Mastering JavaScript Game Development Techniques
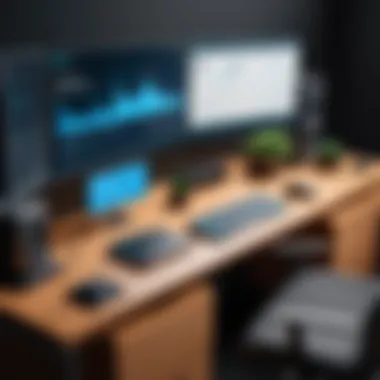
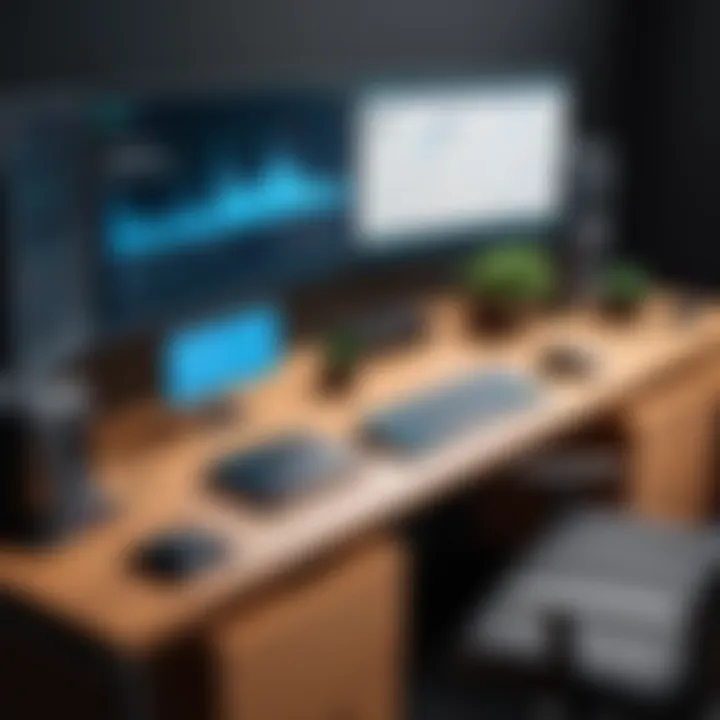
Intro
Creating a game using JavaScript can often feel like embarking on an exciting journey full of twists and turns; it may seem daunting, yet rewarding at the end. This guide aims to break down various stages of the game development process, ensuring that even those new to programming can grasp the fundamental concepts and apply them effectively.
Intro to Programming Language
JavaScript stands as one of the foundational pillars in web development. When you think about interactive web applications or games, JavaScript is often at the center of the action.
History and Background
Originally developed in 1995 by Brendan Eich at Netscape, JavaScript was created to add dynamic elements to web pages. Over the years, it has evolved significantly. As the need for more complex web applications grew, so did the capabilities of JavaScript. Today, it is not just a scripting language but a fully-fledged programming language, integral to modern web development.
Features and Uses
Its versatility makes JavaScript a go-to choice for game development. Here are some reasons why:
- Cross-Platform: Works on various devices and platforms, making it widely accessible.
- Event-Driven: Ideal for creating interactive gaming experiences.
- Framework Support: A myriad of frameworks like Phaser or Three.js help ease the development.
Popularity and Scope
JavaScript is everywhere, and its scope in the gaming landscape has been expanding steadily. Companies like Google and Microsoft harness JavaScript to build engaging experiences, and it's also a popular option among indie developers. According to the latest statistics, JavaScript consistently ranks among the top programming languages, evidencing its widespread acceptance and application.
Basic Syntax and Concepts
Understanding the basic syntax of JavaScript is crucial for anyone looking to develop games. This section will ease you into the fundamental building blocks of the language.
Variables and Data Types
In JavaScript, variables are like containers that hold data values. There are three main keywords to declare variables: , , and . For example:
The above code introduces two variables: one can change but the other remains constant. This distinction is essential in managing game states effectively.
Operators and Expressions
Operators in JavaScript allow developers to perform operations on variables and values. For enhancing a game, you may often use:
- Arithmetic Operators: To handle scoring systems.
- Comparison Operators: To check player conditions.
Control Structures
These structures help dictate the flow of your game. Conditional statements like or loops like and allow you to execute code based on certain conditions, essential for setting up game mechanics.
Advanced Topics
Once youāre comfortable with the basics, diving into advanced concepts will further prepare you for game development.
Functions and Methods
Functions are reusable blocks of code that perform specific tasks. Methods, which are functions associated with objects, play a significant role in object-oriented programming, commonly used in game development for better structure and maintainability.
Object-Oriented Programming
JavaScript supports OOP principles, allowing developers to create objects that represent real-world entities in their games. Creating a player object could look like:
Exception Handling
Errors will happen, and having a strategy to manage them is vital. Try-catch blocks provide a way to handle exceptions gracefully without crashing your game, preserving a smooth user experience.
Hands-On Examples
Nothing beats practical experience when it comes to learning. Here, youāll find simple programs that showcase essential JavaScript functionalities in a gaming context.
Simple Programs
Start with a basic setup like a score tracker:
Intermediate Projects
As you grow more comfortable, consider creating a simple 2D platformer using a framework like Phaser.
Code Snippets
A little snippet for moving a player character could look like this:
Resources and Further Learning
So youāre looking to dive deeper? Plenty of resources can sharpen your skills:
- Recommended Books and Tutorials: Books like "Eloquent JavaScript" offer great insights into the language.
