Mastering Dynamic Programming for Coding Interviews
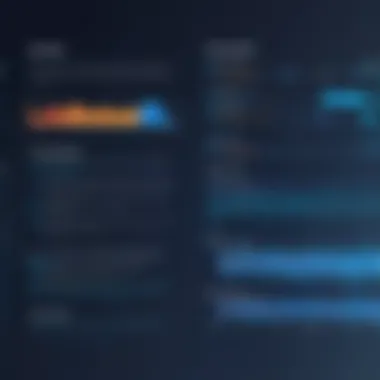
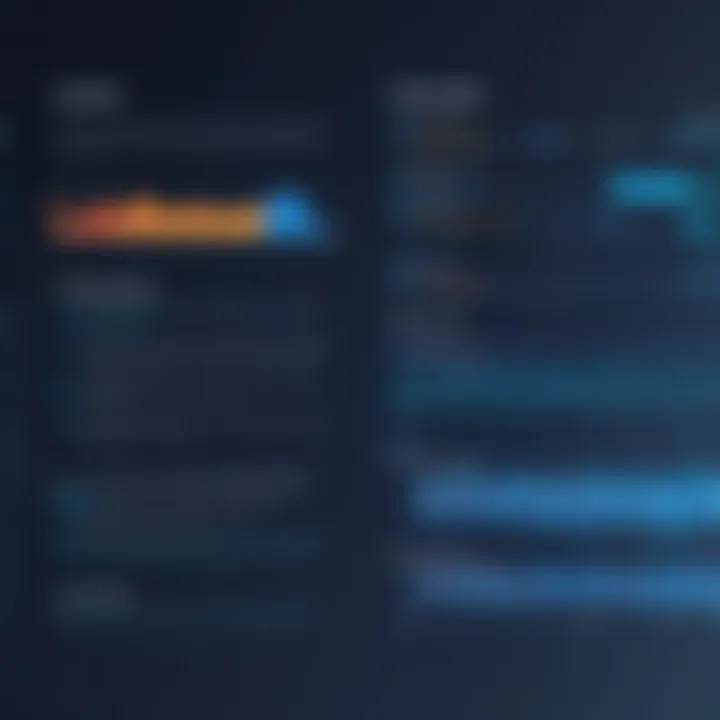
Intro
Dynamic programming is one of those concepts that draws a line between good programmers and exceptional ones, especially in coding interviews. If youāve ever grappled with a problem that feels insurmountable, you might have unconsciously touched on the principles of dynamic programming. This technique is like having a secret weapon in your programming arsenal, helping you break down complex problems into manageable pieces. But what exactly is dynamic programming? How did it become such a focal point in technical interviews?
In this article, weāll explore these questions in depth. Weāll dive into what dynamic programming is, how itās utilized in coding interviews, the common traps candidates fall into, and strategies for overcoming these challenges. Although dynamic programming can seem daunting at first, once you grasp the fundamentals, it starts to clickālike a lock to a key.
The significance of mastering dynamic programming cannot be understated. Interviews at tech giants like Google and Amazon frequently include problems that can only be solved effectively using this technique. Those who arrive prepared demonstrate not just their coding skills, but also their ability to think critically and problem-solve. Are you ready to enhance your coding interview skills? Letās get started.
Understanding Dynamic Programming
Dynamic programming is fundamentally about breaking a problem into simpler, overlapping subproblems and solving each of those only once, storing their solutions for future reference. Imagine it like compiling a cookbook: you donāt need to write out the recipe each time you want to bake a cake. Instead, you note down the key steps once and refer back to them whenever necessary.
Key Concepts in Dynamic Programming
- Optimal Substructure: This principle implies that a problem's optimal solution can be constructed efficiently from optimal solutions of its subproblems.
- Overlapping Subproblems: Unlike divide-and-conquer strategies that tackle distinct subproblems, dynamic programming addresses subproblems that appear multiple times.
Common Approaches
- Memoization: This is a top-down approach where you solve the larger problem first and cache the results of the subproblems to prevent redundant calculations.
- Tabulation: In contrast, this bottom-up approach involves filling out a table based on the solutions to the smallest subproblems first.
Why It Matters in Coding Interviews
The ability to recognize when to use dynamic programming can be a game changer in technical interviews. It showcases a deep understanding of algorithmic design and the ability to handle complexity, which are coveted skills in any software development position.
Aim of This Guide
The aim of this guide is to first, break down the principles of dynamic programming with clear examples, and second, provide strategies to navigate common pitfalls often faced in coding interviews. We will intersperse practical code snippets to clarify the concepts discussed, ensuring a comprehensive understanding.
"In the world of programming, the codes you create tell not just a story, but often reveal your analytical prowess and depth of understanding."
As we move forward, buckle up and prepare for a deep dive into the world of dynamic programming!
Understanding Dynamic Programming
Dynamic programming is a cornerstone concept in computer science and programming interviews. It revolves around breaking down complex problems into simpler subproblems and leveraging solutions to these subproblems to construct a solution for the overall problem. Understanding this methodology not only enhances oneās problem-solving repertoire but also paves the way for more efficient coding practices.
