Mastering Java 8 Programming: A Comprehensive Guide for Software Developers
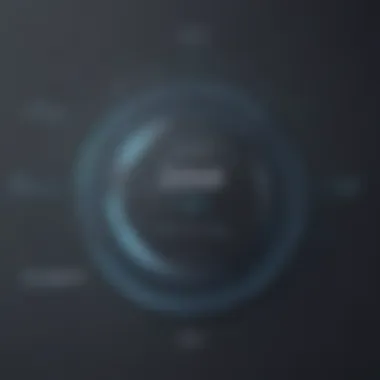
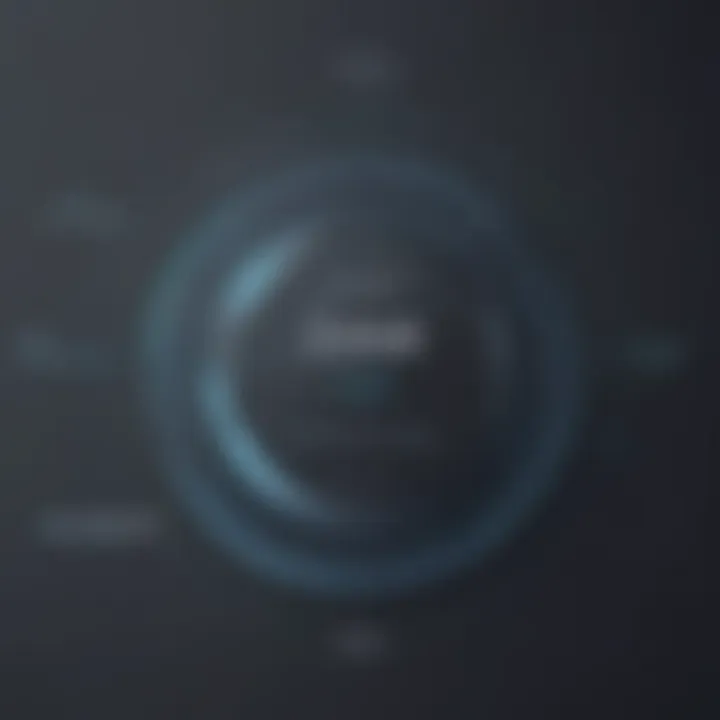
Introduction to Programming Language
Java 8 is a programming language renowned for its robust features and efficient performance in software development. Originating from Sun Microsystems in the 1990s, Java has evolved into a versatile language embraced by programmers worldwide. Its syntax facilitates clear and concise code structuring, aiding beginners in grasping fundamental programming concepts.
History and Background
Java 8 marks a significant milestone in the language's evolution, introducing innovative features like lambdas and streams that revolutionized functional programming practices. These advancements have propelled Java 8 to the forefront of modern software development, enabling developers to write more expressive and concise code.
Features and Uses
The versatility of Java 8 extends to its wide array of features, including enhanced performance with the introduction of functional interfaces and default methods. Its platform independence allows developers to write code once and run it anywhere, minimizing compatibility issues across different operating systems.
Popularity and Scope
Java 8's popularity stems from its scalability and compatibility with a myriad of applications, from web development to mobile app creation. Its broad scope in enterprise-level projects and its integration with industry-standard frameworks like Spring have solidified its position as a go-to language for robust software solutions.
Introduction to Java Programming
Java 8 marks a significant advancement in the realm of programming languages, ushering in a new era of functionality and efficiency. This section delves into the core aspects and innovations that define Java 8, presenting a comprehensive overview for learners of varying levels of expertise.
Understanding the Evolution of Java
Origins of Java
The origins of Java trace back to its inception as a robust and versatile programming language. Java's creation can be attributed to the need for a platform-independent language that could revolutionize software development. By emphasizing concepts like portability and flexibility, Java has cemented its position as a stalwart in the programming community.
Additionally, the object-oriented nature of Java has streamlined the process of building intricate applications, offering a level of reliability and scalability that is unparalleled. This evolutionary trajectory underscores Java's adaptability to changing technological landscapes, making it a cornerstone in the programming domain.
