Mastering Java with W3Schools: A Comprehensive Guide
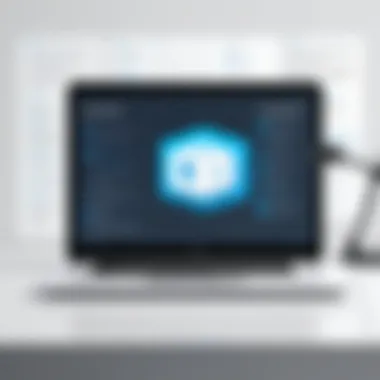
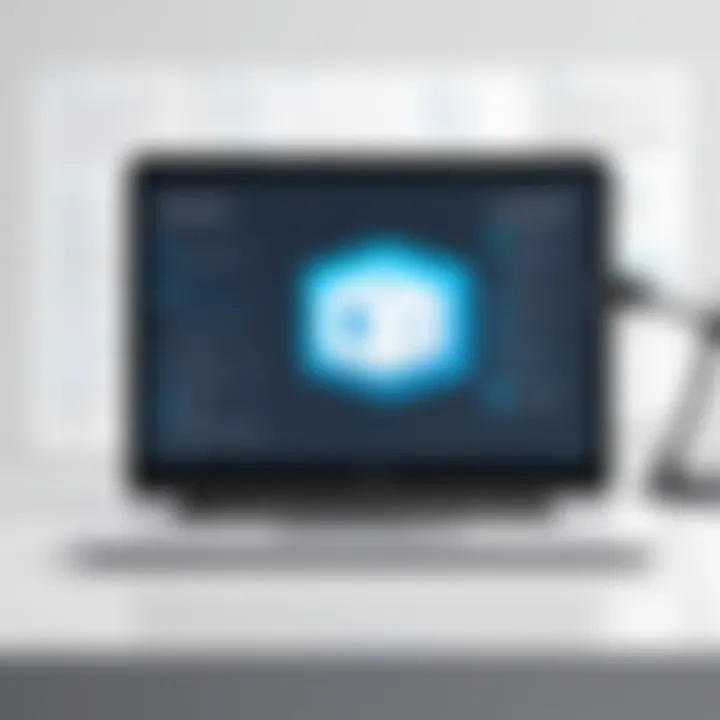
Preamble to Programming Language
Java is a versatile and powerful programming language, renowned for its platform independence and ease of use. It was officially launched by Sun Microsystems in 1995, evolving from small beginnings into one of the most important languages in software engineering. Understanding its history and background helps to appreciate its design decisions and widespread adoption.
History and Background
Java originated from a project known as the Oak Project. The goal was to develop a language that could run on various devices, from home appliances to computer systems. The compelling reason was to create software that is not limited by hardware architecture. As Internet usage soared in the late 1990s, Java garnered attention for its ability to function across platforms, leading to its acceptance in enterprise-level applications.
Features and Uses
Java shines due to its rich set of features, including:
- Platform Independence: Write once, run anywhere (WORA) principle makes Java highly portable.
- Object-Oriented: Promotes tidy code organization through classes and objects.
- Robust and Secure: Built-in garbage collection and a strong type system.
This language is widely utilized in different fields such as web development, mobile application creation, and enterprise software solutions. Its popularity and scope continue to expand, especially in the realm of big data and cloud computing.
Popularity and Scope
According to various statistics, Java regularly ranks among the top programming languages. The TIOBE index and RedMonk’s rankings consistently place it high. Its extensive use in academia and industry ensures a steady commitment to learning and adapting Java skills.
Basic Syntax and Concepts
Starting with the core syntax is essential for anyone eager to grasp Java. Mastering this fundamental knowledge lays the groundwork for understanding more complex concepts.
Variables and Data Types
In Java, a variable is a container that holds data. Each variable in Java has a data type, which defines the kind of data it can store. Common data types include:
- Integer (.int)
- Float (.float)
- Character (.char)
- Boolean (.boolean)
Operators and Expressions
Operators allow you to manipulate variables and values. Java supports various operators:
- Arithmetic: +, -, *, /, %
- Relational: ==, !=, >, , >=, =
- Logical: &&, ||, !
