Mastering JavaScript for Web Page Creation
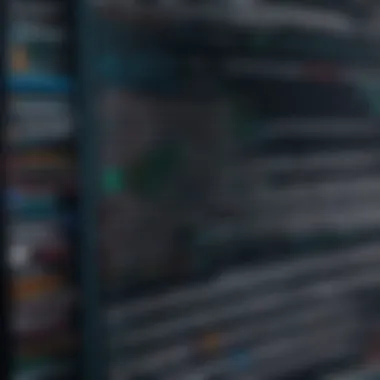
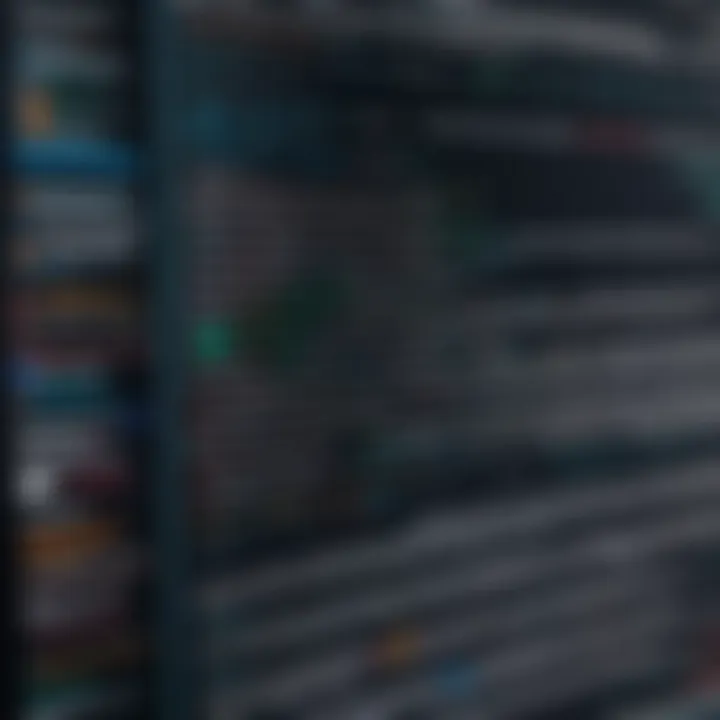
Intro to Programming Language
JavaScript is a versatile programming language that plays a pivotal role in web development. Understanding its significance requires exploring its history, key features, and where it stands in today's technology landscape.
History and Background
JavaScript was created in 1995 by Brendan Eich while working at Netscape. Initially, it was developed to add simple interactivity to web pages. Over the years, JavaScript evolved significantly, becoming a fundamental part of web browsers. The rise of frameworks and libraries such as jQuery, Angular, and React further propelled its usage.
Features and Uses
JavaScript is primarily known for its ability to create dynamic and interactive web content. Key features include:
- Client-side Scripting: It runs in the browser, enabling developers to enhance user experience without needing to contact the server for every interaction.
- Event Handling: JavaScript allows developers to write code that responds to user actions like clicks and keyboard inputs.
- Versatile Applications: Beyond web pages, JavaScript powers game development, mobile applications, and server-side programming via Node.js.
Popularity and Scope
JavaScript's popularity continues to grow. Many web developers consider it an indispensable skill. According to surveys, it consistently ranks among the most used programming languages. This widespread usage is evident in job postings and numerous learning resources.
Basic Syntax and Concepts
To build effective web pages, one must grasp JavaScript's basic syntax and core concepts.
Variables and Data Types
Variables are fundamental in any programming language. In JavaScript, you declare variables using , , or . The data types include:
- String: Represents textual data.
- Number: Represents numerical values.
- Boolean: Represents true or false.
- Object: A collection of key-value pairs.
Operators and Expressions
JavaScript offers various operators:
- Arithmetic Operators: Such as , , , , for mathematical calculations.
- Comparison Operators: Employ or for checking equality.
- Logical Operators: Include , , and for logical operations.
Control Structures
Control structures direct the flow of execution. Key control structures include:
- if Statements: Executes code based on conditionals.
- for Loops: Repeats actions multiple times.
- while Loops: Continues until a condition is met.
Advanced Topics
Delving deeper into JavaScript reveals advanced topics that enhance development skills.
Functions and Methods
Functions are blocks of code designed for reuse. Methods are functions associated with objects. Here is how you define a function:
Object-Oriented Programming
JavaScript supports object-oriented programming (OOP) principles. This promotes code organization and reuse. Objects can have properties and methods, which enhances functionality.
Exception Handling
Error management is essential in programming. JavaScript uses try-catch blocks. With this, developers can manage errors without crashing the application.
Hands-On Examples
Practical experience solidifies understanding. Below are examples illustrating JavaScript functionality.
Simple Programs
Creating a basic program can be as straightforward as this:
Intermediate Projects
Building a simple to-do list application can help reinforce skills. It involves managing user inputs and displaying items dynamically.
Code Snippets
Sharing concise and elegant code segments fosters learning. An example might include toggling visibility of an HTML element:
Resources and Further Learning
Continuous learning is vital in programming. Here are some valuable resources:
Recommended Books and Tutorials
"Eloquent JavaScript" by Marijn Haverbeke is an excellent choice for beginners.
Online Courses and Platforms
Platforms like freeCodeCamp and Codecademy offer immersive coding experiences.
Community Forums and Groups
Engaging with communities such as Reddit or Stack Overflow can provide support and enhance knowledge.
