Mastering the Array Class in C#: A Comprehensive Guide
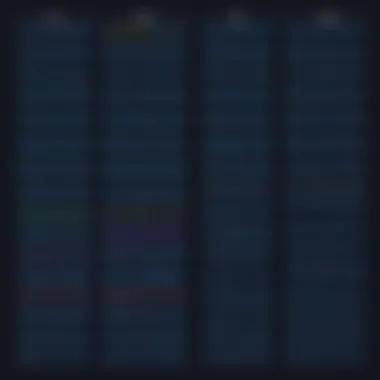
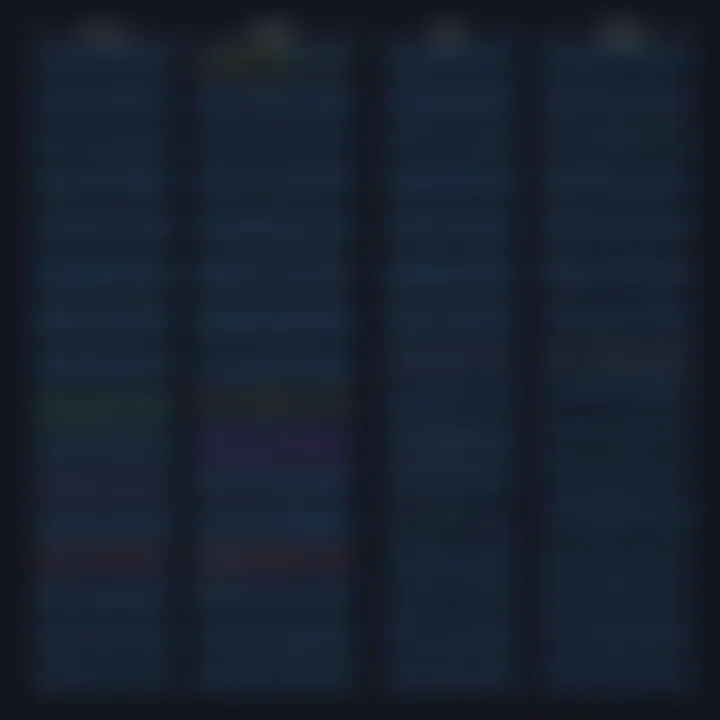
Intro
The Array class in C# serves as a fundamental component in the programming language, offering a straightforward way to store and manage collections of data. By understanding this class, programmers can drastically improve their ability to handle complex data structures efficiently. This article will explore the various aspects of the Array class, including its types, methods, properties, and best practices for performance optimization.
Understanding Arrays in
Arrays are fixed-size collections of elements that share the same data type. They provide a mechanism for grouping related data together, allowing programmers to access and manipulate that data conveniently. In C#, arrays are implemented as objects, which means they come with a variety of built-in methods and properties that can be utilized to accomplish tasks effectively.
Key Characteristics of Arrays
- Fixed Size: An array's size must be declared at the time of creation and cannot be changed later.
- Zero-based Indexing: C# arrays utilize zero-based indexing, meaning the first element is accessed using index 0.
- Homogeneous Data Types: Arrays can only contain elements of the same data type, enforcing data integrity.
"Understanding how to manipulate arrays is crucial for effective programming in C#."
Types of Arrays
In C#, various types of arrays allow for flexibility in data representation and manipulation. Here are the most common types:
- Single-Dimensional Arrays: Linear structures used to hold one set of data.
- Multi-Dimensional Arrays: Used to store data in a table format, allowing rows and columns.
- Jagged Arrays: Arrays of arrays, where each sub-array can have a different length.
Methods and Properties of Arrays
C# provides a rich set of methods and properties related to the Array class:
- Length: This property returns the total number of elements in the array.
- GetLength(): Retrieves the length of a specified dimension in a multi-dimensional array.
- Sort(): A method that sorts the elements of a one-dimensional array.
- Copy(): This method is used to copy elements from one array to another.
