Mastering Entity Framework Core: A Complete Guide
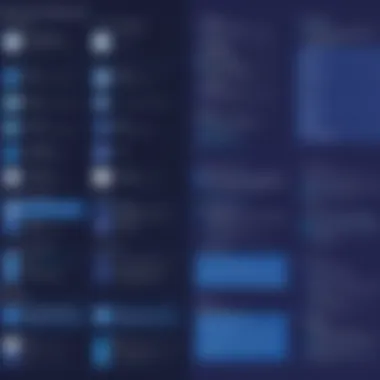
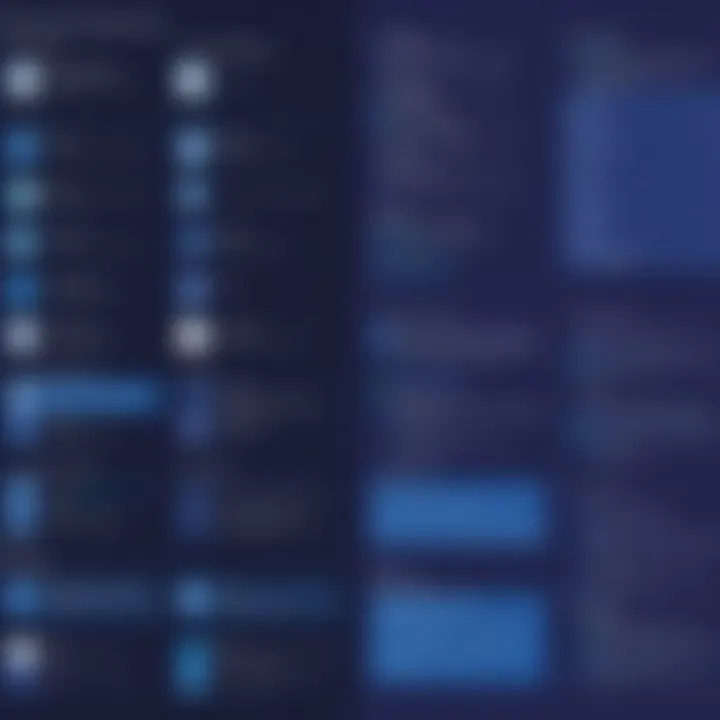
Intro
Entity Framework Core (EF Core) is a modern object-relational mapping (ORM) framework for .NET applications. Its primary purpose is to facilitate data access by allowing developers to work with databases using .NET objects. This leads to efficient data management practices in application development.
EF Core provides a streamlined way to handle data, offering features such as change tracking, lazy loading, and database migrations. One important aspect is that it abstracts the complexities of raw SQL queries. As a result, developers can focus more on the application’s logic rather than struggling with database interactions.
This framework is not just a tool; it represents a shift in how developers approach data management within their applications. Unique architecture makes it lightweight and extensible, catering to modern needs in software development.
In this tutorial, we will explore the essential components of EF Core. We will look into its setup, functionalities, and implementation strategies. The aim is to enhance your understanding of using this powerful ORM tool effectively.
Goals of This Tutorial
By the end of this tutorial, readers will be able to:
- Understand core concepts of EF Core.
- Set up their first EF Core application.
- Implement common data operations with practical examples.
- Identify best practices for using EF Core in real-world applications.
"Entity Framework Core is one of the most preferred tools for data access in modern .NET applications. It bridges the gap between object-oriented programming and relational databases."
Equipped with this knowledge, you are now ready to delve deeper into the world of Entity Framework Core, allowing for an efficient and effective data management approach in your software projects.
Foreword to Entity Framework Core
Entity Framework Core (EF Core) is a modern Object-Relational Mapping (ORM) framework, important for any developer working with data in .NET applications. ORM simplifies the way developers query and manipulate data. Knowing how to use EF Core is fundamental for managing data effectively in various applications.
• It allows developers to work with data as objects, rather than database tables. • EF Core provides a strong foundation for building robust applications that require seamless data management.
With the rapid growth of data-driven applications, a proper understanding of EF Core is critical. Developers can significantly increase their productivity by leveraging its capabilities. EF Core supports multiple database providers, enabling flexibility and extensibility. This framework also manages concurrency and transactions efficiently. Therefore, adopting EF Core can lead to better code maintainability and performance.
"Entity Framework Core is essential for modern application development. It provides developers tools to handle data operations smoothly."
This tutorial will guide you through the essential aspects of EF Core and how to integrate it into your projects efficientlly.
