Mastering Java: A Comprehensive Beginner's Practice Guide for Java Programming Skills Enhancement
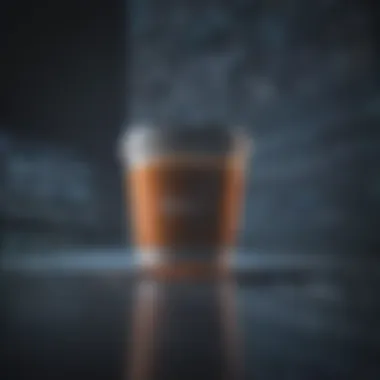
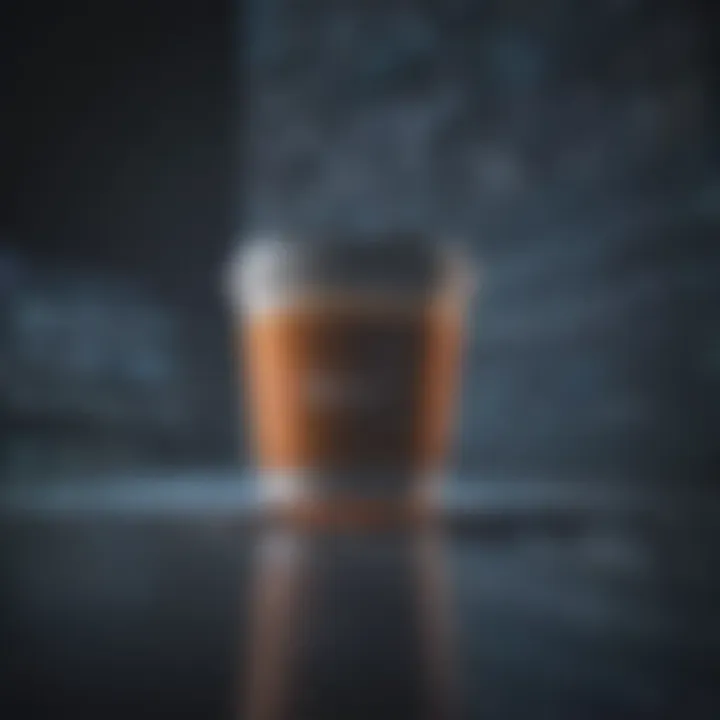
Introduction to Programming Language
Java, a versatile programming language, renowned for its reliability and flexibility, has been a cornerstone in the technological realm for decades. Understanding Java not only serves as a robust foundation for aspiring programmers but also provides a gateway to a myriad of career opportunities across various industries. Exploring the history and background of Java unveils its evolution from inception to becoming one of the most sought-after languages in the world today.
- History and Background: Delving into the origins of Java sheds light on how it was developed by James Gosling at Sun Microsystems in the early 1990s. Initially named Oak, it was later rebranded as Java and released to the public in 1995. Java's primary objective was to design a language for consumer electronic devices, a vision that transformed into its widespread use in web development, enterprise applications, and mobile computing.
- Features and Uses: Java's key features, including platform independence, object-oriented structure, and robust standard library, distinguish it from other languages. Its ability to run on any device with the Java Virtual Machine (JVM) has propelled its popularity in cross-platform development. Java finds its applications in building web applications, mobile apps, enterprise software, and software tools, making it a versatile choice for developers worldwide.
- Popularity and Scope: The widespread adoption of Java in the tech industry highlights its significance and enduring popularity. Java's scalability, maintainability, and performance have solidified its position as a preferred language for businesses and developers alike. With a vast developer community and a plethora of libraries and frameworks, Java offers a supportive ecosystem for individuals embarking on their programming journey.
Introduction to Java Programming
In the realm of programming languages, Java stands out as a versatile and powerful tool. This section of the article delves into the foundational aspects of Java programming that form the bedrock for all future Java endeavors. Understanding Java Fundamentals is crucial for any aspiring programmer, as it lays the groundwork for comprehending more advanced concepts. From Variables and Data Types to Control Flow Statements, each element plays a vital role in shaping a programmer's journey. By mastering these fundamental principles, individuals can unlock a world of possibilities within Java programming.
