Mastering Data Structures: A Guide for Interviews
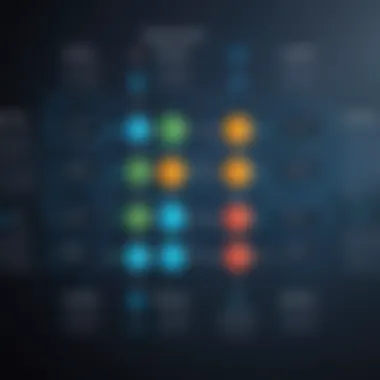
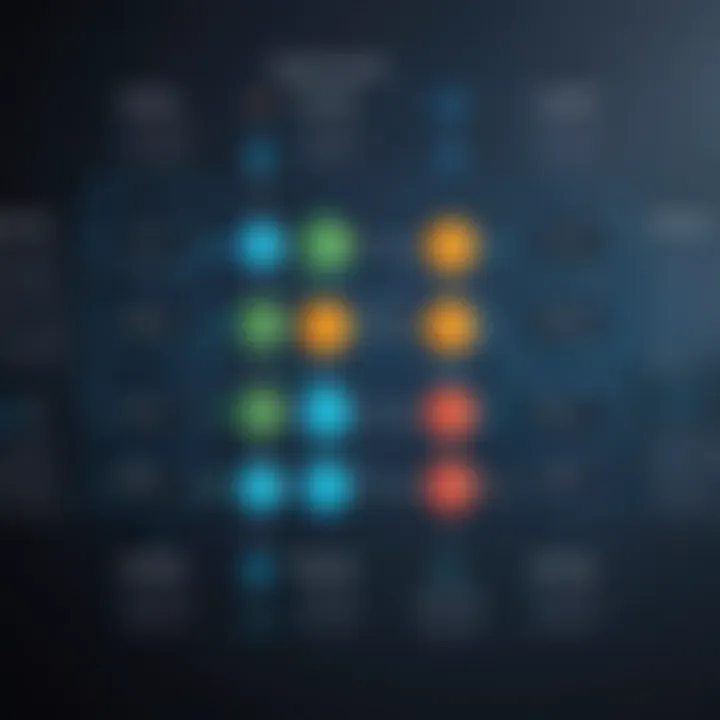
Intro
In the sphere of coding interviews, understanding data structures is paramount. These structures serve as the backbone that supports programming efforts, enabling efficient data management and manipulation. In this article, we shall explore various data structures, their applications, and the reasons they are essential for problem-solving in interviews.
Importance of Data Structures in Coding Interviews
Data structures play a critical role in assessing a candidate's technical skills. Interviewers often use data structure-related questions to gauge problem-solving approaches, analytical thinking, and coding proficiency. Mastery of these structures can significantly enhance one's performance in coding interviews.
Key Data Structures to Understand
- Arrays: Fundamental collections of items identified by indexes. They allow for quick access to their elements but have fixed sizes once initialized.
- Linked Lists: Collections that consist of nodes, each containing data and a reference to the next node. Linked lists are dynamic and can grow or shrink in size.
- Stacks: These follow Last In First Out (LIFO) principle, often used in scenarios like backtracking.
- Queues: Following the First In First Out (FIFO) rule, queues are ideal for handling tasks in order.
- Trees: Structured hierarchically, trees consist of nodes with parent-child relationships. They help in efficient searching and sorting.
- Graphs: Complex structures made of vertices and edges, graphs are vital in optimizing routes and connections.
Strategies for Mastering Data Structures
To excel in coding interviews, focus on practicing problems that involve these structures. Utilizing platforms such as LeetCode or HackerRank can be beneficial.
- Understand Basic Concepts: Each data structure has unique properties and use cases. Grasping these is crucial.
- Solve Practice Questions: Practical experience reinforces learning. Attempt various questions related to each structure.
- Review and Analyze: After solving, review your solutions. Consider different approaches and their efficiencies.
"The mastery of data structures is a step toward coding fluency. The more adept you are, the better your skills will shine in interviews."
Recommended Resources
- Books: "Cracking the Coding Interview" by Gayle Laakmann McDowell offers an excellent overview of common interview questions.
- Online Courses: Coursera and edX provide many courses in data structures that cater to various levels of proficiency.
- Community Study Groups: Engage with forums on Reddit or Stack Overflow. Engaging with peers can help reinforce knowledge.
Mastery of data structures is not just about rote learning but fostering a deep understanding. This concept is vital to addressing real-world programming challenges and excelling during interviews.
Prelims to Data Structures
Data structures are pivotal in the realm of computer science and programming. They serve as the backbone for effective data management and manipulation. Understanding data structures is crucial, especially for individuals preparing for coding interviews. In interviews, employers often assess candidates on their knowledge of various data structures, as this knowledge directly relates to problem-solving capabilities.
Definition and Importance
A data structure is a particular way of organizing and storing data in a computer to enable efficient access and modification. The importance of data structures lies in their ability to optimize the performance of algorithms. For instance, a suitable data structure can vastly improve the efficiency of search operations, data retrieval, and updating records.
Key reasons why data structures matter in coding interviews include:
- Efficiency: Choosing the right data structure can enhance runtime performance.
- Scalability: During system design, the appropriate data structure allows for scalable solutions as data volumes grow.
- Problem Solving: Many programming challenges can only be effectively solved by implementing the correct data structure.