Setting Up the Environment
Setting up the environment is a crucial step when working with Entity Framework Core. This step ensures that all the necessary tools and configurations are in place. An improperly configured environment can lead to various issues during development. Hence, having the right setup facilitates a smoother development process. Proper setup also allows developers to leverage the full capabilities of EF Core, including features like migrations and LINQ queries.
Required Tools and Software
Before diving into development, you will need several tools and software to set up your environment effectively. Here are the essentials:
- Visual Studio or Visual Studio Code: Both are excellent Integrated Development Environments (IDEs) that support .NET development. Visual Studio offers advanced features, while Visual Studio Code is lightweight and extensible.
- .NET SDK: Installing the .NET SDK is necessary as it includes essential tools for building, running, and publishing .NET applications. It ensures you can run EF Core applications efficiently.
- SQL Server or another database: EF Core supports various databases, but SQL Server integration can offer rich data management features. You may also consider SQLite or PostgreSQL, depending on your requirements.
Ensure that the versions of these tools are compatible with .NET and EF Core versions you plan to use.
Installing EF Core Packages
After setting up the IDE and .NET SDK, the next step is to install EF Core packages. These packages can typically be added via the NuGet Package Manager in your IDE. You can run the following commands in the Package Manager Console:
The first command installs the core EF packages, the second command adds the SQL Server provider, and the third command grants access to EF Core command-line tools. This setup is important for managing migrations, which are essential for updating your database schema as your model changes.
Remember to check the official Microsoft documentation for the latest package versions and installation guidance.
Creating Your First Project
Once you have all required packages installed, the next logical step is creating your first project. This process is straightforward. Follow these steps:
- Open Visual Studio or Visual Studio Code.
- Create a new project by selecting "Create a new project" from the initial screen.
- Choose a template, such as "ASP.NET Core Web Application" or "Console Application."
- Proceed with the wizard to set the project name and location, ensuring you select .NET Core as the framework.
- After project creation, open the NuGet Package Manager to install the EF Core packages as previously discussed.
Once your project is set up, you can begin implementing data access logic using Entity Framework Core.
Setting up your environment correctly will form the foundation for further development. This will enhance your ability to execute CRUD operations and implement complex queries efficiently. Each subsequent development phase will build upon this initial setup, allowing for a more structured approach to database management.
Core Concepts of Entity Framework Core
Understanding the core concepts of Entity Framework Core is critical for effectively utilizing this powerful ORM framework. These concepts enable developers to interact with the database in a structured manner, simplifying the complexities of data access. Whether you're new to EF Core or looking to deepen your knowledge, grasping these fundamentals will enhance your programming efficiency and effectiveness in application development.
DbContext Overview
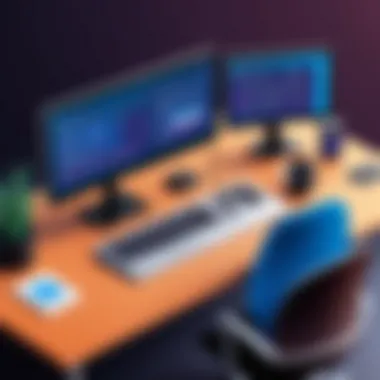
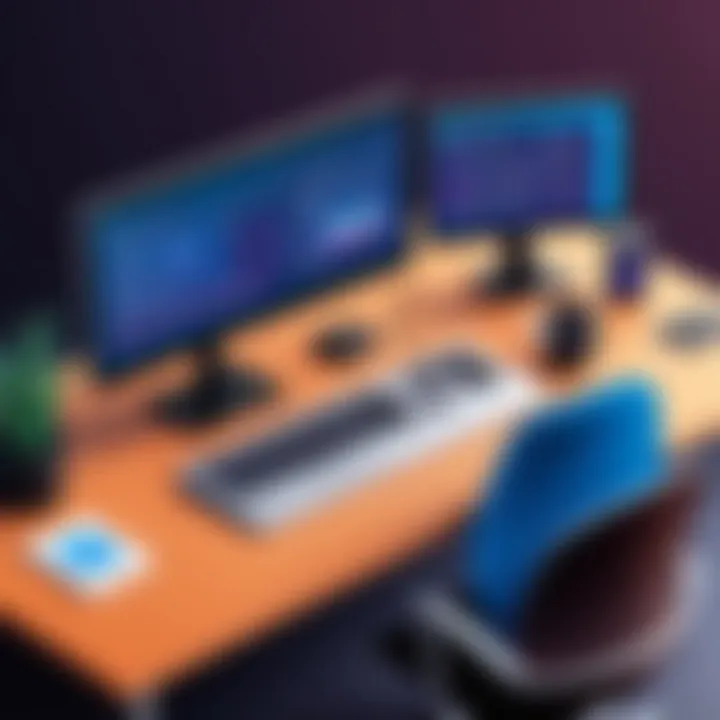
The is the principal class responsible for interacting with the database using Entity Framework Core. It acts as a bridge between your domain or entity classes and the database. Understanding how operates is essential for efficiently performing data operations.