Understanding Java Fundamentals
Variables and Data Types
Variables and Data Types are the building blocks of Java programming. They allow programmers to store and manipulate data efficiently. The key characteristic of Variables is their ability to hold different values, enabling dynamic operations within a program. Data Types, on the other hand, define the type of data a variable can store, ensuring data integrity and efficient memory usage. Understanding Variables and Data Types is essential as it forms the basis for handling information in Java applications, making it a popular choice for developers.
Operators and Expressions
Operators and Expressions form the backbone of Java computations. Operators are symbols that perform specific operations on one or more operands, allowing for arithmetic, logical, and relational calculations. Expressions, comprised of variables, operators, and method calls, evaluate to produce a singular value. Their unique feature lies in their ability to manipulate data and control program flow efficiently. By mastering Operators and Expressions, programmers gain a powerful tool for creating dynamic and interactive Java applications.
Control Flow Statements
Control Flow Statements dictate the flow of execution within a Java program. They include mechanisms such as loops and conditional statements, enabling developers to control how commands are executed. The key characteristic of Control Flow Statements is their ability to direct program flow based on conditions or iteration requirements. Mastering Control Flow Statements is crucial as it empowers programmers to create logic and decision-making processes within their applications, enhancing program flexibility and functionality.
Setting Up Development Environment
Choosing IDE
Choosing the right Integrated Development Environment (IDE) is paramount for Java programmers. An IDE provides a comprehensive suite of tools for writing, testing, and debugging code, streamlining the development process. The key characteristic of an IDE is its user-friendly interface and robust feature set, making it a preferred choice for developers. IDEs offer unique features such as code completion and refactoring, enhancing coding efficiency while ensuring code quality. By selecting the appropriate IDE, programmers can boost productivity and streamline their Java development workflow.
Installing JDK
Installing the Java Development Kit (JDK) is the first step towards building Java applications. The JDK includes essential tools such as the Java compiler and runtime environment, allowing developers to compile and execute Java code. The key characteristic of the JDK is its platform independence, enabling developers to write code once and run it on any Java-supported platform. By installing the JDK, programmers gain access to a rich set of libraries and tools that form the backbone of Java development, accelerating the coding process and enabling robust application creation.
Configuring Build Path
Configuring the Build Path in an IDE is crucial for Java projects. The Build Path specifies the libraries and dependencies required for a project to compile and run successfully. The key characteristic of configuring the Build Path is its ability to manage external libraries and frameworks seamlessly, ensuring project integrity. By setting up the Build Path correctly, developers can avoid compilation errors and runtime issues, enhancing the stability and functionality of their Java applications.
Data Structures and Algorithms in Java
Data Structures and Algorithms play a pivotal role in mastering Java programming. Understanding these concepts provides a solid foundation for efficient code implementation and problem-solving in Java. Data Structures focus on organizing and managing data effectively, while Algorithms deal with solving computational problems systematically. By delving into Data Structures and Algorithms in Java, programmers can enhance their skills in optimizing code performance, memory usage, and overall program efficiency.
