Mastering Debugging: Techniques and Tools for Coders
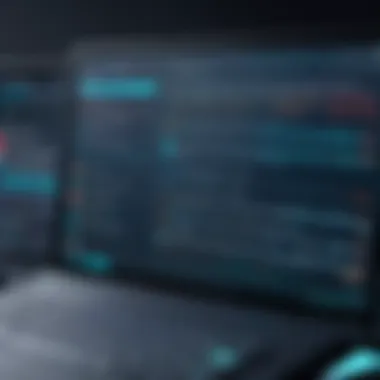
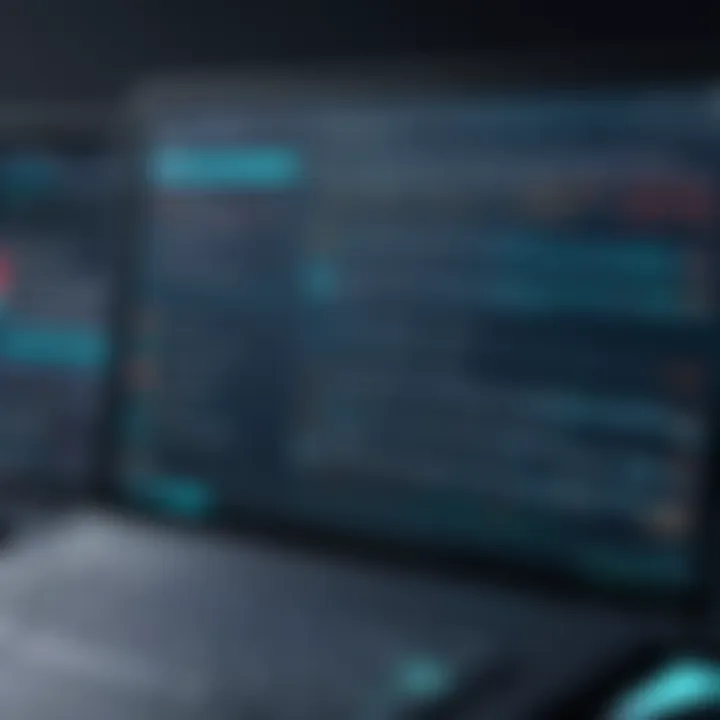
Preamble to Programming Language
Programming languages form the backbone of software development, enabling programmers to convey instructions to a computer efficiently. The journey of computer programming is as old as the very notion of computers themselves. With roots tracing back to early mechanical calculators and leading into the digital age, the evolution of these languages has paved the way for modern software solutions.
History and Background
The first program, created by Ada Lovelace for Charles Babbage’s Analytical Engine, laid the foundation for what would eventually grow into a multitude of languages. Over the decades, languages such as Fortran, COBOL, and C emerged, each tailored for specific tasks. The advent of the internet brought forth new languages such as JavaScript, C#, and Python, each designed to address the needs of a rapidly changing tech landscape.
Features and Uses
Notably, programming languages vary widely in their features. High-level languages like Python and Ruby emphasize readability and simplicity, making them ideal for beginners. In contrast, low-level languages like C and assembly offer finer control over hardware, suited for performance-critical applications.
Each language serves unique purposes:
- Python: Widely used for web development, data analysis, and machine learning.
- Java: Known for its portability and is frequently employed in enterprise applications.
- JavaScript: The language of the web, critical for interactive web pages.
- C++: A favorite in game development and system programming, ensuring speed and efficiency.
Popularity and Scope
In today’s programming landscape, it’s crucial to consider the popularity of different languages. According to surveys, languages like JavaScript, Python, and Java continuously top the lists. Such popularity often reflects a language's community support, libraries available, and the scope of projects that can be undertaken using it.
Understanding these languages provides a valuable background as we delve into the debugging process.
"Debugging is like being a detective in a crime movie where you are also the murderer."
As a budding programmer, one will encounter challenges while coding. Understand that debugging is an essential skill in diagnosing and rectifying these errors.
Up next, we will break down the basic syntax and core concepts that form the foundation of programming languages. Understanding these will significantly aid in efficient debugging practices.
Understanding Debugging
Debugging is a cornerstone in the world of programming. It’s not merely about fixing errors but understanding the intricate web of logic within one’s code. At its core, debugging serves as the bridge between intention and implementation. Mistakes aren't just nuisances; they are golden opportunities to learn and refine one's skills.
This segment shines a light on why comprehending debugging is crucial for anyone venturing into programming. Grasping how to debug effectively can lead to more stable software, ultimately fostering better applications that stand the test of time.
