Mastering the JavaMail API for Java Developers
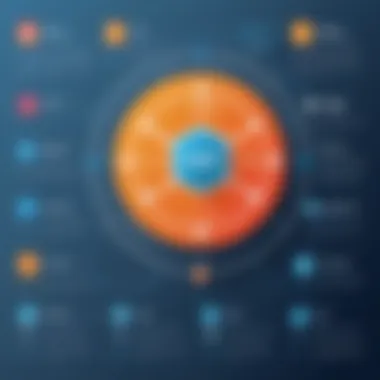
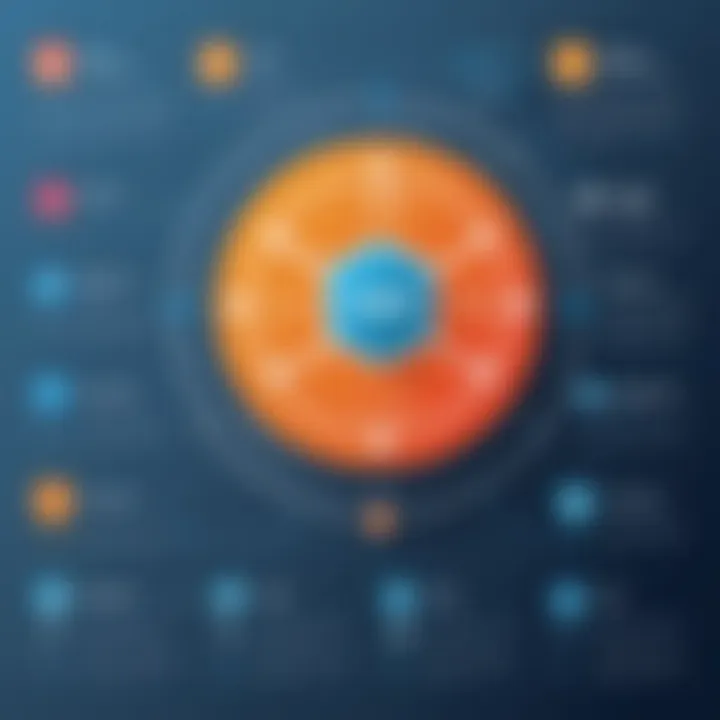
Intro
JavaMail API has become an indispensable resource for Java developers aiming to integrate email capabilities into their applications. Understanding this API is no longer just a bonus; it's a necessity for building modern applications that effectively communicate with users via email.
Email functionality extends beyond simply sending messages; it encompasses features like handling attachments, managing MIME types, and ensuring secure transmission of data. With these components in mind, JavaMail offers a robust framework for managing these aspects efficiently.
Importance of JavaMail API
The significance of JavaMail lies not only in its ability to send and receive emails but also in its versatility. Developers can craft applications that automate notifications, generate reports, or even facilitate user interactions through email—each of these applies to various fields like finance, healthcare, and education. The capacity to integrate email processing within Java applications enables businesses to enhance their operational efficiency.
"With Email being an essential form of communication in the digital age, mastering the JavaMail API is crucial for any budding Java developer seeking to stand out."
What Will Be Covered
This guide will transition from the fundamentals of setting up the JavaMail API to more advanced capabilities. We’ll cover:
- Setting up and configuring the JavaMail API
- How to send emails with ease
- Handling complex tasks such as managing attachments
- Understanding security protocols and their importance
In each of these sections, we will provide hands-on examples and practical insights to enrich your learning experience. By remaining actively engaged with the material, you will develop a solid grasp of the intricacies involved in using the JavaMail API effectively.
Intro to JavaMail API
In today’s digitally driven landscape, email remains a cornerstone of communication, both for personal and business uses. The JavaMail API empowers developers by providing a robust framework to seamlessly integrate email functionalities into their applications. Understanding how to effectively manipulate this API is essential for anyone looking to enhance their Java applications with reliable email delivery solutions.
What is JavaMail?
