Mastering the Go Language: A Comprehensive Guide
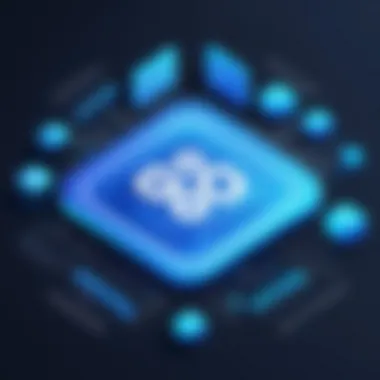
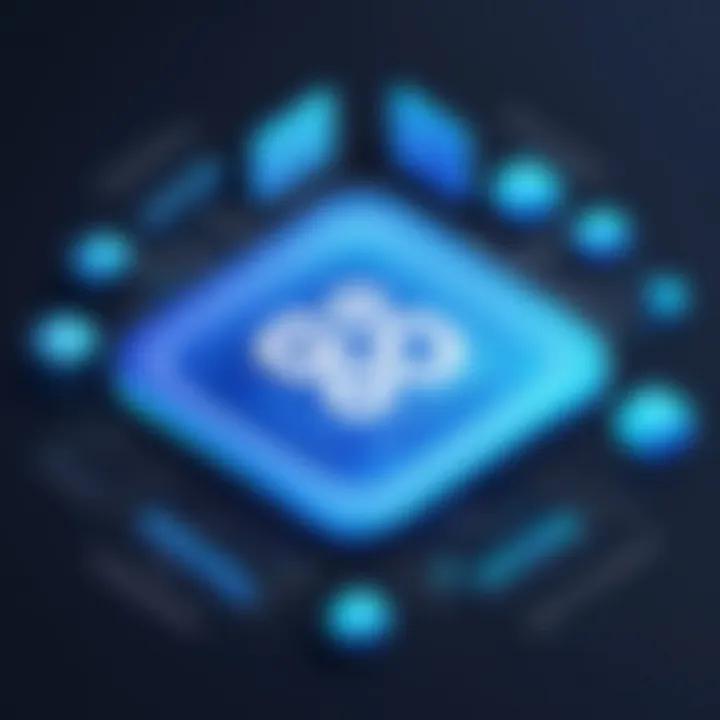
Intro to Programming Language
In the ever-evolving landscape of software development, the Go programming language, often referred to as Golang, has emerged as a powerful tool for modern application building. Originating at Google in 2007, this language was created to address the challenges that developers faced with existing languages. Its first public release came in 2012, marking the beginning of a journey that has gained significant momentum.
Go's design prioritizes simplicity and efficiency, enabling developers to code faster without sacrificing performance. It combines the speed of low-level languages like C with the ease of high-level languages, ensuring that both system-level programming and large-scale web applications can be efficiently developed.
History and Background
The inception of Go can be traced back to frustrations with long compile times and dependency management in other programming environments. The language was born from the desire to streamline these processes while preserving a level of robustness. Some of the architects of Go include notable figures such as Robert Griesemer, Rob Pike, and Ken Thompson, who aimed to create a language that would stand the test of time in a programming world often riddled with complexity.
Features and Uses
One of Go's standout features is its strong concurrency model, which allows developers to run multiple processes simultaneously without running into common threading mishaps. This is achieved through Goroutines and channels that simplify the management of concurrent tasks.
Beyond concurrency, Go boasts features like static typing, garbage collection, and powerful standard libraries. These attributes make it well-suited for a variety of applications, including:
- Web development (using frameworks such as Gin or Echo)
- Cloud computing and microservices
- Command-line tools
- Network programming
Popularity and Scope
Go’s popularity has surged, evidenced by its recognition in various programming communities, particularly on platforms like GitHub. It ranks highly on Stack Overflow’s developer survey as a language that developers are eager to learn, showing a promising trajectory for future growth. Its extensive use in major companies like Google, Dropbox, and Docker showcases its practical utility in both startups and established organizations alike.
In summary, mastering the Go programming language presents an invaluable opportunity for developers. As we delve deeper into the specifics of Go programming, understanding its foundational concepts, structures, and practical applications is essential.
"In a world with an increasing demand for developers, mastering languages like Go puts you ahead of the curve in a competitive job market."
Basic Syntax and Concepts
Understanding the basic syntax of any programming language forms the bedrock on which further knowledge can be built. For those stepping into Go, the language's simplicity will be refreshing.
Variables and Data Types
In Go, declaring a variable is straightforward. You use the keyword followed by the variable name and type. Here’s a quick example:
Go supports several data types including:
- Integers (int, int32, int64)
- Floating-point numbers (float32, float64)
- Strings (string)
- Booleans (bool)
Operators and Expressions
Operators in Go are similar to those in other languages, handling arithmetic, comparison, and logical operations. Here’s how you can perform basic addition:
Control Structures
Control structures determine the flow of the program. Go has several managing structures like , , , and looping with . An example of a simple statement is:
With a solid foundation in Go’s basic syntax and constructs, we can venture into more advanced topics.
Prolusion to Go Language
Go, or Golang as some prefer to call it, has emerged as a significant language in the coding landscape. For those of you diving into programming, understanding Go’s core principles, historical roots, and unique features can lay the groundwork for a successful journey into software development. The importance of this section lies in illuminating the landscape of Go programming, providing context guiding your future explorations.
Historical Context
Go was introduced by Google in 2009, a product born from the desire to address shortcomings observed in other programming languages. The architects of Go included notable figures like Robert Griesemer, Rob Pike, and Ken Thompson. They set out to create a language that combined the efficiency of lower-level languages like C with the ease of use found in higher-level languages like Python.
