Mastering Dynamic Programming: A Complete Overview
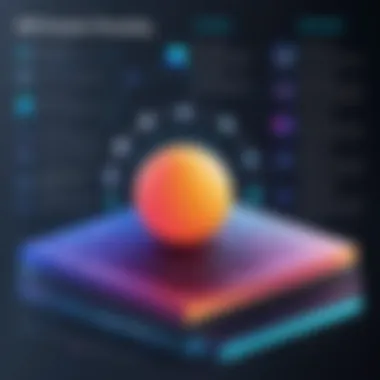
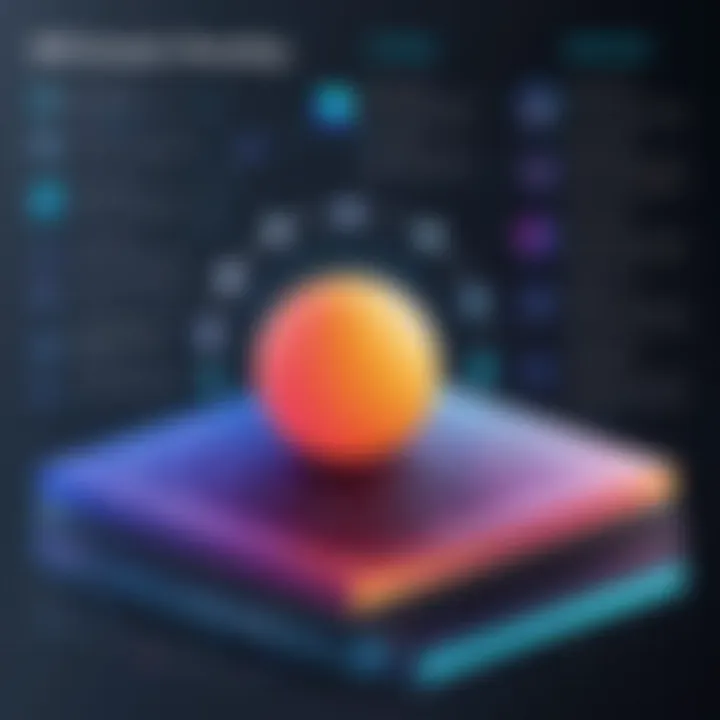
Intro
Dynamic programming (DP) is a methodology that is particularly effective in tackling problems with overlapping subproblems and optimal substructure. Essentially, dynamic programming is about breaking a problem down into simpler parts, solving each part just once, and storing their solutions. This technique shines when faced with computational issues that would otherwise be prohibitively time-consuming. It’s not just a theoretical construct—it's widely applied in various fields such as operations research, computer science, and even economics.
In this guide, we’ll journey through the intricacies of dynamic programming. Consider fixed points like the Fibonacci sequence or the knapsack problem as our touchstones. We’ll start from the fundamentals and progressively tackle more sophisticated topics, ensuring you grasp not only how to implement these techniques but also the underlying principles driving them.
As we delve into the realms of dynamic programming, you’ll find it’s about more than just memorizing algorithms. It's about developing an intuition for when and how to apply DP.
What You’ll Learn
In this comprehensive guide to dynamic programming, expect to uncover the following:
- A clear understanding of dynamic programming principles.
- Step-by-step approaches to solving specific problems.
- Practical examples to solidify your understanding.
- A variety of resources to further your learning.
This journey will equip you with the tools needed to handle complex programming challenges significantly. As programming languages evolve, so does the application of DP, making it a timeless skill for coders of all levels.
Understanding Dynamic Programming
Dynamic programming is not just a mere technique but a transformative approach to algorithm design. It holds significant importance in this article as it lays the groundwork for understanding how to tackle complex problems through breaking them down into manageable pieces. This section will delve into the critical aspects of dynamic programming, shedding light on its definition, historical evolution, and how it stands in comparison to other problem-solving strategies.
Definition and Significance
At its core, dynamic programming is a method used in computer science to solve problems by breaking them down into simpler subproblems. These subproblems are solved independently, and solutions to these intermediate stages are stored for future reference. A classic example can be seen in the Fibonacci sequence, where instead of recalculating values multiple times, storing previous results can significantly enhance efficiency.
The significance of mastering dynamic programming cannot be overstated. It allows programmers to handle complex challenges efficiently. Not only does it improve performance by optimizing run time, but it also equips developers with a versatile toolkit applicable in various domains, ranging from software development to operations research.