Definition and Importance
So, what is debugging? Fundamentally, debugging means identifying and correcting errors in code. It's much like being a detective trying to crack a case; there’s a mystery to solve. When a developer writes code, they often expect it to behave in a certain way. When it doesn't, the onus is on them to dive right in and figure out where the wheels fell off the wagon.
Debugging is not just a buzzword; it’s a critical process for several reasons:
- Efficiency: Debugging directly impacts the speed of development. If a bug festers undetected, it could lead to larger, systemic issues down the line.
- Quality: A meticulous debugging process will ensure a higher quality of code, which translates to better software performance.
- Learning: Each encounter with a bug is a lesson. Understanding the source of an error enhances knowledge and prevents similar mistakes in the future.
- Collaboration: Knowledge of debugging fosters better teamwork, as developers can communicate more effectively about problems and solutions.
What’s important to remember is that debugging isn't a one-size-fits-all approach. Recognizing this can help demystify the process and encourage programmers, especially learners, to adopt an iterative mindset when handling their errors.
Common Errors in Programming
As any seasoned programmer will tell you, errors are as common as rain in April. To better understand debugging, it's beneficial to familiarize oneself with the types of errors often encountered. Here are the main culprits:
- Syntax Errors: These are typos or incorrect language usage that prevent the code from running at all. Think of it like spelling mistakes; the message just doesn't get across.
- Runtime Errors: These occur while the program is executing, often due to unforeseen circumstances, like trying to divide by zero. It’s the point where expectations flip on their head, and you’re left puzzled.
- Logical Errors: The code runs without a hitch, but it doesn’t produce the expected results. This is where understanding the intent behind the code becomes vital.
- Off-by-One Errors: A classic blunder, often encountered in loop constructs or array indexing. Just one misplaced number and it’s like a domino effect.
Each type of error can lead you down a rabbit hole of investigation, revealing not just the problem but offering insights into coding practices and principles that are critical for programming success.
"Debugging is like being the detective in a crime movie where you are also the murderer."
— Anonymous
With these definitions and common pitfalls in mind, developers can approach debugging with a clearer perspective, seeing it not as an obstacle but as part of the journey in programming.
The Debugging Process
Debugging is a critical phase in software development. It’s where you sift through complex code to locate the bugs that cause unexpected behaviors. Understanding the debugging process not only helps in fixing issues but also improves the quality of your software. It’s like being a detective; you gather clues, construct theories, and work towards solving a mystery that impacts the user experience.
In this section, we will explore the various aspects of the debugging process, with an emphasis on the steps you can take, how to formulate hypotheses about potential errors, and verifying your findings effectively. By mastering these elements, you will develop a more solid foundation in programming, leading to stronger and more maintainable code.
Step-by-Step Debugging Methodology
The foundation of an effective debugging process often lies in a systematic approach. This can be broken down into several steps:
- Identify the issue: First and foremost, you need to know what’s going wrong. Often, it’s just a matter of a program not behaving as expected. During this phase, detailed error messages, logs, or user reports provide the initial context.
- Reproduce the problem: This is where you want to replicate the circumstances that lead to the bug. Can you follow the same steps to recreate it? If it’s an intermittent issue, it could take some patience.
- Isolate the cause: Once the bug is reproducible, start narrowing down potential culprits. This might involve temporarily disabling certain features or testing components in isolation.
- Implement a fix: After pinpointing the troublesome code, you can implement the necessary changes. This is typically a critical moment because it’s easy to strangle a bug in its cradle but risk introducing new ones in the process.
- Test your solution: After applying your fix, you should rigorously test to confirm that it indeed solves the problem without breaking anything else.
- Document your findings: Lastly, record what you’ve learned. This might seem tedious, but in the long run, having insights documented will save you time in the future.
