Unlock the Power of Python If Statements: A Comprehensive Guide
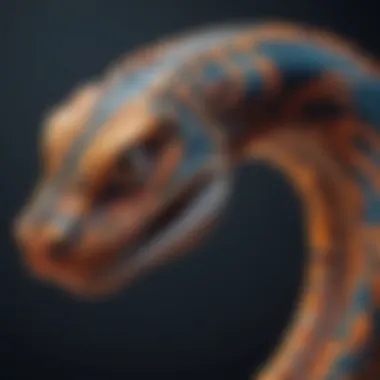
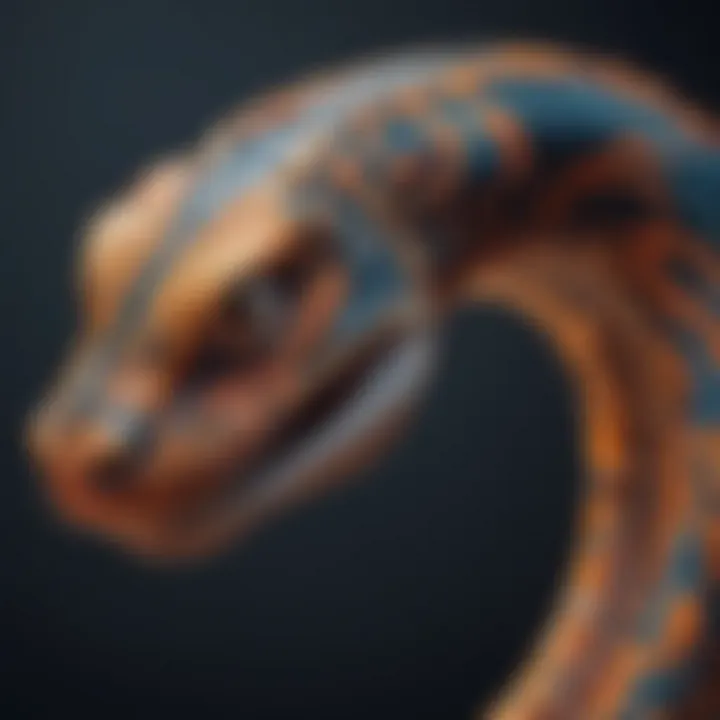
Resources and Further Learning
Embarking on a journey to master if statements in Python is a continuous process of learning and exploration. As learners progress in their programming endeavors, leveraging resources and opportunities for further learning becomes essential to staying updated with the latest trends and practices in Python development.
Recommended Books and Tutorials
Books and tutorials provide valuable insights and in-depth knowledge on mastering if statements and advancing one's Python skills. From beginner-friendly guides to advanced technical manuals, a plethora of resources are available to cater to various learning styles and expertise levels, offering comprehensive coverage of Python's syntax, features, and applications.
Online Courses and Platforms
Online courses and learning platforms offer interactive and engaging experiences for enthusiasts seeking structured learning paths and hands-on practice. With diverse courses covering if statements, programming concepts, and Python projects, learners can enhance their skills, collaborate with peers, and receive guidance from experienced instructors, fostering a supportive and dynamic learning environment.
Community Forums and Groups
Engaging with the Python community through forums, groups, and events opens doors to networking opportunities, knowledge sharing, and collaborative projects. By joining online communities like Reddit's rPython, Python Discord servers, and local Python user groups, programmers can seek advice, exchange ideas, and contribute to the vibrant Python ecosystem, expanding their horizons and nurturing their passion for programming.
Understanding If Statements
In the realm of Python programming, understanding if statements holds paramount importance. It serves as the cornerstone of implementing conditional logic, enabling programmers to dictate different paths for their code based on specified conditions. Grasping the nuances of if statements empowers developers to execute commands selectively, enhancing the overall efficiency and versatility of their programs. Through a detailed exploration of if statements, programmers can attain a robust foundation in controlling the flow of their Python code, a skill essential for mastering the art of programming.
