Mastering C Programming: A Complete Learning Journey
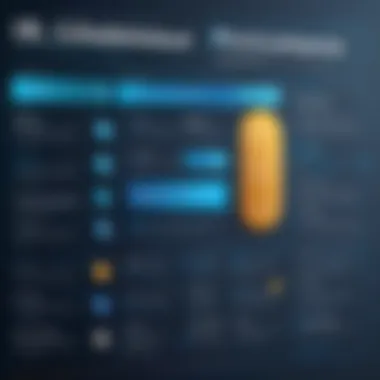
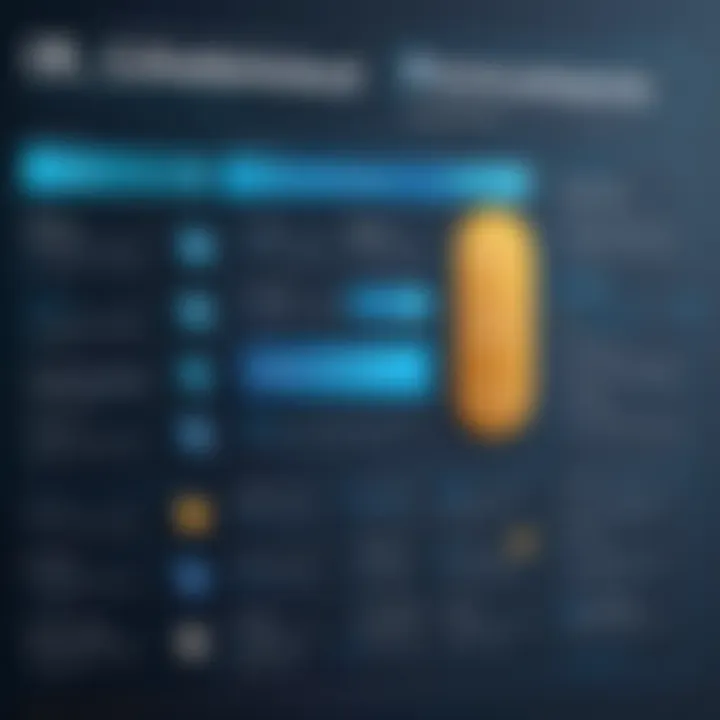
Intro
Programming is an essential skill in today’s technology-driven world. This guide aims to provide a comprehensive overview of C programming, a language established in the early 1970s that has maintained its relevance and utility across various domains of software development. Originating in the development of Unix operating systems, C has become a foundational language that underpins many modern programming languages, making it crucial for both beginners and intermediate programmers to grasp its principles and applications.
History and Background
C programming has a rich history that dates back to its creation by Dennis Ritchie at Bell Labs. Initially designed as a successor to B, it evolved into a powerful language used for system programming. The language’s close relationship with assembly language allows developers greater control over hardware, which is one reason for its widespread adoption in systems programming, embedded systems, and high-performance applications. The introduction of the C Standard Library standardized the functionality across different platforms, allowing programs written in C to run on various hardware with minimal changes.
Features and Uses
C is characterized by its efficiency, speed, and portability. It’s a general-purpose language, primarily used for:
- Operating system development (e.g., Linux)
- Embedded systems (e.g., appliances, automotive systems)
- Software development (compilers, interpreters)
- Game development and graphics programming
Its simplicity enables programmers to implement complex algorithms and data structures effectively, making it a go-to choice for many software engineers.
Popularity and Scope
Despite the introduction of many other programming languages, C continues to hold a prominent position. Its ease of learning and powerful capabilities contribute to its ongoing popularity among computer science students and professionals alike. According to programming surveys and various educational platforms, C remains one of the top languages taught in academic environments. This guide will delve into C programming intricacies, ensuring that learners can harness its capabilities effectively.
"C programming is not just a language; it's a foundation upon which many advanced skills can be built."
By the end of this journey through C programming, readers will be well-equipped to tackle both the fundamental and advanced features of the language.
Preamble to Programming
In the realm of programming languages, few hold as pivotal a role as C. Its influence can be felt across modern computing, powering systems and applications that make our digital lives possible. This introduction serves to lay foundational knowledge for anyone stepping into the world of C programming, showcasing its importance, history, and broad applicability.
History of Language
The C programming language first saw the light of day in the early 1970s, developed by Dennis Ritchie at Bell Labs. It emerged from the need to create a more efficient language for system programming, eventually leading to the development of the Unix operating system. The name 'C' came from its predecessor, B, which was itself a simplified version of an earlier language called BCPL.
C was distinguished by its combination of low-level access to memory and high-level abstractions, striking a balance that many languages often fail to achieve. By the late 1970s, it had gained traction, largely due to Brian Kernighan and Ritchie’s book "The C Programming Language," which became an essential manual for aspiring programmers. Over decades, various standards emerged, with ANSI C (or C89) being a significant milestone, ensuring wider compatibility across sectors.
Importance of in Modern Programming
C's relevance in the programming ecosystem can’t be overstated. Many modern languages, such as C++, Java, and Python, are influenced by its syntax and principles. C's ability to manipulate hardware-level resources while maintaining readability makes it ideal for system-level programming. This characteristic often leads programmers to prefer C for operating systems or embedded systems, where performance is critical.
