Mastering Object Oriented Programming Concepts
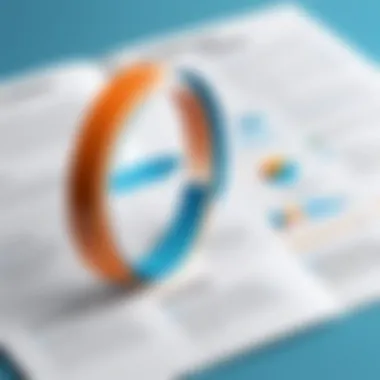
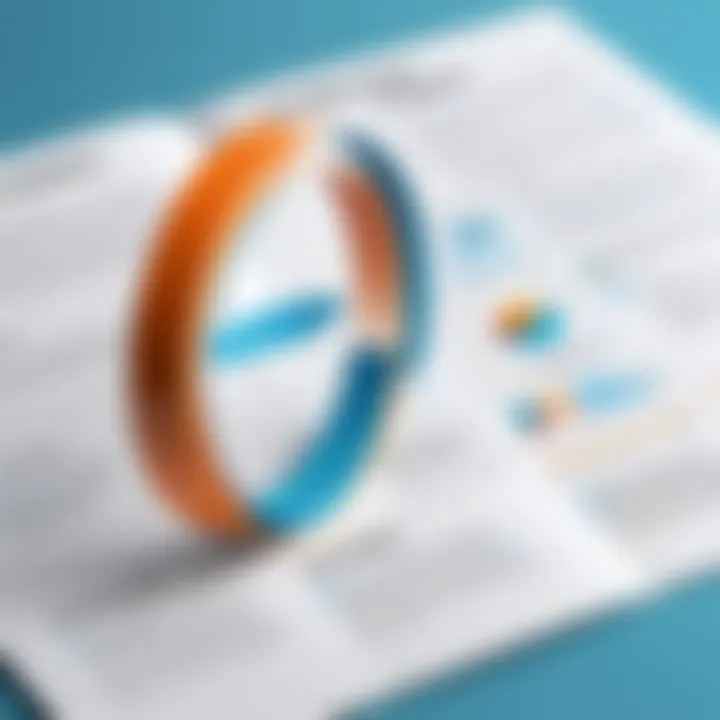
Intro
Understanding programming languages can be like trying to decipher a secret code. It’s essential to grasp the foundational concepts before diving headfirst into any specific area. Here, we're going to explore the ecosystem of programming languages, laying the groundwork for our in-depth discussion on Object Oriented Programming (OOP).
History and Background
Programming languages have a rich history, evolving over decades to accommodate the growing demands of technology. The first high-level programming languages, such as Fortran and COBOL, emerged in the 1950s, making writing code more accessible than the machine-level languages used previously. Over time, as the complexity of software systems grew, so did the need for more structured and organized coding paradigms.
OOP began gaining traction in the 1970s, primarily through the Simula language, which introduced concepts like classes and objects. By the 1980s and 90s, languages like C++ and Java popularized these principles, leading to the widespread adoption of OOP in software development today.
Features and Uses
Why should you care about how programming languages have developed? Well, understanding these features is crucial. Object Oriented Programming emphasizes the use of objects in coding, which can encapsulate data and functionality together. This concept allows developers to create modular, reusable code, making it easier to maintain and scale applications. Common features of OOP include:
- Encapsulation: Bundling data and methods operating on that data within one unit (i.e., an object).
- Abstraction: Hiding complex realities while exposing only the necessary parts.
- Inheritance: Allowing new classes to inherit properties and behavior from existing ones.
- Polymorphism: Enabling objects of different classes to be treated as objects of a common superclass.
These principles are crucial across many fields—web development, app creation, game design, and beyond. Besides, most modern programming languages support OOP to some degree, enhancing their versatility.
Popularity and Scope
With tech giant and startups alike investing heavily in software products, the popularity of programming languages has skyrocketed. According to studies, languages like Python, Java, and JavaScript continuously lead the charge in terms of usage. Their capabilities in handling complex tasks efficiently make them favorite choices among programmers.
