Mastering Authentication in Java: Essential Techniques
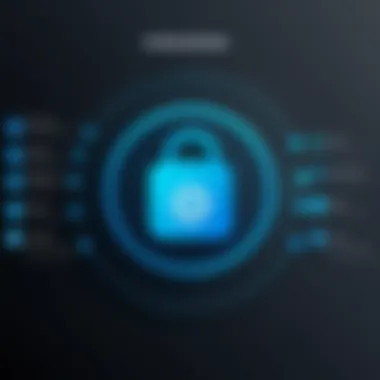
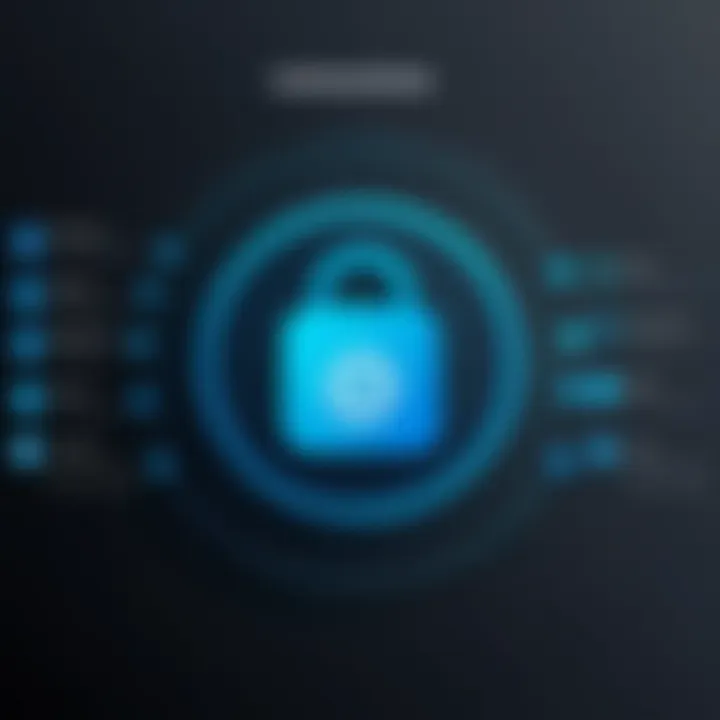
Intro
Authentication is vital in any software application, especially in web-based systems. Understanding how to implement secure authentication mechanisms in Java can greatly enhance the safety of applications. Java offers various frameworks and APIs that streamline the authentication process. This article aims to provide a thorough overview of authentication methods within Java, comparing traditional and modern practices.
As we navigate through Java's authentication landscape, we will discuss the critical aspects developers must consider. This includes exploring key frameworks, analyzing security concerns, and offering practical implementations.
Prelude to Programming Language
History and Background
Java was developed by Sun Microsystems and was released in 1995. Initially aimed at enabling interactive television, Java has evolved significantly to become one of the most widely used programming languages today. Its platform independence and robust security features have made it a preferred choice for web applications and enterprise development.
Features and Uses
Java offers features that contribute to its popularity. Its object-oriented nature promotes code reuse and modularity. Additionally, the automatic memory management provided by the Java Virtual Machine helps prevent memory leaks and improves application stability. This language finds its use in various domains, including web applications, mobile applications, and large-scale enterprise systems.
Popularity and Scope
Java consistently ranks among the top programming languages worldwide. According to various surveys, Java is favored by many developers for its rich set of libraries and community support. The scope of Java is vast, and it is evident in its utilization by major corporations as well as startups.
Basic Authentication Concepts
Authentication is the process of verifying a user's identity. In Java, this can be achieved through various methods, each with its strengths and weaknesses. Let's delve into some essential concepts.
User Credentials
The typical approach involves using usernames and passwords. However, additional factors can be included to enhance security, such as:
- Two-factor Authentication: Requires not just a password but also another authentication method, like a text message code.
- OAut: An authorization framework that enables applications to access user data from other services securely.
Authentication Protocols
Java supports several authentication protocols. Noteworthy ones include:
- Basic Authentication: Simple, but not encrypted by default.
- Digest Access Authentication: More secure than Basic Authentication, but also has limitations.
- Federated Identity: Allows users to authenticate across multiple platforms with a single identity.
Advanced Topics
Implementation of Authentication Mechanisms
The integration of authentication mechanisms varies greatly depending on the framework used. Here, we will highlight a few common frameworks:
- Spring Security: A comprehensive framework that provides authentication and access control.
- Apache Shiro: Known for its simplicity and powerful capabilities.