Furthermore, C's continuous adaptation and usage in education have made it a foundational language in computer science. Many computer science courses begin with C, as understanding its underlying concepts translates well into learning newer languages. With its efficiency and flexibility, C lays down the groundwork for good programming habits, emphasizing structured and logical thinking.
"C is a powerful language that allows you to see what's going on under the hood, making it a favorite for systems programmers and hardware developers alike."
Applications of Language
C’s versatility translates into a wide array of applications across different domains. Here are a few notable ones:
- Operating Systems: The Unix operating system and many of its derivatives are written in C, showcasing its strengths in system-level programming.
- Embedded Systems: Devices ranging from microwaves to medical equipment often use C due to its efficiency and control over hardware.
- Game Development: Many game engines leverage C for performance-critical components, especially in graphics rendering.
- High-Performance Computing: C is frequently seen in scientific computing, where performance is essential for handling large datasets quickly.
Understanding C’s historical context, its critical standing in modern programming, and its diverse applications offers a comprehensive view of why this language is not just relevant but foundational to the evolution of computer programming as we know it today.
Setting Up the Programming Environment
When venturing into the realm of C programming, it’s essential to create a solid foundation. This section delves into Setting Up the C Programming Environment, which is critical for both novices and seasoned programmers. A well-organized setup can dramatically influence your coding experience, making learning smoother and more efficient. Without the right tools, even the best coding ideas can fall flat. The environment you create enables not just the coding, but also the debugging and testing processes, too.
Moreover, understanding how to set things up correctly can save you time and headaches down the line. Whether you are just starting or looking to refine your skills, knowing how to configure your programming environment can have far-reaching implications on your productivity.
Choosing the Right Compiler
The first step in establishing a conducive coding environment starts with selecting the right compiler. A compiler translates your C code into machine language so that it can be executed by the computer. With several options available, making the right choice is crucial. Popular compilers like GCC (GNU Compiler Collection) and Turbo C are often recommended, but the choice may depend on specific needs and platforms.
Factors to consider include:
- Compatibility: Ensure the compiler is compatible with your operating system, whether it's Windows, macOS, or Linux.
- Ease of Use: Some compilers have a more user-friendly interface or documentation that may simplify the learning curve.
- Community Support: A compiler with a strong community readily available for support can be invaluable, especially when you encounter issues.
- Features: Look for features such as optimization capabilities, debugging options, and error reporting that enhance your coding experience.
In the end, the goal is to find a compiler that fits your workflow. GCC is a solid choice for many due to its flexibility, while other options like Clang are also growing in popularity. Choosing the right compiler can mean the difference between a smooth coding journey and one fraught with frustration.
Installing Integrated Development Environments (IDEs)
After selecting a compiler, you may want to consider using an Integrated Development Environment (IDE). An IDE combines a code editor, compiler, and debugger into one cohesive package, streamlining the programming process. It’s like having a Swiss Army knife, where all your essential tools are bundled together, designed to improve efficiency and ease of use.
There are several noteworthy IDEs for C programming:
- Code::Blocks: Renowned for its simplicity and versatility, making it great for beginners.
- Dev-C++: Lightweight and straightforward, perfect for smaller projects.
- Eclipse CDT: A more advanced option that caters to complex projects and offers extensive features.
To install an IDE, follow these general steps:
- Download the installer from the official website of the chosen IDE.
- Run the installer and follow the prompts, making sure to configure the installation according to your preferences.
- Once installed, configure the IDE to use the compiler you have chosen by adjusting the settings in the options menu.
- Finally, test your setup by creating a simple C program to ensure everything works smoothly.
When done properly, this will create a comfortable workspace where coding is seamless, leading to productive sessions. Remember, the right tools make all the difference in coding – so take the time to set your environment up with care!
In summary, setting up your C programming environment is a key first step in your programming journey. Take the time to carefully choose your tools, and they will pay off in improved productivity and ease of learning.
Basic Syntax and Structure
Understanding the basic syntax and structure of C programming is paramount for anyone embarking on the journey of coding. This foundational knowledge serves as the bedrock for all subsequent learning, paving the way for the effective implementation of more complex concepts. A grasp of how C operates essentially shapes one’s ability to write efficient and clear code. When learners dive into C, they quickly find that its syntax is relatively straightforward compared to some modern languages. The predictability helps beginners build confidence as they craft their first code snippets.
Understanding Program Structure
Every C program adheres to a schematic that remains consistent across various applications. At its core, a C program consists of functions, with the function standing front and center, much like a star performer at a concert. This function is where the program begins execution. The minimal structure of a C program can look something like this:
Each function is a block of code designed to accomplish a specific task. The function's name, like , is essential for referencing it within your program. Therefore, naming your functions carefully can enhance code readability.
Apart from functions, global variables and user-defined functions may also feature in a C program. Knowing how these components interact is vital for writing coherent C code.
Data Types and Variables
Data types are the backbone of any programming language. In C, they define the kind of data a variable can hold, influencing the operations you can perform on it. C offers several fundamental data types:
- int: for integers, capable of representing whole numbers.
- float: for floating-point numbers, useful for decimals.
- char: for single characters, like 'a' or 'B'.
- double: for larger floating-point numbers, providing higher precision than float.
Declaring a variable must align with its data type, as shown here:
Learning how to declare variables properly is like learning to set the table before a meal—you need the right arrangement before anything substantial can happen.
