Mastering Database Programming with Python
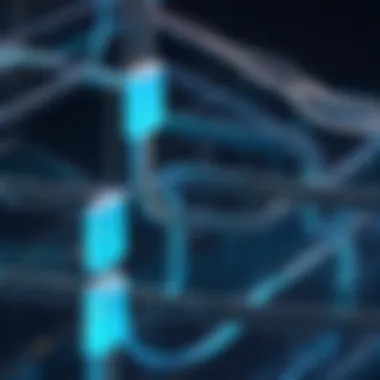
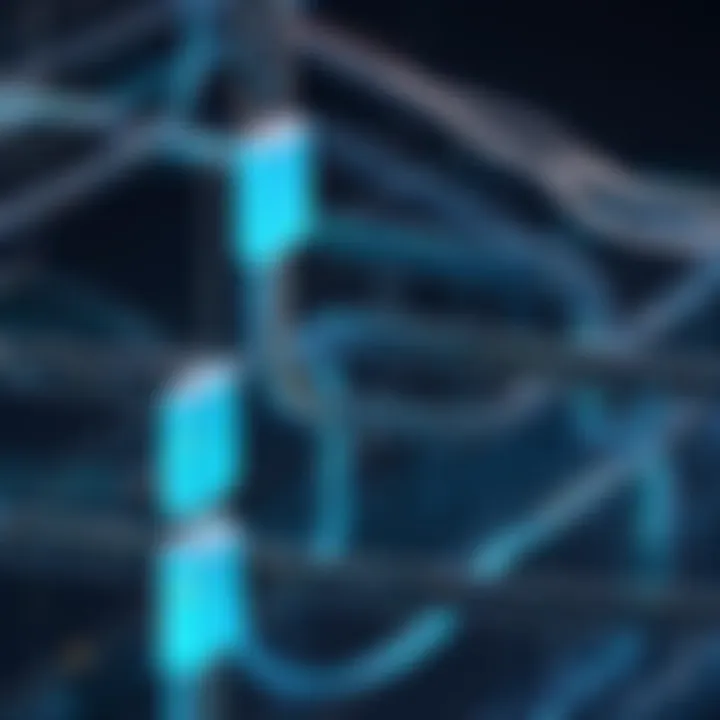
Intro
Python has grown into a powerful tool in the realm of database programming. For students and budding developers, understanding how Python interacts with databases opens a world of possibilities. This section lays the groundwork for diving into the world of Python database technologies, capturing essential concepts and their significance.
Prelude to Programming Language
History and Background
Python's inception dates back to the late 1980s, crafted by Guido van Rossum. Initially designed as a successor to the ABC language, Python was conceived with code readability in mind. Fast forward to today, it stands as one of the premier languages utilized for a variety of applications, with database programming being a key area. Its flexibility and simplicity have made it a go-to choice among developers worldwide.
Features and Uses
Several characteristics contribute to Pythonâs stronghold in database programming:
- Simplicity and Readability: Python's syntax allows developers to express concepts clearly and concisely.
- Rich Libraries: Libraries such as SQLite3, SQLAlchemy, and Django ORM provide powerful tools for interacting with databases seamlessly.
- Community Support: An active community ensures ongoing support, continuous updates, and abundant resources for troubleshooting.
These features enable Python developers to handle various types of databases, from relational databases like PostgreSQL and MySQL to NoSQL databases like MongoDB, with relative ease.
Popularity and Scope
Recent surveys indicate that Python is one of the most sought-after programming languages, especially in fields like data science, machine learning, and web development. Its popularity can be largely attributed to its versatility. With many organizations opting for Python, the skills associated with it can be beneficial in securing various tech roles. The scope ranges from web applications to automation scripts and large-scale data processing tasks.
Basic Syntax and Concepts
Variables and Data Types
In Python, a variable serves as a container for storing data. The dynamic nature of Python allows you to assign any type of data to a variable without specifying its type initially. Here are common data types in Python:
- Integers: Whole numbers like 5, 100, or -25.
- Floats: Decimal numbers like 5.0 or -2.7.
- Strings: Text encapsulated in single or double quotes, such as 'Hello' or "World".
- Booleans: True or False values, often used in conditional statements.
Operators and Expressions
Operators in Python perform operations on variables and values. Common operators include:
- Arithmetic Operators: Such as + (addition), - (subtraction), * (multiplication), and / (division).
- Comparison Operators: Such as == (equal), != (not equal), > (greater than), and (less than).
Expressions combine values and operators, allowing for complex calculations and logical evaluations.
Control Structures
Control structures direct the flow of execution within a program. Here are key concepts:
- Conditional Statements: Using , , and to execute code based on certain conditions.
- Loops: and loops manage repetitive tasks efficiently, iterating over sequences like lists and strings.
Advanced Topics
Functions and Methods
Functions allow programmers to encapsulate code blocks for reuse, leading to cleaner, more manageable scripts. A simple function is defined using the keyword:
Object-Oriented Programming
Object-oriented programming (OOP) in Python is a paradigm centered around the concept of âobjects.â This approach fosters modular design, making code easier to manage. Key concepts include:
- Classes: Blueprints for creating objects.
- Inheritance: Mechanism to create new classes based on existing ones.
Exception Handling
Handling errors gracefully in Python ensures robustness. The and blocks are instrumental in managing potential errors during execution:
Hands-On Examples
Simple Programs
Basic scripts can perform fundamental tasks, like simple database operations using SQLite:
Intermediate Projects
Building a small web application with Flask that interacts with a database illustrates practical implementations of Python database programming.
Code Snippets
Utilizing Python's libraries for effective database operations can drastically reduce the amount of code needed for commonplace tasks.
Resources and Further Learning
Recommended Books and Tutorials
- Automate the Boring Stuff with Python by Al Sweigart: Great for beginners.
- Python Crash Course by Eric Matthes: Offers hands-on projects.
Online Courses and Platforms
- Coursera and Udacity provide numerous courses focusing on Python and database interactions.
Community Forums and Groups
Platforms like Reddit and Stack Overflow host vibrant communities where learners can ask questions and share experiences in database programming with Python.
Python's ability to seamlessly integrate with almost any type of database makes it an indispensable skill for modern developers.
Prelude to Python Database Programming
In modern software development, the role of databases stands out as pivotal. Whether you're creating a simple app, a complex web service, or a data-driven enterprise solution, integrating a capable database system can make or break your project. This discussion serves as an introduction to Python database programming, highlighting its growing importance in the tech landscape.