Formulating Hypotheses
When debugging, crafting hypotheses is akin to making educated guesses. You aim to theorize what might be causing the problems based on the symptoms observed. A few tips for effective hypothesis formulation include:
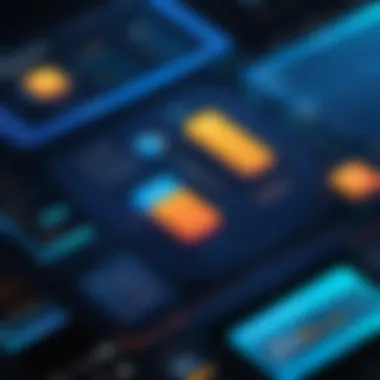
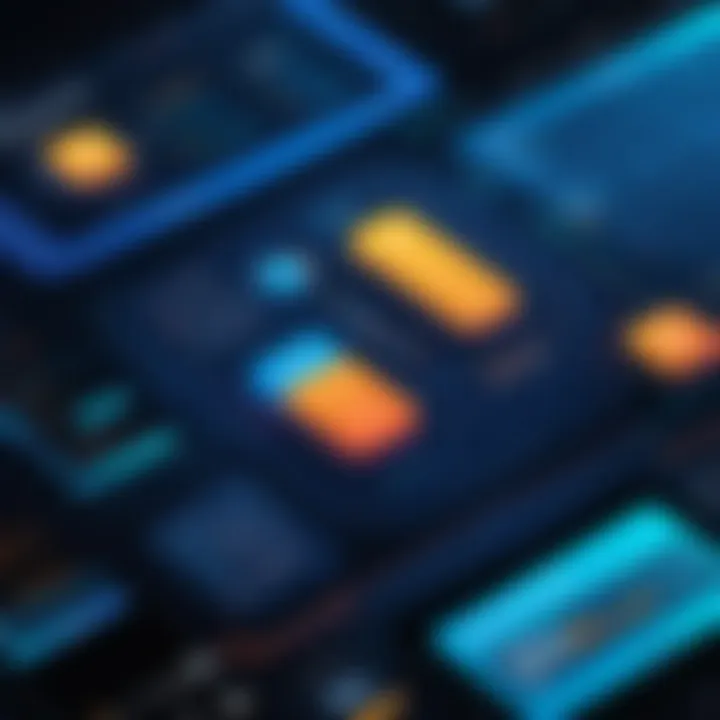
- Analyze error messages: Error messages can provide powerful clues. They often indicate the location and nature of the error.
- Examine recent changes: If a program starts acting strange, consider what changed recently. Was there an update to a library, or perhaps some new code was added?
- Use debugging aids: Tools that help log outputs or monitor variables during execution can assist you in making informed guesses.
By approaching debugging with well-articulated hypotheses, you raise the chances of solving the issue quicker and more efficiently.
Verification of Results
After adjustments are made to the code, it’s paramount to verify that the bug has been successfully squashed. Verification involves various strategies:
- Regression Testing: This method tests the functions that might have been impacted by the fix. Ensure sound functionality across the board.
- Peer Review: Sometimes a second pair of eyes can spot something you may have missed. Engaging with fellow programmers allows for collaborative problem solving.
- Use of Automated Testing: Implementing unit testing frameworks can automate the validation of code, verifying that changes don’t introduce new bugs.
Verification is not just checking; it’s a continual process that ensures software quality.
Types of Debugging Techniques
When delving into the world of programming, understanding various debugging techniques is akin to having a trusty toolbox at your disposal. Each technique serves a distinct purpose, especially when faced with the inevitable errors that crop up in complex code. Using the right method can not only expedite the problem-solving process but also enhance programmers’ overall efficiency. Let’s take a closer look at three primary approaches: static vs. dynamic debugging, manual debugging techniques, and automated debugging approaches.
Static vs. Dynamic Debugging
In the realm of debugging, static and dynamic approaches present two sides of the same coin. Static debugging refers to the analysis of code without executing it. This technique is particularly useful during the early stages of development when the programmer can catch errors through careful inspection of the source code. Tools such as linters can provide real-time feedback on potential issues, helping to keep the code organized and efficient.
On the other hand, dynamic debugging operates on a different wavelength. Here, the code is executed in a controlled environment to observe the behavior of the program during its run-time. This approach can bring to light issues that may not be apparent through static analysis, like memory leaks or unexpected crashes due to specific input scenarios. Using debuggers, programmers can set breakpoints and step through the code line by line, gaining insights into the program’s execution flow.
This duality of static and dynamic techniques underscores the necessity for a comprehensive debugging strategy. While one provides a preemptive view, the other offers a real-time perspective, making them both indispensable in the debugging arsenal.
Manual Debugging Techniques
Manual debugging techniques require a hands-on approach by the programmer. This method is often favored by those who like to maintain close control over their debugging process. Here, the programmer goes through the code line by line, examining variables and program flow to pinpoint problematic areas. Some common techniques include:
- Print Statements: Insertion of print statements at strategic points in the code can help trace variable values and execution paths. It’s simple but effective.
- Code Reviews: Collaboration with peers through code reviews can also shine a light on unnoticed errors. Fresh eyes can spot logical flaws or oversights that one might overlook.