Significance of Java
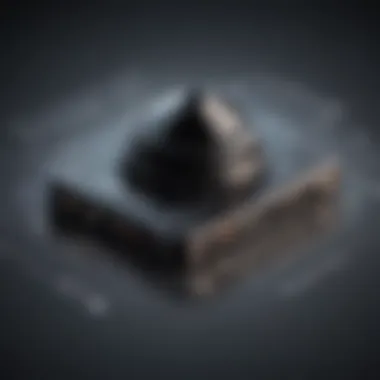
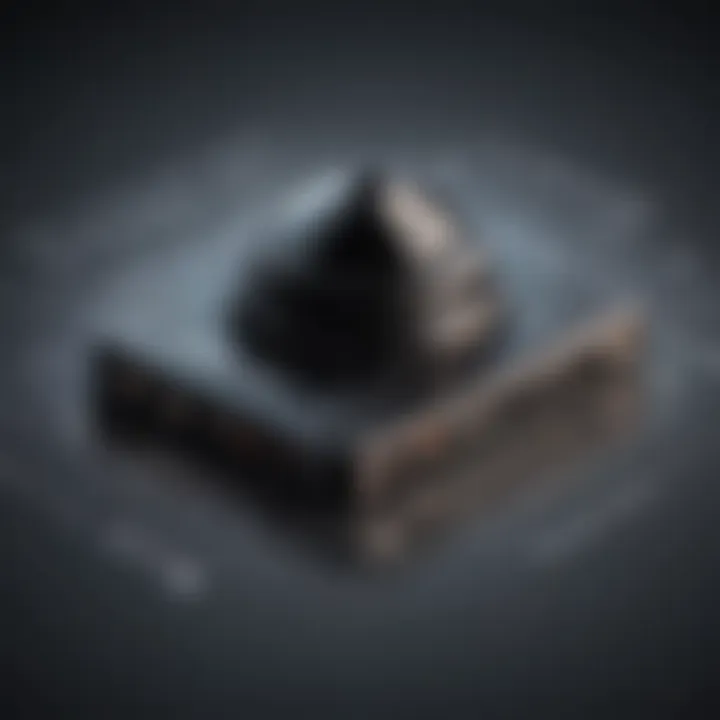
Java 8 represents a pinnacle in the evolution of the language, introducing paradigm-shifting features that have redefined programming practices. The advent of lambdas and streams has enriched the programming experience, empowering developers to write concise and expressive code with ease.
One of the standout attributes of Java 8 lies in its compatibility with functional programming paradigms, enabling developers to leverage the benefits of immutability and higher-order functions. This shift towards functional programming not only enhances code readability but also fosters the development of streamlined and efficient applications.
Features of Java
Lambdas and Functional Interfaces
The incorporation of lambdas and functional interfaces in Java 8 signifies a departure from traditional imperative programming paradigms. By facilitating a more concise syntax and enabling the passing of functions as parameters, lambdas enhance code modularity and flexibility.
Furthermore, the seamless integration of functional interfaces elevates the programming experience, providing a structured approach to implementing functional concepts within Java. This convergence of imperative and functional programming paradigms underscores Java 8's adaptability and versatility, setting it apart as a language tailored for modern development practices.
Streams and Collectors
Streams and collectors in Java 8 offer a novel approach to data processing and manipulation, paving the way for efficient and elegant solutions to complex problems. Streams provide a declarative style of programming, allowing developers to work with data in a more streamlined and functional manner.
In tandem with collectors, streams enhance the processing of collections by enabling operations such as filtering, mapping, and reduction. This dynamic duo not only simplifies the handling of data but also promotes code reusability and maintainability, making Java 8 a formidable contender in the programming landscape.
Core Concepts of Java
Java 8 introduces several essential core concepts that revolutionize the way developers write code. Lambdas, functional interfaces, and the Stream API are at the heart of Java 8, offering streamlined and efficient ways to handle data and operations. By understanding these core concepts, developers can enhance code readability, maintainability, and performance. The benefits of mastering these concepts extend to improved code quality, flexibility, and scalability in software development projects.