JavaMail is an application programming interface (API) that facilitates the sending and receiving of emails. It allows developers to craft messages, manage addresses, and communicate with various email servers. Essentially, what JavaMail brings to the table is an abstraction over the email protocols like SMTP, POP3, and IMAP. This helps developers to simplify the complex tasks of managing email processes without having to directly interact with the underlying protocols. Today’s IT projects require flexible and efficient communication strategies, and JavaMail stands tall in fulfilling those needs.
Why Use JavaMail?
One could ask why a developer chooses JavaMail over other solutions. The answer is multi-faceted:
- Ease of Use: JavaMail simplifies common tasks associated with email handling. Functions that would otherwise require extensive coding can be accomplished with just a few lines using this API.
- Compatibility: It supports multiple protocols and can easily communicate with most email servers, making it highly versatile.
- Integration: For those already working within Java environments, JavaMail offers seamless integration, which not only saves time but also minimizes potential errors that could arise from using disparate frameworks.
- Community and Support: Being a popular solution, JavaMail has a robust community. So, if you hit a snag, chances are someone else has faced it too, and you can find answers or documentation ready to help you out.
Overview of Features
JavaMail is packed with features that cater to a range of email functionalities. Here’s a brief overview:
- Sending Emails: Craft messages and send them using the SMTP protocol.
- Receiving Emails: Retrieve messages sent to you through the POP3 and IMAP protocols.
- Attachments: Send various file types along with your emails. This capability is crucial for business communication.
- HTML Support: Allows for formatted messages, advertisements, and newsletter design in emails, which enhances user engagement.
"With JavaMail API, integrating email functionalities into your Java applications can become as straightforward as pie."
For developers, having a grasp on JavaMail not only broadens their skill set but also streamlines communication solutions in their projects, thereby driving efficiency and effectiveness in deliverables.
Setting Up the JavaMail API
Setting up the JavaMail API is a crucial step for developers who wish to harness the power of email functionality in their applications. This process lays the groundwork for everything that follows, ensuring not only that the right tools are in place but also that they are configured correctly. The right setup can save developers a lot of hassle down the road, making it smoother to implement various email features.
Installation Requirements
Java Development Kit
The Java Development Kit (JDK) serves as the backbone for any Java-related development. It's the essential toolkit that provides all the tools you need to develop Java applications, including the Java Runtime Environment, the Java compiler, and essential libraries. For this particular article, having a solid understanding of the JDK is paramount, since the JavaMail API operates within the Java environment.
A notable characteristic of the JDK is its comprehensive set of tools for both development and debugging. This makes it a popular choice among developers because it simplifies the entire building process. One unique feature of the JDK is its built-in support for Java Language Specification, which ensures that you're writing code that adheres to the specified standards. Though the JDK can be resource-intensive, the advantages far outweigh the disadvantages. By ensuring that your environment is properly set up with the latest JDK version, you will enhance your ability to utilize JavaMail effectively.
Maven or Gradle Setup
When it comes to project management and build automation, Maven and Gradle stand out as top contenders. Both tools streamline the integration of libraries, dependencies, and configurations, making them almost essential for Java projects that wish to utilize JavaMail.
Maven is known for its ease of use and effective dependency management, which saves a lot of time compared to manual management. Gradle, on the other hand, offers greater flexibility and the ability to create custom build logic, which can be beneficial for more complex projects. A unique feature of Gradle is its support for multi-language builds, letting you integrate projects written in different languages seamlessly. In essence, choosing either Maven or Gradle not only simplifies the setup process but makes your project more manageable in the long run.
JavaMail Dependency
The JavaMail Dependency itself is an essential part of the overall setup. This library provides the fundamental classes and interfaces needed to work with sending and receiving emails. Having this dependency included in your project means you can efficiently manage all aspects related to email functionality.
A key characteristic is that the JavaMail library supports a range of protocols, including SMTP, IMAP, and POP3, allowing developers to work with various email services effortlessly. One unique aspect of JavaMail is its extensibility, allowing developers to add custom protocols if needed. Although there can occasionally be issues related to version compatibility, the benefits of this dependency far outweigh any potential drawbacks. By integrating the JavaMail library correctly, you're setting the stage for a successful project.