The importance of mastering dynamic programming lies in its wide-ranging applicability to a myriad of problems. From optimizing resource allocation in logistics to constructing efficient algorithms for complex combinatorial problems, the principles of dynamic programming can be the golden key to unlocking solutions in coding interviews. Not only does it equip candidates with a powerful tool for tackling challenging questions, but it also demonstrates a depth of understanding in algorithm design, which is invaluable in any technical role.
Definition and Importance
At its core, dynamic programming is a method used for solving problems by breaking them down into simpler, overlapping subproblems, storing the results of these subproblems to avoid redundant computation. This reduces the computational overhead and optimizes performance, making it particularly critical when faced with problems that feature exponential time complexity if approached naively.
In coding interviews, knowing how to implement dynamic programming can set candidates apart from others. The interviewers often look for efficient solutions, and understanding dynamic programming allows candidates to propose elegant solutions that others might miss. This skill demonstrates not just technical proficiency but a strategic mindset capable of seeing problems from various angles.
Historical Context
The roots of dynamic programming emerge from the early days of computer science, with its formulation attributed to Richard Bellman in the 1950s. Bellman was working on optimization problems and noticed that many complex problems shared overlapping subproblems, leading to significant computational waste when solved directly. He conceptualized dynamic programming as a systematic approach to handling such scenarios through a methodical breakdown and solution storage, revolutionizing algorithm theory.
Over the decades, dynamic programming evolved and became foundational in several domains, such as operations research, economics, and artificial intelligence. Its historical significance is palpable; it not only contributed to the advancements in algorithm design but also laid the groundwork for modern computational approaches in solving real-world problems.
By understanding the historical evolution of dynamic programming, one appreciates the journey that brought us to its current status as a vital pillar of technical interviews in programming and computer science. This context enriches the learning experience and inspires individuals to harness these principles in their coding endeavors.
"Dynamic programming is like a map; it shows you the best paths to take when traversing through the complex landscape of algorithms."
Principles of Dynamic Programming
Dynamic programming stands as a cornerstone technique in computer science, especially for solving problems with lots of overlapping subproblems and optimal substructures. At its core, dynamic programming is about breaking down complex problems into manageable parts and ensuring that solutions are reused, thus saving time and resources. Understanding these principles can offer a strategic advantage during coding interviews, enhancing both efficiency and effectiveness in problem-solving.
Overlapping Subproblems
In many scenarios, problems may involve calculations of the same results multiple times. This is the essence of overlapping subproblems. For instance, consider the Fibonacci sequenceāa classic example in the dynamic programming realm. If you try to compute Fibonacci using a simple recursive approach without dynamic programming, you'll find a large number of repeated calculations. When calculating , you end up needing and , both of which would also require their previous Fibonacci numbers, repeatedly. This inefficiency blossoms exponentially.
To combat this, with dynamic programming, we can store results of the Fibonacci numbers in a structure like a list or array. This way, once a number is computed, it isn't recalculated. Think of it as writing down a recipe: rather than retracing steps every time, just glance at your notes. This leads to a significant reduction in computational complexity, switching from exponential time to linear time in this case. Essentially, recognizing overlapping subproblems can speed up the approach drastically.
Optimal Substructure
The second principle, optimal substructure, tells us that a problem can be broken down into smaller, simpler parts, which are then solved independently. The trick is that the best solution to the overall problem can be derived directly from the optimal solutions of its subproblems. A typical scenario where this comes into play is in pathfinding problems, like the shortest path in a graph.
Consider a graph with various paths connecting cities. If the path from city A to D passes through B, then the shortest route from A to D is equal to the sum of the shortest route from A to B and from B to D. Thus, you can approach these parts independently, yielding the overall optimal route.
This principle is crucial in scenarios like dynamic programming implementations of algorithms, where we need to ensure that solutions to subproblems guarantee a solution to the main problem. When you design your solution, always ask, "Can this larger problem benefit from smaller problems?" If the answer is yes, you've identified an optimal substructure!
In summary, the principles of overlapping subproblems and optimal substructure lie at the heart of dynamic programming. Recognizing their presence in problems is key to leveraging dynamic programming efficiently.
Understanding these principles not only prepares candidates for technical evaluations but also builds a formidable foundation for more advanced algorithmic designs. Having these lenses to view problems can significantly enhance one's approaches to software development. So, as one delves deeper into dynamic programming, keep these principles in your toolkitāyou'll find they illuminate the path forward.
Common Techniques in Dynamic Programming
Dynamic programming, often referred to in technical discussions, has two primary techniques that stand out: memoization and tabulation. Both methods help reduce the computational burden associated with recursive algorithms by storing intermediate results of computations. The essence of these techniques lies in their ability to tackle problems more efficiently. This leads us not only to theoretical gains but also to practical advantages when faced with time-constrained situations, such as coding interviews. Mastering these techniques can set candidates apart as they navigate complex problems with ease.
Memoization
Definition
Memoization is a technique where you store the results of expensive function calls and reuse them when the same inputs occur again. Think of it as a smart backpack, packing all the solved problems so you donāt have to redo the work. This approach is particularly useful when solving problems with overlapping subproblems, which are common in dynamic programming. The key characteristic of memoization is its focus on saving state; this offers a significant boost in efficiency when dealing with large inputs.
