Mastering Calculator Code: A Developer's Guide
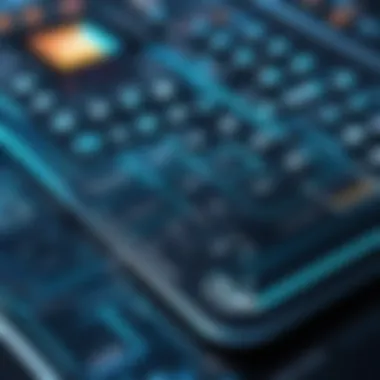
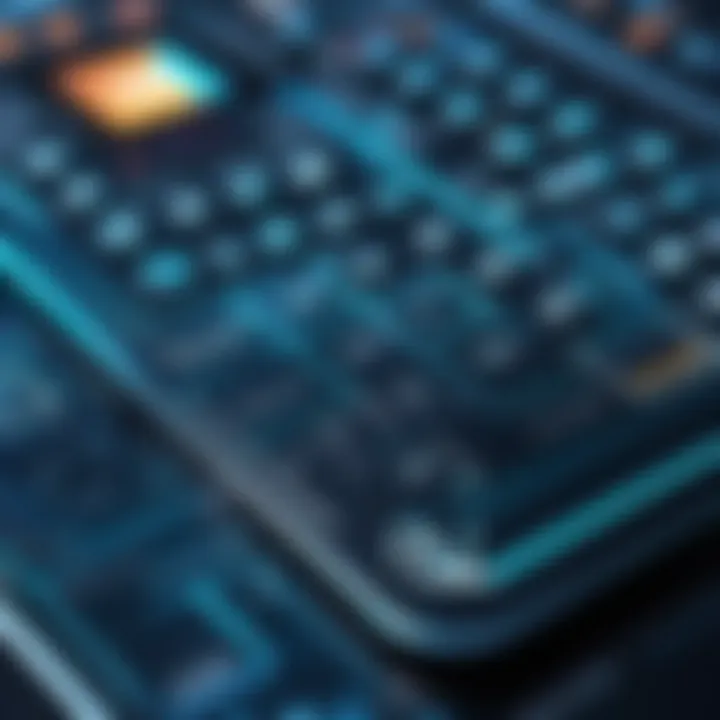
Intro
In the realm of programming, crafting a calculator might seem like a rudimentary task at first glance. Nonetheless, this seemingly simple project serves as a gateway into the intricate world of coding and algorithm design. In fact, calculators encapsulate fundamental principles that extend far beyond mere arithmetic operations. They reflect the essential constructs that form the backbone of programming languages, making them prime candidates for beginners and enthusiasts alike to delve deeper into the nuances of software development.
Importance of Calculator Code
Writing calculator code isn't just about functionality; it's about understanding crucial coding techniques and best practices. One might say that attempting to create a calculator is akin to building a sturdy foundation for a house. If the base is weak, the entire structure could crumble. Hence, grasping the essence of calculator programming equips learners with skills applicable in diverse contexts—be it developing applications or solving complex computational problems.
"A solid grasp of calculator code improves logical thinking and coding capabilities, vital for any programming journey."
Key Topics to Explore
In this guide, readers will uncover a myriad of topics. From history and evolution to hands-on examples, the key points include:
- Introduction to Programming Languages
The evolution of programming languages and their roles in developing calculator functions. - Basic Syntax and Core Concepts
Understanding variables, operators, and control structures that drive calculator applications. - Advanced Topics
Insights into functions, object-oriented programming, and effective exception handling techniques. - Practical Examples
Real-world scenarios that illustrate both simple and complex calculator functionalities. - Resources for Further Learning
Suggestions for books, online courses, and community forums that encourage continuous learning.
The journey into calculator code will unfold layers of learning, making it clear why this topic holds significance for both novices and even seasoned developers looking to reinforce their fundamentals.