Understanding the definition and importance of data structures enables programmers to make informed choices during problem-solving scenarios, particularly in interviews.
Data Structures in Programming Languages
Each programming language provides a set of built-in data structures, along with the ability to create custom ones. For example, languages like Python offer lists, dictionaries, and sets, which translate concepts like arrays and hash tables from classic data structure theory. In contrast, languages such as C++ offer vector and map classes, emphasizing data manipulations in a more manual and controlled manner.
Several benefits of knowing data structures specific to programming languages include:
- Language Proficiency: Familiarity with native data structures enhances a developer's overall proficiency in the language.
- Code Optimization: Knowledge of underlying data structures allows for more optimized code, as developers can select the most suitable structures for their algorithms.
- Enhanced Problem Solving: Different languages present unique data handling capabilities. Understanding these helps in structuring solutions effectively.
Thus, before entering an interview, candidates must grasp how various programming languages implement data structures. This knowledge not only aids in answering conceptual questions but also helps in writing efficient code during technical assessments.
"A strong command of data structures is a distinct advantage in coding interviews."
Types of Data Structures
Understanding different types of data structures is crucial for anyone preparing for coding interviews. These structures serve as the foundation for organizing and managing data efficiently. Knowing them helps in selecting the right structure for specific problems, leading to better performance and, ultimately, success in interviews. There are two main categories: primitive and non-primitive data structures. Each of these has specific characteristics and uses, which we will explore in detail.
Primitive Data Structures
Primitive data structures are the basic building blocks of data handling in programming. They include types like integers, floats, characters, and booleans. The value types that these structures hold are directly represented in the memory. Therefore, they are straightforward to use and manipulate. Understanding these primitives is essential because they often form the base for more complex data types.
- Examples of Primitive Data Structures:
- Integer: Represents whole numbers, important for counting or indexing.
- Float: Used for decimal values, necessary for precise calculations.
- Character: Refers to single letters or symbols, fundamental in text processing.
- Boolean: Indicates truth values, key for decision-making processes.
These structures offer simplicity and efficiency. They can efficiently represent a range of common data types in programming languages. However, they can be limiting when dealing with more complex data relationships or larger datasets.
Non-Primitive Data Structures
Non-primitive data structures are more sophisticated and designed for storing multiple values or collections of data. They can be classified into two categories: linear and non-linear structures. Understanding these types enhances flexibility and allows tackling a broader range of problems.
- Linear Data Structures: Data elements are arranged in a sequential manner. Examples include:
- Non-Linear Data Structures: Unlike linear data structures, the elements are not stored sequentially. Examples include:
- Arrays: Fixed-size collections of elements of the same type, crucial for quick access and manipulation.
- Linked Lists: Consists of nodes where each node contains data and a reference to the next node, enabling efficient insertions and deletions.
- Stacks: Follow Last In First Out (LIFO) principle, useful in scenarios that require reversing data or managing function calls.
- Queues: Operate in First In First Out (FIFO) method, facilitating order management in tasks like scheduling.
- Trees: Hierarchical structures that enable organized data representation.
- Graphs: Composed of nodes connected by edges, useful for representing relationships.
Understanding non-primitive data structures is vital for grasping more complex algorithms and systems. They provide flexibility by allowing for dynamic data sizes and complex relationships.
In interviews, questions around non-primitive structures often focus on applying algorithms effectively to solve problems or improve performance.
Learning about both primitive and non-primitive data structures broadens your capability as a programmer. Emphasizing this knowledge will strengthen your problem-solving skills, which is vital for coding interviews.
Arrays
Arrays serve as fundamental building blocks in computer programming. Their significance cannot be overstated, especially in coding interviews where efficiency and optimization are key. Understanding arrays gives candidates a solid advantage. This section will delve into arrays, covering their structure, operations, and how they are often scrutinized in interviews.
Overview of Arrays
An array is a collection of elements, typically of the same data type, stored in contiguous memory locations. This structure allows for efficient access and manipulation of data. The fixed size of arrays is a compelling factor. Once declared, they offer a consistent way to handle data, simplifying algorithms that require indexing. Arrays also lay the groundwork for understanding more complex data structures like matrices or multidimensional arrays.
For example, in programming languages like Java or C++, arrays are declared and utilized as follows:
Here, is an array holding five integers. The indexing starts at zero, allowing for straightforward retrieval of elements.
