Mastering Java Collections: A Comprehensive Guide
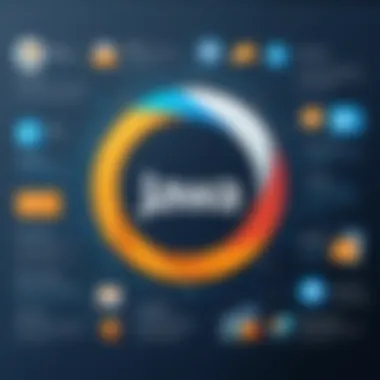
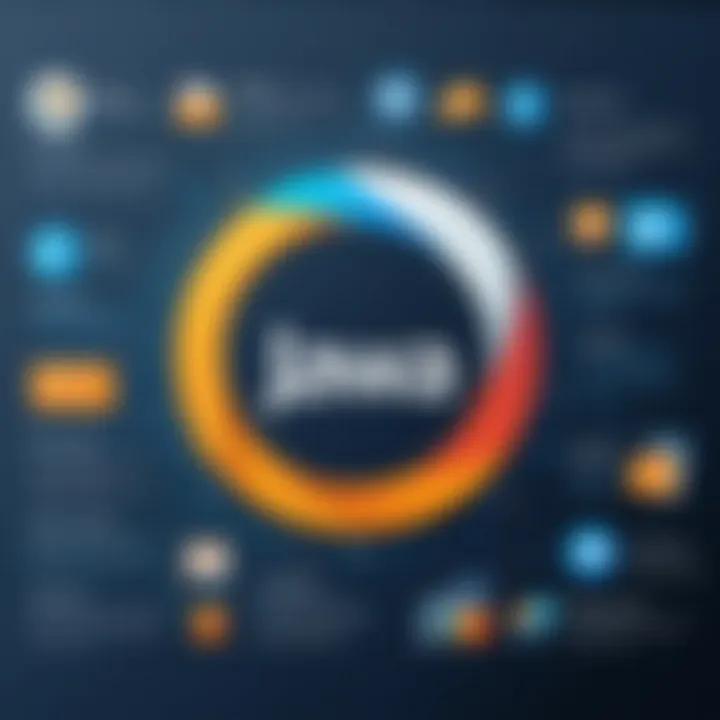
Intro
Collections in Java are a corner stone when it comes to managing groups of objects. At its core, Java Collections provide a way for programmers to handle data in an organized manner. From managing simple lists to complex data structures, the collection framework is designed to optimize performance and usability.
Understanding collections isn’t just about knowing how to use them; it’s about grasping their significance in writing effective code. They can make the difference between a program that runs like a well-oiled machine and one that’s bogged down by inefficiencies. This exploration covers the core interfaces, types, practical implementations, and best practices for navigating Java collections, catering to both newcomers and those with more experience looking to deepen their knowledge.
"The man who moves a mountain begins by carrying away small stones." — Confucius.
To unpack the nuances of collections, it helps to start from a solid foundation. Hence, let’s delve into what makes Java the preferred choice for many programmers, not just in the realm of data structures but as a language overall.
Preface to Programming Language
History and Background
Java, first released in 1995 by Sun Microsystems, was developed with a clear mantra: "Write Once, Run Anywhere." This principle is integral to its appeal, allowing code to be executed on various platforms without modification. Java’s syntax shares similarities with C++, making it accessible for many developers familiar with those languages.
Features and Uses
Java shines through its object-oriented design, enabling programmers to create modular and reusable code. It offers several features like:
- Platform independence: Java applications can run on any device with a Java Virtual Machine (JVM).
- Automatic memory management: Java handles memory allocation and deallocation automatically, reducing the burden on developers.
- Rich standard library: It includes a variety of classes and interfaces, especially in the Collections framework.
Java is widely used in various domains, from enterprise applications to mobile apps. Its stability and security make it a popular choice for large-scale systems.
Popularity and Scope
The popularity of Java remains high, evidenced by its presence in various programming communities. It's not just a language confined to academic settings; Java finds applications in backend development, cloud computing, and scientific computing. With a supportive community and vast resources for learning, it serves as a useful tool for anyone embarking on software development.
Basic Concepts of Collections
Core Interfaces
Java collections revolve around several key interfaces:
- Collection: The root interface from which different collections inherit.
- List: An ordered collection that can contain duplicates. Implementations include ArrayList and LinkedList.
- Set: A collection that does not allow duplicates. HashSet is a popular implementation here.