Operators in Language
Operators form the tools and utensils of any programmer. They’re directed towards performing operations on variables and values. C provides various types of operators:
- Arithmetic Operators: Used for basic calculations (e.g., +, -, *, /).
- Relational Operators: For comparisons (e.g., ==, !=, >, ).
- Logical Operators: Used for logical operations (e.g., &&, ||).
These operators can manipulate data types and variables to produce desired outcomes. For example, combining variables using arithmetic operators allows you to perform calculations seamlessly. Here’s a simple illustration in code:
This snippet sums the integers and stores the result in the variable .
As with any tool, learning to wield these operators effectively can elevate your coding from basic to intermediate levels. A solid understanding of these elements is crucial—like learning to read a map before setting a course for exploration.
"The journey of a thousand miles begins with a single step." – Lao Tzu
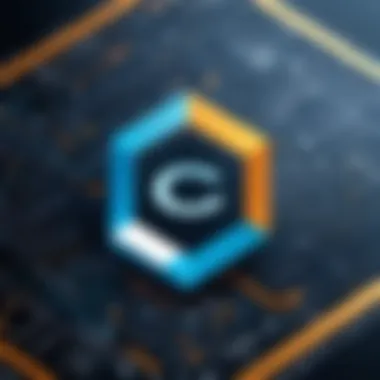
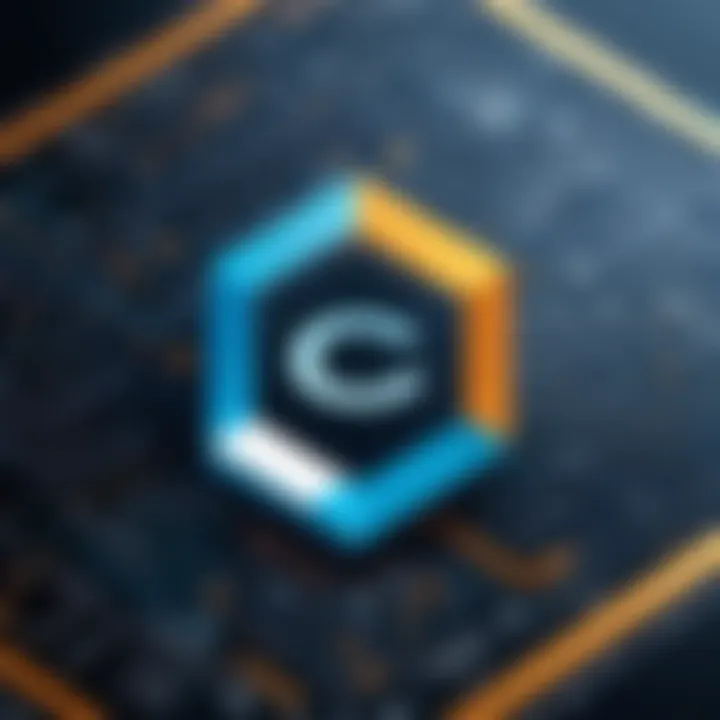
Harnessing the power of syntax, data types, and operators equips you with the knowledge to navigate through the expansive landscape of C programming, laying the groundwork for advanced mastery.
Control Structures in
Control structures are the backbone of any programming language, allowing developers to execute code in a precise and controlled manner. In C programming, they function as gates through which decisions are made and actions taken based on certain conditions. This section will discuss the pivotal role control structures play in managing the flow of a C program.
Control structures can be broadly categorized into two types: conditional statements and loops. Both types serve unique purposes that significantly enhance the functionality of a program. By mastering these constructs, programmers gain the ability to write efficient, readable, and maintainable code.
Conditional Statements
Conditional statements allow programmers to dictate which blocks of code to execute based on specific criteria. At the heart of C programming are the , , and statements, essential for branching logic.
For example, consider a situation where a program needs to determine if a number is even or odd:
In this code snippet, the program checks if the number is divisible by 2; if the condition is true, it executes one line of code; otherwise, it falls into the block.
Benefits of Using Conditional Statements:
- Improved Decision-Making: This allows for complex decision-making processes in applications, enabling dynamic program responses based on user input.
- Enhanced Readability: They facilitate a clear understanding of the program logic, making it easier for others to follow the reasoning behind specific outcomes.
Loops: For, While, and Do-While
Loops are another critical aspect of control structures that facilitate repeated execution of a block of code. In C, the primary types of loops are , , and , each serving its own purpose.
- For Loop: This is ideal for situations where the number of iterations is known beforehand. For example, if you want to print numbers from 1 to 10, the loop is straightforward:
- While Loop: Useful when the number or condition of iterations isn’t predetermined. Here’s an example that continues until a user inputs zero:
- Do-While Loop: This structure ensures that the code block runs at least once before checking the condition. Here’s how it looks:
Key Insight: Understanding loops is crucial for optimizing repetitive tasks. Using them efficiently can reduce code redundancy and streamline processes, making programs faster and more responsive.
In summary, control structures are vital in C programming. They provide the necessary scaffolding to control the flow of execution and enable developers to craft sophisticated programs that respond intelligently to varying conditions. Through effective use of conditional statements and loops, programmers can write code that not only executes efficiently but also remains comprehensible for future development.