As part of this exploration, it's vital to engage actively with the language you choose. Consider the following:
- The community and support available
- The compatibility with other software tools
- Job market demand for that particular language
Prolusion to Object Oriented Programming
Understanding Object Oriented Programming (OOP) is crucial for anyone stepping into the world of software development. OOP isn’t just a coding paradise; it’s a fundamental programming paradigm that structures code in a manner making it more manageable and scalable. By organizing software design within the context of objects that combine data and behavior, OOP not only simplifies complex programming tasks but also promotes code reuse and flexibility.
In today’s fast-paced tech environment, where adapting to new requirements quickly can make or break a project, knowing the principles of OOP is like having a Swiss Army knife. It allows developers to craft solutions that are not only effective but also easier to maintain over time. With this guide, readers will navigate through the core concepts and intricacies of OOP, making it accessible for both beginners and those brushing up on their skills.
Defining Object Oriented Programming
At its core, Object Oriented Programming centers around the concept of objects. An object is a self-contained entity that consists of both data and methods to manipulate that data. This unified approach distinguishes OOP from traditional procedural programming styles, where logic is prioritized over data.
Let’s define the fundamental characteristics of OOP:
- Encapsulation: This principle ensures that the internal representation of an object is hidden from the outside, exposing only what’s necessary through defined interfaces. It’s akin to a well-locked box—what’s inside is none of anyone else's business.
- Inheritance: Through inheritance, a new class can inherit properties and methods from an existing class. This concept facilitates code reusability and establishes a hierarchy among different classes.
- Polymorphism: Polymorphism allows methods to do different things based on the object that it is acting upon. It’s all about flexibility; you write the code once, and then watch it adapt to different situations.
- Abstraction: This principle lets developers focus on complex functionalities while hiding the unnecessary details. It simplifies interaction by allowing users to interact with a simplified model.
With OOP, developers can create programs that mirror real-life interactions between different entities, making their designs more intuitive and aligned with human thinking.
Historical Context and Evolution
A dive into the history of Object Oriented Programming reveals a fascinating journey. The roots of OOP can be traced back to the 1960s with Simula, a pioneer in incorporating objects. Developed in Norway, Simula introduced a revolutionary approach to programming, enabling developers to model real-world problems effectively using objects.
Following Simula, languages like Smalltalk in the 1970s took OOP further by offering a more refined environment, emphasizing messages between objects rather than mere function calls. This period heralded the birth of many OOP principles we embrace today.
The 1980s brought about C++, which grafted OOP concepts onto the powerful C language. This allowed programmers to harness heavy-duty functionalities while enjoying the elegance of OOP. The evolution continued as languages like Java emerged in the mid-1990s, bringing OOP to the mainstream, especially within enterprise applications.
Today, OOP forms the backbone of many modern programming languages, including Python, Ruby, and C#. Its evolution reflects the need for more sophisticated ways to develop software, adapting to the complexities of our ever-changing technological landscape. As we look forward, understanding OOP is not just a technical requirement; it’s become a necessity in the toolbox of any programming enthusiast.
"Object Oriented Programming takes the complexity out of programming, making it easier to develop applications which can grow over time."
By grasping the foundations of OOP, programmers gain insights into effective design and implementation strategies, emphasizing the importance of this paradigm in today’s software development arena.
Core Concepts of OOP
Understanding the core concepts of Object Oriented Programming (OOP) is crucial for anyone venturing into the realm of software development. These principles form the bedrock of many cutting-edge programming languages and frameworks, thus enabling developers to create robust and scalable applications. By familiarizing oneself with these concepts, programmers can better manage complexity in their code, enhance code reusability, and cultivate a clearer, cleaner coding style.