- Online Courses and Platforms: Websites like Codecademy and Udemy provide structured courses.
- Community Forums and Groups: Engaging with communities such as Reddit or Stack Overflow can provide help and inspiration as you navigate your programming journey.
āThe only way to learn a new language is to use it.ā - A truth that holds quite credible in the realms of programming too.
With these foundational insights and resources, you're set to embark on creating games that not only entertain but also delight in the process.
Intro to Game Development in JavaScript
In the ever-evolving digital landscape, game development stands out as an exciting and dynamic field. Delving into game development, particularly through JavaScript, opens a treasure trove of opportunities and enhances one's programming skills. JavaScript empowers developers to create immersive gaming experiences right in the web browser, bridging barriers between coders and creativity. This section sets the stage by illustrating the importance of understanding game development in JavaScript, highlighting the journey from concept to realization and the substantial benefits it offers.
The Evolution of JavaScript in Gaming
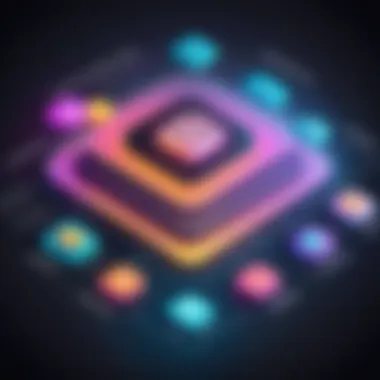
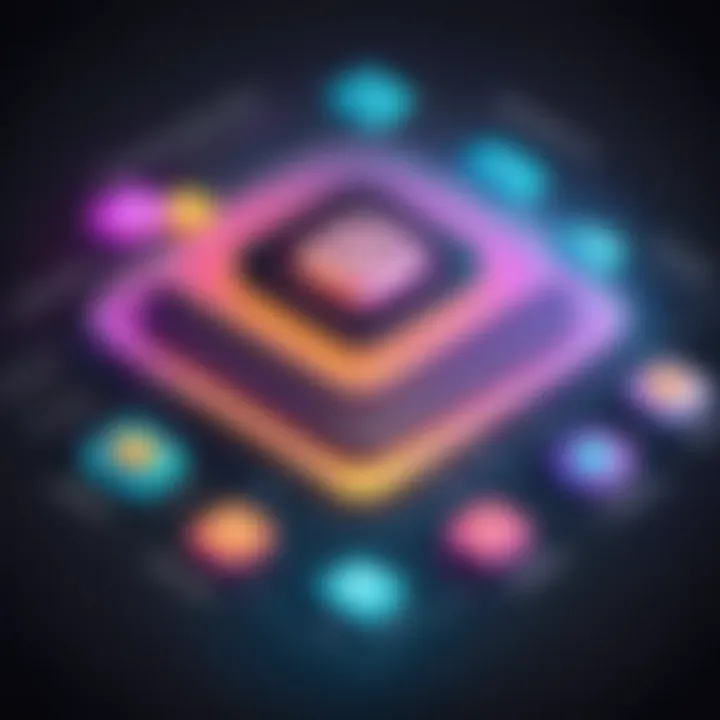
JavaScript might have started as a web scripting tool, but its rise in the gaming industry is nothing short of remarkable. Early web games were often simple and rudimentary, but as browsers became more powerful, so did the potential of JavaScript. Initially, games created in JavaScript faced performance issues compared to counterparts built on other platforms. A balance of innovation and community effort helped spice things up. For instance, advancements like Canvas and WebGL have equipped developers with the tools to harness graphics processing power.
Consider Phaser, a popular HTML5 game framework, which allows developers to craft rich, interactive games. Itās a testament to the language's growth, elevating JavaScript into a full-fledged game development environment. These tools, along with libraries like Babylon.js, demonstrate how the landscape has morphed, driving a new era of game development where web technologies reign supreme.
As a result, JavaScript games are no longer considered second best but are praised for their accessibility and versatility. Today, developers can create complex multiplayer experiences and visually stunning graphics almost effortlessly, laying the foundation for new kinds of players and diversifying the gaming market. The potential of JavaScript continues to expand, becoming a formidable contender in the game development arena.
Why Choose JavaScript for Game Development
When it comes to game programming, choosing the right language is crucial, and JavaScript has carved itself a niche, offering several advantages:
- Wide Accessibility: With JavaScript, your game can be played on any device with a web browserāno need for heavy installations or specific operating systems. This ensures a broader audience.
- Rapid Prototyping: The flexibility of JavaScript makes it easy to quickly build and test new game ideas. Developers can iterate fast, which is essential in todayās fast-paced gaming world.
- Rich Community and Resources: A vast community surrounds JavaScript. From forums like Reddit to comprehensive guides on platforms like Wikipedia, you have access to numerous resources, tutorials, and support to elevate your game development skills.
- Integration with Other Technologies: JavaScript works seamlessly with other languages and technologies. This interoperability means you can use libraries and frameworks to enhance your game's functionalities without getting bogged down by compatibility issues.
Ultimately, JavaScript is not just a coding language; it is a powerful tool that empowers developers to think creatively and innovate.
Setting Up the Development Environment
Setting the right development environment lays the groundwork for any successful game project. Rushing through this step can lead to confusion and frustration down the track. An effectively set up environment allows for a smoother workflow, reducing the time spent fixing errors that often stem from misconfigured settings or missing tools. Plus, understanding how to arrange your workspace encourages better coding habits. It makes troubleshooting simpler, promotes better collaboration with others on your team, and facilitates learning as you gain experience.