Security Concerns
When implementing authentication, several security concerns must be addressed, such as:
- SQL Injection: Properly escaping queries and using prepared statements can help mitigate this risk.
- Session Management: Ensuring that session data is secure and invalidating sessions post-logout is crucial.
Hands-On Examples
How to Implement Basic Authentication Using Spring Security
To implement basic authentication, one can configure Spring Security as follows:
Intermediate Projects
To gain practical experience, one can build a simple Java web application that requires user authentication. Such projects reinforce understanding of the concepts and methods discussed.
Resources and Further Learning
To deepen knowledge on Java authentication, consider the following resources:
- Recommended Books: "Effective Java" by Joshua Bloch for best practices.
- Online Courses: Websites like Coursera and Udacity offer courses on Java security.
- Community Forums: Engage with platforms like Reddit to discuss and clarify doubts with other developers.
Understanding Authentication in Java
Authentication plays a crucial role in securing Java applications and ensuring that user identities are verified before granting access to sensitive resources. This section delves into the essential concepts related to authentication in Java, highlighting its significance and various methodologies used in modern software development. Understanding these principles is not just important for secure coding, but it also shapes how developers approach the design and implementation of applications.
Definition and Importance
Authentication is the process of verifying the identity of a user, system, or entity. In the context of Java applications, it serves as the first line of defense against unauthorized access. The primary goal is to ensure that only legitimate users can interact with an application, safeguarding sensitive data and functionality from potential threats. Without proper authentication mechanisms, applications could be vulnerable to various security risks, including data breaches and unauthorized user activities.
Moreover, authentication influences user experience. A well-designed authentication process can help streamline user access, improving overall satisfaction while maintaining security. Developers must also recognize the impact of authentication on compliance with regulatory standards and organizational policies.
"Inadequate authentication methods can lead to significant security lapses, making it imperative for developers to adopt robust practices."
Types of Authentication Mechanisms
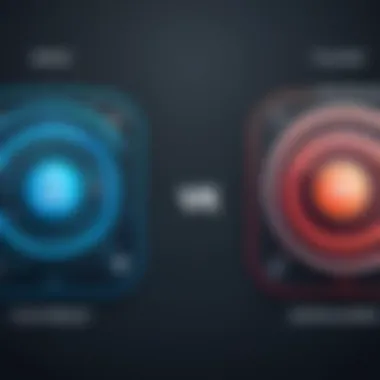
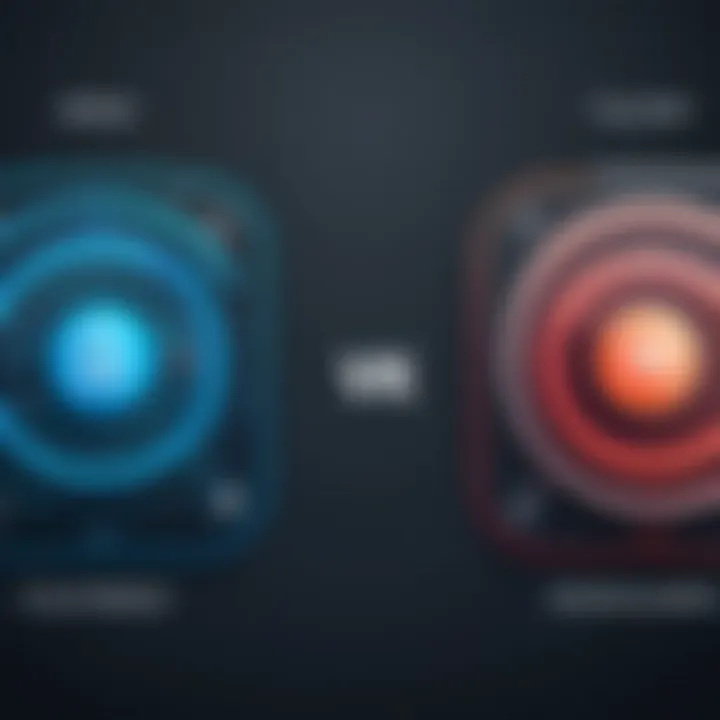
There are several types of authentication mechanisms used in Java applications. Each has its own benefits and considerations:
- Basic Authentication: This is a straightforward method where users provide a username and password. It is typically easy to implement but should be paired with secure transmission protocols, like HTTPS.
- Token-Based Authentication: This method involves generating a temporary token after the user successfully logs in. The token is sent with each request, allowing the server to verify the user's identity without the need for continuously resending credentials.
- Multi-Factor Authentication (MFA): MFA enhances security by requiring users to provide two or more verification factors. This could include something they know (password), something they have (token or mobile device), or something they are (fingerprint).
