. Ensuring that files are correctly processed, validating sizes and types, and implementing error messages and exceptions are fundamental to maintaining the integrity and security of the upload functionality.
Moreover, the Conclusion underscores the significance of enhancing user experience in file uploads. Strategies such as implementing progress bars, establishing feedback mechanisms for successful uploads, and optimizing performance are crucial for creating a seamless and user-friendly upload process.
Security considerations also take center stage in the Conclusion, delving into the imperative need for sanitizing user input, implementing CSRF protection, and preventing file execution vulnerabilities. These measures are essential for safeguarding the application against malicious attacks and maintaining data integrity.
In essence, the Conclusion section encapsulates the essence of mastering PHP form file uploads. By synthesizing the information presented throughout the guide, readers are equipped with the knowledge and skills necessary to navigate the intricacies of PHP form file uploads effectively and securely.