Essential Tools and Software
When diving into game development with JavaScript, having the appropriate tools is non-negotiable. Hereās a rundown of some essential software that can set you on the right path:
- Code Editor: Any decent code editor can help you write and manage your code efficiently. Visual Studio Code stands out due to its extensive features, such as syntax highlighting, terminal integration, and plenty of extensions tailored for JavaScript. Other options include Atom and Sublime Text, each of which offers unique functionalities.
- Version Control System: Git is a must-have for managing your game development project. It helps track changes in your code, enables you to work collaboratively and seamlessly revert to previous versions if something goes awry. Hosting services like GitHub or GitLab can help manage your repositories and provide a platform for collaboration.
- Graphics Editing Software: Tools like GIMP or Adobe Photoshop are important if you plan to create your own game assets. Even if you are opting to use pre-made assets, understanding how to edit images can enhance the unique flavor of your game.
- Audio Editing Software: Integrating sound into your game doesnāt need to be daunting. Audacity is a free software that allows you to edit audio snippets to give your game a rich auditory experience.
- Web Browser DevTools: Since youāll be developing for the web, familiarizing yourself with the built-in developer tools in browsers like Chrome or Firefox can help you debug your code more effectively and inspect game elements in real-time.
Leveraging the right tools not only speeds up your workflow but also enhances your overall programming experience when creating games.
Creating the Project Structure
A well-organized project structure is tantamount to the successful development of a game in JavaScript. It may seem burdensome to take the time to organize files and folders initially but it pays off handsomely in the long run. Building a clean architecture makes it easier to navigate, understand, and debug your code as your project grows in size and complexity.
Hereās how you might structure your game project:
- /assets: This folder can house all your images, audio, and other multimedia files. Organizing files into subfolders ā like /sprites, /sounds ā can save time in locating what you need later.
- /src: Your core game code should live here. It often helps to divide this further into modules for different features, such as /player, /enemies, and /levels.
- /lib: If youāre using third-party libraries, keep them all in one folder. This makes keeping track of updates and dependencies much easier.
- index.html: This is the entry point of your game. It will pull in your main JavaScript files and assets and serve as the backbone of your setup.
- style.css: If youāre utilizing CSS to style your game interface, doing this in a separate stylesheet keeps the design elements organized and maintainable.
By adhering to this organized structure, youāll streamline the development process and avoid many of the headaches associated with messy projects. And as you venture into larger projects, having a solid arrangement will make adding new features and fixing bugs feel more manageable.
A clear project structure reduces confusion and enhances productivity.
Core Concepts of Game Design
Understanding game design is like holding the blueprint of a building before itās constructed. Every brick, every beam needs to be properly placed, or else the structure may crumble. In the realm of JavaScript game development, having a solid grasp on core concepts of game design is not just an option; it's vital. These concepts dictate how players interact with your game and define the overall gameplay experience.
Understanding Game Mechanics
At the heart of any game lies its mechanics. Mechanics act like the rules of a board game; they tell players how to engage with the game world. When designing mechanics, consider elements like actions, goals, and feedback loops. For example, if players must jump over obstacles, the physics behind jumping should be intuitive; otherwise, players could feel like they're fighting against the game rather than playing it.
- Action Types: Identify what actions players can take, such as running, jumping, or building.
- Feedback Loops: Provide instant feedback through animations or sound effects when an action is performed. This makes the gameplay smoother and more engaging.
- Constraints and Challenges: Define what limits players face as they attempt to achieve their goals. This not only raises the difficulty level but also maintains excitement.
An effective game mechanic feels natural yet offers a challenge. If it doesn't mesh well with the gameplay, it may frustrate players. Think about how the popular game "Super Mario" relies on jumping mechanics combined with obstacles, creating a fun and engaging environment.
Designing Levels and Objectives
Crafting levels is akin to writing chapters in a story; each one must build upon the last and lead to a fulfilling conclusion. When designing levels, consider both the layout and the learning curve. Players should feel something is at stake, and their objectives should be clear. Here are some pointers to keep in mind:
- Progressive Difficulty: Ensure levels gradually increase in difficulty, allowing players to master mechanics before introducing tougher challenges.
- Clear Objectives: Clearly define what players need to accomplish in each level. For example, a goal might be to defeat a boss or collect certain items.
- Environmental Storytelling: Level design is a chance to convey your gameās story without words. Use visual cues and scenery to guide players and deepen their immersion.
Remember, levels canāt just be a collection of obstacles or enemies without thought. They should create a narrative that draws players in, making them feel part of something larger. Over time, as players progress from simple tasks to complex challenges, they invest emotionally in completing the game, which can lead to a more satisfying experience.
"Game design is the art of creating experiences that engage players. It blends creativity with technical skills, making the final product a beautiful journey to behold."
Mastering core concepts of game design isnāt merely an academic exercise; itās a prerequisite to developing games that resonate with players. As developers delve into understanding mechanics and crafting levels, they should always keep the playerās experience at the forefront. This approach not only enhances player satisfaction but can also lead to games that gain a dedicated following.
In summary, grasping the essence of game mechanics and the thoughtful design of levels and objectives serves as the bedrock for any successful game built with JavaScript. This sets up the stage for further development, where the foundation can be built upon, creating an engaging and immersive gameplay experience.