Understanding these operators is critical for creating expressions that evaluate to true or false, thereby controlling the logic of programs.
Control Structures
Control structures dictate the flow of execution in a program. Java has several key structures like:
- if-else statements
- switch statements
- loops (for, while, do-while)
Familiarity with these will enable anyone to create dynamic and responsive software.
Advanced Topics
Once the basics are mastered, learners can explore advanced topics that expand their skill set significantly.
Functions and Methods
Functions in Java are blocks of code designed to perform specific tasks. Methods are functions tied to classes. This distinction is key in object-oriented programming.
Object-Oriented Programming
Java is celebrated for its object-oriented nature. Concepts such as inheritance, encapsulation, and polymorphism allow developers to manage larger codebases effectively. Understanding these principles is crucial for writing scalable and maintainable code.
Exception Handling
Exception handling provides a mechanism to handle runtime errors smoothly. Java offers a robust framework through try-catch blocks, making it less likely that a small error can cause a program to crash entirely.
Hands-On Examples
Practical experience reinforces theory. Engaging with real code examples is essential.
Simple Programs
Creating basic programs helps reinforce core concepts. For example, a simple "Hello, World!" program introduces syntax and structure:
Intermediate Projects
As confidence grows, tackle more comprehensive projects that integrate multiple concepts. This could involve building a simple calculator or a text-based game, enabling practical application of functions and control structures.
Code Snippets
Developing a library of reusable code snippets enhances efficiency. Common tasks like sorting arrays or managing user input can be code snippets.
Resources and Further Learning
To deepen understanding, embrace additional learning resources.
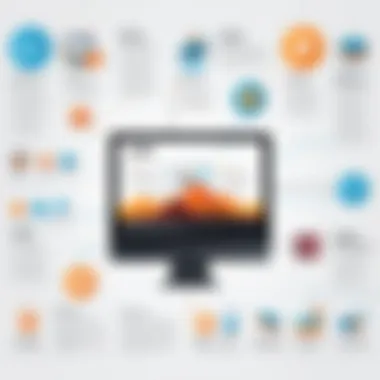
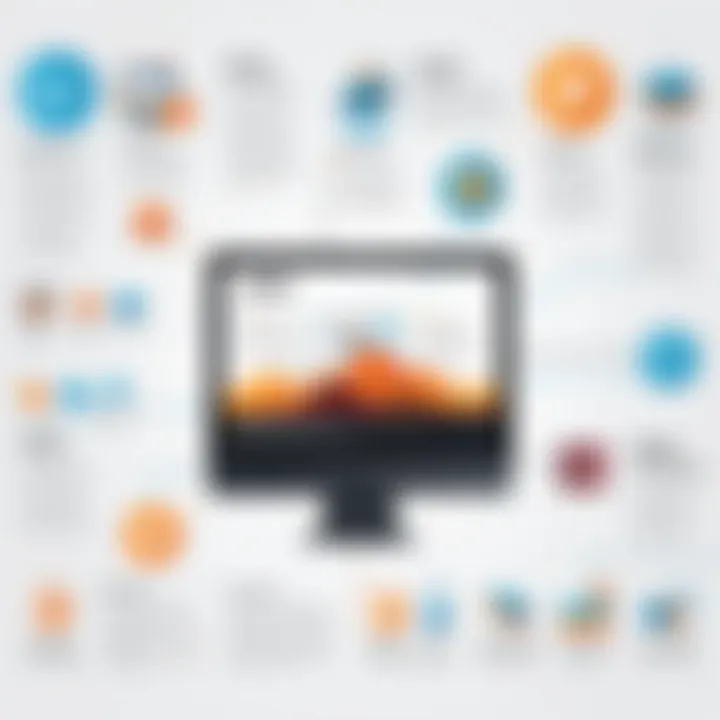
Recommended Books and Tutorials
Several resources stand out:
- "Effective Java" by Joshua Bloch: A thorough guide on best practices.
- "Java: The Complete Reference" by Herbert Schildt: Comprehensive coverage of Java fundamentals.
Online Courses and Platforms
Platforms like Coursera and Udacity offer structured learning paths in Java. W3Schools also provides interactive tutorials tailored for beginners.