Classes and Objects
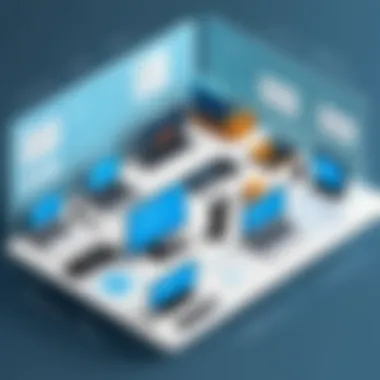
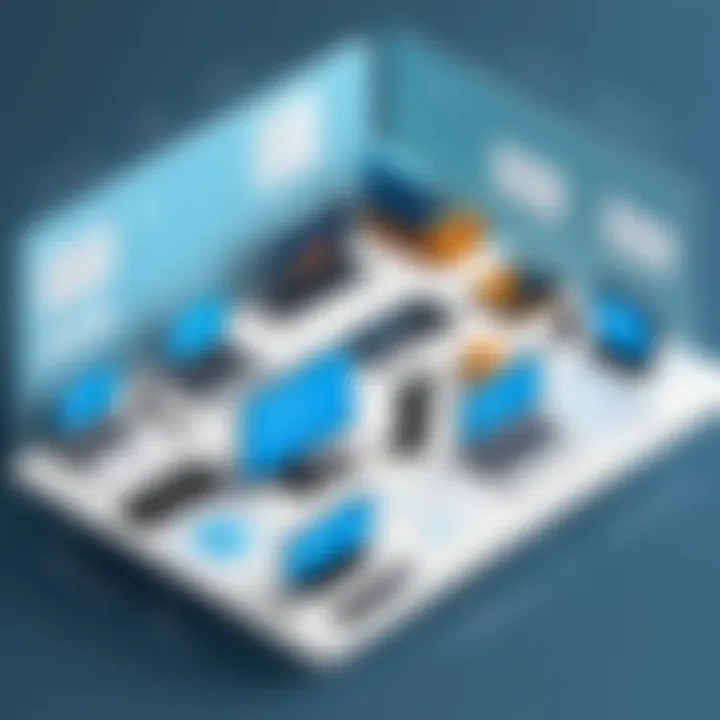
At the very heart of OOP lie classes and objects. A class serves as a blueprint, portraying attributes and behaviors that objects or instances will inherit. For instance, consider a class that holds properties like , , and . Each specific car that originates from this class, like a red sedan or blue hatchback, is an object.
- Benefits of Classes and Objects:
- Reusability: Instead of rewriting code, developers can create new objects from existing classes.
- Organization: Classes help spatially organize code, making it easier to manage and navigate.
This close-knit relationship between classes and objects streamlines many programming tasks, allowing programmers to focus on higher-level design rather than getting bogged down by minutiae.
Encapsulation
Encapsulation is the concept of restricting access to certain components of an object. By doing so, it hides the internal state or logic from the outside world. Think of it as a protective shell. For example, if a class has a method for withdrawing money, this method might check whether the account has sufficient funds before proceeding.
- Key Aspects of Encapsulation:
- Data Protection: Critical data is safeguarded from outside interference.
- Controlled Access: Access to certain methods and attributes can be controlled with public, private, and protected access modifiers.
In effect, encapsulation fosters an environment where the inner workings of an object remain hidden, allowing developers to change those workings without altering external behavior.
Inheritance
Inheritance allows one class to inherit properties and methods from another. This leads to a hierarchy of classes, promoting code efficiency. For instance, if there’s a general class called , classes like and can inherit from it, thereby obtaining its properties like and but also adding their own unique features.
- Merits of Inheritance:
- Code Reusability: Enhances reusability by avoiding duplication of code.
- Extensibility: New functionalities can be added by creating new subclasses.
Inheriting attributes and behavior promotes not only efficiency but also a clear understanding of relationships among various classes, facilitating better maintainability of the code.
Polymorphism
The term polymorphism translates to “many forms.” In OOP, it refers to the way functions or methods can take multiple forms, enabling a single interface for different data types. A classic example is a method that can be used for both and classes, even if each class implements its own version of .
- Advantages of Polymorphism:
- Flexibility: Allows for methods to behave differently based on the object invoking them.
- Maintainability: Makes it easier to update and maintain code as new object types are introduced without affecting existing ones.
Polymorphism truly shines in large applications where it enables more dynamic interactions between objects.
Abstraction
Abstraction is another key pillar of OOP. It involves simplifying complex systems by modeling classes based on essential properties while ignoring unnecessary details. This process can be visualized in user interfaces— users interact with a simple interface without needing to grasp the complexities behind it.
- Key Benefits of Abstraction:
- Simplicity: Helps in focusing on implementation without getting mired in the particulars.
- Modularity: Supports the use of abstract classes and interfaces that can be implemented by multiple subclasses.
