Mastering Form Handling in JavaScript: A Complete Guide
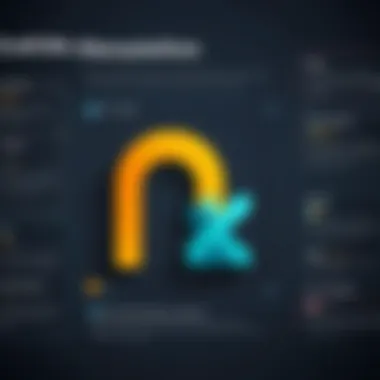
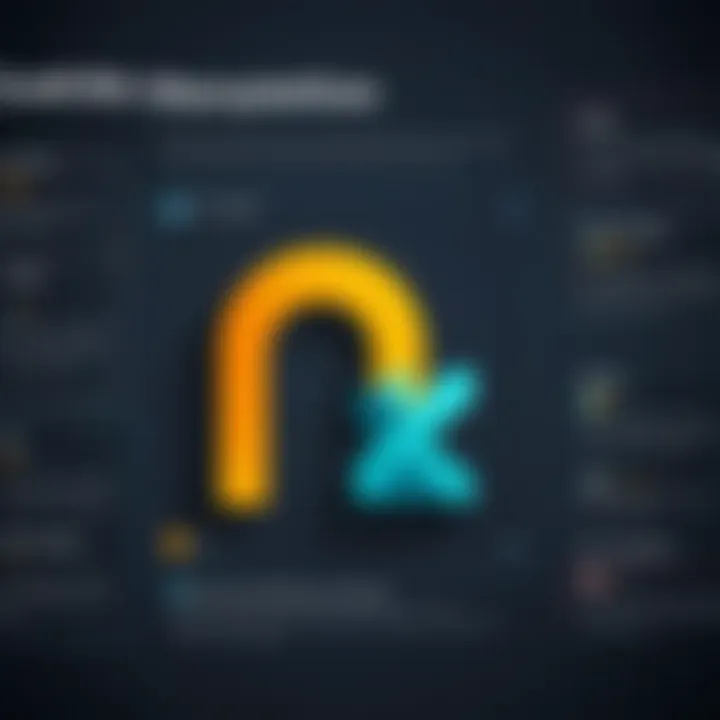
Intro
JavaScript, often hailed as the lifeblood of web development, plays a pivotal role in enhancing interactivity on websites. This article will take you on a journey through the essential aspects of form handling—a crucial part for any developer looking to embrace the nuances of user input. Whether you’re a newcomer feeling your way through the code or someone with a little more experience, this guide will provide a structured and detailed exploration.
Form handling in JavaScript is not just about collecting user information but fostering a seamless user experience. With forms being the primary means for users to interact with web applications, the right techniques in handling forms can significantly impact usability and satisfaction. In this immersive guide, we will cover everything from the basics of DOM manipulation to advanced validation techniques, each section building upon the last.
Moreover, practical examples throughout the article will demonstrate real-world applications of the concepts discussed. This ensures that you not only learn theoretically, but also understand how to apply this knowledge effectively.
As we delve deeper, we’ll emphasize key topics that encapsulate the essence of form handling in JavaScript, ensuring that by the end, you’ll have the tools and insights necessary to implement effective strategies in your projects.
Preface to Form Handling in JavaScript
Forms are the backbone of user interaction on the web. They’re where users input their data, whether it be for signing up, logging in, or providing feedback. A well-designed form can significantly enhance user experience, leading to higher engagement and better conversion rates. In this section, we discuss why understanding form handling in JavaScript is crucial for aspiring developers and programmers alike.
Importance of Forms in Web Applications
Forms facilitate the vital connection between users and websites. Their importance can’t be overstated. Here are some key reasons why forms are essential in web applications:
- Data Collection: Forms serve as a primary method for gathering user input, which can be anything from personal info to survey responses.
- User Authentication: For many sites, forms are the gateway for user account creation or authentication, permitting access to user-specific content.
- Interactivity: They enable interaction and communication with users, formulating a two-way street where users provide information and receive feedback.
- Feedback Mechanism: Forms allow users to express their thoughts on services or products, which can be invaluable for businesses.