Performance Considerations
When working with arrays, performance optimization is essential. Here are some best practices to improve efficiency:
- Avoid resizing arrays frequently, as this can lead to performance overhead.
- Utilize the built-in methods for sorting and searching instead of manual implementations.
- When dealing with large data sets, consider using collections such as ListT> for dynamic sizing.
Finale
The Array class in C# is an essential feature that beginners and intermediates alike must understand. By grasping the key characteristics, types, methods, and performance considerations associated with arrays, programmers can create more efficient and effective code. Embracing arrays is not just a matter of knowing how to use them but understanding when and where they can be most beneficial in your coding practice.
Prelims to Arrays in
Arrays are a fundamental data structure in C#. These are crucial for handling collections of data efficiently. The ability to store multiple items of the same type under a single variable name is powerful. It simplifies data management and enhances the performance of applications. In learning programming, arrays often become one of the first collections introduced. Understanding arrays lays the groundwork for more advanced data structures.
Definition and Purpose
An array is a fixed-size collection of elements, all of which are of the same data type. In C#, arrays are invocable through indexes, meaning individual elements can be accessed randomly using their position numbers. The purpose of arrays in programming is to organize data. It allows developers to group related values together for efficient retrieval and manipulation. Creating an array in C# can be straightforward. For example:
This line of code defines an array that can hold five integers.
Significance in Programming
The significance of arrays cannot be understated. They facilitate data storage, organization, and constant time complexity for access to elements via indices. With creating array instances, programmers can implement numerous algorithms more efficiently. For instance, sorting algorithms often leverage arrays due to their contiguous memory allocation. In programming contexts, arrays can be manipulated through various methods provided by the Array class. Such methods include sorting, copying, and reversing collections with ease. Using arrays can lead to improved code readability and maintainability. Developers must grasp this concept to take full advantage of the array functionalities offered by C#.
"An array is like a canvas where developers can create various data-driven applications seamlessly."
Thus, starting with arrays, programmers can better structure their code and improve it.
Array Class Fundamentals
Understanding the Array class is crucial for any C# programmer. This foundation not only enhances coding efficiency but also promotes better application design. The Array class encapsulates a variety of functionalities that simplify data organization. With arrays, programmers manage collections of data items flexibly and effectively, which is essential in many programming scenarios. In exploring this topic, we can identify key aspects that are beneficial for both beginners and seasoned developers.
Basic Properties of the Array Class
The Array class comes with several core properties that define its usage. These properties include:
- Length: Represents the total number of elements within the array. This property is vital for loop iterations and data manipulation.
- Rank: Indicates the number of dimensions of the array. Understanding rank helps in determining how to access or manipulate multi-dimensional data.
- GetValue and SetValue: Methods allowing retrieval and assignment of elements in the array by their index. This is particularly useful for dynamic data handling.
