Mastering Node.js: In-Depth Guide for Developers
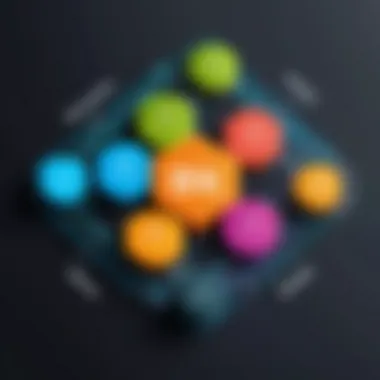
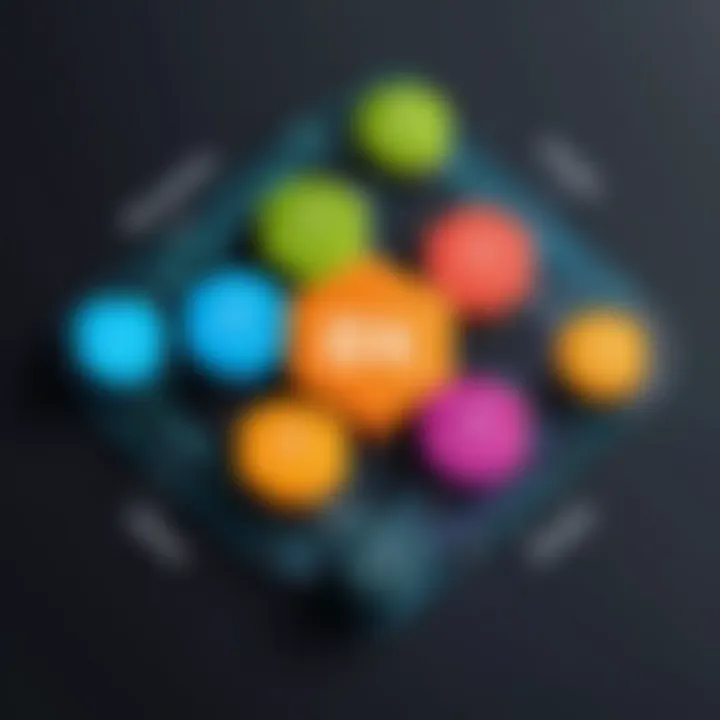
Intro
Node.js is like the silent powerhouse behind many web applications seen today. It has fundamentally changed the landscape of how JavaScript is perceived, transitioning it from a simple client-side script to a robust server-side runtime. As developers look for efficient, scalable solutions for their projects, Node.js offers the tools and framework to deliver that. This section kicks off our journey by exploring the programming language itself, its history, features, and widespread applications.
History and Background
Node.js was crafted in 2009 by Ryan Dahl. Inspired by the need for asynchronous, event-driven programming, it aimed to help developers handle multiple connections concurrently. Built on top of Google's V8 JavaScript engine, Node.js has since expanded to a rich ecosystem of libraries and frameworks. The initial driving force was to improve performance in web applications, especially those dealing with real-time data. Today, it’s a staple for many companies and developers, powering sites like Netflix and LinkedIn.
Features and Uses
What makes Node.js stand out in a crowded field? Here are some of its key features:
- Non-blocking I/O: Node.js uses an event-driven model that makes it lightweight and efficient. It’s capable of handling numerous requests simultaneously, ideal for I/O-heavy operations.
- Single Language: Developers can use JavaScript for both client and server-side, reducing the need for context-switching and streamlining the code flow.
- Rich Ecosystem: The Node Package Manager (NPM) houses thousands of libraries, enabling faster development times and richer applications.
Node.js is utilized for various applications such as web servers, real-time applications (like chats), and APIs. It’s not just limited to websites, either; with its capabilities, it can serve as a platform for microservices and IoT systems.
Popularity and Scope
In terms of popularity, Node.js has gained significant traction over the years. According to various surveys, many developers regard it as a top choice for back-end development. Its shift from a niche framework to a go-to technology shows its reliability and community support. The scope is vast, spanning over e-commerce, social media, and even cloud services. More importantly, it serves both established enterprises and budding startups.
In summary, Node.js is more than just another technology—it’s an evolving framework that plays a crucial role in modern web development. Understanding its background, features, and the areas where it shines is essential for leveraging its potential.
Intro to Node.js
Node.js has rapidly become a hot topic in the world of web development, attracting both novice programmers and seasoned developers alike. At its core, Node.js represents more than just a runtime environment; it's a gateway to building high-performance applications that can handle numerous connections simultaneously. As developers increasingly move towards scalable network applications, understanding Node.js is not just beneficial—it's essential.
The significance of this section lies in setting the stage for understanding everything that follows in this guide. This article outlines key concepts, technological benefits, and practical applications of Node.js, ensuring that the reader grasps why this environment has garnered such a following in the development community.
What is Node.js?