Before Go, developers faced cumbersome processes when building software systems, especially those dealing with concurrency and multi-core processing. Before long, Go started to catch on due to its minimalist design and fast execution. The decision to leverage familiar syntax while introducing new paradigms also contributed to its rising popularity.
Over time, Go has evolved, showcasing robust performance, particularly in cloud computing and microservices. Its emphasis on simplicity—both in syntax and functionality—has helped foster a community keen on collaboration and productivity. Many companies, large and small, have adopted Go, from cloud giants like Amazon and Microsoft to startups developing innovative applications.
Why Learn Go?
Now you might wonder, with so many programming languages out there, what makes learning Go worth your time? Here are a few compelling reasons:
- Performance: Go compiles to machine code, providing exceptional performance similar to C/C++ without their complexities. This makes it an excellent choice for performance-critical applications.
- Concurrency Made Easy: Go’s goroutines and channels simplify the process of concurrent programming. This means you can build responsive applications and services without getting tangled in complex threading issues.
- Simplicity and Clarity: The philosophy behind Go is about enhancing developer productivity. Its straightforward syntax and structure make it easier to read and maintain code, an attractive aspect for budding programmers.
- Strong Community Support: The growth of Go has fostered a vibrant ecosystem full of libraries, frameworks, and community resources. Whether you’re facing a challenge or seeking information, you’re likely to find help.
- Career Opportunities: Knowing Go is increasingly becoming a valuable asset. Many companies seek Go developers, especially in fields like DevOps and cloud engineering. This experience could open doors to exciting job prospects.
This combination of traits establishes Go not just as a language, but as a practical tool for building efficient systems in our increasingly digital world, making it quite relevant for anyone serious about programming.
"In the world of programming languages, Go stands tall due to its unique blend of simplicity, efficiency, and powerful concurrency mechanisms."
By the end of this guide, it’s expected you’ll have a clearer understanding of what Go offers and why it’s a language worthy of your time. To grasp its fundamentals further, it’s crucial you approach it with an open mind, ready to explore its depths.
Fundamental Concepts of Go
Understanding the fundamental concepts of Go is vital for diving deeper into the language and making the most of its features. Go, or Golang as it’s sometimes called, is lauded for its simplicity and efficiency, making the learning process easier, especially for those new to programming. Grasping the foundational principles is like laying the groundwork for a sturdy building; without it, complex structures simply won’t hold.
Basic Syntax and Structure
The basic syntax and structure of Go help set it apart from many other programming languages. At its core, the syntax revolves around readability and simplicity. When you first open a Go file, you’re greeted with a clean layout that emphasizes clarity. For instance, Go uses a straightforward naming convention for variables and functions which makes it easier to understand what each piece of code is doing.
Here’s a sample Go function that illustrates some basic syntax:
Notice how each line serves a clear purpose? The statement indicates which package the code belongs to, while lets us include libraries to simplify tasks. In this example, is a standard library for formatting I/O.
Another important aspect is how Go handles comments. There are single-line comments using and multi-line comments that are enclosed in . This allows developers to include notes or explanations without cluttering the code. Such features make the language approachable, instilling confidence in beginners as they learn by doing.
Data Types and Variables
In Go, understanding data types and how to declare variables is essential. The language has several built-in data types, such as integers, floats, booleans, and strings. This selection allows for a broad range of operations while keeping performance in check.
Declaring variables is straightforward. You can use the keyword, or with Go’s shorthand, you can use the operator for type inference. Here’s an example to illustrate the point:
This flexibility means you can choose the method that fits best depending on the context. The option to declare multiple variables simultaneously also streamlines code, enhancing readability:
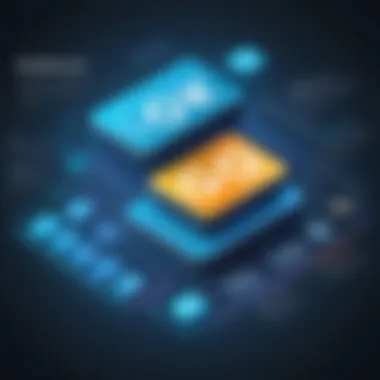
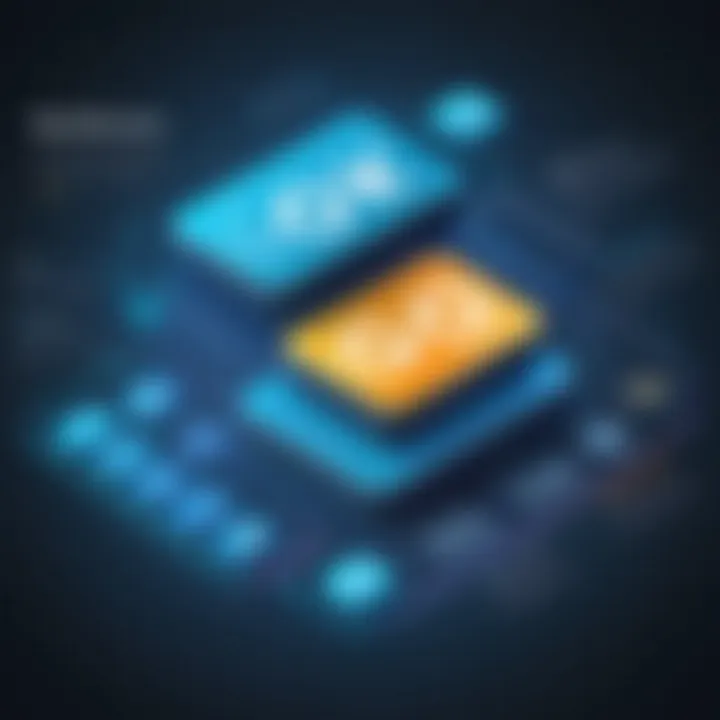
Being familiar with data types and variable declaration is a stepping stone toward more complex programming as it lays the basis for data manipulation and function parameters.