- Single Sign-On (SSO): SSO allows users to authenticate once and gain access to multiple applications without re-logging, simplifying the user experience while controlling access centrally.
- Biometric Authentication: This newer approach utilizes physical characteristics, such as fingerprints or facial recognition, to verify identity. While it offers higher security, implementation can be more complex.
Understanding these mechanisms is crucial for developers. They must evaluate their applications' specific security needs and choose the most suitable methods to protect user data effectively. The selection process should take into account factors like ease of implementation, user experience, and level of security required.
Java Authentication Frameworks
In the realm of Java development, authentication frameworks play a critical role. They provide essential structures that allow developers to efficiently integrate authentication mechanisms within their applications. These frameworks streamline the process of implementing user authentication and authorization while promoting adherence to security best practices. Understanding these frameworks is key for any developer keen on building robust Java applications.
Java Authentication and Authorization Service (JAAS)
JAAS is a vital part of Java's security architecture. It provides a pluggable framework for managing authentication and authorization of users. The main advantage of JAAS is its flexibility; developers can choose different authentication modules based on application requirements. This means that systems can evolve over time without significant changes to the core application. Using JAAS makes it easier to enforce security policies, which is essential for any business-facing application.
JAAS operates on a principle of security realms. Each realm can contain various login modules. This modularization allows for the integration of techniques like Kerberos, X.509, or even usernames and passwords. Here is how JAAS generally works:
- The application requests the user to present credentials.
- JAAS verifies these credentials against the identified login module.
- Upon successful verification, the user is granted permission based on defined roles and policies.
This structured approach is both efficient and effective, making JAAS a popular choice among Java developers.
Spring Security Framework
Spring Security is another powerful framework designed to address authentication and security concerns in Java applications. It is part of the larger Spring ecosystem, which is known for its dependency injection and aspect-oriented programming. One of the core benefits of Spring Security is its comprehensive support for various authentication techniques, including form-based and HTTP Basic authentication.
With Spring Security, developers can enforce security at both method level and URL level, offering granular control over access rights. Moreover, it supports integration with OAut and OpenID Connect, allowing seamless use of social logins and single sign-on features.
Key features of Spring Security include:
- Declarative security through annotations.
- Extensive customization options.
- Support for CORS and CSRF protection.
Understanding Spring Security not only empowers developers to build secure applications but also aids in maintaining user trust. Security is a continual journey in development, and Spring Security provides the tools to navigate this space effectively.
Apache Shiro
Apache Shiro is an open-source security framework that provides comprehensive functionalities for authentication, authorization, session management, and cryptography. It is designed to be flexible, easy to use, and to plug into any Java application.
One notable feature of Apache Shiro is its capability to handle authentication directly from various systems, such as LDAP or databases, making it versatile for different environments. Its intuitive API allows developers to implement complex security scenarios with minimal code.
The advantages of using Apache Shiro include:
- Simplicity and ease of integration.
- Strong session management capabilities.
- Robust support for both web and non-web environments.
The design philosophy behind Apache Shiro ensures that it's easy to learn and implement while providing the necessary tools to maintain secure applications. Its capability to integrate with existing systems makes it suitable for legacy projects as well.
In summary, when developers consider authentication mechanisms, frameworks such as JAAS, Spring Security, and Apache Shiro are central to modern Java applications. Each has its specific strengths and selecting the right framework often depends on the individual project requirements and context.
Implementing Basic Authentication
Implementing basic authentication is a critical topic in the realm of security for Java applications. This form of authentication serves as the foundational layer for verifying user identities. Understanding how to implement it effectively can safeguard sensitive data from unauthorized access. There are several elements and benefits associated with basic authentication that developers must carefully consider.
When done correctly, basic authentication ensures that only users with valid credentials can access specific resources. This method adheres to principles of simplicity and ease of integration, making it a go-to solution for many developers, especially in smaller applications or when rapid deployment is necessary. However, it is crucial to be aware of its limitations. Basic authentication often transmits credentials in an encoded form rather than encrypted. This leaves it vulnerable unless additional security measures like HTTPS are applied.
User Credentials and Storage
User credentials form the very core of the basic authentication process. When implementing basic authentication in Java, developers must decide on how to securely manage these credentials.
- Usernames and Passwords: The most common credentials include usernames and passwords. It is vital that passwords are not stored as plain text. Instead, developers should utilize hashing algorithms, such as bcrypt or PBKDF2, to secure passwords before they are stored in a database.
- Storage Solutions: Common storage solutions for user credentials range from relational databases to NoSQL databases. Each option has its advantages and disadvantages.