Prelude to Calculator Code
Calculator code is more than just a collection of lines in a programming language. It serves as the backbone of computational technologies that we often take for granted in our daily lives. Essentially, calculators translate complex arithmetic into a practical and accessible format. Understanding the purpose and significance of calculator code is crucial for students and budding programmers. This foundational knowledge opens the door to exploring broader concepts in programming, enhancing logic and problem-solving skills.
Understanding the Purpose of Calculator Code
At its core, calculator code is designed to perform mathematical computations. A neatly written algorithm can take user input, process it, and output the result in a way that's both user-friendly and efficient. This purpose may seem straightforward; however, its implications are profound for learners in programming. With a solid grasp of how calculator code operates, one can appreciate underlying principles like flow control, data types, and user interface design.
The capacity to write and manipulate calculator code provides several benefits:
- Familiarity with Basic Operations: Understanding how addition, subtraction, multiplication, and division are handled in code lays the groundwork for more advanced programming tasks.
- Problem-Solving Skills: Dealing with user inputs and handling errors fosters critical thinking, important for any programmer.
- Foundation for Advanced Features: Once the basics are mastered, developers can explore enhancements like scientific functions or graphing, broadening the scope of how calculators can be utilized.
Historical Context of Calculator Development
The story of calculator development is as varied as the devices themselves. From the ancient abacus to modern graphing calculators, each step represents a significant leap in both mathematics and technology. Early calculating devices helped humans tally goods, measure time, and even conduct astronomical observations. With the advent of electronic calculators in the mid-20th century, a new era began, marked by miniaturisation and digital displays.
For learners, it’s effective to grasp how calculators evolved from simple mechanical devices to complex software systems. Notable milestones include:
- The Pascaline, invented by Blaise Pascal in the 17th century, was one of the first mechanical calculators.
- The appearance of electronic calculators in the 1960s, which greatly increased calculation speed and accuracy.
- Finally, the rise of graphing calculators and mobile calculator apps offers expanded functionalities for educational use and professional calculations.
This historical backdrop enriches the understanding of calculator code, grounding it in a context that showcases its relevance and utility throughout human innovation.
Core Components of Calculator Functionality
Understanding the core components of calculator functionality is crucial when developing any calculator application. These are the building blocks that define how users interact with the calculator, and they ensure that the calculator performs the necessary calculations accurately and efficiently. By delving into these components, developers can create a seamless experience that allows users to carry out basic to advanced computations with ease. Focusing on the elements such as arithmetic operations, user input handling, and output display mechanisms enables you to craft a well-functioning application, designed to meet users' needs and expectations.
Basic Arithmetic Operations
Addition
Addition serves as the foundation of all calculations. It allows users to combine two or more numbers to achieve a total. This makes it an essential feature in any calculator. People often find addition straight-forward and intuitive. It’s the first mathematical operation most learn in school and is therefore embedded in our everyday interactions with numbers. The unique feature of addition is its ability to gracefully handle positive and negative numbers alike, making it versatile. One key advantage of addition is that it can be performed in various formats, such as summing up a list of values. But, it may not be as beneficial when dealing with complex mathematical functions that require extreme precision, as cumulative errors can sometimes occur over extended calculations.
Subtraction
On another hand, subtraction is equally pivotal. It represents the removal of one quantity from another, effectively allowing the user to compute the difference. This operation is not only common but also widespread in practical scenarios, such as calculating expenses or determining remaining balance. The distinguishing aspect of subtraction is its straightforward nature, easily understood by most. Its main advantage comes from its direct relationship with addition, as it can be viewed as adding a negative value. However, similar to addition, subtraction can introduce cumulative errors when used repetitively or in sequence with larger datasets, necessitating care and consideration in algorithms.
Multiplication
Multiplication jumps into the fray as a more complex operation. It's essentially a shortcut for repeated addition, which can save time and effort, especially for larger numbers. This operation finds its importance in various fields, from basic budgeting to advanced scientific applications. One notable characteristic of multiplication is its ability to quickly scale numbers, turning small values into large ones with relative ease. Nonetheless, one must be cautious of its drawbacks. For example, multiplying by zero effectively nullifies any number, which can lead to unexpected results if not handled appropriately. Additionally, the order of operations can complicate things when multiple arithmetic operations are involved.