Lambdas in Java
Syntax and Examples
The syntax of lambdas in Java 8 simplifies the process of writing concise and expressive code snippets. By providing a compact way to represent anonymous functions, lambdas enhance code efficiency and reduce boilerplate. For example, instead of writing lengthy anonymous inner classes, lambdas allow developers to pass functionality directly as an argument. This feature significantly streamlines code, making it more readable and maintainable. While the concise syntax of lambdas offers increased productivity and code clarity, beginners may find it challenging to grasp initially.
Benefits of Using Lambdas
The utilization of lambdas in Java 8 brings a myriad of advantages to software development. One key benefit is the facilitation of functional programming paradigms, enabling developers to treat functions as first-class citizens. This functional approach leads to more modular, composable, and testable code. Additionally, lambdas promote parallelism and asynchronous programming, enhancing performance and scalability. However, the foray into asynchronous operations may introduce complexity and debugging challenges for novice programmers.
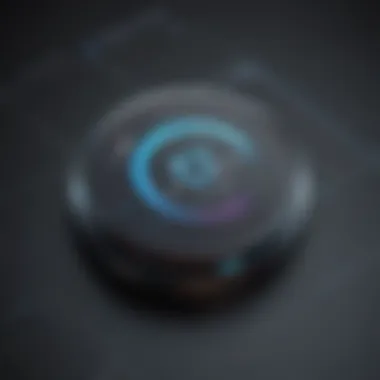
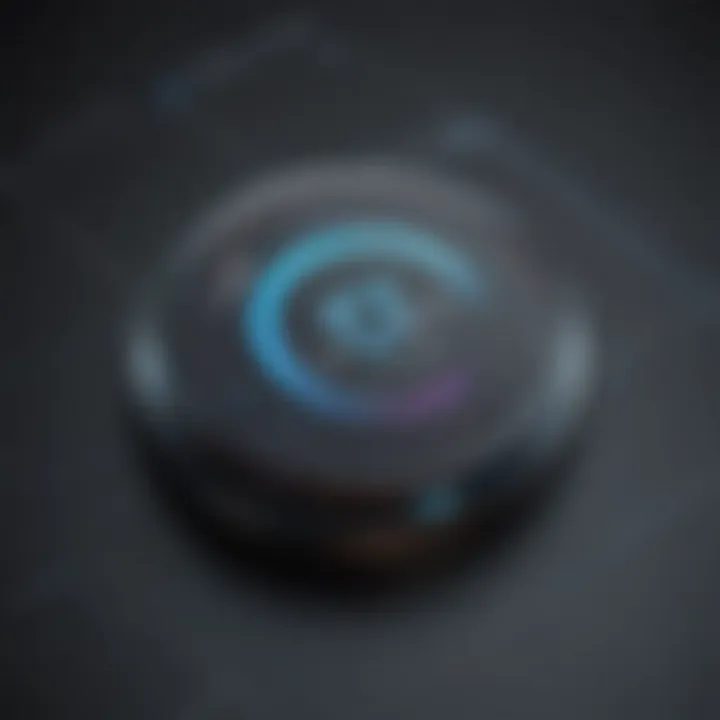
Functional Interfaces
Role in Java
Functional interfaces play a pivotal role in Java 8 by serving as the foundation for lambda expressions. These interfaces contain only one abstract method, making them ideal candidates for lambda operations. By embracing functional interfaces, developers can leverage the power of lambda expressions effectively. The clear and defined structure of functional interfaces promotes code consistency and reusability, fostering good coding practices.
Implementation and Usage
The implementation of functional interfaces in Java 8 is straightforward and versatile. Developers can either use existing functional interfaces from the Java API or create custom interfaces tailored to specific requirements. Through the usage of functional interfaces, developers can encapsulate behavior, promote code modularity, and facilitate the implementation of different functionalities. However, a deep understanding of interface inheritance and method signatures is essential to avoid ambiguity and errors during implementation.
Stream API
Working with Streams
The Stream API in Java 8 offers a declarative approach to process collections of objects. By enabling functional-style operations on streams of elements, developers can perform complex data processing tasks with ease and efficiency. Working with streams allows for concise and expressive code, promoting code readability and maintainability. Moreover, streams support pipelining operations, enabling developers to chain multiple functions to transform data seamlessly.