Functions in Programming
Functions are a cornerstone of C programming, serving as building blocks that help organize and compartmentalize code tasks. Having a well-structured approach through functions not only leads to cleaner code but also makes reusing and maintaining it significantly easier. When you draft a C program, you will likely encounter scenarios where repetitive tasks need streamlined execution. This is where functions shine, encapsulating specific operations and providing a simple interface for other parts of your program to engage with them.
In essence, functions empower programmers to break down a large task into manageable parts, making them indispensable for enhancing clarity and modularity. Furthermore, leveraging functions can improve code readability and ease debugging, as you can focus on individual components rather than getting lost in a sea of procedural logic. Let's explore this concept further by delving into three key aspects of functions: defining and calling them, managing parameters and return values, and understanding recursion.
Defining and Calling Functions
Defining a function in C is quite straightforward. At its basic level, a function comprises a heading that specifies its return type, name, and parameters (if any), followed by a block of code enclosed in braces that outlines what the function will execute.
Here’s a simple example:
In this code snippet, we define a function named that takes two integers as parameters and returns their sum. However, defining a function is just half of the story. To make it work, you must call the function somewhere in your main program.
For instance, calling the function could look like this:
By doing this, you can pass values to your function and ultimately display the result. This abstraction not only keeps things neat but allows for easy updates; altering the function itself means every call will automatically utilize the new logic.
Function Parameters and Return Values
Function parameters and return values are essential for communication within functions. Parameters act as placeholders for actual values, allowing functions to operate on input supplied during the call. The return value, on the other hand, provides the output of the function.
For example, consider a function that calculates the area of a rectangle:
Here, and are parameters of type , and the function returns a value that represents the calculated area. By utilizing function parameters, you can generalize the function's ability to handle different length and width values, adding quite a bit of versatility.
Functions can enhance code quality and reduce errors considerably by ensuring that data types remain consistent across operations.
Building upon this, return values dictate how data can be passed back from a function to the calling code. A function need not return a value at all; you might see the return type as when no return is necessary. Still, when utilizing return values, ensure the type aligns correctly with the expected output in order to prevent any data type conflicts.
Recursive Functions
Recursion is a unique concept in C programming where a function calls itself to solve a problem. This can be particularly handy for problems that can be broken down into smaller, more manageable subproblems, such as calculating factorials or navigating tree structures. The key to successful recursion lies in understanding the base case – the simple case that can be resolved without further recursion.
For instance, here’s an example of a factorial function defined using recursion:
In this code snippet, the function multiplies by the factorial of until it reaches 1. You may notice that with each iteration, you're converging upon a base case, eventually bringing the recursive calls to an end.
While fun and elegant in some scenarios, recursive functions can also lead to increased memory usage and stack overflow errors if not managed correctly. Hence, it is crucial to ensure that your base case is well defined and that recursion does not exceed reasonable limits.
In closing, mastering functions in C programming is fundamental to not just enhance your coding skills but also better comprehend how to create efficient algorithms. Functions simplify complexity, improve reusability, and pave the way for clean, maintainable code.
Working with Arrays and Pointers
When tackling C programming, understanding the relationship between arrays and pointers serves as a cornerstone for maximizing memory management and data structuring capabilities. Both constructs are not just vital from a syntactical standpoint but also essential for writing efficient, high-performing code. They might seem a bit daunting at first, but grasping these concepts can open many doors in software development. Let's dig deeper into the details.
Array Declaration and Initialization
Declaring and initializing arrays in C is straightforward, but it carries significant implications for how we manipulate and access data. An array is a collection of variables of the same type, and they are accessed using an index.
To declare an array, you specify the type of elements it will hold followed by square brackets. For example:
After declaring, you might want to initialize it. This can be done upon declaration:
Alternatively, you can declare an array without initial values:
In this case, the elements will hold garbage values until explicitly assigned. It's always a wise move to initialize your arrays to avoid unexpected behavior. The size of an array must be known at compile time, meaning it should always be a constant expression.
Understanding Pointers
Pointers in C are often described as variables that hold the address of another variable. This means you can directly interact with the memory of your application, which is both powerful and risky. Pointers provide a way to dynamically manage memory and facilitate efficient array manipulations.
To declare a pointer, you start with the type of the variable it will point to, followed by an asterisk. Here’s how you can define a pointer to an integer:
Once you have a pointer, you can assign it the address of an integer variable. For example:
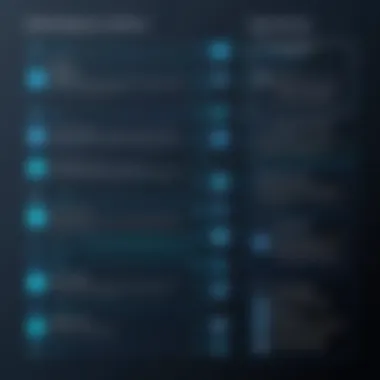
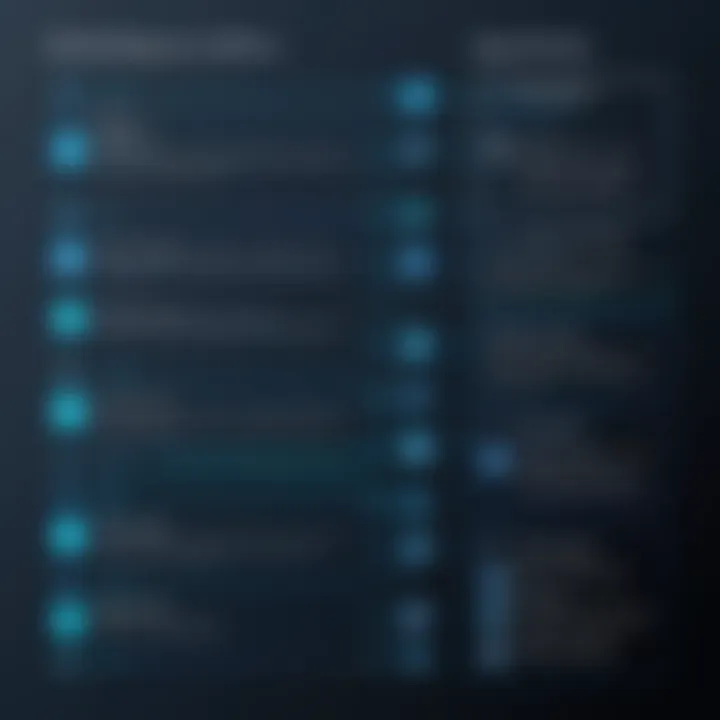
Using pointers also allows you to modify the value of the variable they point to:
Being able to overwrite data this way can lead to powerful programming patterns, but it also poses risks including segmentation faults if mismanaged.
