Mastering Java Spring: A Comprehensive Guide for Building Enterprise Applications
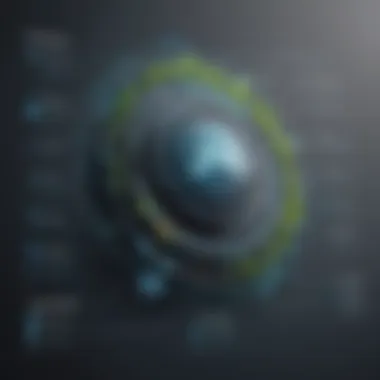
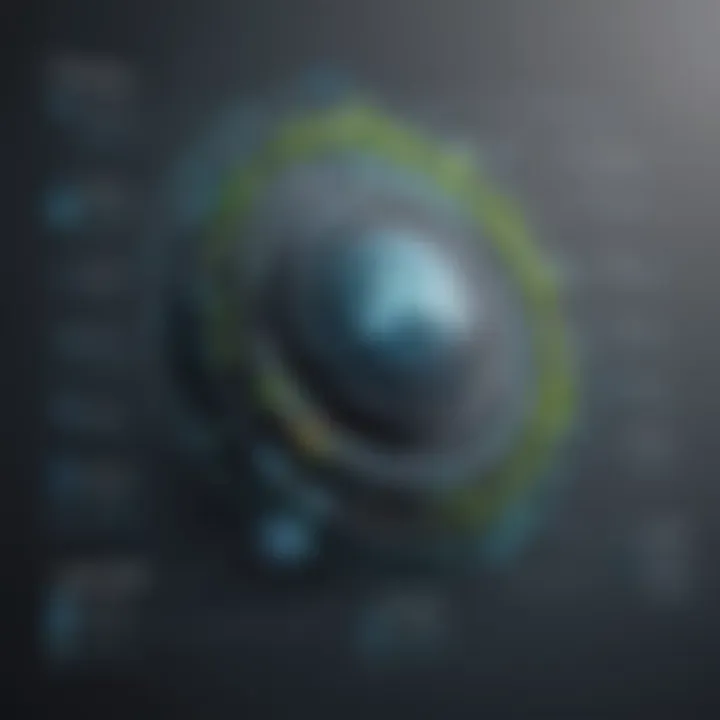
Introduction to Programming Language
Java Spring, a powerful framework for developing enterprise applications, has garnered significant attention in the programming world. With its roots traced back to 2003, Java Spring offers a robust platform for creating scalable and reliable software solutions. Its versatile nature caters to a wide range of development needs, making it a popular choice among developers worldwide.
Basic Syntax and Concepts
In Java Spring, mastering the basics is crucial for a solid foundation in programming. Understanding variables and data types lay the groundwork for efficient data management. Knowledge of operators and expressions enhances code readability and functionality. Control structures like loops and conditional statements dictate program flow, ensuring smooth execution of tasks.
Advanced Topics
Delving deeper into Java Spring unveils a realm of advanced topics essential for comprehensive expertise. Functions and methods streamline code organization and promote reusability. Object-Oriented Programming (OOP) principles form the core of Java Spring development, emphasizing encapsulation, inheritance, and polymorphism. Exception handling equips developers with the ability to manage errors gracefully, enhancing the robustness of applications.
Hands-On Examples
Practical application of Java Spring concepts through hands-on examples accelerates learning. Simple programs help grasp fundamental concepts, while intermediate projects provide exposure to real-world scenarios. Code snippets offer quick solutions to common programming challenges, aiding in developing efficient and optimized code.
Resources and Further Learning
To continue mastering Java Spring, utilizing resources is paramount. Recommended books and tutorials offer in-depth insights and practical exercises for skill enhancement. Online courses and platforms provide comprehensive learning modules tailored to different proficiency levels. Community forums and groups foster collaboration and knowledge sharing within the Java Spring development community.
Introduction to Java Spring
Java Spring is a crucial topic that individuals delving into the realm of enterprise-level application development must grasp. This section serves as the bedrock for understanding the intricacies of Java Spring. It acts as the gateway for beginners and intermediate learners, offering insights into the fundamental concepts that underpin this robust framework.
Understanding the Basics
Overview of Java Spring Framework
Exploring the Java Spring framework provides a profound insight into its architecture and functionalities, making it an indispensable tool for modern application development. The framework's focus on dependency injection and aspect-oriented programming sets it apart from traditional approaches, enhancing modularity and scalability. Embracing the essence of loose coupling and high cohesion, Java Spring empowers developers to create robust and maintainable applications effortlessly.