Using databases with Python enables developers to store and retrieve data efficiently, ensuring that applications can meet user needs without compromising on performance. The methods and libraries available with Python help streamline this process, allowing programmers to focus on more complex tasks rather than getting bogged down by low-level database management details.
The Role of Databases in Software Development
Databases act as the backbone of data management in software applications. It's hard to imagine an application without a way to handle, organize, and query data. In essence, a database provides a systematic way to store, modify, and retrieve information, which can be crucial for applications that require real-time data processing.
Some key aspects of databases in software development include:
- Data storage: Databases allow for structured data storage, ensuring information can be easily accessed when needed.
- Data management: Handling data directly becomes simpler with databases, especially when dealing with large volumes. Data integrity and security are also improved as databases manage these aspects more effectively.
- Scalability: Applications frequently need to grow with user demand. Databases support scalability and can handle increasing amounts of data without degrading performance.
- Collaboration: In team environments, databases facilitate collaboration among developers by providing a central location for data access and modification.
In short, databases provide a foundation for building robust and effective applications. This leads us to why Python is often the language of choice when it comes to database programming.
Why Choose Python for Database Interaction?
Python has rapidly gained traction in various domains, particularly in database programming. The reasons for its popularity extend beyond its well-structured syntax and developer-friendly features. Here are some notable reasons:
- Simplicity: Python's syntax is clean and straightforward, making it accessible even for beginners. This simplicity allows developers to focus more on algorithms and logic rather than getting lost in complex syntax.
- Versatility: Whether youâre working with SQL databases like PostgreSQL and MySQL or NoSQL options like MongoDB, Python can connect and interact with a wide array of database systems.
- Robust Libraries: Python boasts several powerful libraries, such as SQLite, SQLAlchemy, and Pandas. These give developers the tools to manipulate and analyze data efficiently.
- Community Support: Python's vast pool of users and developers means it's easy to find solutions, snippets, and best practices when interfacing with databases.
Choosing Python for database interaction offers a blend of power and simplicity, crucial for both new programmers and seasoned developers alike. With its growing ecosystem and versatility, learning Python database programming becomes an attractive endeavor for anyone looking to make an impact in software development.
"The fastest way to connect with data is to use the right language"
As we delve deeper into the essentials of Python database programming, itâll be crucial to cover not only the practical aspects but also the foundational knowledge that supports these operations. This holistic approach will make your journey into Python database programming enlightening and fully rounded.
Core Concepts of Database Programming
Database programming forms the backbone of modern applications, as it allows for the systematic storage, retrieval, and manipulation of data. Understanding the core concepts of this field is crucial for anyone stepping into Python database programming. It sets a solid foundation for grasping more complex topics later on. Within this context, two fundamental elements stand out: database management systems (DBMS) and the comparison between relational and non-relational databases. Each aspect brings its own set of benefits and considerations that significantly influence how data is managed and utilized in software development.
Understanding Database Management Systems
Database Management Systems are software platforms that facilitate the creation, management, and organization of databases. These systems play a crucial role by providing the necessary tools to seamlessly work with information in a structured manner. When dealing with any programming language, including Python, familiarity with DBMS is not just recommended; itâs essential.
A DMBS primarily serves to:
- Organize data into a coherent structure, making information accessible and manageable.
- Handle concurrent data access, ensuring that multiple users or processes can work with data without stepping on each otherâs toes.
- Ensure consistent data storage and retrieval through a normalized schema.
Moreover, DBMS can be categorized into two broad types: relational databases and non-relational (NoSQL) databases, each of which supports different data models and offers distinct advantages depending on application requirements.
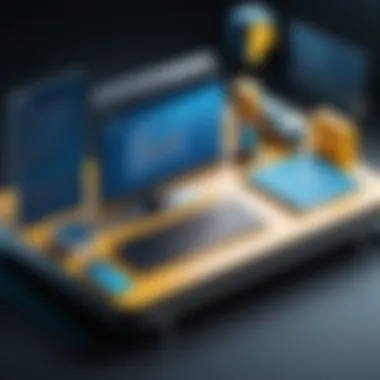
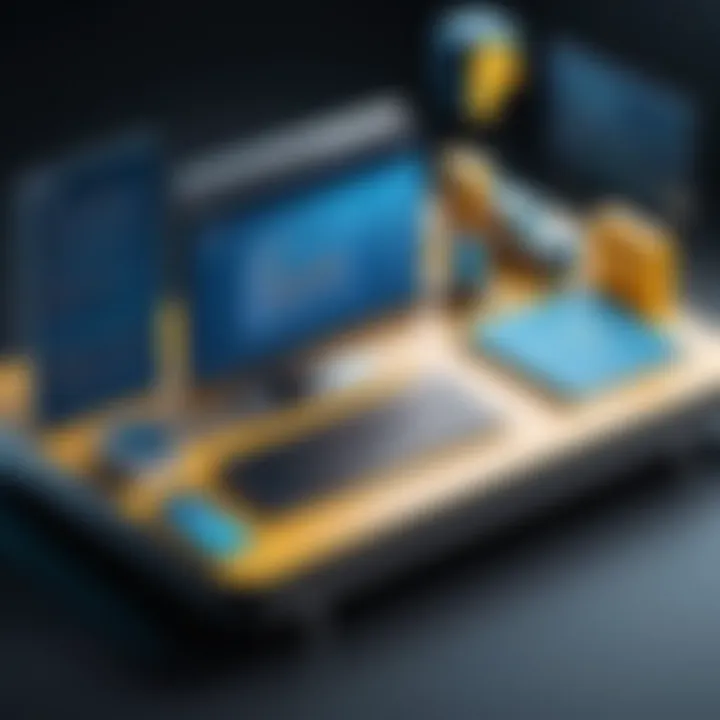
Relational vs Non-relational Databases
When distinguishing between relational and non-relational databases, it becomes clear that both have their perks and are suited for different scenarios.
Relational Databases
Relational databases, such as PostgreSQL or MySQL, utilize structured query language (SQL) to manage data. These databases store information in predefined tables made up of rows and columns. They typically support ACID (Atomicity, Consistency, Isolation, Durability) properties to ensure data integrity and reliability. Here are some characteristics:
- Structured Data: Perfect for applications with fixed data models where relationships between entities are crucial.
- Joins and Complex Queries: Relational databases support joins, enabling complex queries across multiple tables.
- Data Integrity: Enhanced through normalization and constraints that prevent data anomalies.