Node.js is a JavaScript runtime built on Chrome's V8 engine, enabling developers to utilize JavaScript on the server side. This allows for a more unified development approach, where both the client and the server can communicate using the same language. Node.js operates on a non-blocking, event-driven architecture, which makes it particularly suitable for building scalable network applications.
As developers, the ability to write client-side and server-side code in the same language enhances collaboration and simplifies the development process. Node.js leverages JavaScript's asynchronous capabilities to handle multiple connections with ease, which is crucial for real-time applications like chats or online gaming interfaces.
This powerful combination of JavaScript and server-side capabilities opens numerous doors for innovation and efficiency, making Node.js a go-to choice for many in the industry.
History and Evolution
The story of Node.js traces back to 2009 when Ryan Dahl introduced it as a way to create server-side applications using JavaScript. Dahl saw the immense potential for JavaScript beyond the browser, especially given its non-blocking I/O features combined with the enduring popularity of JavaScript itself.
Since its inception, Node.js has undergone considerable evolution. The community has played a vital role in its development, contributing a multitude of modules and packages. This collaborative effort fueled the rise of the Node Package Manager (npm), which hosts an astonishing number of reusable code modules.
Over the years, Node.js has matured significantly, with numerous updates refining its performance and adding features. The introduction of ECMAScript 6 brought syntactic sugar that made writing JavaScript even more enjoyable. The evolution of Node.js reflects the changing trends in software development, adjusting to the diverse needs of developers around the globe.
Why Choose Node.js?
There are several compelling reasons to opt for Node.js in your development projects:
- Scalability: Node.js employs a single-threaded model with event looping, which facilitates handling numerous concurrent connections. This is ideal for real-time applications that require constant interaction.
- Performance: Leveraging the V8 engine allows Node.js to execute JavaScript at remarkable speeds, making it a performance-oriented choice for developers.
- NPM Ecosystem: The npm registry provides access to thousands of libraries and tools, further extending your application's capabilities without the need to reinvent the wheel.
- Unified Language: Working with JavaScript both on the front and back end means developers can manage their workflows more effectively, removing the need for context switching between different programming languages.
In summary, Node.js offers a robust environment for developers looking to create efficient, scalable, and maintainable applications. This introductory section lays the groundwork for a deeper understanding of Node.js, which we will explore further in upcoming sections.
Understanding the Architecture of Node.js
The architecture of Node.js serves as the backbone of its efficiency and capability as a runtime environment. This topic is fundamental because it delves into how Node.js operates under the hood, revealing the magic that transforms JavaScript into a robust solution for server-side applications. Understanding these components is essential for developers as it affects application design, performance, and overall user experience.
Three pivotal elements will be explored in depth: the single-threaded event loop, the non-blocking I/O model, and the Node Package Manager (npm). Each of these aspects contributes significantly to what makes Node.js a popular choice among developers, especially those looking to harness the power of asynchronous programming and real-time client-server interaction.
Single-Threaded Event Loop
The essence of Node.js's non-blocking operation is rooted in its single-threaded event loop architecture. At first glance, one might think that having a single thread could limit application performance, given the multi-threaded capabilities of many other frameworks. However, the opposite is true for Node.js.
With a single thread, Node.js can still handle multiple operations concurrently thanks to the event loop. When a task is initiated, it gets added to an event queue. Instead of waiting for tasks to complete before moving on, Node.js allows other operations to proceed. This is significant because it contrasts starkly with traditional threading models – it allows high scalability while being resource-efficient. Here’s a simple breakdown of how it works:
- Event Trigger: An event occurs in the application, like a notification of incoming data.
- Queue: The corresponding callback function is placed in a queue, waiting to be executed.
- Execution: The event loop picks the callback from the queue and executes it once the call stack is empty.
This model is particularly beneficial for I/O-bound applications, enabling them to handle numerous simultaneous requests without incurring significant overhead, all thanks to the efficiency of the event loop.
Non-Blocking /O Model
Closely tied to the event loop is the non-blocking I/O model, which frees Node.js from the bottlenecks usual in synchronous operations, where one task must complete before moving on to the next. Non-blocking calls allow operations, such as file system queries and network requests, to happen without halting the flow of execution.
When a non-blocking operation is triggered, Node.js will initiate it and promptly return control to other tasks without waiting for the operation to finish. This is a game-changer for performance and responsiveness in web applications. Common examples include:
- Database Queries: Initiate a database request, process user inputs or other tasks, then handle the results when they’re available.
- File System Access: Read or write to the file system without causing a hold-up in the main execution thread.
The result is a streamlined, efficient application capable of managing high loads, especially in real-time scenarios where speed is crucial.
Node Package Manager (npm)
No discussion of Node.js architecture would be complete without mentioning npm, the Node Package Manager. A tool that acts like a treasure trove for developers, npm allows seamless management of packages and dependencies. When your app begins to grow, so does the need for various libraries and frameworks. This is where npm shines, providing a centralized repository for easy package installation and updates.