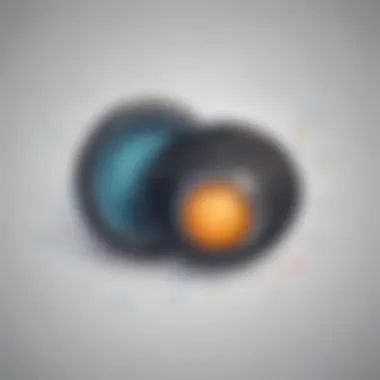
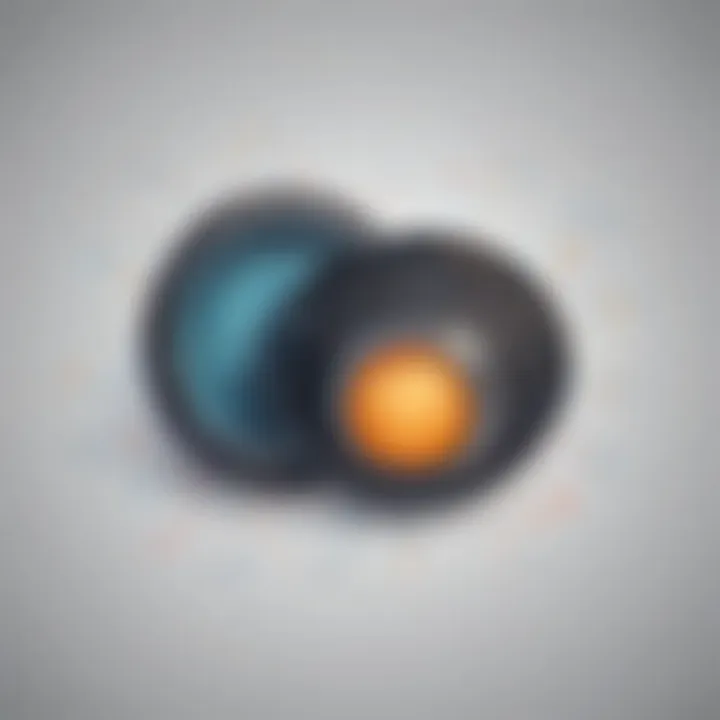
Arrays and Lists Operations
Array Initialization
Array Initialization in Java refers to the process of allocating memory and assigning initial values to array elements. This step is crucial as it sets the size and content of the array, laying the groundwork for efficient data storage and retrieval. The key characteristic of Array Initialization lies in its simplicity and ability to directly specify values during declaration. This approach is a popular choice in Java programming due to its ease of implementation and quick setup, making it ideal for scenarios requiring predefined data structures. However, one disadvantage of Array Initialization is its fixed size, limiting dynamic data manipulation compared to other data structures.
List Iteration
List Iteration involves traversing through elements in a list systematically, usually using loops to access, retrieve, or modify data elements. The key characteristic of List Iteration is its flexibility in handling varying list sizes and structures, accommodating dynamic data management requirements efficiently. This flexibility makes List Iteration a preferred method for iterating through collections of elements in Java, offering enhanced control and functionality. However, excessive use of List Iteration may lead to performance issues in large data sets, requiring optimization strategies for improved efficiency.
Searching and Sorting Techniques
Searching and Sorting Techniques in Java are essential algorithms for organizing and retrieving data systematically. Searching techniques focus on locating specific elements within a dataset, while sorting techniques arrange data in a specified order for easier access and manipulation. The key characteristic of Searching and Sorting Techniques lies in their ability to streamline data operations, improving search efficiency and data organization. These techniques are beneficial in scenarios requiring quick data retrieval or organized data presentation. However, implementing complex search and sort algorithms may lead to increased processing time and memory usage, necessitating optimization for optimal performance.
Working with Loops and Conditional Statements
For and While Loops
For and While Loops in Java are iterative structures that execute a block of code repeatedly based on specified conditions. The key characteristic of For and While Loops is their ability to automate repetitive tasks and control flow based on varying conditions. These loops are popular choices for iterating through arrays, lists, or performing specific actions a set number of times. However, improper use of loops may result in infinite loops or inefficient code execution, requiring careful programming and logical conditions for optimal performance.
If-Else Conditions
If-Else Conditions in Java provide decision-making capabilities based on specified conditions, allowing for branching logic and alternate code execution paths. The key characteristic of If-Else Conditions is their versatility in handling multiple scenarios and controlling program flow dynamically. These conditions are beneficial for implementing conditional logic, error handling, and customized behavior based on specific input conditions. However, nested If-Else structures can result in code complexity and reduced readability, requiring careful design and simplification for maintainable code.
Switch Case Statements
Switch Case Statements in Java simplify decision-making by evaluating a variable against a list of values and executing corresponding code blocks. The key characteristic of Switch Case Statements is their efficiency in handling multiple possible outcomes with a concise and structured approach. This method is beneficial for replacing multiple nested If-Else conditions, improving code clarity and performance. However, Switch Case Statements have limitations in handling complex conditions or ranges of values, requiring consideration for optimal usage and code structuring.