By mastering form handling, developers can create applications that not only meet user needs but also contribute to a seamless and enjoyable browsing experience.
Overview of JavaScript's Role
JavaScript plays an instrumental role in the functionality of forms on web pages. It empowers developers to create interactive experiences, ensuring that forms are not just static but dynamic and responsive to user input. Here’s how JavaScript enhances form handling:
- Validation: JavaScript can perform real-time validation of user inputs, ensuring data correctness before it’s sent to the server. This reduces server strain and can significantly improve user satisfaction by providing immediate feedback.
- Dynamic Changes: With JavaScript, developers can modify form fields dynamically. For example, the visibility of fields can change based on previous user inputs, helping streamline the user experience.
- Asynchronous Processing: Utilizing Ajax, JavaScript allows for submitting forms without reloading the page, improving performance and providing a smoother experience.
In summary, mastering form handling with JavaScript is not merely an optional skill for developers but a vital component for creating effective web applications. It ensures that the forms not only perform as expected but also delight users while safeguarding their data.
"Forms are like the pulse of a web application, capturing the essential data that brings it to life."
Understanding how to leverage JavaScript for form handling sets the foundation for building applications that truly resonate with users.
Understanding the Document Object Model (DOM)
Having a solid grasp of the Document Object Model (DOM) is like having a map in a vast unknown territory. This underlying structure of web documents is not merely a technical term—it's the backbone that supports form handling in JavaScript and creates interactive web experiences. The importance of the DOM cannot be overstated. It provides a structured representation of the document and defines the way documents are accessed and manipulated. This is crucial when handling user inputs in forms, as it allows developers to change the content and structure of a webpage in real time based on user actions.
What is the DOM?
The DOM is a programming interface provided by the browser. It represents the document as a tree of nodes, where each node corresponds to the different components of the webpage: elements, attributes, and text. At its core, the DOM allows scripts like JavaScript to dynamically access and update the content, structure, and style of a document.
For instance, when you submit a form, the JavaScript code interacts with the DOM to retrieve values from input elements. This interaction is crucial for activities such as validating user input or displaying error messages, all of which happen on the fly. Without a proper understanding of the DOM, these tasks could become cumbersome and inefficient, resembling a chore rather than a seamless experience for users.
In simpler terms, think of the DOM as a detailed blueprint of your house; every room, wall, and window is represented. If you want to make changes—whether adding a room or changing the color of the walls—you work off this blueprint. Similarly, developers manipulate the DOM to create responsive and engaging user interfaces.
Navigating the DOM in JavaScript
Navigating the DOM in JavaScript involves traversing this tree structure to find nodes and manipulate them. This process can seem complex at first, but with a bit of practice, it becomes second nature. Here are some key methods used to navigate the DOM:
- : Perhaps the most straightforward, this method retrieves an element based on its unique ID. For example, if you have a form field with the ID "username", retrieves that specific input field easily.
- : This method returns a live HTMLCollection of elements with the specified class name. It can be handy when dealing with multiple elements that share styles or functionalities.
- : Leveraging CSS selectors, this method allows for targeting more complex structures. For instance, pulls the first text input within a container with the class "form-container".
Navigating involves more than just retrieving elements; it also encompasses modifying or creating nodes. Consider this example:
This code snippet illustrates how you can create a new text input dynamically and append it to an existing form, showcasing the power of DOM manipulation. The ability to navigate effectively is crucial for handling forms, as it allows developers to fetch, modify, and validate forms on demand, leading to a much enhanced user experience.
"The DOM is the bridge between the static content of a webpage and the dynamic, vibrant experience JavaScript can create. Without understanding this essential concept, developers would be working with blindfolds on."
For further information on the Document Object Model and its significance, consider exploring more at Wikipedia or deeper insights into its design principles on Britannica.
In summary, understanding the DOM equips developers with the necessary tools to craft responsive and user-friendly forms. With a clear structure for accessing and manipulating page elements, JavaScript integration becomes an art form, enhancing not only functionality but also user satisfaction.
Creating Forms with HTML
Creating forms in HTML is a cornerstone of web development. They serve as the primary tool for collecting user input, which is crucial in many web applications ranging from simple contact forms to complex user registration processes. The way forms are structured can greatly influence how users interact with your website. Thus, understanding how to create effective forms using HTML is an essential skill for anyone learning to program.