Use Cases
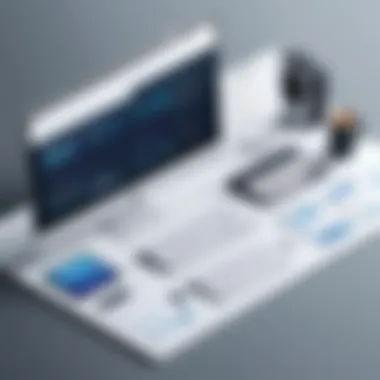
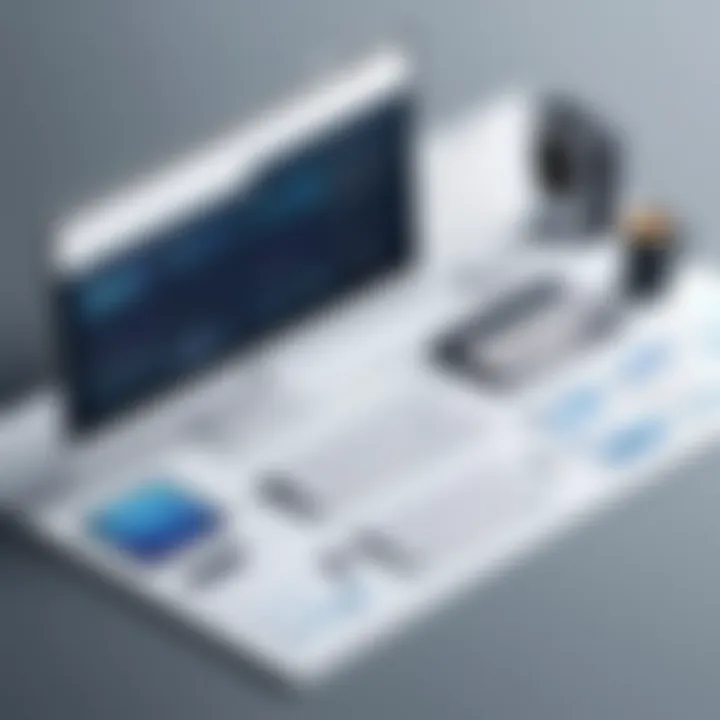
Memoization shines in problems where repeated calculations take place, such as those found in the Fibonacci sequence or the calculation of optimal paths in graphs. For instance, if you're organizing a trip and you need to find the shortest route to various points, memoization will save you time by not requiring the recalculation of already solved routes. One unique feature of memoization is that it can be applied in a top-down approach, making it flexible and intuitive for many programmers, especially those who prefer a recursive perspective.
Limitations
However, memoization is not without its downsides. While it can dramatically reduce time complexity by avoiding redundant calculations, it can consume considerable memory resources to store those intermediate results. For example, if a problem has a high branching factor or extensive call depths, this storage requirement could lead to inefficiencies or even application crashes due to memory overuse. Hence, while it's a beneficial and popular choice for optimizing recursive solutions, developers must weigh its memory cost against performance gain.
Tabulation
Definition
Tabulation, on the other hand, is a method where you solve a problem by filling out a table, typically in an iterative manner. Itās like planning out your grocery list, where you ensure everything's neatly organized before heading to the store. The key characteristics of tabulation are its bottom-up approachāthis allows you to systematically build your solution using previously computed values. This technique not only avoids the overhead that comes with recursive calls but also reduces the risk of running into stack overflow issues.
Use Cases
Youāll find tabulation quite effective in a variety of scenarios. A great example is the computation of the nth Fibonacci number where you build the sequence iteratively, storing results in a table. Problems like the longest common subsequence or even variations of the knapsack problem also benefit from this method. This unique approach of building up from base cases to the larger problem structure often leads to clearer code and can enhance debugging efforts.
Limitations
On the flip side, tabulation may require more upfront structuring and understanding of how to organize your data efficiently. It can lead to increased setup time when programming. Moreover, some problems might be too complex for this linear structure, requiring more nuanced handling. Also, since tabulation often necessitates comprehensive tables, this can lead to high memory usage similar to memoization. Just like with all techniques in dynamic programming, balancing time complexity with space constraints is key to good performance.
In the world of dynamic programming, choosing between memoization and tabulation often determines the efficiency of your solution. Each method has its unique strengths and challenges, and understanding both can be invaluable for anyone preparing for coding interviews.
By mastering these common techniques, candidates can align themselves with effective strategies that enhance their problem-solving arsenal. This is a step in the right direction toward acing those pesky coding interviews.
Dynamic Programming Approaches
Dynamic programming is a powerful tool often employed in tackling complex problems during coding interviews. The goal is to break down the problem into smaller, manageable pieces, thereby making it easier to solve. When it comes to dynamic programming, two main approaches come to the forefront: the top-down and bottom-up methods. Understanding these methods is essential for any programmer aspiring to excel in interviews, as they dictate how one may approach problem-solving with efficiency and clarity.