- Password Salting: To further enhance security, developers can implement a technique called salting. This adds a random value to passwords before hashing, making it significantly harder for attackers to use pre-computed hash tables (rainbow tables) in attacks.
- Relational Databases (e.g., MySQL, PostgreSQL) are well-known for their ACID properties, ensuring data integrity.
- NoSQL Databases (e.g., MongoDB) offer flexibility in data storage but may require careful consideration regarding data consistency.
In summary, robust handling of user credentials is fundamental to the success of basic authentication in Java applications.
Creating a Secure Authentication Process
The process of creating a secure authentication system goes beyond just handling user credentials. Developers must consider multiple factors to ensure that the system remains resilient against attacks.
- HTTPS Utilization: As mentioned earlier, transport of user credentials should always occur over HTTPS to protect against eavesdropping and man-in-the-middle attacks. Configuring the server to enforce HTTPS is a non-negotiable aspect.
- Error Handling: Implementing proper error handling can prevent attackers from gaining insights into the authentication system. Instead of providing specific error messages like "username not found" or "password incorrect", a generic message such as "invalid credentials" can be utilized.
- Session Management: Once authenticated, maintaining user sessions securely is important. Developers should implement timeouts for sessions and offer the ability to log out, thereby minimizing the risk of session hijacking.
- Regular Audits: Periodically reviewing and testing the authentication system helps identify vulnerabilities and ensure that security measures are effective.
To conclude this topic, creating a secure authentication process requires attention to detail and a commitment to implementing best practices. By addressing each of these elements, developers enhance the resilience of their Java applications against potential authentication threats.
Security is not a one-time effort but requires ongoing dedication and vigilance.
Advanced Authentication Techniques
Advanced authentication techniques play a crucial role in enhancing the security of applications. As cyber threats grow more sophisticated, developers must implement more robust methods to protect sensitive user information. This section covers two prominent techniques: token-based authentication and multi-factor authentication (MFA). Each of these methods addresses specific vulnerabilities in traditional authentication systems, thereby ensuring a more secure user experience.
Token-Based Authentication
Token-based authentication is increasingly popular in modern web applications. It allows for a stateless method of user verification, where user credentials are exchanged for a token upon successful login. This token is then used for subsequent requests, eliminating the need to repeatedly send sensitive information like passwords.
Benefits of Token-Based Authentication:
- Statelessness: The server does not need to store user session information, which reduces server memory usage.
- Scalability: This method enhances scalability since it enables the backend to handle more users without compromising performance.
- Cross-Domain Support: Tokens can be easily used across different domains, making it effective for applications built with microservices.
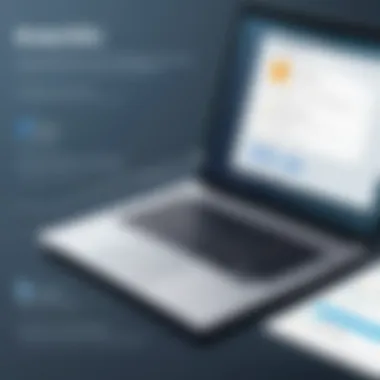
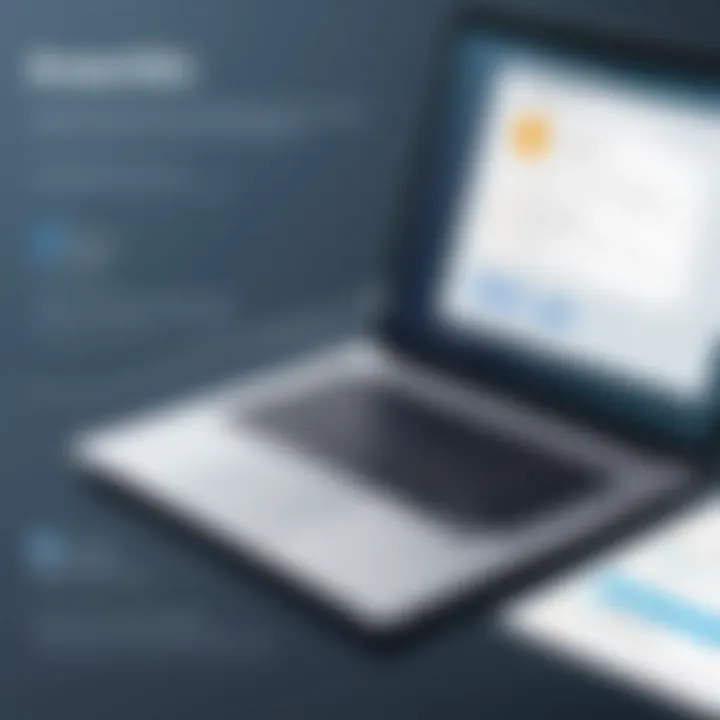
Implementing token-based authentication generally involves the following:
- User logs in with credentials.