Historical Context
Dynamic programming emerged in the mid-20th century, chiefly credited to Richard Bellman. Originally developed to enable complex decision-making processes, Bellman’s work laid the foundation for future advancements in algorithm design. The approach was initially demonstrated in the realm of Economics, where it helped solve multi-stage problems involving resource allocation.
Over the years, thanks to advancements in computing power and algorithmic thinking, dynamic programming has expanded its reach. Early applications were limited, but today, it finds use in numerous fields, including artificial intelligence, game theory, and bioinformatics. Understanding its historical context is crucial as it provides insights into how these advancements have shaped modern applications.
Comparison with Other Techniques
When examining dynamic programming, it's vital to compare it to other algorithmic strategies like greedy algorithms and divide-and-conquer. While the greedy approach makes the optimal choice at each step hoping to find a global optimum, dynamic programming ensures that an overall solution is built from optimal sub-solutions.
- Dynamic Programming: optimally solves problems by storing results of subproblems.
- Greedy Algorithms: makes local optimal choices aiming for a global optimum but does not guarantee it.
- Divide-and-Conquer: breaks down a problem into smaller, independent problems, but does not take advantage of overlapping subproblems.
This nuanced understanding is essential for students and learners as it helps in zeroing in on when to apply dynamic programming over other methods. By grasping these differences, one can better navigate the complexity of algorithms and choose the right method for solving specific challenges.
Core Principles of Dynamic Programming
Understanding the core principles of dynamic programming is foundational for anyone venturing into this field. These principles act as the backbone of the methodology, revealing how dynamic programming makes what seems daunting into manageable pieces. By mastering these concepts, a programmer can tackle a wide array of complex problems efficiently.
Optimal Substructure
At the heart of dynamic programming lies the principle of optimal substructure. This concept essentially means that the optimal solution to a problem can be constructed from optimal solutions to its subproblems. Think of it like a well-constructed house: the integrity of the entire structure depends on the strength and quality of its individual bricks.
For instance, consider the problem of finding the shortest path in a graph. If you know the shortest paths from point A to B and from B to C, you can deduce that the shortest path from A to C includes B. This not only simplifies the way we approach the problem but also facilitates the reuse of previous results, saving computation time and resources.
Here’s an insightful way to view it: If you have to climb a mountain, you focus on reaching one peak at a time. Each peak's ascent should lay a strong foundation for the next. Thus, the optimal path up that mountain can be seen as a series of optimal climbs.
Overlapping Subproblems
Another essential principle is overlapping subproblems. Unlike traditional problem-solving techniques, which typically tackle distinct subproblems independently, dynamic programming exploits the fact that many problems can be broken down into smaller, overlapping subproblems that recur multiple times.
For example, in calculating the nth Fibonacci number, we find that Fibonacci(n) involves Fibonacci(n-1) and Fibonacci(n-2). Both of these subproblems arise repeatedly depending on how far along the sequence you are. By storing the results of these subproblems (a technique called memoization), we avoid recalculating them each time they are needed, vastly improving efficiency.
You might imagine this like a student revisiting study notes. If the student wrote down a solution to a complicated algebra problem, they wouldn't redo the entire problem from scratch; they'd simply refer back to their notes. This approach reduces effort and time significantly.
"The key to effective dynamic programming is recognizing and leveraging the connections between problems rather than viewing them in isolation."
In summary, the principles of optimal substructure and overlapping subproblems are pivotal in dynamic programming. By fully grasping these concepts, programmers can not only make their code more efficient but also simplify the complexity of problems that, at face value, can seem insurmountable. This principled approach lays a solid foundation for tackling a variety of dynamic programming challenges as we further explore this field.
The Dynamic Programming Approach
The Dynamic Programming Approach represents a cornerstone of efficient algorithm design. It encompasses two primary strategies: top-down and bottom-up. Both methods aim to tackle complex problems by simplifying them into manageable subproblems and utilizing previously solved subproblems to inform the current solution. Understanding these approaches is essential for anyone delving into dynamic programming, as this knowledge lays the foundation for solving a variety of challenges across different scenarios and programming languages.
When selecting between top-down and bottom-up strategies, one needs to consider factors such as memory usage, complexity of the problem, and personal familiarity with the concepts. Moreover, the idiosyncrasies of each approach can lead to different performance outcomes based on the nature of the problem at hand.
Top-Down versus Bottom-Up
Memoization in Top-Down
The memoization technique in the top-down approach allows programmers to store the results of expensive function calls and reuse them when the same inputs occur again. This method is particularly advantageous for recursive algorithms where the same subproblems might be recalculated multiple times. By caching results, it reduces the overall computational time significantly.