- Map: A collection of key-value pairs, where keys are unique. HashMap is a commonly used class in this category.
Understanding these interfaces is essential for mastering collections, as they dictate how data is stored and accessed.
Practical Implementations
When using collections, knowing the implementation can make a significant impact on performance. For example, if you need to frequently access elements, ArrayList might be your go-to. Conversely, if insertion and removal operations are more common, LinkedList may serve you better.
Using generics—specifically the diamond operator—can enhance type safety and clarity. For instance,
This explicitly indicates that the list will only hold String objects.
Performance Characteristics
Performance should dictate your choice among collections. Certain collections come with distinct trade-offs. Lists are generally faster for accessing elements, while Sets and Maps offer faster search capabilities for large data sets.
Best Practices for Effective Usage
To ensure you harness the full potential of Java collections:
- Choose the right type of collection based on your needs.
- Regularly assess your data access patterns.
- Leverage Java's built-in methods for common tasks rather than reinventing the wheel.
Resources and Further Learning
To wrap up, enriching your knowledge of Java collections can be greatly enhanced by external resources:
- Recommended Books: "Effective Java" by Joshua Bloch and "Java: The Complete Reference" by Herbert Schildt.
- Online Courses: Platforms like Coursera and Udemy offer comprehensive courses tailored to Java Collections.
- Community Forums: Joining conversations on Reddit or Facebook groups focused on Java can provide additional insights and foster collaboration.
Mastering Java Collections isn ’t just an exercise in programming; it’s about enhancing problem-solving skills. The more you understand these structures, the better you can write efficient and maintainable code.
Intro to Collections in Java
In programming, having the right tools can make a world of difference. Collections in Java are a key part of this toolkit. They allow developers to manage groups of objects with ease and efficiency. Understanding how to use collections is not just beneficial; it's essential for writing clean, maintainable code.
Collections in Java are interfaces and classes that help us store, manipulate, and retrieve data. Think of them as organized boxes where we can keep our toys—sometimes we want them sorted neatly, sometimes we just need to dump them all in. The way collections are designed allows for a variety of functionalities, which suit different programming needs.
Whether you're developing a small application or a large software system, knowing how to work with collections means saving time and resources. A clear focus on these data structures can lead to more efficient algorithms and better memory management, which in turn results in smoother application performance.
What are Collections?
At the core, collections are data structures provided by the Java Collections Framework. They offer a way to treat multiple objects as a single unit. Let's break this down:
- Definition: A collection in Java is an object that groups multiple elements, whether they're numbers, strings, or even other objects.
- Types: There are several types of collections, each serving different purposes, like Lists, Sets, and Maps.
- Flexibility: Collections can dynamically change their size, unlike arrays, which have fixed lengths.
- Interfaces: Collections are often worked with through interfaces, allowing for different implementations, like ArrayLists or HashSets, providing flexibility in code.
The powerful part of collections is their versatility. If you think of data management as handling different sizes of data boxes, collections allow you to pick the right box for the task. Moreover, Java collections support techniques like sorting and searching, which make them indispensable for programmers.
Importance of Collections in Java
Collections are a fundamental aspect of Java programming. Here’s why:
- Efficiency: Using collections can optimize algorithms. For instance, when you need to store unique values, a HashSet is far more efficient than using an ArrayList.
- Ease of Use: Java collections provide built-in methods for common operations. You don’t have to reinvent the wheel for things like sorting or searching.
- Code Readability: By utilizing collections appropriately, your code becomes more understandable. Future maintainers of your code will thank you when they see well-structured collection usage.
- Data Handling: Working with data in bulk or in a specific order becomes a breeze. Collections allow you to focus on what you want to do with the data instead of how it's stored.
- Integration: Collections integrate seamlessly with other Java utilities, like the Stream API, enabling functional programming paradigms that can make data processing even easier.
As we dive deeper into the topic, this article will unpack the various interfaces that make up the Collections Framework. Understanding these key elements is not just for academic knowledge, but a stepping stone to becoming proficient in Java programming.