Community Forums and Groups
Engagement with the community through forums such as Reddit can provide support and insights. Participating in discussions will further solidify understanding and keep current with trends.
"Learning Java not only enhances your programming skills but also opens doors to numerous career opportunities in technology."
Through diligent exploration of Java using resources like W3Schools, students and learners can effectively equip themselves for future challenges in programming.
Understanding Java
Java is a programming language that has stood the test of time. Its significance goes beyond just being a tool for writing code; it serves as a foundational element in many technological applications. Understanding Java means grasping its core principles, strengths, and ubiquity in the software development landscape. This article aims to explore Java comprehensively, highlighting its historical evolution, key features, and practical applications that make it an essential language for both beginners and seasoned programmers.
History of Java
Java was initially developed by Sun Microsystems in the mid-1990s. Its design focused on portability, making it a suitable choice for various platforms. Java introduced the concept of "write once, run anywhere," allowing developers to create software that could run on any device equipped with a Java Virtual Machine (JVM). The language's evolution includes major updates, with concurrent enhancements addressing performance, security, and developer productivity. This history underscores how Java has adapted to changing technological needs while maintaining its core tenets.
Key Features of Java
Java boasts several key features that contribute to its popularity:
Platform Independence
Platform independence is a fundamental characteristic of Java. This feature allows Java programs to operate on any device or operating system that supports the Java Virtual Machine. By compiling Java code into bytecode, it enables execution across diverse environments without modification. This benefit extends to developers, who can write code once and deploy it in numerous settings, enhancing efficiency and collaboration. However, the JVM's behavior can slightly vary across platforms, introducing potential debugging challenges.
Object-Oriented Programming
Java is inherently an object-oriented programming language. This paradigm organizes code into objects that encapsulate data and functionality. The approach bolsters code reusability and maintainability, making it an attractive option for software development. Object-oriented programming promotes a modular structure, allowing developers to troubleshoot and enhance individual components without significant impact on the overall system. Despite its advantages, it may introduce complexity, necessitating a solid understanding of concepts like inheritance and polymorphism.
Robust Security
Security is a pivotal concern in software development, and Java addresses this with a robust security architecture. Java implements multiple layers of security, including bytecode verification and a security manager to enforce access restrictions. The built-in security features mitigate risks, making Java a favorable choice for developing web applications and systems requiring protection against vulnerabilities. However, developers must remain vigilant to understand security best practices to maximize these features' effectiveness.
Automatic Memory Management
Java's automatic memory management, facilitated by garbage collection, simplifies memory management for developers. This feature eliminates the need for explicit memory allocation and deallocation, reducing the likelihood of memory leaks common in other programming languages. Automatic memory management contributes to increased productivity and lessens the cognitive load on developers. However, the drawback lies in the unpredictable nature of garbage collection, which can sometimes introduce performance overhead that developers need to consider carefully.
In summary, these features collectively establish Java as a viable choice for modern software development, offering benefits that enhance functionality and ease of use while presenting some challenges that developers must navigate.
Understanding these core aspects of Java is crucial as it sets the stage for deeper exploration of its application through resources like the W3Schools Java tutorial.
Getting Started with W3Schools Java Tutorial
Beginning the journey with Java programming is significant for those looking to dive into the world of coding. The W3Schools Java Tutorial serves as a valuable resource, especially for students and beginner programmers. This section focuses on how to effectively navigate the W3Schools platform and develop your very first Java program. Understanding this process provides a firm foundation for further exploration of Java’s core concepts and applications.
Navigating the W3Schools Platform
Navigating the W3Schools platform is essential for getting the most out of the learning experience. The site is user-friendly with straightforward navigation menus. You can easily locate Java tutorials under the programming section. Every tutorial integrates examples and exercises that reinforce learning. Furthermore, each topic often links to related concepts. This interconnectedness ensures that you do not have to search extensively for supplementary knowledge, facilitating a smooth learning path.
Creating Your First Java Program
Creating your first Java program could be seen as a rite of passage for newcomers. This section covers the necessary steps, which includes environment setup, writing code, and finally compiling and running the program. Each step plays a crucial role in ensuring that the programming logic is understood and executed correctly.