Introduction to Object-Oriented Programming
Classes and Objects
Classes and Objects form the core concepts of Object-Oriented Programming (OOP) in Java, enabling the creation of reusable code structures and modeling real-world entities. The key characteristic of Classes and Objects is their ability to encapsulate data and behavior into distinct entities, promoting code organization and reusability. This approach is beneficial for designing modular and extensible programs, fostering code maintenance and scalability. However, an overabundance of objects and classes may lead to a complex class hierarchy, impacting code readability and managing class dependencies effectively.
Inheritance and Polymorphism
Inheritance and Polymorphism in Java allow for class hierarchy establishment and flexibility in implementing different behaviors through shared interfaces. The key characteristic of Inheritance and Polymorphism is their capability to promote code reuse, enhance extensibility, and support method overriding for customization. This feature is beneficial for creating flexible and maintainable code structures, facilitating code extension without modifying existing functionality. However, excessive class inheritance levels may introduce hierarchy complexities and increase coupling, necessitating careful design considerations for a balanced and scalable OOP architecture.
Encapsulation and Abstraction
Encapsulation and Abstraction promote data hiding, method access control, and interface definition to isolate implementation details and streamline code maintenance in Java. The key characteristic of Encapsulation and Abstraction is their emphasis on information hiding and simplifying complex systems by encapsulating internal logic. This practice enhances code security, modularity, and reduces coupling, promoting code reusability and ease of modification. However, excessive encapsulation may lead to reduced code visibility and hinder code understanding for new developers, requiring clear documentation and design patterns for effective collaboration and maintenance.
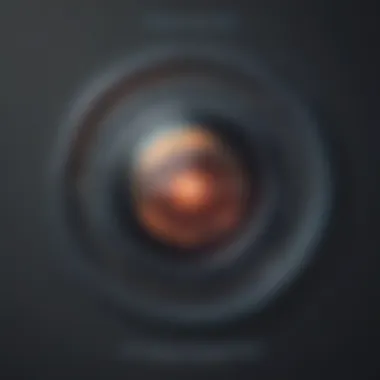
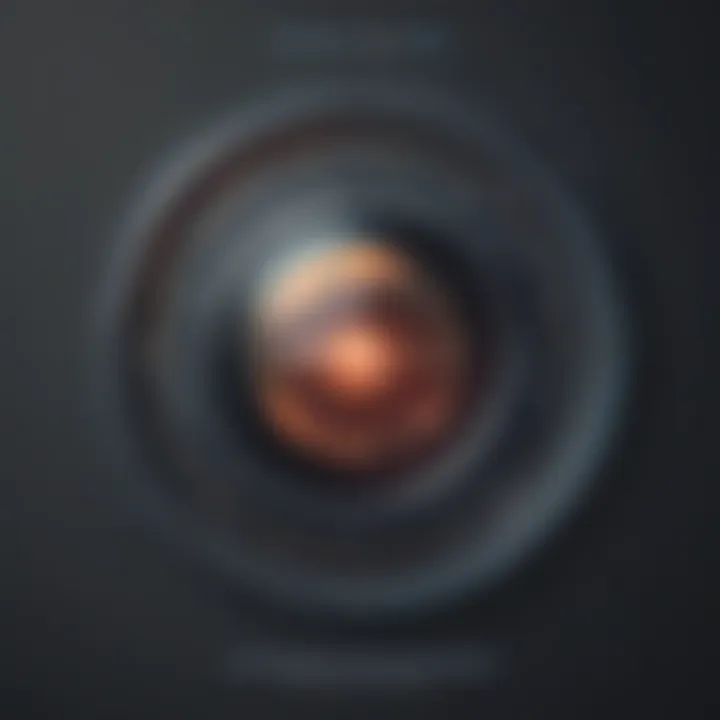
Java Application Development Practices
Java Application Development Practices plays a crucial role in this article by delving into various elements that help in enhancing Java programming skills. By focusing on GUI development with JavaFX, file handling and exception handling, as well as introducing multithreading concepts, this section aims to provide a well-rounded understanding of practical Java application development.