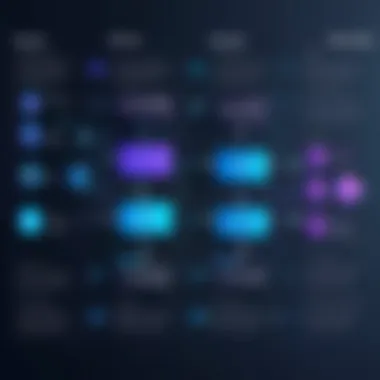
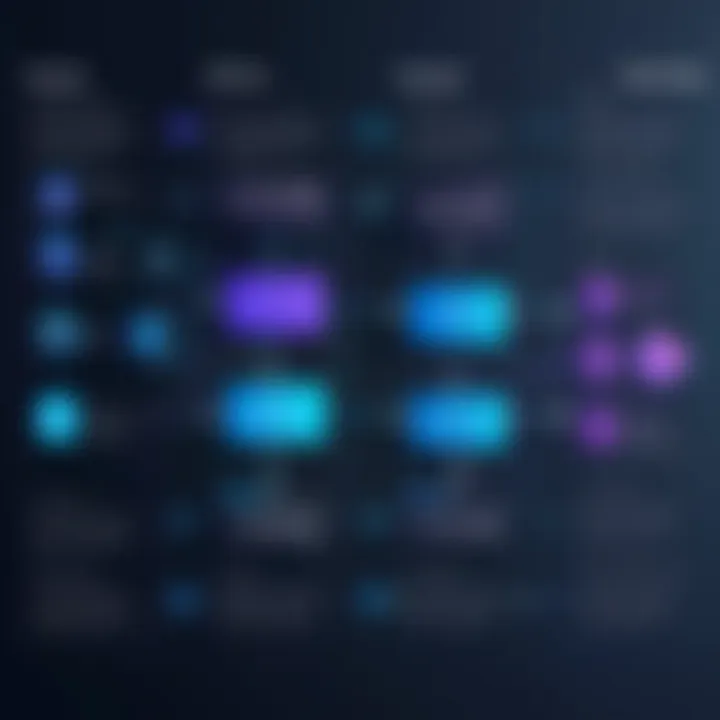
A key characteristic of memoization lies in its simplicity and ease of understanding. For beginners, it's often seen as a more intuitive option since it mirrors the conventional recursive approach most programmers learn first. The unique feature here is that it only computes values as they are needed, which conserves resources until those values are necessary.
However, memoization comes with trade-offs. As results are stored in memory, it can lead to increased space consumption. If not correctly managed, especially for large datasets, the memory footprint can become cumbersome. On the other hand, when properly utilized, it significantly accelerates performance, making it a popular choice in scenarios where redundancy is high, like in the Fibonacci sequence problem.
Tabulation in Bottom-Up
Tabulation is a distinct approach that builds a table iteratively and utilizes it to find the solution to the original problem. Instead of starting from the top (the final solution) and working down to the base cases, it fills in the table from the ground up. This means that, unlike memoization, every possible subproblem is solved before the larger problems, which helps in ensuring that all required data is available at all times.
One standout feature of tabulation is its efficiency in both time and space complexity. Since it computes all possible subproblems ahead of time, you can avoid repeated calculations altogether. This systematic manner often results in faster runtimes, especially for problems involving a large amount of overlapping subproblems.
Nevertheless, tabulation also has its downsides. It demands a more structured approach and requires knowing the size of the problem in advance. Additionally, it’s less friendly for problems that are inherently recursive or require backtracking.
In short, while memoization suits situations where flexibility is paramount, tabulation shines when one values structure and predictability. Choosing the right approach will ultimately depend on the problem characteristics and the programmer’s comfort level.
Choosing the Right Approach
Selecting between top-down and bottom-up approaches in dynamic programming is crucial and can significantly affect the efficiency of your solution. Some factors to consider include:
- Nature of the problem: If it’s recursive and involves overlapping subproblems, memoization might be the way to go. For problems where a structured tabulation can help, consider the bottom-up method.
- Memory constraints: If you are limited on space, memoization could prove advantageous as it only stores necessary computations.
- Performance requirements: Some problems may have strict time constraints that could lead you to prefer one method over the other based on expected runtimes.
"Choosing the right approach in dynamic programming isn’t just about picking a method; it’s about understanding the problem and the resources available to you."
By weighing these considerations, you'll be in a better position to make an informed decision that saves time and optimizes your resources. Mastering the dynamic programming approach is key to effectively tackling both theoretical and practical programming challenges.