Pointer Arithmetic
Pointer arithmetic enables programmers to navigate and manipulate memory locations in a powerful yet concise manner. This means you can perform operations like incrementing a pointer to traverse an array. When you add to a pointer, it moves by the size of the type it points to—not merely by bytes.
For example, if you have:
If you increment as follows:
This capability allows for a compact way of processing array data through iteration. A practical approach could be iterating through an array with a pointer:
This prints the elements of the array without using array indexing. This compact notation is not just aesthetically pleasing but can also be a tad faster in some contexts.
As you dive into arrays and pointers, remember they are intertwined deeply, representing a fundamental aspect of memory manipulation in C.
"Understanding pointers and arrays is critical for diving deeper into C programming and helps cultivate better programming practices."
Incorporate solid practices, such as careful pointer management and diligent memory allocation, to harness the full potential of these powerful tools.
Dynamic Memory Management
Dynamic Memory Management is a crucial aspect of C programming that underpins effective resource utilization. In C, managing memory dynamically allows programmers to allocate memory during the runtime of an application rather than at compile time. This capability provides several advantages, especially when working with data structures whose size is not known ahead of time, such as linked lists or trees. The flexibility of dynamic memory management significantly impacts the efficiency and performance of applications, making it an essential skill for anyone looking to master C programming.
When you introduce dynamic memory management into your code, you're essentially granting your program the ability to make decisions on the fly about memory usage. This leads to optimizing memory allocation, which can dramatically reduce waste. However, with great power comes great responsibility. Poor memory management can result in issues such as memory leaks which can hamper performance and lead to application crashes.
Using malloc and free
Two of the most fundamental functions in C for dynamic memory allocation are malloc and free. Let's dive into how these work:
- malloc: This function reserves a block of memory on the heap of a specified size in bytes. If the allocation is successful, it returns a pointer to the beginning of the newly allocated memory block. If it fails, it returns . Here’s a simple usage example:
In the example above, we reserve memory for an array of five integers. It's crucial to check if the pointer returned is , indicating memory allocation failure, to avoid dereferencing a null pointer.
- free: Once you are done using the dynamically allocated memory, you must release it back to the system. This is done using the free function. Forgetting to free memory creates what’s commonly known as a memory leak.
Memory Leaks: Prevention and Debugging
Memory leaks occur when allocated memory is not released, leading to reduced available memory. This can cause the application to perform poorly or crash when it runs out of memory resources. Here are some tips to prevent memory leaks:
- Always free memory: After you’re finished with the allocated memory, use free to release it. For every call to malloc, there should be a corresponding call to free.
- Track your allocations: Keeping a log of all memory allocated can be beneficial, especially in larger applications. You might consider wrapping malloc and free in custom functions to log usage.
- Use tools: Tools like Valgrind can help track down memory leaks and give you insights into memory usage.
"An ounce of prevention is worth a pound of cure." Keeping a close eye on memory management practices can save time and headaches down the line.
By keeping these considerations in mind, you create a more efficient application that runs smoothly. Remember, mastering dynamic memory management not only enhances your skills as a programmer but also improves your software’s performance in the long run.
File Handling in
File handling is a crucial aspect of C programming that enables developers to perform operations on files, thus allowing persistent storage of data. The ability to read from and write to files is fundamental, especially in applications that need to handle large amounts of data or interact with external sources, such as logs, user configurations, or databases. By incorporating file handling into C programs, developers can go beyond the limitations of temporary data stored in memory, facilitating the management of information over time and across sessions.
The benefits of mastering file handling techniques in C include:
- Data Persistence: By saving data to files, applications can retrieve information even after they are closed and reopened.
- Interactivity with Users: Programs can allow users to input data through files, making applications more versatile.
- Organized Data Management: Files can be structured and organized, enabling efficient data retrieval and storage.
While working with files, there are certain considerations programmers must keep in mind. Proper error handling during file operations is essential to ensure that the program does not crash. Moreover, understanding the different file modes is key to using file handling effectively.
"Proper file handling allows programs to effectively interact with an external environment, ultimately leading to a more dynamic application experience."