Join the conversation on Reddit to share experiences and learn from other developers.
Understanding the Role of JavaScript in Web Development
JavaScript plays a crucial role in web development, serving as one of the primary languages for building interactive and dynamic websites. Unlike HTML and CSS, which primarily focus on structure and style, JavaScript enables developers to enhance user experience through interactive features. This section will explore what JavaScript is, its historical context, and its significance in modern web development.
Defining JavaScript
JavaScript is a high-level, dynamic programming language commonly used in web development. It allows developers to create rich, interactive content on web pages. Fundamentally, JavaScript can manipulate the Document Object Model (DOM), which represents the page structure, enabling changes to the document content and style in real-time. This ability to execute client-side scripts makes it an essential tool in modern web applications.
History of JavaScript
JavaScript emerged in 1995, developed by Brendan Eich while he was at Netscape. Originally named Mocha, it quickly evolved to what we know today as JavaScript. The languageโs birth was driven by the need for a scripting language that would run in web browsers. Over the years, it has undergone significant development, leading to standardization through the ECMA-262 specification. This evolution has made it seamless to integrate with other technologies, enhancing its capabilities.
Importance in Modern Development
Today, JavaScript is one of the core technologies of the web, alongside HTML and CSS. Its importance lies in its ability to add interactivity and responsiveness to websites. Here are some key elements that showcase JavaScript's significance:
- Dynamic Content: JavaScript enables the creation of dynamic content that can change without requiring a page reload. This feature is crucial for modern web applications where user engagement is vital.
- Versatility: JavaScript can function on both the client-side and server-side, thanks to environments like Node.js. This versatility allows developers to use a single language across their entire stack.
- Rich Ecosystem: The language boasts a robust ecosystem of libraries and frameworks, such as React, Angular, and Vue.js, which streamline development processes and enhance maintainability.
JavaScript's flexibility and power have made it a cornerstone of successful web applications, shaping the way developers build and engage with users online.
In summary, understanding JavaScript's role in web development is essential for creating effective web pages. Its capabilities enhance user interactions, making web experiences richer and more engaging. As this article progresses, we will explore practical applications and techniques for mastering JavaScript as part of a comprehensive web development journey.
Getting Started with JavaScript
Getting started with JavaScript is a critical step for anyone delving into web development. JavaScript is not just a scripting language; it is a cornerstone of modern web applications. Its versatility allows developers to create both dynamic user interfaces and complex backend systems. In this section, attention will be on environment setup, writing initial code, and grasping the synergy between JavaScript, HTML, and CSS. This foundational knowledge is indispensable for effective web programming.
Setting Up Your Environment
Choosing a Code Editor
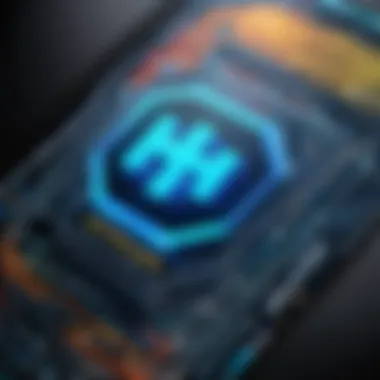
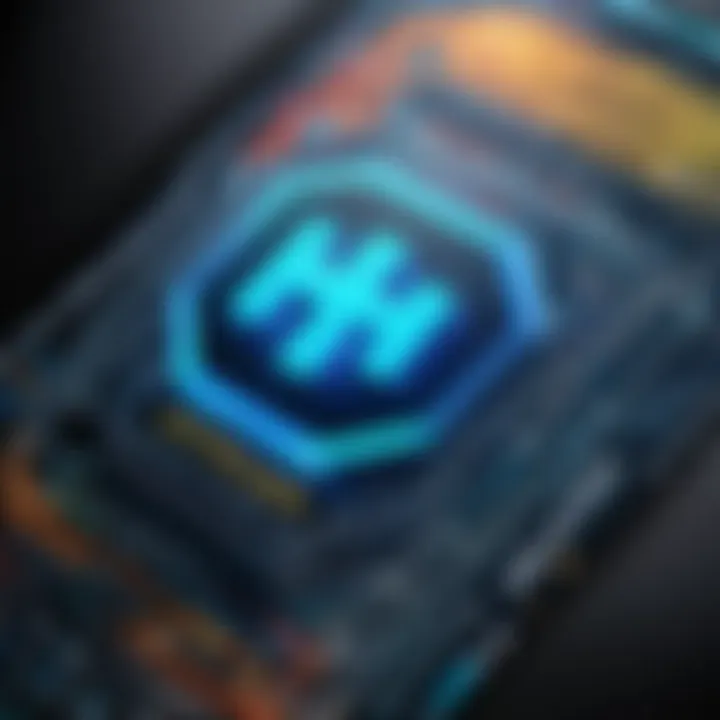
Choosing a code editor is one of the first decisions a developer must make. The code editor serves as the primary workspace for writing, editing, and managing code. It is important to select one that fits personal workflow and preferences. Popular editors such as Visual Studio Code and Sublime Text provide extensive features including syntax highlighting and customizable extensions. Visual Studio Code, in particular, is favored for its integrated terminal and debugger, which enhance productivity.
One unique feature of Visual Studio Code is the extensive library of extensions. These can add functionality like code linting or direct integration with version control systems. However, users may find the abundance of options overwhelming at first.