Division
Last but not least, division plays a vital role in the realm of arithmetic. It essentially shares a total into a specific number of parts, which is particularly useful in scenarios like calculating averages or distributing resources evenly. Notably, division is often seen as an extension of subtraction, though it is less intuitively straightforward. The key aspect of division is its potential to yield fractions, which can lead to more precise results in calculations. However, division also comes with its significant considerations. Dividing by zero is not permissible in mathematics, and implementing guards in the code to handle such instances is essential. Thus, while division is powerful, developers must account for such edge cases to ensure robustness in their calculator applications.
User Input Handling
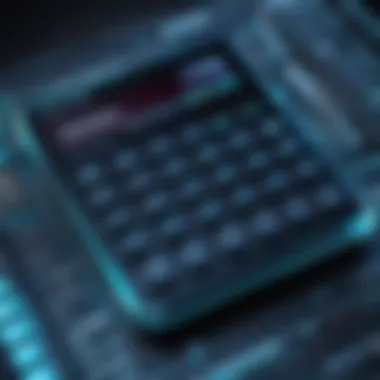
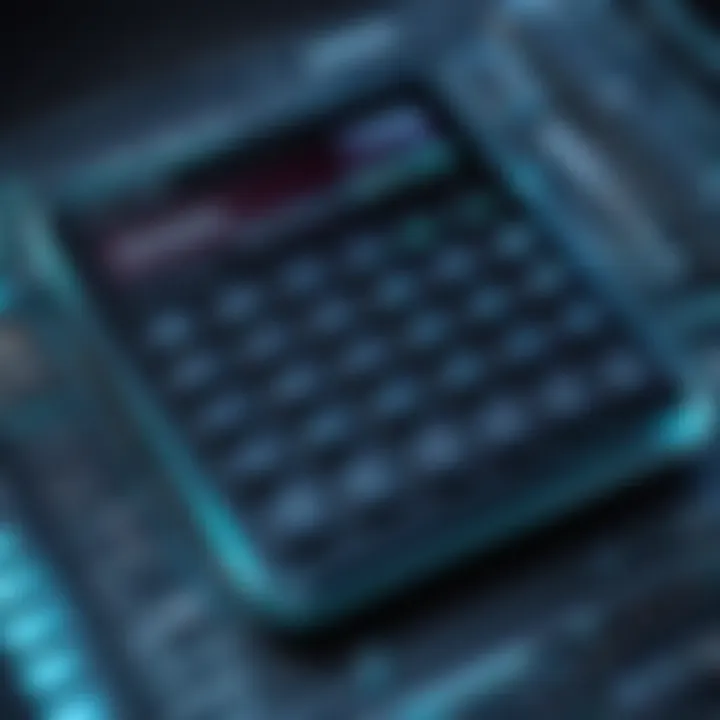
Effective user input handling ensures that a calculator responds correctly to the user’s intentions. It encompasses recognizing user input, validating data for correctness, and managing various input methods, such as keyboard entry or touch interactions. Factors like user experience and accessibility play crucial roles in how well an input mechanism functions. For example, a poorly designed input method might frustrate users, while an intuitive interface can enhance overall usability.
Output Display Mechanisms
Finally, the output display mechanisms bring the results of computations to life. Whether it’s a digital readout or graphical representation, displaying results clearly and understandably is fundamentally important. It’s vital for users to see their inputs alongside the calculated outputs to grasp how conclusion were visualized. Engaging designs, along with clear fonts and intuitive symbols, contribute to an effective display. A well-crafted display will not only reflect accuracy, but also provide feedback, creating a more interactive experience for the user.
The arrangement of these core components into a cohesive calculator application significantly enhances both functionality and user satisfaction. Focused attention to detail across these elements can lead to a robust and efficient calculator design.