Basic Structure of an HTML Form
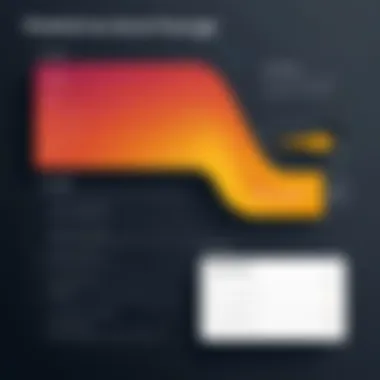
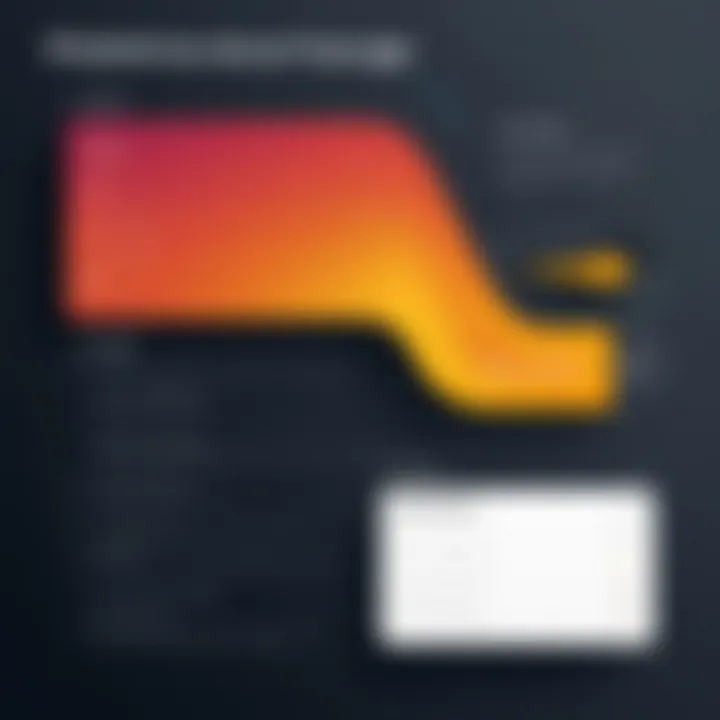
The structure of an HTML form is straightforward yet powerful. At its core, an HTML form is defined by the element, which acts as a container for various input fields. Here’s a simplified breakdown of a typical form:
- Form Element: The tag specifies where user inputs will be gathered. It can include attributes like (where to send the data) and (how to send the data, such as "GET" or "POST").Example:
- Input Fields: Various types are employed to collect data. Ranging from text boxes to radio buttons, each type caters to different kinds of user input.
- Labels: Utilizing the tag enhances accessibility, linking the label text to the corresponding input field. This practice benefits users with screen readers and improves overall user experience.
- Submit Button: Finally, a button, usually of the type , sends the form data for processing when clicked.Example:
The simplest form might look like this:
When creating forms, consider user experience. An effectively structured form guides users intuitively through inputs.
Form Elements and Attributes
Diving deeper into HTML forms reveals a spectrum of elements and attributes that can fine-tune how user data is gathered.
- Input Types: HTML5 expanded the range of input types significantly. Some popular ones include:
- Attributes Explained:
- : For single-line text inputs.
- : Automatically validates email formats.
- : Masks input for secure data entry.
- and : Allow multiple selections or single choice.
- : Dropdown menu for selecting from multiple options.
- : Forces users to fill out a specific field before submission.
- : Gives users a hint about the expected input in a field.
- : Limits the character count for input fields.
These attributes not only enhance functionality but also improve the form’s usability.
Important Note: The structure and attributes you choose have real effects on how users interact with and experience your forms. Keeping forms concise and focused can significantly decrease abandonment rates.
Capturing User Input
Capturing user input is a pivotal aspect of form handling in JavaScript. It’s where the connection between the user and the web application comes alive. Forms act as gateways for users to submit information, whether it’s signing up for a newsletter, placing an order, or even leaving feedback. Without capturing this input correctly, the entire user experience can crumble.
Understanding how to manage and process this input provides numerous benefits, not least of which is enhancing the overall functionality of your web application.