Setting Up Your Environment
Setting up the environment is critical as it defines how you will write and run your code. Often, developers use Integrated Development Environments (IDEs) like Eclipse or IntelliJ IDEA. These tools provide debugging features, code suggestions, and a convenient interface to manage your projects. The benefit of using an IDE is its user-friendliness and the support it offers to catch errors prior to running code. However, some might prefer simpler text editors like Notepad or Visual Studio Code for smaller tasks. This can cause less overhead but might lack advanced features.
Writing Java Code
Writing Java code is where creativity meets logic. In the W3Schools tutorial, you can learn the basics of syntax, data types, and control structures. A key characteristic of Java is its readability, making it easier for beginners to grasp. The tutorial encourages hands-on practice, allowing learners to write their first lines of code in an interactive environment. This practical approach can enhance understanding. However, beginners might feel overwhelmed by the abundance of information initially available.
Compiling and Running
Compiling and running your Java program is vital. Compiling translates your code into machine language. W3Schools uses Java’s command line tools for demonstrating this process, which makes it accessible and easy to follow. By running your program, you can see the output and identify any errors that need fixing. The unique advantage here is that learners not only write but also actively engage in the complete development lifecycle of software. This engagement can foster a deeper understanding and retention of knowledge.
"Learning to write Java code is like learning to play an instrument. Practice is key."
Core Java Concepts
Core Java concepts lay the foundation for understanding Java programming. They are crucial for any learner aiming to master Java, providing the basic tools to build more complex applications later in their journey. In this section, we will explore key aspects such as variables, data types, and control flow statements. These elements are not only fundamental to Java but also enable programmers to solve problems and create efficient code.
Variables and Data Types
Variables are essential in any programming language, including Java. They serve as containers to store data values. By naming a variable, developers can refer to data throughout their program without needing to reference the value directly, making code more readable.
Data types dictate what kind of data can be stored in a variable. Java provides several data types, which include:
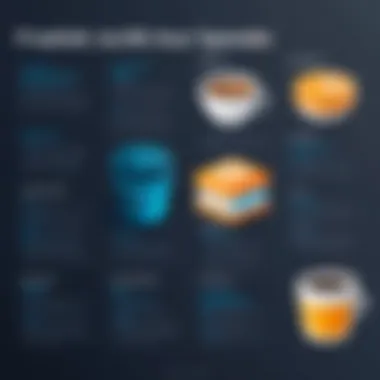
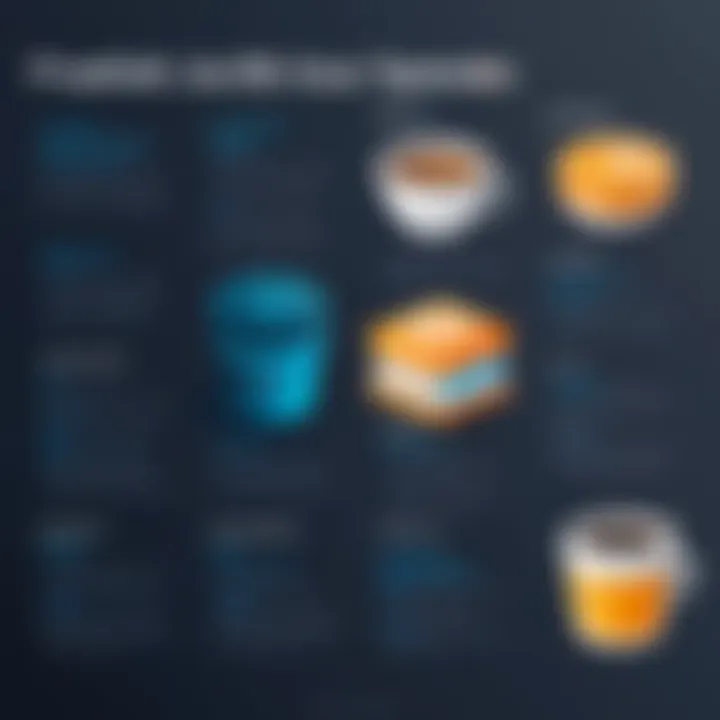
- Primitive types: such as , , , and , which represent simple values.