Introduction to Conditional Logic
Definition of If Statements
The definition of if statements lies at the core of conditional logic in Python. An if statement is a programming construct that allows developers to execute a block of code only if a specified condition is met. This functionality provides programmers with the ability to create decision-making structures within their programs, enhancing the code's flexibility and responsiveness. By understanding the definition of if statements, programmers can efficiently control program flow, leading to more organized and coherent code structures that align with specific requirements.
Importance in Programming
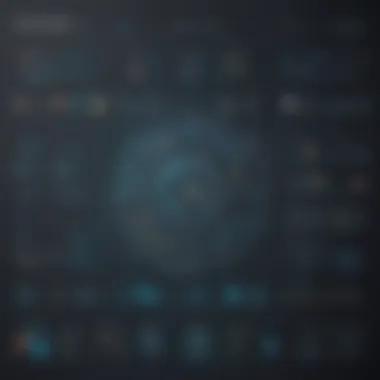
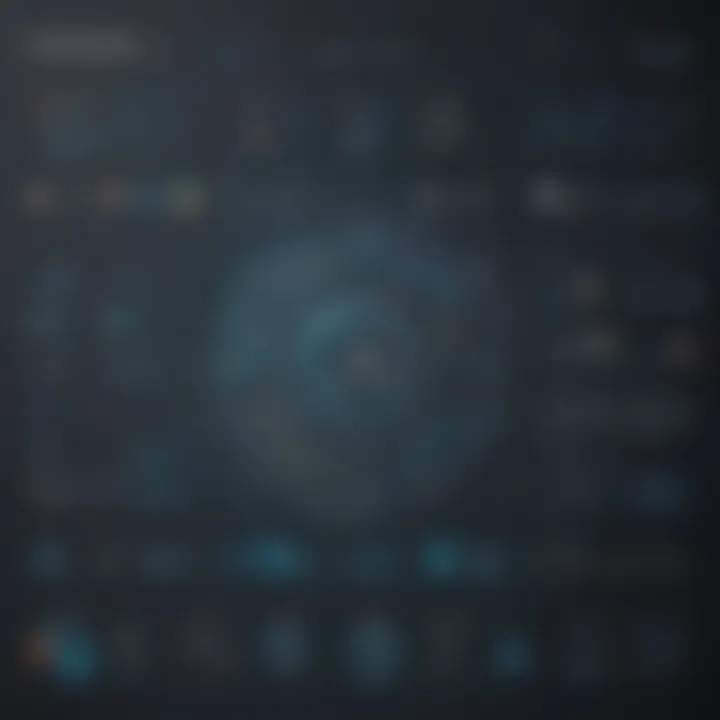
The importance of if statements in programming cannot be overstated. It serves as a fundamental building block for implementing logic and decision-making in codebases, enabling developers to create dynamic and interactive programs. By incorporating if statements, programmers can conditionally execute code based on user inputs, system states, or other defined criteria, adding a layer of intelligence and adaptability to their applications. Understanding the significance of if statements equips developers with the necessary skills to write efficient, responsive, and logical code that caters to diverse scenarios and requirements.
Basic Syntax of If Statements
Syntax Rules
Mastering the syntax rules of if statements is crucial for writing efficient and error-free Python code. The syntax of an if statement typically consists of the keyword 'if,' followed by a condition that evaluates to either True or False. Understanding the syntax rules allows programmers to structure their code effectively, ensuring that conditions are formulated correctly and actions are triggered appropriately. By honing their grasp of syntax rules, developers can write clear and concise if statements that enhance the readability and maintainability of their codebases.
Comparison Operators
Comparison operators play a vital role in defining the conditions within if statements. These operators, such as '==,' '!=,' ',' '', '=,' and '=,' enable programmers to compare values and determine the flow of their programs based on the results. Knowing how to leverage comparison operators empowers developers to create complex conditional expressions, facilitating precise decision-making within their code. By delving into the nuances of these operators, programmers can construct elaborate if statements that capture a wide range of scenarios and possibilities, contributing to the robustness and versatility of their applications.