Furthermore, npm promotes a modular approach to development, letting developers break complex applications into manageable components. This can significantly improve the maintainability of your code. To summarize its key features:
- Installation and Updates: Quickly install or update libraries directly from the terminal.
- Dependency Management: Automatically manage dependency versions, avoiding conflicts and compatibility issues.
- Community Support: Access a vast ecosystem of libraries and frameworks contributed by the community, from utilities to complete frameworks.
"With npm, working with Node.js isn’t just about efficiency, it’s about creativity and collaboration within the developer community."
The architecture of Node.js is crafted beautifully to support efficient, high-performance applications. With a solid grasp of the single-threaded event loop, non-blocking operations, and npm, developers can harness Node.js in ways that were once thought only possible with more bulky frameworks. As we proceed, we will explore the core features that further accentuate these architectural advantages.
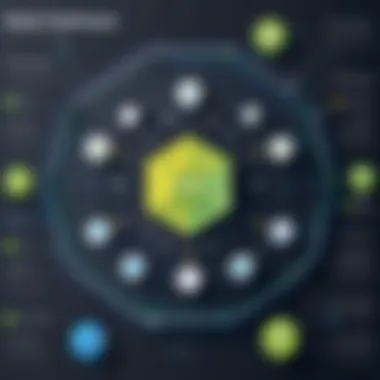
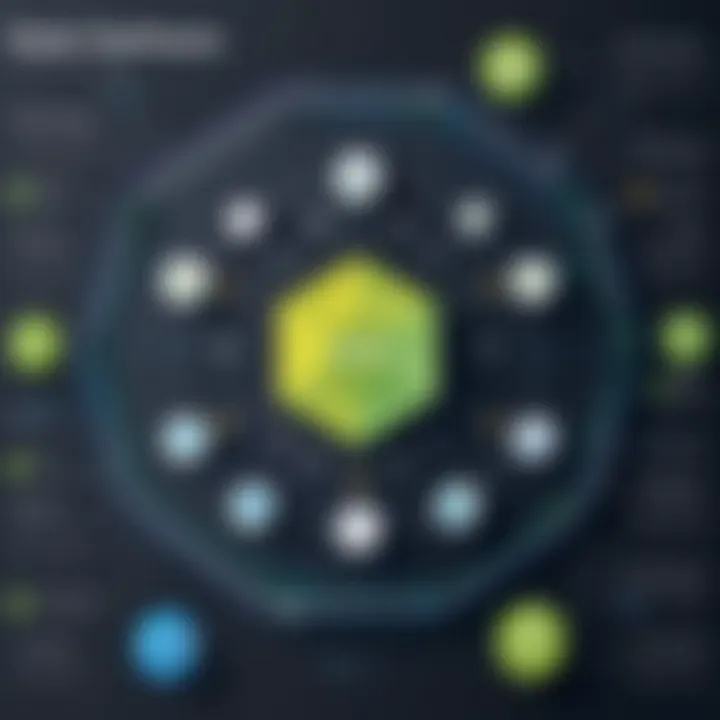
Core Features of Node.js
Node.js stands out in the realm of web development due to its unique and powerful core features. Understanding these features is crucial for anyone looking to harness Node.js effectively. In this section, we will delve into three pivotal characteristics: asynchronous programming, real-time applications, and scalability. Each of these elements plays a vital role in how Node.js operates and what makes it appealing to developers.
Asynchronous Programming
One of the crown jewels of Node.js is its asynchronous programming model. In simpler terms, this means that operations can run in the background without blocking the main execution thread. Developers can fire off tasks and keep the application responsive, which is essential in today’s fast-paced web environment. This model opens doors to enhance performance substantially.
Unlike languages that rely on a synchronous method where one task must wait for another to finish, Node.js allows multiple tasks to be processed concurrently.
"Efficiency is doing things right; effectiveness is doing the right things." – Peter Drucker
As asynchronous programming relies heavily on callbacks and promises, it is important to adeptly manage the flow of your application. The async/await syntax introduced in ES2017 has made this easier, bringing more clarity and structure to async code. For example:
In this example, while your application waits for the data retrieval, it's free to handle other tasks, thereby keeping the user experience smooth.
Real-Time Applications
Another standout feature of Node.js is its capability to build real-time applications effectively. This is particularly relevant in scenarios like chat applications, live notifications, and online gaming. The event-driven architecture of Node.js ensures that it can handle multiple client requests simultaneously without any lag.
This is especially crucial given that users expect instantaneous interaction with features. Platforms like Socket.IO leverage Node.js to establish bi-directional communication channels between clients and servers. By maintaining a continuous connection, data can flow freely, allowing for updates in real-time without the need to refresh the web page.