Common Operations
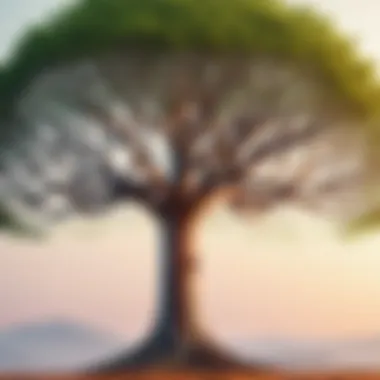
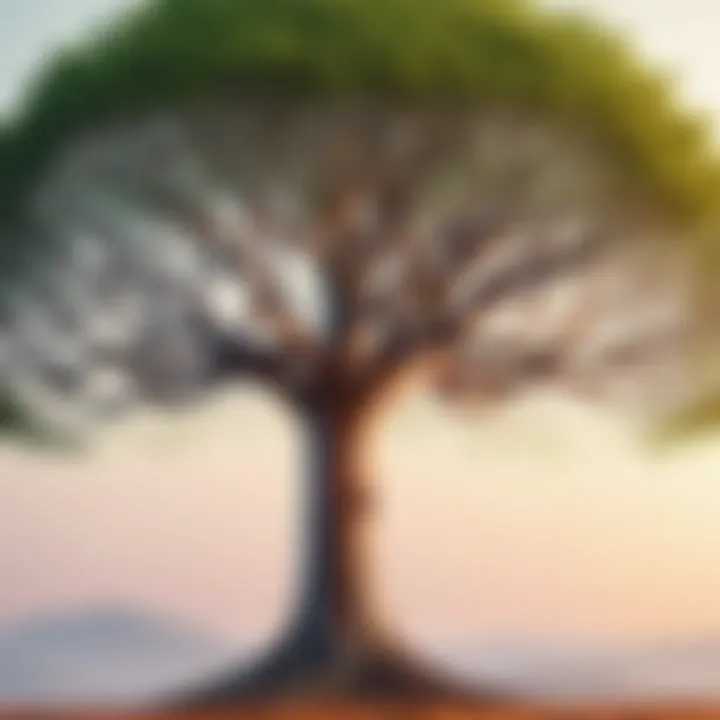
Arrays support several essential operations, each crucial in solving problems efficiently. Key operations include:
- Accessing Elements: Elements in an array can be accessed directly using their index. This operation has a time complexity of O(1).
- Inserting Elements: Adding an element typically involves shifting existing elements to accommodate the new value. This operation has an average time complexity of O(n).
- Deleting Elements: Similar to insertion, deletion requires shifting elements, making it an O(n) operation as well.
- Iterating through Elements: Accessing each element in an array relies on iteration, which is O(n).
Understanding these operations helps identify the situations when arrays are particularly useful. For example, accessing elements quickly makes them ideal for algorithms requiring fast lookups.
Interview Questions on Arrays
In coding interviews, candidates often encounter specific questions designed to evaluate their understanding of arrays. Here are several common types of questions:
- Find the Maximum Element: Given an array, determine the largest number. This tests simple iteration techniques.
- Reverse an Array: This requires manipulation of the array's structure and understanding of in-place versus auxiliary storage.
- Merge Two Sorted Arrays: Candidates must combine arrays while maintaining sorted order, showcasing proficiency in manipulation and efficiency.
"Array problems frequently focus on manipulation and efficiency. Be prepared to explain both your thought process and the optimal approach.”
Overall, mastering arrays is pivotal for success in coding interviews. They not only serve as a primary data structure but also offer insight into more advanced concepts. With practice, candidates can tackle array-related problems with confidence.
Linked Lists
Linked lists are important data structures that offer several advantages for programmers preparing for coding interviews. Unlike arrays, linked lists consist of nodes that hold data and pointers to the next node. This structure allows for efficient memory usage and ease in dynamic memory allocation. The flexibility of linked lists makes them ideal for certain applications where the size of the dataset cannot be predetermined. A deep understanding of linked lists can significantly enhance the problem-solving skills required in interviews.
Understanding Linked Lists
A linked list consists of nodes, which can be understood as the building blocks of the structure. Each node typically contains two main components: the data field and a pointer to the next node. This creates a sequence that can grow or shrink dynamically as data is added or removed. One of the key characteristics of linked lists is that they do not require contiguous memory space, which reduces the likelihood of memory allocation issues found in arrays.
Moreover, linked lists allow for simplified insertions and deletions, which are performed in constant time if the node to be manipulated is known. The downside, however, is that they require more memory for pointers and are less efficient for random access compared to arrays.
Types of Linked Lists
There are several types of linked lists, each with specific advantages and use cases:
- Singly Linked List: Each node points to the next node, forming a one-way chain. This structure is simple and efficient for certain operations like appending items.
- Doubly Linked List: Nodes have pointers to both the next and previous nodes. This allows for two-way traversal of the list but uses more memory due to the additional pointers.
- Circular Linked List: In this structure, the last node points back to the first node, creating a circular connection. This type is useful for applications that require a rotating or circular traversal of data.
Understanding these variations allows programmers to select the most appropriate linked list type for specific problems.
Common Linked List Problems
In coding interviews, questions relating to linked lists often focus on classic problems that test a candidate’s understanding of the structure and its manipulation. Here are some common problems:
- Reversing a Linked List: Interviewers often ask candidates to reverse a linked list in various ways, usually requiring an understanding of in-place algorithms.
- Detecting Loops: This problem requires finding cycles in a linked list, often solved using techniques such as Floyd’s cycle-finding algorithm.
- Merging Two Sorted Linked Lists: Candidates may be asked to combine two sorted linked lists into a single sorted list, testing their ability to traverse and manipulate the data.
- Finding the Middle Element: This problem typically uses the slow and fast pointer technique to identify the middle of a linked list effectively.
Mastering these problems and having a strong grasp on linked lists will significantly enhance success at coding interviews.
Stacks
Stacks are fundamental data structures that play a crucial role in many programming scenarios. They follow the Last In, First Out (LIFO) principle, meaning that the most recently added element is the first to be removed. Understanding stacks is essential for programming interviews, as they are often used to evaluate a candidate's skills in object-oriented design and algorithmic thinking. Stacks help in managing function calls, parsing expressions, and handling backtracking algorithms, to name a few applications. Through proper mastery of stacks, candidates can demonstrate their ability to solve problems effectively under time constraints.