- Lifecycle Management: Each instance of represents a session with the database. It is best to create a new instance for each unit of work rather than reusing the same instance, as this can lead to issues with stale data.
- Entities and Change Tracking: keeps track of changes made to entity instances. This mechanism ensures that only the modified data is sent to the database during the method call.
- Configuration and Customization: You can configure to suit your application need by overriding methods or setting options in the constructor. For example, you can define connection strings and logging options.
In short, mastering is crucial for leveraging the full capabilities of EF Core and ensures optimal database interactions.
Entity Classes and Configurations
Entity classes represent the data model in application and are directly mapped to database tables. Configurations play a pivotal role in defining how these classes interact with the database. Understanding these configurations is essential for ensuring data integrity and optimizing database performance.
- Defining Entity Classes: Each entity class corresponds to a table in the database. Properties of the class represent columns. For example, if you have a class, it may have properties like , , and .
- Fluent API vs. Data Annotations: You can configure entities using either Fluent API or Data Annotations. Fluent API is more flexible and powerful, allowing complex configurations. Data Annotations provide a straightforward, attribute-based way to configure entity properties directly in the class definition.
- Navigational Properties: These properties are crucial for defining relationships between entities. For instance, if a has many , you can define a navigational property in the class to link them.
Understanding how to define and configure entity classes effectively not only allows for clear structure but also enhances maintainability in your application.
Relationships in EF Core
Entity Framework Core provides several ways to define relationships between entities. Mastering these relationships is essential for accurate data modeling and retrieval.
- One-to-One Relationships: This occurs when a single entity instance is related to another single entity instance. For instance, a may have an associated .
- One-to-Many Relationships: One of the most common relationships. A can have multiple . This can be defined using navigational properties in both classes to facilitate data access.
- Many-to-Many Relationships: With EF Core, this type of relationship can be established using join tables. For example, can enroll in multiple , and each can have multiple .
To create and manage relationships effectively, it is crucial to understand how foreign keys work in EF Core. Properly configuring relationships ensures data integrity and optimizes queries.
In summary, a deep understanding of the core concepts of Entity Framework Core, including , entity classes, and relationships, is fundamental for effective data management. These principles lay the groundwork for productive application development and can significantly enhance the quality of your software solutions.
Working with the Database
Working with the database is a core functionality of Entity Framework Core. This section explains the importance of effectively managing database interactions within applications. Understanding how to properly connect, configure, and manipulate databases using EF Core is crucial for maximizing its benefits. It allows developers to execute complex queries, enabling applications to retrieve and store data efficiently.
Database management includes multiple elements such as selecting the correct database provider, configuring connection strings, and applying migrations. Each of these aspects is essential to ensure that data operations are seamless and reliable. By utilizing EF Core, one can leverage an abstraction layer that simplifies data access while maintaining performance.
Database Providers Supported by EF Core
Entity Framework Core supports various database providers, making it versatile for different applications. The widely used providers include:
- Microsoft SQL Server: A critical choice for applications that operate in a Microsoft environment. It integrates fully with the .NET ecosystem.
- SQLite: A lightweight, file-based database. It is perfect for smaller applications or testing.
- PostgreSQL: Known for its rich feature set and performance. This provider is preferred for applications needing advanced database capabilities.
- MySQL: Another popular open-source database. It is well-suited for web applications needing a reliable database backend.
Each provider has unique attributes and performance characteristics, thus, the choice depends on the specific requirements of the project.
Configuring the Connection String
The connection string is fundamental to establishing a link between your application and the database. It contains essential information, such as the server name, database name, and authentication credentials. A properly configured connection string ensures smooth communication between the application and the database.
A connection string can look like this:
In EF Core, you typically set the connection string in the file. Here is an example:
It’s pivotal to securely manage credentials and keep sensitive information like passwords confidential. Using environment variables is a common practice to achieve this.
