Mastering Form Generation in PHP with Best Practices
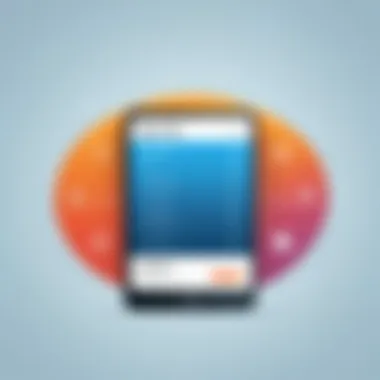
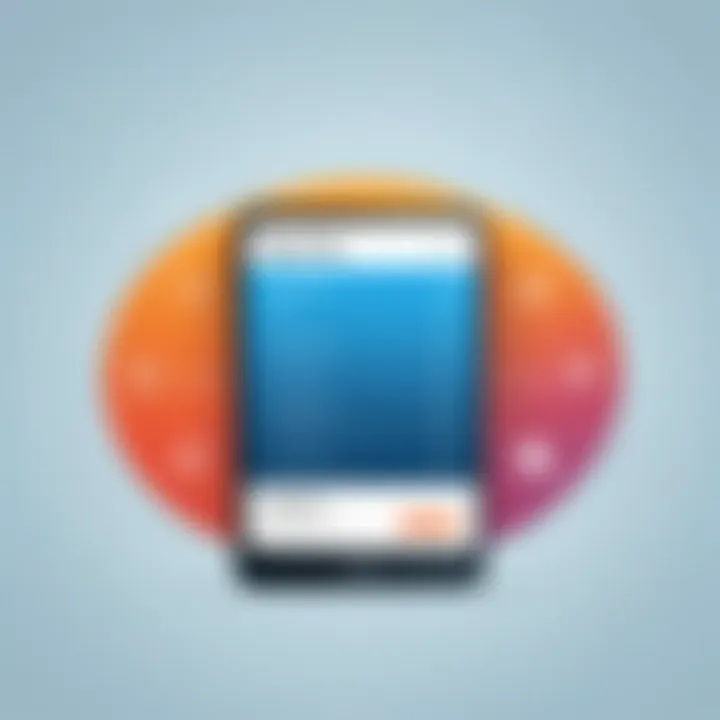
Intro
In the world of web development, forms are one of the most essential components of user interaction. It allows users to input their data and send it to server-side applications for processing. PHP has established itself as a preferred language for many developers for creating forms due to its simplicity and flexibility. Understanding how to generate forms using PHP could be the differentiator in building more functional and user-centric web applications.
History and Background
PHP, which stands for "Hypertext Preprocessor," was created in the mid-1990s by Danish-Canadian programmer Rasmus Lerdorf. Initially, it served as a set of Common Gateway Interface (CGI) binaries written in the C programming language. Over the years, PHP has evolved into a dynamic server-side scripting language, becoming be a powerful tool for web development. As the need for interactive elements on websites increased, PHP grew to meet these demands, making form creation a lot more intuitive and straightforward.
Features and Uses
One of the standout features of PHP is its ability to seamlessly integrate with HTML, making it easier to incorporate forms into web pages. The language adds functionality with minimal overhead, allowing developers to focus more on user experience rather than on complex coding hurdles. PHP enables data validation, error handling, and even session management, making it a robust framework for form generation.
Popularity and Scope
As one of the most widely-used languages for web development, PHP powers a significant portion of websites, including well-known platforms like WordPress and Facebook. Its simple syntax and strong community support contribute to its popularity. For budding developers and students, learning PHP can open doors to a plethora of opportunities, especially in the realm of full-stack development.
Through this article, we aim to lay a foundation for understanding how to effectively generate, validate, and process forms in PHP, addressing both novice learners and those who possess a basic understanding of the language.
Foreword to PHP Forms
In the digital age, forms are the lifelines through which users communicate with web applications and services. PHP forms play a crucial role in enabling this interaction. When you think about it, forms serve as a bridge between the users and a program, allowing for capturing data input and providing feedback. In this section, we'll explore what PHP forms are, their purpose, and why they are significant in web development.
Creating effective forms can greatly enhance user experience. They can simplify a userās journey through a website, collecting information efficiently while ensuring a smooth process. Being aware of the critical aspects of PHP forms is beneficial not just for developers but also for stakeholders who want to understand how data exchange works in web applications. So, what makes forms important in PHP?
- Data Collection: Forms allow for easy gathering of user data, whether itās a simple email subscription or complex user profiles.
- Interactivity: They provide interactivity on web applications, enabling users to perform specific actions like submitting feedback or registering for events.
- User Feedback: Well-designed forms facilitate feedback collection, thus tasting user sentiments about products or services.
Understanding the implications of forms will lead to better design decisions and ultimately more successful applications.
What is a Form in PHP?
A form in PHP is essentially an HTML form that users can fill out and submit to a server for processing. This involves the use of the PHP programming language to handle the data sent via the form. On a fundamental level, forms collect input and allow developers to work with it, making them a vital component of interactive web development.
The basic structure of a PHP form includes elements such as:
- Input fields: Areas where users type specific information.
- Buttons: These initiate the form submission process.
- Action URLs: The destination where the collected data will be sent when the user hits 'submit'.
Forms can contain various elements, including text boxes, checkboxes, radio buttons, and dropdown menus, designed for specific data collection needs.