Basics of Stacks
A stack operates like a collection of items stacked on top of each other. You can only add or remove items from the top. This behavior is implemented through two primary operations: push and pop. The push operation places an item on top of the stack, while the pop operation removes the top item. The top of the stack can also be accessed without removing the item using the peek operation.
Here are some key characteristics of stacks:
- LIFO Structure: The last element added is the first to be removed.
- Dynamic Size: The stack can grow or shrink as items are added or removed.
- Limited Access: Items can only be added or removed from the top, making access to elements more restricted compared to other data structures.
Implementing a stack can be done using arrays or linked lists, and each method has its advantages. Arrays provide faster access and require fewer memory allocations, but linked lists allow for a dynamic size without needing to resize arrays.
Applications of Stacks
Stacks have various important applications in programming:
- Function Call Management: Stacks are used to keep track of active function calls in programming languages through the call stack.
- Expression Parsing: Compilers use stacks to parse and evaluate expressions, particularly for handling operators and parentheses.
- Undo Mechanism: In applications, stacks are often used to implement undo functionalities, where the last action can be reversed easily.
- Backtracking Algorithms: Many algorithms that require exploring all possible options, like solving mazes or puzzles, use stacks to backtrack.
Using stacks effectively can lead to more organized and efficient code structures. Recognizing when to use a stack can greatly improve program performance in scenarios that require maintaining order of operations.
Sample Interview Questions
To prepare for coding interviews, it helps to familiarize yourself with typical stack-related questions. Here are some examples that can aid in your studies:
- Implement a stack using an array. Describe the operations and any challenges you face.
- How would you reverse a string using a stack? Discuss your approach and its efficiency.
- Explain how a stack can be used to check for balanced parentheses in an expression. What algorithm would you use?
- What is the space complexity of a stack implemented using a linked list? Discuss cases where one implementation might be preferred over the other.
Understanding the underlying principles and practical implementations of stacks can greatly enhance your problem-solving capabilities in technical interviews.
By mastering these aspects, candidates can articulate their thought process effectively while demonstrating a solid grasp of fundamental data structures.
Queues
Queues are a fundamental data structure that is crucial for understanding various algorithms and systems. They operate on the principle of First In First Out (FIFO), meaning the first element added to the queue will be the first one removed. This property makes queues especially useful in scenarios where order matters, such as scheduling tasks, managing resources, and dealing with asynchronous data. In coding interviews, the ability to manipulate queues can demonstrate proficiency in programming and problem-solving.
Queue Fundamentals
At its core, a queue has a few basic operations that are essential for management. These operations include:
- Enqueue: Adding an element to the back of the queue.
- Dequeue: Removing the front element from the queue.
- Peek/Front: Accessing the front element without removing it.
- IsEmpty: Checking if the queue is empty.
These operations can often be implemented using arrays or linked lists, but they come with their own set of performance implications. In terms of time complexity, both enqueue and dequeue operations take O(1) time in a well-implemented queue.
Types of Queues
Understanding the different types of queues can enhance your ability to select the appropriate queue for a given problem. Here are the main types:
- Simple Queue: The basic FIFO structure where the first element inserted is the first out.
- Circular Queue: A queue where the last position is connected back to the first position. This structure optimizes memory usage and can prevent overflow.
- Priority Queue: Elements are removed based on priority, rather than the order in which they were added. This is useful in algorithm design where certain tasks need immediate attention.
- Double-Ended Queue (Deque): Elements can be added or removed from both ends. This flexibility can start to solve more complex problems efficiently.
Each type of queue serves specific use cases, from task scheduling to managing resources effectively in memory.
Interview Problems Involving Queues
Queues often appear in coding interviews, and candidates should be prepared to tackle problems that test their understanding of this structure. Common problems include:
- Implementing a Queue Using Stacks: This problem tests your understanding of both queues and stacks and how to manipulate their behaviors.
- Implementing a Circular Queue: Candidates may be asked to implement a circular queue from scratch, which tests knowledge of memory management and algorithm design.
- Breadth-First Search (BFS): This algorithm, commonly used in graph traversal, employs a queue to manage the order of node visits.
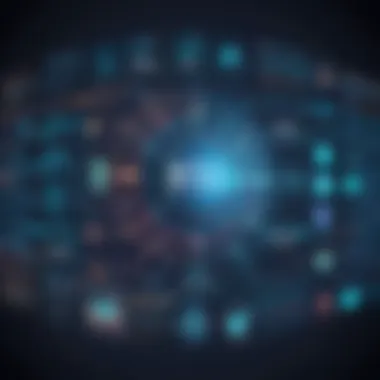
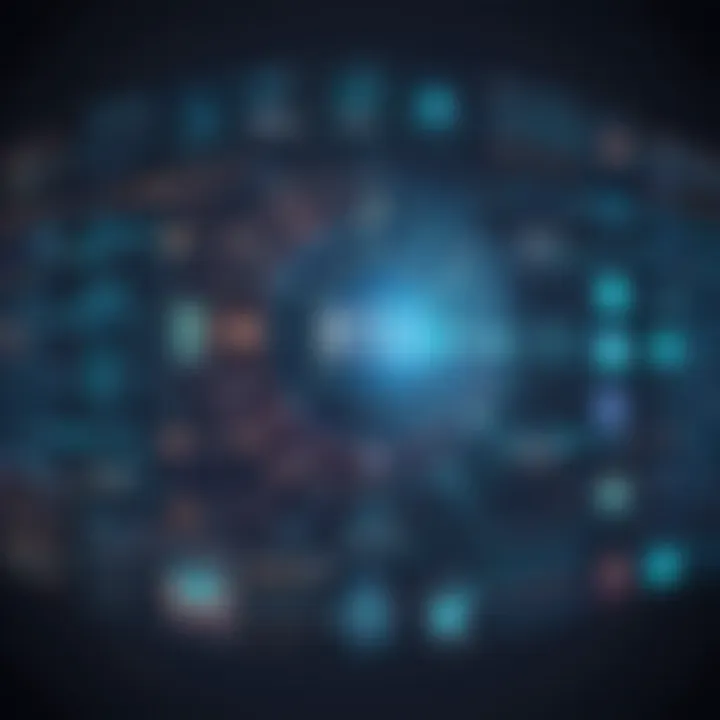
Being familiar with these problems and practicing them can significantly improve readiness for coding interviews.