Applying Migrations
In Entity Framework Core, migrations are a way to keep the database schema in sync with the application model. Migrations are critical when changes are made to entity classes or configurations. They allow developers to create, modify, and delete tables without losing existing data.
To apply a migration, you can use the Package Manager Console or the command line. The command is:
This will scaffold a migration, which can then be applied using:
Applying migrations ensures that your database structure evolves alongside your application’s requirements. It is essential for maintaining data integrity and consistency throughout the lifecycle of the project.
Migrations enable developers to manage database changes efficiently, reducing the risk of errors during updates and deployments.
CRUD Operations with EF Core
CRUD stands for Create, Read, Update, and Delete. These are the four basic operations that you can perform on data in any application. In the context of Entity Framework Core, understanding how to implement these operations is critical for effective data management. Each operation serves a unique purpose in handling data. Here, we will explore their significance, best practices, and considerations when working with Entity Framework Core.
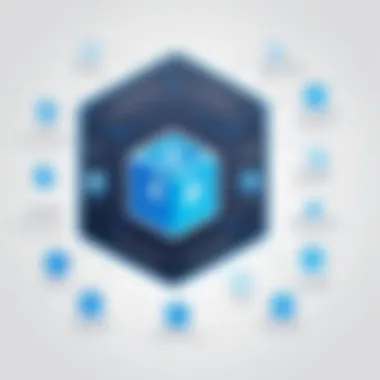
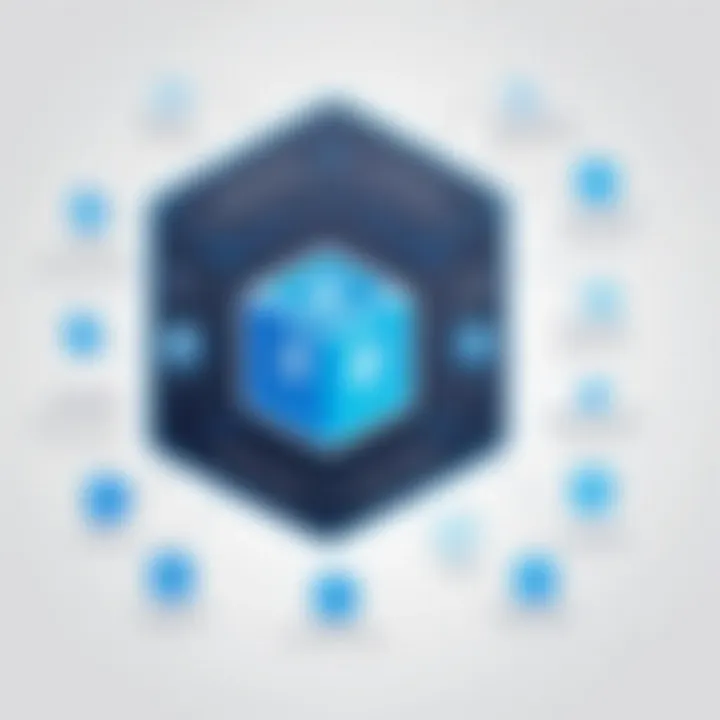
Creating Records
Creating records is the first step in persistence. It involves adding new data entries into your database. In Entity Framework Core, this is primarily achieved using the method of the class. When you create a new record, EF Core automatically sets up the relevant properties such as keys or timestamps based on your model configuration.
For example, to create a new record, you might have:
When you call , EF Core sends commands to the database to insert the new record. This might seem simple, but there are several aspects to consider, such as validation and handling possible exceptions. It is crucial to ensure that the data is in a valid state before attempting to save, preventing runtime errors that can disrupt your application.
Reading Records
Reading records is fundamental to retrieving data stored within your database. In EF Core, you can use LINQ queries to fetch records efficiently. This can be done through methods such as , , or with filtering using . For instance:
Using LINQ allows for complex querying capabilities. Whenever you read data, consider how much information you need and try to avoid fetching unnecessary records. This optimization can greatly enhance the performance of your application, especially when dealing with large datasets.
Updating Records
Updating records is essential to keeping your application’s data current. In Entity Framework Core, updating an entity can be achieved with the method. First, you must retrieve the entity you wish to modify, alter its properties, and then save the changes.
Here's a brief example:
It is important to be cautious when updating records. This can lead to overwriting important data if handled improperly. Additionally, consider implementing versioning or timestamps to track changes effectively.