Programming Languages and Calculator Code
The world of calculator code is a vast expanse where different programming languages converge, each bringing its unique abilities and considerations to the table. Understanding the interplay between various languages and calculator functionality is essential for both aspiring developers and seasoned programmers looking to broaden their repertoire. This section digs into the significant role that programming languages play in the creation and robustness of calculator applications.
A key component of this topic is recognizing how each language offers specific advantages, potentially impacting what can be developed. Some languages are better suited for rapid prototyping, while others allow for more intricate designs or optimizations. For instance, Java is renowned for its portability, which means a calculator written in Java has the potential to run on any device equipped with the Java Virtual Machine. In contrast, C and C++ might offer more control over system resources but come with complexities that can hinder speedier development.
Let’s explore practical implementations across Java, C, and C++. Each section will reveal how unique characteristics of these languages contribute to the overall effectiveness of calculator applications.
Implementing Calculator Code in Java
Java has earned a reputation as a solid choice for developing applications, including calculators. Its platform independence, robust libraries, and OOP principles make it a favored option among many developers.
Setting Up the Development Environment
Setting up the development environment forms the backbone of any programming task, including calculator development in Java. Choosing Java means opting for a structured approach where Java Development Kit (JDK) must be installed, alongside an Integrated Development Environment (IDE) like Eclipse or IntelliJ IDEA.
A noticeable feature of setting up such an environment is the ease it offers for navigation and editing. The IDE can provide automatic code completion and hints, helping developers avoid common pitfalls and syntax errors. However, it can also lead to over-reliance on the IDE, which might affect understanding the core Java language itself. Nevertheless, this structured environment allows for a smoother, more efficient coding experience.
Sample Code Implementation
Illustrating how to implement a sample calculator program in Java brings clarity to its application. A simple implementation could include methods for addition, subtraction, multiplication, and division, neatly encapsulated in a class.
This kind of sample code acts as a solid foundation. It showcases principles of modularity, making it easier to expand functionalities. Yet, simplistic implementations run the risk of lacking proper error handling, which can be a common oversight when developing calculators.
Troubleshooting Common Java Issues
No code is perfect, and troubleshooting is as pivotal as the coding itself. Common issues arise, like null pointer exceptions or ArrayIndexOutOfBounds. Learning how to efficiently debug these errors contributes immensely to the overall development process.
The key characteristic here is that Java’s robust community support and documentation make it easier to find solutions or alternative approaches to common coding issues. Still, debugging can be time-consuming, particularly for newcomers who may struggle with interpreting stack traces or error messages, making effective debugging strategies an essential learning curve.
Creating a Calculator in
Developing calculators in C requires an in-depth understanding of basic programming concepts, especially regarding memory management and code efficiency.
Code Structure and Design
The structure of the code in C significantly affects performance and readability. A well-structured program often follows a top-down approach, where you define the main functionality before delving into specifics.
C is lauded for its efficiency, which is crucial in performance-sensitive applications like calculators. However, a complex structure can complicate readability, translating into a steeper learning curve. Developers must strike a balance between power and simplicity.
Handling Edge Cases in
Handling edge cases in C, such as dividing by zero or overflow scenarios, ensures that the calculator behaves predictably under unusual circumstances. This consideration enhances user experience by preventing crashes or erroneous outputs.
C requires explicit checks for these cases, which might seem laborious but is essential for robust applications. While this explicitness allows for precision, it also demands diligence from the programmer to cover every conceivable scenario.
Best Practices for Coding
As programmers work on building calculators in C, adopting best practices can dramatically enhance code quality. Practices such as maintaining clear comments, using meaningful variable names, and breaking functions into smaller, manageable parts stand out.
These approaches prevent the infamous “spaghetti code” and make future modifications simpler. Yet, there ’s a tendency among developers to underprioritize comments, assuming code will be clear later on. This perspective often leads to confusion when revisiting code after some time.