- Rubber Duck Debugging: Explaining the code to an inanimate object or a colleague can lead to new perspectives and understanding. It’s about verbalizing thought processes that might clarify complex ideas.
While manual debugging might seem time-consuming, it fosters a deep understanding of the code’s inner workings. Programmers often describe this technique as a learning experience, enhancing their ability to write better code in the future.
Automated Debugging Approaches
With advancements in technology, automated debugging methods have gained traction, much to the delight of many in the programming community. Automated tools can catch errors in real-time and often serve as a safety net during the development process. These tools can vary:
- Static Analysis Tools: Programs like SonarQube and ESLint analyze code without executing it and can detect issues ranging from stylistic choices to more critical bugs. These tools help maintain code quality and enforce best practices.
- Automated Testing Frameworks: Utilizing frameworks such as JUnit (for Java) or Pytest (for Python), developers can automate tests to ensure that their code functions as intended across various scenarios. These tests can be run frequently, providing immediate feedback on code changes.
- Continuous Integration/Continuous Deployment (CI/CD) Tools: Employing CI/CD pipelines automates the deployment and testing processes, catching bugs earlier in the life cycle than traditional methods would.
Embracing automated approaches can streamline the debugging process significantly, allowing developers to focus their energies on more complex problem-solving tasks. As the saying goes, "work smarter, not harder."
The balance between manual and automated debugging is key. Each has its place, and effectively combining them can create a robust debugging strategy that capitalizes on the strengths of both approaches.
Debugging Tools and Environments
The realm of debugging is as much about the tools and environments we engage with as it is about our methodologies. When considering error resolution in programming, thoughtful selection of debugging tools can provide significant advantages. These tools not only enhance efficiency but also cater to the specific needs of different programming languages and environments. Understanding these tools is crucial; it’s the kind of knowledge that sets apart an average developer from an exceptional one.
Integrated Development Environments (IDEs)
Integrated Development Environments, often called IDEs, are at the forefront of modern software development. These applications combine multiple developer tools into one cohesive environment, allowing programmers to write, test, and debug code seamlessly. Popular examples of integrated development environments include Visual Studio, Eclipse, and JetBrains' IntelliJ IDEA.
With features like syntax highlighting, code suggestions, and built-in debuggers, IDEs drastically reduce the cognitive load during coding sessions. They allow live debugging, which means developers can set breakpoints and inspect variables as the program runs. This real-time insight often leads to quicker identification of issues.
"An effective IDE is akin to having a trusty sidekick; it highlights what you miss and makes the journey smoother."
Moreover, the community surrounding popular IDEs often contributes plugins that further enhance debugging capabilities, adapting the environment to users’ specific needs. However, some may argue that the extensive features can be overwhelming for beginners. Learning to navigate an IDE is a skill worth developing, as it significantly boosts productivity.
Standalone Debuggers
Standalone debuggers, on the other hand, offer a focused approach to error resolution. Tools like GDB for C/C++ or the Python Debugger (pdb) are designed specifically for debugging tasks. These tools often provide advanced features tailored for hunting down elusive bugs. For instance, GDB allows users to inspect the program's memory, variable values, and even control the execution flow.
A standalone debugger can be less intimidating than an IDE for developers who prefer simplicity. The command-line interface found in tools like GDB requires a different skill set but allows for a deep dive into the mechanics of the code without the distraction of a graphical user interface.
Using a standalone debugger often enhances understanding of the code being worked with. It forces one to engage more actively with the program's behavior, making it a great choice for learners who seek to comprehend the fundamentals rather than rely solely on automated tools.
Version Control Systems
Version Control Systems (VCS) play a pivotal role in debugging, especially when working within teams or on larger projects. Tools like Git offer more than just version tracking. They enable developers to create branches, experiment safely, and revert to earlier states of the code with ease.
Incorporating a VCS into your debugging workflow allows one to isolate changes that introduced bugs. For instance, if a new feature caused the program to crash, one can quickly examine the changes made in that specific commit. This granularity is invaluable and significantly reduces the time spent on sifting through lines of code to identify the problem.
Additionally, using a VCS facilitates collaboration among team members. When multiple developers are working concurrently, merging changes becomes vital. It's common that bugs are introduced through merge conflicts, which can be resolved efficiently with the help of version control tools.