Control Structures
Control structures in Go permit developers to dictate the flow of programs in a logical manner. They include decision-making constructs like statements, loop structures like , and switch cases. Mastery over these structures can dramatically enhance code effectiveness and adaptability.
Consider the statement in Go:
It reads cleanly and allows for straightforward branching, which is fundamental in programming. As for loops, Go employs the statement, which can replicate functionality seen in loops from other languages. This versatility supports diverse programming needs:
Being comfortable with control structures means you can manage program execution flow effectively, allowing for the creation of complex logic within applications.
It's worth noting that Go encourages simplicity, so even complex tasks can often be accomplished in a clear fashion.
Effective Learning Strategies
Effective learning strategies are the backbone of mastering the Go programming language. In a landscape awash with programming languages, Go stands out for its simplicity and efficiency. Yet, diving into it can feel like being thrown into the deep end of a pool without a life jacket. That’s where these strategies come into play, guiding learners through the waters of Go with purpose and direction.
Firstly, setting structured goals makes a world of difference. When you know what you want to achieve, you can create a roadmap that suits your needs. This approach not only enhances motivation but also helps maintain focus. Learning without a clear destination leads to drifting. Therefore, breaking down the vast world of Go into smaller, digestible sections can lead to more profound understanding.
Equally important is the matter of resources. The internet is a treasure chest of information, but not all resources are created equal. Choosing the right ones saves time and fosters a more effective learning experience. An abundance of tutorials, courses, and community forums exists, but being discerning can make all the difference. Learning strategies must take into account the varied learning styles of individuals as well.
"Good strategies do not just help in setting out goals and choosing resources, they can also create a sense of community, connecting learners to one another."
As we look closer, it becomes evident that blending these two elements can forge a sustainable learning path, paving the way for a solid grasp of Go's capabilities and, ultimately, its application.
Setting Learning Goals
The practicability of setting learning goals is paramount in the journey to master Go. These goals act like signposts, providing direction and ensuring that learners have a clear focus on what they want to achieve. Without specific goals, progress can feel aimless.
To start, consider asking questions like: What aspect of Go do I find fascinating? Is it the concurrency model? The simplicity of syntax? Or perhaps the ability to build scalable web applications? Pinpointing these interests can steer your learning process more effectively.
Here are a few strategies for setting effective learning goals:
- SMART Goals: Specific, Measurable, Achievable, Relevant, and Time-bound. This framework aids in crafting goals that have substance and purpose.
- Short-term and Long-term Goals: Establish what you want to achieve in the near future as well as in the long haul. This layering helps maintain a balance between immediate outcomes and broader aspirations.
- Reflect on Progress: Periodically reviewing your goals can provide new insights and adjustments as needed. This reflection can keep your learning experience both dynamic and relevant.
Choosing the Right Resources
Navigating the world of resources available for learning Go can be overwhelming. With countless options at hand, it is crucial to filter what will provide the most value. Having the right materials means you're not just spinning your wheels, but actually making tangible progress.
When evaluating resources, consider these factors:
- Quality of Content: Look for materials that are well-reviewed and respected within the Go community. A reputable course or book can often be worth its weight in gold.
- Language and Style: Resources that match your language proficiency will make understanding concepts more attainable. If you struggle with jargon and complex explanations, you may lose interest quickly.
- Format Preference: Everyone grasps information differently—some may prefer video tutorials, while others benefit more from hands-on exercises or reading.
- Community Engagement: Resources that encourage interaction, such as forums or group projects, can enhance the learning experience. Consider platforms that integrate discussion sections or peer reviews.
Practical Exercises for Mastery
In the journey of mastering the Go programming language, engaging in practical exercises stands as one of the most vital components of effective learning. It's easy to consume theory, but applying knowledge through hands-on experiences solidifies understanding. Practical exercises not only enhance computational skills but also equip learners with the ability to think critically and troubleshoot programs creatively.
Coding Challenges
Coding challenges serve as a bridge between learning concepts and practical application. These challenges push a developer to think outside the box, encouraging them to apply their knowledge under constraints. Whether it's solving problems on platforms like LeetCode, HackerRank, or Codewars, each of these platforms presents unique problems that require innovative solutions.
- Concept Reinforcement: By tackling these challenges, learners review and reinforce basic syntax and data structures. They become adept at honing in on logic and control structures in real scenarios.
- Timed Environment: Coding challenges often simulate real-world stressors, such as time constraints, helping participants manage their skills under pressure. It builds mental resilience, which is crucial in any programming career.
- Score Tracking: These platforms typically track progress, allowing learners to see how they stack up against peers.
Coding challenges can range from simple exercises, like writing a function to check for prime numbers, to more complex algorithms involving data manipulation. They often include a wide array of difficulty levels, making them adaptable for all stages of learners. Plus, as you engage in these challenges, you'll discover new tricks and approaches that sharpen your programming arsenal.
Project-Based Learning
Project-based learning takes it a step further. Rather than solving isolated problems, learners are immersed in entire projects that encourage them to think like developers. This approach provides a tangible end-result, which further deepens understanding and retention of knowledge.
- Real-World Application: Building a project, be it a web app using Go's net/http package or a command-line tool with flags and arguments, links theory to practice. It helps learners grasp how Go interacts with other systems and libraries.
- Portfolio Development: Each project can be showcased in a portfolio, which will be invaluable for future job applications. This isn't just practice; it's creating work that can impress potential employers.
- Collaboration & Version Control: Many projects require collaboration, pushing learners to refine their soft skills. Tools like GitHub allow you to not just manage code but also collaborate effectively with others, replicating real work environments.
Taking on an ambitious personal project can help solidify a learner's confidence. They might build a simple web service or automate a repetitive task with Go. This not only helps in gaining proficiency but also serves as a launching pad for personal interests in technology.