- Reference types: which refer to objects and any instance of a class, typically more complex than primitive types.
The importance of understanding variables and data types lies in how they control memory usage and performance efficiency. A solid grasp of these concepts can lead to better programming practices, and ultimately, more effective code.
Control Flow Statements
Control flow statements dictate the direction the program takes during execution. They allow the program to make decisions based on conditions, leading to dynamic behavior. Understanding these statements is critical in shaping logic within Java applications. There are three primary types:
If-Else Statements
If-else statements allow for conditional execution of code blocks. They evaluate boolean expressions and determine which path of code to follow based on whether those expressions are true or false.
A key characteristic of if-else statements is their flexibility. They can handle complex decision-making scenarios by nesting multiple conditions. This makes them beneficial for many programming tasks, such as user input validation.
One unique feature of if-else statements is their simplicity in syntax. This makes them accessible for beginners. However, a potential disadvantage is that deeply nested if-else statements can lead to convoluted code, which may be hard to read and maintain.
Switch Cases
Switch cases are another form of control flow that offers a more structured way to handle multiple potential outcomes based on a single variable.
The key characteristic of switch cases is that they can replace long chains of if-else statements with cleaner requests. This readability makes them advantageous in scenarios where a variable can assume many values. They also allow adding new cases easily without modifying existing logic significantly.
However, there are drawbacks. Switch cases only work with certain data types, such as or . This limitation can hinder their use when dealing with complex data. Additionally, without a default case, missing options can lead to unexpected behavior.
Loops: For, While, Do-While
Loops enable repetitive execution of code, which is vital for tasks that involve iterating through data collections or performing actions until certain conditions are met.
The key feature of loops is their efficiency in executing code repeatedly without the need for redundancy. For instance, the for loop is ideal for iterating over arrays, while while and do-while loops are useful for repeating an action while a condition is true.
Each loop has its advantages. The for loop provides a compact syntax, making it easy to manage counters, while while loops afford more flexibility by allowing conditional checks before entering the loop. However, improper management of loops can lead to infinite loops, a common pitfall in programming that can crash the system. Thus, understanding these control flow statements enhances overall programming proficiency in Java.
"Control flow statements in Java allow you to dictate the logic and functionality of your programs effectively, making them essential elements in programming."
Overall, mastering these core Java concepts equips learners with essential skills that will serve as a foundation for more advanced topics in Java programming.
Object-Oriented Programming in Java
Object-oriented programming (OOP) is a fundamental paradigm in Java. It helps developers organize code through the use of objects, which are instances of classes. This approach not only enhances code reusability but also promotes clear and maintainable systems. Java employs OOP principles such as encapsulation, inheritance, and polymorphism. Each of these concepts plays a significant role in how Java is structured, making it more efficient and accessible for both new and experienced programmers.
OOP is essential in Java for various reasons. It allows for a better modeling of real-world problems, making it easier to understand and relate to code. Furthermore, OOP optimizes code organization, making it easier to locate and fix issues, and it fosters collaborative programming by allowing different parts of a system to be developed independently. Overall, OOP contributes to a more structured and logical approach to coding, which is highly beneficial for creating robust applications.
Classes and Objects
Defining Classes
Defining classes is a cornerstone of object-oriented programming in Java. A class serves as a blueprint for creating objects. This blueprint specifies the attributes and behaviors that the objects will possess. A main characteristic of a class is its ability to encapsulate data and methods, providing a clear structure to the codebase. This encapsulation makes it easier to manage complex codebases by grouping related functionalities and data together.
The popularity of defining classes in Java comes from the benefits they offer. One key advantage is the ease of maintenance. Changes made to a class propagate to all its instances, allowing for manageable and consistent updates across the application. However, an important consideration is ensuring that classes are defined with proper clarity and purpose. Poorly structured classes can lead to confusion and difficulties in debugging.
Unique features of defining classes include access modifiers. They dictate the visibility of the class and its members, allowing developers to implement stricter controls over how data is accessed and manipulated. While this adds a layer of security, it requires careful planning of how classes interact with one another.