Top-Down Approach
The top-down approach refers to a recursive technique where the problem is divided into subproblems, which are solved individually. The results of these smaller problems are stored, often through a process called memoization. This technique prevents the same calculation from being performed multiple times, essentially caching outcomes to save time. Here's how you can see this works:
- Start with the main problem: Define your larger issue.
- Break it down: Identify the smaller subproblems involved.
- Solve recursively: Use functions to solve these subproblems, calling them as needed.
- Cache results: Store the results so that if they arise again, the program can access the stored outcome.
For instance, in calculating the Fibonacci sequence, a naive implementation would be to repeatedly calculate Fibonacci numbers. Instead, a top-down approach would store values, like so:
This method is especially beneficial when the input size is not overly large, as recursive calls can eat up memory and stack space quickly. Care must be taken to ensure that the base cases are defined accurately, to avoid unnecessary depth in the recursion.
Bottom-Up Approach
The bottom-up approach, unlike its top-down counterpart, builds up the solutions iteratively. It starts from the smallest subproblems and gradually solves larger problems by using previously computed results in a table or array format. This method is more space-efficient and often less prone to issues like exceeding the recursion limit.
- Initialize your table or array: This will store results for all subproblems.
- Begin with the base cases: These are the simplest forms of the problem.
- Iterate through the range: Fill in the table or array by solving each subproblem leading up to the larger problem using the stored results.
For instance, in solving the Fibonacci sequence using a bottom-up method, you can do:
This method is particularly useful in scenarios where you want to limit memory usage or optimize for iterative problem-solving. As a result, it can often run faster than the top-down approach in many practical applications.
In summary, understanding both approaches allows programmers to select the best method for the problem at hand. The top-down approach with memoization can save time at the cost of memory, while the bottom-up approach might be slower but typically uses less memory overall. Grasping these concepts is crucial not only for coding interviews but also for applying dynamic programming effectively in real-world applications.
Recognizing Dynamic Programming Problems
To tackle dynamic programming effectively, it's crucial to identify problems that can be solved using this method. Recognizing these problems enables programmers to approach challenges in a structured way, leading to efficient solutions that often outperform naive strategies. Mastery of this topic aids in not only solving a variety of coding problems but also in excelling during technical interviews, where time and clarity matter significantly.
Key Indicators
When you're faced with a coding problem, there are specific indicators that hint at the applicability of dynamic programming. The two main indicators to watch out for include:
- Recursive Structure: If the problem can be broken down into subproblems that resemble the original problem, it's a key sign. For instance, calculating the nth Fibonacci number can be expressed recursively, where . This path leads directly to overlapping subproblems, a hallmark of dynamic programming.
- Optimal Substructure: If an optimal solution to a problem can be constructed from optimal solutions to its subproblems, then the problem is likely a candidate for dynamic programming. This characteristic allows for effective use of solutions already computed to solve larger problems.
Identifying such patterns can make a world of difference in simplifying complex problems and steering clear of brute-force solutions.
Problem Categories
In dynamic programming, problems generally fall into distinct categories based on their nature and the approach required to solve them. Each category showcases specific characteristics, influencing how one might deploy dynamic programming principles.
Sequence Problems
Sequence problems often involve finding the longest, shortest, or most significant sequence from given data, such as strings or lists. The famous Longest Common Subsequence (LCS) problem is a typical example. This type of problem is beneficial for the overall goal of this article as it illustrates how dynamic programming can be used to tackle complex string manipulations efficiently.
Key Characteristic: The sequential nature makes it easier to visualize the solutions as they build upon previous results.
Unique Feature: These problems often feature a natural recursive structure, facilitating their transformation into dynamic programming problems.
Advantages: Utilizing dynamic programming leads to improved runtime from exponential to polynomial, which is substantial when dealing with longer sequences.
Optimization Problems
Optimization problems are tasked with finding the best solution from a set of feasible solutions, making them a fundamental aspect of dynamic programming. The classic Knapsack Problem exemplifies how dynamic programming can be applied to weigh various options and maximize value.
Key Characteristic: Solutions must be carefully computed as they can be influenced by a combination of variables or constraints.
Unique Feature: The use of a decision-making process to establish which elements to include in the solution can lead to varied outcomes depending on the approach.
Advantages: Dynamic programming allows for systematically building optimal solutions by addressing smaller decisions but can also increase complexity if there are numerous potential options to consider.
Partitioning Problems
Partitioning problems require dividing data into parts that meet certain criteria. The Subset Sum problem illustrates this partitioning, where the goal is to find a subset of numbers from a given set that adds up to a specific target.
Key Characteristic: The need to evaluate combinations of elements makes this category particularly tricky, as it may seem non-exhaustive.
Unique Feature: These problems often allow for clever schemes to reduce the search space by establishing strict criteria for valid partitions.
Advantages: Applying dynamic programming here streamlines the process, allowing candidates to practically manage often complex decision spaces, thereby enhancing efficiency.