To sum it up, practical exercises—like coding challenges and project-based learning—are non-negotiable in mastering Go. They push learners to explore beyond the books and tutorials, fostering a deeper connection with the language. As you embark on this journey, remember:
"Practice does not make perfect. Perfect practice makes perfect."
This adage rings true. So, the next time you sit down to learn Go, prioritize your practical exercises; they hold the key to mastering the language.
Leveraging Online Resources
In today’s digital age, tapping into online resources becomes essential for anyone looking to learn Go programming. The vast array of online materials not only supplements the traditional methods of learning but also makes it possible to acquire knowledge at one’s own pace and convenience. Understanding how to effectively utilize these resources can greatly accelerate your journey towards mastering Go.
Online Courses and Tutorials
Online courses have paved the way for self-guided learning, providing structured paths that are often hard to find elsewhere. Platforms like Udemy and Coursera host a myriad of courses, tailored to various skill levels, from complete beginners to seasoned programmers. Here are some tangible benefits of these online offerings:
- Structured Learning: Courses typically follow a logical sequence, ensuring that you build on your knowledge gradually.
- Variety of Formats: Whether you prefer videos, written materials, or interactive content, there’s a resource out there catering to your preferred learning style.
- Access to Experts: Often, courses are led by experienced instructors who can offer insights that might not be easily accessible through books or blogs.
A few courses truly standout:
- "Learning Go" on Coursera: This provides a comprehensive overview of the language and its key concepts.
- "Mastering Go" on Udemy: A deeper dive into more complex topics, perfect for those already comfortable with the basics.
Furthermore, many tutorials available online complement these courses well. Websites such as Go by Example (https://gobyexample.com/) offer practical examples that can help solidify your understanding.
Community Forums and Discussion Groups
The value of engaging with online communities can’t be overstated. Platforms like Reddit (https://reddit.com/r/golang) and various forums dedicated to programming languages act as lively hubs for exchange and support. Connecting with other learners or seasoned professionals can provide several advantages:
- Problem Solving: Got stuck on a coding issue? Chances are someone else faced a similar problem. Forums serve as a great resource for troubleshooting and finding solutions.
- Networking Opportunities: Interactions often lead to connections within the industry, helping you to expand your professional circle.
- Real-World Insights: Community members often share their experiences and challenges, offering a glimpse into the practical applications of Go.
It’s worth investing time to engage with the community. Actively participating in discussions can deepen your understanding and expose you to new perspectives. Whether you’re asking questions or providing answers, this kind of interaction is invaluable.
Involvement in the developer community not only fosters learning but also keeps you updated on the latest trends and best practices in Go programming.
Leveraging these online resources effectively creates a rich learning environment that encompasses not just gathering information, but engaging with it on multiple levels. As you venture into these realms, your understanding and skills will grow, paving the way towards mastery in Go.
Advanced Go Topics
Diving into the realm of advanced Go topics marks a pivotal point in your understanding and application of the Go programming language. These subjects not only refine your expertise but also empower you to tackle complex programming challenges with confidence. Concurrency and error handling are two of the most crucial elements in Go, each serving specific needs and enhancing overall efficiency and robustness. Let's take a closer look at these essential topics, their benefits, and why they deserve your attention.
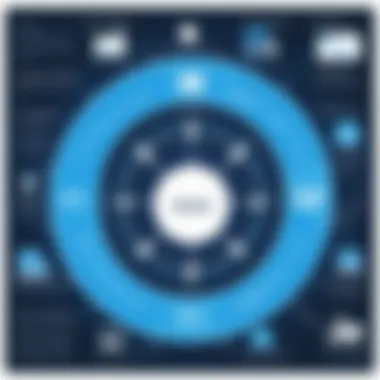
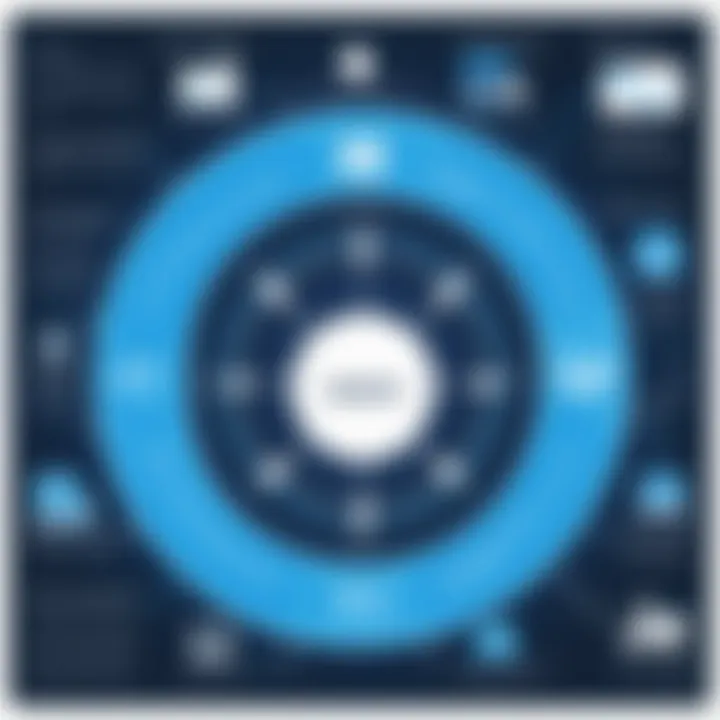
Concurrency in Go
Concurrency is often seen as the backbone of modern programming, and Go has integrated this concept into its very architecture. The ability to handle multiple tasks simultaneously, without the fuss of traditional threading models, gives programmers a significant edge. Through goroutines, Go lets you spin up lightweight threads with minimal overhead, allowing your applications to scale more seamlessly.