Applications of PHP Forms
PHP forms find their importance not just in theory but also in practice across numerous domains. Here are some applications:
- User Registration: Websites often require users to fill out registration forms, capturing necessary details for account creation.
- Surveys and Feedback: Businesses use forms to gather opinions about their services or products, helping to improve their offerings.
- E-commerce: In online stores, forms are essential for capturing billing information, addresses, and feedback during checkout.
- Contact Forms: These allow potential customers to reach out without needing to share an email address.
"Forms not only act as a tool for input but also are a mirror reflecting user intent and preference."
Setting Up Your Environment
Setting a well-structured environment is the bedrock for effective form generation using PHP. Without proper setup, even the best of coding techniques can fall flat. Understanding the nuances of your development tools beforehand can save time and headaches down the road. Furthermore, a clean environment helps ensure that your development process flows smoothly, leading to fewer errors when you finally deploy your form. So letās dive into the nitty-gritty of these essential components.
Required Software and Tools
Before penning down your first line of PHP, you'll need to gather some software and tools. Hereās a rundown, which might come in handy:
- PHP: The very heart of your forms. Download the latest version from the official PHP website.
- Web Server: Apache or Nginx are popular choices. If you're looking for ease of use, XAMPP or WAMP bundles everything in one packageāperfect for beginners.
- Database Management System (DBMS): MySQL is a widely used option, especially when it works in concert with PHP.
- IDE or Text Editor: A good editor like Visual Studio Code, Sublime Text, or Notepad++ can make your coding experience smoother.
- Browser: For testing, always have a browser ready (Chrome, Firefox, etc.) to see your forms in action.
Remember, each of these tools serves a specific purpose, so having the right setup ensures that you can focus entirely on your code.
Configuring a Local Server
Configuring a local server might seem a daunting task at first, but itās all about connecting the right dots. Once you set it up, you'll have a reliable environment where you can test and troubleshoot your PHP forms without the hassle of uploading them to an external server. Hereās how you can go about it:
- Download a Server Package: If you opt for XAMPP or WAMP, follow their installation wizards. They typically come with PHP, Apache, and MySQL bundled together, so you get everything you need in one go.
- Install and Start the Server: After installation, launch the control panel for the chosen server. Start Apache and MySQL services; these are crucial for running PHP scripts and serving your database.
- Create a Project Directory: Navigate to the serverās root directory. In XAMPP, it's usually . Create a new folder for your projectāin this case, letās call it .
- Write Your First Script: Inside your project folder, create a new PHP file (e.g., ). You can now write your HTML and PHP code for forms.
- Access via Browser: Open your web browser and type in . If all went well, you should see your code rendered.
Setting up a local server isnāt just a good practice; itās a crucial stepping stone towards mastering PHP forms. A local server allows you to experiment with different features without the fear of breaking something in a live environment.
This foundational step in the process fosters a powerful workflow, enabling you to efficiently develop and test your forms. With a set environment, youāre equipped to tackle the complexities that come with form generation using PHP.
Basic Structure of a PHP Form
When diving into creating forms with PHP, it's crucial to understand the basic structure of a PHP form. Why's that? Well, the structure is akin to the foundation of a houseāwithout a strong base, everything kind of crumples, right? A well-defined structure defines how the inputs are gathered, how PHP interprets them, and ultimately, how data is processed. This section illuminates important elements, benefits, and considerations surrounding form structure that can enhance your PHP programming journey.
Creating a Simple HTML Form
Now, let's roll up our sleeves and get our hands dirty. Creating a simple HTML form is the first step towards mastered form handling in PHP. A typical form includes tags such as , with attributes defining the method (GET or POST) and the action URL where the form data should go.
Hereās a straightforward example:
This piece of code outlines a simple form with two inputsāname and email. The action attribute directs the form to the file, where youād handle the submitted data. It's critical to choose the right method. The POST method is often preferred for most forms since it doesn't display data in the URL, enhancing security for sensitive inputs.
Form Elements and Attributes
Every element in a form plays a unique role, much like instruments in an orchestra. Understanding these elements and their attributes is pivotal for rich user interaction. Some key types of elements you may include are:
- Text input: As used for names, addresses, etc.
- Email input: For valid email submissions, offering automatic validation.
- Radio buttons: To provide single-choice answers among options.
- Checkboxes: For allowing multiple selections from a set of options.
- Dropdown lists: A compact way to present choices without cluttering the form.
Moreover, utilizing attributes effectively adds layers of functionality. Using the attribute, for instance, ensures that users fill out necessary fields before submission, thus reducing the risk of incomplete submissions.
A blend of elements and proper attributes contributes to a smoother user experience, making your form not just functional, but also user-friendly.
A small but mighty tip: Always remember to use the right for your inputs. It enhances accessibility for users who depend on screen readers.
As you start building forms, keep these insights in mind. The goal isn't just to gather data, but to facilitate an intuitive interaction. Adapt the elements and attributes to the needs of your users. By doing this, you lead your form to be both comprehensive and responsive to user actions.