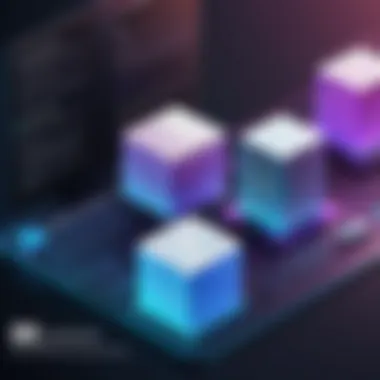
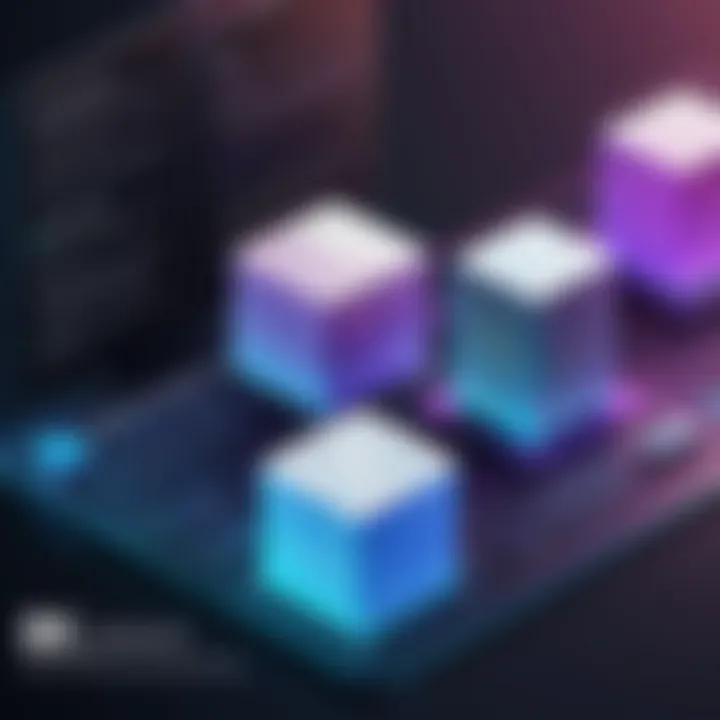
In summary, recognizing dynamic programming problems involves understanding key indicators, which lead to classifying them into appropriate categories, such as sequence problems, optimization problems, and partitioning problems. Mastering these elements will arm candidates with the insight needed to excel in dynamic programming and, subsequently, in technical interviews.
Strategies for Coding Interviews
In the realm of coding interviews, mastering dynamic programming is not just advantageous; itās often the key that unlocks the door to success. Employers highly value it due to its capacity to simplify problems that may seem overwhelmingly complicated at first glance. Candidates who adeptly navigate through dynamic programming challenges demonstrate not only technical proficiency, but also a structured approach to problem-solving that interviewers find appealing.
This section explores three main strategies that can help candidates turn their coding interviews into opportunities for displayin their skills and creativity.
Breakdown of the Problem
Before jumping into coding, itās vital to dissect the problem at hand. This means understanding the requirements and identifying parts that can potentially fit into dynamic programming frameworks. Hereās a simple approach to breaking down a problem:
- Read the problem multiple times: It may sound basic, but a thorough read can help catch nuances that can influence how you approach the solution.
- Identify input and output: Clearly define what you are given and whatās expected in return. This creates a foundation to build on.
- Spot patterns or subsequences: Often, dynamic programming problems involve repetitive calculations or paths. Identifying these early can save time later.
- Understand constraints: A clear understanding of constraints will steer decisions towards time efficiency and memory usage, both critical in interviews.
Effective problem breakdown encourages clarity and precision, allowing candidates to visualize the methods required without feeling overwhelmed.
Identifying Base Cases
Base cases are the backbone of any dynamic programming solution. Without them, the recursive formulas become meaningless, and confusion reigns. Base cases represent the simplest instances of a given problem. They act as the foundation upon which further computations evolve. Itās crucial to identify these early for effective recursion or iteration.
- Ask yourself: What is the smallest version of this problem? Often in sequence-related problems, you can find the base cases, like for the Fibonacci sequence, which is that and .
- Write them down explicitly: Having a clear reference of your base cases helps minimize errors during implementation.
- Test against edge cases: Consider special instances, such as empty inputs or extremely large numbers, to ensure your solution holds under various circumstances.
By taking encapsulated and straightforward base cases into account, the decision-making process during coding will flow more smoothly.
Formulating Recurrences
Once the pieces of the puzzle are neatly laid out before you, the next step is to formulate the recurrence relation. This is where your intuition and understanding of the problem should converge into a coherent function that describes how to derive your answer from previously solved subproblems.
- Analyze how one case relates to another: Think of it as building a pyramid; each new block builds on the previous layers. For instance, in the context of the Knapsack Problem, you frame recurrences around whether to include or exclude an item.
- Be precise in your language: Use clear variable naming to avoid confusion, whether it's , , or any custom term that reflects your logic.
- Write examples: Speak your thoughts aloud. Sometimes a quick example can clarify what your recurrence should look like.
Formulating the right recurrence relation directly affects the efficiency of the solution and the computational ceiling.
Understanding these strategies is essential to recognize and tackle dynamic programming cases head-on. They foster a methodical mindset that can dramatically bolster performance in coding interviews.