The advantages of mastering concurrency in Go are manifold:
- Efficiency: Goroutines are cheap; you can run thousands without breaking a sweat,
- Simplicity: With channels, managing communication between goroutines feels almost intuitive.
- Performance: Applications become more responsive, as blocking operations don't stall the whole program.
Implementing concurrency can be as mundane as having a separate goroutine for each background task:
This small snippet creates a simple interplay between the main function and a goroutine, demonstrating how the two can run without stepping on each other's toes.
Error Handling
Another cornerstone of programming that deserves your attention is error handling. Go's approach here may be a departure from conventional methodologies, but it brings a straightforwardness that many find appealing. Instead of relying on exceptions, Go opts for a multiple return value method, where functions return an error alongside the actual result. This design philosophy emphasizes clarity and forces the developer to consider error cases actively.
Mastering error handling in Go means:
- Predictability: It's easier to understand the flow of code when error handling is explicit.
- Control: You decide how and when to address issues, providing robust error management tailored to your application.
- Simplicity: Avoiding complex error propagation simplifies both writing and reading the code.
An example to illustrate this concept:
This code first checks for zero division, ensuring you get feedback when something goes amiss rather than letting your program crash unexpectedly.
Integrating Go with Other Technologies
Integrating Go with other technologies is essential as it broadens its application scope, giving developers the ability to create versatile and high-performing applications. With Go’s strong emphasis on concurrency and performance, its integration capabilities with various systems enhance its utility in modern software development. This section will explore two major areas of integration: databases and web development, shedding light on their significance and benefits.
Using Go with Databases
Databases are a crucial aspect of any application, as they store and manage data efficiently. Go offers powerful capabilities when it comes to database integration. Go's standard library includes the package, which provides a flexible interface for working with different database systems. Here are some notable points regarding Go's interaction with databases:
- Simplicity and Efficiency: Go’s strong suit is its simplicity. Connecting to a database can often be a breeze, thanks to the straightforward methods provided. Whether you're implementing a PostgreSQL, MySQL, or SQLite backend, clear documentation and community support make Go an attractive choice.
- Concurrency: Go’s goroutines allow for seamless handling of multiple database connections. This feature is particularly advantageous when dealing with web applications where requests to the database can be numerous and simultaneous.
- ORM Support: While Go doesn’t come with a built-in ORM (Object-Relational Mapping), popular libraries like GORM and SQLBoiler fill this gap. These tools reduce boilerplate code while ensuring that developers can interact with databases using Go's expressive syntax.
- Connection Pooling: Go's built-in features allow the creation of connection pools, which optimize the resource management necessary for heavy database interactions.
Implementing a database in Go is as simple as writing:
This snippet demonstrates how straightforward it can be to connect and execute queries against a database.
Web Development with Go
The realm of web development has embraced Go for its efficiency and scalability. It’s common to find Go employed in backend programming, handling everything from REST APIs to full-fledged web applications. Here’s why Go stands out in this field:
- Fast Performance: Go compiles directly to machine code, leading to rapid execution times which are critical for application performance, especially for web servers.
- Robust Standard Library: Go provides a powerful package that allows developers to create web servers with minimal code overhead. Building an HTTP server can be done in a matter of seconds.
- Microservices Architecture: The lightweight nature of Go improves service interactions in microservices architecture. Developers can easily create small, independent services that communicate over HTTP, enabling the building of scalable applications.
- User-Friendly: Go’s simplicity translates to quicker onboarding for new developers. This is particularly beneficial for startups or teams requiring agility in their development processes.
To illustrate the basics of web server setup in Go:
Here, a simple web server is implemented that responds with a "Hello, world!" message. The ease with which such servers can be set up plays into Go’s popularity in web development circles.
Go’s ability to integrate smoothly with databases and to power web applications makes it an essential tool in the developer's toolbox in today’s technology landscape.
Through these integrations, Go proves its flexibility and strength as a programming language capable of handling modern software requirements.
Testing and Debugging in Go
Testing and debugging in Go are not just tasks to perform; they are fundamental practices that elevate the quality of your code. In the world of programming, catching bugs before they escalate can mean the difference between a seamless application and an experience fraught with errors. Testing goes hand-in-hand with debugging, offering peace of mind that your code behaves as expected. Baker’s dozen of reasons exist to prioritize these tasks, but let’s highlight a few massive ones. It improves code reliability, makes the onboarding process easier for new team members, and enhances collaboration by providing shared understanding. All of which are crucial in today’s fast-paced development environments.
Writing Tests
Writing tests in Go involves a straightforward approach. Go has a built-in testing framework that allows developers to create unit tests, integration tests, and even benchmark tests seamlessly. Here's how to get going:
- Use the package: It’s included in the Go standard library. You simply import it like this:
- Create a test function: Functions should start with followed by a name that describes what is being tested. For example:
- Run the tests: You can run tests using the command line. Just type in the package directory that contains your tests, and it will execute them all at once.
- Test coverage: Go provides a great way to measure test coverage, too. When you run , it'll tell you how much of your code is being executed by tests.
Writing tests provides not just the benefit of identifying bugs early but also serves as living documentation for your code. It encapsulates what the code is supposed to do, helping both current and future developers.
Debugging Techniques
Debugging is an art unto itself. While writing tests helps prevent bugs, debugging is about getting to the bottom of issues that have already appeared. Here are some solid techniques anyone can adapt:
- Print Statements: Sometimes the classic approach works best. Placing print statements () to check variable values mid-execution can give immediate insights into what's going wrong.
- Use the Go Debugger: Go offers a debugger called Delve, which allows you to set breakpoints, inspect variables, and step through code. You start it by installing Delve using . Once set up, you can dive deep into the execution flow.