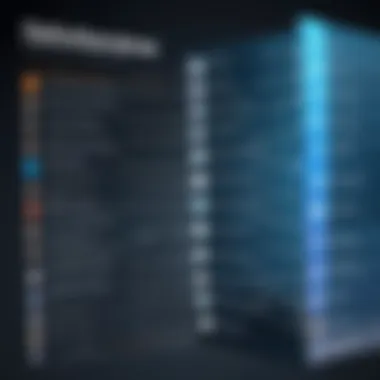
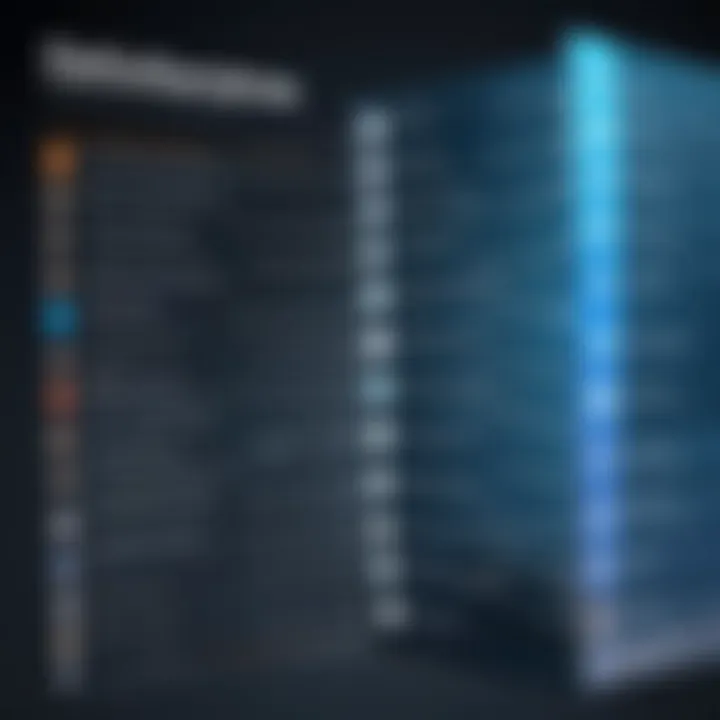
"The only way to do great work is to love what you do." - Steve Jobs
Understanding collections represents a love for efficient, effective coding. Let's embark on this learning journey to master collections in Java.
Core Interfaces of Java Collections
A deep dive into Java collections requires a solid grasp of the core interfaces that form the backbone of this vital framework. These interfaces serve as blueprints for various data structures, guiding developers in selecting the right tool for their needs. A well-rounded understanding of these core interfaces not only enhances code efficiency but also fosters better coding practices.
List Interface
The List interface is a fundamental part of Java’s collections framework, which allows the storage of an ordered collection of elements. Lists are also great for accessing and manipulating elements based on their indices, making them vital in numerous applications.
Consider your typical use case: you need to maintain a sequence of items, maybe a list of names or a row of scores in a game. Here, and are the primary implementations of the List interface. The provides fast random access to elements thanks to its underlying array structure, while the excels in scenarios with extensive insertions and deletions.
Key points to consider when using the List interface include:
- Order of Elements: The order in which elements are inserted is the order in which they will be iterated.
- Duplicates Allowed: Unlike sets, lists allow duplicate values which can be invaluable in certain situations.
- Rapid Access: Standard operations such as adding and retrieving elements execute at different speeds. For example, accessing an element in an is generally faster than in a due to its array backing.
Set Interface
In contrast, the Set interface is all about uniqueness. This interface dictates that no two elements in a set can be identical, making it crucial for applications that necessitate the prevention of duplicates. Think of an online voting system where one user should only vote once; a set becomes an appropriate choice here.
With implementations like and , choosing the right type of Set depends on the requirements of your application.
- HashSet provides constant time performance for most operations but does not maintain any order of elements.
- TreeSet, on the other hand, keeps elements sorted in their natural order or as determined by a provided comparator.
Important features of the Set interface include:
- No Duplicates: Any attempt to add a duplicate entry simply won't have any effect.
- Enhanced Performance: For operations like checking membership, sets offer a fast way to achieve this, especially with hash-based implementations.
Map Interface
When it comes to key-value pairs, the Map interface is paramount. It allows you to store associations between elements, thus making it easy to retrieve values based on their corresponding keys. In everyday scenarios, think of a phone book where names (keys) correspond to phone numbers (values).
Common implementations like and each serve distinct purposes:
- HashMap offers performance advantages for insertions and lookups but has no specific order.
- TreeMap on the other hand sorts the map based on the natural ordering of keys or a specific comparator, ensuring that you can process elements in a sorted manner.
The benefits and considerations surrounding the Map interface include:
- Key Uniqueness: Each key in a map is unique while values can repeat; this is pivotal for many data-driven applications.