Non-relational Databases
On the other hand, non-relational databases like MongoDB or Cassandra embrace flexibility. They are often used for large sets of distributed data and can handle unstructured or semi-structured information. Key features include:
- Scalability: More suitable for horizontally scaling across multiple nodes, making them ideal for large data volumes.
- Document or Key-Value Storage: Data can be stored in a way that matches application needs without a rigid schema.
- Faster Writes: Typically provide better write performance than relational models, accommodating rapid data changes.
"Understanding the types of databases and their mechanisms is crucial for any developer. It determines not just how you write code, but how your application interacts with the digital world."
Choosing between these database types hinges upon your application requirements, data structure, and expected load. Each has its unique advantages and drawbacks, but understanding their core principles will undeniably lead to more informed design decisions in Python database programming. With a solid grasp of these basic concepts, programmers can better tackle advanced programming tasks that capitalize on Pythonâs capabilities in data management.
Essential Python Libraries for Database Programming
In the realm of database programming, choosing the right tools plays a pivotal role in the development process. The essential libraries in Python that facilitate database management not only provide ease of connectivity but also bring a whole suite of functionalities to the table. In this part of the article, we will dive into three critical libraries: SQLite, SQLAlchemy, and Pandas. Each of these libraries shines brightly in its own niche but collectively enhances the Python programming experience when working with databases.
SQLite in Python
SQLite is often the go-to choice for developers looking to administer lightweight applications. This file-based database doesnât necessitate a dedicated server, making it an ideal option for smaller projects or for those just dipping their toes into the waters of database programming.
When using SQLite with Python, the module comes into play. Developers enjoy a straightforward syntax to interact with this library. This ease of use is particularly beneficial for beginners.
Hereâs a quick code snippet illustrating how you might connect to a SQLite database:
The simplicity of SQLite makes it a favorite for many developers. Furthermore, its portability â being a single file â is stupendous, allowing easy migration across different systems. This makes SQLite incredibly useful in applications such as small web applications, mobile apps, or even when you just need a reliable data store for prototyping ideas.
SQLAlchemy - The ORM Toolkit
Next up is SQLAlchemy, which has a reputation that precedes it. As an Object Relational Mapper (ORM), it allows developers to interact with databases in a more intuitive way. Instead of writing heaps of SQL queries, you can handle database operations using Python classes and methods, which can be especially appealing for those inclined towards an object-oriented programming style.
One of the notable benefits of SQLAlchemy is its flexibility. It can work with a variety of database backends â be it PostgreSQL, MySQL, or SQLite, you name it. How about this â with just minor code changes, you can switch from one database system to another, which can save a lot of headaches when considering scalability.
A basic example utilizing SQLAlchemy to define a table and insert records can be seen below:
With SQLAlchemy, one can manipulate data using Python's syntax, which can significantly reduce the learning curve for those familiar with the language but not with SQL. While it does come with a steeper learning curve than SQLite, the long-term benefits â such as improved maintainability â are well worth it for many developers.
Pandas for Data Analysis
Last but not least, letâs talk about Pandas. While it's primarily known for data manipulation and analysis, it proves to be an invaluable asset when it comes to working with database data. With its powerful data frame capabilities, one can quickly load data from databases, manipulate it, and analyze it, all within the same environment.
Pandas simplifies the data analysis process significantly. You can read SQL queries directly into a Pandas DataFrame, allowing for immediate inspection and analysis, which can streamline experimental approaches to data exploration. Hereâs a common way of using Pandas to read from a SQL database:
Using Pandas, one can perform all kinds of data manipulations like filtering, grouping, and even visualizations while maintaining a connection to any SQL database. It truly elevates the data analysis phase, making it simpler and more efficient.
In summary, whether you're working on a small project with SQLite, leveraging the robustness of SQLAlchemy as an ORM toolkit, or diving into data analysis with Pandas, these essential libraries will significantly enrich your Python database programming experience.
Setting Up Your Python Environment
Setting up your Python environment is, without a doubt, a crucial foundation for successful database programming. This stage is where your journey begins, forming the bedrock for all subsequent development work youâll embark on. Having a well-structured environment not only enhances your coding productivity but also simplifies the process of managing dependencies and libraries that are essential for working effectively with databases. The benefits of an organized setup canât be overstated: speed, efficiency, and clarity in your coding endeavors.
Key Factors to Consider:
- Version Control: Ensure you're using a consistent version of Python, ideally the latest stable release, as various libraries may drop support for older versions within no time.
- Virtual Environments: Embrace the idea of virtual environments. They allow you to create isolated spaces for your projects, preventing conflicts between library dependencies.
- Package Management: Familiarize yourself with package managers like . This tool simplifies the installation and management of libraries critical for your database workloads.
The way you set up this environment significantly affects not just your current project, but future ones as well. Getting it right from the start minimizes compatibility issues that could rear their ugly heads down the line.
Installing Python and Required Libraries
To kick off, you need to install Python itself along with the libraries you're going to use. The Python Software Foundation provides an easy-to-navigate site where you can grab the latest version. You can download it from Python's official website.
Once you have Python installed:
- Open a terminal or command prompt:
- Install : This should come bundled with your Python installation, but if it doesnât, follow the instructions on the official site. Use the command to make sure itâs set up.
- Create a virtual environment: Utilize the commandReplace with your environment name. This step is like crafting a little silo for your projectâs needs and dependencies.
- Activate the environment:
- Install required libraries:
Itâs helpful to have packages like , , and handy for development. Use commands liketo grab these tools in one go.
- On Windows, use
- On macOS/Linux, use
By setting everything up this way, you protect your main Python installation from any package chaos that might arise during your development process.
Connecting to Databases from Python
Once your environment is ready, the next step is to connect Python to your chosen database. This is where the real magic happensâenabling you to execute commands, manage data, and craft sophisticated interactions.
Start by deciding which database you want to connect to. Different databases have different connection methods:
- SQLite:
SQLite is often the starting point for beginners. Itâs lightweight, serverless, and comes included with Python. Connecting is as easy as: - PostgreSQL:
If you prefer a more robust solution, libraries like are needed. Install it using pip if itâs not on your system. Then, set it up:
Once the connection is established, donât forget to handle it properly: close the connection when done to avoid any unwanted resource hogging!
Always remember: A well-defined environment and robust connections are the keys to a smooth programming experience!
In summary, dedicating time to set up your environment and connecting properly to databases establishes a reliable foundation for successful Python database programming. This groundwork helps to streamline and ease your programming journey, ensuring that you can focus more on enhancing your skills and less on dealing with setup headaches.