Installing Node.js
Installing Node.js sets the stage for running JavaScript server-side. This allows developers to use JavaScript beyond just client-side scripting. Node.js is known for its asynchronous capabilities, which enable handling multiple requests efficiently. It is a popular choice because it simplifies the process of building scalable network applications.
A distinct advantage of Node.js is the robust ecosystem of libraries available through npm (Node Package Manager). This allows quick access to numerous libraries that can simplify coding tasks. However, beginners might face a learning curve due to the asynchronous programming model Node.js employs, which is different from traditional synchronous programming.
Using Browser Developer Tools
Browser Developer Tools are essential for debugging and optimizing web applications. Every major web browser, such as Chrome and Firefox, comes equipped with these tools. They offer features such as inspecting HTML elements, monitoring network activity, and profiling performance. It gives immediate feedback on how changes affect the webpage.
The ability to directly edit HTML and CSS in real-time is a key benefit of using these tools. This facilitates rapid testing and adjustment. However, newcomers might feel daunted by the complexity and depth of features provided, but gaining familiarity with them is worth the effort.
Writing Your First JavaScript Code
Creating a Basic HTML File
Creating a basic HTML file is the starting point for any web project. HTML structures the content on a webpage. It defines elements such as headings, paragraphs, and links. The simplicity of HTML makes it a favorable choice for beginners.
The essential component is the DOCTYPE declaration, which informs the browser about the type of document. A simple HTML file might look like this:
The unique feature of creating a basic HTML file is that it lays the groundwork for integrating JavaScript. Even a simple HTML document can demonstrate the interplay between structure and interactivity.
Incorporating JavaScript
Incorporating JavaScript into an HTML document adds interactivity and engagement. This can be achieved either by placing tags directly in the HTML file or linking to an external JavaScript file. The former is useful for small scripts, while the latter is practical for larger projects.
For example:
The flexibility of incorporating JavaScript enhances the overall functionality of a web page. However, improper integration can lead to performance issues or script loading errors.
Running Your Code
Running your code is where the practical application happens. Most web browsers can directly render HTML and JavaScript. Simply opening the HTML file in a browser will display the output. Developers can also utilize local servers for more complex applications that require backend processing.
The key advantage of running code in this manner is immediate feedback. Developers can see changes in real time, facilitating a smoother development cycle. However, relying solely on a browser may not showcase certain server-side features, which necessitates alternative environments for comprehensive testing.
HTML and CSS: The Building Blocks of a Web Page
The integration of HTML and CSS lays the foundation for any web page. HTML provides the structure, while CSS is responsible for styling. Understanding both is crucial for achieving an effective layout and user experience. Without this knowledge, a JavaScript developer may struggle to manipulate elements on a page effectively. Therefore, mastering HTML and CSS is essential for leveraging the full potential of JavaScript and enhancing web development skills.
Understanding HTML Structure
HTML Tags and Elements
HTML consists of tags that define various elements within a webpage. Tags are the basic building blocks, often containing opening and closing tags such as for paragraphs or `` for divisions. This distinct characteristic of HTML allows developers to create structured documents that browsers can render correctly.
One key advantage of HTML tags is their semantic meaning. For instance, using , , and can improve the clarity of code. This clarity is beneficial when developers revisit the code or when other programmers collaborate on the project. However, improper use of tags can lead to confusion or accessibility issues, which must be taken into account.
The Document Object Model (DOM)
The Document Object Model, or DOM, is a programming interface for HTML and XML documents. It represents the structure of a document as a tree of objects, where each node is an object representing a part of the document. This feature is pivotal since it allows JavaScript to interact with the page elements dynamically.
The key characteristic of the DOM is its ability to reflect changes made by JavaScript in real-time. For example, if a JavaScript function adds a new element to the DOM, it will immediately appear on the webpage without needing a refresh. On the downside, manipulating the DOM can be performance-intensive, especially with large-scale applications, which developers must consider when optimizing their code.
Styling with CSS
Inline Styles vs. External Styles
When it comes to styling a webpage, developers can choose between inline styles and external stylesheets. Inline styles involve adding CSS directly to the HTML elements using the attribute, while external styles are placed in a separate file linked to the HTML document.
The primary advantage of external styles is maintainability. Changes can be made in a single file, allowing for easier adjustments across multiple pages. In contrast, inline styles can lead to cluttered code and are more challenging to manage, especially in larger projects. Still, inline styles may be beneficial for quick, one-time overrides if needed.
CSS Selectors and Properties
CSS selectors allow developers to target HTML elements for styling. By using selectors like class, ID, or element types, one can apply styles effectively. This is a significant feature of CSS, enabling a broader application of styles without repetitive code.
The benefits of understanding selectors and how properties work cannot be overstated. Effective use of selectors ensures clean and efficient stylesheets, which enhances website performance. However, some developers may struggle with specificity, where the order and hierarchy of selectors can lead to unexpected styling results. Understanding the nuances of these concepts is vital for delivering a well-designed user experience.
"Mastering HTML and CSS is crucial before delving into JavaScript; they are the core elements that provide structure and style to a web page."
This section highlights the foundational role that HTML and CSS play in web development. By grasping how these technologies work together, developers can progress to utilizing JavaScript more effectively, creating dynamic and functional web pages.
Integrating JavaScript with HTML and CSS
Integrating JavaScript with HTML and CSS is crucial in web development. This combination allows for dynamic interactions and style modifications which enhance user experience significantly. JavaScript brings HTML to life, enabling developers to create responsive and interactive interfaces. This section will explore how these elements work together and the benefits they offer.