Common Dynamic Programming Problems
Dynamic programming is a powerful tool for tackling a myriad of problems that often appear deceptively simple on the surface. Understanding these common dynamic programming problems is significant not just for honing problem-solving skills but also for practical applications in various fields such as computer science, operations research, and artificial intelligence. Each of these problems has its unique set of challenges and learning them enriches one’s programming arsenal. By dissecting intricate problem statements, one benefits from improved critical thinking and analytical skills.
Fibonacci Sequence
The Fibonacci sequence is often the first encounter students have with dynamic programming. It’s a simple sequence where each number is the sum of the two preceding ones, usually starting with 0 and 1. While the math is simple, calculating Fibonacci numbers recursively leads to inefficiency due to overlapping calculations. The naive recursive version recalculates values multiple times, making it exponentially slow.
To solve this efficiently, one can leverage memoization or tabulation. By storing already computed values, we can repurpose this data instead of recalculating it. This method simplifies complexity from exponential time to linear time. Here’s a straightforward example using memoization:
This snippet illustrates how to improve performance dramatically. It's not just about finding a number in a sequence; it lays the groundwork for more complex algorithms.
Knapsack Problem
The Knapsack Problem centers around resource allocation and optimization. Imagine a thief faced with a knapsack that can carry a limited weight. Each item has both a weight and a value, and the objective is to maximize value without exceeding the weight limit. This problem is notable because it encourages strategizing to achieve the best outcome.
Dynamic programming offers a way to break down the problem into manageable parts, allowing for a systematic approach. You can create a table that tracks the maximum value achievable for every sub-problem determined by the combination of items up to the capacity. Specifically, the time complexity of this algorithm is O(nW), where n is the number of items and W is the capacity of the knapsack. This is substantially more efficient than brute-force methods, which can quickly become unwieldy.
Longest Common Subsequence
Finding the Longest Common Subsequence (LCS) between two sequences is a quintessential example of how dynamic programming can simplify complex relationships. Suppose you have two strings, and you want to find the length of the longest sequence that can appear in both, albeit not necessarily contiguously. It’s a search for patterns and optimization in data, useful in fields like bioinformatics for DNA sequencing.
Using dynamic programming, the problem translates into constructing a 2D array to store lengths of common subsequences up to each character position of the two strings. The recursive relations guide the filling of this table effectively. The LCS has a time complexity of O(mn), with m and n as the lengths of the two sequences.
Edit Distance
Edit Distance, also known as Levenshtein distance, quantifies how dissimilar two strings are by counting the minimum number of operations needed to transform one string into the other. The operations typically considered are insertion, deletion, and substitution. This concept is not only academically intriguing but finds practical implementation in applications like spell-checkers and DNA sequence analysis.
Dynamic programming is particularly effective for computing edit distance due to its overlapping subproblems. Similar to LCS, a 2D array can be leveraged to keep track of the costs associated with substrings. The final result resides in the cell that reflects the distance for the entire length of both strings. The naive approach can be inefficient for long strings, but dynamic programming reduces the time complexity to O(mn).
"The beauty of dynamic programming is its ability to simplify a complex problem through thoughtful decomposition, transforming it into manageable pieces that are both insightful and efficient."
In summary, grasping these core dynamic programming problems amplifies one’s mastery over a critical computational technique. Recognizing and applying the principles of dynamic programming to real-world challenges offers a rewarding triumph over computational limits.
Dynamic Programming in Different Programming Languages
The implementation of dynamic programming can vary significantly across programming languages, each offering unique features and capabilities. Understanding how dynamic programming operates within different languages not only enriches a programmer’s toolkit but also highlights the importance of language choice in solving algorithmic challenges efficiently. Mastering dynamic programming in languages like Java, C, and C++ can lead to more effective solutions that leverage the nuances and strengths of each language.
Dynamic Programming with Java
Java, being an object-oriented language, provides a robust framework for implementing dynamic programming solutions. Its rich APIs and built-in data structures enhance problem-solving flexibility. Java’s emphasis on code readability and maintainability is beneficial when tackling complex dynamic programming problems.
Consider a classic example, the Fibonacci sequence. Here’s an illustration of dynamic programming through memoization in Java:
In this code snippet, by storing previously calculated results, Java minimizes redundant calculations, showcasing how dynamic programming can drastically cut down on computation time.