Configuring Your Environment
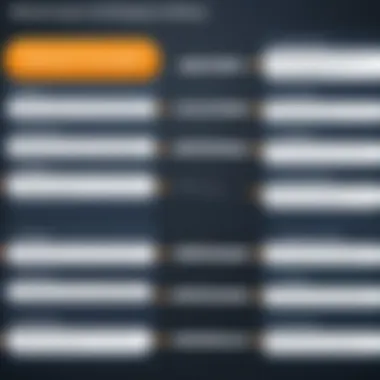
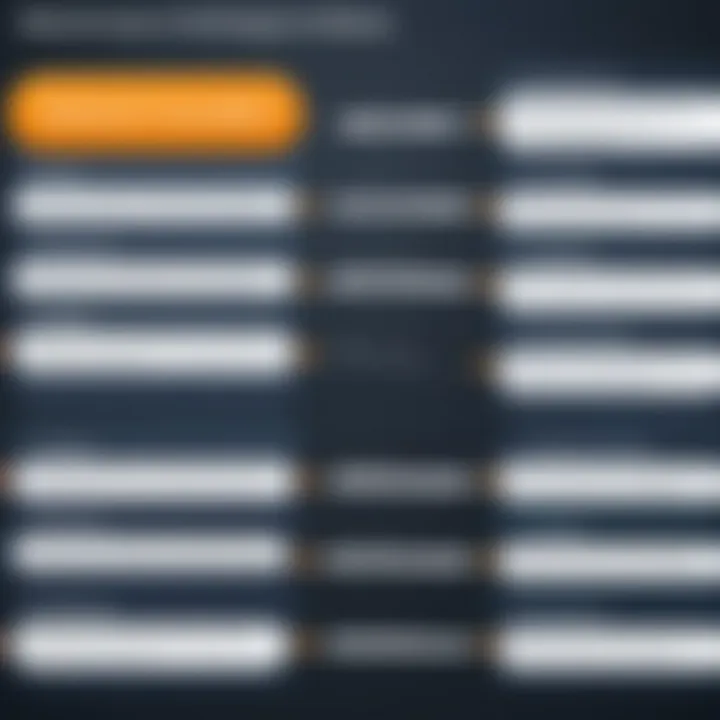
The configuration of your development environment is as important as the installation requirements. This phase involves setting up your Integrated Development Environment (IDE) and defining your project structure, which are critical for efficient coding.
IDE Setup
Setting up your IDE is a key element of configuring your environment for JavaMail development. An IDE like IntelliJ IDEA or Eclipse provides robust support for Java development. These tools offer features such as code completion, error detection, and debugging support—all designed to make development easier.
A notable characteristic is that modern IDEs facilitate quick and easy integration of libraries, including JavaMail. For instance, you can simply add the JavaMail dependency in the project settings without diving deep into manual configuration. A unique advantage of using an IDE is the visual interface it provides, making it easier for newcomers to navigate the coding landscape. Although some developers prefer lighter-weight code editors for their speed, the comprehensive features of an IDE generally make it a beneficial choice.
Project Structure
Defining a clear project structure is essential for managing your JavaMail projects efficiently. This involves organizing your packages and classes in a logical and easily navigable manner. Effective project structure helps maintain code readability, thus streamlining future updates or debugging.
The key characteristic of a well-structured project is that it allows for better collaboration among developers working in teams. One unique feature to consider is organizing your resources, such as images or email templates, into distinct folders. This approach can significantly reduce confusion and improve the overall workflow. While laying out a project structure might require some initial thought, its long-term advantages make it a worthy investment for anyone looking to use JavaMail to its full potential.
Remember: A careful setup and configuration can save you a world of trouble as you start diving deeper into email functionalities. Your success hinges on the choices you make in these early stages.
Basic Operations with JavaMail
When diving into the JavaMail API, understanding the Basic Operations is crucial for harnessing its full power. These operations are fundamental to implementing email functionality within any Java application. Mastering these components not only adds invaluable features to your software but also enhances user experience significantly. By concentrating on sending and receiving emails, developers can create applications that effectively communicate and deliver information.
Sending Emails
Sending emails is often the first interaction one has with the JavaMail API. It's the lifeblood of any application that requires email notifications or direct communication with users. The process can seem intricate at first, but breaking it down into smaller steps can simplify the undertaking.