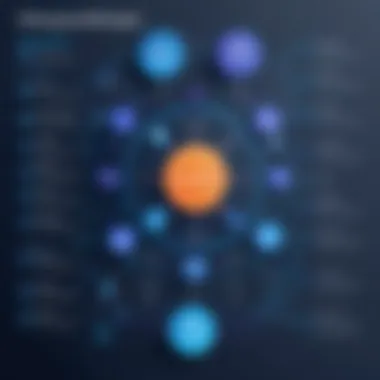
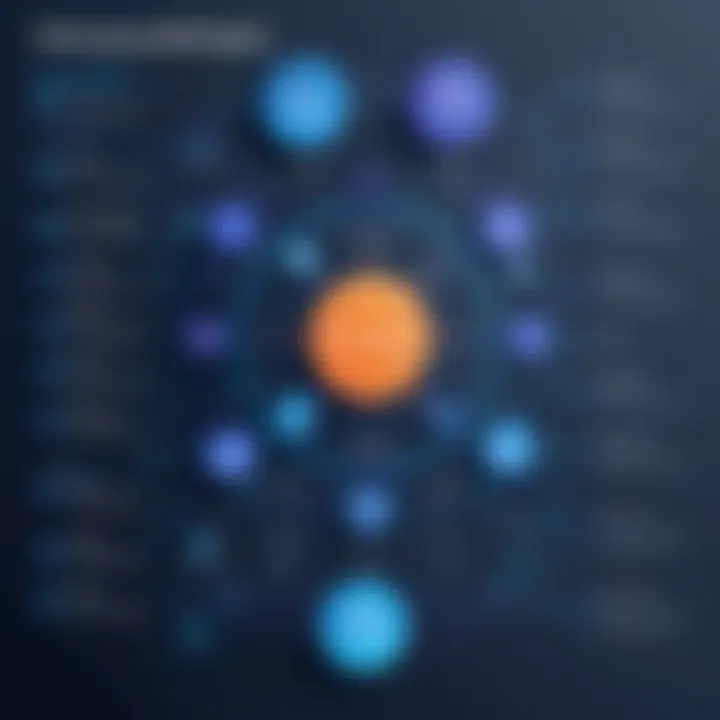
Epilogue
In summary, understanding the tools and environments available for debugging is integral to mastering the art of programming. Whether leaning towards integrated environments, standalone tools, or version control systems, each offers unique benefits that can streamline the debugging process. As one explores the nuances of these tools, the journey becomes less about simply fixing bugs and more about enhancing the code quality and development process.
Debugging in Various Programming Languages
Debugging is a fundamental skill for programmers, regardless of the language they use. Each programming language has its own unique characteristics, syntax, and potential pitfalls that can lead to errors. Understanding how to debug effectively in these environments is crucial for both novice and experienced coders alike. This section delves into the nuances of debugging in key programming languages: Java, C, C++, and Python. By examining the specific challenges and solutions that arise in each of these languages, one can enhance their debugging capabilities and ultimately improve the software development process.
Debugging in Java
Java developers are often faced with a robust set of tools and features that can streamline the debugging process. One of the core advantages of Java is its exception handling framework, which provides a clear way to manage errors. When an error occurs, an exception is thrown—this can be caught using blocks. This immediate feedback helps isolate issues.
Debugging Tips for Java:
- Use the debugger: Integrated Development Environments like Eclipse or IntelliJ IDEA come with powerful debugging tools. Step through your code line by line to observe the flow and inspect variables.
- Log messages: Utilize the logging framework to capture important information at runtime. A well-placed log statement can clarify where an error surfaces.
- Unit tests: Java's JUnit framework allows for writing tests to ensure code correctness before deploying to production. This proactive approach reduces the chance for bugs to arise.
"In Java, catching exceptions isn’t just a safety net; it’s part of the art of coding. A well-crafted exception handling mechanism makes your software resilient."
Debugging in
Debugging in C can often feel like walking a tightrope, given the direct manipulation of memory. Errors such as segmentation faults or buffer overflows can arise unexpectedly, making a debugger an important ally in this environment. Particularly, tools like (GNU Debugger) are invaluable for stepping through code and examining memory.
Key Considerations for Debugging in C:
- Pointer mismanagement: Always double-check your pointer declarations. A simple dereferencing of a null pointer can bring your entire application down.
- Memory leaks: Utilize tools like Valgrind to detect improper memory allocations and ensure that memory is managed properly.
- Compile with warnings: Use flags like with gcc to enable all important warnings. This can catch potential problems early in development.