Example Problems
In the realm of dynamic programming, delving into example problems serves not just as a practical exercise but also as a crucial learning experience. These problems illuminate the core concepts and techniques of dynamic programming, helping learners recognize patterns and apply structured approaches to problem-solving. By dissecting actual coding interview questions, candidates can strengthen their understanding, gain confidence, and develop the skills needed to tackle complex challenges.
Fibonacci Sequence
The Fibonacci sequence is a classic example of dynamic programming that offers a straightforward illustration of both its principles and applications. The essence of this problem lies in its recursive nature, where each number is the sum of the two preceding ones. Though the sequence itself is simple, the challenge arises in calculating large Fibonacci numbers efficiently.
Using a naive recursive approach can lead to excessive computations, as the function repeatedly solves the same subproblems. This inefficiency can be easily avoided with dynamic programming techniques like memoization or tabulation. Hereās a brief code snippet highlighting both methods:
In interviews, presenting the Fibonacci sequence not only tests a candidateās understanding of recursion but also their grasp of optimization through dynamic programming. It leads to discussions about efficiency and performance optimization, essential skills for any coding interviewee.
Longest Common Subsequence
The Longest Common Subsequence (LCS) problem pushes candidates to think critically about string matching and optimal solutions. Given two sequences, the task is to identify the longest subsequence that appears in both. This problemās importance in computing arises because LCS has practical applications in DNA sequencing, file comparison, and version control systems.
Similar to the Fibonacci sequence, LCS has overlapping subproblems, making it a perfect candidate for dynamic programming. Candidates often recognize that the problem can be solved using a two-dimensional table, where each entry represents the length of the LCS for the corresponding prefixes of the two sequences. The recurrence relation typically looks like this:
- If the last characters of the sequences match, then:LCS(X[0..m-1], Y[0..n-1]) = 1 + LCS(X[0..m-2], Y[0..n-2])
- If they do not match, the table values are derived from the maximum LCS values by excluding one character:LCS(X[0..m-1], Y[0..n-1]) = max(LCS(X[0..m-2], Y[0..n-1]), LCS(X[0..m-1], Y[0..n-2]))
Understanding LCS can significantly bolster a candidateās problem-solving toolkit, enabling them to craft tailored solutions for related tasks in interviews.
Knapsack Problem
The Knapsack Problem is another fundamental dynamic programming example often showcased in coding interviews. It revolves around a common scenario where a thief must maximize the total value of items he can carry in a bag without exceeding its weight capacity. This problem quickly becomes complex as various combinations of items must be assessed, linking back to concepts of resource allocation and decision-making.
Two versions of the Knapsack Problem exist: the 0/1 knapsack, where each item can either be included or excluded, and the fractional knapsack, where items can be taken fractionally. The 0/1 version is particularly suitable for dynamic programming, demanding a table to store optimal solutions for every weight limit with respect to the items considered.
The recurrence relation for the 0/1 Knapsack might be visualized like this:
- If the weight of the current item is more than the knapsack's remaining capacity, ignore it:
K(i, w) = K(i - 1, w) - If the item can fit, calculate maximum value, deciding whether to include it or not:
K(i, w) = max(K(i - 1, w), K(i - 1, w - weight[i]) + value[i])
By mastering the Knapsack Problem, candidates are not only learning an algorithm but also the art of optimizing choices within constraints, a vital skill in technical interviews.
Example problems around dynamic programming help candidates internalize the methodologies required to excel in coding interviews. Attaining proficiency in these examples fosters a clear understanding of key concepts and their applications.
Common Mistakes in Dynamic Programming
Dynamic programming is a nuanced field within computer science that often trips candidates during coding interviews. Grasping its concepts is one thing, but mastering its applications can feel like a tightrope walk. Recognizing common mistakes is crucial in navigating this landscape effectively. By being aware of these pitfalls, candidates can refine their problem-solving skills and increase their chances for success. This section dissects two prevalent mistakes: ignoring base cases and misdefining recurrences. These errors can lead to incorrect solutions, wasted time, and frustration.
Ignoring Base Cases
Base cases are the bedrock of dynamic programming solutions. They represent the simplest instances of a problemāthose that can be solved directly without further subdivisions. Ignoring these cases can throw a wrench in your entire solution.
When tackling a dynamic programming problem, one must always begin by defining base cases clearly. For example, in computing the Fibonacci sequence, if you forget that the first two Fibonacci numbers are 0 and 1, your entire series could be skewed.
The significance of base cases cannot be overstated. They not only serve as starting points for building the solution but also help in preventing infinite loops during the recursion process. A poorly defined base case often results in erratic behavior of the algorithm, leading to timeouts and errorsāsomething you certainly want to avoid during an interview.
To sidestep this common blunder, it's advised to:
- Write down base cases before diving into the recurrent relationship.
- Test your algorithm with edge cases, as these often correlate to base cases.
- Ensure your base cases accommodate all possible inputs to avoid runtime surprises.