CRUD Operations in Python
CRUD operationsâstanding for Create, Read, Update, and Deleteâare the backbone of database management. Understanding these operations is pivotal for anyone looking to effectively manipulate data within a database using Python. Through these operations, users can perform essential functionalities that drive applications, making CRUD a fundamental skill for developers.
When you think about managing data, imagine a boardroom filled with employees. Each employee represents a row in your database table, where they have specific attributes like name, position, or salary. If you want to add a new employee, update their info, check who's there, or even remove someone, thatâs CRUD in action. By mastering these operations, youâre essentially learning how to resonate with the data.
Creating Data Records
Creating data records is your first step into the world of database operations. The ability to insert new data into your database is crucial, especially when youâre dealing with dynamic information. For instance, consider a customer feedback system; every time a customer submits a review, you want to create a new record that includes their thoughts and the date of submission. This operation often resembles a straightforward task in code but carries significance in the fullness of your database.
In Python, creating records typically utilizes the SQL INSERT statement. Here's how you can do it using SQLite:
This code snippet showcases a basic implementation of inserting records. Adjusting this pattern allows the expansion of your application as new data constantly streams in.
Reading Data from Databases
Reading data is where practical implementation really shines. It allows you to query your database, fetch data, and present itâan element crucial for applications that need to display information. For example, an e-commerce site might want to pull up product details whenever a user clicks on a category. This operation often involves SELECT statements.
In Python, reading data looks something like this:
When executed, this code fetches all records in the table and prints each. Itâs vitalâthis operation ensures you have continuous access to your data, allowing users to see what's available smoothly.
Updating Existing Records
Updating existing records can be critical when the data needs to be refreshed. Imagine a scenario where you need to modify the address of a client in your database. This operation not only fixes inaccuracies but also boosts the relevance of your stored information. In a real-world application, users may change their preferences or update their credentials. This means your system must adapt to these changes without creating duplicates or losing information.
To update a record in Python, you would typically employ the SQL UPDATE statement:
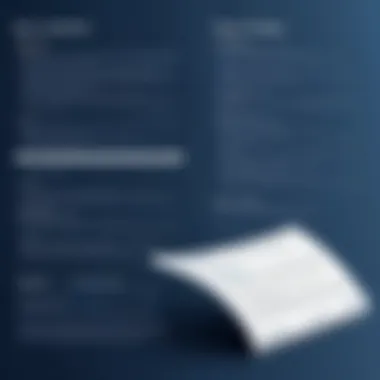
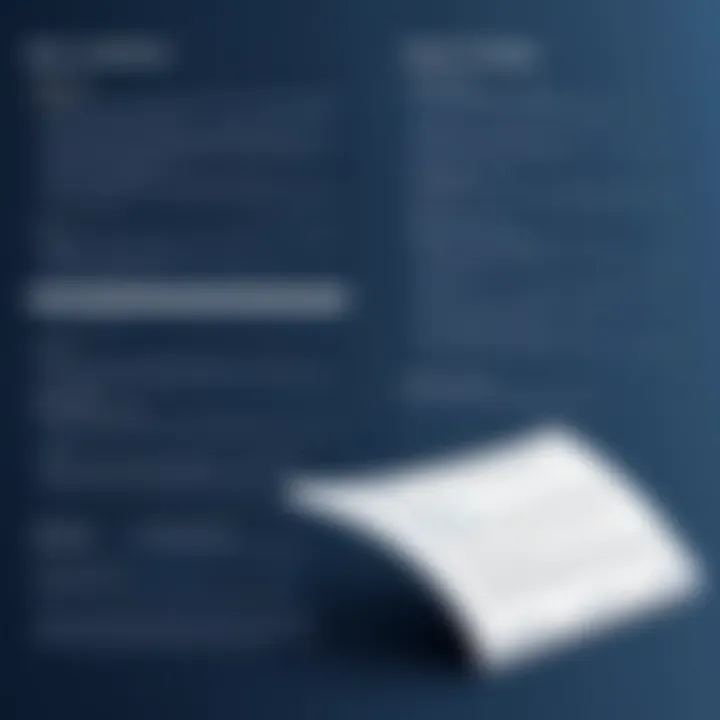
This snippet effectively changes an existing review in the database. It reflects the importance of keeping data fresh and accurate.
Deleting Data Records
Last but not least, deleting records plays a pivotal role in database management. Sometimes, it becomes necessary to clean houseâbe it outdated records, erroneous entries, or data that no longer serves its purpose. This operation is straightforward but should be approached with care; after all, mistakes can lead to data loss. A good practice is to establish soft delete strategies where data can be marked as deleted rather than being permanently removed.
Using Python to delete records would involve the SQL DELETE statement:
In this case, the review with an ID of 1 is removed from the database. Having the right permissions and protocols in place ensures that deletions happen without jeopardizing the integrity of your overall dataset.
In summary, mastering CRUD operations equips you to effectively handle data in Python database programming. Each operation plays a critical role in data management, allowing developers to maintain, access, and manipulate data seamlessly, strengthening the effectiveness of your applications.
Handling Transactions in Python Databases
Managing transactions is a vital aspect of database programming, particularly when working with Python. Transactions ensure that a series of operations are executed in a controlled manner, maintaining the integrity and consistency of the database. Imagine youâre in a restaurant, placing an order that requires several steps: selecting a dish, providing payment, and a waiter delivering it. If any step fails, such as the dish not being available or payment being declined, the entire order process should be reversed. This analogy simplifies what transactions do in databasesâthey either complete entirely or not at all.
Understanding Transactions
In a broad sense, a transaction is a sequence of operations treated as a single unit. If something goes wrong during one of these operations, the transaction can be rolled back to maintain the database's pristine state.
Key properties, often referred to as ACID (Atomicity, Consistency, Isolation, Durability), underpin this concept:
- Atomicity: Ensures that all operations within the transaction are completed successfully. If any operation fails, none are executed.
- Consistency: Guarantees that a transaction will bring the database from one valid state to another valid state, maintaining data integrity from start to end.
- Isolation: Makes certain that transactions do not interfere with one another, even if they occur simultaneously.
- Durability: Once a transaction is committed, it remains so regardless of future system failures.
Understanding these aspects is crucial for developers, as it directly impacts how they will design and implement database operations in Python.
Implementing Transactions in Code
In Python, implementing transactions can be done efficiently using libraries designed for database interaction, such as SQLite or SQLAlchemy. Hereâs a brief look at how this can be put into practice with SQLite:
Code Example:
This code snippet does a few things:
- It starts by establishing a connection to the SQLite database.
- The statement initiates a transaction, allowing subsequent operations to be treated as a singular unit.