Importance of Java Spring in Application Development
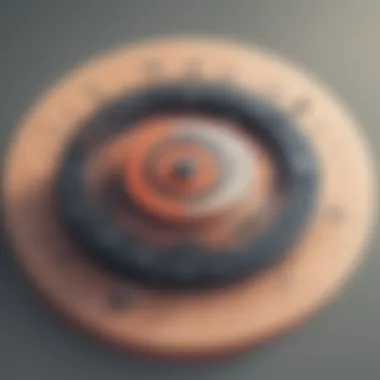
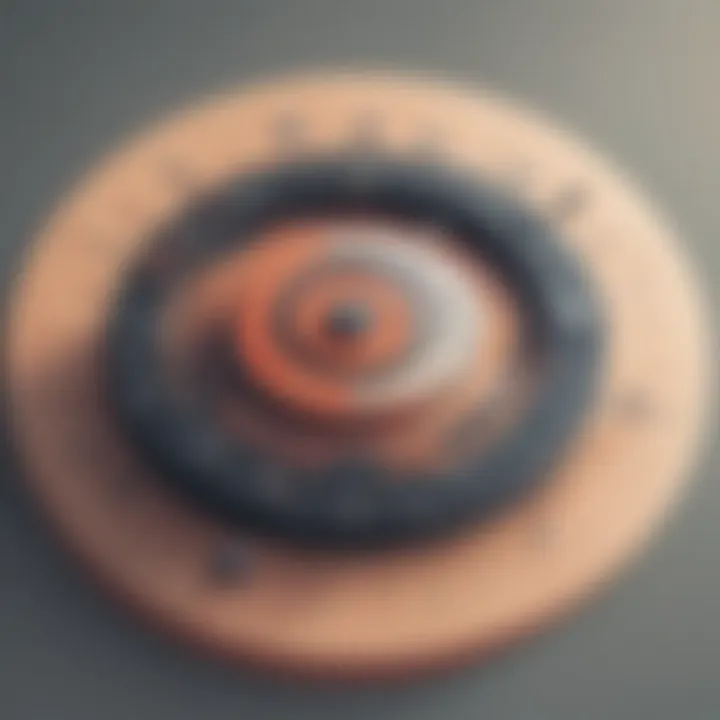
Understanding the significance of Java Spring in the realm of application development is paramount. Java Spring offers a comprehensive solution for addressing challenges related to enterprise application complexities, facilitating agile development and seamless integration. Its versatility in handling diverse aspects such as security, data access, and microservices development underscores its relevance in modern software engineering practices.
Setting Up the Development Environment
Installing Java Development Kit (JDK)
The installation of the Java Development Kit (JDK) marks the initial step in preparing the development environment for Java Spring. By incorporating the JDK, developers gain access to essential tools and libraries required for Java application development. Setting up the JDK ensures compatibility with various operating systems and ensures a seamless development experience.
Configuring Integrated Development Environment (IDE)
Configuring the Integrated Development Environment (IDE) is pivotal in optimizing the development workflow for Java Spring projects. Integrating the IDE with necessary plugins and extensions streamlines coding, debugging, and testing processes. Selecting an IDE that aligns with the project requirements and developer preferences enhances productivity and facilitates collaborative development efforts.
Core Concepts of Java Spring
In the landscape of Java Spring, mastering the core concepts is paramount for aspiring developers. This section delves into the fundamental pillars that uphold the framework, serving as the bedrock for sophisticated application development. Understanding the essence of Dependency Injection and Aspect-Oriented Programming is pivotal for harnessing the full potential of Java Spring. From simplifying complex structures to enhancing code modularity, these concepts offer a paradigm shift in software design.
Dependency Injection
Understanding Inversion of Control (IoC)
At the core of Dependency Injection lies the principle of Inversion of Control (IoC), a revolutionary paradigm altering how components interact within an application. IoC shifts the control of object creation and management from the objects themselves to an external entity, promoting loose coupling and increased flexibility. This mechanism allows developers to achieve greater separation of concerns, facilitating maintenance and extensibility in Java Spring applications. Embracing IoC empowers programmers to focus on business logic without being entangled in object instantiation details, streamlining development processes.
Implementing Dependency Injection in Java Spring
Implementing Dependency Injection in Java Spring entails integrating external dependencies into a component, enhancing reusability and testability. By decoupling components and their dependencies, developers can dynamically inject collaborating objects, promoting scalability and enhancing code readability. Java Spring's dependency injection container simplifies object wiring, enabling seamless interaction between disparate modules. This approach fosters modular design, promoting easier integration of new functionalities and facilitating unit testing for robust application development.