Such capabilities are not limited to specific use cases; they extend to various industries—from e-commerce platforms updating inventory levels live to collaborative tools enabling team members to work together seamlessly.
Scalability
When it comes to scalability, Node.js shines brightly. The framework allows developers to create applications that can grow alongside demand without a hefty rebuild. This is due to its non-blocking architecture, which efficiently manages concurrent connections.
There are two types of scalability to consider:
- Vertical Scaling: Adding more resources (CPU, RAM) to your existing server.
- Horizontal Scaling: Adding more machines to handle the load.
Node.js excels particularly in horizontal scaling, making it adept for distributed computing environments like cloud services. By creating microservices architecture, developers can spin off individual services within an application, distributed across multiple servers, thereby enhancing performance and manageability.
In addition, tools like Docker can be integrated with Node.js, making deployment and scaling even simpler by packaging applications and their dependencies into containers.
Overall, the core features of Node.js—its asynchronous programming model, ability to build real-time applications, and robust scalability—provide a solid foundation for modern web applications. Take time to explore these aspects, as they are essential for crafting high-performing, user-friendly applications.
Setting Up a Node.js Environment
Setting up a Node.js environment is a crucial step for any developer looking to dive into server-side programming. It provides the foundational framework needed to run JavaScript code on your server instead of the browser. Without this, the potential to harness the power of Node.js remains untapped. This section will walk you through the essentials of installation, initial app creation, and debugging methods—all key elements for successful Node.js development.
Installation Process
Getting your hands dirty begins with the installation process. Thankfully, Node.js installation is straightforward. You'll typically start by visiting the official Node.js website, where you'll find downloadable binaries tailored for your operating system. Choosing between the LTS (Long-Term Support) version or the Current version can be a bit muddling. The LTS version is often recommended, especially if stability is your aim, while the Current version introduces the latest features, albeit at the risk of potential instability.
- Download the Installer: Locate the installer suited for your system—Windows, macOS, or Linux.
- Run the Installer: Follow the straightforward prompts. On Windows, make sure to check the box that adds Node.js to your PATH; failure to do so can complicate your setup later on.
- Verify Installation: After installation, open your command line interface and run . If it returns your Node.js version, you've successfully set the stage for your coding endeavors.
"Installation is half the battle; what you build next defines your journey."
Creating Your First Node.js App
Once your Node.js environment is installed, it’s time to create your first application. This initial app can be as simple as a "Hello World" program, a rite of passage in the programming world. Use your favorite text editor or integrated development environment (IDE) and follow these steps:
- Create a New Folder: Designate a directory for your project, which could be something like .
- Initialize the Project: Open your command line inside this directory and run . This command generates a file that documents your project and its dependencies.
- Write Your Code: Create an file in this folder and input the following code:
- Run the App: Execute your code by typing in the command line. Head over to your browser and type . You should see "Hello World" displayed, marking a significant milestone in your journey with Node.js.
Debugging Node.js Applications
Debugging is as essential to development as coding itself. When you're deep in the trenches of your application, knowing how to troubleshoot effectively can save you time and frustration. Node.js provides some built-in debugging tools, and here's how you can harness their power.
- Using the Built-in Debugger: Start your application using the debug flag with . This opens the Node.js debugging tool where you can set breakpoints and inspect variables.
- Console Logs: While it may seem basic, console logging using is invaluable for understanding what’s happening in your code. You can see the flow of execution in real time.
- Using Development Tools: Consider using tools like Visual Studio Code, which has built-in debugging support for Node.js, simplifying your debugging process significantly.
- Error Handling: Always implement error handling in your code using blocks to gracefully manage unexpected issues that might arise during runtime.
By mastering these debugging techniques, you'll be able to nip problems in the bud and keep your application running smoothly.
In summary, setting up your Node.js environment involves more than just installation. It requires an understanding of creating functional applications and being adept at debugging. As you delve deeper into the Node.js ecosystem, these skills will form the bedrock of your success.
Node.js and APIs
Node.js has fundamentally changed how developers approach web applications, especially when it comes to building robust and scalable APIs. In a world where applications require seamless communication between the client and server, Node.js stands out due to its event-driven, non-blocking architecture. This makes it an ideal choice for creating APIs that handle multiple requests efficiently without breaking a sweat.
Building RESTful APIs
When we talk about APIs, RESTful APIs are the gold standard that many developers aspire to build. REST (Representational State Transfer) relies on a set of principles for creating web services. It uses standard HTTP methods like GET, POST, PUT, and DELETE, which makes it familiar for any web developer.
Node.js, combined with Express.js, simplifies the process of designing these APIs. Here’s how:
- Simplicity: Creating routes in Node.js is straightforward. With Express.js, you can set up relevant endpoints quickly, where each route corresponds to an API function.
- JSON Support: Node.js natively handles JSON, which is the most common format for data exchange in REST APIs. This allows easy reading and sending of data.