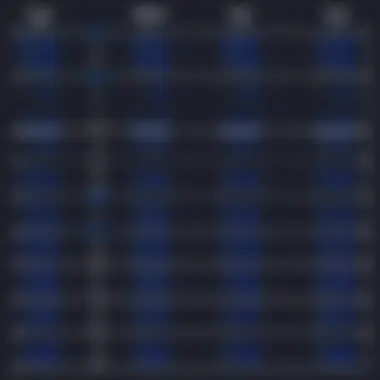
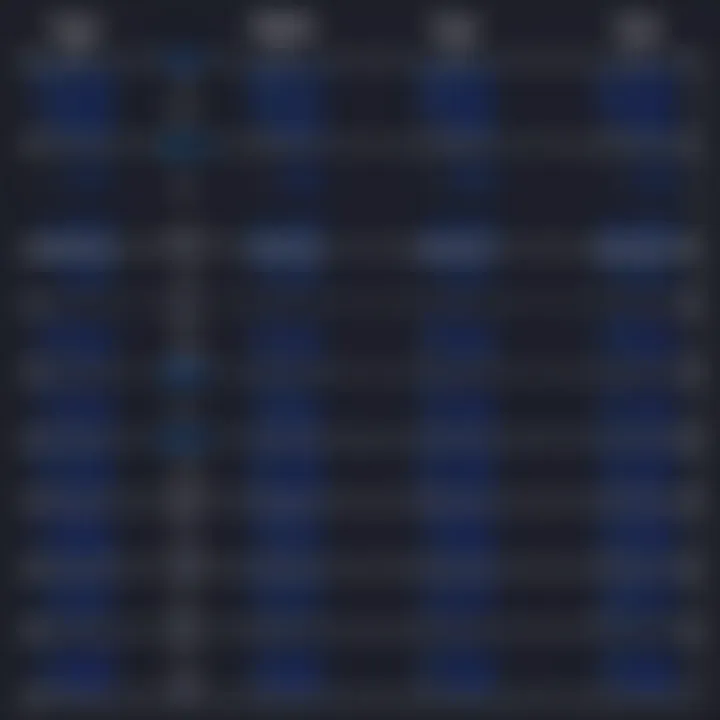
These properties not only facilitate data management but also assist in understanding array behavior. Mastering these basic properties lays the groundwork for more advanced manipulation techniques.
Understanding Multi-Dimensional Arrays
Multi-dimensional arrays are arrays of arrays, allowing the storage of data in two or more dimensions. They are often used in scenarios where data representation mimics real-world structures, like tables or matrices. In C#, it’s possible to declare a two-dimensional or even a three-dimensional array.
For example, to declare a simple two-dimensional array in C#:
This simple declaration initializes a grid of integers. Working with multi-dimensional arrays involves understanding how to access data using multiple indices, enhancing the ability to solve complex problems.
Jagged Arrays versus Rectangular Arrays
When comparing jagged arrays to rectangular arrays, the distinctions are important for optimal data management. A rectangular array is a multidimensional array where each row has the same number of elements. In contrast, a jagged array can have rows that vary in length.
- Rectangular Array Example:
- Jagged Array Example:
Choosing between a jagged or rectangular array is often dictated by the requirements of the application. Jagged arrays are more flexible with storage, while rectangular arrays may provide performance benefits in specific situations.
Understanding the differences between these types of arrays can significantly influence the performance and design of your applications.
Mastery of these array fundamentals provides the groundwork for further exploration into array methods and practices.
Creating Arrays in
Creating arrays is a fundamental skill in C#. This section aims to illuminate the process and considerations involved in effectively creating arrays within the C# programming environment. Understanding how to create arrays provides the groundwork for storing and manipulating data in an efficient way. Arrays facilitate data organization, making them essential for both simple and complex programming tasks.
Declaring and Initializing Arrays
Declaring and initializing arrays in C# is straightforward. An array declaration involves specifying the type of elements it will hold, followed by square brackets, and finally, the name of the array variable. For example:
This code snippet indicates that is an array designed to hold integers. Initialization occurs when you assign values to the array. This can be done in various ways.
- Static Initialization: This method allows you to assign values at the time of declaration. For example:Here, is initialized with five integers at once.
- Dynamic Initialization: Arrays can also be initialized after declaration. First, you define the array’s size and then allocate values:In this example, a loop is used to fill the array.
The flexibility in how you can declare and initialize arrays empowers programmers to adapt their code to various needs.
Dynamic Arrays in
Dynamic arrays in C#, unlike static arrays, can adjust their size during runtime. This feature is crucial when the number of items is not known before execution. The class is often used in such situations
Here’s how it works:
- Using the List Class: You can initiate a list similar to an array but it grows automatically. For example:
- Flexibility: Lists can grow as required. For instance, if you keep adding numbers, the list expands dynamically.
Using dynamic arrays is particularly advantageous when processing collections with uncertain lengths. Just remember that dynamic arrays may consume more memory due to their flexibility, so consider memory management as you design your application.
Important: Dynamic arrays are not strictly arrays but offer array-like functionalities, making them versatile for various programming scenarios.