This essential feature allows developers to break down complicated systems into manageable parts, enhancing clarity and effectiveness in design communication.
In summary, these core concepts of OOP—classes and objects, encapsulation, inheritance, polymorphism, and abstraction—coalesce to form a framework that not only promotes cleaner and more efficient coding but also reflects real-world relationships. Mastery of these principles profoundly impacts a programmer's ability to craft sophisticated software systems.
Importance of OOP
Object Oriented Programming (OOP) is a cornerstone of modern software development, offering a robust framework that reflects real-world scenarios. Its importance lies in the way it simplifies complex programming tasks through the use of objects, which encapsulate data and behavior. This method not only enhances code reusability but also promotes better organization within applications.
Advantages in Software Development
- Modularity: One of the primary benefits of OOP is that it encourages modularity. By creating distinct classes and objects, developers can focus on specific tasks without getting bogged down by extraneous details. This approach fosters clean code practices and makes it easier to collaborate on larger projects.
- Reusability: Through inheritance, one class can inherit attributes and methods from another, reducing redundancy significantly. This means that once a developer creates a class, they can reuse that class in various applications without having to rewrite code.
- Ease of Maintenance: When you think about it, maintaining a well-structured codebase is similar to keeping a tidy workspace; the more organized it is, the easier it is to find things. OOP's emphasis on encapsulation and abstraction helps make the code more understandable. Bugs can be traced and fixed more effectively, as each class acts as a separate module.
- Scalability and Flexibility: As projects grow, the need to scale up becomes paramount. OOP allows for easy scalability, enabling organisations to adjust and extend their systems as needed without redoing entire codebases. This flexibility is invaluable in today's fast-paced development environments, where changes and new requirements are the norm.
- Improved Collaboration: In a team setting, when developers adhere to the principles of OOP, they can work more harmoniously. Each team member can focus on their respective classes, and as long as the interfaces remain consistent, integration among different parts of the application will be seamless.
OOP in Real-world Applications
Object Oriented Programming isn’t just an academic concept; it’s deployed in various ways across industries:
- Game Development: Games often have complex interactions that can be encapsulated within classes. Each object, like a player, enemy, or item, can hold its own state and behavior, making it easier to manage gameplay dynamics.
- Web Applications: Frameworks like Ruby on Rails and Django utilize OOP principles to structure their applications, allowing results-driven product development. The MVC (Model-View-Controller) architecture divides responsibilities in a way that emphasizes separation of concerns.
- Simulation Software: OOP finds a natural fit in simulation software, where physical entities and their interactions can mimic behaviors in the real world. For instance, each object in a flight simulation could represent specific aircraft dynamics, loading various states into a cohesive unit.
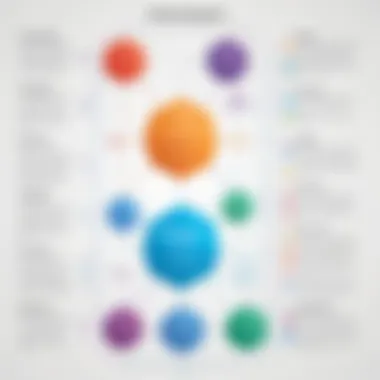
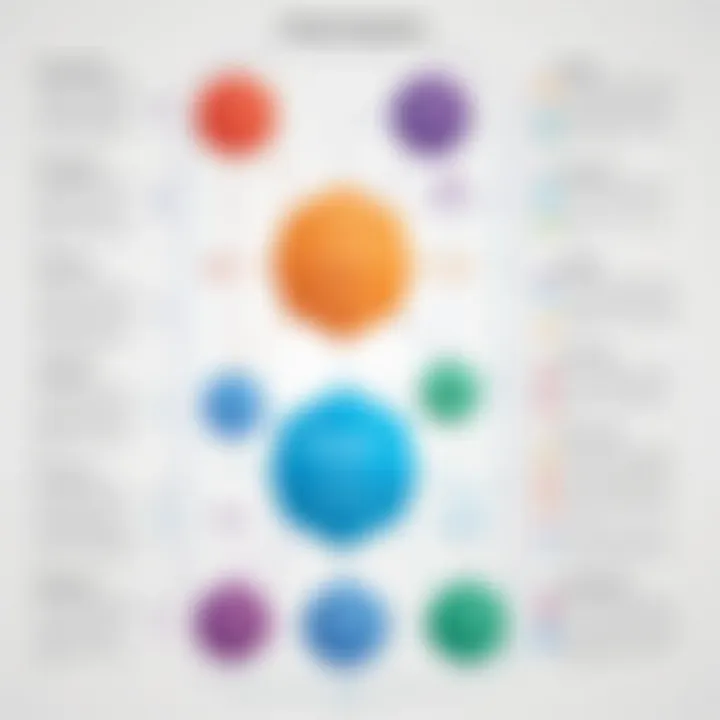
In essence, OOP serves not just to make programming more intuitive but also to bridge the gap between abstract concepts and their tangible applications. As industries continue to evolve, the significance of OOP will undoubtedly resonate through innovative solutions across various domains.