JavaScript Fundamentals for Game Development
JavaScript stands as the backbone of web-based gaming experiences, providing a dynamic and interactive platform for developers. Having a grasp of the fundamental elements of JavaScript contributes greatly to the overall success of a game. Understanding these key components can seamlessly lead to better design decisions, optimized performance, and an engaging user experience. In this section, we will explore the core concepts that every game developer should be familiar with, from the basics of variables to the principles of object-oriented programming.
Variables and Data Types
Variables serve as the building blocks of any JavaScript program, akin to containers that hold data throughout the life of your game. Understanding how to define and use variables is crucial, as it allows developers to manage game states, store player progress, and keep track of essential game metrics like scores.
JavaScript offers several data types:
- Primitive Types: These include , , , , and . Each serves a distinct purpose. For instance, you might use to track a playerās score and to indicate whether a player has completed a challenge.
- Reference Types: This includes objects, arrays, and functions. Objects are particularly useful for grouping related data, such as combining a player's name, score, and level information.
Given the need for flexibility in gaming, the nuanced understanding of data types allows developers to harness the full potential of JavaScript. A mistake in data handling can lead to bugs or even crashes, driving home the idea that a solid foundation in variables and types is not just helpful but essential.
Functions and Control Flow
Functions in JavaScript allow game developers to break down complex tasks into manageable chunks, promoting reusability and cleaner code. Much like crafting a detailed recipe, where each step brings a dish closer to perfection, functions lay out instructions for specific game tasks such as scoring, level advancement, and user inputs.
Control flow is equally important; it directs the sequence in which code executes based on certain conditions. The use of conditional statements like and can adjust gameplay dynamically, changing challenges based on player skill level or in-game events. For instance:
This simple code checks if a player outscored their previous best, thus providing immediate feedback and motivation to engage with the game further. Mastering functions and control flow enhances creativity and flexibility in game design, enabling responsive and immersive gameplay.
Object-Oriented Programming Principles
Delving into Object-Oriented Programming (OOP) principles opens another door to advanced JavaScript use in gaming. OOP enables developers to create clear structures, promoting better organization and reuse of code, which is particularly beneficial when scaling up a game.
In JavaScript, OOP principles such as encapsulation, inheritance, and polymorphism allow for the creation of complex game mechanics without making the code unwieldy.
- Encapsulation: This principle allows developers to bundle data and methods that operate on that data together. For example, a class can encapsulate properties like , , and methods like or .
- Inheritance: Building upon existing structures is invaluable. A class can inherit from a more generic class, allowing for easier expansion of game features and ensuring consistency through shared traits.
- Polymorphism: This principle lets developers write code that can work on different types of objects, leading to greater flexibility. For instance, both and could implement functions, but their implementations differ based on the game context.
By adopting OOP principles, JavaScript developers can streamline their game development process, leading to well-structured and easily maintainable code.
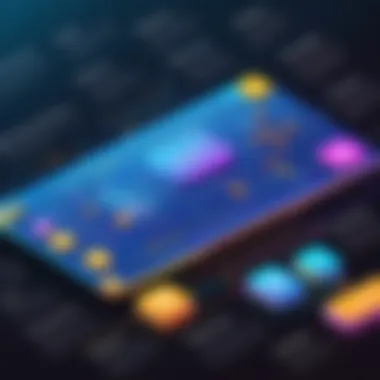
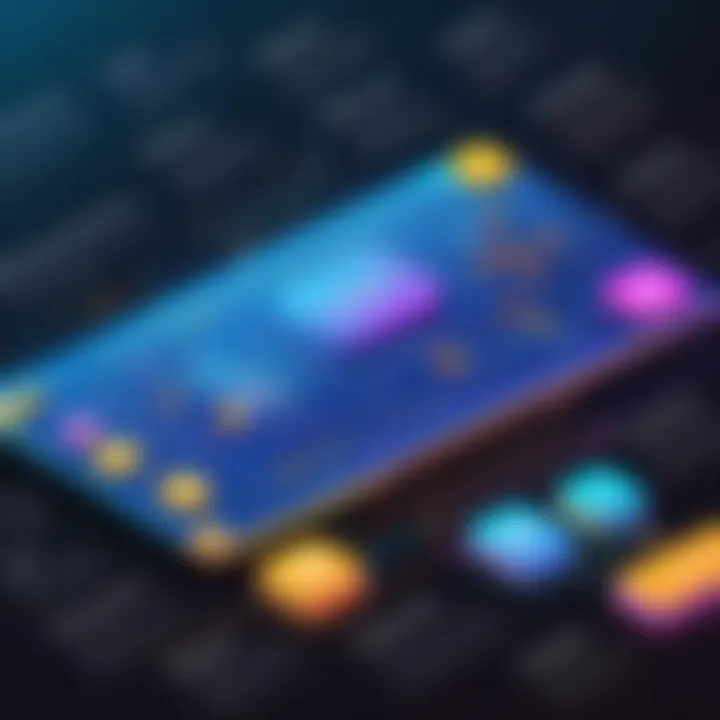
A deep understanding of JavaScript fundamentals can not only elevate the quality of a game but also empower a developer to innovate and create unique gaming experiences.
In summary, mastering JavaScript fundamentals such as variables, functions, and OOP principles lays a strong foundation for successful game development. These concepts interconnect to help create not just functional games but also captivating and enjoyable experiences for players.
Building the Game: Step by Step
When it comes to game development, building the game step by step is like following a recipe to bake a cake. You can't just throw all the ingredients together and expect it to turn out ok. This process is crucial, as it allows developers to systematically tackle each component that makes up the game. Undoubtedly, taking it step by step helps in streamlining the workflow and reducing errors. Each element of the game, from graphics to code logic, requires attention, and this structured approach allows for adequate focus on each detail, ensuring everything fits in the right place.