Manipulating the DOM
Selecting Elements
Selecting elements is a key aspect of using JavaScript in web development. It involves identifying specific elements in the Document Object Model (DOM) so the script can manipulate them. The primary characteristic of element selection lies in its ability to dynamically target and interact with received data. This capability makes selecting elements a beneficial choice in web applications.
The unique feature of this process is its flexibility. For instance, developers can select elements by their tag names, classes, or even based on specific attributes. This flexibility allows for precise targeting, increasing the script's effectiveness. However, efficiency can become an issue if the selection process relies on complex queries. This may impact rendering performance, especially in larger applications.
Modifying Content and Attributes
Modifying content and attributes is an extension of selecting elements. Once an element is selected, developers can alter its inner content or its attributes. This contributes greatly to creating a dynamic web experience. The key characteristic of this process is the ability to change displayed information without needing to reload the page. Such flexibility is vital for modern web applications.
One unique feature is the ease with which attributes can be manipulated. For example, changing the attribute of an image based on user interaction is straightforward. However, developers must be careful; incorrect modifications can lead to errors or unexpected behavior in the application. The advantage here is the increased interactivity, while the disadvantage might include potential bugs if not handled properly.
Event Handling
Understanding Events
Understanding events is a fundamental aspect of creating interactive web applications. Events are actions that occur in the browser, such as clicks, key presses, or form submissions. Their significance lies in how they enable developers to create responsive behaviors in web applications. It is beneficial because events can initiate functions that execute various tasks when triggered.
A unique feature of events is their categorization. Events can be classified into two main types: event bubbling and event capturing. This helps developers control event flow, enhancing the script's efficiency. However, complexity can arise in complex interfaces where multiple events interact, calling for careful design to avoid conflicts.
Creating Event Listeners
Creating event listeners is critical for engaging users effectively. An event listener is a function that waits for a specific event to occur, allowing the script to react accordingly. This characteristic renders JavaScript an excellent choice for interactive web development.
The unique feature of event listeners is their ability to be attached to various nodes in the DOM. Developers can monitor events for multiple elements simultaneously. However, this can also lead to performance issues if too many event listeners are created. Striking a balance is key to maintaining optimal performance while enabling interactivity. Therefore, understanding and implementing event listeners effectively will significantly impact the user experience on the site.
"Integrating JavaScript with HTML and CSS creates a powerful trifecta essential for any modern web developer. Every interaction shapes user experience, making it vital to master these connections."
By understanding these elements, web developers can create powerful, interactive, and user-friendly applications.
Dynamic Content with JavaScript
Dynamic content is one of the cornerstones of modern web development. Using JavaScript, developers can create interactive experiences that respond to user inputs in real-time. This capability allows for a more engaging user experience, as web pages can display content that changes based on actions, such as clicks or form submissions. In this section, we will explore how JavaScript achieves dynamic content and the benefits of using it effectively.
One key benefit of dynamic content is its ability to improve user engagement. When users see content that adapts to their actions, they are likely to spend more time on the page. This not only enhances user satisfaction but can also lead to higher conversion rates for businesses. Yet, developers must consider performance implications. Too much dynamic content can lead to slower load times, particularly if not optimized properly.
Using Functions
Functions serve as fundamental building blocks in JavaScript. They allow developers to group code into reusable blocks that can be executed when needed. This reduces redundancy and helps maintain a cleaner code structure. Functions can simplify complex processes, making it easier to manage large codebases.
Defining Functions
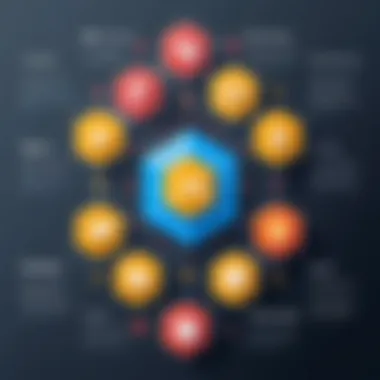
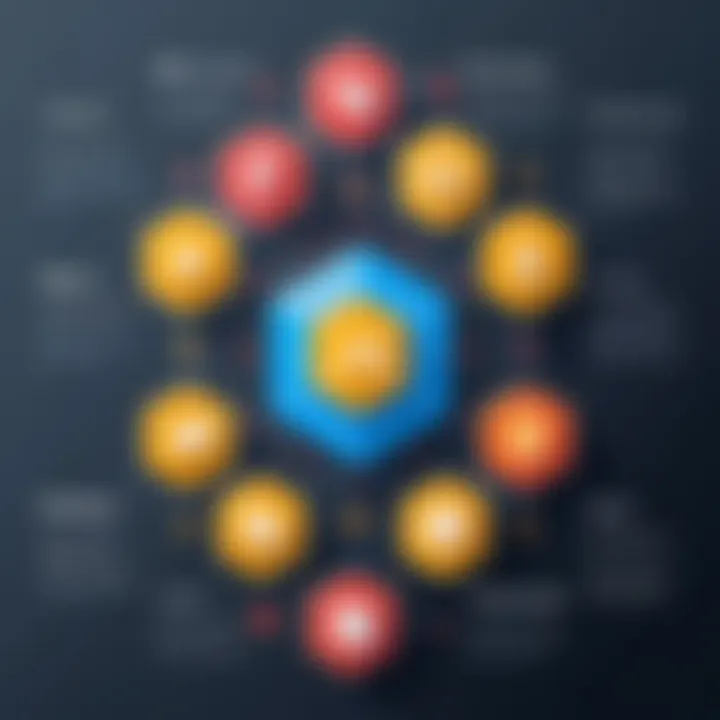
Defining functions involves specifying a set of instructions that can be executed when the function is called. A key characteristic of functions is their reusability. Once defined, a function can be called multiple times, each time with different parameters. This reusability makes functions a beneficial choice for organizing code logically.