- Setting Up the Message: You start the process by creating a object. This object represents the email message and allows you to set various properties like sender, recipient, subject, and body content.
- Establishing the SMTP Connection: Next, establish a connection to an SMTP server using , which lets you configure properties for auth, debug options, and more.
- Transport Layer: Finally, the class sends the message. You can use methods like to dispatch your email. This all ties together to form a seamless experience for the user, whether it's a newsletter, a verification email, or a simple notification.
Here's a brief code snippet to illustrate sending an email:
This code demonstrates the barebones of email sending. It showcases how to piece together the various facets that make the transmission successful. With this knowledge, you can customize the email further, adding attachments or using HTML formatting.
Receiving Emails
On the flip side, receiving emails can also be tackled with the JavaMail API. This aspect is essential for applications that require real-time updating of information or notifications.
Receiving emails typically involves connecting to a POP3 or IMAP server. While POP3 is straightforward for simply downloading mail, IMAP allows for more interactive email handling. Here’s how you can approach it:
- Connecting to the Server: First, establish a connection to your email provider using their specific server settings. This can be achieved through the class, similar to sending emails.
- Fetching Messages: Once connected, you can fetch messages using the class. The messages can be retrieved and processed as needed, making it practical for integrating incoming emails into your application logic.
- Processing the Emails: Finally, you can handle the emails fetched, whether by displaying them to users or triggering actions based on the content.
Here's a compact code example for receiving emails:
Learning these basic operations is like laying the first bricks of a sturdy foundation, upon which you can build powerful communication tools.
Advanced Email Features
When diving deeper into email integration within Java applications, advanced email features stand as pivotal components that broaden functionality and enhance user experiences. Harnessing the full potential of the JavaMail API requires an understanding of these features, especially in today’s digital landscape where interactivity and information dissemination are key. By leveraging the advanced capabilities of JavaMail, developers can ensure their applications are not just functional but also engaging.
Handling Attachments
One of the most practical applications of email technology is doing attachments. With JavaMail, incorporating attachments into your email becomes a seamless endeavor. Attachments can range from documents to images, presentations, and even zipped folders. By allowing users to send critical files alongside their messages, you empower them with stronger communication tools.
When handling attachments with JavaMail, there are a couple key practices to keep in mind. First, ensure that the file paths are accurate to avoid sending errors. A common approach is to use the class. For instance:
In this code snippet, two objects are created—one for the message and another for the attachment. Such a setup allows for clear and organized email composition. Moreover, when sending multiple attachments, it’s beneficial to use a container, which helps maintain a streamlined structure:
This results in a refined email that holds both the message and attachments together, ensuring recipients get everything in one package. Finally, remember to manage large files efficiently and provide necessary feedback to users about upload progress, especially in applications where responsiveness is crucial.
HTML and Multipart Emails
In a world teeming with visual stimuli, plain text emails can often feel lackluster. HTML emails, on the other hand, provide a dynamic avenue to engage recipients. They allow embedding images, hyperlinks, and styling that enhances the overall aesthetic and clarity of communication. JavaMail supports the creation of HTML-formatted emails, which enable developers to craft visually appealing messages that resonate with users.