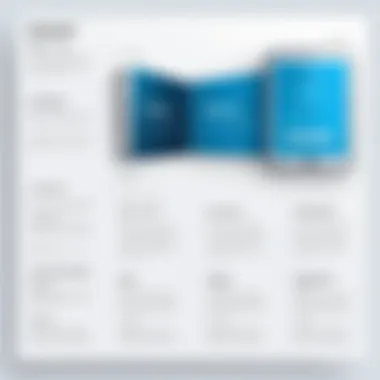
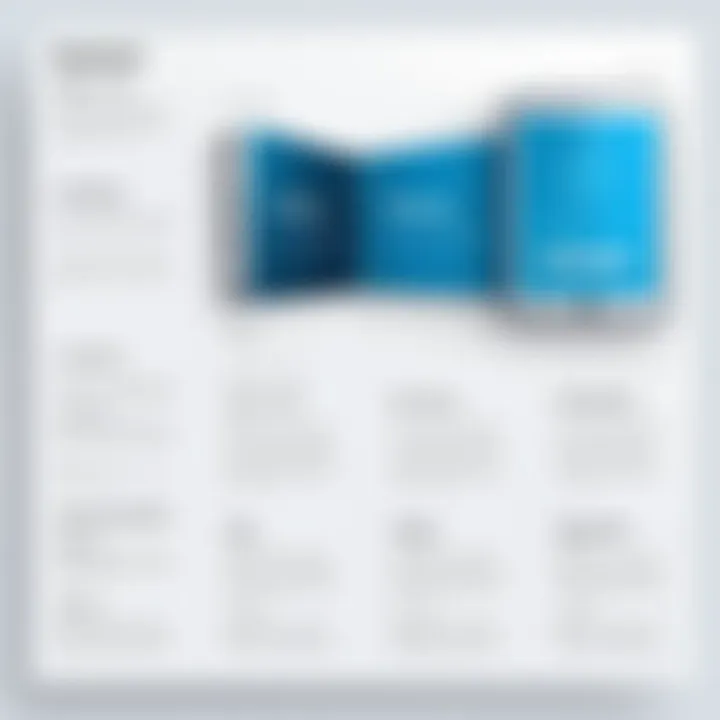
Form Methods and Action Attributes
When diving into the realm of PHP forms, understanding the methods and action attributes is crucial. These two elements shape how data is sent to the server and provide the necessary groundwork for any form to work effectively. They are not just mere specifications; they represent essential strategies for efficient data handling, ultimately determining how the submitted data integrates with the server-side code. The right approach to form methods and action attributes can enhance user experience, optimize performance, and bolster security measures.
GET vs POST Method
The distinction between the GET and POST methods is foundational in web development. Both methods transmit data, yet they serve different purposes and work in unique ways.
- GET Method: This method appends data to the URL. Itās typically used for requests where data retrieval is required without altering any server-side state. It is visible to the user in the address bar, making it easier to bookmark and share. However, it is limited in size, as URLs have character limits, and sensitive data should never be transmitted via GET.
- POST Method: This method sends data in the body of the HTTP request, thus keeping it hidden from the user. Itās more widely used for submitting forms that include sensitive information or require extensive data transfer.
- Use Cases: Ideal for search queries or when filtering data. For instance, a URL like demonstrates how parameters are sent.
- Considerations: Always remember that any information sent through GET can be seen by anyone with access to that URL.
- Use Cases: Suitable for login forms, payment processing, or situations where users submit private details.
- Considerations: While POST does not have the URL length restrictions faced by GET, server-side scripts must be prepared to handle bigger payloads and validate data properly.
Defining Action URLs
The action attribute in a form specifies where the data should be sent upon submission, acting like a destination guide in the digital landscape. It is critical to craft action URLs that point to the correct PHP scripts or endpoints responsible for processing the data submitted by users.
- Syntax: Generally, an action URL may look something like . This indicates that when a user submits the form, the data will be sent to the file on the server.
- Absolute vs. Relative URLs: Developers have the choice between using absolute or relative URLs. An absolute URL specifies the complete path (like ), whereas a relative URL simply points to a file within the current domain. Depending on the project structure and deployment practices, one may be more appropriate than the other.
"Choosing the right action URL is like setting a course for your ship; get it wrong, and you might end up in uncharted waters."
- Validation and Security: It is wise to ensure that the action URL targets a secure script capable of properly validating inputs. This step prevents malicious data injections. Use sufficient security protocols, such as HTTPS, whenever the action involves sensitive user data.
- Fallback Options: Lastly, itās also a good idea to implement fallback options in case the PHP script fails. Perhaps redirecting the user to a user-friendly error page could maintain a good user experience.
In summary, the action URL is a crucial component to direct where form submissions go, reinforcing why this understanding is vital in PHP form generation. By keeping these considerations in mind, developers can create forms that are not only functional but also secure and user-focused.
Validating Form Input
Validating form input is crucial in the realm of PHP forms, acting as the shield against erroneous data leading to potential chaos in your application. Whenever a user submits their information through a form, thereās an inherent risk that the input may be incorrect, incomplete, or even malicious. Validation serves as a gatekeeper ensuring that the data received aligns with what your program expects, thereby safeguarding both the functionality of your code and the integrity of any stored data.
Client-Side Validation Techniques
Client-side validation falls at the user's doorstep, providing instant feedback before data even reaches your server. To put it simply, itās like having your friend proofread your essay before you hand it in. Users appreciate this because it enhances their experience significantly ā they can correct small mistakes on-the-fly, like leaving out their email when signing up for a newsletter.