- Efficient Lookups: Retrieving values via keys is typically conducted in constant time for hash maps, making them suitable for applications like caches and dictionaries.
"Understanding the core interfaces is the first step to mastering Java collections. They provide the foundation on which collections are built and significantly influence your program's design and performance."
By knowing these interfaces well, developers can pick and choose the right structure to optimize their code. Each interface brings its own strengths to the table, and a user who is familiar with these can greatly enhance the quality and performance of their Java applications.
Understanding Collection Types
Understanding the types of collections in Java is key to wielding the full power of this programming language. Each collection has its own set of characteristics, making them suitable for different scenarios. Here, we will explore several important collection types that Java offers, each with their unique benefits and considerations.
ArrayList Characteristics and Use Cases
ArrayLists are likely the most widespread type of collection you'll encounter in Java. They allow you to store a dynamically sized array of objects. This flexibility means you don’t have to declare the size upfront, which is great for most applications where the number of elements can change.
Key Characteristics:
- Dynamic Size: Unlike arrays, ArrayLists can expand dynamically without needing to create a new array and copy elements.
- Random Access: They provide fast access to elements as they permit random access using an index, making getting items quick and efficient.
- Insertion & Deletion: Adding or removing elements can be slow, especially if done at the beginning of the list since it often requires shifting elements.
Use Cases:
- Ideal when you need to frequently read data.
- Great for applications where order matters, but the size is uncertain, such as shopping carts or playlists in multimedia applications.
LinkedList Advantages and Disadvantages
LinkedLists are another versatile structure that is made up of nodes. Each node contains the data and a reference to the next node, allowing for efficient insertions and deletions.
Advantages:
- Efficient Insertions/Deletions: Inserting or removing elements is very quick, especially when you’re modifying the ends of the list.
- Memory Efficiency: LinkedLists do not have to maintain their size. Since they are dynamically allocated, they are memory efficient for managing large data volumes.
Disadvantages:
- Slow Random Access: Accessing elements isn’t as fast as ArrayLists, as it requires traversal from the head.
- More Memory Usage: Each node has an overhead due to the extra pointers, leading to higher memory use compared to ArrayLists, particularly for small datasets.
HashSet: When to Use It
HashSet is part of the Java Collections Framework and implements the Set interface, which means it does not allow duplicate elements. Here, the benefits of the HashSet shine brightly.
Key Features:
- Unordered Collection: HashSet does not guarantee the order of elements, which can be advantageous or disadvantageous depending on your needs.
- Constant Time Complexity: Searching for an element, adding, and removing elements occurs in constant time, provided the load factor and threshold are managed well.
When to Use It:
- When you need fast operations on collections of non-duplicate elements.
- Useful in scenarios like tracking unique visitors on a website or in scenarios where quick lookups are essential.
TreeSet: Benefits and Limitations
TreeSet also implements the Set interface but arranges its elements in a naturally sorted order.
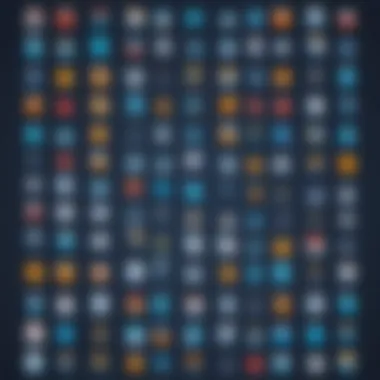
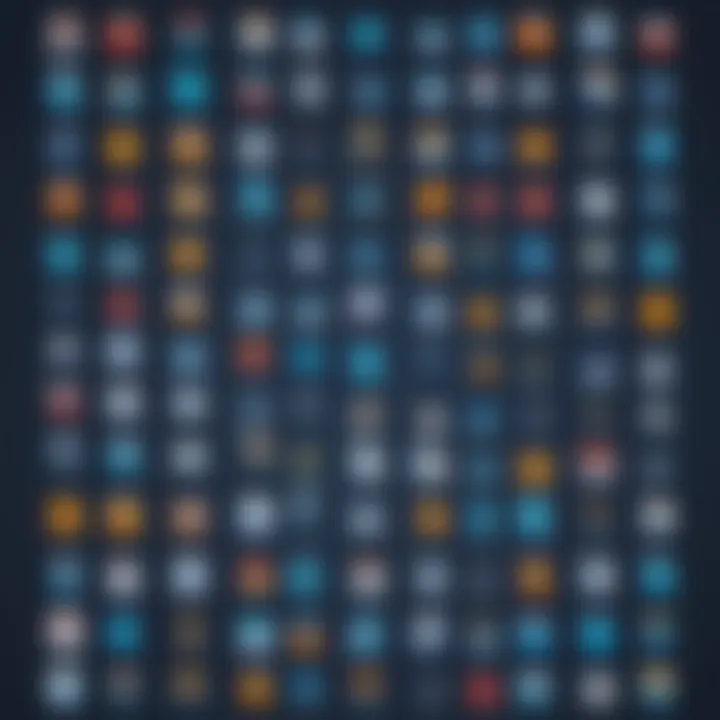
Benefits:
- Sorted Order: It maintains elements in a sorted manner, which is beneficial if you need to retrieve elements in a specific order.