Debugging in ++
C++ inherits all of C's challenges and adds complexity with its object-oriented features. The presence of classes, inheritance, and polymorphism introduces new types of errors. Understanding how to navigate these challenges means knowing your tools well. Both Visual Studio and CLion offer comprehensive debugging functionalities tailored for C++.
Effective Debugging Tips in C++:
- Understand constructors and destructors: Be aware that improper use can lead to resource mismanagement. Debugging memory leaks often falls back to these functions.
- Smart pointers: When utilizing C++11, favor smart pointers over raw pointers to automate memory management and reduce the complexity of debugging as they prevent many common errors.
- Static analysis tools: Tools like CPPCheck can provide insights into potential bugs before runtime, catching things at compile time instead.
Debugging in Python
Python's simplicity is part of its charm, yet debugging can still prove challenging, especially when working with dynamic types. Python’s built-in debugger, , is user-friendly and can step through scripts interactively. Moreover, Python's clear error messages direct developers effectively to the source of problems.
Best Practices for Python Debugging:
- Leverage Exception Handling: Use blocks to manage the unexpected behavior gracefully, avoiding crashes and providing clear feedback on what went wrong.
- Debugging with IDEs: Many IDEs like PyCharm offer graphical debugging tools that simplify stepping through code and inspecting variables.
- Test-Driven Development: Encouraging a TDD approach can minimize bugs at the outset. Write tests before the code to ensure it meets defined requirements.
Ultimately, debugging across different programming languages requires adapting skills to the specifics of each language. Whether it's navigating pointer arithmetic in C or managing class hierarchies in C++, mastering debugging methods is essential to a programmer’s toolkit. Understanding and applying these principles can significantly enhance the development workflow and lead to cleaner, more efficient code.
Best Practices for Effective Debugging
Effective debugging is a crucial skill in programming that significantly impacts the quality of software solutions. By employing best practices, developers can streamline their debugging process, enhance accuracy in identifying errors, and ultimately produce more reliable code. Here, we will explore key elements such as code documentation, refactoring strategies, and collaboration techniques that embody effective debugging practices.
Code Documentation
Keeping detailed code documentation plays a foundational role in debugging. It serves as a road map for you and your team, outlining the thought process and the rationale behind specific coding decisions. Good documentation can make it easier to grasp the overall flow of the project, facilitate onboarding new team members, and shorten the time needed to resolve issues.
For example, consider documenting not just what a function does but also how inputs are processed and what output to expect under different conditions. This information can be invaluable when something goes awry. It avoids the 'guessing game' and provides context, which is particularly helpful when diving back into a project after some time.
- Benefits of Code Documentation
- Reduces the time spent understanding existing code
- Eases collaboration among team members
- Serves as a reference for future debugging efforts
One practical approach is to use comments judiciously, which means not overloading your code with unnecessary notes, but ensuring that critical parts are well explained. Additionally, consider supplementary documentation like UML diagrams or flowcharts for complex logic.
Code Refactoring Strategies
The concept of code refactoring can sometimes sound overly technical, yet it's a friend in disguise for effective debugging. It involves revisiting and improving the inner workings of existing code without altering its external behavior. This can reveal hidden bugs and enhance code readability.
Refactoring can be as simple as breaking down large functions into smaller ones. This makes it easier to pinpoint where an issue might be lurking. Refactoring is not just a one-time activity; it should be an ongoing process throughout the software development life cycle.
Here are effective strategies for refactoring:
- Modularizing Code: Create smaller, reusable components.
- Simplifying Logic: Aim for clarity over complexity.
- Removing Redundancies: Avoid duplicate code, which can lead to multiple points of failure.
By adhering to these strategies, you can refine your code, making it easier to navigate and debug.