Creating Objects
Creating objects is the next logical step after defining classes. In Java, objects represent real-world entities and are crucial in encapsulating state and behavior. The process of creating an object involves calling the constructor of the class, which initializes the object. A crucial aspect of object creation is that each object has its own unique state, allowing multiple instances of a class to operate independently.
The key characteristic of creating objects is that they bring classes to life. They allow developers to utilize the functionalities defined in the class while maintaining the individuality of each instance. This is particularly beneficial in applications where multiple similar objects are required, such as user profiles in a social media platform.
A unique feature of object creation is its impact on memory management. Each object occupies memory, and understanding how to efficiently manage object lifecycles is essential for performance. Java's garbage collection mechanism helps manage memory automatically, reducing the risk of memory leaks. However, unnecessary object creation should be avoided as it can lead to performance overhead.
Inheritance and Polymorphism
Inheritance and polymorphism are two fundamental concepts in Java's object-oriented model. Inheritance allows a new class to inherit properties and methods from an existing class. This not only promotes code reuse but also establishes a natural hierarchical relationship between classes. For example, a class could serve as a parent class for and subclasses, each inheriting general bird traits while implementing specific behavior.
Polymorphism enables methods to perform different tasks based on the objects calling them. This aspect supports flexible code and contributes to cleaner designs. When combined with inheritance, polymorphism allows for defining a single interface with multiple implementations, fostering ease of code maintenance and scalability.
Advanced Java Concepts
The section on Advanced Java Concepts is crucial in understanding the depth and flexibility of the Java programming language. Mastery of these concepts is essential for developers aiming to create complex applications and systems. This segment explores critical components such as Exception Handling and the Java Collections Framework, both of which significantly enhance a programmer’s ability to manage errors and data structures efficiently.
Exception Handling
Try-Catch Blocks
Try-catch blocks are a fundamental part of Java’s error handling mechanism. They allow developers to anticipate potential runtime errors and manage them gracefully. The key characteristic of try-catch blocks is their structure, which separates normal code execution from error handling code. This makes it a beneficial choice for this article as it illustrates a proactive approach to programming, ensuring more robust applications.
A unique feature of try-catch blocks is that they let a programmer continue execution after an error occurs, rather than terminating the program. This advantage minimizes disruptions, ensuring a smoother user experience. However, excessive use of try-catch blocks can lead to code that is harder to read and maintain.
Throwing Exceptions
Throwing exceptions is a key mechanism in Java for indicating that a problem has occurred during program execution. It contributes significantly to error management by informing the program to stop executing at a point where an issue arises. The main characteristic of throwing exceptions is its ability to propagate errors up the call stack, giving higher-level methods the chance to handle them.
This method is beneficial because it promotes clean code and separation of concerns. However, developers must use it wisely. Poorly managed exceptions can lead to lost error information or overly complex code structure, which complicates debugging and error tracing.
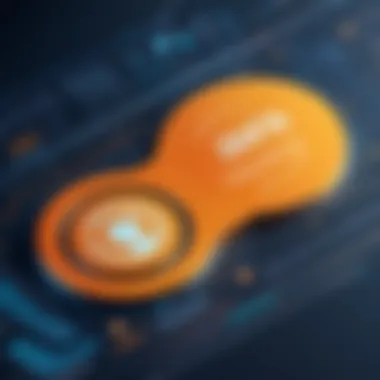
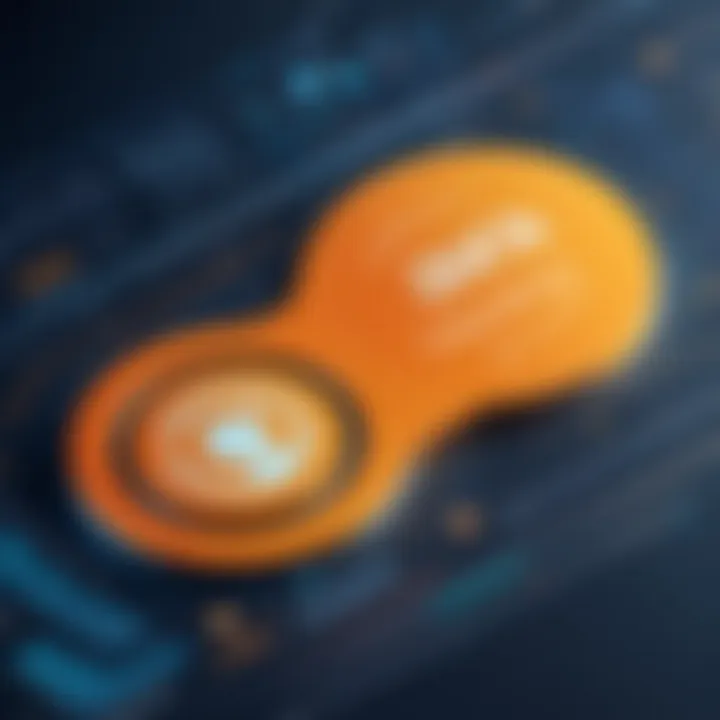
Java Collections Framework
Overview of Collections
The Overview of Collections presents a structured way to manage groups of objects. Collections in Java are significant for their efficiency and ease of use. The key characteristic of the Java Collections Framework is its broad set of interfaces and classes that facilitate data management, including lists, sets, and maps. This framework is crucial for this article as it enhances developers’ skills in handling data dynamically.