Deleting Records
Deleting records is the final operation in the CRUD cycle. This is done using the method of the class. Before deleting any record, it is advised to confirm whether the record to be removed truly should be deleted. This can prevent accidental data loss.
An example of deleting a record is:
Again, always validate the operation, especially in business-critical applications. Consider implementing a soft delete strategy if retaining data integrity is paramount.
Always ensure to handle exceptions gracefully to maintain user experience and application stability.
Understanding these CRUD operations with Entity Framework Core lays the foundation for data handling in your application, allowing for effective data management strategies.
Querying with Entity Framework Core
Querying is a crucial aspect of working with Entity Framework Core (EF Core). It enables developers to retrieve and manipulate data stored in a database efficiently. Understanding the querying capabilities of EF Core is essential for anyone looking to utilize this framework effectively. The querying process can shape the performance and responsiveness of applications. Being aware of different querying strategies and their implications is vital.
LINQ Queries in EF Core
Language Integrated Query (LINQ) serves as the backbone for querying data in EF Core. It provides a syntactically integrated way to compose queries directly in C#. LINQ queries can be written in two distinct styles: query syntax and method syntax. The choice depends on the developer's comfort and the specific use case.
Example of LINQ Query
Consider a scenario where we want to retrieve a list of products from a database:
This example demonstrates using a lambda expression to filter products with a price greater than 100. The use of ToList() executes the query and fetches the results.
Benefits of using LINQ:
- Type Safety: Errors are caught at compile time, enhancing reliability.
- IntelliSense Support: Developers can take advantage of IDE features for easier query writing.
- Composability: Queries can be built in a modular way, facilitating more complex queries with ease.
Raw SQL Queries
While LINQ is powerful, there may be instances where raw SQL queries are preferred. This can occur for complex queries that are easier to write in SQL or to use database-specific features. EF Core allows developers to execute raw SQL queries directly against the database.
Example of Raw SQL Query
To run a raw SQL query, the following syntax is used:
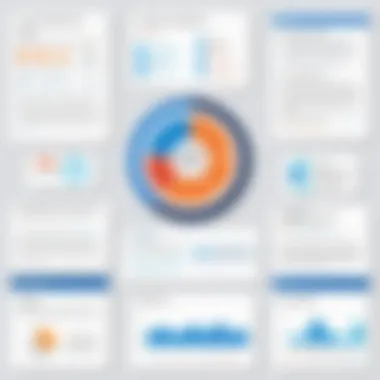
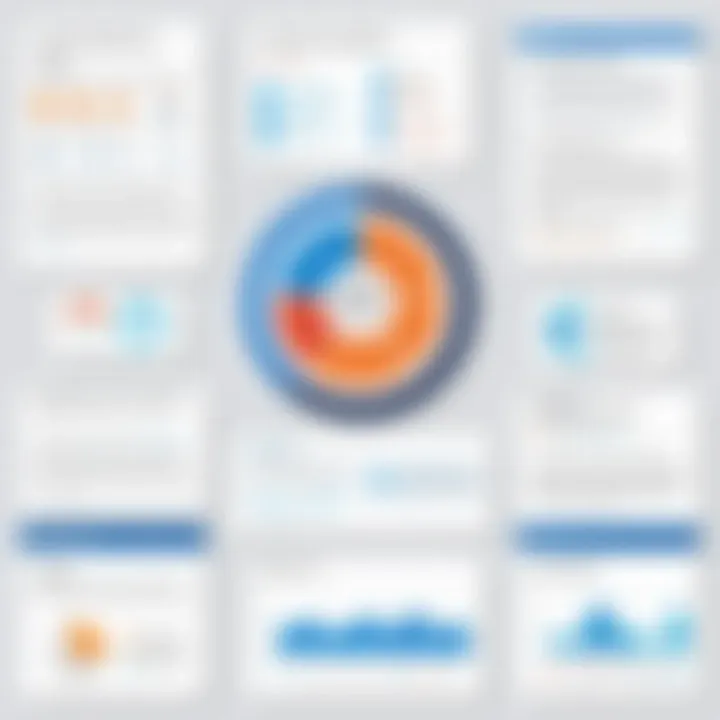
This approach grants flexibility but may sacrifice some of the type safety that LINQ provides. It is advised to use raw SQL sparingly and only when necessary.
Considerations for raw SQL queries:
- Performance: Direct SQL can be more efficient for certain operations.
- Maintainability: Raw SQL may reduce readability and increase difficulty in managing changes.
- Security: Ensuring protection against SQL injection becomes critical; developers should always validate input.