Programming Languages and OOP
Understanding the interplay between programming languages and Object Oriented Programming (OOP) is crucial for anyone stepping into the world of coding. Different programming languages have their unique ways of handling OOP principles, impacting how developers implement these concepts in real-world projects. The right choice of language can lead to clean, maintainable, and scalable code. Conversely, using the wrong language can make OOP implementation cumbersome and less efficient.
Languages like Java, C++, and Python each approach object-oriented principles with their specific syntax, capabilities, and paradigms. This variety isn't just academic; it's practical. For instance, choosing Java for a large enterprise application might provide benefits in terms of performance and stability. In contrast, Python's simplicity might be more suitable for rapid prototyping and experimentation.
Moreover, understanding how OOP fits into different programming languages helps learners to visualize and realize the advantages of OOP, like reusability and modularization. It's like picking the right tool for the job—each language equips developers with distinct features to tackle varying programming challenges.
"Every language has its spirit; understanding that spirit is the key to effective programming."
Java and OOP Principles
Java is often held up as a quintessential Object Oriented Programming language. It paves the path for learners to grasp fundamental OOP concepts. The principles of encapsulation, inheritance, and polymorphism are exceptionally clear in Java, making it easier for budding programmers to understand and apply OOP in real-world applications. In Java, everything revolves around objects, which means that even functions must be encapsulated within classes. This tight encapsulation is pivotal for managing complex software designs.
Key Aspects of Java’s OOP Principles:
- Encapsulation: Java encourages hiding the internal state of an object. By using access modifiers, developers can control visibility and maintain the integrity of their data.
- Inheritance: This principle allows new classes to inherit properties and methods from existing classes, fostering code reusability and organization.
- Polymorphism: Java enables methods to do different things based on the object that it acts upon, which simplifies code management.
++ and OOP Practices
C++ is a language that builds on the foundations of C while integrating OOP principles in a versatile manner. Unlike some strictly object-oriented languages, C++ gives programmers the flexibility to use both procedural and object-oriented programming styles. This mix allows developers to choose the best approach for their specific task, providing them a broad palette to work with.
Important Practices in C++ OOP:
- Multiple Inheritance: Unlike Java, C++ allows a class to inherit from multiple classes, which can be powerful but requires careful management to avoid complexity, known as the "Diamond Problem".
- Constructors and Destructors: C++ employs special member functions that manage the allocation and deallocation of resources, ensuring that objects release their resources appropriately.
- Templates: C++ supports generic programming through templates, making it possible to create functions and classes that can operate with any data type without the need for duplication.
Python’s Approach to OOP
Python's take on Object Oriented Programming is often described as elegant and straightforward. It allows for rapid development while still supporting OOP principles wholeheartedly. Unlike Java or C++, Python adopts a more permissive syntax, enabling programmers to write less code while achieving the same functionality.
Distinct Features of Python’s OOP:
- Duck Typing: Python uses a principle that suggests an object's suitability is determined by the presence of certain methods and properties, rather than the actual type of the object itself.
- Dynamic Typing: Variables in Python can change types in the course of program execution, allowing more flexibility in how objects are created and manipulated.
- Simplicity of Syntax: The language makes it easy to define classes and create objects, emphasizing readability and ease of use, which is perfect for those learning to program.