To send an HTML email, you can use the same principles outlined in the multipart handling previously discussed. Here’s a sample way to achieve this:
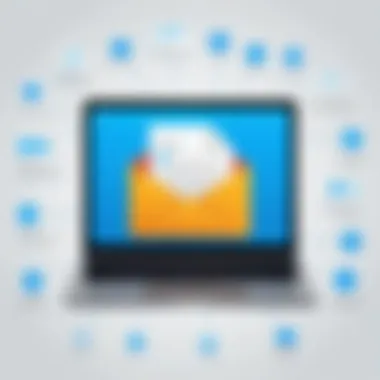
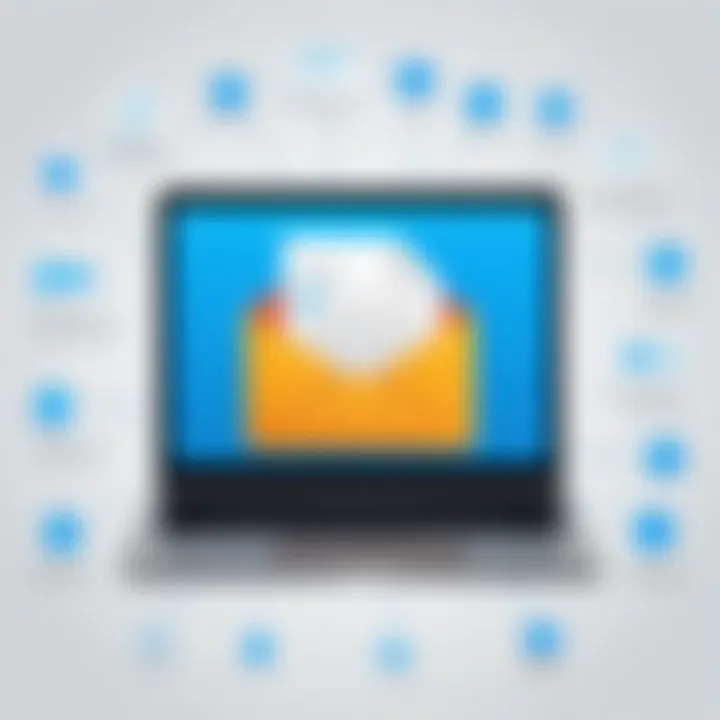
The above snippet sets the content type of the email to "text/html," making it possible to include elements like headers and paragraphs in HTML format. Such formatting greatly improves readability and engagement.
Moreover, creating multipart emails combines both plain text and HTML. This serves as a fallback mechanism—if a recipient’s email client doesn’t support HTML, they will still receive the plain text version.
In creating your multipart email:
By incorporating both formats, you cater to a broader range of email clients, ensuring your messages retain their intended appearance regardless of user settings.
Authentication and Security
When it comes to sending emails through Java applications, authentication and security are not just fancy extras; they're the backbone of any robust emailing system. The reality is that without solid authentication, you’re opening the floodgates for spam and potential hacks. So let’s get down to brass tacks — securing the transmission of your information should be a top priority.
The significance of this topic lies in its ability to protect sensitive information. Authentication ensures that only authorized users have access to your email services. Security protocols like SSL and TLS encrypt the data being transmitted, which prevents prying eyes from sneaking a look at what you are sending or receiving. This is crucial for businesses that handle sensitive data and personal information. In short, when you get the security aspects wrong, it can lead to serious repercussions, not to mention a tarnished reputation.
SMTP Authentication
SMTP (Simple Mail Transfer Protocol) authentication is the method used to verify a sender's identity. Think of it as checking the credentials of a person before allowing them to enter a secure building. In the world of JavaMail, implementing SMTP authentication is fairly straightforward.
To enable SMTP authentication, you would generally provide your email username and password. Here’s a basic idea of what the code looks like:
This snippet sets up SMTP authentication that allows your Java application to send emails securely by validating your identity with the mail server. Just make sure not to hard-code sensitive data like passwords; this is a rookie mistake. Instead, consider environment variables or configuration files that aren’t stored in public repositories.
Using SSL and TLS
When you think about putting your messages in envelopes for safe delivery, SSL (Secure Sockets Layer) and TLS (Transport Layer Security) function just like that. They add an additional layer of security by encrypting the connection between your application and the mail server, ensuring that all data is scrambled and unreadable by any nosy individuals who might intercept it.
To implement SSL/TLS in JavaMail, you must adjust a few properties. The following showcases how you can do just that:
Here you see , which tells JavaMail to use TLS. What’s critical to understand is that you want to connect over secure ports — for instance, 587 is commonly used for SMTP with TLS. This practice ensures that the communication channel remains as secure as a vault.
In today’s digital world, implementing effective authentication and security measures not only safeguards your emails but also helps build trust with your users.
In summation, investing the time to integrate SMTP authentication and SSL/TLS encryption into your JavaMail implementation yields more than just enhanced security — it creates a reliable emailing environment that sets the stage for smooth operations.
Troubleshooting Common Issues
Addressing issues that arise while using the JavaMail API is crucial for developers who are looking to implement email functionality in their applications efficiently. The ability to troubleshoot common problems not only ensures smoother operations but also enhances the overall user experience. Within this section, we will examine the typical hurdles developers face and how to navigate through them effectively.