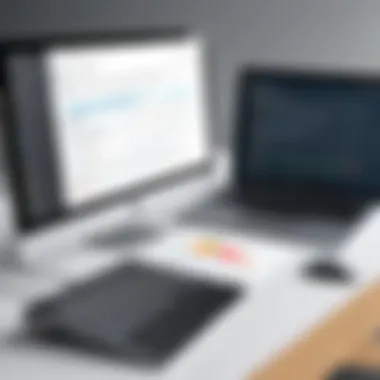
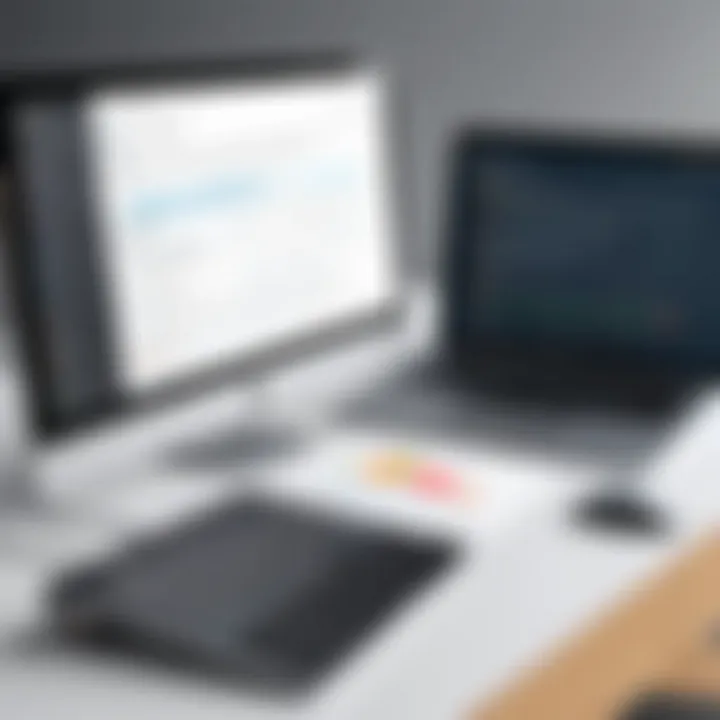
Collaborative Debugging Techniques
In the world of software development, twining forces can mean the difference between finding solutions quickly or being stuck in a rut. Collaborative debugging techniques leverage the collective intelligence of the team. Engaging with your peers when tackling particularly gnarly bugs can lead to insights that one individual might overlook.
Pair programming is one effective approach where two developers work together at the same workstation. This allows for immediate feedback, fostering a dynamic environment where problems can be addressed on the fly. Another often overlooked technique is conducting code reviews regularly. These reviews help catch potential issues before they escalate into major debugging sessions.
Moreover, platforms such as Reddit's programming community provide a space to seek help from fellow coders, offering multiple perspectives on debugging issues that may arise.
"Alone we can do so little; together we can do so much." – Helen Keller
Challenges in Debugging
Debugging is more than just a routine process; it is a crucial aspect of coding that determines how effectively a program can run. While each programmer might look at code with unique lenses, the reality is that debugging offers hurdles that can sometimes feel like climbing a mountain. Whether the programmer is a beginner or has years of experience under their belt, these challenges can be daunting. Understanding these issues is vital, as they influence strategy and approach, ultimately impacting productivity and code quality.
Complexity of Code
With the modernization of programming languages and the increasing use of libraries and frameworks, the complexity of code is at an all-time high. Codebases are no longer confined to a few hundred lines of instructions; they can stretch into the tens of thousands. This complexity can lead to several situations:
- Interdependent components: One change in code can ripple through other modules, causing unforeseen errors.
- Margin for error: As complexity increases, so does the probability of bugs slipping through unnoticed.
- Difficulty in tracing errors: When something malfunctions, pinpointing the issue becomes a Herculean task. Developers may have to sift through layers of code to identify the root cause.
When dealing with complex codes, it's essential to implement structured approaches to facilitate navigation. Tools like static code analyzers can assist in reviewing the code without executing it, catching issues before they can cause significant problems.
"In a tangled web of code, clarity can seem like a distant relative. It's vital to have strategies in place that give your mind a clear pathway through the confusion."
Dealing with Legacy Code
Legacy code, by its very definition, can be a double-edged sword. On one hand, it is often an old but functional system that is invaluable for an organization. On the other, it can present a host of challenges for debugging. When programmers step into legacy systems, they encounter several roadblocks:
- Lack of documentation: Many legacy systems do not come with adequate documentation, leaving programmers to guess at the intentions behind certain lines of code.
- Obsolete technology: Older programming languages and frameworks may not even function on modern machines, requiring additional effort to emulate or adapt.
- Resistance to change: Often, businesses hesitate to update or refactor legacy code due to the perceived risks involved. Fear of new bugs or failure can inhibit necessary updates.
Strategies to deal with legacy code can include gradual refactoring and establishing clear documentation that captures the essence of the existing code for future work. Engaging in peer code reviews can also shed light on potential issues and improve collective understanding of older systems.
Utilizing these techniques not only mitigates risks but can also often yield improvements to performance and efficiency, turning a daunting challenge into a fruitful endeavor.