There are two primary categories involved: developing common game elements and implementing game logic. These are interdependent and crafting them carefully can create a cohesive gaming experience that is engaging and functional.
Developing Common Game Elements
Within any game, common elements serve as the building blocks that engage the player. Here, we cover three main facets of these elements: assets, user interface design, and game state management.
Assets: Graphics and Sounds
In game development, assets comprise graphics and audio that help create the world the player interacts with. The importance of these elements cannot be overstated; visuals and sounds enhance the immersive quality of the game. Unique graphics can give your game a distinct flavor, while sound effects can trigger emotional responses from players. The key characteristic of assets lies in their ability to evoke a sense of presence. For instance, in a racing game, the roar of an engine or the sound of tires squealing enhances the excitement and realism.
However, managing these assets comes with its own challenges. High-quality graphics can be memory-hungry, sometimes bogging down lower-end devices. Therefore, you need to strike a balance between quality and performance.
User Interface Design
Designing an intuitive user interface (UI) is essential for guiding players through the game. A well-designed UI can lead to a smooth gaming experience, helping players easily navigate options and understand controls. The clarity of action buttons, on-screen indicators, and menus can make or break how players engage with the game. If a player finds themselves frustrated figuring out how to shoot or use an item, they may quickly lose interest.
What sets effective UI design apart is its ability to integrate seamlessly into the gameplay while remaining visually appealing. However, overly intricate designs can lead to confusion, making game navigation cumbersome. Thus, simplicity is often the best approach, especially when targeting beginners or a wide audience.
Game State Management
Game state management handles the various states your game can have. Think of it as the brain of the operation; it decides when to show the main menu, pause the game, or display the game over screen. Having a robust management system ensures that transitions between these states are smooth and without hiccups, promoting a consistently engaging player experience.
An effective game state management system could be implemented through a state machine. This approach is beneficial as it keeps the game's structure organized, allowing you to easily add or remove states as required. Nevertheless, it can be a bit complex for beginners to grasp, but mastering it can significantly improve your game's performance and user experience.
Implementing Game Logic
Game logic is where the character of the game comes to life. This involves the application of rules and mechanics that dictate how players interact with the game world. Central aspects include collision detection and scoring systems.
Collision Detection
Collision detection defines how objects within the game interact with one another. Imagine a platforming game where a character jumps on a platform; collision detection ensures that when the character hits the platform, they land correctly instead of falling through. A fundamental aspect of any game is accurately identifying interactions which, if not addressed properly, can ruin the gameplay experience.
Being able to implement collision detection effectively can lead to increased game realism and satisfaction for players. However, it may become computationally expensive as the number of objects increases. So, itās crucial to utilize efficient algorithms to prevent lag or performance issues from emerging.
Scoring and Player Progression
Scoring systems are vital as they help track player success and encourage engagement. They provide feedback and motivation to players, reinforcing their efforts. A well-thought-out scoring system not only reflects player achievement but also can be used as a tool for progression, unlocking new levels or items based on scores. Such mechanics tap into the psychological aspect of gaming, pushing players to strive for improvement.
However, itās critical to strike the right balanceātoo easy a scoring system could diminish challenge, while too challenging can lead to frustration, potentially driving players away.
To sum up, building a game step by step enables developers to divide and conquer each element effectively, leading to a fulfilling gaming experience. Ensuring that each aspectāassets, UI, state management, game logic, collision detection, and scoringāis addressed comprehensively goes a long way in developing a standout game.
Enhancing User Experience
When diving into game development, a common goal is to create not just a playable product but one that players genuinely enjoy. The phrase "user experience" often gets thrown around, but in the realm of game design, it holds particular significance. A well-crafted user experience can mean the difference between a game thatās quickly cast aside and one that keeps players returning for more.
At its core, enhancing user experience involves understanding how players interact with your game. Itās about creating an immersive environment that hooks them from the get-go. This includes carefully considering elements like sound design, visual aspects, and overall gameplay mechanics.
The benefits of focusing on user experience are manifold:
- Increased Engagement: A game that feels good to play tends to hold a player's attention longer.
- Positive Feedback: Satisfied players are more likely to share their experience with others, growing your audience.
- Improved Retention: Players will return to a game that resonates well with them, leading to a loyal following.
However, achieving this is no small feat. Developers must weigh various factors, including how different aspects interact with each other and how they can serve the gameplay. This is where elements like sound and animations come into play.
"User experience isn't just about playing; it's about feeling the game."
Adding Sound and Music
Sound is often the unsung hero in game design. It can set the mood, build tension, and even provide feedback on a player's actions. Think about your favorite games ā the sound design is likely one of the reasons theyāre memorable. When players hear a catchy tune or the satisfying sound of collecting a reward, it enhances their overall experience.
Why is sound important?
- Emotional Engagement: Sound can evoke emotions in ways visuals sometimes cannot. A well-composed soundtrack can draw players deeper into the narrative.
- Tactile Feedback: The sounds associated with actions (like jumping or collecting items) provide crucial feedback. A thud when landing after a jump adds a sense of realism to the experience.
- Setting the Scene: Ambient sounds ā like the sounds of nature or city life in the background ā can help establish the gameās world, making it feel more immersive.
Incorporating sound requires careful planning. Youāll want to consider the following:
- Quality Matters: Invest in good sound assets; poor quality can take away from the experience.