Configuration Errors
Configuration errors are some of the most common issues that Java developers encounter when working with the JavaMail API. These errors often stem from incorrect setup in the properties used to establish the email connection.
For instance, a frequent error is not setting the property properly, which can cause the email-sending mechanism to fail. Here's a simple check-list to prevent configuration errors:
- Verify Email Server Details: Ensure that the SMTP server address and port number are correct. If the server requires authentication, the correct username and password must be included in the properties.
- Check for Typos: Sometimes, the simplest errors can stem from typographical mistakes in property names or values. A misspelled key can lead to the API not recognizing critical parameters.
- Use Consistent Formats: For certain properties like , ensure that the value is a string and specified correctly—"true" or "false" without quotes is essential.
- Consult Documentation: When in doubt, referring to official documentation might illuminate the issue at hand. The JavaMail API Documentation is a great resource for specifics.
"The devil is in the details." - Unknown
This adage holds especially true in programming, where minor oversights can snowball into frustrating roadblocks.
Network Connectivity Issues
Network connectivity issues represent another significant barrier to seamless email communication. These issues can arise due to a variety of reasons, making it imperative for developers to be aware of common pitfalls.
Here’s a couple of typical connection problems and how to tackle them:
- Firewall Settings: Firewalls can block outgoing connections to SMTP servers. Checking the firewall settings to ensure that the necessary ports (commonly 25, 587, or 465) for SMTP traffic are open can resolve this.
- ISP Restrictions: Some Internet Service Providers restrict the use of SMTP for sending emails, especially when using port 25. If your application fails to connect, it's worth inquiring if your ISP enforces such restrictions.
- Timeouts: Long delays can lead to timeouts when the server is unresponsive. Adjusting the timeout settings in your email session setup can help alleviate these frustrations. Here's a simple property setup:
- Testing Connectivity: Tools like telnet can be useful in testing if you can reach the SMTP server on the desired port. Example command:
By understanding and diagnosing these connectivity issues, developers can save themselves time and effort in the long run.
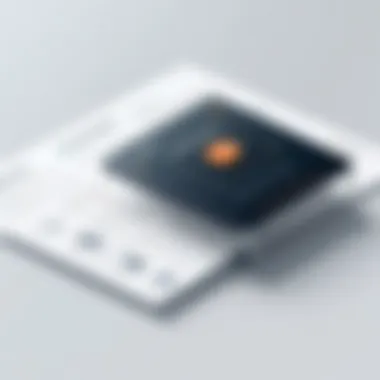
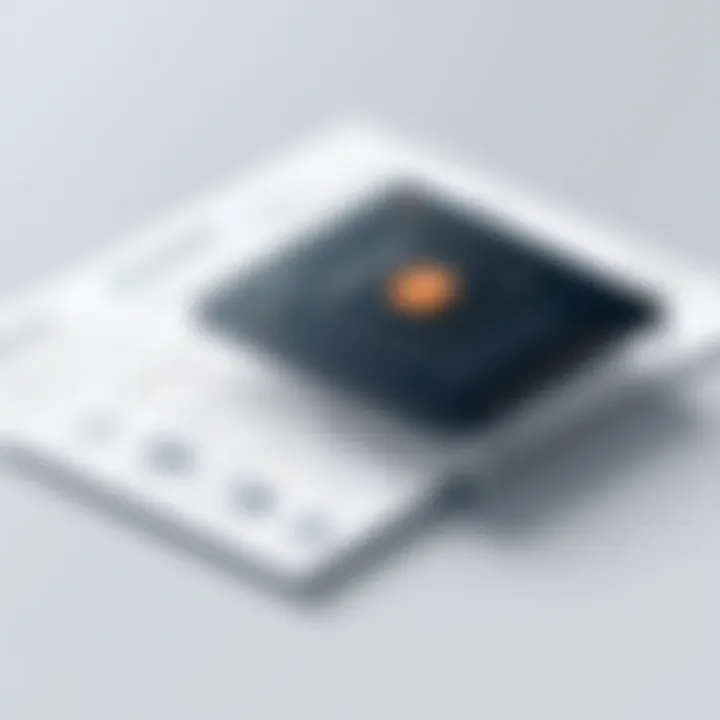
Best Practices for Using JavaMail
When we engage with JavaMail, embracing best practices becomes a cornerstone of effective implementation. Ensuring that emails are sent, received, and processed correctly is not merely a matter of coding; it directly impacts user experience, application performance, and security. Optimal usage of JavaMail can significantly enhance reliability, making it essential for developers who wish to harness its full potential while avoiding common pitfalls. Here, we delve into two specific pillars of best practices: performance optimization and code maintenance.