- Go with Error Handling: Go forces you to deal with errors explicitly. A well-placed error check can often lead you directly to the heart of explanations for unexpected behavior. For instance:
- Profiling: Sometimes you're not just dealing with logic errors, but performance issues. Using the Go tool for profiling can help you identify bottlenecks in your code. Running can give you insights into where your logic might be failing in terms of performance.
Debugging is not just about fixing errors; it's about understanding the system as a whole and ensuring that each part works harmoniously.
Career Prospects with Go
The relevance of Go in the current tech landscape cannot be stressed enough. As businesses continually search for efficient, scalable, and straightforward solutions, Go programming is increasingly becoming a hot commodity in the job market. This section explores what makes Go appealing in various industries, what potential job seekers might expect, and how to prepare for a successful career utilizing this language.
Job Market Overview
The job market for Go developers has seen remarkable growth. According to industry analyses, there’s an increasing demand for professionals proficient in Go. Major tech companies and startups alike are adopting Go for its exceptional performance and efficient concurrency handling. Sectors like cloud computing, financial services, and DevOps have recognized Go’s power in building robust applications.
Here are a few key trends to consider:
- Rising Popularity: Surveys show Go is becoming one of the top programming languages among developers. Increasingly employers are posting jobs that prefer Go expertise.
- Salary Expectations: Reports indicate that positions for Go developers often come with competitive salaries. An average Go developer’s salary can be higher compared to those using more traditional languages.
- Job Roles: Positions range from backend and web developers to DevOps engineers and cloud architects. The skill set demanded often combines knowledge of Go with containerization technologies like Docker and Kubernetes.
Given the versatility of Go, job seekers can find positions across various sectors, signaling a healthy outlook for aspiring programmers who invest time in mastering the language.
Preparing for Interviews
Job interviews can often feel like a gamble. However, when you prepare with the right strategies, you'll boost your chances of landing that job. Here are some tips on how to ace your Go-related job interviews:
- Understand Go Fundamentals: Make sure you have a grip on Go basics—understanding its syntax, data structures, and common libraries. Practice fundamental coding challenges that will likely come up.
- Show Practical Experience: Be ready to discuss projects where you’ve applied Go. Whether it's a personal endeavor or an open-source contribution, real-world application matters. Don't just talk the talk—walk the walk.
- Mock Interviews: Engage in mock interviews to get comfortable with common questions. You can use platforms like reddit.com or local meetups to find practice partners.
- Algorithm and Data Structure Questions: While specific language knowledge is crucial, you will frequently encounter algorithm challenges. Brush up on your problem-solving skills, focusing on how Go differs from other languages in handling such tasks.
- Stay Updated: Make sure to read up on the latest developments in Go. Knowing recent updates and community practices can impress interviewers and showcase your enthusiasm.
- Behavioral Questions: Don’t underestimate the importance of soft skills. Be prepared to discuss your problem-solving process, teamwork examples, and how you handle critique.
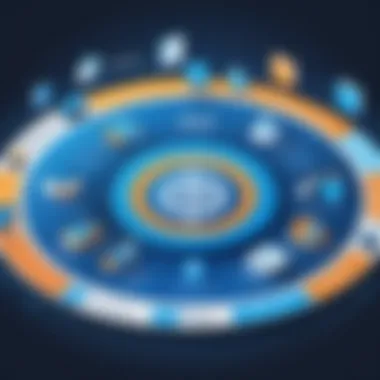
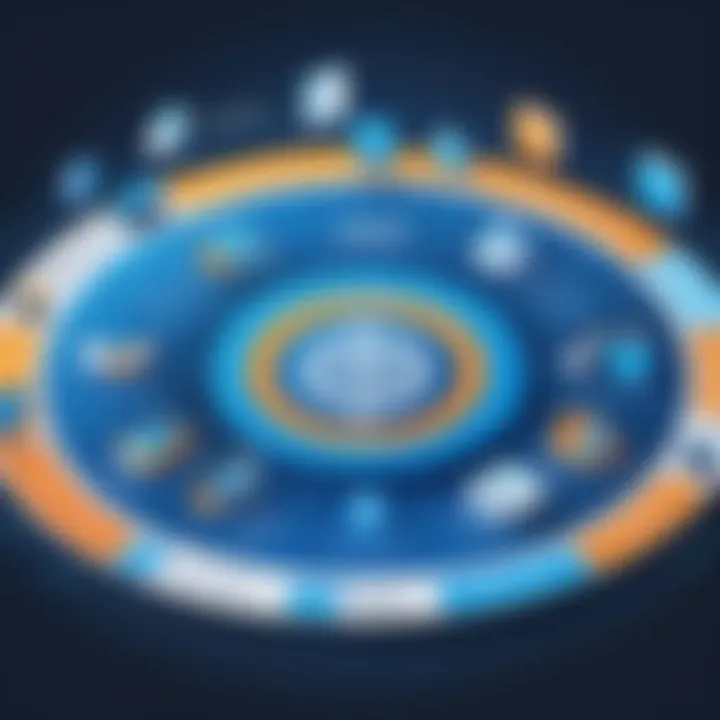
"It's not just about knowing how to code—companies want to see how you think."
Reflecting on these tips will not only make you a more attractive candidate but also prepare you for the dynamic world of technology using Go. As you continue to build your skills, remember that the path to landing a job in Go development requires perseverance, but the rewards in terms of career satisfaction and opportunities are well worth the effort.
Contributing to Open Source Projects
Contributing to open source projects holds particular significance for learners of the Go programming language. These projects not only act as a fertile ground for honing coding skills, but they also offer a chance to engage with the vibrant developer community. Dive more deeply, and you'll find that these contributions bring several compelling benefits.