The primary considerations include choosing the right input methods, ensuring accessibility, and validating inputs to maintain data integrity. A well-implemented mechanism for capturing input can significantly influence user satisfaction and, ultimately, their likelihood of returning to your site.
Event Listeners and Their Role
Event listeners are essentially the eyes and ears of a web application. They allow developers to respond to user actions like clicks, typing, or even form submissions in real-time. For instance, when a user types in a text box or clicks a button, an event fires. The idea is simple but powerful: you can write a function that runs when these events occur.
Here’s an example of how this can look in code:
In this snippet, when the form is submitted, instead of letting the browser handle it immediately, we stop it in its tracks with . This allows us to control what happens next - such as validating inputs or sending data to a server via AJAX. On top of that, event listeners provide a smooth user experience, making interactions feel instantaneous and lively.
Fetching Input Values Dynamically
Fetching input values dynamically is crucial when dealing with forms where user input can change frequently. Imagine a scenario where a user fills out a multi-step form. If you only capture the data once at submission, you risk losing important information if there are mistakes along the way.
Using JavaScript, you can pull these values as users make changes. Let’s say we have a form input for an email address:
With this setup, the application logs the value of the email input every time the user types something. This is especially useful for validation checks or to provide live feedback to users, enhancing the form's interactivity.
Not only does this approach improve user engagement, but it also minimizes the chances of submitting erroneous data, aligning perfectly with best practices for modern form handling.
Form Validation Techniques
Form validation is a critical part of web development, serving as a gatekeeper for data that flows into an application. It's akin to checking your bags before a trip to ensure you’ve packed everything necessary. In the realm of JavaScript, mastering form validation techniques not only enhances the user experience but also bolsters the security of your web application.
Effective validation can prevent a host of issues, including data corruption, unauthorized access, and fraud. As developers, we must pay attention to the nuances of how validation affects usability and security. A seamless validation process ensures users can input data freely while safeguarding the application from invalid or malicious information.
Client-Side vs. Server-Side Validation
When it comes to validation, there are two main approaches: client-side and server-side. Each has its strengths and weaknesses, creating a complementary relationship in many applications.
Client-Side Validation: This type of validation happens in a user's browser before the form is even submitted. Think of it like having a bouncer at the front door who checks that everyone is wearing the right attire before they enter the club.
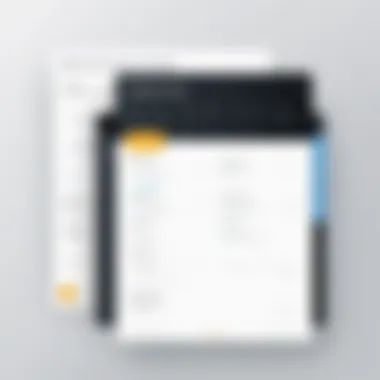
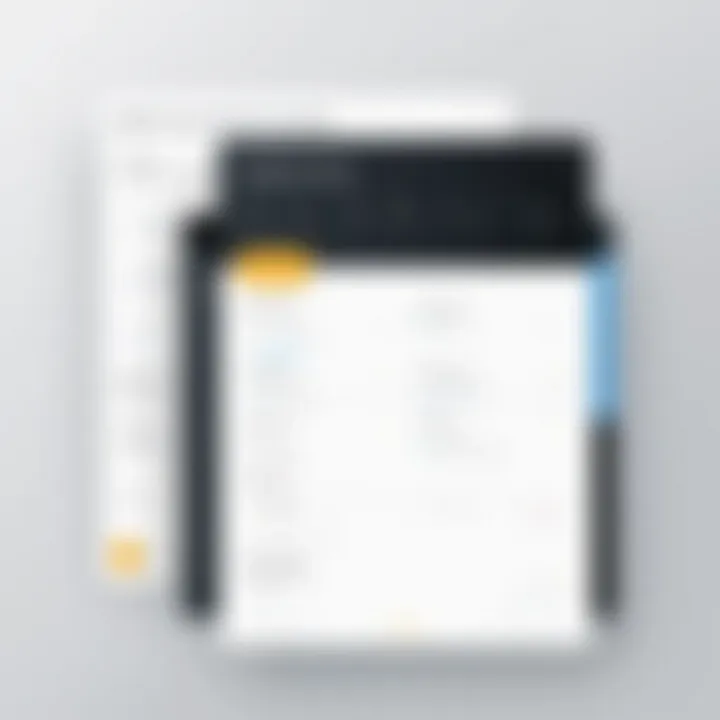
- Benefits:
- Considerations:
- Immediate feedback to users; no need for a round trip to the server.