Reading from and Writing to Files
Reading and writing data to files forms the backbone of file handling in C. To perform these operations, C provides a set of standard file handling functions, which are part of the C Standard Library. The most commonly used functions for these tasks are , , , and .
- Opening a File: Before performing any read or write operations, a file must be opened using . This function requires two arguments: the name of the file and the mode for opening it. Here’s a brief look at some common modes:
- Writing to a File: Once a file is opened in write mode, you can write data to it using functions like . Here’s a quick example:
- Reading from a File: To read data from a file, use or . You can pull data line by line or in a formatted way. An example of reading data line-wise looks like this:
- : Open the file for reading.
- : Open the file for writing; if the file exists, it will be truncated.
- : Open the file for appending.
Handling errors during these operations is crucial. Always check if the file pointer returned by is , indicating a failure to open the file.
File Pointers and Modes
Understanding file pointers and modes is paramount for effective file handling. In C, a file pointer is a variable that points to a location in a file. It allows you to keep track of where you are in the file while reading or writing data. The file pointer must be of type , which is defined in the standard I/O library.
When you open a file, it creates a file pointer that you can use in your subsequent file operations. The state of this pointer is updated with every read or write operation. Here's a rundown of typical file modes:
- Read Mode (): This mode is used for reading existing files. In this mode, if the file does not exist, the operation fails.
- Write Mode (): This is typically used for creating new files or overwriting existing ones if they exist.
- Append Mode (): Useful when you want to add data to the end of an existing file without altering its current content.
Exploring Advanced Concepts
When delving deeper into C programming, we encounter the realm of advanced concepts that extend beyond basic syntax and structures. This section is vital for reinforcing the understanding and application of C language in more complex scenarios. Mastering these elements not only enhances code efficiency but also builds a solid foundation for tackling real-world programming challenges. A significant component in any programmer’s toolkit, understanding advanced concepts equips learners to write more robust and maintainable code, making it essential for those who aspire to excel in the field.
Structures and Unions
Structures and unions are paramount in C programming, offering ways to group different data types into a single entity, enhancing the organization of complex data.
What are Structures?
A structure is a user-defined data type that allows the combination of different types of variables under one name. Think of a structure as a blueprint for creating various data forms. For example, if you are working on a project to keep track of books in a library, you may define a called that includes the title, author, and year of publication. Here’s a simple example:
Using structures helps streamline the management of related data. Imagine trying to handle each book's details separately; it would quickly become a tangled web of variables. By utilizing structures, you encapsulate that data neatly, making it easier to manage and understand.
Understanding Unions
Unions, on the other hand, are somewhat similar to structures but with a key distinction: they save memory. Union allocates enough memory for the largest data type, allowing you to store different types of data in the same location but only one at a time. This can be particularly useful in scenarios where memory efficiency is a concern.
Here, can store an integer, a float, or a character, but only one of those at any given moment. This flexibility allows you to conserve memory while managing multiple data types.
Key Takeaways
- Structures are suited for bundling data together for easier access and management. They provide a way to combine various data types into a cohesive structure, enhancing readability and maintainability.
- Unions offer a memory-efficient means of storing different data types, though at the cost of accessing only one at a time. This can be a double-edged sword; careful planning is necessary to use unions effectively without losing track of what data type is currently stored.
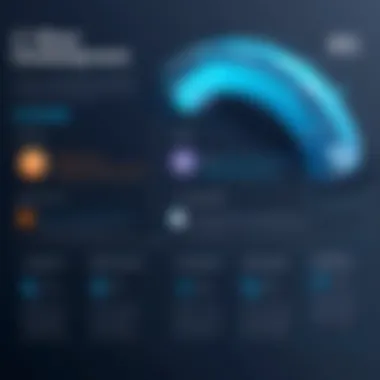
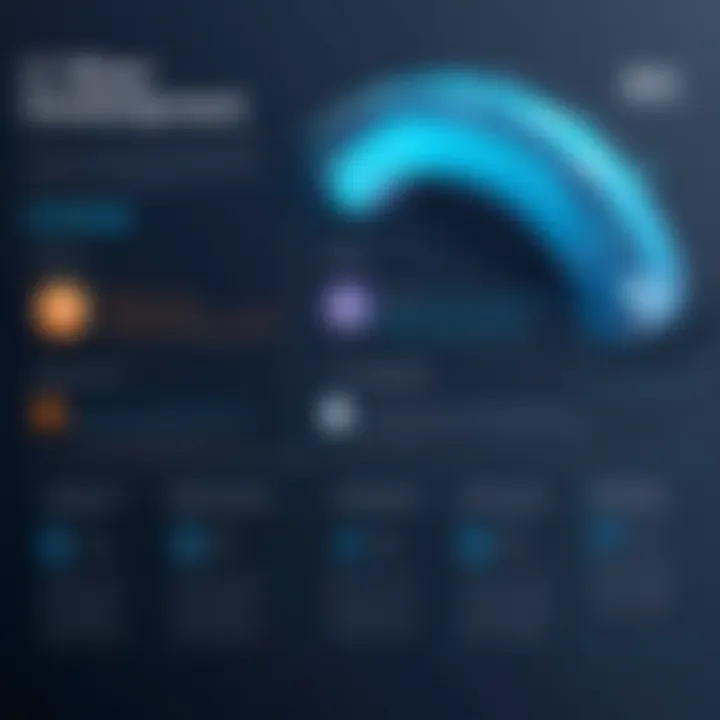
In summary, while structures and unions may seem like simple constructs, they carry significant weight within the programming world. Understanding when and how to use them can elevate your programming game, driving your code towards functionality and efficiency, both crucial in advanced C programming.