Building a Calculator with ++
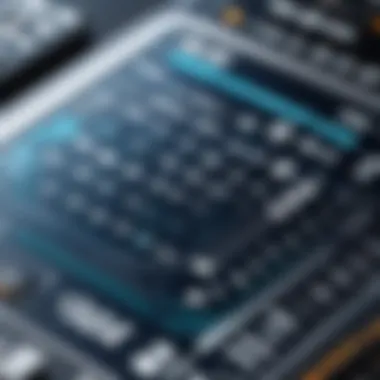
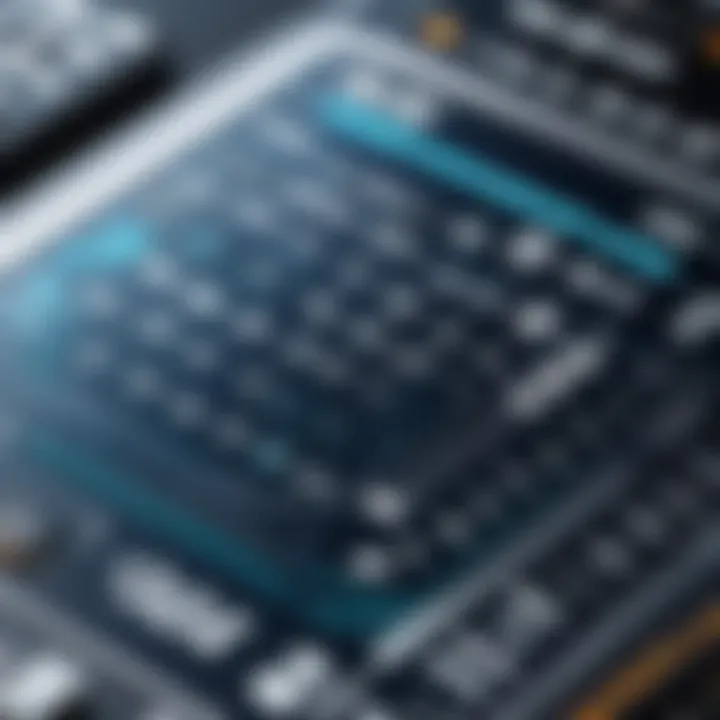
C++ amplifies the experience of programming a calculator with its object-oriented features. It introduces more advanced paradigms, enhancing organizational structures.
Utilizing Classes and Objects
With C++, leveraging classes and objects leads to a more organized code structure. By encapsulating functionality within classes, developers can build more flexible and maintainable calculators.
The key benefit lies in code reuse; classes can be extended to create new types of calculators without starting from scratch. However, this might encourage a less straightforward coding style for those who are more accustomed to procedural programming.
Memory Management Considerations
Memory management in C++ is a significant consideration. The language allows for dynamic memory allocation, which can enhance performance. However, it also places the onus on developers to manage memory responsibly, introducing risks such as memory leaks if not handled properly.
This duality of power and responsibility is a compelling aspect of C++. It fosters deep learning but can discourage newcomers who might be more comfortable with managed memory environments like Java.
Creating User-Friendly Interfaces
Finally, a user-friendly interface is the feather in the cap for any calculator app. In C++, libraries such as Qt or SFML make it feasible to design attractive GUIs.
The importance of good user experience cannot be overstated, as it directly impacts usability. However, creating these interfaces may come with additional complexity and a steeper learning curve compared to console-based applications. Developers must be ready to navigate these challenges to realize aesthetically pleasing and functional calculators.
Algorithm Design for Calculator Code
When it comes to creating a calculator that performs reliably in various situations, the design of the algorithms is crucial. Algorithm design lays the groundwork for not just how the calculator processes numbers and symbols, but also how it expects to handle complex scenarios like division by zero or input errors. A well-structured algorithm enhances user experience, making a calculator not only functional but also robust against erroneous inputs. This section delves into the key elements of algorithm design and demonstrates how they contribute to the overall effectiveness of calculator code.
"An algorithm is like a recipe; it tells the program how to mix the ingredients to achieve the desired dish."
The crux of algorithm design for calculator code lies in its ability to systematically break down processes into manageable steps. By doing so, errors can be minimized and efficiency can be maximized.