Various JavaScript techniques can be employed here, such as:
- HTML5 Attributes: Using attributes like , , and can enforce validation rules right in the browser.
- Regular Expressions: These powerful tools can check if the input matches the desired format, such as verifying a phone number or an email address.
- JavaScript Functions: Custom scripts can provide tailored validation logic, allowing for deeper checks like ensuring a password meets specific criteria.
For example, you might implement a simple JavaScript function to ensure an email address follows the correct format:
"A stitch in time saves nine" ā this old proverb illustrates the value of catching errors early through client-side validation.
However, it's essential to understand that client-side validation alone is insufficient. Savvy users might bypass these checks, so you must still validate on the server-side.
Server-Side Validation Importance
Once the data reaches your server, the second line of defense kicks in through server-side validation. This process ensures that even if data skews off the tracks at the client-side level, your application remains resilient against poor or harmful inputs.
Consider these points:
- Security: Server-side checks are critical for protecting your application from harmful data injections like SQL injection or XSS attacks. By validating and sanitizing inputs, youāre reinforcing your applicationās defenses.
- Consistency: While client-side checks can be bypassed, server-side validation guarantees that all data stored in your database is reliable. This plays a significant role in ensuring that your application runs smoothly and delivers accurate outputs.
- Error Handling: While server-side validation might sometimes return errors, this isnāt a downfall. Instead, it gives you an opportunity to communicate effectively with users about what went wrong, which is vital for improving overall user satisfaction.
Using PHP to handle form submissions typically means checking inputs with functions like for sanitization. For example, to validate an email:
In summary, both client-side and server-side validation play indispensable roles in the data input lifecycle. Together, they form a comprehensive approach to safeguarding your PHP applications against erroneous and potentially malicious user input.
Processing Form Data
Processing form data is the linchpin in the entire form generation process using PHP. This operation encapsulates how collected information transforms into a usable format for applications, be it for feedback, user registration or any other purpose. Without an effective way to process this data, the whole exercise of creating forms risks being a mere exercise in futility, a complex web of code lacking real-world applications.
When users fill out forms, they are not just typing into fields; they are transmitting vital information with expectations regarding its treatment. Thus, understanding the dynamics and nuances of processing form data elevates the developersā expertise. It's not just about simply grabbing data; it's about handling it smartly.
Using PHP Super Globals
At the heart of any PHP form processing are the superglobal arrays, notably and . These built-in variables serve as the conduits through which data travels from a clientās browser to a server. When a user submits a form, PHP automatically fills these arrays with the data entered by the user.
To illustrate:
This snippet is a classic example. When the form is submitted, the PHP engine processes the input, checking the request method and mapping user inputs to variables. Understanding how to adeptly use these superglobals enhances clarity and effectiveness in form handling.
Moreover, leveraging these arrays means that one can quickly display or manipulate data.
- Enhanced efficiency: With superglobals, data retrieval is straightforward and consumes less processing power.
- Data accessibility: Since these arrays are always available, developers can reference them anytime they like, sparing the trouble of declaring global variables explicitly.
Persisting Data to a Database
Writing the data to a database is where the real power of form processing comes to life. Just collecting information wonāt help unless it's stored somewhere useful. Using PHP's library of functions in combination with databases, like MySQL for instance, allows you to create, read, update, and delete information effectively.
The process typically involves:
- Connecting to the database.
- Preparing SQL queries to insert or manipulate the data.
- Executing those queries to bring about the desired changes in the database.
Hereās a brief code example:
This simple approach outlines data persistence, employing a prepared statement to secure the input data before executing the query. Storing user information this way not only fosters better organization but also opens the door for further analysis, reporting, and user engagement.
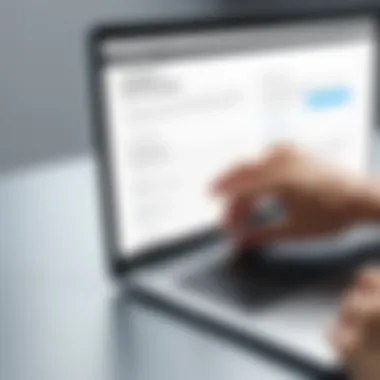
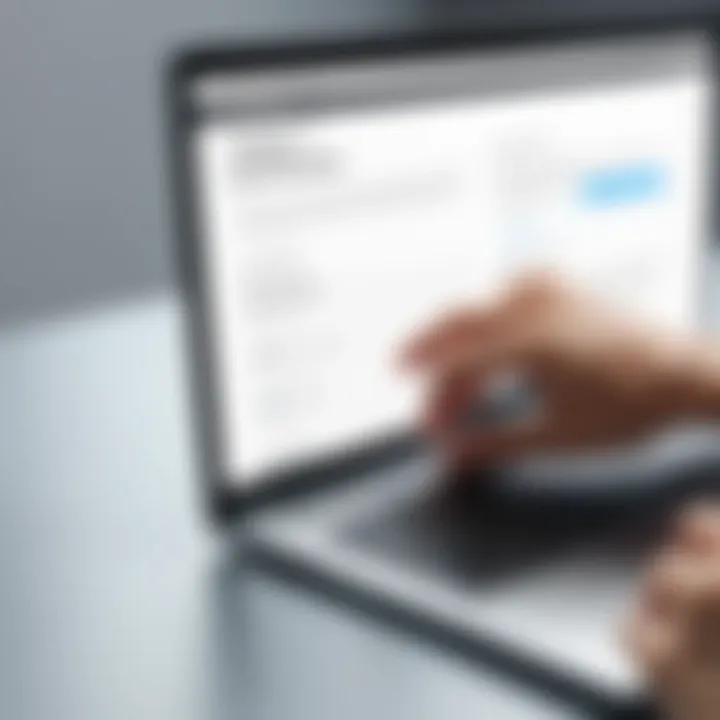
In summary, processing form data stands as a central theme in this discussion. Without a firm grasp on utilizing PHP superglobals and integrating data persistence into a database, the unfolding of sophisticated applications remains just out of reach.