- Middleware: Express’ middleware can manage requests efficiently, enabling functionalities such as logging, authentication, or error handling before reaching your final route handler.
A simple example of constructing a RESTful endpoint might look like this:
This code creates a basic API that returns a list of users at the endpoint . It shows just how easily you can begin building your RESTful service with Node.js.
Using Express.js Framework
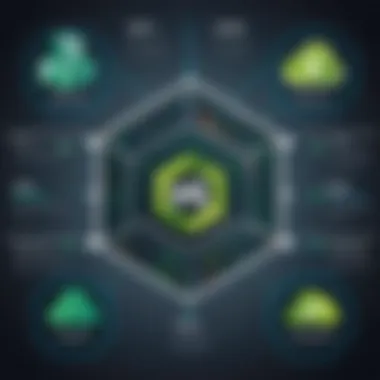
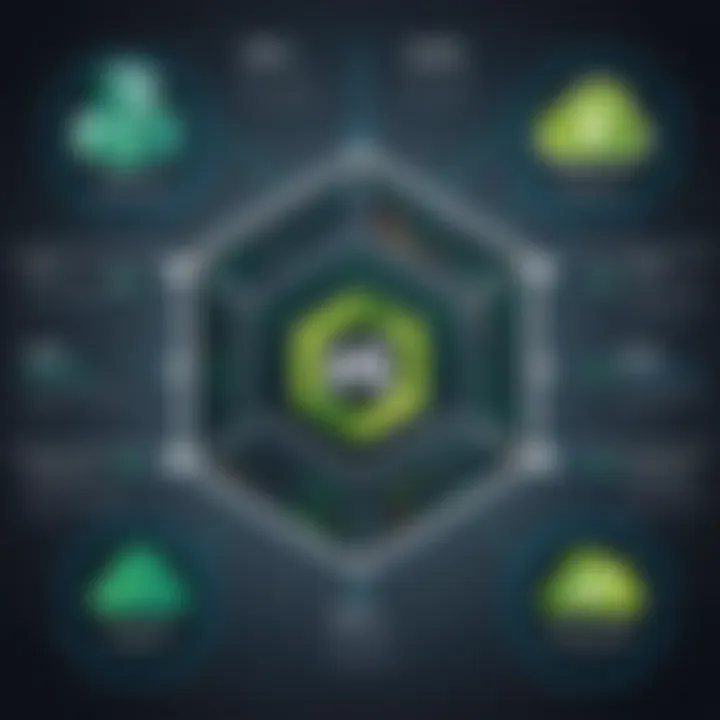
Express.js is essential for developing applications in Node.js. It provides a minimalist approach, allowing you to focus on building your application without worrying about every little detail of server setup. Here are some key benefits of using Express.js:
- Robust Routing: Express allows developers to define multiple routes efficiently, which is crucial for a well-structured API.
- Simplified Middleware: The framework tracks the request and response cycle, so you can attach middlewares at any step, allowing for custom handlers as per the request flow.
- Community Support: With a vast ecosystem of plugins and middleware, Express.js thrives in developer communities, providing solutions for common tasks. You can find a plethora of resources on sites like Reddit or Wikipedia.
Handling API Authentication
As applications grow more complex, API security becomes a top priority. Authentication ensures that users are who they say they are when accessing your APIs. In Node.js, you can implement various methods of authentication, like:
- Token-Based Authentication: JSON Web Tokens (JWT) are commonly used to authenticate users. After the user logs in, the server generates a token that the client must send with each request.
- OAuth: If you’re interfacing with services like Google or Facebook, utilizing OAuth allows users to log in without exposing their passwords, making your API interaction smoother and more secure.
To illustrate, if you were to validate a JWT in an Express.js route, you might implement middleware like this:
This middleware checks if a token is present and valid before allowing access to protected routes. It's not just about functionality; it’s a critical part of ensuring users' data security and integrity within your API.
The use of Node.js for building APIs is not merely a technical preference; it’s a strategic decision that aligns with efficient and scalable application design.
Database Integration with Node.js
In the realm of web development, effective database integration is paramount. Node.js is not just about handling server requests; it’s about making sense of data that flows through applications. Understanding how Node.js interacts with various databases allows developers to choose the right tools that make their applications robust and performance-oriented.
Using MongoDB
MongoDB stands out as a leading NoSQL database that pairs beautifully with Node.js. Its ability to store data in a flexible, JSON-like format resonates well with the way JavaScript operates. This synergy opens the door for streamlined data manipulation within applications. Developers appreciate MongoDB's scalability and its capacity to handle massive datasets, which is essential for modern web applications.
One common pattern is using Mongoose, an ODM library that simplifies interactions with MongoDB. It allows for modeling data and defining schemas in a clean way. For instance:
This short code snippet highlights how easy it is to set up a user model, ensuring that data is structured and validated appropriately.