Aspect-Oriented Programming (AOP)
Exploring AOP Concepts in Java Spring
Diving into Aspect-Oriented Programming unveils a novel way to address cross-cutting concerns in Java Spring applications. AOP enables developers to encapsulate cross-cutting functionalities into aspects, eliminating code duplication and enhancing maintainability. By abstracting common functionalities such as logging, security, and transaction management, developers can streamline code organization and reduce redundancy. Leveraging AOP in Java Spring empowers developers to modularize concerns that span multiple modules, improving code maintainability and promoting reusability across diverse components.
Applying AOP in Cross-Cutting Concerns
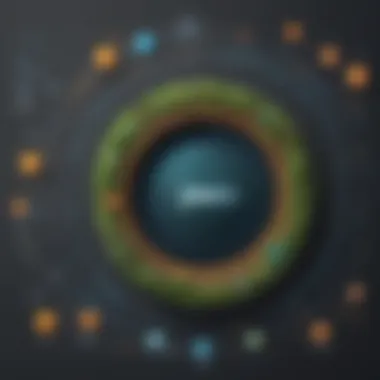
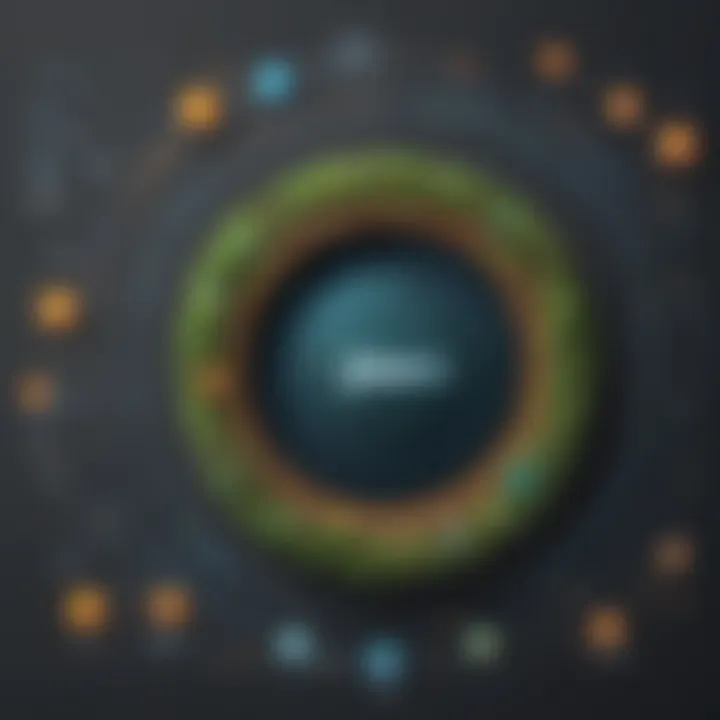
Applying AOP in addressing cross-cutting concerns revolutionizes the way developers handle shared functionalities in Java Spring applications. By weaving aspects into the existing codebase, programmers can achieve a more cohesive and structured approach to managing common features. This methodology enhances the separation of concerns, allowing developers to focus on business logic while abstracting cross-cutting functionalities into reusable aspects. Through AOP, Java Spring facilitates a cleaner and more efficient codebase, enhancing scalability and promoting agile development practices.
Working with Java Spring Components
When delving into the realm of mastering Java Spring, understanding the core components is paramount. Working with Java Spring Components is a crucial aspect covered in this article to equip readers with the knowledge and skills necessary for developing robust enterprise applications. This section addresses pivotal elements such as Controllers, Views, Data Access, and more.
Controllers and Views
Creating Controllers in Java Spring
Exploring the creation of Controllers in Java Spring sheds light on how these components serve as the backbone of application logic. Controllers act as intermediaries between user input and application functionality, enabling seamless interaction within the software. Leveraging Java Spring's capabilities in creating Controllers ensures clean, organized code structure and facilitates the implementation of business logic effectively. The innate flexibility of Controllers in Java Spring allows for easy integration with various view technologies and data sources, making them a preferred choice for this guide.
Rendering Views using Thymeleaf
Incorporating Thymeleaf for rendering views amplifies the user interface experience in Java Spring applications. Thymeleaf, with its elegant and natural templates, simplifies the process of embedding dynamic content in web pages. Rendering Views using Thymeleaf adds a layer of abstraction that enhances code readability and maintainability. The seamless integration of Thymeleaf with Java Spring empowers developers to create visually appealing and interactive user interfaces efficiently. While the learning curve may exist, the benefits of using Thymeleaf for view rendering in this context outweigh any initial challenges.