Enumerated Types
Enumerated types, or , are particularly useful when you need to represent a set of related constants in your code. This feature enables you to create a new type that consists of a list of named integer constants. By assigning names to these constants, you add clarity to your code while avoiding the confusion that can arise from using arbitrary numerical values.
Defining an Enum
Defining an enum is quite straightforward:
The days of the week now have meaningful identifiers rather than just numerical values. This makes your code easier to read and maintain.
Advantages of Using Enums
- Improves Code Clarity: Replacing magic numbers with descriptive names makes the code far easier to follow.
- Type Safety: Enums provide better type-checking, preventing potential bugs that can occur when using plain integers.
- Namespace Management: Enums help in organizing constants and avoiding naming collisions.
In wrapping up this exploration of advanced C concepts, it becomes clear how structures, unions, and enumerated types contribute significantly to writing efficient and effective code. Each plays a unique role in managing data, offering powerful tools to programmers aiming to master the complexities of the C language.
Programming Best Practices
C programming, while powerful and efficient, carries with it a set of best practices that can greatly enhance not only the quality of your code but also its maintainability and readability. Understanding these practices is vital for anyone wishing to pursue a serious journey in programming. C has a reputation for being complex, but the solidity and precision gained from adhering to best practices can alleviate potential pitfalls.
When you adopt best practices, you ensure that your code is not only functional but also comprehensible for others (and yourself when you revisit your work). Here, you will learn about two essential subtopics: commenting and code documentation, as well as error handling techniques, both of which contribute significantly to writing robust code.
Commenting and Code Documentation
Commenting your codegoes beyond merely leaving notes for others; it’s about fostering an environment where future developers can grasp the purpose of your decisions and understand the flow of logic. Think of comments as your code's personal translator—navigating others through the complexity of logic you've woven together. Here are some key points on why commenting is important:
- Clarity: It clarifies the purpose of your code sections, functions, and variables.
- Time-saving: Reduces the time it takes to debug or enhance the code in the future.
- Collaboration: Aids team members in understanding your contributions.
When commenting, be concise yet informative. For example, avoid stating the obvious like:
Instead, provide context:
Additionally, code documentation tools like Doxygen can help automate the generation of documentation from annotated source code files. A well-prepared documentation strategy can be a game-changer in various project stages.
"Good code is its own best documentation."
— Steve McConnell
Error Handling Techniques
In the world of programming, errors are as inevitable as the sunrise. As such, error handling becomes a crucial aspect of a robust C application. Implementing strategies to handle errors gracefully not only helps in providing a better user experience but also makes debugging more straightforward for you. Here are some practices to consider:
- Use Return Values: Functions should return specific error codes, often utilizing enums for clarity. Check these values after function calls to catch issues early.
- Assertions: Utilize assertions to catch failures in debugging phases. This will halt program execution if the condition fails, revealing where the logic goes awry.
- Error Logging: Implement logging for error tracking, as it can reveal patterns and pitfalls
For instance, here’s a simple error handling demonstration in a file handling scenario:
By paying attention to error conditions and taking appropriate actions, you can significantly increase the robustness of your C programs and reduce runtime surprises.
Debugging and Testing in
In the realm of C programming, debugging and testing stand as the bedrock upon which reliable software is crafted. The importance of these activities cannot be overstated; they are essential for ensuring that code not only runs but performs its intended function without unwelcome surprises. Debugging is the art of identifying and rectifying errors in the code, while testing involves verifying that the code behaves as expected under various scenarios.
Understanding the nuances of debugging and testing can elevate a programmer’s skill set significantly. A well-structured debugging methodology streamlines the process of troubleshooting. On the other hand, thorough testing safeguards against future pitfalls, ensuring the longevity and resilience of software.
- Preventing Issues: Catching errors early not only saves time but also reduces costs associated with fixing buggy code late in the development cycle.
- Enhancing Quality: Regular testing helps foster better code quality by enforcing best practices during development.
- Building Confidence: Well-tested applications instill confidence in developers and users alike, as bugs are minimized, leading to smoother functionality.
Overall, mastering debugging and testing practices is invaluable in the C programming landscape. It prevents grief down the line and is pivotal in crafting clean, effective code.
Common Debugging Techniques
Debugging can often feel like searching for a needle in a haystack, but various techniques can ease frustration. Here are some tried-and-true methods to tackle debugging in C:
- Print Statements: Simple yet effective. By adding print statements throughout the code, programmers can track the flow of execution and inspect variable states. For example:
- Using a Debugger: Most IDEs come equipped with debugging tools that allow step-by-step execution, letting you see what's happening in real-time. Tools like GDB (GNU Debugger) are incredibly useful for setting breakpoints, watching variables, and controlling the flow of execution.
- Segmentation Faults: These are pesky issues that arise when your code crashes. Understanding where these faults occur is crucial. Using tools like Valgrind can help identify where illegal memory accesses happen and how to fix them.
- Code Review and Pair Programming: Sometimes, a second set of eyes can spot issues you might have missed. Engaging in code reviews promotes knowledge sharing and different perspectives.