- Server validates credentials and generates a token.
- Token is sent back to the client.
- Client stores the token (often in local storage or cookies).
- For each request, the client sends the token in the header.
This mechanism securely ensures that only verified users can access protected resources, reducing the risk of interception and replay attacks. However, it is essential to secure the token itself, for instance, by using HTTPS for all communications.
Multi-Factor Authentication (MFA)
Multi-Factor Authentication (MFA) adds an extra layer of security beyond traditional login methods. MFA requires users to present two or more verification factors to gain access to an application or online account. This extra step decreases the likelihood of unauthorized access, even if a password is compromised.
Common Factors Used in MFA:
- Something you know: A password or PIN.
- Something you have: A mobile device or hardware token.
- Something you are: Biometric verification like fingerprints or facial recognition.
Benefits of Multi-Factor Authentication:
- Enhanced Security: Even if a password is stolen, the chances of unauthorized access remain low without the second factor.
- User Trust: Implementing MFA can enhance user trust, knowing that their accounts are well protected.
To implement MFA in a Java application, developers can use libraries to facilitate multi-factor verification. Examples include integrating services such as Google Authenticator or using SMS-based verification. Proper implementation requires careful consideration of user experience; the process should not be overly cumbersome, as this may lead users to abandon the authentication process.
"Implementing advanced authentication techniques is not just a trend but a necessity in today's digital landscape."
In summary, token-based authentication and multi-factor authentication are pivotal in establishing secure application environments. By employing these techniques, developers can significantly mitigate security risks while ensuring a smoother user experience.
Integrating Third-Party Authentication Services
Integrating third-party authentication services is a pivotal aspect of modern application development. By leveraging established authentication providers, developers can offload the complexity of managing user credentials while enhancing security. This integration not only streamlines user management but also improves the user experience by allowing seamless login processes.
The benefits of third-party authentication services include:
- Increased Security: Third-party providers like Google, Facebook, and others implement robust security measures. This reduces the likelihood of vulnerabilities in your application.
- User Convenience: Users prefer not to remember multiple passwords. Allowing them to log in using existing accounts can increase user retention and satisfaction.
- Faster Development: It speeds up the development process. Developers don’t have to create and maintain authentication systems from scratch.
However, there are considerations.
- Dependency on External Services: Relying on a third-party service means that issues on their end can directly impact your application’s functionality.
- Potential Privacy Concerns: Users may have reservations about sharing their information with third parties.
- Integration Complexity: Setting up OAuth or OpenID can introduce complexities in application architecture.
Integrating such services is not simply about adding functionality. It requires a structured approach, ensuring that the integration aligns with the application's overall goals, especially in terms of security and usability.
OAuth 2. Overview
OAuth 2.0 is a protocol that allows applications to obtain limited access to user accounts on an HTTP service. It does this by allowing users to authorize third-party applications without sharing their credentials. The protocol facilitates a secure delegation of access and is widely adopted in various applications, especially those that require a single sign-on feature.
The main components of OAuth 2.0 include:
- Resource Owner: The user or entity who owns the data.
- Resource Server: The server hosting the user’s data for which access is requested.
- Client: The application requesting access to user data.
- Authorization Server: The service that authorizes the client to access the resource owner’s data.
To initiate the process, the client requests authorization from the user. Upon approval, the user receives an authorization grant, which is exchanged for access and refresh tokens. This method provides a layered security approach by ensuring that sensitive information is never exposed to the client directly.
OpenID Connect
OpenID Connect is an authentication layer built on top of OAuth 2.0. It provides a framework to verify the identity of users based on authentication performed by an Authorization Server. This enhances the capabilities of OAuth with an ID token that provides information about the user.
Key features of OpenID Connect include:
- Identity Verification: It confirms the identity of users. This is essential for applications requiring high security, as it reduces the risk of impersonation.
- Standardized: OpenID Connect uses standard JSON Web Tokens (JWT), making it easier to integrate across different platforms and programming languages.
- User Information: It enables applications to retrieve user profile information in a secure manner, promoting a personalized user experience.
Understanding Security Best Practices
In the realm of software development, particularly within Java applications, the establishment of robust security measures is more than a preference—it is a necessity. Authentication serves as the first line of defense against unauthorized access, and it is critical to understand best practices to safeguard user data and maintain system integrity. Security best practices not only help in fortifying the authentication process but also in fostering user confidence. This section will elucidate key practices that developers must adopt to enhance authentication security.