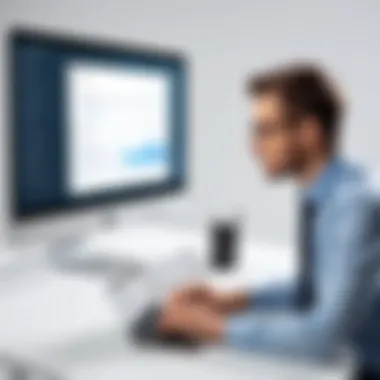
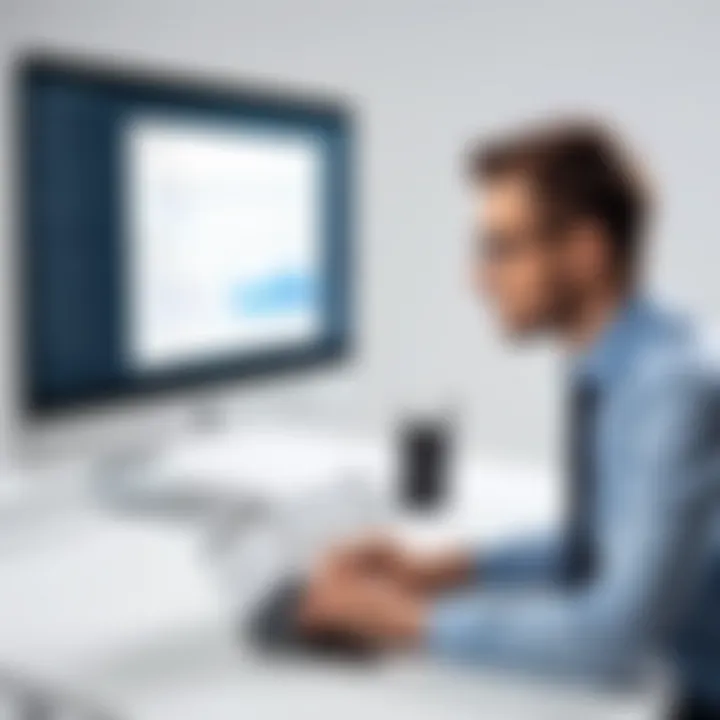
Misdefining Recurrences
Armed with a strong foundation, transitioning to the recurring relationships is the logical next step. But here's where many candidates big-time misstep. Misdefining recurrences can result in an inaccurate representation of the problem at hand, yielding incorrect outputs.
Recurrences are mathematical expressions that describe how the solution can be constructed from solutions of smaller subproblems. If these expressions are flawed, the entire architecture of your solution becomes shaky. A common scenario arises when candidates skimp on examining how to break the problem down accurately. For example, in the classic Knapsack problem, failing to account for either including or excluding an item correctly can skew the result completely.
Consider the following suggestions to ensure that your recurrences are correctly defined:
- Review similar solved problems to gain intuition on constructing recurrences.
- Verify the logical flow of your narrative. Each decision in your recurrence should clearly explain how it leads to the total solution.
- Create a simpler version of the problem to grasp the correct recurrence relationship before tackling the full version.
"In dynamic programming, clarity in defining both base cases and recurrences sets the stage for a successful solution."
By paying close attention to these aspects, candidates can navigate the tricky waters of dynamic programming problems more effectively. The objective is to not only finish in time but also to arrive at sound solutionsāsomething every interviewer looks for.
Tips for Practicing Dynamic Programming
Practicing dynamic programming is quite important for anyone gearing up for coding interviews. Not only does it enhance oneās problem-solving skills, but it also builds a solid foundation for approaching some tricky questions that appear frequently in interviews. Understanding this topic can help you glide through some of the most complicated algorithmic challenges thrown at candidates.
Effective Practice Platforms
When diving into dynamic programming, choosing the right practice platforms can really make a difference. Here are some worthwhile options to consider:
- LeetCode: This site is famous for its vast array of coding problems, including a dedicated section for dynamic programming. It allows you to filter problems by difficulty and topics, so finding the right fit is a breeze.
- HackerRank: Another good platform known for coding challenges. They offer specific challenges that require dynamic programming techniques, helping reinforce your skills through practice.
- CodeSignal: It provides a unique twist with their arcade section, where you can solve problems to progress through levels, including many that touch on dynamic programming.
- GeeksforGeeks: Not only does this site deliver practice problems, but it also provides detailed explanations and articles that cover dynamic programming concepts in depth.
These platforms not only let you practice but often offer community discussions where you can glean insights from othersā approaches to the same problem. This feedback loop can deepen your understanding.
Establishing a Study Routine
Creating a study routine tailored specifically for dynamic programming can boost not only your skills but also your confidence. Hereās how to do it right:
- Set Clear Goals: Decide how many problems you wish to solve each week. Having specific targets helps keep motivation high.
- Divide and Conquer: Break down complex problems into simpler components. Spend time understanding the core concepts behind the problems, especially the nuances of memoization and tabulation.
- Regular Revision: Make it a point to regularly engage with previously solved problems to solidify your understanding. Going back will help you see how your thought process has progressed.
- Time Yourself: When practicing, occasionally set a time limit for solving problems. This mimics the pressure of a real coding interview and helps you work on your speed.
- Peer Programming: Team up with friends or fellow learners. Discussing your thought process can reveal new strategies and approaches, increasing your flair for problem-solving.
By keeping your practice regular and structured, youāll notice improvement in your ability to tackle dynamic programming problems over time.
"Practice is the best of all instructors." - Publilius Syrus
Staying diligent is key. With the right platforms and a solid routine, youāll carve out your path to mastering dynamic programming in no time.