- If all operations succeed, is executed to save changes. However, should an error occur, the will undo any changes made during that transaction.
Using transactions in this manner optimizes data management while helping to safeguard against data corruption. As you delve deeper into database programming, mastering transactions will undoubtedly enhance the reliability of your applications.
Error Handling in Database Operations
Effective error handling is akin to having a safety net in the vast world of database programming. Just as high-wire performers need to be prepared for unforeseen tumbles, developers must also anticipate mishaps that arise when interacting with databases. Missteps can lead to data loss, corrupt transactions, or worse, a tarnished reputation of the application. Thus, focusing on robust error handling is not merely an afterthought but a fundamental aspect of database operations.
Errors can manifest in various forms due to factors like incorrect SQL queries, connection issues, or even unexpected data types. The risks associated with these can be substantial. Proper management of these errors empowers programmers to create resilient applications that not only recover gracefully but also offer better user experiences. By acknowledging potential pitfalls and implementing sound error handling practices, developers can maintain integrity and functionality in their applications.
Common Database Errors
When navigating the terrain of database programming, knowing the common pitfalls is crucial. Here are some frequent errors developers may encounter:
- Syntax Errors: Mistakes in SQL commands can lead to execution failure, whether it's a missing comma, a misspelled keyword, or incorrect parameter usage.
- Connection Failures: Often stemming from network issues or wrong credentials, these errors prevent any database interaction from taking place.
- Timeout Errors: If the database takes too long to respond, operations may time out, and developers need to have safeguards in place.
- Data Integrity Issues: These occur when constraints such as foreign keys or unique indexes are violated, leading to failed insertions or updates.
- Transaction Errors: Situations where transactions cannot complete due to various reasons like deadlocks or lock timeouts.
Identifying these issues early helps streamline your debugging efforts and contributes to more stable applications.
Best Practices for Error Handling
An effective error handling strategy can make the difference between a seamless user experience and the dreaded spinning wheel of death. Here are some best practices to consider:
- Implement Comprehensive Logging: Capture detailed logs for every error that occurs. This aids in tracking issues and speeding up the debugging process.
- Use Try-Except Blocks: In Python, utilize and blocks to gracefully handle exceptions. This method allows the program to continue running even if an error occurs.
Code that may cause an error
db.execute(query) except DatabaseError as e: print(f'An error occurred: e')
Moreover, stored procedures can encapsulate business logic. For instance, if calculations tied to business rules exist, placing these rules within a stored procedure keeps logic consistent and helps in maintaining data integrity. However, it's also crucial to consider potential drawbacks. If not carefully managed, too many stored procedures can lead to convoluted databases that are difficult to understand and manage. Also, code becomes harder to maintain since itâs separated from the application logic, making debugging a more challenging task.
Remember: Stored procedures elevate performance but come with added complexity. Always weigh the trade-offs based on your application's needs.
Database Caching Techniques
Caching is an indispensable technique that can significantly boost the performance of database applications. Essentially, caching involves storing copies of frequently accessed data to reduce the number of requests sent to the database, thereby improving response times.
Implementing caching can be done at different layers and there are various strategies to do so. For instance:
- In-memory caching: This strategy stores data in RAM for quick access. Libraries like Redis or Memcached can be utilized for this. Retrieving information from memory is considerably faster than fetching it from a database.
- Result caching: If certain queries yield results that donât change often, caching those results can save considerable computation time. Itâs particularly useful in read-heavy applications but be cautious with updates, as cached data may become stale.
- Database-Level Caching: Some databases offer built-in caching mechanisms. Understanding how to leverage these features effectively can lead to reduced load on database servers and improved application throughput.
Consider a web application where the same data is requested repeatedly. Without caching, each request travels to the database server, leading to additional latency. By employing caching, the first request fetches the data, which is then stored. Any subsequent requests can pull from this cached data rather than hitting the database again.
In terms of implementation:
In short, the worth of understanding these complex topics is not just in their individual benefits but also in how they cumulatively enhance the reliability and efficiency of Python applications interacting with databases. By leveraging stored procedures and caching techniques, developers can create robust applications that can handle increased loads while maintaining optimal performance.
Testing and Debugging Database Applications
In the world of Python database programming, the precision of one's code is critical. Testing and debugging are crucial components that protect the integrity of your applications. When developers write code to handle database interactions, a misstep in the logic can lead to serious issues, such as data corruption or application crashes. Recognizing the importance of these practices can save time and resources in the long run.
Why Test and Debug?
The smooth functioning of a database-driven application hinges on rigorous testing. First off, itâs about ensuring reliability. A thorough test regimen helps identify flaws before they reach production, safeguarding user experience. Debugging, on the other hand, involves the process of locating and correcting those pesky errors that often pop up. Effective debugging not only resolves current problems but also provides insights for future development.
Here are some key elements to consider:
- Identify Vulnerabilities: Testing illuminates potential weaknesses in your code.
- Prevent Regression: It helps ensure that fixes donât create new issues.
- Enhance Performance: Continuous testing can reveal bottleneck areas that slow down database queries.
The benefits of adopting a solid testing and debugging strategy cannot be overstated. By catching issues early, you minimize the risk of bigger headaches later on. Moreover, it boosts the confidence of developers, allowing them to write and refactor code without the nagging fear of introducing defects.
Unit Testing Database Interactions
Unit testing focuses on small, isolated pieces of codeâspecifically functions or modulesâensuring they behave as expected. In database programming, this means verifying that your functions for creating, reading, updating, and deleting data perform their tasks correctly, independently from the overall application.
To effectively unit test database interactions, one might employ tools like pytest or unittest. These frameworks allow developers to write tests that cover various scenarios, such as verifying if a record is correctly added to the database or if a query returns the expected results.
Hereâs a concise example using unittest for a function that retrieves a userâs details:
This simple test checks that calling with a specific user ID returns the expected name. Itâs concise, easy to understand, and directly verifies that a critical database interaction works as anticipated.
Debugging Common Database Issues
Debugging demands not only technical skill but also an analytical approach to pinpointing issues. In database applications, it often revolves around unexpected outputs from database queries, connection failures, or transaction conflicts.
Common issues can include:
- Incorrect SQL Queries: Typos in SQL statements can lead to syntax errors or failed executions.
- Connection Errors: Problems such as timeouts or authentication failures can halt your application.
- Data Integrity Issues: Unexpected null values or duplicates might signal that validation processes need attention.