A unique feature of defining functions is the ability to accept parameters, allowing for versatile behavior. However, overcomplicating functions with too many parameters can lead to confusion. Developers should strive for clarity while defining functions to ensure that they remain easy to understand and maintain.
Function Parameters and Return Values
Function parameters are variables passed to functions, and return values are the results produced by those functions. This concept is crucial for providing input to functions and obtaining output. A key aspect of using parameters is flexibility; developers can create generic functions that perform various tasks based on the input values.
Understanding return values is equally important. The ability to receive results from functions allows for dynamic content generation. This facilitates more interactive user experiences on a webpage. However, improper handling of return values can lead to unexpected behaviors, making it essential to manage this aspect carefully.
Implementing Loops and Conditionals
Loops and conditionals are essential for controlling the flow of JavaScript applications. They help in executing certain parts of code based on specific conditions, which is vital for creating dynamic content.
For Loops
The for loop is a powerful control structure used to execute a block of code multiple times. It is particularly useful for iterating over arrays or collections. One key characteristic of the for loop is its ability to control the number of iterations through its initialization, condition, and incrementation statements. This structure makes for loops a popular choice among developers.
For example, a for loop can be used to dynamically generate a list of items from an array, allowing content to be created on demand. However, care should be taken to avoid infinite loops, which can cause browsers to become unresponsive.
If Statements
If statements provide a method for executing code based on certain conditions, allowing for decision-making within scripts. This structure is designed to evaluate conditions and execute corresponding code blocks based on the outcome. The key characteristic of if statements is their straightforward syntax and clear logic, making them intuitive to use.
If statements are advantageous in the context of dynamic content as they enable developers to display different content based on user interactions. However, too many nested if statements can complicate code and make it less readable, thus developers should aim for balance in their usage.
Advanced JavaScript Concepts
Understanding advanced JavaScript concepts is vital for developers who aim to enhance their programming skills. These concepts build on the fundamentals and provide a deeper understanding of how JavaScript works. With knowledge of these advanced topics, programmers can write more efficient and powerful code. They can also create complex applications that utilize modern programming techniques.
Prelims to Objects
JavaScript is an object-oriented language. This means that objects are central to its design. Objects allow developers to group related data and functions together. This structure improves code organization and reusability.
Object Properties and Methods
Object properties are characteristics or attributes defined within an object. Methods are functions associated with those objects. A key aspect of object properties is that they hold data relevant to that object. For instance, if there is an object representing a car, properties might include color, model, and year. Methods can define behaviors like starting the engine or honking.
The significant benefit of using object properties and methods is encapsulation. This concept allows developers to keep related data and functionality together. This makes code easier to manage and update.
One unique feature of object properties is the ability to use getter and setter functions. These functions allow controlled access to properties. However, this adds complexity.
Creating Objects
Creating objects is a fundamental skill in JavaScript. There are several ways to create an object, such as using object literals, constructors, or the method. Each method serves different use cases.
A popular way to create objects is through constructors. Constructors allow for the creation of multiple instances of an object without code duplication. For example, you can create a constructor to generate several car objects.
The unique feature of constructors is that they support inheritance. However, this can also lead to potential misunderstanding among beginners regarding prototype chains.
Working with Arrays
Arrays are essential in managing collections of data. They provide a way to store multiple values in a single variable. The importance of arrays is evident in many applications. They allow for efficient data manipulation and access.
Array Methods
There are several methods associated with arrays. These methods allow developers to perform actions such as adding, removing, and iterating over elements. The key characteristic of array methods is their ability to simplify coding tasks.
For example, the method can transform each element in an array, ensuring cleaner and more readable code.
However, overusing such methods can lead to less optimal performance if not used carefully. Developers must understand when to apply specific methods to avoid inefficiencies.
Iterating through Arrays
Iterating through arrays is a critical operation in programming. It allows you to access each item within an array and perform actions individually.
There are different methods for iteration, including loops, , and the newer loop. The primary benefit of iterating through arrays is the flexibility it provides. Developers can apply various operations as needed, from simple logging to complex data transformations.
It is essential to choose the correct iteration method according to the specific need. For instance, using for non-blocking operations is effective. However, it has limitations compared to traditional loops regarding breaking out of the loop early.
Ultimately, advanced JavaScript concepts allow for more sophisticated application development, showcasing developers' skills and versatility. Understanding and leveraging these principles can significantly enhance one's ability to solve programming problems.
Utilizing JavaScript Libraries and Frameworks
The incorporation of JavaScript libraries and frameworks has transformed the landscape of web development. They provide developers with tools that streamline the process of creating dynamic and responsive websites. By utilizing well-established libraries and frameworks, developers can increase productivity and enhance code maintainability. This section will delve into two prominent libraries, jQuery and frameworks like React and Vue.js, which are widely adopted in modern web applications.