Dynamic Programming with
C is known for its speed and efficiency, making it a great choice for performance-critical applications. The manual memory management in C offers developers the ability to optimize their programs at a low level, which can be leveraged well in dynamic programming scenarios. Here’s how you can solve the same Fibonacci problem using an iterative approach with a bottom-up technique:
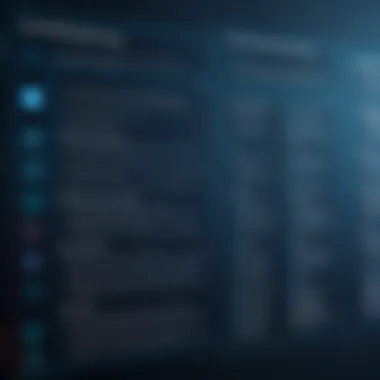
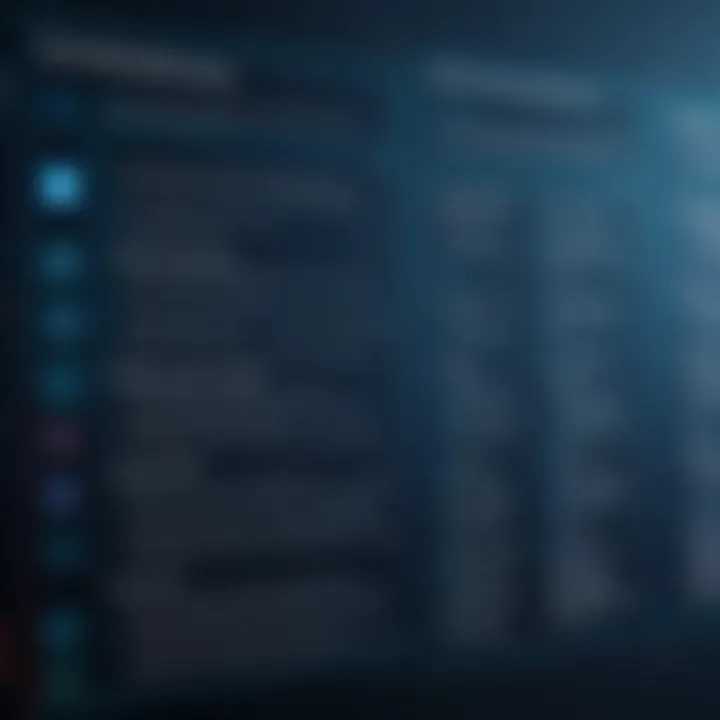
In this example, the space required for storing intermediate results can be minimized further, making C an ideal option when optimizing performance and memory usage is crucial.
Dynamic Programming with ++
C++ builds on the foundation of C, offering strong object-oriented capabilities along with features like Standard Template Library (STL) that facilitate dynamic programming implementations. The STL provides containers that can simplify the coding process and allow programmers to focus on logic rather than data structure management. Here’s a simple code snippet demonstrating the Knapsack Problem using C++:
With C++, you can optimize both time and space complexity while enjoying the benefits of high-level programming. In summary, each programming language offers its distinct advantages for implementing dynamic programming. Java shines with its readability and object-oriented features, C offers maximum efficiency, and C++ combines these strengths nicely with its powerful libraries.
Mastering dynamic programming across multiple languages can enhance problem-solving efficiency, making you a more versatile programmer.
By understanding how to implement dynamic programming in Java, C, and C++, you'll be equipped with critical skills that allow you to tackle a diverse range of algorithmic challenges proficiently.
Practical Tips for Mastering Dynamic Programming
Diving into dynamic programming can feel like navigating a labyrinth at times. It’s a powerful technique, and getting a grip on it is essential for any programmer looking to elevate their skills. Practical tips can act as your compass, guiding you through intricacies with more confidence.
The importance of practical tips lies in their ability to demystify the process and provide actionable insights. By understanding how to approach problems and which resources can aid your learning, you will find yourself better equipped to tackle complex challenges.
Practice and Application
Practice makes perfect, as the old saying goes, and this is especially true when it comes to dynamic programming. Engaging with problems regularly is crucial. Familiarity breeds not only understanding but also intuition. Start small, perhaps with simpler problems like the Fibonacci sequence, before moving on to more complicated scenarios, such as the knapsack problem.
Hands-on experience with varied problems will build your analytical thinking skills. It encourages you to identify patterns and articulate steps clearly. The more you code, the easier it becomes to translate a problem statement into a structured approach.
Utilizing Online Resources
There’s a treasure trove of online resources available for mastering dynamic programming. Whether it's through structured courses or challenge platforms, online offerings are expansive and can cater to varied learning styles.
Online Courses
Online courses present a structured way to absorb concepts surrounding dynamic programming. With these, you often find a blend of theoretical understanding and practical exercises. One of the standout characteristics of online courses is their flexibility; you can learn at your own pace, which is a boon for busy students.