- Reduces server load by catching errors early on.
- It's reliant on the browser, which means it can be bypassed if someone turns off JavaScript in their browser settings.
- Can lead to inconsistent behaviours if different browsers handle validation differently.
Server-Side Validation: Once the client-side checks are passed, server-side validation steps in. This is akin to a double-check by the security personnel inside the event. Even if someone gets past the bouncer, they may still need to go through another round of validation at the door inside.
- Benefits:
- Considerations:
- This validation is performed on trusted server code, making it much harder for users to manipulate.
- It allows for more comprehensive checks that account for logic and data integrity.
- There's an inherent delay due to the round trip to the server.
- Users may experience frustration if they are not given immediate feedback about valid inputs.
In practice, a combination of both client-side and server-side validation is recommended for robust data handling.
Implementing Basic Validation Rules
Creating basic validation rules in JavaScript is straightforward and can greatly enhance the user experience. Here, we will explore a few essential rules to consider when validating form inputs.
- Required Fields:
Ensuring certain fields must be filled out. This could be as simple as checking if an input field is empty: - Email Format:
Validation rules can check whether an entered email address is structured correctly. A regular expression is often used for this: - Password Strength:
It’s important to enforce rules that require the password to contain a mix of letters, numbers, and special characters to enhance security. A potential validation could look like this:
By implementing these basic validation rules, developers can prevent many common input issues right at the outset. These checks offer users a smoother journey in their interaction, ensuring that their information is correctly formatted before it ever reaches the server.
Advanced Form Handling Features
In the realm of web development, the capability to adeptly manage forms can considerably influence user interaction. Advanced form handling features in JavaScript are not merely enhancements; they serve as pivotal tools that streamline data collection and boost user satisfaction. This section delves into two critical aspects of advanced form handling: dynamic forms and AJAX submissions, elucidating their significance and practicality in real-world applications.
Dynamic Forms with JavaScript
Dynamic forms, often likened to chameleons, are versatile components that adapt based on user actions or inputs. They allow developers to construct engaging and efficient user interfaces by changing the form's structure without reloading the page. Here’s how they operate:
- Conditional Fields: Imagine a scenario where a user selects a specific option that requires additional information. With dynamic forms, you can unveil extra fields only when necessary, ensuring that users aren’t overwhelmed with irrelevant questions.
- Real-Time Validation: As users fill out their information, dynamic forms can provide instant feedback. For instance, if someone types an email address that doesn’t meet format criteria, they can receive immediate alerts. This not only enhances data quality but also significantly reduces user frustration.
- Interactive Components: Utilizing various input types such as sliders or date pickers makes forms more manageable and pleasant to use. This interactivity can make tedious tasks, like filling out lengthy forms, feel less burdensome.
Implementing dynamic forms often involves using to track user inputs and manipulate the DOM accordingly. Here’s a simplified example:
Ajax for Asynchronous Submissions
Ajax, an acronym for Asynchronous JavaScript and XML, is another powerhouse when it comes to advanced form handling. It allows forms to send and receive data without disrupting the user experience—think of it as a magician behind the curtain, performing its tricks unseen.
- Immediate Feedback: When users submit a form through AJAX, they can see response messages without necessitating a page refresh. This seamless interaction keeps users engaged and informed. For example, if a user submits their information, an AJAX call can relay whether the data was accepted or if there were any errors, all without the jarring interruption of a full-page reload.
- Partial Data Updates: AJAX not only streamlines user submissions but also allows for partial data updates on the page. Users don't have to navigate away from their current form to see updates or results, which keeps them from feeling lost or sidetracked.
- Performance Efficiency: By loading only necessary data rather than the entire page, AJAX substantially reduces load times. This is especially relevant in mobile contexts, where data speed can be a vital consideration.