Enhancing Forms with PHP
Enhancing forms using PHP isnāt just a nice-to-have; itās a critical part of web development that can lead to better user experience, improved data management, and more reliable form processing. Forms are often the gateway through which users interact with websites, so making them as functional and appealing as possible is essential. By integrating PHP into forms more strategically, developers can enhance their forms in diverse waysāfrom embedding PHP directly into HTML to creating forms that adjust dynamically according to user inputs. This section will explore these crucial elements in detail.
Embedding PHP in HTML
Embedding PHP directly into HTML is akin to weaving a seamless tapestry where the backend programming and the front-end design come together effortlessly. By using PHP snippets in an HTML document, developers can tailor forms dynamically based on various conditions such as user authentication levels or previous choices made by users. For instance, you might want to change form names or values based on the type of user logged in. Hereās a simple example:
In this snippet, the value of the username field is pulled directly from a variable and displayed in the input box. If a user enters their username, it will populate that field again on form submission, ensuring they donāt have to type it all over.
The major benefits of this method include:
- Customization: Tailors user experience based on real-time data.
- Data Control: Prevents incorrect data submission by having values set according to specific rules.
- Efficiency: Allows for cleaner code and more maintainable forms.
Dynamic Form Creation Techniques
Dynamic form creation takes the concept of embedding PHP a step further. Rather than just adjusting existing fields, dynamic techniques allow developers to build forms that can change their structure based on user interactions. This can be extremely useful when dealing with complex forms that require multiple steps.
For example, consider a scenario where users can select their interests from a dropdown, and based on that selection, an additional section emerges for more detail, say a checkbox group. Using JavaScript along with PHP enhances this by making it possible to fetch data from the server asynchronously and display additional fields without making the user reload the page.
Hereās an overview of how it might work:
- User selects a category from a dropdown.
- AJAX request is sent to fetch relevant data from PHP.
- New fields are created and displayed based on the AJAX response.
Typically, you would use a combination of PHP code that gets executed when the user selects an option and JavaScript to manage the dynamic aspects. Such techniques not only streamline user interactions but also enhance the quality of submitted data.
To summarize, optimizing forms using PHP is not merely about functionalityāit also involves creating an inviting and effective interface for users. By embedding PHP in HTML and adopting dynamic form creation methodologies, developers can significantly enrich user engagement and satisfaction.
Security Best Practices
In today's digital landscape, security is paramount, especially when dealing with forms that collect user information. Security best practices in PHP form generation are not just ancillary concerns; they're fundamental components that protect both users and developers alike. Neglecting security can lead to disastrous outcomes, including data breaches, unauthorized access, and loss of user trust. This section will delve into key elements, benefits, and considerations surrounding security practices to ensure robust form handling in PHP applications.
Preventing SQL Injection
One of the most notorious security threats encountered in PHP forms is SQL injection. This occurs when an attacker manipulates user inputs to execute arbitrary SQL code, potentially gaining access to sensitive data. The severity of SQL injections canāt be overstated; they can compromise the database integrity and expose personal information. The good news is, there are effective ways to prevent these attacks, starting with the use of prepared statements. This technique ensures that SQL queries are sent separately from user data, mitigating the risks of malicious input.
Another practice involves thoroughly validating and sanitizing user input. By adhering to specific formatting requirements or using built-in functions like , you can safeguard against unexpected values. To summarize, here are some key strategies to prevent SQL injection:
- Utilize prepared statements and parameterized queries.
- Validate and sanitize input data rigorously.
- Implement least privilege principles in database access.
- Use ORM (Object-Relational Mapping) frameworks like Laravel's Eloquent, which abstracts SQL interactions.
"An ounce of prevention is worth a pound of cure." This adage rings particularly true in the realm of security.
Cross-Site Scripting (XSS) Prevention
Cross-Site Scripting, commonly known as XSS, occurs when an attacker injects malicious scripts into content that is served to users. Unlike SQL injection, XSS exploits the trust a user has in a particular site. There are three main types of XSS: stored, reflected, and DOM-based. The ramifications can range from unauthorized actions on behalf of the users, to compromising their personal data.
Preventing XSS is crucial for maintaining user integrity and trust. Some effective strategies include:
- HTML Encoding: Ensure that user input is encoded when rendering within HTML. Functions like play a vital role here.
- Content Security Policy (CSP): Implementing CSP can drastically reduce the chances of XSS attacks by restricting the sources of executable scripts.
- Input Validation: Similar to SQL injection prevention, validating and sanitizing inputs helps avoid any user-provided data from being executed as code.
When constructing forms, developers need to remain vigilant. Regularly updating libraries and frameworks to their latest versions also ensures that security patches are applied promptly, reinforcing the defenses against XSS attacks.