Flowcharts and Pseudocode
One of the best ways to convey the logic behind an algorithm is through flowcharts and pseudocode. Flowcharts represent the workflow visually, enabling programmers to grasp the sequence of operations at a glance. Each shape within a flowchart has a specific meaning:
- Oval: Start and end points.
- Rectangle: Instructions or operations.
- Diamond: Decision points that guide the flow based on conditions.
Pseudocode, on the other hand, is a method of coding that uses plain language to outline steps without getting bogged down by the syntax of a specific programming language. This approach allows you to focus on logic rather than specifics. Here is a simple pseudocode example for a basic calculator:
By laying out your algorithm in this manner, you can quickly identify potential pitfalls and improve upon them before delving into actual code.
Recursive vs. Iterative Approaches
When crafting the algorithms that drive calculator functionality, the choice between recursion and iteration often comes into play. Both methods have their strengths and weaknesses that are worth considering in the context of calculator design.
- Recursive Approaches: This method involves a function calling itself to solve a problem, breaking it down into smaller, more manageable sub-problems. Recursion can lend itself well to certain calculations, such as handling complex mathematical functions like factorials and Fibonacci sequences. However, you must be wary of stack overflow errors that can occur if too many recursive calls happen.
- Iterative Approaches: In contrast, iteration employs a loop to repeat a set of instructions until a condition is met. Iterative algorithms are generally more memory-efficient since they avoid the overhead of numerous function calls. This approach is often preferable for basic operations like addition and subtraction because it tends to be simpler and more straightforward.
Ultimately, the choice between recursion and iteration often boils down to the problem at hand and the specific requirements of the calculator you're designing. Thoughtful consideration of these elements can improve the robustness and efficiency of your calculator code.
Testing and Debugging Calculator Code
In the landscape of coding, the importance of testing and debugging calculator code cannot be overstated. These practices act as the backbone of creating robust applications that serve not only their intended purpose but also maintain a level of reliability that developers strive for. When calculators, whether simple or complex, are designed, they must function correctly under various conditions. This is where rigorous testing and efficient debugging come into play. Ensuring precision in mathematical computations and smooth user interactions is paramount.
Understanding how to effectively test and debug allows programmers to identify and rectify bugs that could potentially derail the calculator's intended functionality. In the early stages, it helps in building a solid foundation; later on, it adds refinement. By adhering to systematic testing methodologies, developers can significantly reduce the risk of miscalculations or system failures. This means better performance in real-time applications, ultimately giving users a more seamless experience.
Unit Testing Methodologies
Unit testing is a fundamental practice that ensures individual components of the calculator code are functioning as expected. It focuses on isolating each piece of code and verifying its correctness with various test cases. For instance, if you're implementing a function that adds two numbers, you can create a series of tests that check not only standard addition but also edge cases, like large numbers or unexpected types.
Some methodologies worth considering include:
- Test-Driven Development (TDD): This methodology encourages writing tests before the actual code. It ensures that every code functionality is validated from the very beginning. Although it requires more upfront work, it often leads to cleaner and better-structured code.
- Behavior-Driven Development (BDD): This approach focuses on the behavior of the application, using natural language to describe the workings of the code. BDD often leads to clearer specifications, making it easier for non-developers on a team to understand the tests.
Consider utilizing testing frameworks like JUnit for Java, or Google Test for C++. These tools streamline the process, allowing for systematic testing that can be integrated into your development workflow. By embracing unit testing, developers can catch errors long before they reach the end-user, thus fostering trust and reliability in their code.