Data Access with Spring Data
Configuring Data Sources
Efficient data management is critical in enterprise applications, underscoring the significance of configuring data sources in Java Spring. The configuration of data sources facilitates seamless connectivity with databases, ensuring data retrieval and manipulation occur smoothly. Java.
Performing CRUD Operations with Spring Data JPA
Administering Create, Read, Update, and Delete (CRUD) operations forms the backbone of database interactions in Java Spring applications. Spring Data JPA simplifies the implementation of these operations, offering a streamlined approach to database management. Executing CRUD operations with Spring Data JPA enhances the efficiency of data handling, promoting scalability and maintaining consistency within the application landscape.
Advanced Topics in Java Spring
In this section, we delve into the crucial Advanced Topics in Java Spring, which is pivotal for mastering this comprehensive guide. Advanced topics play a significant role in enhancing developers' skills and knowledge, especially in building complex enterprise applications. By understanding and implementing advanced concepts, developers can elevate their applications' performance, security, and scalability. Furthermore, diving into advanced topics equips learners with the necessary expertise to tackle real-world application challenges effectively.
Security in Spring Applications
Implementing Authentication and Authorization:
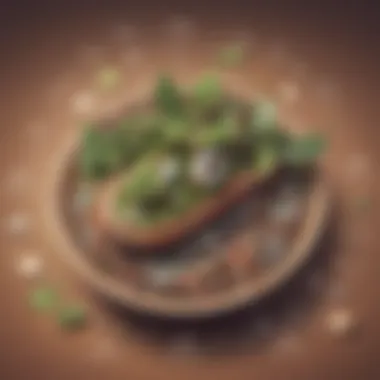
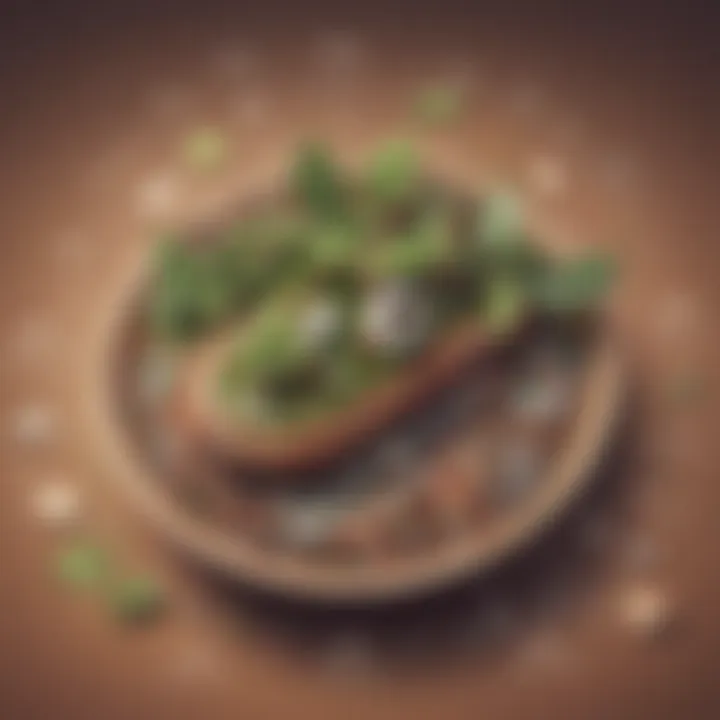
The Implementation of Authentication and Authorization in Spring Applications is a critical aspect that ensures the security and integrity of an application. By incorporating robust authentication mechanisms, developers can validate users' identities and control access to sensitive resources effectively. Authentication verifies the user's identity, while authorization determines the permissions and privileges granted to them. Utilizing these security measures enhances the overall security posture of the application, safeguarding it against unauthorized access and potential threats. Implementing Authentication and Authorization is indispensable in developing secure and reliable applications, making it a foundational element in Java Spring development.
Securing RESTful APIs with Spring Security:
Securing RESTful APIs with Spring Security is imperative in ensuring the confidentiality, integrity, and availability of data transactions over APIs. By leveraging Spring Security's robust features, developers can implement authentication, authorization, and encryption mechanisms to protect sensitive data exchanged between client and server. This helps prevent unauthorized access, data breaches, and man-in-the-middle attacks, ensuring the seamless and secure operation of RESTful APIs. Furthermore, Spring Security provides flexibility in configuring security policies and protocols, allowing developers to tailor security measures according to the application's specific requirements and compliance standards. Overall, incorporating Spring Security in API development adds an extra layer of protection, instilling trust and reliability in data communication.