User Experience Considerations
User experience (UX) is a crucial aspect of web development that can greatly influence the effectiveness of PHP forms. A well-designed form can be the difference between a user completing their task or abandoning it in frustration. Itās not just about making it look nice; itās about ensuring it's intuitive and easy to navigate. Prioritizing user experience leads to higher satisfaction rates and ultimately boosts conversion outcomes.
When we talk about user experience in the context of forms, it involves a range of elements. Firstly, itās about understanding the user's journey. From the moment they land on the site to hitting that submit button, every click should feel seamless. Ensuring clarity of the instructions alongside a well-structured layout can significantly ease the process.
Designing Intuitive Forms
Designing intuitive forms starts with an awareness of what the users need. It's essential to keep the design simple. A cluttered form not only overwhelms users but also diverts their attention from the core objective. You should keep the number of fields to a minimum, incorporating only those necessary for the desired outcome. Consider chunking information into sections if a form requires multiple inputs. This can make lengthy forms feel less daunting.
Another important principle is to follow logical grouping and ordering. Group related fields together; for example, personal details can be separated from payment information. Clearly labeled fields can guide the user seamlessly through the process; if they know which information goes where, they are likely to fill it out with less hesitation.
"The easier the form is to fill out, the fewer the barriers to submission."
Here are other considerations for designing intuitive forms:
- Use placeholders and tooltips to guide users on what to enter.
- Provide examples under text fields where appropriate to clarify expectations.
- Utilize mobile-friendly designs, as many users will access forms via mobile devices.
- Highlight required fields clearly, possibly with an asterisk, so users understand what is necessary to proceed.
Feedback Mechanisms for Users
Once a user interacts with a form, feedback plays an essential role in their experience. Providing timely and clear feedback can greatly influence how users perceive the form's usability. For instance, when a field is filled incorrectly, immediate feedback allows the user to correct their mistake without waiting for the submission. This can be achieved through inline validation messages, which inform about errors as they happen rather than at the end of the process.
Additionally, confirmation messages are vital once a form is submitted. They inform users that their input has been received successfully. This could simply be a message displayed on the same page, or redirecting them to a thank you page.
Implementing visual cues is another effective strategy. Using colors or symbols (like checkmarks) can indicate whether a field is completed correctly, enhancing clarity and reducing user frustration. Based on the user's interaction, tailor messages to address specific issues or confirm successful submissions. Moreover, having clear and easy options for users to go back or edit their input without starting over entirely can contribute positively to their experience.
Incorporating these feedback mechanisms not only assists users in completing their forms but also builds trust and an overall positive perception of your site. An excellent user experience ensures users are more likely to return, and perhaps even recommend your site to others.
Common Challenges and Solutions
In the realm of PHP form generation, users inevitably encounter a variety of challenges. This section addresses the common obstacles faced while creating and managing forms, offering practical solutions aimed at enhancing your skills and understanding.
Having a solid grasp of these challenges improves not only the efficiency of your forms but also their overall effectiveness and user satisfaction.
Troubleshooting Form Issues
Form issues can spring from numerous sources, leading to user frustration and diminished trust. A broken form can spell disaster for any website needing user interaction, causing visitors to search for alternatives. Here are some frequent issues you might face:
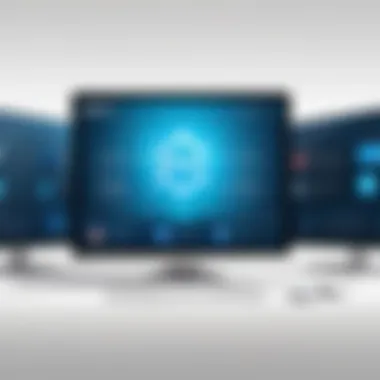
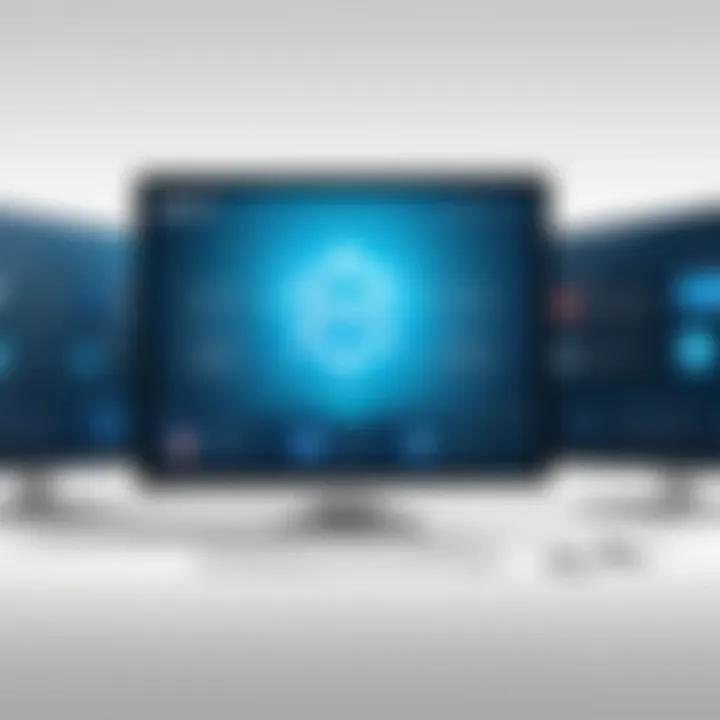
- Incorrect Action URL: If the action attribute in your form doesn't point to the right URL, submissions can go awry. Double-check the specified URL to ensure it directs to the intended script.