To tackle these issues, employing debugging techniques like logging can offer insights into what went wrong. For instance, a developer might add debug logs before and after database operations:
This log records the data being inserted and confirms when the operation is done. When issues arise, these logs act as breadcrumbs, revealing what occurred at each step of the process.
"The essence of program debugging is the art of removing bugs from software that can turn a perfectly written program into a real headache."
Taking the time to develop effective testing and debugging strategies not only minimizes risks but also accelerates development time down the line. As one delves into the complexities of Python database programming, these practices stand as vital foundations that boost confidence and contribute to a successful project.
Security Considerations in Database Programming
When delving into the realm of database programming, itâs vital to acknowledge the security aspects that protect sensitive data from prying eyes and ensure the integrity of information. Security considerations are not mere afterthoughts; they are foundational elements that significantly influence how applications are designed and implemented. From safeguarding user information to maintaining compliance with regulations, a thorough understanding of security in database programming is paramount.
The potential consequences of neglecting security can range from data breaches, resulting in financial loss and reputational damage, to legal repercussions. Therefore, addressing the vulnerabilities inherent in database management is not only about protecting assets but also about fostering trust among users. In this section, we explore two crucial aspects of database security: the prevalence of SQL injection vulnerabilities and the best practices that can be employed to secure access to databases.
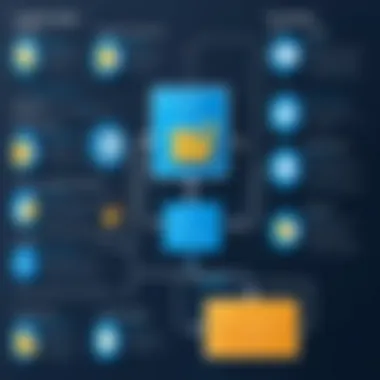
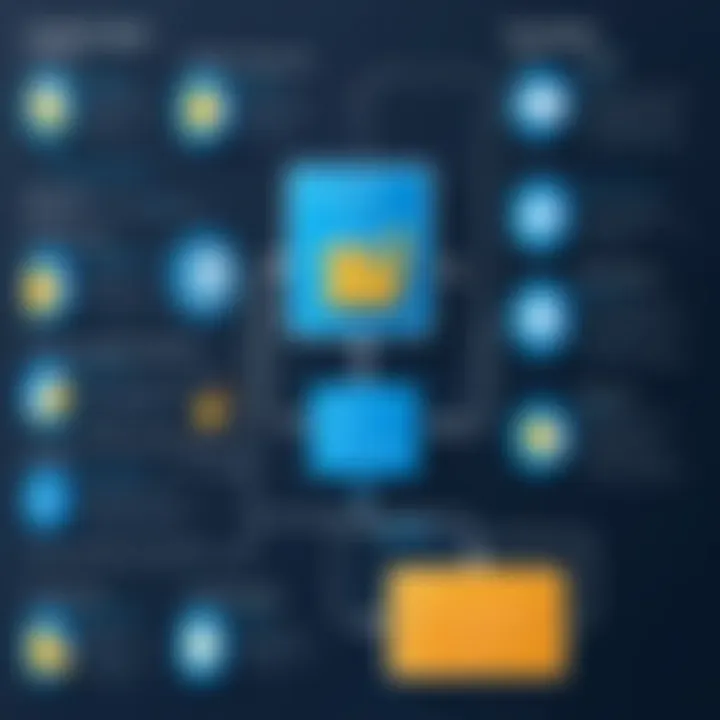
SQL Injection Vulnerability
SQL injection is one of the most common, yet devastating security threats in database programming. Essentially, it occurs when an attacker exploits vulnerabilities in an application's software to execute malicious SQL commands. These commands could allow an intruder to view or manipulate sensitive data, and in severe cases, to gain administrative rights over the database itself.
This form of attack typically happens when user inputs are not properly validated or sanitized before being used in SQL statements. For example, consider an application where user credentials are validated using the following naive approach:
Here, if an attacker enters a specially crafted username, they could easily manipulate the query to bypass authentication.
To mitigate such risks, developers must adopt preventive measures like:
- Prepared Statements: Using parameterized queries can greatly reduce the risk of SQL injection, as it differentiates between commands and data.
- Input Validation: Always ensure that inputs conform to expected types, formats, and lengths. For instance, check that a username is alphanumeric and does not exceed a certain length.
- Database Permissions: Restrict user permissions to only what is necessary. Limiting access rights significantly minimizes the impact of a potential attack.
Best Practices for Secure Database Access
Implementing security measures is not solely the responsibility of the database or the application; itâs a collective effort that involves clear policies and best practices. Hereâs a rundown of strategies to enhance the security of database access:
- Use of Encryption: Always encrypt sensitive data, both at rest and in transit. This ensures that even if data is intercepted, it remains unreadable to unauthorized parties.
- Regular Security Audits: Conduct frequent audits of database access logs and user activity to identify anomalies or unauthorized access attempts early.
- Implement Multi-factor Authentication: Adding an extra layer of authentication can make it harder for attackers to gain access, even if they acquire user passwords.
- Keep Software Updated: Ensure that the database management systems and related frameworks are up to date to prevent exploits stemming from known vulnerabilities.
Remember: A strong security posture helps not only in protecting data but also in building a reputation for reliability and trustworthiness.
In an era where data breaches are a daily occurrence, integrating security considerations into the database programming process is non-negotiable. Understanding SQL injection vulnerabilities and adhering to best practices for secure database access can work miracles in fortifying your applications against potential threats.
Performance Optimization in Database Queries
Performance optimization in database queries is a critical aspect of programming that can significantly influence the efficiency and usability of applications. Slow database queries can bottleneck application performance and provide a frustrating user experience. Consequently, understanding how to enhance query performance is not just an added skill; it's essential for any serious developer.
When it comes to database applications, performance issues can arise from various factors such as inefficient queries, lack of indexing, and poor database design. Optimizing these queries can lead to faster response times, reduced server load, and improved overall application performance. Given the complexity of modern applications, where multiple database calls occur simultaneously, neglecting query optimization could result in significant delays and lost revenue opportunities.
Consider the following elements and benefits of optimizing database queries:
- Reduced Latency: By optimizing queries, you can significantly lower the time it takes to fetch data from the database. Low latency translates to quicker responses, which is crucial for user satisfaction.
- Lower Resource Consumption: Efficient queries consume fewer server resources, allowing more concurrent users to access the database without straining the server.
- Scalability: As applications grow, the database must manage increasingly large data sets. Optimized queries can handle this growth more gracefully, ensuring that performance doesn't degrade as demand increases.