- Volume Control: Ensure players can adjust sound levels as needed. Not everyone plays in the same environment.
- Use Plugins or Libraries: Consider using libraries like Howler.js to manage audio efficiently.
Incorporating Animations and Visual Effects
When it comes to animations and visual effects, they play a critical role in making gameplay smooth and captivating. Players should feel as though every action they take has a corresponding visual response. From character movements to flashy effects when achieving milestones, itās these small details that keep players engaged.
Hereās why animations are essential:
- Fluidity of Motion: Well-crafted animations can make gameplay feel more intuitive. A character that moves smoothly across the screen appears more lifelike and responsive.
- Immediate Feedback: Quick animations conveying player action, such as a character flashing when hit or enemies bursting into particles when defeated, signal success or failure efficiently.
- Visual Storytelling: Animations can convey backstory or character development without needing to lay out excessive text. A moment of despair conveyed through a characterās slumped shoulders can resonate on a personal level.
Some considerations in incorporating animations and effects:
- Performance Impact: Be mindful of game performance. Too many animations or heavy visual effects can slow things down, especially on less powerful machines.
- Consistency: Ensure the style of animations matches the overall aesthetic of the game. A mismatch can confuse or disengage players.
- Testing Is Key: Finally, always test your animations across different devices and settings to ensure everything runs smoothly.
By focusing on both sound and visual effects while developing your game, you're not just adding bells and whistles. You're carefully crafting an environment that elevates gameplay, making each player's experience uniquely enjoyable. Remember, it's these details that can turn a good game into a great one.
Testing and Debugging the Game
Testing and debugging are foundational pillars in the realm of game development. They ensure that your game runs smoothly and provides a positive experience for players. A game filled with bugs can ruin the fun and frustrate players, leading to poor reviews and lackluster engagement. Hence, addressing these issues is not just a matter of fixing problems but an essential part of delivering a quality product.
Let's consider the often underestimated aspect of testing. It encompasses a wide range of activities, from looking out for glitches in gameplay to ensuring that the user interface behaves as intended. When players face unexpected crashes, or when essential functions, like saving progress, fail, it can lead to widespread dissatisfaction. Thus, meticulous testing not only enhances gameplay but also builds trust with your audience.
Identifying Common Bugs
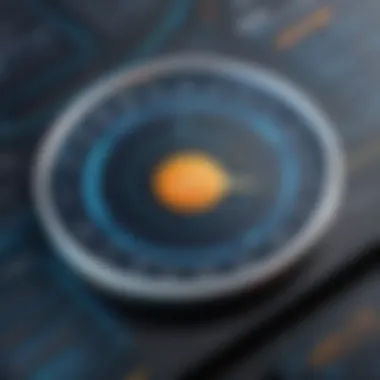
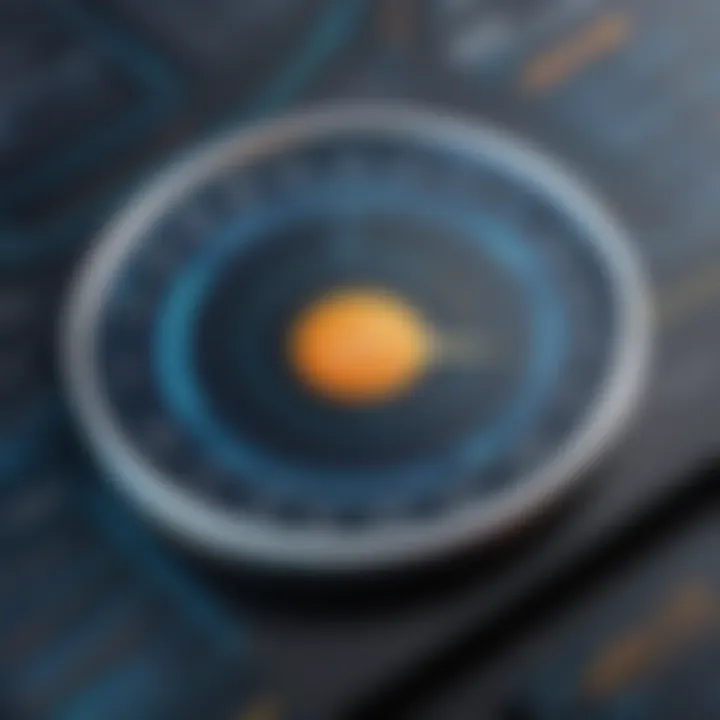
When developing a game, being familiar with potential bugs can save significant time and effort. Here are a few types to keep an eye on:
- Gameplay Bugs: These could manifest as objects not behaving correctly or incorrect scoring after a player action. For instance, if a player jumps but doesn't land correctly, it can lead to confusion and frustration.
- Visual Bugs: Graphics glitches may happen when assets don't load as intended. Imagine a character wearing mismatched outfits or erratic animations causing a disjointed feel during play.
- Audio Bugs: Sound cues may not play at the right time or could overlap, creating a chaotic audio landscape that takes away from the atmosphere.
- Performance Bugs: The game might run sluggishly on some devices due to inefficient resource management. This scenario is especially common with JavaScript games running on diverse hardware configurations.
Any developer should strive to uncover these issues early, ideally while still in the development environment. This early realization can significantly reduce the need for large-scale fixes later in the project life cycle.