- Range View Operations: The ability to create subsets based on range allows for intricate data manipulation.
Limitations:
- Performance Overhead: Given that it relies on a Red-Black tree structure, performance can take a hit when it comes to insertion and deletion relative to HashSet.
- Not Ideal for Unordered Data: If the order is irrelevant, using TreeSet might be overkill.
HashMap vs TreeMap: A Functional Comparison
When it comes to associative arrays in Java, HashMap and TreeMap are two heavyweights. Each has unique capabilities that fit specific use cases better than the other.
HashMap:
- Provides average constant-time performance for get and put operations. It does not maintain any order among its entries.
- Uses hashing, which allows for very efficient search times.
TreeMap:
- Implements the NavigableMap interface and maintains a sorted order of its keys.
- All operations are logarithmic time complexity, making it slower compared to HashMap under heavy loads.
Conclusion:
- Use HashMap for efficiency when the order of entries doesn't matter.
- Opt for TreeMap when you want to keep your keys sorted.
That's in short the key functionalities and characteristics of these collection types in Java. Being aware of these details can equip you with the knowledge needed to make the best choice for your programming tasks.
Iterating Through Collections
When working with collections in Java, one fundamental aspect that cannot be overlooked is how to effectively iterate through these data structures. This concept is not just a mere technicality; it impacts performance, maintainability, and readability of code. Different collection types come with their idiosyncratic ways of iterating, and understanding these can help you write more efficient and elegant programs.
Iterating through collections can be viewed as the bridge between your data and your logic, allowing you to transform, retrieve, or manipulate data as needed. Without appropriate iteration techniques, one's ability to harness the full potential of Java collections would be significantly hampered.
Using for-each Loop
The for-each loop, or enhanced for loop, represents one of the simplest ways to iterate over collections. It provides a clean syntax, minimizing boilerplate code. Many developers appreciate its ease of use and clarity, allowing one to focus more on what is being done with the elements rather than how to access them.
Here's a brief comparison:
- Simplicity: A for-each loop reduces the chance of errors and improves readability significantly.
- Safety: It automatically handles the retrieval of elements without exposing the underlying structure of the collection.
An example of using a for-each loop with an ArrayList can look like this:
This not only iterates through the collection but does so in a manner that is immediately understandable. Keep in mind, however, that while the for-each loop is generally more efficient, it doesn't allow for concurrent modification or removal of elements during iteration.
Utilizing Iterators
While for-each loops are easy-going and typically favored, iterators offer a more granular level of control over the iteration process. They are particularly useful when you need the capability to modify the collection while iterating. The iterator interface grants methods like , , and , which provides flexibility not available with the for-each loop.
Example:
In this code snippet, the iterator allows for the removal of an element safely. During iteration, this avoids the risk of , which could occur if you attempted to modify a collection through a traditional for loop.
Stream API for Collection Operations
With Java 8 and beyond, the introduction of the Stream API revolutionized the way collections can be processed. Streams enable a more functional programming approach to manage collections and provide a wide array of operations, from filtering to mapping and collecting results.
Using streams can make your code cleaner and more expressive. For example:
This snippet filters the to find those that start with "A" and prints them. The use of streams efficiently chains operations in a way that is both readable and concise. The benefits include:
- Composability: Stream operations can be linked together, allowing for complex queries in a simplified manner.
- Lazy Evaluation: Streams can optimize performance as they process items only when necessary.
The Stream API is more than just a convenience; it promotes a different way of thinking about data processing, leaning towards immutability and stateless operations.