Intermediate and Terminal Operations
The distinction between intermediate and terminal operations in the Stream API is crucial for understanding stream processing. Intermediate operations, such as map and filter, transform the stream elements and return a new stream without triggering their execution. On the other hand, terminal operations, like forEach and reduce, initiate the stream processing and produce a result. Understanding the difference between these operations is essential to harness the full potential of the Stream API in Java 8.
Advanced Topics in Java
In the realm of Java 8 programming, understanding advanced topics is paramount to mastering the language completely. Advanced Topics in Java 8 delve into intricate concepts that showcase the depth and complexity of the language. These topics are designed to challenge developers and push their skills to new heights. By exploring Advanced Topics in Java 8, programmers can enhance their proficiency, solve more complex problems, and elevate their software development prowess. From Optional Class to Date and Time API, these advanced topics offer invaluable insights and tools for creating robust and efficient Java applications.
Optional Class
Handling Null Values
Handling null values is a crucial aspect of programming in Java, especially when dealing with uncertainties or missing data. The Optional Class in Java 8 provides a streamlined solution for handling null values effectively. By encapsulating a value as either present or absent, the Optional Class eliminates the risk of NullPointerExceptions, enhancing code reliability and robustness. This feature simplifies error handling and encourages developers to write cleaner and more resilient code. Embracing the Optional Class for handling null values fosters good programming practices and promotes code safety in Java 8 applications.
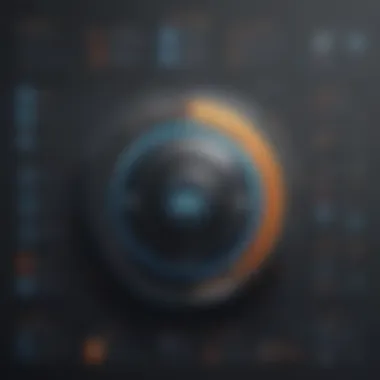
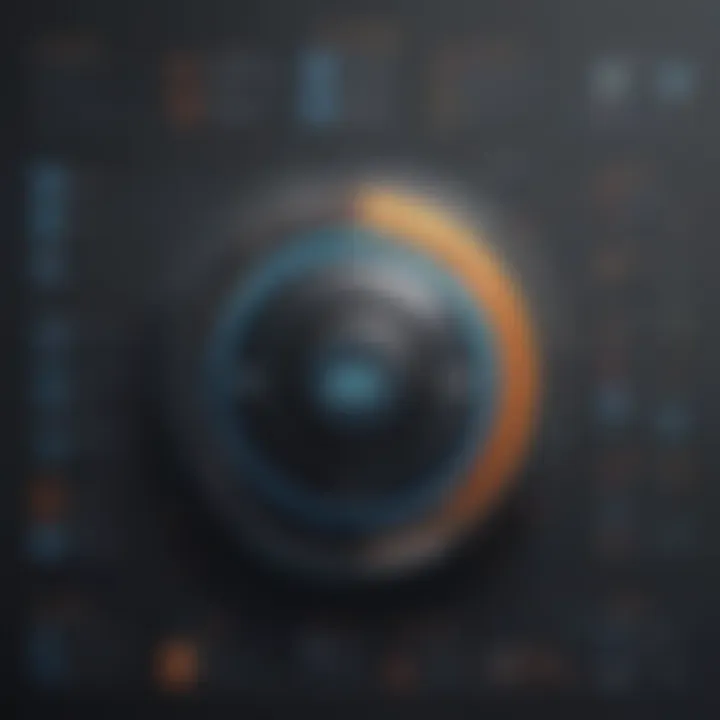
Use Cases and Examples
Exploring the use cases and examples of the Optional Class in Java 8 reveals its versatility and practicality in real-world scenarios. Developers can employ the Optional Class to refactor legacy code, improve readability, and streamline exception handling. By utilizing Optional in method parameters, return types, and collection operations, programmers can enhance code clarity and maintainability. Moreover, the Optional class promotes functional programming paradigms by encouraging the use of map, filter, and flatMap operations. Leveraging the Optional class in Java 8 empowers developers to write concise, expressive, and error-resilient code, optimizing their software development process.