Performance Optimization
From the get-go, performance is paramount in any application. With JavaMail, optimizing performance can improve response times, especially when dealing with bulk mail or handling large attachments. Here are some focal points to consider:
- Connection Pooling: Create a pool of connections rather than establishing a new connection for each email. This practice conserves resources and minimizes the overhead of connection setup times.
- Batch Processing: If your application needs to send several emails at once, gather them up and process in batches. Instead of sending emails one at a time, this saves on network latency and can drastically improve speed.
- Threading: Implement multithreading to send emails in parallel. This requires careful management to prevent overwhelming the mail server and to maintain a balance between speed and resource consumption.
- Use of Efficient Protocols: Depending on your use case, choosing between SMTP, IMAP, or other protocols can also impact performance. For instance, IMAP is often preferred for managing large mailboxes efficiently.
Incorporating these practices can lead to noticeable performance gains in how your JavaMail implementation handles various use cases, ensuring that your emails don't just go out—they soar.
Code Maintenance
Writing code that works is one thing; writing code that stays workable is another challenge entirely. Maintenance is crucial to keep your JavaMail implementation resilient against both time and changes. Here are some pragmatic approaches:
- Modularization: Break down your email functionality into reusable modules. Instead of having one monolithic class, separate the concerns related to sending, receiving, and processing emails. This makes it easier to manage, test, and modify.
- Documentation: Maintain clear and articulate documentation within your code. Sometimes, two months later, it can be tough to remember why you opted for a specific solution, so a brief comment can be a lifesaver.
- Error Handling: Implement robust error handling practices. Emails could fail due to various reasons: invalid addresses, connection issues, etc. Capture these errors with well-defined responses, making rekindling these messages or debugging seamless.
"A well-maintained codebase is a gentle whisper; a failing one is a scream that drowns productivity."
- Regular Updates: Keep your JavaMail library and dependencies updated to avoid vulnerabilities and bugs. Newer versions often come with performance enhancements or additional features that can be beneficial.
Ultimately, investing in these aspects of code maintenance not only keeps your application running smoothly but also enhances your adaptability to future changes, reducing future workload.
Adopting these best practices in JavaMail propels you towards agile and efficient application development.
Real-World Applications
When considering the practical implementation of JavaMail API, recognizing its real-world applications proves crucial for any Java developer. This clarity aids not just in understanding how to use the tool but also in appreciating its significance in modern software developments. With various possibilities on the horizon, it's essential to tap into the benefits, challenges, and nuances associated with JavaMail's integration into existing systems.
Integrating JavaMail with Web Applications
Integrating JavaMail into web applications is like adding a feather to the cap of any project. The excitement of sending automated emails can be a game changer for user engagement and operational efficiency. Think of a web application handling user registrations. When a user signs up, an immediate welcome email can enhance user experience; more importantly, it assures them that they are part of the community.
To implement this integration effectively, developers often choose frameworks like Spring Boot. This allows a seamless configuration of email services. The simplicity of establishing such workflows opens up a plethora of notification possibilities. For instance, consider the following scenarios that highlight the benefits:
- Transactional Emails: Notifications regarding successful account creation, password resets, or order confirmations can all be automated.
- Promotional Emails: Sending newsletters or exclusive offers can boost user retention and conversion rates.
Moreover, using JavaMail ensures that these communications maintain a level of personalization, which is something users value highly in today’s digital landscape. However, it's worth noting that handling email lists responsibly, adhering to anti-spam regulations, and ensuring secure data transmissions should never be overlooked.