Tip: Always review the basic queue operations before the interview. This can save valuable time on problem-solving.
By understanding queues, their types, and their role in algorithmic problems, you can sharpen your skills and approach coding interviews with increased confidence.
Trees
In the realm of data structures, trees occupy a pivotal position. They are hierarchical structures that allow for efficient organization and manipulation of data. In coding interviews, understanding trees can set candidates apart. Trees help illustrate relationships between data points, making them essential for representing both simple and complex datasets. The flexibility of trees, alongside their varied representations, brings about multiple benefits in real-world applications like databases and file systems.
One of the significant aspects of trees is their ability to facilitate efficient searching and sorting. This characteristic is crucial during interviews since many problems demand optimal solutions. Moreover, trees provide hierarchical organization, which is beneficial for representing relationships and structures, such as folders in a file system or the structure of a family tree.
Tree Structures Explained
A tree consists of nodes and edges, forming a connected graph without cycles. The top node is called the root, while nodes without children are terminal nodes or leaves. Trees can have different characteristics based on how they are organized. For instance, a full tree has all nodes either fully filled or leaf nodes. Conversely, a balanced tree maintains an even distribution of nodes among subtrees, leading to faster operations.
The depth or height of a tree is also essential. The depth refers to the length from the root to any node. In interviews, knowing how to calculate the depth or height of a tree can be vital. It impacts time complexity when evaluating the efficiency of algorithms utilizing tree data structures.
Binary Trees Versus Binary Search Trees
Binary trees are a specific type of tree where each node has at most two children. They are foundational for many algorithms and applications. On the other hand, binary search trees (BST) offer additional constraints. In a BST, the left child node has a value less than its parent, while the right child node has a value greater. This property allows for efficient searching and inserting of values.
The choice between binary trees and BSTs depends on the problem at hand. If the goal is to maintain sorted data with efficient search operations, BSTs are preferable. However, when structure is more critical than data ordering, binary trees can serve better.
Common Tree Algorithms
Several algorithms operate on trees, offering various functionalities. Some common algorithms include:
- Traversal Algorithms: In-order, pre-order, and post-order traversals help in visiting all nodes in a specified order. These are particularly crucial during tree search operations.
- Search Algorithms: Finding a specific node or value utilizes tree properties to reduce search time.
- Insertion and Deletion Algorithms: Adding or removing nodes while maintaining the structure is another essential skill in manipulating trees.
- Balancing Algorithms: Techniques such as rotations in AVL trees keep the tree balanced, ensuring optimal performance.
Understanding these algorithms and their applications in trees is vital in interviews where efficiency and time complexity are evaluated.
Interview Questions Related to Trees
When preparing for coding interviews, familiarity with tree-related questions can enhance problem-solving skills. Some common interview questions include:
- Explain the differences between binary trees and binary search trees.
- Write a function to traverse a binary tree in a pre-order manner.
- Describe how to find the height of a tree.
- Given a binary tree, implement a function to check if it is balanced.
- Explain how to find the least common ancestor in a binary search tree.
These questions test not only knowledge of trees but also the ability to apply that knowledge effectively. Practicing these can prepare candidates for the complexities of real interview scenarios.
Graphs
The significance of graphs in programming and data structures should not be underestimated. In many applications, relationships between elements are represented as graphs. This provides a powerful way to model real-world scenarios, as they can describe networks such as social connections, pathways in maps, and dependencies in tasks. Understanding graphs is crucial for problem-solving in coding interviews where you may need to demonstrate your knowledge of graph manipulation and traversal techniques. Knowledge in graphs will empower you to tackle many algorithmic challenges effectively.
Graph Basics
Graphs consist of vertices and edges. A vertex, or node, is a fundamental part of the graph representing entities. An edge symbolizes a connection between two vertices. These connections can be directed or undirected, which means they can have a specific direction or be bidirectional. Moreover, graphs can be weighted or unweighted. Weighted graphs have edges with associated costs, while unweighted ones treat all edges equally.
There are two primary representations for graphs: adjacency list and adjacency matrix. The adjacency list is more space-efficient, especially for sparse graphs, while the adjacency matrix can be easier for identifying relationships between nodes quickly. Understanding these representations is fundamental to efficiently implementing algorithms.
Graph Traversal Techniques
Graph traversal methods are essential for exploring and manipulating the structure of graphs. The most common techniques are Depth-First Search (DFS) and Breadth-First Search (BFS).