Logical Operators in If Statements
AND, OR, NOT
Logical operators like AND, OR, and NOT allow developers to combine multiple conditions within if statements, expanding the scope of decision-making in Python programs. These operators enable programmers to build intricate logic structures by specifying how different conditions should interact to trigger specific actions. By mastering the application of logical operators, developers can create sophisticated if statements that cater to intricate scenarios and requirements. Understanding the behaviors of AND, OR, and NOT within if statements is essential for writing code that responds intelligently to various stimuli and inputs.
Combining Conditions
The ability to combine conditions in if statements provides developers with the flexibility to address complex scenarios in their programs. By merging multiple conditions using logical operators, programmers can create elaborate decision trees that accurately reflect real-world requirements. This capability allows developers to orchestrate intricate logic flows, ensuring that their code behaves as intended under a myriad of conditions. By exploring the techniques for combining conditions within if statements, programmers can craft robust and adaptive applications that exhibit a high degree of responsiveness and intelligence.
Implementing If Statements
When delving into the world of Python programming, understanding and mastering If Statements is crucial. Implementing If Statements allows programmers to introduce conditional logic into their code, enabling them to execute specific blocks of code based on predetermined conditions. This section serves as a gateway to harnessing the power of decision-making in Python programs efficiently.
Single-Line If Statements
Syntax and Examples
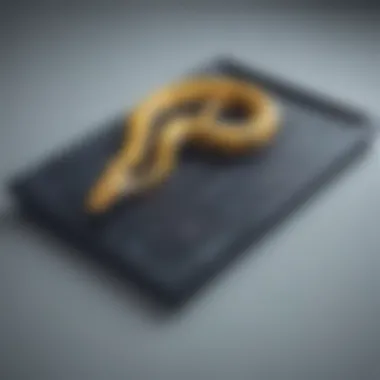
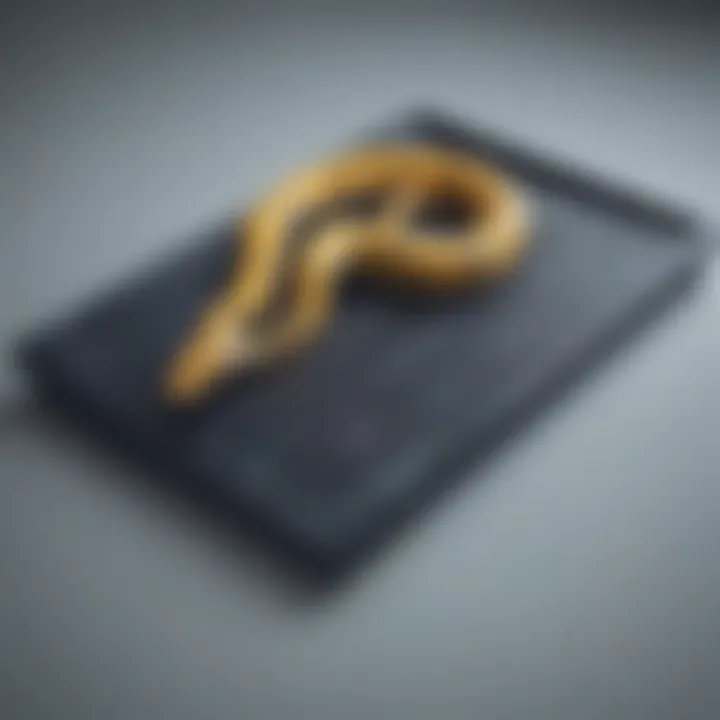
Single-Line If Statements, with their concise syntax and immediate execution, offer a streamlined approach to conditionally run code. The key characteristic of Single-Line If Statements lies in their ability to condense simple conditional checks into a single line, enhancing code readability and reducing complexity. This concise structure proves beneficial when quick decisions are to be made based on straightforward conditions. While offering brevity and efficiency, Single-Line If Statements may lack readability for complex conditions due to their compact nature, a trade-off that programmers must consider when leveraging this syntax.