Testing Strategies for Game Development
Effective testing is a blend of art and science. Here are key strategies to consider as you fine-tune your game:
- Unit Testing: This entails testing individual components. Testing functions and methods separately ensures each one works as designed. In JavaScript, frameworks like Jest can be particularly useful for this.
- Integration Testing: After unit tests confirm individual components, it's crucial to check how they work together. This step helps identify issues that might arise when combining different parts of your game, such as user interactions affecting game states.
- Beta Testing: Once initial testing phases wrap up, bringing in external players can provide fresh perspectives. They might encounter bugs or gameplay challenges you hadnāt noticed. Consider using platforms like Reddit to recruit beta testers.
- Automated Testing: Using tools for automated tests can save time and ensure your game continually meets standards. Automating repetitive tasks means you can focus on more complex aspects of game design.
- Playtesting: This informal testing method involves letting players experience your game and gather feedback based on their gameplay. Observing real players can reveal fundamental issues that you might otherwise overlook.
"Testing is not just a phase; itās an integral part of development that shapes the player's experience."
By implementing these strategies, you can catch issues early, saving you a world of headaches down the line. Keep in mind that diligent testing leads not only to fewer bugs but also to a more polished and enjoyable game at launch.
Optimization Techniques
In the realm of game development, especially when crafting games using JavaScript, the term optimization often pops up. Discussing this topic is crucial because an optimized game not only runs more smoothly, but also provides a better user experience. As gamers evolve and expect high-performance gameplay, developers must use techniques that enhance both considerations. So, what really goes into optimizing a game? Letās explore the specifics, benefits, and iterative considerations that come into play.
Improving Performance
One of the fundamental aspects of optimizing a game is improving its performance. This encompasses several strategies that help the game flow seamlessly, ensuring a delightful experience for players. Here are some key components:
- Efficient Rendering: Avoid excessive redraws for objects on the canvas or screen. Using techniques like dirty rectangles or culling can reduce the workload significantly. For example, only rendering whatās visible on-screen can save precious resources.
- Resource Management: Managing assets efficientlyālike images, sounds, and animationsāis vital. Instead of loading everything at once, consider lazy loading where resources are fetched as needed, reducing initial load time.
- Use of a Game Loop: The game loop is where the action happensāit updates game state and renders scenes. An effective game loop optimizes fps (frames per second). Using libraries like requestAnimationFrame instead of setInterval can help achieve smoother animations.
- Profiling and Monitoring Tools: Tools such as Chromeās Developer Tools or third-party applications like Unity Profiler offer insights into your gameplay performance. They allow developers to identify bottlenecks and refine their code during the development cycle.
Reducing Load Times
Load times can make or break the gaming experience, especially in a fast-paced environment. Here are effective techniques to consider:
- Minification and Compression: Minification removes unnecessary characters from code, including spaces and line breaks, while compression (like Gzip) reduces file sizes. These practices cut down on load time significantly.
- Image Optimization: Large images can delay load times. Use image formats like WebP or consider compressing images without losing quality. Additionally, utilizing spritesheets can reduce the number of HTTP requests made to load graphics.
- Content Delivery Network (CDN): Implementing a CDN can distribute game content across various servers worldwide, allowing users to download assets from locations closer to them, hence speeding up the loading process.
- Preloading Assets: Ensure that critical assets are preloaded before the game starts. This means that while the player is watching an intro or loading a main menu, background files like music or stage designs can load, minimizing wait times when entering the actual gameplay.
By applying these optimization techniques, developers can create games that not only play better but also engage players immediately, transforming a potentially frustrating experience into an enjoyable one.
"Optimizing a game is like tuning an instrument; every minor adjustment contributes to a harmonious performance.ā
In closing, remember that optimization is an ongoing process. As players provide feedback, you may need to revisit and enhance various elements of your game. The balanced relation between performance and player experience is a constant strive in the game development journey.
Publishing and Sharing Your Game
Publishing and sharing your game is not just the finish line of your development journey; itās the gateway to connecting with an audience. This section dives into the critical aspects of making your game accessible and enjoying it with the wider gaming community. Once all the hard work is done, the thrill of sharing your creation makes it all worthwhile. Moreover, understanding the ins and outs of publishing can ensure that your game reaches the right players and gains the traction it needs to succeed.
Choosing the Right Platform
While pondering over where to publish your game, several platforms can come to mindāeach presenting unique advantages and considerations. Whether you're leaning towards mobile, PC, or a web-based platform, itās important to evaluate a few key factors.
- Audience Reach: Different platforms cater to different audiences. For instance, publishing on Steam might land you in front of hardcore gamers, while Kongregate might attract casual players. Think about who you want as your players.
- Monetization Options: Having a solid monetization strategy is key. You might consider offering your game on itch.io, where you can choose a pay-what-you-want model, or going the freemium route on mobile through Google Play Store. Each platform has its own nuances, so investigate the potential revenue streams available.
- Technical Specifications: Each platform will likely have different required specifications. For web games, consider browsers and compatibility across varied devices, while PC might focus more on system capabilities.
- Community Features: Platforms like Reddit or Facebook provide opportunities to engage with players, share updates, and harness feedback. Getting involved in the community can create a buzz around your game.
When choosing the right platform, keep in mind that it reflects your vision and can be the launchpad for your game's success.
Marketing and Promotion Strategies
After youāve decided where to share your game, the next step is to ensure that it doesnāt get lost in the digital shuffle. Marketing is essential in gaining visibility and attracting players. Here are some effective strategies:
- Social Media Presence: Create a buzz by sharing development updates and engaging with followers on platforms like Twitter and Instagram. Behind-the-scenes content or sneak peeks can generate excitement.