GUI Development with JavaFX
Creating User Interfaces
In Java Application Development Practices, creating user interfaces using JavaFX is a fundamental aspect imperative for developing visually appealing applications. This practice allows programmers to design interactive interfaces efficiently, enhancing user experience. By harnessing the power of JavaFX's graphical capabilities, developers can craft responsive and dynamic user interfaces. The flexibility and extensive toolkits available in JavaFX make it a popular choice for UI development, enabling developers to create modern and engaging interfaces easily. However, like any tool, JavaFX also has its complexity, requiring a learning curve to master its intricacies.
Event Handling
Event handling in JavaFX is a critical component that enables applications to respond to user interactions effectively. By incorporating event handling mechanisms, developers can define specific behaviors for different user actions, such as button clicks or mouse movements. This feature empowers developers to create interactive and responsive applications that cater to diverse user inputs. The event handling capabilities of JavaFX streamline the process of managing user interactions, enhancing the overall usability of Java applications. However, mastering event handling in JavaFX necessitates a solid understanding of its event-driven architecture.
FXML Design Patterns
FXML design patterns offer a structured approach to designing JavaFX interfaces, enhancing the development process of user interfaces. By incorporating FXML files to define the layout of JavaFX applications, developers can separate the presentation layer from the application logic. FXML facilitates the creation of visually appealing interfaces by providing a declarative XML-based markup language. This approach simplifies UI design and maintenance, improving the overall modularity of Java applications. While FXML design patterns enhance productivity in UI development, integrating them seamlessly into Java projects requires familiarity with the underlying principles.
File Handling and Exception Handling
In the realm of Java Application Development Practices, file handling and exception handling are indispensable aspects that contribute to building robust and error-tolerant applications. By mastering the techniques of reading and writing files, developers can efficiently manage data persistence and retrieval in Java programs. Additionally, effective exception handling mechanisms ensure graceful error recovery and program stability. The utilization of try-catch-finally blocks enhances fault tolerance in Java applications, allowing for proper resource management and error handling. However, implementing robust file and exception handling strategies demands meticulous attention to mitigating potential risks and ensuring code reliability.
Introduction to Multithreading
The introduction to multithreading within the Java Application Development Practices section introduces developers to the world of concurrent programming, enabling the execution of multiple threads simultaneously. By exploring concepts like creating threads, synchronization techniques, and thread pools, developers can leverage the power of parallel processing to enhance application performance. Multithreading empowers applications to handle complex tasks efficiently, improving responsiveness and scalability. While creating threads in Java allows for parallel execution of tasks, synchronization techniques and thread pools facilitate resource sharing and task management. However, implementing multithreading requires a deep understanding of thread safety and synchronization to mitigate concurrency issues and ensure program integrity.
Java Best Practices and Coding Standards
In this section, we delve into the crucial topic of Java Best Practices and Coding Standards. Understanding and implementing industry best practices is paramount in Java programming to ensure efficient, maintainable, and scalable code. By adhering to these standards, developers can enhance code quality, improve collaboration, and streamline the development process. Java Best Practices encompass a wide array of principles like naming conventions, code readability, error handling, and performance optimization. Implementing Coding Standards ensures uniformity across the codebase, making it easier to understand, maintain, and debug. Striving for excellence in coding standards can elevate a developer's proficiency and credibility in the programming community.
Code Optimization Techniques
Efficient Memory Usage:
Efficient Memory Usage is a critical aspect of Java programming that focuses on minimizing memory consumption while maximizing performance. By optimizing memory usage, developers can avoid memory leaks, reduce overhead, and enhance the overall efficiency of their applications. Employing techniques like object pooling, avoiding unnecessary object creation, and optimizing data structures can significantly impact the memory footprint of a Java application. Efficient Memory Usage plays a vital role in improving the responsiveness and scalability of software systems, especially in resource-constrained environments.