- Depth-First Search (DFS) searches as far as possible down one branch before backtracking. It uses a stack (either implicitly via recursion or explicitly) and is good for situations where you want to explore paths exhaustively.
- Breadth-First Search (BFS) explores all neighbors at the current depth prior to moving on to nodes at the next depth level. It uses a queue to maintain order of exploration. BFS is particularly useful in finding the shortest path in unweighted graphs.
Both algorithms are fundamental for solving numerous problems, including finding connected components, detecting cycles, and performing topological sorting.
Key Graph Problems in Interviews
Several graph-related problems frequently appear in coding interviews. Familiarizing oneself with these can greatly aid preparation. Here are a few notable examples:
- Shortest Path Problems: Algorithms like Dijkstra's and Bellman-Ford are used to find the shortest paths from a source to other nodes. This problem tests understanding of weighted graphs and efficient search strategies.
- Cycle Detection: Identifying cycles in directed and undirected graphs is a common problem. This may require the application of DFS or Union-Find techniques.
- Connected Components: Finding connected components in a graph tests candidates on both DFS and BFS methods of traversal.
- Topological Sorting: This problem involves ordering vertices in a directed acyclic graph. It assesses understanding of graph structures and traversal methods.
By mastering these essential concepts related to graphs, candidates can approach interviews with confidence and clarity. Adequate preparation in this area will enhance problem-solving skills and understanding of complex systems.
Hash Tables
Hash tables are critical data structures that facilitate efficient data retrieval and storage. Their significance in coding interviews cannot be overstated, as they offer fast access to data through unique keys. This unmatched performance, particularly in scenarios demanding swift lookups, makes a thorough understanding of hash tables essential for aspiring programmers.
Preamble to Hash Tables
A hash table organizes data in a way that enables quick access using keys. The key is transformed into an index through a hash function, allowing for rapid retrieval. Each entry in a hash table consists of a key-value pair. The value can be any data type, while the key usually remains unique.
One of the major advantages of using hash tables is their average time complexity for operations. Insertion, deletion, and lookup can generally be performed in constant time, O(1), although this can vary depending on factors like hash collisions. Hash tables thus become a powerful tool in programming, especially when efficiency is paramount.
Collision Resolution Techniques
Collisions occur when two keys hash to the same index. Effective resolution techniques are necessary to handle these situations. Two primary methods are widely employed:
- Chaining: Each index in the hash table points to a list of entries with the same hash value. When a collision occurs, the new entry is added to the list at that index. This approach is flexible, allowing for dynamic changes in the list size.
- Open Addressing: If a collision happens, this method finds an alternative index for the new entry within the hash table. Strategies like linear probing or quadratic probing can be used to find the next available index. While it minimizes memory usage, it can increase the time for lookups if clusters form.
"Hash tables are not merely about data storage; they are about efficient retrieval, making complex problems simpler and faster."
Choosing the right collision resolution technique depends on the specific requirements of the application and the expected load on the hash table. Understanding these techniques can be a valuable asset during technical interviews.
Sample Questions on Hash Tables
Familiarity with hash tables leads to a set of common interview questions that test understanding and application. Here are a few examples:
- Explain how a hash table works. What are its operations?
This question assesses knowledge of basic principles, including hashing, key-value pairs, and the significance of a hash function. - What is a hash collision, and how do you handle it?
Candidates are expected to discuss collision concepts and describe various resolution strategies. - Implement a hash table from scratch.
This question evaluates a candidate's coding skills and their grasp of the intricacies involved in hash tables. - Describe a scenario where a hash table is not a suitable data structure.
Understanding limitations is crucial. Candidates should identify contexts where hash tables may fall short, such as in ordered data access.
Mastering hash tables can greatly enhance your problem-solving skills and coding efficiency. Dive deeper into their operations, applications, and variations to prepare effectively for your interviews.
Performance Analysis
Performance analysis is a crucial aspect in the study of data structures, especially when it comes to coding interviews. Understanding how different data structures operate under various conditions helps in making informed choices about which structure to use for a specific problem. Analyzing performance mainly focuses on two key areas: time complexity and space complexity. This analysis provides insights into the efficiency, speed, and resource usage of algorithms tied to data structures.