Password Management Guidelines
The foundation of authentication often lies within the management of passwords. Strong password policies are essential as they help mitigate the risk of unauthorized access. Here are several guidelines that can be implemented:
- Complexity Requirements: Users should be required to create passwords that contain a mix of uppercase and lowercase letters, numbers, and special characters. This complexity hinders the ability of attackers utilizing automated tools to crack passwords.
- Password Length: The minimum length for passwords should be specified, ideally at least 12 characters. Longer passwords make it more difficult for brute-force attacks to succeed.
- Regular Updates: Policies should mandate periodic password changes. This limits the amount of time a compromised password can be used.
- Password Storage Techniques: It is vital to store passwords securely. Utilize algorithms like BCrypt or Argon2 to hash passwords. Never store passwords in plain text or use outdated encryption techniques.
- Two-Step Verification: Implement an additional layer of security by requiring users to verify their identity through a second method, such as SMS or email confirmation. This reduces the risk associated with stolen passwords.
Adopting these guidelines leads to the creation of a stronger security posture. By emphasizing password strength and secure handling, developers can mitigate many of the common vulnerabilities that arise from poor password practices.
Secure Transmission of Credentials
Once credentials are established, their transmission poses another area of security concern. Implementing secure methods for credential transmission is crucial to protect data from interception. Here are essential considerations:
- Use HTTPS: Ensure all data exchanged between client and server is encrypted using HTTPS. This prevents eavesdropping and man-in-the-middle attacks.
- Transport Layer Security (TLS): Utilize TLS protocols to secure communication channels, ensuring that sensitive information, like authentication tokens or passwords, cannot be easily intercepted.
- Session Management: Once authenticated, manage user sessions securely. Implement session timeouts and allow users to log out, ensuring that inactive sessions do not remain open unnecessarily.
- Avoid URL Parameter Usage: Do not transmit sensitive information via URL parameters. Instead, use headers or body payload in POST requests to prevent exposure in server logs.
- Log Security Events: Keep logs of authentication attempts, especially unsuccessful ones. This aids in detecting potential attacks and responding promptly to security incidents.
By implementing secure transmission practices, developers greatly reduce exposure to risks associated with credential theft. This attention to detail in the authentication process is essential for building trust and safeguarding user information effectively.
"Security is not a product, but a process."
Ultimately, understanding and applying security best practices in Java authentication leads to a significantly more secure application, fostering reliability and offering substantial protection against various cyber threats. Adhering to these guidelines creates an environment where both developers and users can engage confidently, knowing that measures are in place to protect sensitive information.
Performance Considerations in Authentication
When developing applications that rely on authentication mechanisms, understanding performance considerations is essential. The way an application handles authentication can significantly impact user experience, system scalability, and overall efficiency. Poor performance in authentication systems can result in delays that frustrate users and potentially lead them to abandon the application. This section delves into critical aspects of handling authentication loads and caching authentication results, providing practical insights into optimizing these processes.
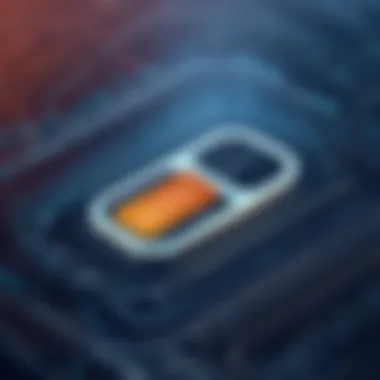
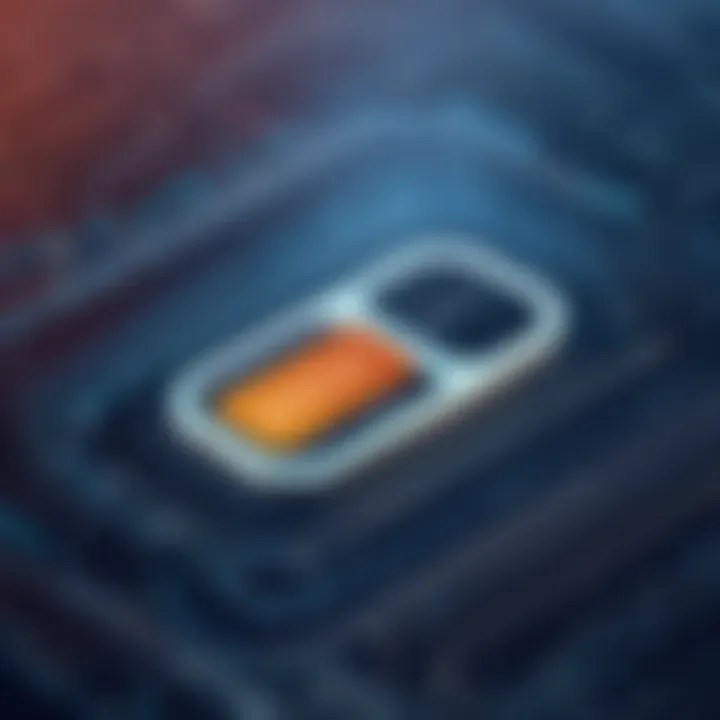
Authentication Load Handling
Handling authentication load is crucial, especially for applications with a vast user base. When numerous users attempt to access an application simultaneously, the authentication service must efficiently process these requests without bottlenecking system resources.