For instance, platforms like Coursera or edX often partner with reputable universities to offer high-quality content. A unique feature of these courses is their community forums, where students can discuss problems, share insights, and gain additional perspectives. However, on the flip side, some learners may find them overwhelming due to the sheer volume of information available.
Programming Challenges
Engaging in programming challenges builds both skill and confidence. Websites like LeetCode and HackerRank offer a myriad of problems specifically designed around dynamic programming. The beauty of these challenges lies in their diversity, ranging from beginner to expert levels.
One key characteristic of programming challenges is the instant feedback you get; when you submit a solution, you often receive immediate evaluation. This helps in understanding what works and what doesn’t. It's an exhilarating way to push personal boundaries and attempt to solve problems under constraints, which mirrors real-world scenarios. Yet, tackling these challenges can sometimes lead to frustration, especially when you hit a wall.
Collaborative Learning
Finally, don’t underestimate the power of collaborative learning. Engaging with peers can provide fresh insights and motivate you to tackle tougher problems. Study groups or coding bootcamps can serve as platforms for discussing strategies and sharing resources.
Advanced Techniques in Dynamic Programming
Dynamic programming is more than just an algorithmic strategy; it’s a powerful toolkit that allows programmers to dissect and solve complex problems elegantly. Within this realm lie several advanced techniques that enhance the effectiveness of dynamic programming approaches. Emphasizing these techniques enriches your toolkit, enables you to optimize your code, and improves your ability to tackle dynamic programming challenges across various scenarios. Let’s delve into these advanced techniques, highlighting key strategies and practical implications.
Space Optimization Techniques
When dealing with dynamic programming problems, maintaining an efficient use of space can significantly influence performance, especially when input sizes are enormous. Traditional dynamic programming solutions often require substantial memory allocation to store intermediate results, which can bog down performance or even lead to memory overflow.
Space optimization techniques come into play to address these concerns. There are a few notable methods to effectively manage memory:
- State Compression: This strategy involves identifying the essential components of state information and compressing it. Instead of storing all values in a table, you only keep track of the minimum necessary variables. For example, when calculating the Fibonacci sequence, rather than using an array to store all values, you can simply maintain the last two computed results.
- Iterative Approach: Rather than employing deep recursion, some problems can be transformed into iterative solutions that use a slim amount of space. This often entails reworking the recursive formula into a loop while maintaining only the current and previous states.
Here's a simple example of how you might implement the Fibonacci sequence using space optimization:
This example illustrates that, even with a linear time complexity, you can effectively optimize space usage from O(n) to O(1), making it both memory-efficient and quicker.
Advanced Problem Solving Strategies
Mastering dynamic programming involves not just understanding basic techniques but also implementing advanced problem-solving strategies that can tackle quirky problems efficiently. Here are several strategies that can prove invaluable:
- Bitmasking: This is an advanced technique that uses binary representation to handle subsets or combinations of data effectively. By encoding possible states or solutions in bits, you can perform operations in a compact form. Bitmasking can help particularly with problems involving sets or multi-dimensional states, reducing complexity significantly.
- Segment Trees and Fenwick Trees (Binary Indexed Trees): For certain types of dynamic programming problems—especially those requiring frequent updates and queries—these tree structures offer an efficient means to manage ranges and queries. They allow you to balance preprocessing time against query time dramatically, which can be essential in competitive programming.
- Topological Sorting for DAGs: In cases where your problem forms a directed acyclic graph, employing topological sorting can provide a neat way to ensure that all dependencies are resolved before you calculate the final answers. This is particularly useful in project scheduling and dependency resolution problems.
Overall, leveraging these advanced strategies allows you to look at problems from unique angles. The adaptability these methods provide can be a game changer, especially when faced with challenges that first appear implausible to solve with standard dynamic programming approaches.
By incorporating these advanced techniques into your programming repertoire, you not only increase your ability to solve problems more effectively but also prepare yourself for the subtle nuances of real-world applications of dynamic programming. As challenges vary, these methods provide robust frameworks through which solutions can be crafted, reinforcing the importance of remaining agile in your problem-solving approach.
"With great power comes great responsibility," as the saying goes; an adept understanding of dynamic programming's advanced techniques ensures you're well-prepared to leverage its full potential in any scenario.