To execute an AJAX form submission, you might use the API. Here’s how you can structure it:
Error Handling in Form Submissions
Error handling in form submissions is crucial for creating a seamless user experience and ensuring effective data management. When users interact with forms—be it for registrations, feedback, or purchases—it's imperative that any errors occurring during submission are addressed intelligently. This not only protects the integrity of the data collected but also enhances the user's trust and satisfaction with the web application.
A well-structured error handling process can greatly minimize user frustration. It informs them about what went wrong and guides them in rectifying the issues. For example, if a user forgets to fill in a required field, a clear error notification will prompt them to address it right away. Errors can manifest from different sources: connectivity issues, incorrect data formats, or even unexpected server responses. Addressing these problems should be considered a part of the web application’s overall functionality.
By implementing proper error handling, web developers can ensure that users receive constructive feedback, increasing the likelihood of successful form submissions. This section breaks down common error scenarios, as well as how to craft user-friendly error messages that facilitate smoother interactions.
Common Error Scenarios
When it comes to form submissions, users encounter various types of errors. Recognizing these scenarios helps in anticipating user needs and troubleshooting effectively. Here are some prevalent error types:
- Required fields left blank: Users often forget to fill essential information. This can be something obvious like a name or email address.
- Invalid formats: Sometimes, users input information in incorrect formats, such as a phone number with letters instead of digits or an email without an "@" sign.
- Exceeding character limits: Many forms have restrictions on input lengths. Users may overlook these while typing.
- Network issues: Poor internet connectivity can lead to failed submissions or timeouts, causing confusion.
- Session timeouts: If a user takes too long to submit the form, their session may time out, resulting in a loss of data.
Recognizing these scenarios is the first step in effective error management in form handling. It’s about being proactive rather than reactive.
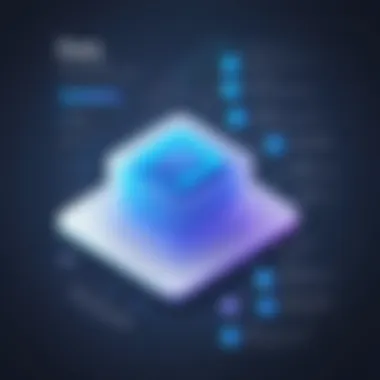
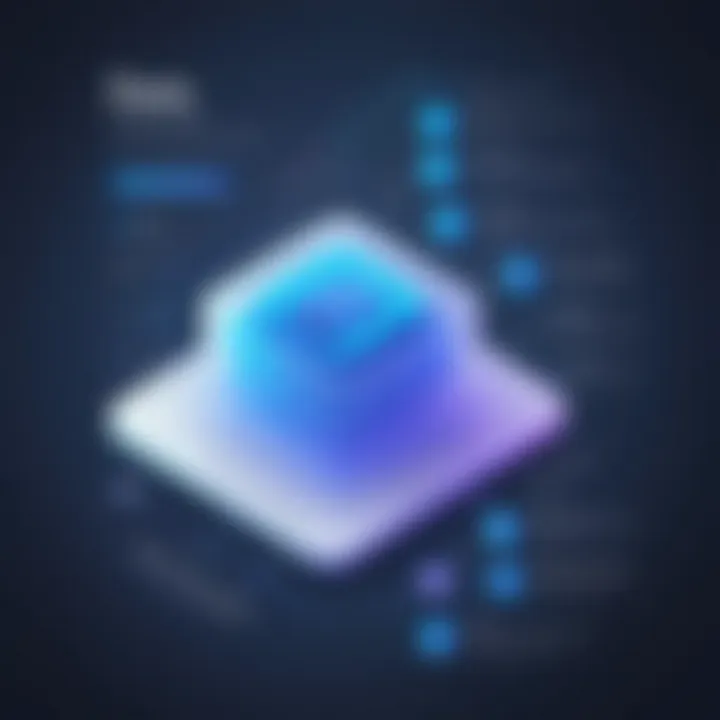
User-Friendly Error Messages
Crafting user-friendly error messages is a fine art that can greatly improve the user experience. Vague or overly technical messages can confuse users more than help them. Here are tips for effective error messaging:
- Be clear and concise: Avoid jargon. A simple message like "Please enter your email address" is much more helpful than "Input Error: A mandatory field is missing."