Multi-Line If Statements
Indentation Rules
In Python, Indentation Rules play a pivotal role in Multi-Line If Statements, determining the scope of conditional blocks. The indentation signifies the beginning and end of code blocks, enforcing a structured and organized coding style. By adhering to indentation rules, programmers ensure code clarity and maintainability, reducing the risk of logic errors and enhancing code comprehensibility. This approach, although demanding strict adherence, results in code that is visually appealing and easier to debug.
Nested If Statements
Nested If Statements introduce a hierarchical decision-making process, allowing for the evaluation of multiple conditions within one another. The key characteristic of Nested If Statements is their ability to handle complex relationships between conditions, offering a layered approach to decision-making. While providing flexibility in designing intricate logic flows, nested structures can lead to code complexity and diminish readability if not implemented thoughtfully. Programmers must strike a balance between nesting levels to maintain code clarity and logic flow.
Chaining If-Elif-Else Statements
Usage and Best Practices
Chaining If-Elif-Else Statements streamlines decision-making by sequentially evaluating multiple conditions until a true condition is met. This method enhances code interpretability by presenting a structured approach to handling various scenarios. The key characteristic of this approach lies in its ability to prioritize conditions, ensuring that only the relevant block of code is executed. By following best practices in chaining conditions, programmers can create succinct and logical code structures, promoting maintainability and readability.
Advanced Techniques
Short-Circuit Evaluation
Explanation and Examples
Short-circuit evaluation is a pivotal concept in Python programming, where logical expressions are evaluated selectively to enhance performance. This method allows the interpreter to skip unnecessary evaluations once a certain condition is met, optimizing code execution. A significant characteristic of short-circuit evaluation is its efficiency in processing complex conditional statements, especially when dealing with multiple conditions. By strategically using short-circuit evaluation, programmers can boost the speed and performance of their code, making it a popular choice for optimizing if statements within Python programs. While the feature enhances runtime efficiency, it is essential to carefully consider its application to avoid unintended side effects that may impact the program's logic negatively.
Ternary Operator
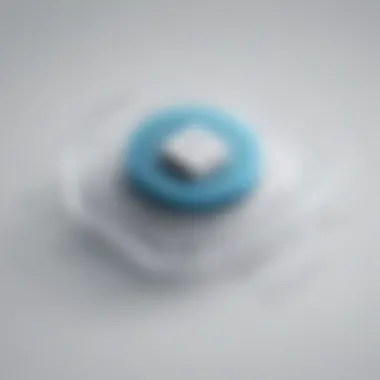
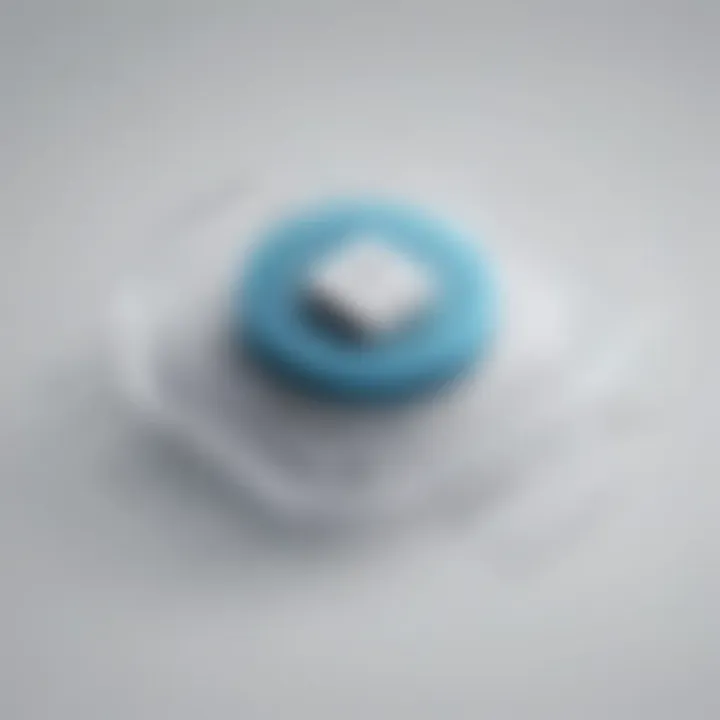
Syntax and Applications
The ternary operator in Python serves as a concise and pragmatic way to write ifelse statements in a single line, simplifying code structure and improving readability. This operator's key characteristic lies in its ability to condense conditional statements into a compact form, making code more concise and intuitive. In this article, the ternary operator offers a beneficial approach to handle conditional expressions efficiently, particularly in cases where brevity and simplicity are prioritized. Although the ternary operator aids in code optimization and clarity, it may pose limitations in readability for complex conditions, requiring a balance between succinctness and comprehensibility within the codebase.