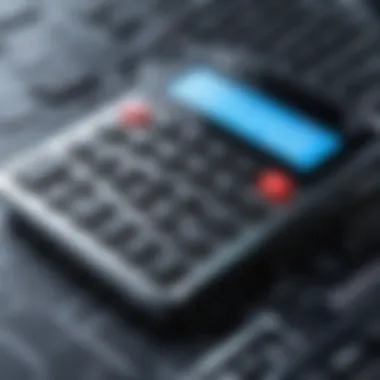
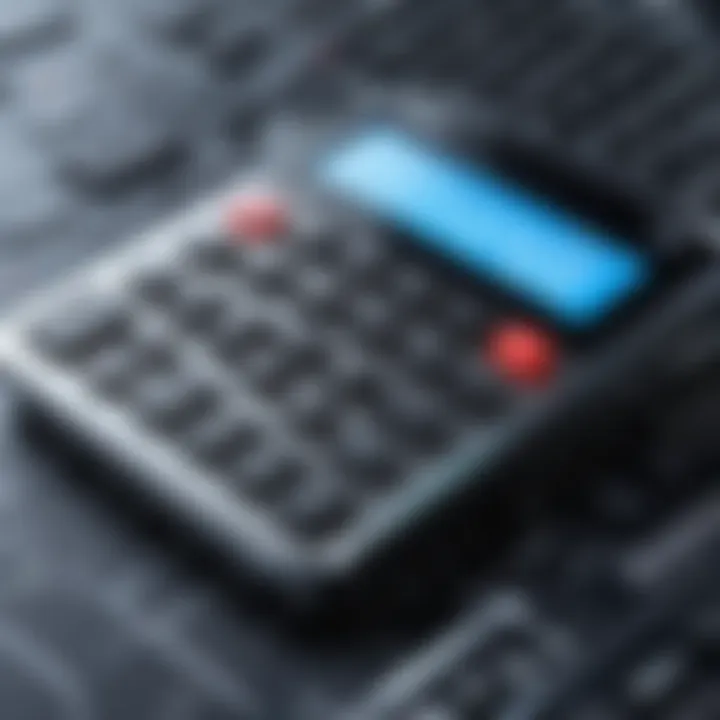
Common Bugs and Troubleshooting
The journey of writing calculator code often leads to encountering common bugs. These pitfalls are not exclusive to new coders; even seasoned programmers can trip over them. Understanding these issues can save time and improve the overall quality of the software.
Here are a few frequent bugs in calculator code:
- Floating Point Errors: One notorious issue is due to the nature of floating point representation in computers, leading to precision errors in decimal calculations. This can yield surprisingly incorrect results.
- Division by Zero: Attempting to divide by zero can cause runtime errors. Implementing error handling to manage this gracefully is crucial.
- Operator Precedence Misunderstandings: If the code doesn’t correctly prioritize operations, it can produce wholly unexpected results.
To troubleshoot these issues effectively, one can take a systematic approach:
- Log Outputs: Adding logging statements can help in tracking down where things go awry. Understanding the flow of data through the code can pinpoint the trouble spots.
- Use Debuggers: Tools like Visual Studio's debugger or GDB for C++ allow programmers to step through the code line by line, making it easier to identify where logic may have failed.
- Peer Reviews: Sometimes, a fresh pair of eyes can catch things you might miss. Engaging peers for code reviews provides not only validation but also new perspectives on potential issues.
"An ounce of prevention is worth a pound of cure." This saying holds especially true in programming. By prioritizing testing and debugging from the get-go, developers can avoid mountains of headaches down the line.
Enhancing Calculator Functionality
In today’s fast-evolving tech landscape, enhancing calculator functionality transcends mere arithmetic. It’s about pushing the boundaries of what calculators can do, transforming them into robust tools for students, engineers, and everyday users. By integrating advanced features, programmers can ensure calculators not only perform basic functions but also solve complex problems, making their applications invaluable across multiple domains.
Enhancing functionality leads to several benefits, including improved user experience and increased utility. For instance, students using calculators with scientific and graphing capabilities can tackle higher-level mathematics effectively. Therefore, when considering enhancements, a programmer should evaluate user needs and the specific context in which the calculator will be used.
Adding Advanced Features
Scientific Calculations
One of the most notable features that enhance calculators is their capacity for scientific calculations. This aspect allows users to perform operations such as trigonometric functions, logarithms, and exponents. The key characteristic of scientific calculators is their ability to handle a wide variety of mathematical operations which direct support the needs of students and professionals alike.