Asynchronous Queries
Asynchronous programming is becoming increasingly important for responsive applications. EF Core supports asynchronous querying to enhance performance during data retrieval operations. By using the async/ await pattern, developers can prevent blocking the main thread and maintain application responsiveness.
Example of Asynchronous Query
An example of an asynchronous query looks like this:
Benefits of asynchronous operations:
- Responsiveness: User interfaces remain responsive while waiting for queries to execute.
- Scalability: More concurrent operations can be handled, effectively utilizing resources.
In summary, mastering querying with EF Core opens up numerous possibilities. Different querying strategies, from LINQ to raw SQL to asynchronous techniques, equip developers to build efficient and responsive applications.
Performance Considerations
In the realm of Entity Framework Core, performance considerations are pivotal for ensuring efficient database interactions. Developers often encounter challenges that can lead to suboptimal application performance. Hence, understanding the various factors affecting efficiency can significantly uplift the application experience. This section delves into two crucial aspects: Tracking vs. No-Tracking queries and Query Optimization Techniques.
Tracking vs. No-Tracking Queries
Entity Framework Core provides two distinct types of queries: Tracking and No-Tracking. Understanding the differences between these is essential for optimizing performance in different contexts.
Tracking Queries
Tracking queries keep track of the changes made to the entities retrieved from the database. This allows the context to automatically detect changes and update the database accordingly upon saving. While this feature is beneficial for applications that need to maintain a consistent state and frequently update data, it can lead to overhead in performance, especially when dealing with large datasets.
- Use Cases: Ideal for applications with frequent edits or complex business logic.
- Drawbacks: Additional memory usage and slower performance, particularly in read-heavy scenarios.
No-Tracking Queries
On the contrary, No-Tracking queries do not track the retrieved entities. This means that upon executing these queries, no entity will be monitored for changes. By opting for No-Tracking, you reduce memory usage and increase speed, making these queries particularly effective for read-only scenarios.
- Use Cases: Suitable for applications where entities are only required for display or reporting.
- Benefits: Reduced tracking overhead, resulting in faster execution.
To opt for No-Tracking, use the method in your queries:
Query Optimization Techniques
Improving query performance is crucial for any application using Entity Framework Core. Below are some practical techniques that can enhance the efficiency of your database interactions:
- Select Only Required Columns: Instead of fetching all columns from an entity, fetch only those that are necessary. This minimizes the amount of data transferred.
- Paging: Implement paging to avoid loading all records into memory at once. This can be achieved using the and methods.
- Compiling Queries: For frequently executed queries, consider compiling them. This reduces the overhead of parsing and planning the query each time it's executed.
- Batch Updates and Deletes: When modifying multiple records, batch processing can reduce the number of round trips to the database.
By thoughtfully applying these techniques, developers can avoid common pitfalls and ensure their applications perform efficiently, ultimately leading to a better user experience.
Epilogue
The conclusion of this article highlights the critical aspects of mastering Entity Framework Core. Understanding Entity Framework Core is not merely an academic pursuit; it has real-world implications for software development. This framework allows developers to interact with databases using .NET objects, simplifying data manipulation and leading to efficient application design. With its capabilities, developers can focus more on the business logic, thus increasing overall productivity.
Key benefits of emphasizing Entity Framework Core in this article include its easy integration with various databases, support for LINQ queries, and providing a clear structure for managing data. Moreover, the robust community backing ensures continued innovation and support, making it an excellent choice for both beginners and experienced developers.
Considerations around Entity Framework Core also deserve mention. While it streamlines many tasks, developers must understand its limitations, such as performance trade-offs when managing large datasets compared to raw SQL queries. Therefore, a balanced perspective on using this tool is essential for effective data management in any application development process.
"Entity Framework Core enables developers to work with data in a more object-oriented way, thus reducing the amount of code required for common operations and increasing maintainability."
Summary of Key Points
- Importance of Entity Framework Core: It streamlines database interactions and improves productivity.
- Core Features: LINQ support, easy integration, and efficient data manipulation.
- Considerations: Understanding of performance implications and when to prefer raw SQL.
Further Learning Resources
To solidify your understanding and keep updated with Entity Framework Core, consider the following resources:
- Entity Framework Core Documentation
- Wikipedia on Entity Framework
- Reddit Community for discussions and tips.
- Online courses on platforms like Coursera or Pluralsight focused on Entity Framework Core.
These resources will provide you with deeper insights, practical examples, and a supportive community to enhance your learning journey.