- Load Balancing: Implementing load balancing strategies can distribute user authentication requests across multiple servers. This prevents any single server from becoming overwhelmed, thereby improving response times. Load balancers can use algorithms like round-robin or least connections to optimize request distribution.
- Asynchronous Processing: Utilizing asynchronous processing allows the system to handle authentication requests without keeping users waiting in a queue. Instead of blocking operations, non-blocking I/O operations can improve throughput and enhance the responsive nature of the application.
- Scalable Architecture: Developing applications with a microservices architecture can enhance the scalability of authentication processes. Each service can handle its load independently, making it easier to adjust resources based on traffic demand.
- Monitoring Tools: Using tools such as ELK stack or Prometheus for monitoring authentication requests can provide insights into system performance. These insights can guide optimization strategies, ensuring that potential issues are addressed proactively.
"Performance optimization in authentication not only boosts user satisfaction but also enhances the security posture of an application."
Caching Authentication Results
Caching authentication results is another vital aspect of performance considerations. By storing the outcomes of authentication requests for subsequent use, applications can significantly reduce the overhead of processing repeated requests.
- Benefits of Caching: Caching reduces unnecessary database queries for commonly accessed user authentication data. This can lead to faster authentication times and decreased server load.
- Cache Expiration Policies: Implementing effective cache expiration policies is critical. Stale authentication information can pose security risks. Setting reasonable time-to-live (TTL) values ensures that cached data is refreshed periodically while still providing performance benefits.
- Choosing the Right Cache: Leveraging tools like Redis or Memcached can enhance cache management for authentication. These in-memory data stores offer high performance and can handle large volumes of requests efficiently.
- Integration with Session Management: Caching can also be integrated with session management systems to ensure that user sessions are readily available, further minimizing the delay in authentication processes. When a user logs in, their authentication status can be cached for future requests, enhancing both speed and efficiency.
In summary, addressing performance considerations in authentication involves multiple strategies, including load handling and caching. By implementing these techniques, developers can create robust applications that not only perform well under pressure but also maintain a secure and efficient authentication process.
Testing Authentication Mechanisms
Testing is a critical aspect of developing robust authentication systems in Java. Authentication mechanisms are designed to ensure that only authorized users can access sensitive information or features within an application. Without thorough testing, vulnerabilities can remain hidden, exposing applications to security risks.
Effectively testing authentication mechanisms can help identify flaws in the implementation, ensure compliance with security standards, and improve the overall user experience. There are a range of strategies that assist developers in validating the integrity of their authentication processes.
Unit Testing Strategies
Unit testing focuses on testing individual components of an application in isolation. This is particularly essential for authentication processes, as even minor issues can cause significant security weaknesses. Here are some key strategies for unit testing authentication mechanisms:
- Test User Input Validation: Ensure that all user inputs, especially credentials, are properly validated. This includes checking for length, character restrictions, and null values.
- Mock Dependencies: Use mocking frameworks to simulate interactions with external services, like databases or third-party authentication providers. This helps verify that your integration points are functioning correctly without relying on these services during testing.
- Test for Error Handling: Ensure that proper error messages are returned for invalid credentials or failed authentication attempts. This helps prevent leaking information that could be useful for an attacker.
- Security Assertions: Incorporate assertions that check for specific behaviors or states. For example, ensure that a user’s session token is only valid after successful authentication.
Here’s a simple example of a unit test using JUnit:
This ensures that both valid and invalid credentials are handled appropriately, highlighting the importance of comprehensive testing.
Security Testing Methodologies
Security testing goes beyond unit tests, focusing on assessing an application's authentication from a security perspective. Various methodologies can be applied:
- Penetration Testing: This involves simulating attacks on the authentication mechanism to identify vulnerabilities. It allows developers to proactively discover weak points in their system.