Prologue to jQuery
jQuery is a classic library that simplifies HTML document traversal and manipulation. Its design philosophy encourages simplicity and ease of use. With just a few lines of code, developers can achieve tasks that would normally take many more with vanilla JavaScript. For example, the command to select an element and hide it can be done in one line:
Selecting Elements with jQuery
Selecting elements with jQuery is a key aspect of its utility. The function can take a string selector to easily access DOM elements. One key characteristic is that jQuery supports a wide variety of selectors that extend beyond CSS selectors. This flexibility allows developers to work more intuitively with elements, making it a practical choice for many.
Advantages of using jQuery include:
- Reduced complexity in code.
- A large community with abundant plugins and resources.
However, one disadvantage is that it adds an extra layer of abstraction that may not be necessary for smaller projects. Therefore, understanding the appropriateness of jQuery in the scope of a project is critical.
jQuery Event Handling
Another significant feature of jQuery is its event handling capabilities. It simplifies the process of binding events to elements and enables seamless interactions. For example, attaching an event listener to a button click is straightforward:
Key characteristics of jQuery event handling include:
- Unified event model for cross-browser compatibility.
- Ability to handle multiple events using one function.
The popularity of jQuery in this realm lies in its streamlined approach. Nevertheless, it's essential to consider its performance impacts in more complex applications, where native event handling may provide better performance.
Overview of React and Vue.js
React and Vue.js are modern frameworks that further enhance JavaScript's capabilities for building complex interfaces. Their component-based architectures encourage reusability and modularity. This approach leads to more organized and manageable code, ultimately improving collaboration among developers.
Components and Props in React
In React, every UI element is treated as a component. This enables developers to break down user interfaces into modular pieces. Components can receive data from their parent component through props. A key characteristic of this approach is that it promotes reusability. Once defined, components can be reused in different parts of the application.
Benefits of using components in React include:
- Simplified code structure.
- Easier debugging and testing.
The trade-off can be the initial learning curve associated with component logic, especially for beginners. However, developers often find that once understood, this methodology greatly enhances their efficiency.
Understanding Vue Instances
Vue.js, similarly, operates on a component-based architecture. However, it introduces a unique aspect with its Vue instances. Each Vue instance manages its own data and lifecycle. This encapsulation allows for a clear organization of code.
Key aspects of Vue instances include:
- Reactivity, allowing automatic updates when data changes.
- Integration with HTML, making it intuitive for developers familiar with traditional web technologies.
One significant advantage is the ease of learning, making Vue.js a popular choice for those new to frameworks. However, the flexibility of Vue can lead to inconsistencies in larger projects if not carefully managed.
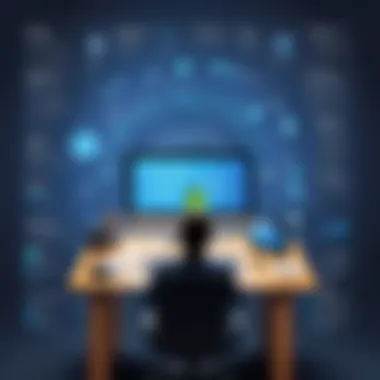
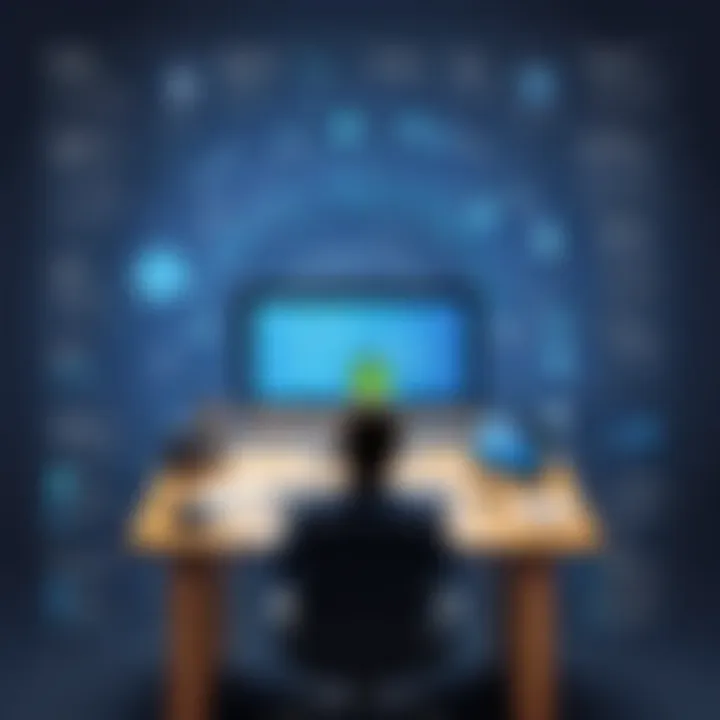
This synthesis of jQuery along with React and Vue.js showcases how JavaScript libraries and frameworks contribute significantly to efficient web development. Understanding their unique features and practical applications will empower developers to build capable and maintainable web pages.
Debugging and Testing JavaScript Code
Debugging and testing are vital skills for any JavaScript developer. The process of debugging involves identifying and resolving errors in your code. Testing ensures that your applications behave as expected. Both practices contribute to the overall quality of your web applications. Confidence in your code can significantly enhance the development process, leading to efficient and stable applications. Without effective debugging and testing, developers risk deploying faulty code, which can lead to poor user experiences and increased development time.