Using If Statements with Functions
Passing Arguments
Integrating if statements with functions enables programmers to control the flow of logic based on input parameters passed to the function. Passing arguments plays a fundamental role in customizing function behavior, allowing for dynamic responses within the code. This aspect enhances the adaptability and versatility of functions, catering to various scenarios and requirements. The key characteristic of passing arguments is its capability to inject flexibility into the functions, tailoring their responses based on specific conditions. By leveraging passing arguments effectively, developers can create highly adaptable and responsive functions that cater to diverse use cases. However, it is crucial to manage argument passing carefully to maintain code clarity and avoid overcomplicating the function interface.
Returning Values
Integrating return values with if statements and functions facilitates the extraction of results based on defined conditions, enhancing the output customization of functions. This feature enables functions to provide different outputs based on varying conditions, increasing the versatility and usability of code components. The key characteristic of returning values is its role in defining varying outcomes within functions, aiding in decision-making processes and result customization. By judiciously utilizing return values in conjunction with if statements, developers can streamline logic flow and output generation, contributing to more efficient and adaptive code structures. However, careful consideration is necessary to ensure consistent and coherent handling of return values to maintain code predictability and reliability.
Common Pitfalls and Best Practices
Error Handling
Handling Indentation Errors
Handling indentation errors stands as a pivotal aspect of effective coding in Python. The indentation error is a distinctive characteristic that emphasizes the significance of properly aligning code blocks in Python. By addressing indentation errors promptly, developers can maintain the logical structure of their code, thereby preventing misinterpretations by the Python interpreter. Recognizing and rectifying these errors contribute to improved code readability and execution, fostering better programming practices within the Python environment.
Avoiding Ambiguity
In the context of if statements, avoiding ambiguity plays a critical role in enhancing the clarity and precision of your code. Ambiguity can lead to confusion and erroneous outcomes, potentially impacting the functionality of your program. By adhering to clear and concise logic in your conditions, you eliminate room for misinterpretation and reduce the likelihood of unexpected results. Avoiding ambiguity promotes code stability, ensuring that your Python scripts operate as intended, thereby streamlining the development process for optimal outcomes.
Code Readability
Using Descriptive Conditions
Utilizing descriptive conditions in your code contributes significantly to its readability and comprehensibility. Clear and descriptive conditions provide insight into the logic and decision-making process within your if statements, making the code more accessible to both developers and collaborators. By employing descriptive conditions, you can enhance the maintainability of your codebase, facilitating easier troubleshooting and modification when necessary. This practice aligns with industry standards for code documentation and promotes effective communication among team members, fostering a collaborative programming environment.
Optimizing Code Structure
Optimizing code structure serves as a fundamental practice in enhancing the efficiency and organization of your Python programs. By structuring your code logically and systematically, you can improve its scalability and maintainability, promoting long-term sustainability. Well-optimized code structure streamlines the development process, allowing for easier code maintenance and expansion as your projects evolve. This approach enhances code performance and readability, underscoring the importance of thoughtful design and planning in maximizing the effectiveness of your if statements in Python.