First off, getting involved in open source projects can be a golden ticket for practical experience. Unlike theoretical exercises, working on real-world projects helps you understand how Go interacts with different technologies. You’ll often find that collaborating on code adds a layer of complexity that isn’t often captured in tutorials or lectures. This hands-on practice helps you internalize debugging and problem-solving in ways that books simply can’t teach.
Additionally, participating in open source not only builds your technical capabilities but also enhances your soft skills. Collaborating with others requires clear communication, consideration of feedback, and coordination in a team. These skills are often just as valuable as the coding expertise itself, especially when you transition to professional environments.
Another pivotal aspect is the professional networking opportunity that comes with open source contributions. You meet and work with other developers, some of whom might be industry veterans. Establishing connections through projects can open doors to future job opportunities. Being part of a project often means you’ll be more memorable to potential employers who value initiative and collaboration.
Lastly, contributing to open source can bolster your resume. You can showcase your work on platforms like GitHub, which provides employers an insight into your coding style and problem-solving approach. This leaves a lasting impression that mere credentials sometimes can’t replicate.
"Contributing to open source projects not only builds code but also builds skills, networks, and your professional identity."
Finding Projects
So, where do you start when it comes to finding open source projects to contribute to? The options can be a bit overwhelming, but here are some strategies to narrow down your search:
- Leverage GitHub: This is the go-to platform for open source projects. Use GitHub's search feature to filter repositories by language (Go), stars, and recent activity. Look for projects that align with your interests or that maintain a desire for contributors.
- Explore Awesome Go: The Awesome Go repository features a compilation of high-quality Go projects. This sorted list allows you to discover and explore different sectors ranging from web development to tools for developers.
- Join Go Community Forums: Platforms like Reddit or specialized Go forums often highlight projects seeking contributors. Engaging in these communities can also help you meet other developers with similar interests.
- Attend Meetups: Local or virtual coding meetups might promote specific projects needing help. It’s also a chance to meet project leaders and ask how you might contribute.
Finding the right project can sometimes feel like searching for a needle in a haystack, but following these tips can streamline the process.
Best Practices for Contributions
Once you’ve identified a project, how do you step up to the plate? Here are some best practices to ensure your contributions are effective and welcomed:
- Read Contribution Guidelines: Most projects have a CONTRIBUTING.md file that outlines how to contribute properly. Ignoring these can lead to wasted efforts.
- Start Small: Initially, tackle simple issues or documentation updates. This allows you to familiarize yourself with the codebase and create a good rapport with the project maintainers.
- Communicate: Before diving into larger issues, don't hesitate to ask for clarification or share your ideas. Open dialogue fosters a positive environment and generates teamwork.
- Follow Code Standards: Adhering to the established coding style of the project is crucial. Consistency in style enhances readability and keeps the code understandable for every contributor.
- Test Your Code: Before submitting your contribution, make sure to test it appropriately. Automated tests are often a part of open source projects, and running these ensures that your changes don’t break existing functionality.
- Submit Thoughtful Pull Requests: When you're ready to submit your changes, include a clear title and an engaging description that outlines what you've done and why.
By following these practices, you will increase your chances of successfully becoming a valued contributor. It's not just about the code; it's about building relationships and growing together in the Go ecosystem.
Building a Portfolio
Building a portfolio is a crucial step for any developer, especially in the world of programming languages like Go. It acts as a bridge between your skills and potential employers or clients, showcasing what you know and what you've done. In the tech industry, a well-curated portfolio can often speak louder than a resume. A strong portfolio not only demonstrates your technical capabilities but also reflects your creativity, problem-solving skills, and dedication to your craft.
When assembling your portfolio, consider including the following elements:
- Diverse Projects: Include a variety of projects that highlight different aspects of Go programming. Whether it’s a simple console application or a web server, showcasing diversity can display your range.
- Code Quality: Highlight projects with clean, well-structured code. Employers appreciate maintainability and readability, so make sure to follow best practices.
- Live Demonstrations: If possible, host your applications online. This allows potential employers to interact with your work firsthand, proving your competencies in a dynamic way.
- Documentation: Good documentation is a hallmark of a professional developer. It shows that you take your projects seriously and can communicate your ideas effectively.
In essence, a thoughtful portfolio not only serves to impress but also communicates your growth as a developer. As competition intensifies in the job market, an appealing portfolio can make the necessary difference.
Showcasing Projects
When it comes to showcasing projects in your portfolio, aim for quality over quantity. Here’s how to effectively present your works:
- Select Projects Wisely: Choose projects that best represent your abilities and interests. Ideally, select five to seven pieces where you can clearly show your expertise in Go.
- Detail Your Role: Explain your contributions in each project. Were you responsible for the entire project, or did you collaborate with others? Be clear about what you did and what technologies you utilized.
- Provide Context: Explain the problem each project was designed to solve. Context helps viewers understand the significance of your work and how it fits into real-world scenarios.
- Visuals Matter: Include screenshots or videos. Visual elements grab attention and can convey complex information rapidly.
- GitHub Links: Link to your actual code on platforms like GitHub. This allows others to see your code directly and assess your skills further.
"Your portfolio should tell a story, not just show your work. Engage the viewer with a narrative that connects each project."
Personal Branding Strategies
Once you have your projects organized and showcased, consider how you present yourself through your personal branding. This plays a pivotal role in how others perceive you within the Go community and the tech world at large.
- Consistent Online Presence: Maintain consistency across your social media profiles and professional platforms. Use the same handle where possible, and make sure your profiles are up to date. This establishes a cohesive identity.
- Create a Personal Website: A personal website acts as your online business card. Include your biography, contact information, and links to your work. Make it visually appealing and easy to navigate.
- Engage with the Community: Participate in Go-related community forums on platforms like Reddit or Stack Overflow. Share insights, answer questions, or write blogs. Visibility in these spaces builds your reputation as a knowledgeable individual in the field.