Using SQL Databases
Though NoSQL databases have gained traction, SQL databases like PostgreSQL and MySQL remain popular choices. They offer strict schema definitions, which can be advantageous when working with structured data. In scenarios where relationships among data points are complex, using SQL databases can provide clarity and reliability.
Many developers opt for libraries like Sequelize or Knex.js to facilitate SQL queries when working with these types of databases. An example using Sequelize, could look like this:
This snippet illustrates how a user model is defined, embracing both the clarity of SQL and the power of Node.js.
ORM and ODM Explained
The concepts of ORM (Object-Relational Mapping) and ODM (Object-Document Mapping) are crucial in helping developers interact with databases effectively.
ORM arises in scenarios where developers use relational databases to convert data between incompatible types in object-oriented programming languages. This allows for easy mappings and reduces the need to write raw SQL queries, speeding up development.
ODM, on the other hand, is tailored for document-based databases like MongoDB. An ODM acts as an intermediary that facilitates the use of JavaScript objects when manipulating database records, ensuring that developers can continue leveraging JavaScript’s syntax and behaviors in their data operations.
Using ORM and ODM frameworks abstracts much of the complexity involved in data interaction, empowering developers to focus on the broader application logic while ensuring data integrity.
In summary, whether you lean toward MongoDB's flexibility or the structured pathways of SQL, the integration of databases with Node.js enhances the capabilities of web applications. By selecting appropriate tools, developers can create software that's scalable, maintainable, and ready to meet real-world demands.
Advanced Node.js Concepts
In today’s web development landscape, grasping advanced concepts in Node.js is crucial for developers aiming to build scalable and efficient applications. This section outlines the significance of these concepts, their applications, and the benefits that come along with mastering them in Node.js development.
Microservices Architecture
Microservices architecture is a modern approach that breaks down applications into smaller, manageable services. Each service handles a specific function, communicates through APIs, and can be deployed independently. In Node.js, this architecture aligns perfectly with its lightweight and event-driven nature. Here are a few key advantages of using microservices with Node.js:
- Scalability: Each microservice can be scaled independently based on demand without affecting others.
- Flexibility: Developers can use different programming languages or frameworks for various services.
- Resilience: Failure in one service does not necessarily compromise the entire application, allowing for improved fault tolerance.
Microservices enable more agile development cycles and can significantly reduce the time it takes to bring new features to market.
To implement microservices, Node.js frameworks such as Express or NestJS are popular choices because they facilitate API creation and routing. When building microservices, it's crucial to implement robust communication between services, often using HTTP, gRPC, or message brokers like RabbitMQ.
Testing in Node.js
Testing is an indispensable part of the software development lifecycle. With Node.js, developers can implement various testing strategies, from writing unit tests to performing integration tests. Here’s a breakdown of common practices in testing Node.js applications:
- Unit Testing: This focuses on testing individual components of your code, which can be efficiently done using testing frameworks like Mocha or Jest.
- Integration Testing: It tests how different modules work together, ensuring that the overall application functions as expected.
- e2e Testing: End-to-end testing verifies the entire application flow from start to finish. Tools like Cypress are well-suited for this purpose.
A strong testing strategy helps catch bugs early and ensures that your application is robust. Automated tests save time and provide confidence in code changes, especially when working within larger teams.
Performance Optimization Techniques
Performance is a critical factor in any application’s success. Node.js, being asynchronous and event-driven, inherently supports high-performance applications. However, developers must implement additional optimization techniques to boost efficiency further:
- Use Clustering: Node.js runs in a single-thread, but with clustering, multiple instances of the application can be run on separate threads. This leverages multi-core processors.
- Caching: Utilize caching mechanisms like Redis to store frequently accessed data, reducing the load on databases.
- Compression: Implement tools like Gzip to compress responses and reduce data transfer, leading to faster load times.
- Database Optimization: Indexing and careful query management can significantly reduce database response times.
By focusing on these optimization techniques, developers not only enhance application responsiveness but also improve user satisfaction.
In summary, advanced Node.js concepts such as microservices architecture, rigorous testing frameworks, and performance optimization methods hold the keys to developing robust, scalable, and efficient applications. These aspects empower developers to take full advantage of Node.js capabilities and create solutions that stand out in a competitive market.
Best Practices for Node.js Development
When it comes to developing applications using Node.js, adhering to best practices is essential. These practices not only enhance code quality and maintainability but also ensure robust performance, making it easier for developers to manage their projects. With the growing popularity of Node.js, embracing these principles can help maintain a competitive edge in the ever-evolving landscape of web development.