- Be specific: Instead of a general error message, tell the user exactly what went wrong. For instance, if a password doesn’t meet the requirements, specify that the password must be at least eight characters long and include a number.
- Positivity counts: Instead of "That’s incorrect," try "Let’s fix that! Here's what to do:"
- Implementation of tooltips: Sometimes, it can be helpful to provide guidance right next to the input fields. For example, a small note under the email field could state, "Must look like name@example.com."
- Visibility: Ensure that error messages are prominently displayed. They should catch the user's eye without being overly distracting.
By following these practices, you not only enhance their experience but also aid in reducing form submission errors. Using tools like animations or colors can also assist in emphasizing these messages appropriately.
Remember, effective error handling doesn’t just fix mistakes—it prevents them from happening and builds a strong connection between the application and its users.
In the end, good error handling and clear communication create a positive impression of your web application, enhancing its perceived professionalism and reliability.
Security Considerations
In the digital age, securing user input is paramount, particularly when dealing with forms—where the interaction between user and application often takes place. Neglecting security can lead to serious ramifications, including data breaches, compromised user information, and diminished trust. This section of the article focuses on identifying vulnerable points in form handling and lays out strategies to bolster security against prevalent threats.
Forms are gateways through which attackers can infiltrate applications. Ensuring that these points are secure requires vigilance and a proactive approach to coding practices. Here, we will look closely at two major areas: preventing Cross-Site Scripting (XSS) and input sanitization techniques.
Preventing Cross-Site Scripting (XSS)
Cross-Site Scripting (XSS) is a common vulnerability often exploited by hackers. In its essence, XSS allows an attacker to inject malicious scripts into web pages viewed by other users. These scripts can steal cookies, session tokens, or other sensitive information and can be particularly devastating for web applications that rely heavily on client-side scripting. To mitigate this risk, developers must implement robust security measures.
One vital strategy is to implement Content Security Policy (CSP), which acts as a security layer preventing unauthorized scripts from executing. CSP allows developers to delineate which resources are permitted to load and execute.
Here are some practical steps to prevent XSS:
- Escape User Input: Always escape user-entered data when rendering it on the page. This ensures that the input is treated as data rather than executable code.
- Use Frameworks with Built-in Protection: Most modern frameworks, like React or Angular, automatically escape content. Leveraging these can significantly reduce risks.
- Validate Input on Server-Side: While client-side validation is essential, server-side checks are necessary for true security. Validate inputs to ensure they match expected formats and eliminate any harmful content.
"Prevention is always better than cure, especially in the world of cybersecurity."
Input Sanitization Techniques
Input sanitization is the process of cleaning user input to ensure it is secure before it’s processed or stored. Sanitization should be a fundamental part of your form handling practices. Understanding common types of inputs users can provide is key, since each may require different handling methods.
When sanitizing user input, consider the following techniques:
- Removing Unwanted Characters: Stripping out dangerous characters, such as HTML tags, can prevent a host of attacks. Regular expressions can be useful here, as they allow targeting specific patterns.
- Whitelisting Values: Instead of merely blocking harmful inputs, define a list of acceptable inputs. This ensures that only valid data is allowed through. For example, if a field expects an integer, implement checks to ensure the input meets that criteria.
- Encoding Outputs: When displaying user data, encoding outputs ensures that any potentially harmful code is rendered harmless. Utilize HTML entity encoding for this purpose; for instance, converting `` to prevents the tag from being interpreted as HTML.
Best Practices for Form Design
The design of web forms is not merely an aesthetic exercise; it’s a vital part of user interaction that can directly impact the usability and efficiency of any application. In the realm of JavaScript, following best practices in form design enhances user experience and can lead to higher conversion rates. By prioritizing both functionality and intuitiveness, developers can create forms that not only look good but also work seamlessly across various devices.
User Experience Considerations
User experience (UX) is at the heart of effective form design. When users encounter a form, their primary goal is often straightforward: to submit information quickly and accurately. A few critical considerations come into play here:
- Clarity: Forms should be as clear and concise as possible. Users should know what information is required without playing a guessing game. Use descriptive labels right next to each input field. For instance, instead of a generic "Name" label, consider labeling inputs with "First Name" and "Last Name."
- Logical Flow: The order of fields should follow a logical sequence. When users can quickly identify how to move from one field to the next, completion becomes easier. Group related information together, such as placing all contact-related fields in one section.