- Cost Efficiency: For cloud-based database solutions, reduced resource usage can result in lower operational costs, allowing businesses to redirect funds to other critical areas.
In the realm of Python database programming, there are two principal strategies that can be employed to achieve performance optimization: indexing and analyzing query execution plans.
Indexing for Speed
Indexing is one of the cornerstones of database performance optimization. Think of an index as a roadmap of the data stored in your database. Just like a table of contents in a book, an index allows the database engine to find specific rows of data without having to wade through every record in the table.
The use of indexes can dramatically speed up data retrieval operations. Here are a few key points about indexing:
- Types of Indexes: The most common type of index is a B-tree index, which organizes data in a way that allows for quick access. Other types include full-text indexes, hash indexes, and spatial indexes. Each serves a specific purpose based on the nature of queries.
- Trade-offs: While indexes improve read operations, they can slow down write operations (inserts, updates, and deletes) because the indexes need to be maintained. Therefore, itâs crucial to find the right balance for your applicationâs needs.
- Choosing Indexes Wisely: Not every column should be indexed. Over-indexing can lead to wasted resources and diminished performance. Columns frequently used in WHERE clauses or as join keys are often suitable candidates for indexing.
Pro tip: Always monitor your database performance before and after implementing indexes to ensure they are providing the intended benefit.
Analyzing Query Execution Plans
Another important aspect of optimizing database performance is the analysis of query execution plans. When a query runs, the database optimizer creates a plan detailing how it intends to retrieve the requested data. Examining this plan allows developers to understand potential bottlenecks and inefficiencies.
- EXPLAIN Keyword: Most relational database management systems provide functionality to obtain the execution plan of a query via the EXPLAIN keyword. This will reveal how the database processes your query, showing which indexes are used, the order of operations, and data access methods.
- Identifying Issues: By reviewing execution plans, developers can spot issues such as:
- Continuous Monitoring: As your application evolves and data grows, keeping an eye on the query plans and making adjustments as necessary is crucial. Regular reviews can lead to ongoing optimized performance.
- Full table scans where indexes should be used.
- Unnecessary joins or subqueries that can be simplified.
- Operations that could benefit from additional indexing.
"Performance optimization is not a one-and-done task. It's an ongoing process that requires attention and adjustment."
Deployment of Database Applications
When it comes to the lifecycle of any software system, deployment often stand as a crucial juncture. In the realm of Python database programming, this stage is the bridge between development and real-world usage. Successfully deploying a database application not only demonstrates the efficacy of your coding skills but also ensures that your applications perform as expected under actual conditions. This section aims to unpack the nuances surrounding the deployment of database applications, outlining its significance along with the pivotal considerations and advantages associated with it.
Deploying database applications involves several steps, including preparing the infrastructure, deploying the application, and ongoing management. Here are a few salient elements to consider:
- Infrastructure Planning: Before you jump into the thick of deployment, itâs essential to plan your infrastructure. Use cloud platforms like AWS, Google Cloud, or Azure which offer performance, scalability, and security.
- Configuration Management: Ensure your database configurationsâsuch as connection strings, user permissions, and resource allocationâare correctly set up. Tools like Ansible and Terraform can simplify this task.
- Backup Strategies: Incorporating robust backup practices is non-negotiable. This protects against data loss and maximizes uptime for users.
"The real test of any application comes after deployment. Itâs not just about writing codeâitâs about ensuring it works effectively in the wild."
By systematically addressing these aspects during deployment, you can enhance your applicationâs reliability, security, and performanceâa trifecta that contributes to overall user satisfaction.
Preparing for Production
Getting ready for a production environment is like getting a house in order before guests arrive. It goes beyond merely uploading code to a server; it includes meticulous checks for readiness. Here are key steps to consider for preparation:
- Testing Thoroughly: Before launching, ensure that your code, especially database interactions, is rigorously tested. Implement unit tests and integration tests that mimic real-world scenarios. Testing frameworks such as pytest or unittest can be your best friends here.
- Load Testing: Simulate potential peak traffic using tools like Apache JMeter or Gatling. This helps to understand how your application behaves under stress and identify potential bottlenecks.
- Monitoring Setup: Establish monitoring systems that track database performance and application health. Services like Datadog and Prometheus can automate this task, providing insight into operations in real-time.
- Security Measures: Ensure your application is secure before launch. This includes setting database user roles correctly, and implementing encryption for data in transit and at rest.
Taking the time to prepare the application for production means fewer headaches down the line, making the deployment smoother.
Monitoring Database Performance Post-Deployment
Once youâve taken the plunge and deployed your application, the real work begins. The performance of your database must now be monitored consistently to ensure it meets the demands of users. Monitoring goes far beyond just keeping an eye on number of connections or error logs; it encompasses various metrics:
- Query Performance: Regularly analyze slow-running queries. Use tools like EXPLAIN in SQL to dissect their efficiency. Sometimes small adjustments can yield significant performance upgrades.
- Resource Utilization: Keep tabs on CPU, memory, and disk I/O usage. Alerting systems can inform you when usage spikes, preventing potential outages before they occur.
- User Activity Monitoring: Track user activity and behavior within your application to identify patterns. This can provide insights for future optimizations or features.
- Backup Verification: Regularly check the integrity of your backups. You never know when you might need them, and the last thing you want is to discover a backup is corrupt when you need it most.
Monitoring doesnât just safeguard your applicationâit empowers you to tweak and adjust your database as needed, ensuring smooth operations over time. Ultimately, establishing an early monitoring routine can prevent minor issues from snowballing into major obstacles.
Case Studies in Python Database Programming
In the ever-evolving landscape of database management, case studies serve as real-world snapshots that illustrate how Python is harnessed to tackle complex data challenges effectively. Often, itâs through these cases that learners grasp abstract concepts, seeing them roll into practical applications. This section delves into the importance of scrutinizing case studies, paving the way for impactful discussions about methodologies and results.
One fundamental benefit of analyzing case studies is that they provide concrete examples of success. Instead of merely learning the theory behind database operations, individuals can see how organizations like Netflix or Spotify employ Pythonâs library ecosystem to enhance user experience and streamline data handling. Itâs these stories that highlight the adaptability of Python in various sectors, from e-commerce to finance, and even social media platforms.
Moreover, they allow learners to appreciate the integration of best practices within real-world scenarios. When exploring the intricate dance of Python with databases, students gain insights into the pitfalls to avoid and strategies to implement.
Real-world Applications of Python in Databases
Practical applications of Python in database management cover a broad spectrum, illustrating versatility across various industries.