In summary, whether you choose Java, C++, or Python, understanding how each language implements OOP concepts is vital for advancing your programming skills. Each offers unique strengths that cater to different project needs and preferences, shaping the way developers think about and implement object-oriented solutions.
Common OOP Design Patterns
Design patterns are like the secret sauce that gives your coding the right texture, flavor, and presentation. They’re time-tested solutions to recurring problems in software design, making the development process smoother and more efficient. In the context of Object Oriented Programming, these patterns can lead to more maintainable, scalable, and robust applications—all the essentials for any aspiring programmer.
By understanding the various design patterns, developers can avoid reinventing the wheel. Instead of struggling to develop your solutions from scratch, you can lean on established patterns that have been proven effective through countless applications. More importantly, they enhance communication among programmers. When you say "Observer Pattern," everyone’s on the same page. Let’s dissect the main categories of these design patterns: Creational, Structural, and Behavioral.
Creational Patterns
Creational patterns deal with object creation mechanisms, trying to create objects in a manner suitable to the situation. Think of them as the blueprint for producing houses. You wouldn’t use the same design for a small townhouse and a sprawling mansion. Similarly, creational patterns accommodate different objects based on the context.
Key Patterns in this Category:
- Singleton: Ensures that a class has only one instance and provides a global point of access to it. Imagine a scenario where you only want one print manager for a document; a Singleton ensures that duplication doesn’t mess up your resources.
- Factory Method: This pattern creates objects without specifying the exact class of object that will be created. Like ordering a drink at a café without knowing the drinks on the menu—just ask the barista, and they'll whip something up based on your preference.
- Builder: It separates the construction of a complex object from its representation so the same construction process can create different representations. Consider it like assembling a model aircraft. Different builders will give you planes that look different but are created in a similar way.
Structural Patterns
Structural patterns focus on how classes and objects can be combined to form larger structures. They’re all about composition and relationships. These patterns help ensure that if one part of a system changes, the entire system doesn’t need to do a complete overhaul. It's a bit like a well-designed Lego set; you can swap out pieces without tearing the whole thing down.
Key Patterns in this Category:
- Adapter: This pattern allows incompatible interfaces to work together. It's like using a European plug in an American socket: you just need the right adapter to connect.
- Decorator: Allows behavior to be added to individual objects, either statically or dynamically, without affecting the behavior of other objects from the same class. This is akin to adding layers of painting to a canvas:
- Composite: This pattern enables you to compose objects into tree structures. You can treat individual and composite objects uniformly, like a team of employees reporting both as a group and as individuals.
Behavioral Patterns
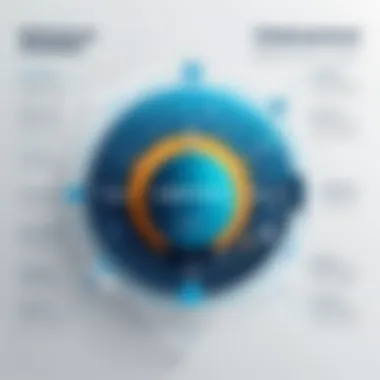
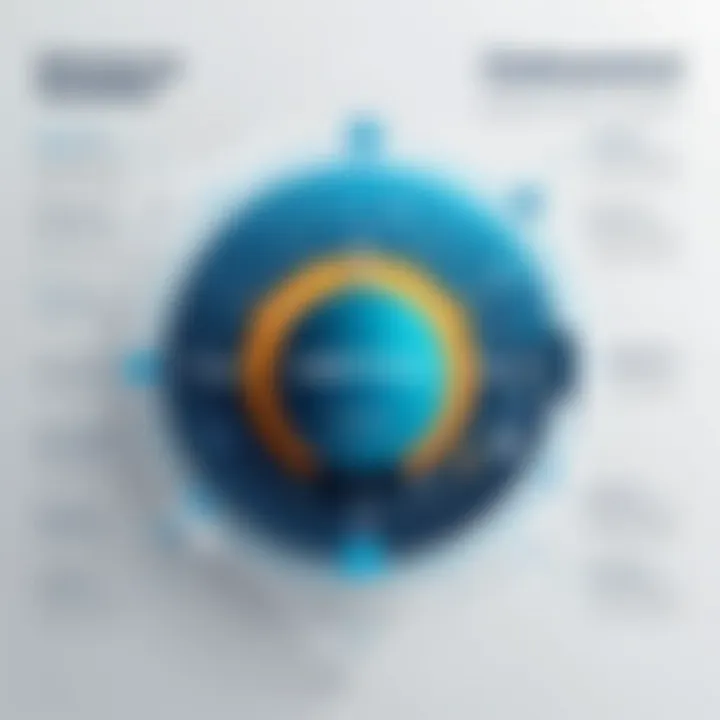
Behavioral patterns are concerned with algorithms and the assignment of responsibilities between objects. Rather than focusing on the structure of objects, they deal with how objects interact with each other. It’s the social dynamic of your code; how your classes play nice together goes a long way in determining the efficiency of your application.