- Feedback Mechanisms: Providing immediate feedback can dramatically improve user satisfaction. If a user has made an error, it’s critical to highlight that issue in real time. For example, if the email format is incorrect, a gray text message could pop up below the input field, letting the user know exactly what to fix please.
- Minimalism: Less is often more. Avoid asking for unnecessary information to reduce user fatigue. Research has shown that forms with fewer fields often yield better conversion rates. Sometimes, less is more when creating engaging forms.
"Simplicity is the ultimate sophistication."
These foundational user experience elements create an environment where users feel comfortable and empowered to complete forms.
Mobile Responsiveness of Forms
In our current digital landscape, a significant portion of users interacts with websites via mobile devices. It becomes imperative for forms to be mobile-friendly. Consider the following practices to ensure that forms perform well on smartphones and tablets:
- Responsive Design: Use CSS frameworks like Bootstrap, or apply media queries to ensure your forms adapt gracefully to varying screen sizes. This means buttons should be appropriately sized; inputs should use full width for ease of touch.
- Touch-Friendly Controls: Avoid small links or buttons that are hard to tap on mobile. Make sure that your buttons are large enough for users to interact with comfortably. This reduces user frustration and improves the likelihood of successful form completion.
- Simplified Input Methods: Leverage mobile advantages by applying input types specific to the kind of data being entered. For instance, using for email fields brings up the appropriate keyboard automatically, making things much easier for users.
- Testing Across Devices: Regularly testing forms on various devices and screen sizes can uncover usability issues that might not be immediately obvious during initial development. Tools like BrowserStack can facilitate this testing process.
By applying these best practices, you can significantly enhance both the functionality and aesthetic appeal of forms within your web applications. The end goal is to create a seamless experience where users can effortlessly engage without frustration.
End
In wrapping up our exploration of form handling in JavaScript, it’s crucial to reflect on the significance of the concepts discussed. The handling of forms is a pivotal yet often overlooked aspect of web development. It bridges the gap between user interactions and the underlying system, allowing for the gathering of valuable data. Understanding the nuances of form handling not only enhances the user experience but also empowers developers to create more responsive and reliable applications.
Recap of Key Concepts
Throughout this guide, several core topics have been addressed, each contributing to a holistic understanding of form handling:
- The Importance of Forms: Forms act as the primary interface for user interaction with web applications. They are essential for collecting data, whether it be for user registration, feedback, or transactions.
- DOM Manipulation: Given that forms exist within the Document Object Model, mastering the manipulation of the DOM is key for creating dynamic interactions, ensuring that developers can adjust form behaviors based on user actions in real time.
- Validation Techniques: Both client-side and server-side validation methods play a crucial role in ensuring data integrity and security. Learning how to implement effective validation prevents common errors that can frustrate users and compromise data integrity.
- Error Handling: Acknowledging the potential for errors in form submission is vital. By designing user-friendly error messages, developers can enhance usability, turning potential pitfalls into constructive feedback that guides users toward successful interactions.
- Security Measures: Techniques like input sanitization and mechanisms to prevent cross-site scripting safeguard applications and users alike from malicious activities.
Further Learning Resources
To gain a deeper insight into form handling and related concepts, here are several valuable resources:
- MDN Web Docs - An excellent source for comprehensive documentation on JavaScript and the DOM. They provide in-depth articles and tutorials on form handling that are highly regarded in the developer community. You can find valuable insights at MDN Web Docs.
- W3Schools Online Web Tutorials - This platform offers interactive examples and try-it-yourself code snippets to reinforce learning about forms in HTML and JavaScript. Visit W3Schools to explore their tutorials.
- Stack Overflow - A community-driven site where developers can ask questions and share knowledge. Searching for specific form handling issues can yield practical solutions and insights from experienced programmers. Check it out at Stack Overflow.
- YouTube Tutorials - Video tutorials can provide visual and practical demonstrations of form handling techniques. Many educators and experienced developers share their insights on platforms like YouTube, making complex topics easier to grasp.
In summary, mastering form handling in JavaScript involves more than just writing code; it requires an understanding of user experience principles, data validation, and security practices. By continuously learning and applying these concepts, developers can create more intuitive and secure web applications.