Common Debugging Techniques
Debugging can be approached through various techniques. Here are some common methods:
- Console Logging: This technique involves adding statements in your code to track variable values and flow of control. It is a simple yet effective method for understanding what is happening within your application.
- Breakpoints: Modern browsers provide tools to set breakpoints in your code. This allows you to pause execution and inspect variable states at any given point. It provides greater insight into your code's execution path.
- Debugging Tools: Tools like Chrome DevTools offer a wide range of debugging features, including performance profiling and network inspection. Familiarity with these tools is essential for streamlining the debugging process.
- Error Stack Traces: Pay attention to error messages and stack traces when issues arise. They provide valuable information about where an error occurred and can lead you directly to the source of the issue.
Investing time in mastering these techniques is beneficial for both novice and experienced developers. Effective debugging can save hours of development time, making it a crucial aspect of your skillset.
Prelude to Testing Frameworks
Testing frameworks provide structured environments to automate the testing process in JavaScript applications. They enable developers to write test cases that verify the functionality of their code, ensuring that updates do not break existing features. Utilizing testing frameworks can lead to higher quality applications and more maintainable code.
Mocha
Mocha is a flexible testing framework for JavaScript that runs on Node.js and in the browser. Its key characteristic is its versatility, allowing for the integration of various assertion libraries. This flexibility makes it easy to customize your testing setup. Mocha provides a simple interface to write and organize tests, which is its biggest appeal. One major advantage is that it allows asynchronous testing, suitable for modern JavaScript applications. However, one disadvantage might be the initial learning curve due to its extensive functionality, which can overwhelm beginners.
Jest
Jest is a popular testing framework developed by Facebook, primarily used for React applications. Its primary strength lies in its simplicity and zero configuration setup. This makes it a great choice for developers looking to get started quickly. One distinctive feature of Jest is its built-in mocking capabilities. This enables the isolation of components during testing, thus improving accuracy and reducing side effects. The downside is that Jest may not fit every project, especially those that do not use React, limiting its flexibility compared to other frameworks.
Effective testing is not an optional aspect of software development. It is essential for maintaining quality and reliability in your applications.
By exploring these tools and techniques, developers can enhance their debugging and testing workflows, ensuring robust JavaScript applications.
Best Practices in JavaScript Development
Best practices in JavaScript development are crucial for producing high-quality code that is clean, efficient, and maintainable. As web applications continue to grow in complexity, it becomes essential to prioritize these practices. By adhering to best practices, developers can minimize bugs, improve performance, and enhance collaboration among team members.
The primary benefits of following best practices include easier code maintenance, improved readability, and reduced errors. Clean code not only helps the original developer but also facilitates future updates by others. Furthermore, when multiple developers work on the same project, consistency in coding styles and practices aids in understanding the codebase more rapidly.
Writing Clean and Maintainable Code
Writing clean and maintainable code is at the core of JavaScript best practices. This includes using consistent naming conventions for variables and functions. Descriptive names help give context, making the purpose of elements clear at a glance. For instance, using as a function name is better than a vague .
Additionally, structuring the code into smaller, reusable functions promotes the DRY (Don't Repeat Yourself) principle. This approach means that rather than rewriting the same logic, developers can call the function whenever needed, reducing redundancy and the potential for errors.
Another aspect is the organization of files and directories. This can quickly become chaotic if not handled properly. For example, keeping JavaScript files organized by functionalities, such as and , allows for easier navigation and understanding of the applicationโs structure.
Optimizing Performance
Optimizing performance in JavaScript is vital for delivering a smooth user experience. There are several strategies developers can employ. Two critical areas are minifying code and understanding asynchronous programming.
Minifying Code
Minifying code involves removing unnecessary characters from the code without changing its functionality. This process decreases file sizes, leading to faster loading times for web pages. Key characteristics of minification include removing whitespace, comments, and shortening variable names.
Minification is a popular choice among developers because it significantly improves performance. A reduced file size means less bandwidth is used during data transfer. However, the drawback is that minified code is often difficult to read and debug. Developers should maintain a separate, readable version of their scripts while using tools to automate the minification process when deploying.
Understanding Asynchronous Programming
Understanding asynchronous programming is another vital aspect of optimizing JavaScript performance. In simple terms, asynchronous programming allows the execution of code without waiting for the previous operation to complete. This helps in managing tasks that take time, such as API calls and file reading, without blocking the main thread of execution.
The key feature of asynchronous programming is its efficiency. It allows more tasks to run concurrently, leading to better application responsiveness. However, it can introduce complexity, especially with error handling and maintaining code flow. Developers must be aware of using promises and async/await to handle these tasks effectively.
Applying best practices in JavaScript is not just a recommendation; it is essential for achieving robust and scalable applications.
By implementing these practices, developers set a solid foundation for their projects, ensuring functionality while paving the way for a smooth development experience.
Exploring APIs with JavaScript
The exploration of APIs, or Application Programming Interfaces, is a pivotal aspect of modern web development, especially when working with JavaScript. APIs facilitate the interaction between different software applications, allowing developers to integrate diverse functionalities into their web pages. As developers create increasingly complex applications, the ability to communicate with external services becomes crucial. This section aims to highlight the significance of APIs, their components, and how they can enhance the capabilities of web applications built with JavaScript.
What Are APIs?