The unique aspect of using the Collections Framework is that it abstracts the underlying implementations, allowing developers to focus on the higher-level design of their applications. This design makes handling data simpler, although the complexity of the framework can sometimes be a disadvantage for beginners.
Using Lists, Sets, and Maps
Using Lists, Sets, and Maps is essential to effectively work with data. Each of these structures serves a distinct purpose, making it easier to organize and manage information in Java applications. The key characteristics are:
- Lists: Ordered collections that allow duplicates, useful for scenarios where order matters.
- Sets: Unordered collections that do not allow duplicates, ideal for ensuring uniqueness.
- Maps: Key-value pairs that enable quick lookups based on keys.
This approach is beneficial because it provides flexibility in how data is stored, accessed, and manipulated. However, choosing the wrong collection type can lead to performance issues, making it crucial to understand when to use each type.
"Understanding the Java Collections Framework is essential for writing effective and optimized Java applications."
Practical Applications of Java
Understanding the practical applications of Java is essential for students and aspiring programmers. Java is a versatile language used in many areas, including web development, mobile applications, and enterprise solutions. Its robust features and platform independence make it a popular choice across various industries. By recognizing how Java can be applied in real-world scenarios, learners can better appreciate its significance and utility, thus motivating them to delve deeper into mastering this programming language.
Java in Web Development
Java plays a crucial role in web development. Several web frameworks utilize Java, including Spring, Hibernate, and JavaServer Faces. These frameworks facilitate the creation of dynamic and robust web applications. The popularity of Java in this domain stems from its object-oriented principles and security features. With Java, developers can create scalable and maintainable web applications while ensuring a high level of security.
Moreover, many content management systems, such as Apache Roller, are built on Java, providing additional functionality for managing web content efficiently. The Java Virtual Machine (JVM) enables web applications to run on any platform, making it a well-suited choice for developers aiming for cross-platform compatibility.
In summary, Java’s extensive libraries and frameworks simplify web development processes, allowing programmers to focus on creating innovative solutions.
Java for Mobile Applications
Java's impact on mobile app development cannot be overstated. As the primary language for Android development, Java provides a stable and efficient environment for building high-performing mobile applications. Android Studio, the official integrated development environment for Android, utilizes Java extensively.
The language allows developers to access a vast array of tools and APIs designed specifically for mobile development. This robust environment enables the seamless creation of UI/UX elements, allowing for optimized user engagement.
Furthermore, Java's exception handling and automatic memory management are advantageous in mobile application development. These features ensure that the apps are not only performant but also less prone to crashes, enhancing user experience.
Common Pitfalls in Java Programming
Addressing common pitfalls in Java programming is critical for learners aiming to enhance their coding skills. Understanding these issues not only minimizes frustration but also fosters a more effective learning experience. Java, while being user-friendly when comprehended fully, has its share of complexities that can trip up novice programmers. Thus, focusing on these pitfalls aids in refining coding practices, leading to better program performance and reliability.