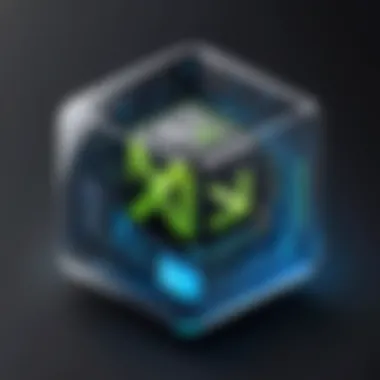
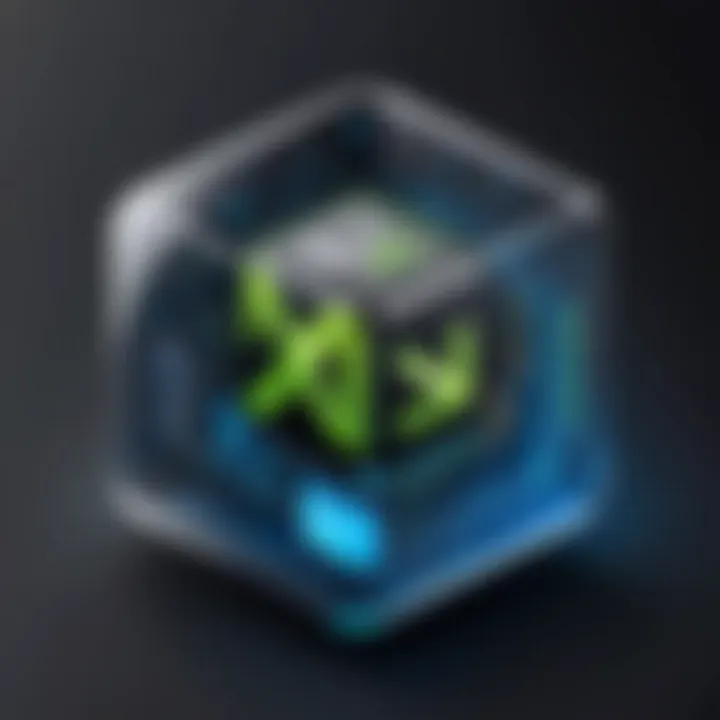
Code Quality and Maintainability
In the realm of software development, writing clean and maintainable code can be the difference between flourishing projects and those that flounder. For Node.js applications, this principle holds particularly true. The effectiveness of a codebase hinges on its readability and ease of updates. Here are some points to consider:
- Consistent Coding Style: Establishing a style guide places all developers on the same page. Following conventions, such as indentation, naming conventions, and comment usage, makes the code more navigable.
- Modularization: Splitting the application into smaller, manageable modules allows for easier testing and isolation of features. This not only speeds up the development process but increases reusability.
- Comprehensive Documentation: Both inline comments and external documentation play a critical role. Including clear descriptions of functions and parameters helps new developers quickly understand the underlying structure.
By focusing on these aspects, developers can create a code base that stands the test of time and is adaptable to changing requirements.
Security Considerations
Security should always be at the forefront of any software development process, and Node.js is no exception. Given its increasing use for creating server-side applications, understanding security considerations is crucial for protecting user data and credentials. Here are some of the key focus areas:
- Input Validation: Always validate user input to prevent injection attacks, particularly in databases. Libraries like Joi can help ensure that only expected formats are processed.
- Avoiding Sensitive Information Exposure: Keep sensitive data, such as API keys, away from the code by using environment variables. Utilizing tools like dotenv can streamline this process.
- Regularly Update Dependencies: Node.js relies heavily on packages. Outdated packages can harbor vulnerabilities. Using tools like npm audit aids in identifying and fixing known security issues.
Ignoring security can lead to severe consequences, so integrating these practices into development routines is non-negotiable.
Monitoring and Logging
Effective monitoring and logging provide essential insights into application performance and user behavior. They also reveal potential issues before they escalate into major problems, making them fundamental aspects of Node.js development. Here are several practices:
- Centralized Logging: Implement a centralized logging solution that aggregates logs from various services. Tools like Winston or Morgan can facilitate this.
- Real-Time Monitoring: Use monitoring solutions like New Relic or Datadog that offer real-time insight into application health. This can help catch bottlenecks or downtime early.
- Error Handling: Implement systematic error handling that captures unhandled errors and unexpected behaviors. Utilize tools such as Sentry, which sends detailed reports whenever an error occurs.
Monitoring and logging are not merely post-development routines; they are ongoing practices that ensure a Node.js application remains efficient and user-friendly.
In summarizing, following best practices in Node.js development significantly enhances application quality and security. These principles not only facilitate smoother operation but also pave the way for future growth and feature expansion, ensuring developers can respond swiftly to changing needs.
Common Issues and Troubleshooting
When diving into the murky waters of Node.js, it's crucial to acknowledge the common issues developers face. Understanding these problems not only saves time but enhances the overall experience. From beginners to those with more experience, everyone encounters hiccups along the way. Issues might stem from various sources like coding errors, environment misconfigurations, or unexpected behavior due to the event-driven model. Positively confronting these challenges is what separates a novice coder from an experienced developer. Let’s take a closer look at each concern and how to approach them.