Performance Considerations
When it comes to collections in Java, performance considerations hold significant weight. As developers, understanding how our choice of collection impacts the efficiency of our applications can save a lot of headaches down the line. Efficient coding not only makes our programs faster but also optimizes resource utilization, which is crucial in today’s computing landscape where performance can make or break user experience.
Time Complexity of Collections
Time complexity, in simple terms, refers to how the runtime of an algorithm or collection operation grows as the size of the input increases. It’s a big deal when we talk about collections because different collections have different time complexities for their key operations like addition, removal, searching, and traversal.
Here’s a quick breakdown of how some common collections stack up:
- ArrayList:
- LinkedList:
- HashSet:
- TreeSet:
- Add: Average O(1) when appending to the end, O(n) if resizing.
- Remove: O(n) since it may need to shift elements.
- Get: O(1) since it allows direct access to elements.
- Add: O(1) for both adding at the front or back.
- Remove: O(1) if you know the node to remove, otherwise O(n).
- Get: O(n) since it must traverse to the position.
- Add: O(1) on average due to hashing.
- Remove: O(1) on average for similar reasons.
- Contains: O(1) on average, but poor performance can occur with many collisions.
- Add: O(log n) due to the underlying red-black tree structure.
- Remove: O(log n) as well.
- Contains: O(log n) since it must traverse the tree.
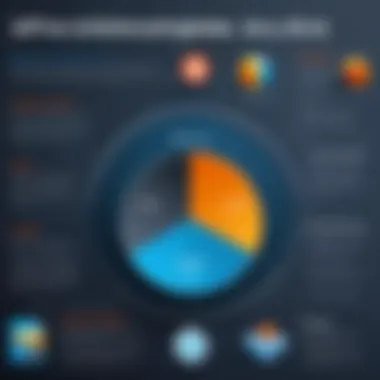
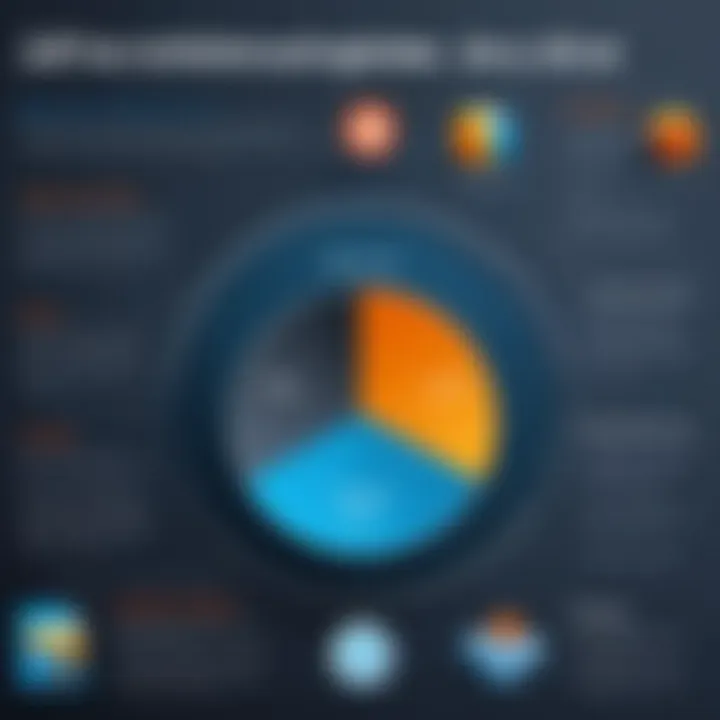
Maintaining an understanding of these time complexities is pivotal when designing systems that need to scale. If you're choosing a collection, consider what operations you’ll perform most often, and select accordingly. A thorough understanding prevents the common pitfall of choosing a collection type that seems intuitive without considering its performance implications in actual use cases.
Space Complexity Insights
Space complexity, on the other hand, refers to the amount of memory space required by your collection in relation to the data it holds. Using collections efficiently means not just considering the time required for operations but also their memory footprint.
To illustrate how this works in practice:
- ArrayList: Uses a single contiguous memory block. If the size doubles and elements exceed capacity, it incurs a sizable overhead due to the need to copy the data.
- LinkedList: Each element stores a reference to the next and/or previous elements, meaning larger memory usage per element. However, it can efficiently manage memory in terms of growth.
- HashSet and TreeSet:
- HashSet in essence grabs memory proportional to the number of items stored but is sensitive to its load factor.
- TreeSet provides a balance, utilizing more memory for the structure of the tree, but offers order which can be beneficial in certain scenarios.