Microservices Development
Designing Microservices Architecture:
Designing a Microservices Architecture is a fundamental aspect of modern application development, emphasizing modularity, scalability, and resilience. By decomposing monolithic applications into independent microservices, developers can achieve greater flexibility, agility, and maintainability in their architectures. Microservices facilitate independent deployment, scaling, and management of services, enabling seamless integration and coordination across the application ecosystem. The key characteristic of Microservices Architecture lies in its decentralized nature, allowing teams to work on different services concurrently while ensuring looser coupling and high cohesion. However, designing Microservices Architecture requires careful consideration of communication protocols, data consistency, and fault tolerance mechanisms to guarantee the overall system's robustness and reliability.
Inter-Service Communication with Spring Cloud:
Inter-Service Communication with Spring Cloud plays a pivotal role in orchestrating communication and coordination between microservices within a distributed system. Spring Cloud provides a comprehensive toolkit for implementing service discovery, load balancing, fault tolerance, and distributed configuration, easing the development and management of microservices-based applications. By leveraging Spring Cloud's capabilities, developers can seamlessly integrate microservices, establish resilient communication pathways, and ensure efficient service interactions. Additionally, Spring Cloud facilitates the implementation of cross-cutting concerns such as logging, monitoring, and security across services, promoting consistent operational practices and enhancing system observability. However, developers need to carefully design communication patterns and error-handling mechanisms to mitigate potential bottlenecks and failures in inter-service communication, ensuring the overall stability and performance of the microservices architecture.
Best Practices and Tips
In the expansive realm of Java Spring, explicit attention to best practices and tips is elemental for the proficiency of developers. It serves as the quintessential compass in navigating the complexities of Java Spring towards optimal outcomes. Understanding and implementing best practices and tips not only streamline development processes but also enhance the robustness and scalability of applications. Engaging with best practices ensures code readability, maintainability, and efficiency, leading to the creation of high-quality software solutions. For beginners and intermediates venturing into the intricacies of Java Spring, adherence to these guiding principles can be transformative, fostering a deep comprehension of coding standards and architecture design paradigms.
Code Optimization
Writing Efficient and Maintainable Code
Within the domain of Java Spring, writing efficient and maintainable code stands as a pivotal pillar for software development excellence. This facet emphasizes the importance of crafting code that is not only performant but also easily comprehensible and modifiable. The cornerstone of efficient code lies in its ability to execute tasks swiftly while maintaining a clear structure that promotes code reuse and extensibility. By adhering to best practices such as meaningful variable naming, modular design, and efficient algorithm usage, developers can optimize their codebase for enhanced performance and readability. Writing efficient and maintainable code not only accelerates development cycles but also minimizes the risk of errors and bugs, fostering a more stable application ecosystem. Embracing the ethos of writing efficient and maintainable code equips developers with the tools to create software solutions that are agile, scalable, and future-proof.
Utilizing Java Spring Annotations
In the Java Spring landscape, leveraging annotations serves as a potent mechanism for simplifying configuration and enhancing the flexibility of applications. Java Spring annotations offer a concise and declarative approach to define components, dependencies, and behaviors within the application context. By incorporating annotations such as @Autowired, @Component, and @RestController, developers can streamline configuration processes, reduce boilerplate code, and improve the overall readability of their codebase. The intrinsic beauty of Java Spring annotations lies in their ability to encapsulate complex functionalities into simple declarations, providing a more intuitive and streamlined development experience. By harnessing the power of annotations, developers can expedite development workflows, facilitate code maintenance, and adhere to best practices in Java Spring architecture design.
Testing Strategies
Unit Testing with JUnit
Unit testing with JUnit embodies a cornerstone in the Java Spring development cycle, ensuring the individual components of an application function as intended. By dissecting application modules into isolated units and validating their behaviors through automated tests, developers can detect bugs early in the development process and safeguard the integrity of their codebase. JUnit, with its rich suite of assertion methods and testing annotations, empowers developers to write robust test cases that validate the functionality of individual units in isolation. Adopting unit testing practices with JUnit not only enhances code quality and reliability but also instills confidence in the application's behavior, paving the way for seamless integration and deployment cycles.
Integration Testing with Spring Test
Integration testing with Spring Test epitomizes the amalgamation of various application components to verify their collaboration and interoperability. By testing the integration of individual units, services, and data sources within the application context, developers can ensure that the system functions harmoniously as a whole. Spring Test provides a comprehensive suite of tools and utilities for setting up application contexts, managing transactions, and executing integration tests across different layers of the application stack. This meticulous testing approach validates the end-to-end functionality of the application, identifying potential integration issues and bottlenecks before they manifest in production environments. Embracing integration testing with Spring Test fortifies the robustness and reliability of Java Spring applications, affirming their resilience in dynamic enterprise landscapes.