- Networking: Attend events and meetups. Networking face-to-face can be just as powerful as online engagement. When you meet others, it builds real connections that can help in your career development.
Ultimately, strong personal branding complements an effective portfolio. It portrays you as a well-rounded professional, giving you an edge in a competitive field.
Staying Updated with Go Developments
Keeping abreast of developments in the Go programming language is not merely a suggestion; it’s a necessity for anyone serious about mastering the craft. The tech landscape evolves at breakneck speed, and Go is no exception. Staying updated ensures that learners and developers are aware of the latest features, best practices, and community discussions that can enhance their programming skillset. There are a myriad of resources available, each offering distinct advantages that can greatly benefit learners at all stages.
"In programming, learning is continual. To be the best, you must stay informed and adaptable."
Some specific elements to consider include:
- New Language Features: Knowing about the latest updates and enhancements in Go can dramatically improve coding efficiency. For instance, understanding how to utilize the newer capabilities introduced in recent versions can make the difference between average and exceptional code.
- Best Practices: The Go community often discusses optimal ways of coding, so having your finger on the pulse can lead to more readable, maintainable, and performant code. Engaging with these discussions can help you understand subtext in coding conventions that textbooks may not cover.
- Networking Opportunities: Staying involved within the Go ecosystem allows for interaction with like-minded individuals and industry experts. This could lead to job opportunities or collaborations on projects. Networking often unveils doors that remain closed to those who do not engage.
All these benefits culminate to make a strong case for why one should invest time in staying updated with the language’s developments.
Following Key Contributors
In the realm of programming, few things are more enlightening than keeping tabs on the individuals who drive the conversation. Key contributors to the Go language often share insight that might not be readily available in conventional learning materials. Following these individuals on platforms like Twitter or GitHub offers a twofold benefit: you gain direct access to their wisdom while integrating yourself into the pulse of the coding community. A few notable figures include:
- Rob Pike: One of the founders of Go, his insights into design decisions can provide clarity on why the language operates as it does.
- Russ Cox: Another core contributor, often shares technical nuances and updates surrounding the Go language.
- Go Blog: The official Go blog curates thoughts from multiple contributors, explaining recent changes in detail. Following this can keep you well-informed about latest events.
To further amplify this knowledge, actively engaging with their posts allows for stimulated discussions and learning opportunities.
Participating in Events and Conferences
Conferences and events serve as the melting pot for new ideas and networking in the Go community. Events like GopherCon are not only about absorbing information; they provide opportunities to connect face-to-face with peers and influencers in the field. Here are some reasons why attending these events is instrumental:
- Workshops and Talks: Many conferences offer hands-on workshops led by experienced Go developers. These sessions allow you to gain skills quickly, often tailored to current industry needs.
- Community Networking: Engaging with fellow attendees can open further avenues. Whether it’s brainstorming ideas or collaborating on future projects, no connections are wasted in the programming world.
- First-Hand Experience of Trends: Conferences often showcase cutting-edge projects or future directions the language may take, which you wouldn’t typically see in literature. This firsthand information allows you to stay ahead of the curve.
You might find these events listed on platforms such as Reddit or the official Golang website for upcoming conferences.
Overall, both following key contributors and participating in events converge to keep your knowledge of Go current, relevant, and actionable.
The End
The conclusion of this guide is a vital piece for anyone venturing into the Go programming language. It serves as a snapshot, summarizing the core insights and emphasizing why Go holds a significant spot in the programming landscape today. By this point, readers have been exposed to various facets of Go, from its historical roots to advanced topics like concurrency. This section pulls all the threads together, reinforcing the key learnings.
Summarizing Key Points
Reflecting on everything discussed, several key points stand out:
- Foundational Concepts: Understanding Go's basic syntax, data types, and control structures is crucial for building a solid base. This makes it easier to tackle more complex topics later on.
- Effective Learning Strategies: Goal-setting and resource selection can dramatically affect your learning path. By choosing quality materials and defining achievable milestones, learners can navigate Go's vast resources more efficiently.
- Practical Exercises: Engaging with real-world projects and coding challenges is essential. This hands-on approach helps solidify knowledge and fosters problem-solving skills.
- Community Engagement: Joining forums, like those on Reddit and Facebook, can provide invaluable support. The shared experiences and insights from the Go community can enhance one’s learning journey, making it feel less daunting.
- Career Prospects: The job market for Go developers is robust, with companies increasingly recognizing the value of Go in modern cloud applications and system programming. A well-built portfolio can greatly improve job prospects in this field.
In summary, the consolidation of this knowledge primes learners for what comes next — deeper dives into Go and its many applications.
Next Steps in Learning Go
After grasping the fundamental aspects covered in this guide, what’s next? Here are some concrete steps to continue on the Go journey:
- Engage with Advanced Materials: Look into advanced Go topics, such as network programming, microservices, and refining concurrency skills. Resources may include online courses, books, or even coding boot camps.
- Contribute to Open Source Projects: Participating in open-source projects is a practical way to apply skills and connect with others in the community. Platforms like GitHub host many such projects that welcome contributions.
- Build Your Own Projects: Start small, perhaps with a personal project that interests you. Not only does this provide a canvas to apply what you've learned, but it also helps in developing a portfolio to showcase to future employers.
- Seek Mentorship: Engaging with someone experienced in Go can accelerate your learning. They can provide guidance, answer queries, and help navigate around common pitfalls.
- Stay Updated: The tech landscape evolves rapidly. Following Go's key players on social media or subscribing to relevant newsletters can keep you informed about the latest advancements and best practices.
In closing, mastering Go is an enriching journey filled with challenges and opportunities. Embrace it wholeheartedly, and the rewards will reflect your dedication.