Time Complexity
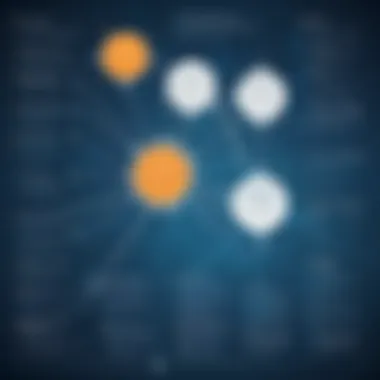
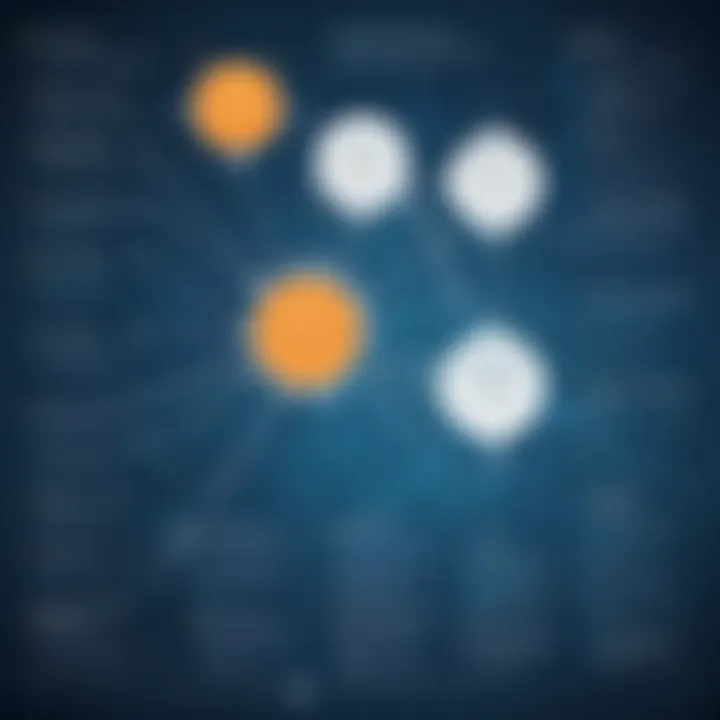
Time complexity measures the amount of time an algorithm takes to complete as a function of the length of the input. It is crucial because coding interviews often demand efficient solutions. Interviewers are less interested in just a working solution; they often seek an optimal one.
The idea is to express this time through Big O notation, which classifies algorithms based on their performance in relation to their input size. Common time complexities include:
- O(1): Constant time - the algorithm takes the same amount of time regardless of input size.
- O(log n): Logarithmic time - the algorithm's time grows logarithmically as the input size increases.
- O(n): Linear time - the time grows linearly with the input size.
- O(n^2): Quadratic time - the performance is proportional to the square of the input size.
Understanding these complexities allows programmers to evaluate algorithms swiftly, deciding which approach is appropriate based on the context of the problem. For instance, using a hash table may allow O(1) time for lookups compared to a list's O(n) time.
Space Complexity
Space complexity refers to the amount of memory an algorithm uses in relation to the input size. It is also expressed in Big O notation. Efficient use of memory is significant in programming, particularly when dealing with large data sets or when running in environments with limited resources.
Key aspects of space complexity include:
- O(1): The algorithm requires a constant amount of space regardless of the input size.
- O(n): The space required scales linearly with the input size.
- O(n^2): The space grows as the square of the input size.
Considerations: When approaching problems, one must balance time complexity and space complexity. For example, a more memory-efficient algorithm might take longer to run, which can be unacceptable in scenarios requiring fast responses.
Balancing time and space complexity is essential for designing efficient algorithms.
Practical Applications of Data Structures
Data structures serve as the backbone of effective programming practices. Understanding their practical applications allows programmers to solve complex problems with efficiency. By using the right data structure, one can optimize performance and manage data effectively.
Data Structures in Real-World Applications
Data structures are pivotal in many real-world applications. They are not just abstract concepts but are utilized in various domains such as web development, game development, and data analysis. For instance, arrays are commonly used for managing lists because of their ability to provide fast access to data. Linked lists offer flexibility in memory utilization, ideal for applications that require frequent insertion and deletion of elements.
Consider how social media platforms, like Facebook, utilize hash tables to quickly retrieve user data. Each user has a unique identifier, and hash tables minimize the time taken to access this information, showcasing the essential role of data structures in enhancing user experience.
In the realm of gaming, trees are often used for representing hierarchical data, such as game maps or object-oriented programming structures. The ability to traverse these trees efficiently results in smooth gameplay and robust performance.
Choosing the Right Data Structure for the Problem
Selecting an appropriate data structure requires a solid understanding of the problem at hand. Factors to consider include:
- Nature of the Data: Analyze how data will be stored and accessed. For instance, if frequent searches are needed, binary search trees or hash tables might be preferred.
- Operations Needed: Identify the kind of operations that will be performed most often, such as insertions, deletions, or lookups. For heavier insertions and deletions, a linked list may be more suitable than an array.
- Memory Constraints: Different data structures have varying memory usage. Linked lists may use more memory compared to arrays but offer more efficient insertions.
- Performance Requirements: Evaluate the performance implications. Understanding time and space complexities helps in making informed choices. For example, if space is a premium and data access speed is crucial, compact structures like hash tables should be favored.
"Efficient algorithm design relies heavily on the correct choice of data structure."
Ultimately, becoming adept at selecting the right data structure comes with experience and practice. It is evident that practical knowledge of data structures can significantly enhance programming proficiency and lead to successful problem solving in interviews and beyond.
By applying these concepts, programmers can confidently approach coding problems, enhancing both their performance in interviews and their overall development skills.
Strategies for Mastering Data Structures
Mastering data structures is crucial for anyone preparing for coding interviews. Developers can expect questions related to various data structures and their applications in problem-solving. The strategies employed can significantly impact the effectiveness of one's study routine and comprehension of the subject. Understanding these techniques helps in grasping how to use different data structures proficiently in real-world scenarios and coding interviews alike.
Important elements of mastering data structures include effective learning resources and hands-on practice techniques. These components ensure that the knowledge gained is not merely theoretical but practical as well. Furthermore, fully grasping these strategies aids candidates in communicating their thought processes effectively during interviews, which is often as crucial as the solution itself.
Learning Resources
When it comes to learning data structures, having the right resources is key. Numerous materials are available that cater to different learning styles. Here are some recommended types of resources:
- Textbooks: Books like "Introduction to Algorithms" by Thomas H. Cormen provide in-depth theoretical insights paired with practical examples.