- Data Analysis and Reporting: Companies like Dropbox utilize Python libraries such as Pandas and SQLAlchemy to manage and analyze large data sets efficiently. They can generate insightful reports that help in decision making.
- Web Development: Django, a popular Python framework, includes robust ORM capabilities that simplify database interactions. It allows developers to craft data-driven web applications while ensuring security and performance.
- Data Migration and ETL Processes: Organizations frequently rely on Pythonâs powerful data handling capabilities during migration projects. For instance, using libraries such as Airflow, companies can automate ETL processes, pulling data from various sources into a centralized database without breaking a sweat.
Each of these applications reflects Python's capability to adapt and scale according to specific business needs.
Success Stories of Database-Driven Projects
Examining success stories of database-driven projects encourages understanding of the tangible impacts Python can have in the business realm. These narratives often highlight not just the technology used, but also the strategic decisions that led to positive outcomes.
- One striking example can be seen in the work of Airbnb. The platform handles a massive volume of data about user listings and transactions. Utilizing Pythonâs extensive libraries, theyâve developed an efficient database solution that supports their complex operations while ensuring a seamless user experience.
- Another noteworthy case is that of Instagram. They transitioned to PostgreSQL, leveraging Python to enable quick iterations on features, integrating user feedback seamlessly into their development cycle. This adaptability has proven crucial as they scale.
"Learning through real-world examples not only reinforces theoretical knowledge but also inspires creativity and innovation in practical applications."
Future Trends in Python Database Programming
In the ever-evolving landscape of technology, the realm of database programming is no exception. Future trends in Python database programming are essential to comprehend as they influence how we manage, interact with, and leverage data in applications. A keen understanding of these developments not only prepares programmers for forthcoming challenges but also empowers them to harness innovative solutions, leading to better data management and analysis.
Emerging Technologies and Their Impact
With the rapid advancement of technology, some emerging trends like cloud computing, serverless architecture, and increased automation in database management are gaining traction.
- Cloud computing: This has fundamentally changed the way databases are hosted and scaled. More companies are opting for cloud services offered by providers like Amazon Web Services (AWS) and Google Cloud Platform (GCP). Database administrators can focus on the functionality without worrying about the hardware.
- Serverless architecture: This trend means developers can deploy database functions without managing any infrastructure. In practice, it allows for more agile development and quick iterations, reducing the time spent on backend setups and more on logic and functionality.
- Automation: Tools that automate routine database tasksâsuch as backups, updates, and performance monitoringâare becoming standard. Python is adapting beautifully through libraries that integrate with these tools, making it seamless for developers to set up and maintain databases practically and effortlessly.
These technologies not only enhance efficiency but also democratize database management, allowing smaller teams to operate at a scale previously reserved for larger enterprises.
The Growing Role of AI in Databases
Artificial intelligence is reshaping database programming in significant ways. The potential for AI to enhance data handling and decision-making processes cannot be overstated. Here are a few impacts worth noting:
- Intelligent Query Processing: AI algorithms can optimize database queries, reducing execution time and boosting overall efficiency. This feature is immensely useful when dealing with large amounts of data.
- Predictive Analytics: Through machine learning, AI can analyze historical data to predict future trends, giving businesses a clear competitive edge. Using Python libraries such as TensorFlow, developers can create models that are integrated directly with their databases.
- Automated Data Management: AI streamlines data management tasks such as data cleaning, transformation, and integration. This process speeds up how quickly data can be accessed and utilized for insights, essential for fast-paced environments.
- Enhanced Security Measures: AI can help identify patterns and anomalies in database activity that signify potential security threats. By using Pythonâs machine learning capabilities, developers can build systems that proactively guard against unauthorized access and anomalies.
In general, the infusion of AI into database management creates possibilities for innovation that weren't conceivable a decade ago. As programmers, especially those using Python, adapt to these trends, they will find themselves standing on the frontline of a technological revolution, equipped with the tools to not just keep pace but to lead the charge.
Closure and Final Thoughts
In this fast-paced digital era, the significance of Python database programming cannot be overstated. It serves as a crucial bridge between data handling and application functionality. Throughout this article, weâve examined varied aspects of database programming with Python, highlighting its potential in both simplifying complex tasks and enhancing developersâ capabilities. Understanding these foundational elements offers a springboard to more advanced concepts, promoting better retention of the subject matter.
The key lies in recognizing how effective database management can lead to streamlined workflows and better decision-making in business contexts. Pythonâs versatility, paired with robust libraries, provides a powerful toolkit for both budding and experienced programmers. With this framework, users can dive into data manipulation, query optimization, and transactional processes seamlessly.
Recap of Key Takeaways
- Role of Databases: Central to software development, databases underpin reliable data storage and retrieval, enhancing application functionality.
- Python's Strengths: High-level syntax and rich libraries make Python an attractive choice for database interaction. Libraries like SQLite, SQLAlchemy, and Pandas streamline workflows.
- CRUD Operations: Fundamental to database manipulation are Create, Read, Update, and Delete operations, essential for full lifecycle management of data.
- Transaction Handling: Understanding how transactions work prevents data corruption, ensuring data integrity.
- Error Management: Implementing robust error-handling mechanisms is vital for producing resilient applications, saving developers from many potential headaches.
- Performance Considerations: Indexing and analyzing query execution plans lead to more efficient data retrieval, vital in todayâs data-driven environment.
- Security Practices: Guarding against vulnerabilities like SQL injection is imperative in safeguarding sensitive information.
- Future Trends: Anticipating developments in AI-enhanced databases is key to staying ahead in programming landscapes.
Continuing Your Database Programming Journey
Now that youâve gained a solid grounding in Python database programming, it's time to take practical steps towards continuing your journey. Below are some actionable paths to enhance your skills further:
- Hands-on Practice: Engage in personal projects that require database interaction. This could involve data analysis, web applications, or automation scripts.
- Community Learning: Join forums and communities such as Reddit or local coding groups to exchange knowledge and tackle challenges collectively.
- Explore Advanced Topics: Delve deeper into topics like machine learning integrations or data visualization using libraries such as Matplotlib or Seaborn.
- Stay Updated: The tech world evolves rapidly. Regularly read articles, participate in workshops, or follow subject matter experts on platforms like Facebook to keep your skills sharp.
- Build a Portfolio: Document your projects in a portfolio. This not only showcases your skills but also facilitates networking opportunities.
By approaching this subject with curiosity and dedication, the doors to new possibilities are wide open. Keep on pushing boundaries, and embrace the journey ahead!