- Unresponsive Controls: These can arise when JavaScript conflicts with the form's native behavior. Validating that the JavaScript is functioning without causing disruptions is crucial.
- Missing Required Fields: Users may overlook essential fields. Implementing client-side validation can prompt users before submission, enhancing the overall experience.
"An ounce of prevention is worth a pound of cure."
To address these issues, one approach is to utilize a systematic debugging method. Follow these steps:
- Inspect the Code: Use browser developer tools to analyze requests and responses.
- Add Logging: Implement logging mechanisms within your PHP code to track variables and flow.
- Test Extensively: Execute multiple test cases to ensure all aspects of the form work as intended.
Handling Form Data Errors
Errors in handling form data can lead to significant problems in web applications, especially regarding user input. Mistakes in processing can affect the credibility of your site. Thus, correctly managing errors in data is critical to maintain data integrity.
The types of common errors include but are not limited to:
- Invalid Input Types: Users may enter values that do not match the expected format. For example, a phone number might contain letters instead of digits. Validate input format to ensure compliance.
- SQL Errors: When persisting data to a database, improper formatting can lead to SQL errors. Use prepared statements to mitigate this risk effectively.
- Incomplete Data Submission: Sometimes, users may submit forms with missing information. Create a well-defined validation first, then display clear messages that guide users on how to correct their submissions.
To effectively handle these errors, consider employing the following strategies:
- Implement Validation Rules: This should be done for both client-side and server-side processing. A double-check approach fortifies the form against invalid submissions.
- User-Friendly Error Reporting: Itās essential to communicate errors clearly. Instead of tech jargon, provide straightforward messages that inform the user about what went wrong and how to fix it.
- Logging Errors for Future Improvements: Maintain logs of what users submit compared to what the system processes. This data can reveal patterns or persistent issues that need addressing.
Through these strategies, you not only fix immediate errors but also enhance the user experience in the long run, ensuring a smoother interaction with your forms.
Form Adaptation for Mobile Devices
Creating forms that are user-friendly requires acknowledging the environments in which these forms will be accessed. Today, with the surge of mobile device usage, adapting forms for mobile is not just a nice-to-have; itās a must. When you think about it, nearly everyone seems to have a smartphone glued to their hands, making it crucial for forms to work seamlessly on these devices. If a form is hard to navigate on a mobile screen, users may quickly lose interest and abandon the process altogether. This would inevitably affect conversion rates and overall user satisfaction.
A well-adapted mobile form can lead to quick interactions, higher completion rates, and ultimately better user experiences. Itās worth diving into some specifics to truly grasp the benefits and considerations of mobile adaptation.
Responsive Design Principles
Responsive design is like a chameleon; it changes based on the surrounding environment. When implementing forms, the layout must adapt fluidly to different screen sizes. Utilizing CSS media queries plays a key role here, allowing for adjustments in layout elements based on the deviceās proportions. Key principles include:
- Fluid Grid Layouts: Using percentages for widths instead of fixed pixel sizes allows for greater flexibility. This ensures elements resize depending on the screen size.
- Viewport Meta Tag: This critical tag in the HTML document helps to ensure that the mobile devices render the page at the width of the device rather than at a larger desktop width, making forms more suitable for smaller screens.
- Size and Spacing: Buttons and input fields should be large enough to tap easily. Increasing padding between clickable elements helps in reducing mistakes during filling out forms.
By integrating these principles, youāre not only accommodating users on mobile but also enhancing their overall experience. Elements should flow naturally as users switch from portrait to landscape, providing an adaptive experience without hassle.
Testing on Different Devices
Nothing beats good ol' testing when it comes to validating the effectiveness of your mobile forms. Ensuring that forms perform well across various devices can become a bit tricky but very rewarding. Given the plethora of devices and screen sizes available today, here are some strategies to streamline your testing process:
- Browser Developer Tools: Most modern browsers offer tools that simulate how a webpage looks on different devices. Google Chromeās Developer Tools allow you to preview your form on various resolutions without needing the actual devices.
- Physical Device Testing: While emulators are helpful, nothing substitutes the actual experience. Testing on different smartphones can expose real issues that you might not catch with developer tools.
- User Feedback: Collecting opinions from actual users can help identify pain points that you might not have considered. Their insights will lead you to enhance the experience further.
It's imperative to keep an eye on popular devices within your target user demographic since some form fields or layouts might behave differently across platforms and browsers.
"A mobile-friendly form can mean the difference between a completed sign-up and a dreaded abandoned cart."
Integrating JavaScript with PHP Forms
Integrating JavaScript with PHP forms is essential for creating interactive and user-friendly web applications. While PHP efficiently handles server-side logic, bringing JavaScript into the mix allows for a seamless user experience by undertaking operations in real-time. This combination of technologies helps to enhance form functionality in various ways, including improved validation, dynamic content updates, and an overall more responsive form interaction.