Reducing Code Redundancy:
Reducing Code Redundancy is a fundamental principle in software development that emphasizes eliminating repetition and promoting code reusability. By identifying and refactoring redundant code segments, developers can enhance code maintainability, simplify debugging, and improve overall productivity. Utilizing functions, classes, and inheritance hierarchies effectively can help in reducing code redundancy and achieving a more concise and manageable codebase. Reducing Code Redundancy not only aids in enhancing code readability but also minimizes the likelihood of errors and facilitates future code modifications.
Improving Performance:
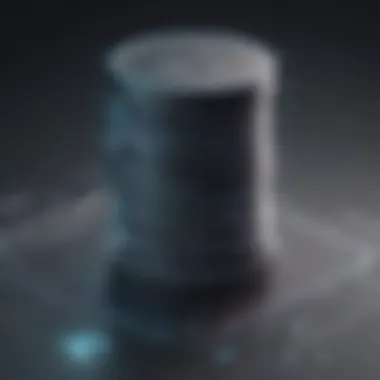
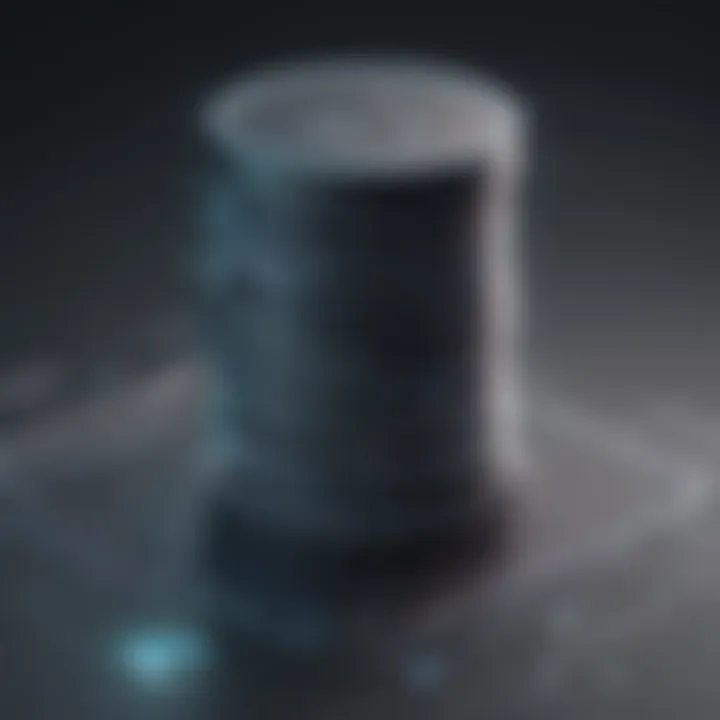
Improving Performance is a core objective in software development that aims to enhance the speed, responsiveness, and efficiency of applications. By optimizing algorithms, data structures, and resource utilization, developers can boost the performance of their Java programs significantly. Profiling, benchmarking, and optimizing critical code paths are essential strategies to identify performance bottlenecks and address them effectively. Improving Performance is vital in meeting user expectations, scalability requirements, and ensuring the competitiveness of software products in the market.
Documentation and Unit Testing
Javadoc Comments:
Javadoc Comments play a key role in documenting Java code, providing insights into the purpose, functionality, and usage of classes, methods, and variables. By incorporating descriptive comments directly into the code, developers can enhance code understandability, facilitate maintenance, and promote code reusability. Javadoc Comments also enable automatic generation of documentation, enhancing project documentation consistency and developer collaboration. Embracing Javadoc Comments as a documentation practice can foster better communication, code quality, and project scalability.
JUnit Framework:
The JUnit Framework is a popular unit testing framework in Java that enables developers to automate testing procedures, verify code functionality, and identify defects early in the development cycle. By writing test cases using JUnit annotations, developers can ensure code correctness, detect regressions, and promote code reliability. JUnit Framework offers a structured approach to writing and executing tests, making it easier to validate code changes, refactor code confidently, and maintain code integrity throughout the software lifecycle.