Memory Management Issues
Memory management is a vital aspect of Java that programmers cannot afford to overlook. Although Java utilizes automatic garbage collection to manage memory, improper handling of objects can still lead to performance degradation. One of the primary memory management issues is memory leaks. A memory leak occurs when the programmer unintentionally retains references to objects that are no longer needed. Even though these objects are unreachable, they continue occupying memory, thereby causing waste.
To minimize this risk, it's essential to:
- Carefully assess object references.
- Nullify references when they are no longer needed.
Another common issue is the excessive creation of temporary objects, which can lead to additional overhead. In performance-sensitive applications, this can be detrimental.
Overall, understanding and proactively addressing memory management issues is crucial. Solid knowledge in this area of Java programming will ensure efficient memory usage and improved application performance.
Debugging Java Applications
Debugging is an essential skill for any programmer, and Java is no exception. The debugging process can become cumbersome without the right tools or techniques. Java provides a range of built-in debugging tools that are essential for identifying bugs and optimizing code.
A common pitfall in debugging is overlooking the fundamental aspects of code execution. For instance, ignoring the Java stack trace can lead to misunderstandings regarding where an error originates. Monitoring trace outputs can give insight into the flow of the program that leads to exceptions or other issues.
When debugging, consider employing techniques such as:
- System.out.println() for simple output checks.
- Utilizing Java Debugger (JDB) to step through code.
- Integrating IDE debug tools, which provide breakpoints and variable inspections.
By ensuring these tools and techniques are part of the debugging process, programmers reduce the time spent tracing bugs. Learning to navigate through common debugging traps will foster a more robust understanding of Java, ultimately benefitting the learning journey.
"Understanding common pitfalls puts you on a faster track to becoming proficient in Java programming."
Through awareness of memory management issues and effective debugging practices, learners can avoid the usual traps that hinder their progress and enhance their coding capabilities in Java.
Resources for Further Learning
In the landscape of programming, the journey of learning does not have a fixed endpoint. Resources for further learning are crucial elements in the acquisition of knowledge and skill enhancement. They serve as guides, offering insights into complex topics, providing updated information, and facilitating self-paced learning. In this article, we will explore the significance of such resources, focusing on books and online courses.
Utilizing a diverse set of learning materials can enrich your understanding of Java. Each resource offers unique perspectives and techniques for grasping core concepts and advanced features. Readers should consider their preferred learning style—whether they thrive in structured environments or prefer self-directed exploration—and seek resources accordingly.
Books on Java Programming
Books remain a reliable source for in-depth understanding of Java. They often provide comprehensive coverage of subjects, including theory and practical examples. Individual titles may focus on fundamentals, advanced concepts, or specific frameworks, catering to various levels of expertise. Below are some impactful books worth considering for Java programming:
- "Effective Java" by Joshua Bloch: This book is known for its solid advice and best practices, beneficial for both beginners and experienced programmers.
- "Java: The Complete Reference" by Herbert Schildt: This extensive guide covers everything from basic syntax to advanced features, making it a valuable resource.
- "Head First Java" by Kathy Sierra and Bert Bates: Through its distinctive teaching style and visuals, this book makes complex concepts accessible to newcomers.
Books allow for deep dives into topics at one’s own pace. They also offer additional exercises and reference material for revision and practice, further augmenting the learner's journey.
Online Courses and Tutorials
Online courses and tutorials provide a more interactive way to learn Java. They often combine video lectures, quizzes, and hands-on projects, enhancing engagement and retention. Platforms such as Coursera, Udemy, and edX offer structured courses tailored to different skill levels. Here are some popular online resources worth exploring:
- Coursera's "Java Programming and Software Engineering Fundamentals": This specialization contains several courses covering essential programming concepts and best practices in Java.
- Udemy's "Java Masterclass: Become a Superheroo Java Programmer": A comprehensive course marketed to complete beginners that progresses to advanced topics.
- edX's "Introduction to Java Programming": Offered by reputable institutions, this course provides foundational knowledge and hands-on experience.
With online courses, learners often benefit from community forums and direct feedback from instructors. This interactive element can significantly enhance comprehension and problem-solving skills.
Engaging with a variety of resources is essential. Each book, course, or tutorial can bring different insights, making the learning process rich and diverse.