- Online Courses: Platforms such as Coursera and edX offer structured courses focusing on data structures and algorithms, often designed by universities.
- Video Tutorials: Websites like YouTube host numerous channels dedicated to programming concepts, including data structures. These can be excellent for visual learners.
- Coding Platforms: Sites like LeetCode and HackerRank not only provide coding challenges but also have sections specifically for practicing data structures and algorithms.
- Forums and Communities: Engaging with communities on platforms like Reddit can offer real-world advice, resources, and suggestions that books and courses might not cover. These communities often share insights about trends in technical interviews.
It is beneficial to combine several types of resources. This mix exposes learners to various methods of explaining concepts and enhances understanding.
Hands-On Practice Techniques
Practical experience is essential in mastering data structures. Here are techniques to enhance hands-on learning:
- Solve Problems Daily: Regularly tackle problems that focus on specific data structures to build fluency. Set a goal to solve X number of problems weekly.
- Build Projects: Create small projects that implement various data structures. For instance, developing a simple application like a task organizer can utilize lists, stacks, or queues.
- Use Visualization Tools: Tools such as VisuAlgo can help visualize how different data structures operate and help reinforce learning.
- Pair Programming: Working with a partner can provide fresh perspectives on problem-solving techniques and enhance understanding through discussion.
- Mock Interviews: Practice with a peer, simulating a real interview scenario. This practice not only enhances coding skills but also develops communication abilities.
In sum, mastering data structures requires both theoretical knowledge and practical application. Combining different types of resources with consistent hands-on practice can lead to a thorough comprehension of data structures, which is vital for coding interviews.
Interview Preparation Tips
Effective interview preparation is essential for anyone looking to excel in coding interviews, particularly when data structures are involved. These tips focus on sharpening your skills, enhancing your knowledge base, and building the confidence to tackle challenging questions. Proper preparation goes beyond just reviewing concepts; it encompasses strategy and technique.
Effective Study Plans
Creating a structured study plan is fundamental to mastering data structures in preparation for interviews. A well-organized plan prevents procrastination, promotes efficient learning, and sets clear goals. Start by outlining a timeline that encompasses each major category of data structures.
- Identify Key Areas: Focus on the important data structures such as arrays, linked lists, stacks, queues, trees, graphs, and hash tables. Allocate more time to areas where you feel less confident.
- Set Daily Goals: Aim to cover specific concepts each day. For example, spend one day on arrays, followed by linked lists the next day.
- Incorporate Varied Resources: Use textbooks, online courses, or platforms like Coursera or edX to deepen understanding. Engaging with diverse materials can reinforce concepts in different ways.
- Schedule Regular Reviews: Consistent review sessions help retain information. Revisit previous topics to solidify knowledge. This helps improve recall during interviews.
Structure your study sessions to include:
- Conceptual learning
- Hands-on coding practice
- Reviewing common interview questions
- Mock exercises to simulate real interview conditions
Mock Interviews and Practice
Mock interviews serve as critical practice to simulate the actual interview experience. They can greatly reduce anxiety and improve performance in high-pressure situations. Practicing under timed conditions can replicate the atmosphere of a real interview. Here are some strategies for effective mock interview preparation:
- Pair with a Peer: Find a study buddy to conduct mock interviews. This can provide feedback and allow you to experience diverse question formats.
- Utilize Online Platforms: Websites like Pramp or LeetCode connect you with other programmers for free mock interview sessions. This is a great way to experience varied problems and receive constructive criticism.
- Record Your Sessions: Recording your practice interviews allows you to review your performance analytically. Observe your problem-solving approach, communication skills, and areas needing improvement.
- Focus on Feedback: After each mock interview, assess what went well and what didn’t. Make adjustments to your approach in subsequent sessions based on the critiques received.
Practice isn't just about repetition; it’s about learning from mistakes and refining your approach.
Ultimately, the fusion of a strong study plan with rigorous practice through mock interviews will better prepare you for real coding interviews. Cultivating these skills will help you approach data structures confidently, displaying not only technical ability but also problem-solving proficiency.
Epilogue
A well-rounded grasp of the material enables candidates to not only perform well but also enhances their confidence. Each data structure discussed serves a unique purpose and offers distinct advantages in different scenarios. Candidates need to consider the context of problems they may face during interviews.
Investing time in practicing data structures can lead to improved problem-solving skills. This not only benefits interview performance but can also enhance one’s programming skills in real-world applications.
On the whole, the conclusion serves as a reminder that data structures are not just theoretical constructs; they are practical tools that can define a programmer's success. Understanding these structures and their intricacies can lead to effective coding practices and delivering successful outcomes in coding interviews.
Recap of Key Points
- Data structures are foundational for programming and critical in coding interviews.
- Understanding various types such as arrays, linked lists, stacks, queues, trees, graphs, and hash tables is imperative.
- Performance analysis is crucial to evaluate time complexity and space complexity.
- Practical applications highlight the relevance of data structures in real-world scenarios.
- Efficient study and practice strategies are necessary for mastering these concepts before interviews.
Final Thoughts on Data Structures in Interviews
Ultimately, as technology continues to evolve, the fundamental principles of data structures remain steadfast. They are timeless tools that every programmer should master. The journey may be challenging, but it is integral to a successful programming career.