Scientific calculations stand out as a popular choice in this article, primarily because they cater to those delving into physics, engineering, and advanced mathematics. A unique feature of scientific calculators is their storage functions, enabling users to save interim values and reuse them in subsequent calculations.
However, these advanced capabilities may come with disadvantages, such as the potential for user confusion. Newcomers might feel overwhelmed by the plethora of functions available, leading to errors in calculations. Yet, once navigated properly, the advantages far outweigh these initial challenges.
Graphing Capabilities
Another transformative addition to calculator functionality is graphing capabilities. This feature allows users to visualize equations, making it easier to grasp the behavior of mathematical functions. The beauty of graphing calculators lies in their ability to represent complex relationships in an intuitive manner.
Graphing capabilities are considered a beneficial enhancement in our article, seeing that they empower students and researchers to analyze data effectively. The unique features of these calculators include dynamic graphing options where users can modify equations in real-time and see instantaneous updates on the graph display.
Nevertheless, while graphing enhances usability, it also has its downsides. One major drawback is the steeper learning curve associated with mastering graphing functionalities. Users might initially struggle to comprehend how to manipulate the graphs or interpret the outputs effectively. However, persistence will lead to proficiency, proving that the investment in time pays dividends in the long run.
"An effective calculator goes beyond simple computations; it’s a gateway to understanding complex concepts."
In essence, enhancing calculator functionality through features like scientific calculations and graphing capabilities allows users to experience mathematics and science in broader and deeper ways. The focus on practical application enables a smarter approach to learning and problem-solving, ultimately enriching users' understanding of subjects often deemed challenging.
The End and Further Resources
As we wrap up this comprehensive guide on calculator code, it's crucial to recognize the importance of the concepts we've covered. The world of programming is vast and intricate, and calculator code is a prime example of how fundamental principles can translate into practical applications. Understanding how to develop a calculator isn't just about the code itself; it's about grasping the logic and reasoning that underpins programming as a whole.
In this article, we explored several essential elements, ranging from core functionalities to testing and debugging practices. The tools and techniques discussed provide a robust framework for coding calculators, which can subsequently be adapted to more complex applications. Moreover, the journey of programming is continuous, making it vital to seek out further education and resources to refine and expand your skills.
Recap of Key Concepts
In our exploration, we delved into various sections crucial to calculator coding:
- Core Components: We outlined fundamental arithmetic operations and how user inputs are processed. This understanding forms the backbone of any functional calculator.
- Algorithm Design: Concepts such as flowcharts and the importance of choosing between recursion and iteration were highlighted, essential for efficient coding.
- Testing: We examined effective unit testing methodologies and common issues that may arise, emphasizing that good testing practices can save time and resources down the line.
Revisiting these topics helps solidify the foundations laid in this guide and assists in transitioning to more advanced programming tasks.
Recommended Reading and Tutorials
To further your journey in programming, exploring additional literature and tools can significantly enhance your learning curve. Here are two resources that stand out:
Books on Advanced Programming
Books focusing on advanced programming concepts, such as "Clean Code: A Handbook of Agile Software Craftsmanship" by Robert C. Martin, are instrumental in building a deeper understanding of coding practices. They offer insights into writing maintainable and efficient code, a key characteristic for any aspiring developer. The practical examples and real-world scenarios enhance learning by showing how to apply abstract concepts effectively. While some might find such reads dense, their unique features, like clear guidelines on code refactoring and design patterns, make them a favored choice for those looking to up their programming game.
Online Coding Platforms
Platforms such as Codecademy and LeetCode have gained popularity for their interactive approach to learning. They offer hands-on practice that can be invaluable for grasping concepts discussed in this guide. These platforms often provide instant feedback, allowing learners to identify and rectify mistakes in real-time. However, one drawback can be the necessity of consistent internet access and potential subscription fees. Still, the advantages in adapting to varied learning styles make them a worthy consideration for anyone looking to enhance their programming skills.
Continuing education enhances one's programming understanding and opens new doors in career possibilities. Staying curious, practicing often, and making use of these resources will propel you toward coding proficiency.