Resources for Deeper Understanding
Understanding dynamic programming deeply requires more than just reading a few articles or tutorials. A well-rounded grasp of the subject combines theory with practice, and that's where various resources come into play. Engaging with these resources can demystify concepts, provide hands-on experience, and enhance problem-solving abilities. When it comes to dynamic programming, the journey can be challenging, but having the right tools can make a world of difference.
In coding interviews, it's not just about knowing the theory; itās about applying it effectively. Here's where books, online courses, and coding challenges become invaluable. They offer structured learning paths and opportunities for practice that cater to diverse learning styles. Going through these resources helps solidify your skills, making you more confident and prepared for technical interviews.
Books
Books provide a comprehensive insight into dynamic programming, often setting a strong foundational knowledge. Here are some noteworthy recommendations:
- "Introduction to Algorithms" by Thomas H. Cormen et al.: This book dives into various algorithms including dynamic programming. It's a bit dense but offers a treasure trove of information and problem breakdowns.
- "Elements of Programming Interviews" by Adnan Aziz, Tsung-Hsien Lee, and Amit Prakash: This book is enriched with examples that walk through the thought process behind dynamic programming solutions.
Books like these transform abstract concepts into relatable problems, making it easier to grasp the intricacies involved.
Online Courses
With the boom of e-learning platforms, numerous online courses have emerged that teach dynamic programming in practical ways. Here are some popular courses:
- Coursera - "Data Structures and Algorithm Specialization" by University of California, San Diego: This series covers dynamic programming concepts and includes coding assignments that challenge you to apply what you've learned.
- Udemy - "Mastering Data Structures & Algorithms using C and C++": This course addresses dynamic programming with various practical scenarios, enhancing both understanding and coding skills.
- edX - "Algorithm Design and Analysis" by University of Pennsylvania: Engage with a curriculum that balances theoretical knowledge and practical applications in dynamic programming.
Courses like these often come with interactive components, allowing learners to get immediate feedback, which is essential for mastering the concepts.
Coding Challenges
Practicing through coding challenges can't be emphasized enough when mastering dynamic programming. Various platforms provide extensive collections of coding problems tailored to help sharpen your skills:
- LeetCode: Known for its array of coding problems, LeetCode has a specific section dedicated to dynamic programming challenges. These can help you gain practical experience and familiarize you with interview formats.
- HackerRank: Offers contests and practice problems focusing on dynamic programming, making it a fantastic platform for honing your coding craft.
- CodeSignal: Another valuable resource, it provides a variety of dynamic programming challenges along with a scoring system that tracks your progress.
Start tackling problems from these platforms on a regular basis. Even just a few problems each week can make a marked difference in knowledge retention and confidence.
Embracing these resources will not only boost your understanding but also prepare you for real-world challenges and coding interviews.
As you explore these books, courses, and practice problems, remember that consistency is key. A balanced approach combining these elements will ultimately lead you to expertise in dynamic programming, paving the way for success in coding interviews.
Final Thoughts on Dynamic Programming
Dynamic programming marks a cornerstone of algorithmic thinking, particularly relevant in the realm of coding interviews. Its ability to transform complicated problems into manageable, step-by-step solutions is nothing short of vital. Building a strong foundation in dynamic programming allows aspiring developers to approach challenges not just with memorized solutions but with a methodical mindset. This thought process dramatically influences performance during technical interviews, where clarity and efficiency are paramount.
Long-Term Benefits
Engaging deeply with dynamic programming yields not only immediate results but also long-term advantages. Here are some notable benefits:
- Enhanced Problem-Solving Skills: By mastering this technique, candidates learn to dissect complex problems into simpler ones ā a skill that comes into play beyond interviews.
- Increased Job Opportunities: Employers hold candidates with a strong grasp of dynamic programming in high regard. This is due to the technique's relevance in various technical positions involving algorithm design and optimization.
- Foundation for Advanced Concepts: Once you understand dynamic programming, grasping other advanced topics like graph algorithms and optimization strategies becomes less daunting.
"Dynamic programming is like eating an elephant; you can only tackle it one bite at a time."
These elements combine to create a robust skill set that extends far beyond coding interviews.
Navigating the Learning Curve
The journey to mastering dynamic programming can seem overwhelming. However, breaking the process down simplifies it considerably. Here are several strategies that may aid newcomers:
- Start Small: Begin with basic problems before venturing into more complex scenarios. Start with the Fibonacci sequence or simple combinatorial problems.
- Visualize the Process: Diagramming your thoughts can often clarify the relationships between various components within a problem. Crafting a tree or table can help illuminate how subproblems relate.
- Practice Regularly: Consistency is key. Allocate time each week to solve dynamic programming problems. Websites like LeetCode and Codewars offer tailored practice.
- Learn from Mistakes: Examining errors and misjudgments during problem-solving helps refine your understanding. Keeping a journal of problem-solving attempts may also highlight useful patterns or approaches over time.
In summary, although the path to proficiency in dynamic programming may be fraught with challenges, the rewards are profound. A well-thought-out approach can make the learning curve less steep and open doors to a world of opportunities.