Working with Array Methods
In C#, arrays are powerful tools for organizing and managing data. However, their true potential unfolds when you understand and utilize the methods available in the Array class. These methods can streamline operations and enhance your programming efficiency. Knowing them not only contributes to performance improvements but also simplifies the coding process.
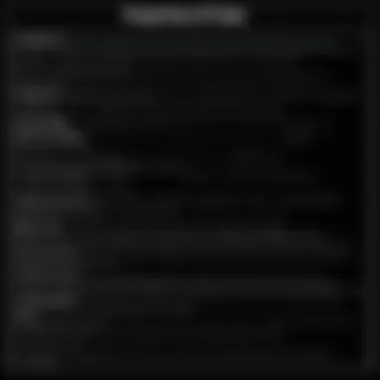
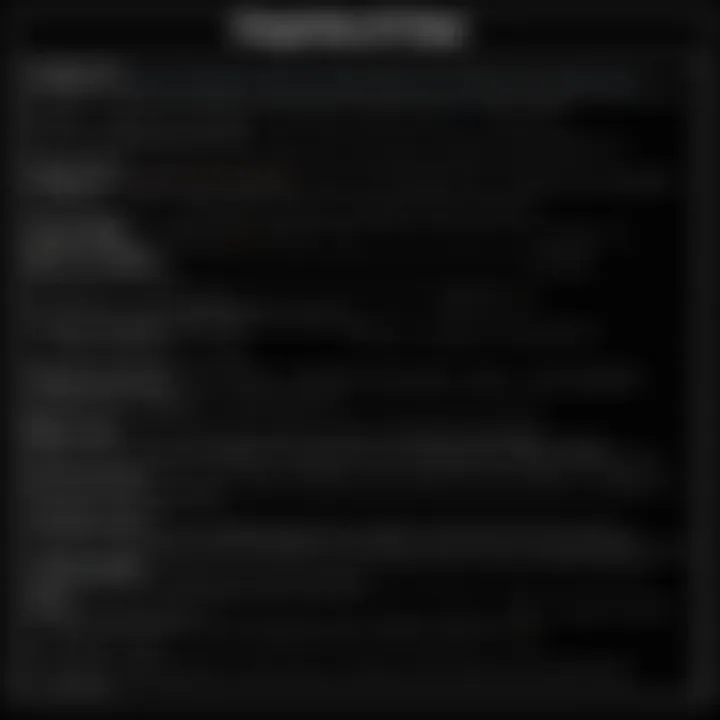
Key aspects of working with array methods include the ability to sort and manipulate data easily. This article will cover several vital methods that simplify common tasks with arrays. By understanding how to apply these methods effectively, programmers can avoid common pitfalls and improve the overall quality of their code.
Common Methods Available in the Array Class
Sort
Sorting is often essential for data presentation and algorithm efficiency. The Sort method allows for quick organization of array elements. It can handle both primitive types and custom objects, provided you implement IComparable. The key characteristic of Sort is its algorithm efficiency, often using quicksort, which benefits large datasets significantly.
Advantages of Sort include simplicity and speed, making it a popular choice for both beginners and experienced programmers. However, it does have a unique feature: it sorts the array in place, meaning the original order is lost. This requires caution if the original sequence is significant.
Reverse
The Reverse method offers a straightforward means to invert the order of elements in an array. This method is particularly useful in scenarios where you may need to traverse data in a backward manner. The key characteristic of Reverse is its ability to handle any array, regardless of type.
One benefit of Reverse is its efficiency in terms of computational resources. This method operates directly on the array without creating a new one. However, it also alters the original data structure, similar to Sort. Understanding this behavior is crucial when maintaining the integrity of your data.
Copy
Copying arrays is often necessary when data manipulation requires retaining the original set. The Copy method efficiently clones elements from one array to another. Its fundamental characteristic is its ability to manage shallow copies of the arrays.