- Build a Website: Having your own space on the web can make a significant impact. Use it to house game trailers, demos, and links to where the game can be downloaded or played, creating a one-stop-shop for interested players.
- Utilize Influencers: Reach out to gaming influencers or streamers who can showcase your game to their audience. A well-placed review or playthrough can significantly enhance visibility.
- Community Engagement: Donāt underestimate the power of communities. Engage with forums like Reddit or dedicated gaming Discord servers. Sharing your game there can invite immediate feedback and organically grow your player base.
- Launch Promotions: Consider offering promotions during launch such as discounts or limited-time offers. This creates urgency and can draw in early adopters.
"Marketing is no longer about the stuff you make, but the stories you tell." ā Seth Godin
Integrating these marketing tactics can help in crafting a narrative around your game that captivates potential players. Each strategy can enhance your game's reach, building a community that supports your creative endeavor.
Common Challenges in Game Development
Game development is an exciting journey, filled with creativity and innovation. However, it also comes with its fair share of hurdles. Understanding these challenges is crucial for anyone venturing into building games with JavaScript. Addressing these issues up front can save developers both time and frustration. In this section, we will illuminate two significant challenges: managing project scope and dealing with feedback and revisions.
Managing Project Scope
In any project, or especially a game development project, keeping an eye on scope is essential. Project scope refers to all the work involved in delivering a finished game. Without a clear scope, it's easy to go overboard. You might start with a simple concept and then suddenly find yourself entangled in features that werenāt part of the original idea.
To manage scope effectively, it's beneficial to:
- Set Clear Goals: Establish what your game will be about, the genres youāll tap into, and the features it needs. Being locked in on a vision helps maintain focus.
- Prioritize Features: Not all game elements are born equal. Decide which features are must-haves and which can be saved for a later version. This way, the core experience remains intact even if everything doesn't make it to launch.
- Regular Reviews: Implementation stages are opportunities for reflection. Check-in meetings can be a chance to assess progress and pivot on any nemesis of scope creep.
Still, itās important to remember that sometimes my expectations donāt match reality. Keeping a flexible attitude towards project scope will help in adapting to unexpected changes without losing sight of your goals.
Dealing with Feedback and Revisions
When your game starts taking shape, feedback is both a gift and a burden. On one hand, itās golden; on the other, it can feel like a maze. Having an open ear to critiques can significantly enhance your game, but it can also lead to frustration if not managed properly. Here are some ways to navigate feedback and revisions:
- Establish Feedback Channels: Make it simple for testers to provide constructive feedback. This can be through forums like Reddit or feedback forms on your game website.
- Filter Constructively: Not all feedback will be beneficial. Learn to differentiate between actionable insights and noise. What resonates with your vision? For instance, if someone says the game is too hard, consider whether itās frustratingly hard or challenging in a good way.
- Plan for Revisions: No game is perfect upon first launch. Be prepared for adjustments post-release. Create a roadmap for updates based on user feedback and any discovered bugs.
"The greatest enemy of creativity is the self-doubt that creeps in during the feedback stage. Prioritize what to change based on clear prioritization."
Ultimately, managing both project scope and feedback efficiently leads to a more polished final product. It's a balancing act, but one that can significantly uplight the gaming experience. By recognizing these challenges ahead of time, you can better prepare yourself for the reality of game development and focus on crafting an engaging and successful game.
End
In the realm of game development, arriving at a conclusion is often more than just wrapping things up. Itās a moment of introspection about the journey taken. This article has traversed the multifaceted world of developing games in JavaScript, illuminating several key points that are vital for aspiring developers.
Reflections on the Game Development Process
Reflecting on the game development process reveals the depth and intricacies involved. One cannot merely look at game creation as coding alone; itās an art form where creativity dances hand-in-hand with logical structure. Each segment of this guide offered insights not just into practical coding, but also the design philosophies underpinning engaging games. Projects often have their twists and turns, akin to navigating a maze, but these challenges sharpen problem-solving abilities and foster innovative thinking.
To encapsulate, a well-thought-out game combines various elementsāart, sound, mechanicsāand stitches them into a narrative that captivates players. Itās crucial to continually seek feedback throughout the development process; after all, the perspective of an outsider can reveal blind spots that even seasoned developers might miss. Netting a feedback loop can save time and ensure that the final product resonates with its audience.
Future Trends in JavaScript Gaming
Peering into the crystal ball of JavaScript gaming trends reveals exciting advancements and transformations on the horizon. One significant trend is the gradual emergence of WebAssembly, which complements JavaScript by allowing developers to write performance-heavy code in languages like C or C++. This technology opens doors for creating more complex games that run smoothly in browsers, enhancing the overall user experience.
Moreover, thereās a growing interest in incorporating artificial intelligence into games. AI has the potential to redefine player interactions and behaviors, making games more immersive. Imagine playing a game where patterns of engagement can shift based on user actions, creating a tailored experience.
Furthermore, the shift towards cross-platform gaming hints at a future where players can enjoy the same game across varying devices seamlessly. This trend is paramount for keeping players engaged, allowing them flexibility without being tied to a single medium. As developers tap into frameworks like Phaser or Three.js, the opportunities to create stunning visuals and seamless gameplay are boundless.
In summary, the landscape of JavaScript gaming is continually evolving, and as these trends unfold, developers must remain adaptable and curious. The journey of game development is ongoing, with new tools and technologies emerging regularly, offering fresh prospects for creative exploration.