- Vulnerability Scanning: Automated tools can scan applications for common security vulnerabilities such as SQL injection or cross-site scripting, specifically targeting authentication functionalities.
- Code Reviews: Regularly reviewing the authentication codebase helps catch any potential security issues early. Peers can provide valuable insights that might be overlooked.
- Use of Security Standards: Familiarize and comply with security frameworks like OWASP. Following these guidelines can lead to more secure authentication implementations.
Regular testing of authentication mechanisms is not just a good practice; it is essential for maintaining user trust and protecting sensitive data.
By adopting a thorough approach to both unit and security testing, Java developers can significantly enhance the reliability and security of their authentication processes. This not only protects the application but also ensures a better experience for users.
Common Pitfalls and Challenges
Identifying common pitfalls in authentication is essential for any developer working with Java applications. Gaps in authentication can lead to security breaches, data leaks, and compromised user information. Understanding these challenges allows developers to create more robust systems. Addressing potential weaknesses early in the development process can save time and resources later. This section explores two crucial aspects: weak password policies and handling authentication errors.
Weak Password Policies
A weak password policy poses significant risks to the security of an application. Many developers may underestimate the value of enforcing strong password practices. Weak passwords can be easily exploited with brute-force attacks or social engineering techniques. Therefore, it is necessary to ensure that users create passwords that meet certain complexity requirements.
Thus, certain measures should be incorporated:
- Minimum Length: Require a minimum of 8 to 12 characters.
- Complexity Requirements: Encourage a mix of uppercase letters, lowercase letters, numbers, and special characters.
- Password Expiration: Implement a policy for regular password changes, like every 90 days.
- Multi-Factor Authentication (MFA): Adding an extra layer of security by requiring more than just a password.
For example, creating user accounts with these stricter rules significantly reduces the chance of unauthorized access. Developers should also communicate the importance of strong passwords to users to increase theyr overall security awareness.
"A strong password is your first line of defense against potential breaches."
Handling Authentication Errors
Errors during the authentication process can create challenges for both users and developers. Ineffective handling of these errors can lead to poor user experiences and may even expose sensitive information.
It is vital to implement a systematic approach to handle errors effectively:
- User-Friendly Messages: Provide clear messages without revealing specifics about what went wrong. For example, rather than stating if a username or password is incorrect, say, "Login failed. Please try again."
- Rate Limiting: Protect against brute-force attacks by limiting the number of login attempts. This is critical to ward off automated attacks.
- Logging and Monitoring: Maintain logs of failed login attempts for analysis, which helps identify potential attack patterns.
- Appropriate Redirects: After failed attempts, redirect users back to the login page rather than exposing another page where they might see more sensitive information.
By focusing on these strategies, developers can create more resilient authentication processes that enhance security and improve user experience.
Future Trends in Java Authentication
The landscape of authentication in Java is on the verge of significant evolution due to emerging technologies and changing user expectations. Understanding these trends is crucial for developers who aim to build secure and efficient applications. As the digital landscape grows more complex, so too do the methods employed to protect user identities and credentials.
Decentralized Identity Solutions
Decentralized identity is gaining traction as a method to enhance security and privacy. This approach enables users to manage their own identities without relying heavily on centralized authorities. With the rise of blockchain technology, decentralized identities offer improved user control and reduced risks associated with data breaches.
Some benefits include:
- Enhanced Security: Users hold their identity information, minimizing the risk of centralized data exploits.
- Privacy Control: Users can choose what information to share and with whom.
- Interoperability: One identity can work across various platforms and services, simplifying user experiences.
In Java, integrating decentralized solutions can be done using libraries like Hyperledger Indy, which provides a framework for building decentralized identity systems. Developers will need to consider the transition from traditional methods of authentication to these innovative frameworks.
Integration with AI and Technologies
Artificial Intelligence (AI) and Machine Learning (ML) technologies are poised to reshape Java authentication in various ways. These tools help to analyze user behavior and identify anomalies that could indicate security threats. By leveraging AI and ML, developers can create adaptive authentication systems that enhance security while minimizing friction for legitimate users.
Key considerations for integrating AI and ML in authentication include:
- Behavioral Biometrics: Monitoring unique user behaviors, like typing patterns or mouse movements, provides another layer of security.
- Risk-Based Authentication: This involves assessing the risk level of each login attempt, allowing for more stringent checks in high-risk situations.
- Fraud Detection: Machine learning algorithms can detect unusual patterns, alerting administrators to potential security breaches.
By using AI and ML in Java applications, developers can create more resilient authentication mechanisms that evolve with threats.