Key Patterns in this Category:
- Observer: This pattern establishes a one-to-many dependency between objects so that when one object changes state, all dependents are notified and updated automatically. Great for scenarios involving a user interface where multiple components need to respond to events.
- Strategy: This pattern enables a family of algorithms to be defined and encapsulated, making them interchangeable. It’s like choosing different driving routes based on the amount of traffic.
- Command: It turns a request into a stand-alone object that contains all the information about the request. Handy when you want to implement undo operations, essentially keeping a history of commands.
Understanding these patterns is crucial for any serious programmer. By applying them effectively, you avoid common pitfalls and set yourself up for long-term success.
When integrating these design patterns into your coding projects, remember they serve as guides rather than dogmas. The adaptability and context of each pattern make them powerful tools in any developer’s toolkit.
Best Practices in OOP
Understanding best practices in Object Oriented Programming is crucial for crafting applications that stand the test of time. Employing these practices not only enhances code quality but also boosts maintainability and performance. Each principle serves as a guiding star for developers, helping them navigate complexities in code structure and design. With a thoughtful approach, programmers can avoid common pitfalls and create software that is both robust and adaptable.
Writing Clean Code
Clean code is the bedrock upon which successful software is built. It contributes to readability, making it easier for new developers and future maintainers to jump in without feeling lost. When writing clean code, consider the following:
- Descriptive Naming: Names matter – use meaningful variable and function names. For instance, instead of naming a variable , try , which instantly reveals more information.
- Consistent Formatting: Adhering to a consistent indentation style aids in comprehension. Utilizing tools like Prettier can standardize this across a project.
- Commenting and Documentation: While the ultimate aim is to make the code self-explanatory, leave comments where the logic may not be evident at a glance. Use comments wisely: too many can clutter, but too few can lead to confusion.
When implemented effectively, clean code drastically reduces debugging time and enhances collaboration among developers. In fact, a study revealed that well-structured code can lead to a 40% increase in development speed in large teams.
"Simplicity is the soul of efficiency." – Austin Freeman
Maintaining Code Flexibility
Flexibility in code design allows it to adapt to changing requirements without needing a complete overhaul. This adaptability is essential, particularly in agile development environments. Here are some important strategies for maintaining code flexibility:
- Use Interfaces: Defining interfaces allows for different implementations. For example, if you have various payment methods like credit card, PayPal, and cryptocurrency, an interface can unify them, making it easy to add or modify payment options in the future.
- Follow SOLID Principles: The SOLID principles guide clean architecture and help to mitigate issues in extending functionality. These principles encourage developers to create systems that are easier to maintain and less prone to bugs.
- Keep Functions Short and Focused: Functions should do one thing well. This small scope makes it much easier to test, debug, and update functionality.
Keeping code flexible may seem challenging over time, but it pays off when your system needs to evolve. Changes become seamless, and response to user feedback accelerates.
In summary, adopting best practices in OOP is not just a flavor of the month. It builds the backbone of a reliable and maintainable codebase, setting up developers for success in the long run. Exceptional practices like writing clean code and maintaining flexibility not only enhance productivity but also increase the overall quality of software applications.
Challenges in OOP Implementation
Navigating the waters of Object Oriented Programming (OOP) can sometimes feel like sailing against a headwind. While OOP brings a wealth of benefits—like improved code organization and reusability—it’s not without its fair share of hurdles. Understanding these challenges is vital for both newcomers and those familiar with programming languages. By identifying pitfalls and performance issues before they arise, learners can maintain clarity in their code and sidestep unnecessary headaches.