APIs are sets of rules and protocols that allow different software components to communicate. They serve as an intermediary between different services, enabling data exchange. In the context of web development, APIs often function as bridges between the server and the client. For instance, when a user interacts with a web application that retrieves data from a database, an API is typically used to manage these requests and responses. Understanding the structure and purpose of APIs is essential for developers looking to create dynamic and responsive websites.
Making API Calls
To utilize an API, developers must understand how to make API calls. These calls can be categorized into various types, such as GET, POST, PUT, and DELETE, each serving specific functions in data management.
Using Fetch API
The Fetch API is a modern interface that provides a straightforward way to make network requests and handle responses. Its main characteristic is its promise-based approach, which simplifies asynchronous operations in JavaScript. Compared to traditional XMLHttpRequest, Fetch is easier to read and write, making it a favored choice among developers.
Using Fetch API, a developer can initiate requests with minimal code, which enhances productivity. The unique feature of the Fetch API is its ability to handle various types of requests while maintaining a clean and consistent syntax. However, itโs important to note that Fetch does not natively reject the promise on HTTP error statuses. This means that checking for status codes in the response is necessary for error handling. Overall, this API contributes significantly by streamlining the process of making requests and handling responses, thereby improving the efficiency of JavaScript applications.
Handling Responses
Once an API call is made using Fetch, the next crucial step is handling the responses received from the server. The primary aspect of handling responses is parsing the data returned from the API.
A key characteristic of handling responses is the necessity for developers to manage the data in formats like JSON or XML. In the case of JSON, which is the most common format, developers can easily convert the response using the method provided by the Fetch API. This capability allows the integration of the response data into the web application seamlessly.
The unique feature of handling responses includes error checking and managing various response scenarios, such as server errors or network issues. This can empower developers to create robust applications that respond gracefully to problems. While handling responses can be straightforward, it requires careful consideration to ensure that data is processed correctly.
"Understanding how to make and handle API calls is essential for enhancing your web applications."
Beyond the Basics: Moving Forward as a Developer
As you delve into the intricacies of JavaScript, it becomes paramount to recognize the significance of progressing beyond foundational skills. This section aims to equip developers with valuable insights and resources that can augment their capabilities and enhance their marketability in the ever-evolving tech landscape.
This journey entails not only mastering the syntax of JavaScript but also understanding its various applications in real-world scenarios. Engaging in projects, continued education, and connecting with communities of like-minded individuals creates a robust framework for growth. Let's explore the pathways available to those looking to elevate their skills.
Building a Portfolio
Project Ideas
Creating a diverse portfolio is a vital aspect of showcasing your abilities as a developer. When picking project ideas, it is crucial to focus on projects that demonstrate both your technical skills and your creativity. For instance, designing a personal blog or a portfolio website allows you to showcase your style and proficiency in HTML, CSS, and JavaScript.
One key characteristic of effective portfolio projects is their relevance to current trends. Integrating popular APIs or frameworks, like React or Node.js, can make your projects more appealing to potential employers. On the other hand, simplicity should also be considered; well-executed, straightforward projects can be just as impressive.
A unique feature of practical projects is their capacity to evolve over time. You can iterate on your projects, adding new functionalities and enhancing user experience based on feedback and learning. The downside can sometimes be the overwhelming feeling of needing to perfect every detail, which might lead to procrastination. In this context, it is important to adopt a growth mindset and prioritize learning over perfection.
Showcasing Skills
Showcasing your skills stems from effective presentation of your projects. Whether through a formal portfolio website or a platform like GitHub, it is important to present your work in a way that is accessible and engaging.
A key characteristic of showcasing skills is clarity. Clear documentation about your projects helps others understand your thought process and technical decisions. This clarity can position you as a knowledgeable candidate in the job market.
Furthermore, a unique aspect of showcasing skills is the opportunity for collaboration and feedback. Engaging with others through project showcases can lead to constructive criticism that sharpens your abilities. However, it is vital to balance the act of seeking feedback and maintaining your voice as a developer. Too much reliance on external validation can dilute your unique style.
Continuing Education and Resources
Online Courses
Continuing education plays a critical role in keeping skills fresh and relevant. Online courses offer flexible and structured learning opportunities that can cater to your specific needs. With platforms like Coursera, Udemy, and Khan Academy, you can find a broad range of topics tailored to various proficiency levels.
The key characteristic of online courses is their structured curriculum, which often includes hands-on projects that reinforce learning. This facilitates a practice-oriented approach, enhancing the retention of concepts. Meanwhile, the downside is the potential for course overload. It's imperative to choose courses wisely, focusing on the ones that align best with your learning objectives.
Moreover, most online courses are updated regularly to reflect the latest in technology and programming languages, keeping you at the forefront of industry standards. This adaptability can make learning more engaging and relevant.
Programming Communities
Participating in programming communities is an excellent way to foster learning and growth. Platforms like Reddit, Stack Overflow, and various discord servers offer a wealth of resources and support. Within these communities, you can ask questions, seek help with code challenges, and share your insights.
A key advantage of being part of programming communities is the exposure to diverse perspectives. Interacting with individuals from various backgrounds can enrich your understanding and broaden your approach to problem-solving. However, one potential disadvantage is the noise factor; with so many opinions, it can be difficult to discern which advice is valuable.
In summary, moving forward as a developer involves a blend of building a standout portfolio, leveraging online education, and engaging with programming communities. Each of these aspects contributes to a versatile skill set that is crucial for success in the tech industry. Stay proactive in your learning, and you'll find opportunities for growth and advancement.