Understanding these elements allows developers to make nuanced decisions about how to implement their collections. If your application is intended to run on devices with limited memory, or if scalability is a key factor, you wouldn’t want your choice of collection to add unnecessary overhead.
Ultimately, performance considerations can help developers balance time and space complexity. Ignoring these could lead to slowdowns and excess resource usage.
Knowing the performance characteristics of collections becomes a potent tool in the Java programmer’s toolkit—ensuring elegance in code and efficiency in execution.
Best Practices for Using Collections
Using collections in Java comes with its own set of challenges and considerations. When you find yourself knee-deep in programming tasks, understanding how to navigate through collections effectively can make a world of difference. This section provides crucial insights into best practices for using collections, ensuring that your Java applications are both efficient and robust. Selecting the right collection type, minimizing the memory footprint, and securing thread safety are integral components that no developer should overlook.
Choosing the Right Collection Type
Not all collections are created equal. Each type serves a unique purpose, and understanding these distinctions is paramount. When faced with the decision of which collection to use, consider the following elements:
- Data Organization: Using a when you require ordered access allows you to easily access elements by index. In contrast, a is your go-to for ensuring uniqueness without caring about the order.
- Performance Requirements: Each collection has its performance characteristics. For example, offers quicker random access but slower insertions and deletions compared to . If you must frequently add or remove items, the latter might be the better option.
- Use Cases: Think about what you need. A is suitable for lookups based on keys, while a will keep elements in a sorted order for you.
Using the right collection type can save you from making code adjustments later and can also contribute to maintaining code clarity.
Minimizing Memory Footprint
In any Java application, memory efficiency is important. An oversized collection can lead to wasted memory and sluggish performance. Here are strategies to minimize your memory footprint when working with collections:
- Prefer Primitive Types: Whenever possible, use collections that directly store primitive types instead of wrapping them in objects. For instance, if you're primarily dealing with integers, using an or specialized collections can have benefits.
- Use Collections Sparingly: Only maintain collections with the necessary items. Removing items when they're no longer needed helps keep your memory usage in check.
- Choose Appropriate Implementations: Implementations like have different memory usage characteristics compared to . Sometimes, using a smaller collection can dramatically reduce memory consumption.
By taking such measures, you'll ensure your program runs smoother and is more scalable over time.
Ensuring Thread Safety
In multithreaded applications, thread safety is not just a nicety—it’s a necessity. Without thread safety, you risk facing unpredictable behaviors that can lead to bugs that are hard to track down. Here are some approaches to ensure your collections are thread-safe:
- Synchronized Collections: The Java Collections Framework comes with built-in synchronized wrappers. You can use to create a thread-safe list.
- Concurrent Collections: Consider using concurrent collections such as and . These are designed for high concurrency and have better performance in a multithreaded environment compared to synchronized collections.
- Avoid Concurrent Modification: Keep an eye on how collections are modified while they are being iterated. Making changes to a collection while iterating through it can cause a , which can trip up your entire application.
Emphasizing thread safety in your design can be the difference between a smooth-running application and a tangled mess of races and data corruption.
Always remember, the right knowledge leads to better decisions, particularly when it comes to optimizing Java collections.
Advanced Collection Techniques
When it comes to Java, using advanced collection techniques is not just a nice-to-have; it’s essential. These approaches empower developers to create collections that are tailored to specific requirements and performance metrics. This section highlights the significance of diving deeper into Java's collection framework, which is not only about using predefined data structures, but also about extending and customizing these structures to solve unique challenges.
Custom Collection Implementations
In many scenarios, out-of-the-box collection types don’t fit the bill. This is where custom collection implementations come into play. Creating a custom collection allows you to build a data structure that perfectly matches your application's requirements, giving you the flexibility to manage data storage and retrieval efficiently.
But why should one bother doing this? Here are key reasons:
- Tailored Performance: Standard Java collections might not be optimized for every use case. By making your own collections, you can design specific performance enhancements that match the data characteristics you expect.
- Specialized Behaviors: Sometimes, you might need a collection that enforces certain business logic or behaviors. By implementing your own structure, you can easily embed these expectations into the collection's core.
- Memory Management: Custom implementations can be designed with particular attention to how memory is managed, which can result in better overall resource allocation and lower memory consumption.
For example, if you're dealing with a large volume of data, creating a sparse array could be advantageous compared to using a traditional ArrayList, especially when many entries might not be utilized. Below is a simple way to start a custom collection:
This snippet sets you on the path to developing a class that could cater to specific needs while adhering to the Java Collections Framework.