Date and Time API
LocalDate and LocalDateTime
The Date and Time API in Java 8 introduces LocalDate and LocalDateTime classes to address the complexities of date and time manipulation. LocalDate represents a date without a time component, while LocalDateTime combines date and time information. These classes offer developers a modern and comprehensive approach to working with date and time values, allowing for precise calculations and formatting. In Java 8, LocalDate and LocalDateTime enhance date-related operations, such as parsing, comparison, and arithmetic, simplifying the handling of temporal data in applications.
Formatting Dates
Formatting dates is a crucial aspect of software development, ensuring that date values are presented clearly and consistently to users. The Date and Time API in Java 8 provides versatile options for formatting dates according to specific patterns and locales. Developers can customize date formatting with ease, catering to diverse user preferences and regional conventions. Formatting dates using the Date and Time API enhances the user experience, making applications more user-friendly and professional. By leveraging the formatting capabilities of Java 8's Date and Time API, programmers can create dynamic and visually appealing date representations in their software projects.
Best Practices and Performance Tips
Best Practices and Performance Tips play a crucial role in this article, offering invaluable insights for beginners and intermediate learners diving into Java 8 programming. Understanding the significance of efficient coding practices and performance optimization is essential to mastering software development. By focusing on specific elements like code readability, efficiency, and maintainability, developers can enhance the quality of their programs. Incorporating best practices ensures standardized coding patterns, reducing errors and promoting collaboration among team members. Performance tips, on the other hand, guide developers in improving the runtime behavior and memory utilization of their applications. By outlining considerations such as algorithm efficiency, resource management, and system scalability, this section equips readers with the necessary tools to write optimized Java 8 code.
Effective Java Coding
Avoiding Common Pitfalls
When it comes to 'Avoiding Common Pitfalls' in Java 8 coding practices, developers must pay particular attention to prevalent mistakes that can hinder program functionality and performance. By identifying and steering clear of common pitfalls like null pointer exceptions, memory leaks, and inefficient data structures, programmers can ensure the robustness and reliability of their code. Emphasizing defensive programming techniques and rigorous testing procedures can significantly reduce the occurrence of such issues, leading to more stable and efficient software implementations. Focusing on error handling, input validation, and code refactoring strategies enables developers to create resilient Java 8 applications that deliver consistent performance and user satisfaction.
Optimizing Code
In the realm of 'Optimizing Code,' developers delve into enhancing the efficiency and effectiveness of their Java 8 programs. Prioritizing optimization techniques such as algorithmic improvements, caching mechanisms, and resource utilization strategies can significantly boost the overall performance of software applications. By identifying and fine-tuning inefficient code segments, developers can eliminate bottlenecks and streamline program execution. The key characteristic of code optimization lies in achieving maximum functionality with minimal system resources, striking a balance between speed, memory footprint, and scalability. Leveraging advanced profiling tools and performance metrics aids developers in identifying optimization opportunities and implementing tailored solutions to enhance code performance.
Performance Tuning
Benchmarking Streams
'Benchmarking Streams' in Java 8 involves assessing the efficiency and reliability of stream processing operations within software applications. Conducting thorough performance benchmarks helps developers evaluate the speed, memory usage, and processing capabilities of Java 8 streams. By comparing different stream processing techniques and analyzing their impact on application performance, developers can make informed decisions to optimize code execution. The key characteristic of benchmarking streams lies in quantifying the performance metrics of various stream implementations, identifying bottlenecks, and proposing enhancements to streamline data processing operations.
Improving Application Efficiency
The aspect of 'Improving Application Efficiency' encompasses strategies and practices aimed at enhancing the overall performance and responsiveness of Java 8 applications. By focusing on factors like algorithm optimization, database query tuning, and resource management, developers can significantly boost the efficiency of their software solutions. Implementing techniques such as asynchronous processing, thread pooling, and cache utilization facilitates smoother and faster application behavior. The unique feature of improving application efficiency lies in the ability to leverage scalability options, distribute workload effectively, and cater to increasing user demands seamlessly. Balancing performance enhancements with resource constraints ensures that Java 8 applications deliver optimal user experiences while maintaining operational stability and responsiveness.