Using a combination of these techniques enhances the debugging prowess of a C programmer, contributing to a more robust final product.
Unit Testing in
Unit testing forms the backbone of a proactive approach to software development. It involves writing tests that cover individual components or functions of the program. Having a systematic approach to unit testing can help verify that each part of your code operates as intended. Here's what you need to know:
- Frameworks: There are several frameworks available for unit testing in C, including CUnit and Check. These frameworks provide the tools necessary to create tests efficiently, manage test cases, and generate reports on test results.
- Test-Driven Development (TDD): Embracing TDD can greatly benefit your programming efforts. With TDD, developers write tests before the actual code. This shifts the focus to building reliable code from the outset, encouraging simpler designs and fewer bugs.
- Best Practices: Keep tests small and focused. A unit test should purposefully test one aspect of functionality. Furthermore, ensure tests are repeatable; they should give the same results every time you run them, barring any code changes.
Unit testing not only helps catch bugs early but also serves as a reference point for future development, improving comprehension of the codebase. By integrating debugging and testing into your workflow, you establish a strong foundation for success in C programming.
"An ounce of prevention is worth a pound of cure."
Through effective debugging and rigorous testing, C programmers can build applications that not only function correctly but are also efficient and maintainable, paving the way for future innovations in software development.
and Software Development
C programming language holds a significant place in the landscape of software development. Understanding its principles is not just about learning to write code; it encompasses grasping how software interacts with hardware, systems, and other high-level programming languages. In this section, we will explore the pivotal role of C in system programming and how it integrates with other languages, providing insights that are crucial for budding programmers.
The Role of in System Programming
C is often deemed the "mother" of modern programming languages. Its design gives programmers fine control over system resources. Unlike many higher-level languages which abstract these details away, C's ability to manage memory and system processes makes it indispensable in system programming.
For instance, systems such as operating systems, device drivers, and embedded systems predominantly rely on C. Why? Because C allows developers to write low-level code that interacts directly with hardware, a feat not as easily accomplished in some higher-level languages. This capability translates into greater efficiency and speed, crucial in system-oriented tasks. It's akin to having the keys to unlock every door in a house, allowing programmers to fully customize what they want to achieve.
Here are some benefits of using C in system programming:
- Performance: C can be more efficient than many interpreted languages. This is vital in systems where speed and low memory usage are key.
- Portability: Programs written in C can run on various platforms with minimal changes, a necessary feature for system-level applications.
- Direct Manipulation: C provides pointers that allow direct memory management, enabling performance optimizations that high-level languages wouldn't allow.
Integration with Other Languages
While C stands strong on its own, it doesn't operate in a vacuum. Its ability to integrate with other programming languages enhances its functionality and applicability. For example, many frameworks and libraries written in C can be harnessed by languages like Python or Java, creating powerful combinations that tap into the strengths of each language.
Benefits of integrating C with other languages include:
- Performance Enhancement: For performance-critical parts of applications, developers can implement those sections in C while maintaining the rest of the application in a more user-friendly language.
- Access to Libraries: Many libraries are written in C due to its efficiencies. By integrating C, developers gain access to powerful tools and libraries that otherwise would not be available in higher-level languages.
- Legacy System Support: In some environments, legacy systems are built around C. Understanding how to work with C is crucial for maintaining and updating these systems effectively.
"The ability to understand and utilize C is more than a skill; it's a powerful weapon in the arsenal of a programmer, enabling them to navigate the complexities of software development with confidence."
To understand more about C programming's historical significance and its evolution, consider visiting resources like Wikipedia) or Britannica.
Finale and Future of Programming
Recap of Key Learnings
Let's take a moment to reflect on the core concepts we’ve covered throughout this guide. Key learnings include:
- Understanding the Basics: We began with the fundamentals of C programming, including its historical significance and the essential syntax that underpins the language.
- Control Structures and Functions: We delved into control structures like loops and conditionals, which are integral to decision-making in programming. Moreover, we examined functions, a critical component for achieving code reusability and modularity.
- Memory Management: The handling of memory using features like pointers and dynamic allocation was a crucial aspect, showcasing how C gives programmers fine-grained control over system resources.
- File Handling and Advanced Concepts: We covered how to read from and write to files, while also exploring complex data structures such as structures and unions which allow for more sophisticated data management.
- Best Practices and Debugging Techniques: Understanding how to write clean code, along with effective debugging and error handling mechanisms, is vital for any developer aiming to produce robust applications.
In summary, the knowledge gained about C programming equips learners with the capabilities to tackle real-world problems using an efficient approach.
Looking Ahead: The Evolution of Language
As we look to the future, it is clear that C programming will continue to play an influential role in software development. Despite the emergence of numerous programming languages and paradigms, C remains relevant for several key reasons:
- System-Level Programming: C is frequently used for developing system software like operating systems and embedded systems. Its ability to interface directly with hardware makes it irreplaceable in this domain.
- Portability and Compatibility: The standardization of C has ensured that programs written in it can run on different hardware and operating systems with minimal changes, thus promoting portability.
- Foundation for Other Languages: Many modern languages, such as C++, Java, and Python, have their roots in C. Understanding C lays a strong foundation for mastering these languages, making it incredibly relevant for budding programmers.
- Continuous Development: The language itself has seen enhancements over the years, with updates and new standards ensuring that it adapts to the changing realities of software development.