Using Collections in Concurrency
In today’s multi-threaded applications, handling collections in a concurrent environment is a critical skill. Using collections in concurrency requires an understanding of how threads interact with shared data and the potential pitfalls that can arise if proper care isn't taken. Without the right techniques, data could become corrupted or lead to unexpected behaviors in your application.
Some key components to consider include:
- Concurrent Collections: Java provides a set of thread-safe collections such as ConcurrentHashMap and CopyOnWriteArrayList. These are built to handle situational race conditions that would typically occur in a multi-threaded context. They allow safe operations without the external synchronization that can often bottleneck performance.
- Immutable Collections: Keeping collections immutable can also be a smart strategy. An instance like ensures that once the list is created, it cannot be altered by any thread, thus removing the risk of concurrent modifications.
- Locking Mechanisms: In cases where custom collections are needed, using locks can provide another layer of safety when accessing shared resources. However, this must be done judiciously to not hinder performance.
"Concurrency can be tricky, but with the right collections, it’s manageable. Embrace the tools Java provides!"
By harnessing these advanced techniques, developers can manage collections more effectively and safely, maximizing performance and ensuring the integrity of their data even in a rapidly changing environment. Conclusively, understanding and implementing advanced collection techniques isn’t just a feature; it’s a necessity for any serious Java developer.
Closure and Further Reading
As we wrap up our journey through the landscape of Java Collections, it's essential to reflect on the critical takeaways. The exploration of collections equips both novice and seasoned programmers with the tools necessary for effective data management. Understanding the significance of collections not only enhances code efficiency but also fosters a deeper comprehension of Java's architecture.
In this article, we discussed various aspects of collections—from core interfaces like List, Set, and Map to their specific implementations such as ArrayList, HashSet, and HashMap. Each type serves unique purposes and performance characteristics, which can vastly influence your applications. An emphasis was placed on best practices; knowing when and how to deploy these collections can make a substantial difference in both memory usage and execution speed.
Moreover, mastering advanced collection techniques, including custom implementations and concurrency, arms developers with adaptable strategies to tackle intricate programming challenges. The value of learning about collections extends beyond their individual components; it lies in the understanding of how these structures interrelate and function within larger systems.
"In the world of programming, understanding collections is akin to knowing your tools; it's not just about having them, but knowing how to use them wisely."
Summary of Key Points
- Understanding Collections: Recognizing what collections are and why they're important in Java programming.
- Core Interfaces: Familiarity with core interfaces like List, Set, and Map and their nuances.
- Collection Types: Insight into different types of collections and their respective benefits and limitations.
- Performance: Analyzing time and space complexities leads to more efficient application design.
- Best Practices: Emphasizing the selection of the right collection type and strategies for memory management and thread safety.
- Advanced Techniques: Employing custom collections and practices in concurrent Java scenarios.
Resources for Advanced Learning
For those eager to delve deeper into the world of Java Collections and related programming topics, consider the following resources:
- Wikipedia on Collections Framework: A foundational article covering the Java Collections Framework in detail.
- Britannica on Programming Languages: A broader context in which Java and its collections can be understood.
- Reddit's Java Community: A vibrant forum for discussions, questions, and resources on Java programming and collections.
- Facebook Groups for Learning Java: Connecting with fellow learners and professionals can be invaluable for enhancing your skills.
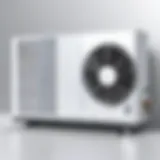
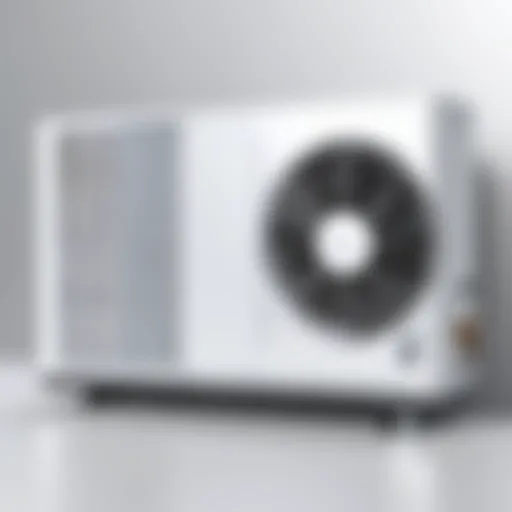