Future Trends in Debugging
Debugging has always been a critical component of software development, but its landscape is constantly evolving. By keeping pace with emerging technologies, developers can enhance their debugging processes and improve their overall productivity. The future trends in debugging, particularly the integration of advanced tools and collaborative approaches, are important as they propose solutions to persistent challenges. These developments strive to optimize the error-fixing process while ensuring that the software remains reliable and efficient.
Integration of AI in Debugging
Artificial Intelligence (AI) is proliferating across various sectors, and its integration into debugging is a trend that cannot be overlooked. AI-powered debugging tools can analyze vast amounts of code much quicker than a human developer. These tools utilize machine learning algorithms to identify patterns and predict common errors, which can help in spotting bugs even before they arise.
Some key advantages of AI in debugging include:
- Enhanced Efficiency: By automating repetitive tasks, developers can focus more on complex problems, allowing for higher productivity.
- Predictive Error Detection: AI can learn from historical data to pinpoint potential issues before they become severe, which can drastically reduce downtime.
- Adaptability: With machine learning capabilities, these tools can improve over time, enabling continuous learning and innovation in error detection practices.
As an example, tools like DeepCode and Snyk are already making waves by leveraging AI for identifying vulnerabilities, thus changing the debugging narrative forever.
Real-time Collaboration Tools
The demand for effective teamwork in software development has led to the rise of real-time collaboration tools. These tools empower teams to work together seamlessly, regardless of their physical locations. Instant communication and shared coding environments can significantly reduce the time it takes to address bugs.
Benefits of employing real-time collaboration tools include:
- Immediate Feedback: Team members can receive instant input on their code changes, allowing for quicker iterations.
- Enhanced Problem Solving: Multiple perspectives can converge on solving a problem, facilitating creative solutions that might not have arisen in isolation.
- Shared Understanding: A collaborative approach fosters a collective understanding of the code base among team members, which can minimize misunderstandings and errors in the future.
Popular tools like GitHub and Slack have integrated features that support real-time collaboration, making effective debugging a team effort.
"The future of debugging lies in harnessing technology and collaboration, making it easier and faster to deliver high-quality software."
Closure
Debugging stands as a cornerstone in the realm of software development, bridging the gap between mere code and fully functional applications. As we wrap up this comprehensive guide, it's clear that acknowledging the importance of debugging is not just academic; it impacts every aspect of programming, from efficiency to user satisfaction.
Summation of Key Points
In our journey through the intricate landscape of debugging, we've touched upon several key aspects:
- Understanding Debugging: We laid the groundwork by defining debugging, illustrating its significance in the software lifecycle and identifying common programming errors that often trip developers up.
- The Debugging Process: We examined a systematic approach to debugging, encompassing step-by-step methodology, formulating hypotheses to address issues, and verifying results to ensure problems are adequately resolved.
- Types of Debugging Techniques: Different methods of debugging, including static and dynamic approaches, manual versus automated techniques, highlight the diversity of tools and strategies available to developers.
- Debugging Tools and Environments: Discussion about Integrated Development Environments, standalone debuggers, and version control systems showcased various platforms that aid in the debugging process.
- Debugging Across Languages: Each programming language has its quirks; understanding how debugging applies in Java, C, C++, and Python allows developers to tailor their strategies effectively.
- Best Practices for Effective Debugging: Emphasis on code documentation, refactoring strategies, and collaborative debugging reinforced the idea that effective debugging goes beyond mere technical skills.
- Challenges in Debugging: We highlighted the complexities involved in modern software development, particularly with legacy code and the increasing intricacy of codebases.
- Future Trends: We looked ahead to how AI and real-time collaboration tools are poised to reshape debugging practices, ensuring that developers can keep pace with evolving technology.
The Importance of Debugging in Software Development
Debugging isn't simply a chore to check off a list; it’s a vital process that ensures the integrity and quality of software output. Without it, developers risk shipping applications riddled with bugs that can lead to catastrophic failures and user dissatisfaction. A few key areas where debugging plays a pivotal role include:
- Enhancing Code Quality: Through debugging, programmers cultivate cleaner, more efficient code, which is crucial for maintainability and scalability.
- User Experience: A bug-free application contributes to a seamless user experience, minimizing frustration and maximizing usability. That's a big deal when you're vying for user loyalty.
- Development Time and Costs: Identifying and resolving issues early in the development process saves time and reduces costs, which can otherwise balloon if bugs linger undetected.
In summary, the process of debugging is indispensable in software development. It not only addresses immediate errors but also contributes to long-term success for developers and companies alike. Understanding and mastering debugging techniques can mean the difference between a successful application and one that flounders due to unresolved issues.