Using JavaMail in Enterprise Solutions
In larger ecosystems, the role of JavaMail morphs into something even more profound. Within an enterprise, communication needs extend beyond mere notifications. JavaMail can facilitate sophisticated solutions such as bulk email campaigns, internal announcements, or automated reports, proving itself as an indispensable asset.
For example, in a corporate scenario, let’s say a quarterly performance report needs to be circulated among hundreds of employees. With JavaMail, this can be done swiftly—with a few lines of code, the report is generated and sent out seamlessly. The key benefits include:
- Scalability: Easily enhances with growth; as your user base expands, JavaMail maintains performance while sending increased volumes of emails.
- Integration with Other Systems: JavaMail can be combined with other APIs for tasks such as data extraction or CRM system updates, allowing for a more unified approach to communication.
However, developers should be mindful of configuring these systems in a way that allows for effective monitoring of email flows and potential challenges such as email deliverability issues. Keeping tabs on bounce rates or spam complaints positions businesses to maintain a positive brand reputation.
"The future of email communication lies not just in sending messages but in managing relationships. Using tools like JavaMail effectively can establish a significant edge in this competitive landscape."
In summation, whether for web applications or extensive enterprise solutions, JavaMail API plays a vital role in shaping how organizations communicate. By making informed choices about integration and usage, developers can harness its full potential, turning everyday tasks into streamlined operations and enhancing user interactions across the board.
Future of JavaMail API
The JavaMail API, a longstanding fixture in the Java ecosystem, continues to be relevant in today's fast-evolving tech landscape. The future growth of this API hinges on its ability to adapt to the shifting needs of developers and the email landscape at large. On the technology front, advancements in email protocols, security features, and user interface improvements play a critical role. Understanding these elements isn't just a nice-to-have; it's essential for any developer looking to stay ahead of the curve. The insightful direction of this topic matters because it informs stakeholders about what to anticipate moving forward and how to plan effectively for app development.
Trends in Email Technology
Being attuned to the latest trends in email technology can give developers a competitive edge. Here are several key trends to keep in mind:
- Increased Use of AI: Artificial intelligence is reshaping how emails are created, filtered, and prioritized. Tools leveraging machine learning can automatically categorize emails and enhance spam detection.
- Enhanced Security Protocols: As phishing attacks grow more sophisticated, the implementation of advanced security measures such as DMARC and DKIM is on the rise. Developers need to implement these protocols in their applications to safeguard users.
- Rich Media Emails: The demand for visually compelling and interactive emails is on the rise. Incorporating media such as videos or podcasts directly in emails can significantly elevate user engagement.
- Open Standards Compliance: There's a growing emphasis on ensuring that all email technologies adhere to open standards. Following these standards helps maintain interoperability and user trust.
- Email as a Channel for Marketing Automation: More businesses are embracing email as a crucial component of their marketing strategies, utilizing automation tools to reach audiences effectively. Developers can build integrations with these tools to streamline efforts.
Staying up to date with these trends can empower developers to leverage JavaMail API for innovative applications that meet user expectations and industry demands.
Roadmap for JavaMail Development
Examining the roadmap for JavaMail development gives an insightful perspective on where the API might be headed. This roadmap outlines planned features, enhancements, and maintenance practices, all of which are vital for future updates:
- Continued Support for Modern Protocols: As new email protocols emerge, JavaMail's commitment to supporting them will be key. Ongoing updates to facilitate changes in standards can establish JavaMail as a leading choice for developers.
- Improved Documentation and Resources: A concerted effort to revamp existing documentation and provide abundant resources is fundamental. Developers thrive on reliable documentation that supports seamless implementation.
- Increased Community Support: Building a robust network of community resources can lead to better support systems for JavaMail users. Active forums and open-source contributions can drive innovation.
- Cross-Platform Compatibility: Enhancing JavaMail’s functionality across various platforms, including mobile and cloud settings, ensures that developers can deploy email solutions wherever they’re needed.
- Regular Updates and Maintenance: Committing to regular maintenance schedules and updates can prevent the API from becoming obsolete. Ensuring compatibility with newer Java versions is a foundational aspect.
By aligning with these elements on its development roadmap, JavaMail will position itself not just as a tool, but as an integral part of modern application development.