The benefits of using JavaScript with PHP forms are numerous. Firstly, it can significantly reduce the amount of server load by validating user inputs on the client side before the data reaches the server. This means users receive immediate feedback about their submissions, such as being notified if a required field is left blank, or if the data entered doesn't meet the specified criteria. Apart from validation, using JavaScript allows developers to implement interactive elements that enhance the usability of forms.
Though the benefits are clear, integrating JavaScript with PHP requires careful consideration. Developers should be aware of potential issues regarding browser compatibility and loading times, ensuring that enhancements in user experience do not come at the cost of application performance. Moreover, itās crucial to remember that client-side validation should never replace server-side validation. Each layer serves a purpose and should be utilized accordingly to maintain security.
"Integrating JavaScript with PHP not only makes your forms smarter but also boosts user engagement. Users appreciate instant feedback, and integrating these technologies helps achieve that."
AJAX for Real-Time Validation
AJAX, or Asynchronous JavaScript and XML, is a game changer when it comes to validating form inputs in real time. Unlike traditional form validation that requires a page reload to submit data, AJAX allows specific sections to communicate with the server without affecting the rest of the page. This means that as users fill out a form, they can instantly see whether the data they are entering is valid.
For example, consider an email field. Using AJAX, as soon as the user types their email address, a request can be sent to the server to check if the email is already registered. If it is, a message could immediately appear, informing the user without needing to wait until they submit the form. Hereās a simplified outline of how the AJAX validation works:
- The user types into an input field.
- An AJAX request is triggered after a short delay (to prevent excessive requests).
- The server processes the request and checks the input data.
- A response is sent back to the client, updating the user interface accordingly.
This not only enhances user satisfaction but also minimizes unnecessary server load by filtering out incorrect inputs before they reach the server. The implementation of AJAX requires JavaScript skills and knowledge of how to handle asynchronous requests, but the payoffs in form usability are manifold.
Dynamic Content Updates
Dynamic content updates via JavaScript enriches PHP forms by making them more engaging and flexible. Instead of presenting users with static forms, developers can create forms that change based on user input in real-time. This could range from showing additional fields when a certain checkbox is selected to dynamically adjusting options in dropdowns based on prior selections.
For instance, if a user selects a country from a dropdown list, JavaScript could be utilized to fetch relevant state or province options automatically. This enhances the user experience by simplifying form completion. Here's a common example of how dynamic updates may occur:
- User selects a country from the list.
- JavaScript makes an AJAX call to fetch state data related to that country.
- The form updates the state dropdown with the fetched data without needing to reload the entire page.
Dynamic content updates lead to forms that feel more natural and less cumbersome, reducing frustration associated with long forms. However, developers should keep in mind that while these dynamic features enhance usability, they also increase the complexity in development. Rigor in testing becomes crucial to ensure all scenarios are covered and that the user experience remains smooth.
Ending and Future Trends
The world of PHP forms is not just a fleeting trend; itās a cornerstone of web development that continues to evolve. As we look toward the future of PHP form generation, various elements emerge that are deserving of attention. One essential aspect is the growing importance of user experience. Forms are often the first point of interaction between users and applications, making them crucial for satisfying engagement. In todayās tech-savvy environment, users expect forms to be not only functional but also intuitive and aesthetically pleasing. Thus, adopting modern design principles is no longer a luxury but a requirement.
Additionally, the importance of security cannot be overstated. With data breaches becoming increasingly common, developers must ensure that their forms are robust against common threats like SQL injection and cross-site scripting. Leveraging PHPās improved security features is vital as we forge ahead, letting us create safer, more reliable forms.
Another consideration is the integration of advanced technologies. The evolution of JavaScript frameworks and libraries like React or Angular influences PHP forms. Developers can build dynamic, interactive forms that respond to user input in real-time, enhancing usability. Implementing AJAX for validation while keeping the overall experience smooth is integral to modern form design. This fusion of PHP and JavaScript will likely dominate the landscape of web development in the coming years, offering an exciting frontier for developers.
"The best way to predict the future is to create it."
Summarizing Key Takeaways
To cap off our journey through the intricacies of PHP forms, consider these vital takeaways:
- User Experience is Paramount: Always prioritize the ease of use and aesthetic appeal of your forms.
- Security is Non-Negotiable: Implement security best practices to safeguard against vulnerabilities.
- Adapt and Update: Keep abreast of new technologies and trends to ensure your forms remain relevant and effective.
- Dynamic Features: Utilize JavaScript for enhanced interactivity and responsiveness.
These points encapsulate a roadmap for informed decision-making as you embark on or continue with PHP form development.
The Evolution of PHP Forms
Reflecting on the evolution of PHP forms reveals significant advancements over the years. Initially, forms served merely as data collection tools. Today, they've transformed into sophisticated elements critical to user interaction. Early PHP forms were relatively static, requiring refreshing the entire page upon submission. However, the advent of techniques like AJAX has revolutionized how forms operate, allowing for seamless data submission without losing the page context.
Moreover, PHP frameworks like Laravel and Symfony offer built-in features to streamline form creation, validation, and security. This evolution signifies a shift toward more comprehensive and user-friendly approaches in form handling. Developers now benefit from tools that emphasize maintainability and scalability, paving the way for more complex applications.
In years ahead, we can anticipate continued transformation driven by emerging technologies such as AI and machine learning, potentially introducing smart form-filling and predictive analytics. The journey of PHP forms is far from over ā itās a dynamic landscape full of opportunities and challenges.