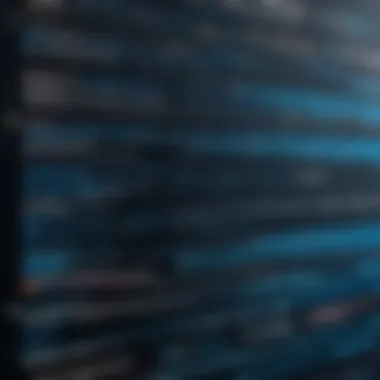
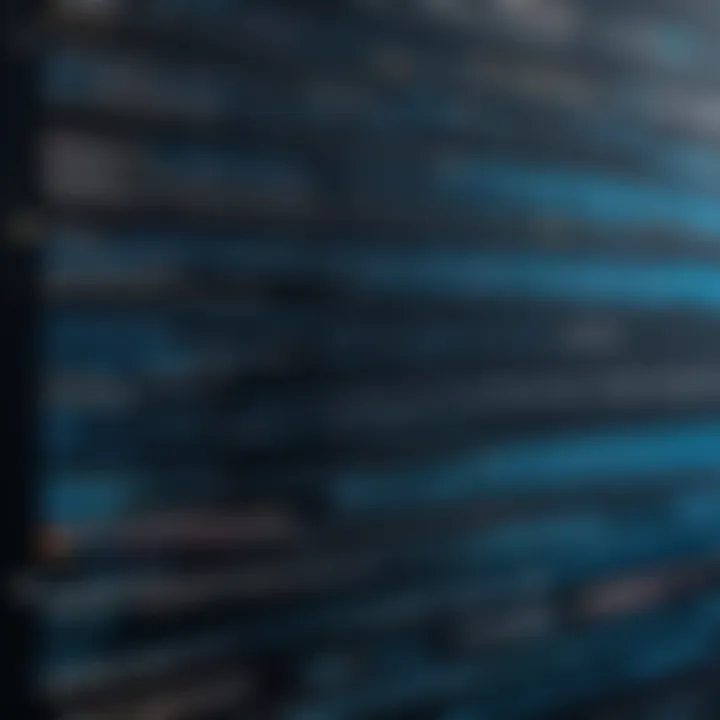
Understanding Complexity Analysis
In the landscape of algorithm design, the concepts of time and space complexity serve as fundamental pillars that support the efficient application of dynamic programming. These not only influence performance but are also critical indicators of an algorithm's scalability and reliability under varying conditions. Grasping how these complexities interplay provides invaluable insight into the viability of a dynamic programming solution, especially in resource-constrained environments.
Time Complexity
Time complexity represents the amount of computational time an algorithm requires to run as a function of the input size. It's crucial to understanding how different algorithms compare in speed and efficiency, allowing programmers to select the most appropriate solution based on specific requirements. The nuances of time complexity deepen in dynamic programming, where overlapping subproblems can lead to significant optimizations.
When dealing with recursive algorithms, a naive implementation might run redundantly through the same calculations multiple times. Here’s where dynamic programming comes into play, using techniques like memoization to enhance efficiency by storing previously computed values.
To illustrate, let’s consider the Fibonacci sequence. A straight-up recursive approach can lead to exponential time complexity due to repeated work. In contrast, an implementation using dynamic programming can reduce it to linear time complexity:
The transition from exponential to linear time complexity not only optimizes execution but also enhances user experience in applications relying heavily on computational resources. Understanding these time metrics is essential, especially when deciding how to structure a specific dynamic programming problem for the best performance possible.
Space Complexity
Space complexity, on the other hand, evaluates the amount of memory an algorithm utilizes as a function of input size. This is crucial, especially in applications where memory resources could be limited, or optimal use of available memory is desired. As dynamic programming often requires the storage of previously computed results, understanding space complexity can be fundamental in crafting efficient solutions.
In many dynamic programming solutions, one might encounter scenarios where space usage can be excessive. For example, in the case of solving the Fibonacci sequence, while memoization reduces time complexity, it can lead to increased space usage for storing results. However, strategic approaches exist to reduce space complexity, such as transitioning from storing all results to only retaining the last two computed values:
By analyzing both time and space complexities, programmers are better equipped to strike a balance between efficiency and resource allocation. This enables them to choose or tailor algorithms that not only meet performance criteria but also function effectively within the constraints of their computational environments.
"The analysis of complexity shapes the foundation of algorithmic thinking, guiding programmers toward optimized solutions."
In summary, delving into complexity analysis opens the door to mastering the intricacies of dynamic programming, underscoring its relevance in practical applications. By understanding both time and space considerations, learners can equip themselves with a robust framework for tackling complex problems head-on.
Dynamic Programming in Real-World Applications
Dynamic programming, a powerful tool in computing, transcends theoretical problems and finds its way into real-world scenarios across various fields. The significance of applying dynamic programming in these settings cannot be understated. It enables efficient solutions to problems laden with constraints and intricacies, optimizing processes that would otherwise become infeasible due to time or resource limitations.