Handling Errors Effectively
Errors are inevitable in any programming language, and Node.js is no exception. Yet, how you handle these errors can profoundly impact the performance and maintainability of your applications. One of the first steps is to utilize the built-in error handling mechanisms. Node's try-catch and promise rejection handling are fundamental techniques. Here’s a simple example worth noting:
Moreover, the importance of logging cannot be overstated. Using tools like Winston or Morgan can greatly improve your ability to catch errors before they escalate into major problems.
"An ounce of prevention is worth a pound of cure."
Listening to your application is crucial; after all, you can't fix what you don't know is broken. Setting up uncaught exception listeners let you catch those pesky issues that slip through the cracks.
Managing Dependencies
As your Node.js application evolves, so do its dependencies. Managing these can be a double-edged sword. On one hand, npm makes it remarkably easy to install and update packages. On the other hand, neglecting dependency management can lead to version conflicts or security vulnerabilities. It is important to periodically review your dependencies and utilize tools like npm outdated to keep tabs on what's being used.
Here are some practical recommendations:
- Use semantic versioning to understand the impact of updates.
- Regularly audit your dependencies to find vulnerabilities. You can run for this.
- Avoid unnecessary packages; every added library adds complexity.
Keeping a tight grip on dependencies helps stave off issues before they begin.
Resolving Performance Bottlenecks
Performance can often be a silent killer in application development. Node.js excels at handling concurrency, but there are still places where it may stutter. Understanding where bottlenecks are occurring is essential to improving your application.
One common issue lies in blocking code, which can interrupt the main thread. It is prudent to utilize the async capabilities of JavaScript whenever possible. Use callbacks, promises, or the async/await syntax to keep your app responsive.
Using profiling tools like Chrome DevTools or Node.js's built-in --inspect flag can help you identify where the bottlenecks are occurring. Here’s a quick code snippet to illustrate async functionality:
Lastly, optimizing your database queries can significantly enhance performance. Use indexing wisely and avoid excessive database calls.
By addressing these common issues proactively, not only can you enhance your productivity but also create a more stable and efficient Node.js application.
Node.js in the Real World
Node.js has fundamentally shifted the landscape of web development, making it a cornerstone in today’s tech stack. Its non-blocking I/O model and event-driven architecture allow it to handle numerous connections simultaneously. This efficiency has opened the doors for applications ranging from sophisticated online platforms to simple web services.
Case Studies of Successful Applications
Numerous organizations have adopted Node.js to enhance their performance and scalability. For instance, Netflix, a giant in the realm of streaming, employs Node.js in its user interface. By enabling a highly responsive and seamless experience, Netflix has improved its loading speeds considerably, catering to millions of subscribers simultaneously. The LinkedIn mobile app is another notable case. They migrated from Ruby on Rails to Node.js, which resulted in a significant cut in the resource consumption of their servers. This transition highlights how Node.js can effectively manage demanding applications without breaking a sweat.
Key benefits observed from these applications include:
- Enhanced Speed: Quick response times due to its asynchronous nature.
- Scalability: Managing larger loads with fewer resources.
- Ease of Development: Utilizing JavaScript both for client and server sides simplifies the workflow for developers.
"Node.js allowed us to build scalability right into our architecture from the start."
– Former Engineering Lead at LinkedIn
Industry Adoption and Trends
The ongoing trend towards microservices architecture is being fueled by Node.js’s lightweight framework. In various industries, businesses are recognizing the benefits of breaking down monolithic applications into smaller, manageable services. Industries such as finance and e-commerce are particularly drawn to this model because it facilitates faster deployment and continuous integration. Companies like Walmart have successfully leveraged Node.js to enhance their e-commerce platform, resulting in improved load times during peak shopping seasons.
Moreover, as real-time data processing becomes increasingly crucial, sectors like gaming and social media are embracing Node.js. Its ability to manage WebSockets enables developers to build applications that need real-time updates, such as online games and chat applications like Slack. As digital transformation accelerates, more organizations are likely to incorporate Node.js to stay competitive.
The Future of Node.js
Looking ahead, Node.js is poised to keep evolving. The Node.js Foundation continues to drive innovation with regular updates, ensuring that it remains relevant in a fast-paced environment. Upcoming features such as support for ES modules are expected to enhance the development experience, aligning Node.js with current JavaScript standards.
There's also a buzz around serverless architecture. As companies seek to reduce the overhead of managing server instances, combining Node.js with serverless functions could become a popular avenue. This shift allows developers to focus on writing code rather than managing infrastructure, further enhancing productivity.
In summary, Node.js has solidified its place in the tech industry, shaping how applications are built and deployed. Its successful application in various organizations serves as a testament to its robustness and versatility. As we look onward, the integration of emerging technologies and concepts will likely push Node.js to new heights, further solidifying its future in the world of web development.
For more in-depth information on Node.js, check out its entry on Wikipedia.