Test-Driven Development:
Test-Driven Development (TDD) is a development practice that advocates writing test cases before implementing the actual code functionality. By following the TDD approach, developers can clarify requirements, design software incrementally, and validate code behavior systematically. TDD emphasizes continuous integration of testing into the development process, fostering a robust testing culture, and allowing for rapid feedback loops. Practicing Test-Driven Development can lead to code quality improvements, efficient bug detection, and accelerated development timelines.
Version Control and Collaboration
Using Git:
Using Git is a widely adopted version control system that enables developers to track changes, collaborate on projects, and manage code repositories effectively. Git provides features like branching, merging, and distributed version control, facilitating seamless collaboration and code integration among team members. By leveraging Git's functionality for version control, developers can maintain a history of project changes, revert to previous versions, and resolve conflicts efficiently. Using Git promotes code transparency, project accountability, and streamlines the software development workflow.
Pull Requests:
Pull Requests are a key collaborative feature in Git-based development workflows, allowing developers to propose code changes, review modifications, and merge code branches systematically. By creating pull requests, developers can solicit feedback, conduct code reviews, and ensure code quality before integrating changes into the main codebase. Pull Requests promote code visibility, foster team collaboration, and establish a structured process for code contributions. Embracing Pull Requests as a collaboration practice enhances code review efficiency, knowledge sharing, and project traceability.
Code Reviews:
Code Reviews play a pivotal role in maintaining code quality, identifying defects, and sharing knowledge within development teams. By conducting thorough code reviews, developers can catch bugs early, enforce coding standards, and promote code consistency across projects. Code Reviews encourage collaborative learning, peer feedback, and continuous improvement in software development practices. Emphasizing regular code reviews can instill a culture of excellence, accountability, and knowledge sharing within development teams.
Conclusion and Further Learning
In the realm of Java programming, the Conclusion and Further Learning section serves as a pivotal juncture for budding coders. This section encapsulates the essence of the entire guide, summarizing the key learnings and paving the way for continued growth. By delving into practical projects and exercises, readers can absorb concepts in a hands-on manner, solidifying their grasp on Java programming fundamentals. Exploring these projects not only enhances technical skills but also fosters creativity and problem-solving abilities essential for mastering Java. This section acts as a bridge for learners, encouraging them to apply acquired knowledge in real-world scenarios, preparing them for more advanced challenges.
Practical Projects and Exercises
Building Calculator Application
The Building Calculator Application stands as a cornerstone in the journey of Java proficiency. This project delves into the intricacies of Java syntax and logic flow, offering learners a practical way to reinforce their understanding. Its systematic approach to implementing mathematical operations equips beginners with essential problem-solving skills. The Calculator Application's user-friendly interface and functionality make it an ideal choice for honing programming abilities. By tackling this project, individuals can comprehensively grasp the importance of modular design and efficient coding practices, setting a strong foundation for mastering Java.
Implementing Data Structures
Implementing Data Structures represents a significant milestone in Java programming skills development. This project delves into organizing and managing data efficiently, emphasizing the importance of algorithmic thinking and data manipulation. By implementing various data structures like arrays, lists, or trees, learners enhance their problem-solving capabilities and optimize code performance. The project's focus on data organization and retrieval strategies not only reinforces Java concepts but also nurtures a strategic approach to programming. Understanding data structures is crucial for writing robust and scalable applications, making this project indispensable for aspiring Java developers.
Creating Multithreaded Applications
Creating Multithreaded Applications unlocks the realm of concurrent programming in Java. This project explores parallel execution and resource synchronization, enabling learners to design efficient and responsive applications. By incorporating multi-threading concepts, individuals gain insights into handling complex tasks concurrently, optimizing system resource utilization. The unique feature of multitasking in Java enhances application efficiency and user experience, making multithreaded applications a valuable addition to one's programming repertoire. Engaging with this project equips programmers with the skills to develop high-performance applications, boosting their proficiency in Java programming.