Common Pitfalls
When embarking on an OOP journey, programmers often stumble upon certain common traps that lead to confusion and suboptimal code. Here are some of these frequent pitfalls:
- Overcomplicating Designs: It’s tempting to create elaborate class hierarchies and intricate object interactions. However, a convoluted design can be harder to understand and maintain. Keeping things simple and intuitive is key.
- Neglecting Encapsulation: This is a cornerstone of OOP, meant to hide object details. When developers choose not to protect data or allow uncontrolled access, it can lead to unintended consequences, making it difficult to track how data is manipulated.
- Misusing Inheritance: While inheritance can simplify code reuse, it can also create tight coupling between classes. Overextending class hierarchies can lead to complications when refactoring code in the future.
- Ignoring the Single Responsibility Principle: Each class should have a single responsibility. If you find a class doing too much, it might be a signal to break it down. This simplifies debugging and enhances code clarity.
Overcoming these pitfalls requires practice and a thoughtful approach to design. Here’s a focus on some examples to clarify these points.
"Simplicity is the ultimate sophistication.”
Performance Considerations
Performance is another area where OOP can encounter challenges. The principles and benefits of OOP, while enriching, can sometimes come at a cost to the system’s efficiency. Key considerations to keep in mind include:
- Memory Overhead: Objects typically consume more memory compared to data structures due to features such as encapsulation and methods. This is essential when working with large datasets or systems where resources are limited.
- Indirection Cost: The object-oriented paradigm often introduces layers of indirection, especially with polymorphism. Utilizing interfaces and abstract classes can slow down performance due to additional lookups and method dispatching.
- Garbage Collection: Many OOP languages rely on garbage collection to manage memory, which helps but can cause unpredictable pauses during execution. For performance-sensitive applications, this can be a crucial factor to consider.
- Slower Initial Learning Curve: Because of the need to understand multiple concepts—like inheritance, polymorphism, and encapsulation—beginners might face a steeper learning curve. Simplifying projects or concentrating on practical applications can help to mitigate this.
Closure: The Future of OOP
As we stand at the crossroads of technology and innovation, the future of Object Oriented Programming (OOP) beckons with numerous possibilities. This conclusion aims to reflect on the significance of OOP in software development and the avenues that lie ahead for programmers and developers alike.
The essence of OOP revolves around its ability to model real-world entities and relationships through classes and objects. This characteristic plays a pivotal role in making code more intuitive. The structured approach fosters better organization of complex codebases, which is increasingly essential as projects evolve and scale in size.
Evolving Trends in Software Development
The world of software is always on the move, and the trends in OOP are no different. Here are some notable directions to watch for in the coming years:
- Increased Use of Microservices: As applications grow larger and more intricate, there's a noticeable shift towards using microservices architecture. OOP principles align well with this trend, enabling developers to create modular components that can interact seamlessly.
- Hybrid Programming Paradigms: Imitating nature’s design, future programming languages may adopt hybrid approaches that combine OOP with functional programming. This mix could offer the best of both worlds, enhancing code reusability along with immutability and statelessness.
- Focus on Maintainability: The importance of maintainability cannot be overstated. As software is not static but a living entity, practices focusing on clean code and documentation will gain prominence. OOP’s encapsulation and abstraction features make it a suitable fit for these requirements.
- AI and OOP: The rise of artificial intelligence will also impact how we use OOP. Techniques like machine learning can leverage OOP principles to create more intuitive models. This would aid in predictive programming where systems adapt and learn from interactions over time.
- Remote Collaboration Tools: With remote working becoming more the norm, tools that support collaboration in OOP environments will surge. Expect to see advanced IDEs and version control systems that facilitate global teamwork on OOP projects.
"The future belongs to those who prepare for it today." - Malcolm X
In summary, OOP stands as a foundational pillar in software development. Its principles continue to influence modern programming and will shape the technologies and methodologies of the future. As we embrace these evolving trends, it is vital for students and developers to remain adaptable and continuously hone their skills in this enduring paradigm. The road ahead is ripe with promise, and those who navigate it with an understanding of OOP will likely find themselves well-equipped to tackle whatever challenges arise.