With its hallmark of breaking down problems into manageable components, dynamic programming provides both flexibility and clarity. As we explore its real-world implications, we'll see how this methodology paves the way for more sophisticated solutions in various domains, enhancing both functionality and innovation.
Dynamic Programming in Artificial Intelligence
In the vast realm of artificial intelligence (AI), dynamic programming plays a critical role, particularly in areas such as reinforcement learning and decision-making processes. Here, algorithms often need to evaluate numerous potential outcomes based on various inputs. Dynamic programming streamlines this by breaking down a complex decision-making process into simpler, recursive ones.
Key Applications:
- Reinforcement Learning: Algorithms like Q-learning utilize dynamic programming principles to update the value of actions based on past experiences, allowing machines to make informed decisions.
- Natural Language Processing (NLP): Techniques such as Viterbi algorithms apply dynamic programming to optimize sequences of events, invaluable for tasks like speech recognition.
- Computer Vision: Dynamic programming helps in optimizing the segmentation of images, which is critical for identifying and classifying objects within visual data.
The capacity to handle vast datasets with many variables makes dynamic programming especially appealing. Implementing these techniques not only enhances learning efficiency but also reduces computational costs significantly.
Dynamic Programming in Operations Research
Operations research (OR) focuses on making optimal decisions based on mathematical methodology and analytical reasoning. Within this sphere, dynamic programming shines in managing resource allocation and scheduling problems. It permits complex decision-making tailored over multiple stages—think of it as navigating a maze where each turn affects future paths.
Notable Use Cases:
- Production Scheduling: Firms utilize dynamic programming to optimize production sequences, facilitating lower costs and improved delivery times.
- Supply Chain Management: By evaluating various supply routes and timings, dynamic programming allows companies to minimize transportation costs and maximize efficiency.
- Network Design: Here, dynamic programming algorithms can devise optimal layouts to ensure smooth information flow while minimizing redundancy.
In operations research, the adoption of dynamic programming ensures systematic problem-solving. It allows practitioners to evaluate potential scenarios over time, ultimately leading to informed choices that benefit both the organization and its stakeholders.
"Dynamic programming not only enhances decision-making but also simplifies processes that would otherwise be overwhelmingly complex."
By embracing dynamic programming in these real-world applications, businesses and researchers alike stand to gain significantly. Whether it's through improved efficiency or insightful analyses, this technique continues to be an indispensable ally in solving intricate challenges in today's fast-paced world.
Closure
The conclusion section is not merely an epilogue but a vital crux of the article that encapsulates the essence of what has been covered. By reflecting on the journey through dynamic programming, we've delved into a myriad of concepts ranging from foundational principles to intricate applications across various domains. This reflection prompts one to consider what they have gained alongside how these insights can help with future endeavors in programming.
Dynamic programming, when mastered, offers significant advantages. Not only does it equip programmers with the ability to tackle complex problems with efficiency, but it also fosters a deeper understanding of algorithm design. Emphasizing the importance of methodologies like top-down and bottom-up approaches facilitates the selection of the appropriate strategies tailored to each unique problem case.
Furthermore, considering the role of dynamic programming in real-world applications such as artificial intelligence and operations research, one can appreciate its relevance in contemporary tech landscapes.
In summary, mastering dynamic programming allows one to hone their analytical skills, thereby enhancing both problem-solving capabilities and programming proficiency.
Reflecting on Mastery
The journey to mastering dynamic programming is filled with challenges and rewards. Reflection is key in solidifying knowledge and understanding the application of learned techniques. It's common for students to wrestle with concepts at first, but through continuous practice, the light bulb moments will come as familiarity builds.
As learners, it's essential to revisit previous sections periodically. This could be through coding challenges in platforms like leetcode.com or discussing concepts on forums like reddit.com, which can shine a new light on tried-and-true principles. Each new problem adds layers to the understanding of foundational techniques and enhances one's ability to think critically about solutions.
Here are key points to consider when reflecting on your mastery of dynamic programming:
- Practice Regularly: Like any skill, the more you engage with dynamic programming, the more intuitive the concepts become.
- Collaborate and Discuss: Engaging with peers not only reinforces personal understanding but often sparks insights that may not have been considered before.
- Keep Learning: Technology evolves, and so must your skills. Staying updated through online courses and resources will help you stay sharp.
"The only way to achieve mastery is through consistent learning and practice."
Ultimately, mastery in dynamic programming leads to increased confidence in problem-solving abilities and a more profound appreciation of the nuances within algorithm design. This is not just about learning; it’s about evolving as a programmer.