This method stands out for its straightforward syntax and functionality. It provides flexibility in programming by allowing partial array copying. However, it's essential to understand that Copy does not perform deep cloning. This means, if the source array contains reference types, both arrays will reference the same objects.
Clear
The Clear method is useful for resetting an array to its default values without creating a new instance. It effectively sets specified elements of the array to their default type values, which is particularly beneficial for initializing lists before reuse.
Clear's primary benefit is memory efficiency, as it allows you to reuse existing arrays rather than creating new ones. However, it destroys the original data, which should be kept in mind during implementation. This method is best utilized when you are confident the original data is no longer needed or has been processed elsewhere.
Array Manipulation Techniques
After familiarizing yourself with the methods available in the Array class, it becomes essential to understand how to manipulate arrays efficiently. Array manipulation techniques can streamline coding practices and improve program performance.
Key techniques include item insertion, deletion, and overall modification of array content. Each method discussed in this section plays a unique role in ensuring that arrays are leveraged to their maximum potential, encouraging effective data management in C#.
By incorporating these methods into regular programming tasks, developers can augment their coding arsenal, leading to cleaner, effective, and more maintainable code.
Performance Considerations
In the realm of programming, the efficiency of data structures can significantly influence application performance. The Array class in C# is no exception. Understanding performance considerations is crucial for optimizing memory usage and reducing execution time. This section will dissect memory management alongside the efficiency of array operations to underscore how these factors can affect overall application performance.
Memory Management with Arrays
Memory management is a vital aspect of using arrays effectively. In C#, arrays are reference types, which means they are stored on the heap. This allocation can lead to increased overhead if not managed properly. When declaring an array, the amount of memory allocated is determined by its length. If the size is not properly anticipated, it can result in wasted memory space.
- Garbage Collection: C# employs garbage collection to manage memory automatically. When an array is no longer needed, the garbage collector can reclaim that memory. However, this process can introduce latency, especially in applications that frequently allocate and deallocate memory.
- Memory Fragmentation: Over time, allocating and releasing memory can lead to fragmentation. This can reduce performance when the program needs to allocate larger contiguous blocks of memory, as it may have to search for available space across the heap.
- Initialization Costs: Arrays require initial population of memory with default values. For large arrays, this can become time-consuming and can add to the overall latency of an application.
Mitigating these issues involves careful planning of array usage and size, along with a good understanding of how the garbage collector works.
Efficiency of Array Operations
The efficiency of array operations directly impacts the performance of an application. Arrays in C# support a variety of operations, such as accessing elements, sorting, and iterating. Each of these operations has its own performance characteristics.
- Accessing Elements: Accessing an element in an array is an O(1) operation. This means it is very efficient to retrieve data, making arrays suitable for scenarios where quick access is essential.
- Sorting Operations: When it comes to sorting arrays, the performance can degrade depending on the algorithm used. The built-in Array.Sort method uses a quicksort algorithm, which has an average time complexity of O(n log n). However, if the array is already sorted or nearly sorted, performance may improve.
- Iterating Through Elements: The speed of iterating through the elements in an array is also notable. Looping through elements with a simple for loop is generally fast, but can become less efficient if using complex logic inside the loop.
"Proper management of performance in array operations can lead to highly efficient applications that better utilize system resources."
To summarize, understanding the performance considerations around memory management and the efficiency of array operations is paramount when using arrays. It allows developers to create applications that are not just functional, but also responsive and efficient. This knowledge is essential for anyone engaging with C#, especially students and beginners who seek to build robust programs.
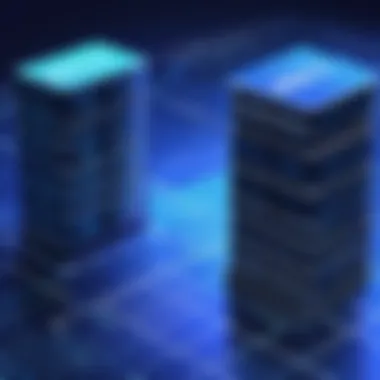
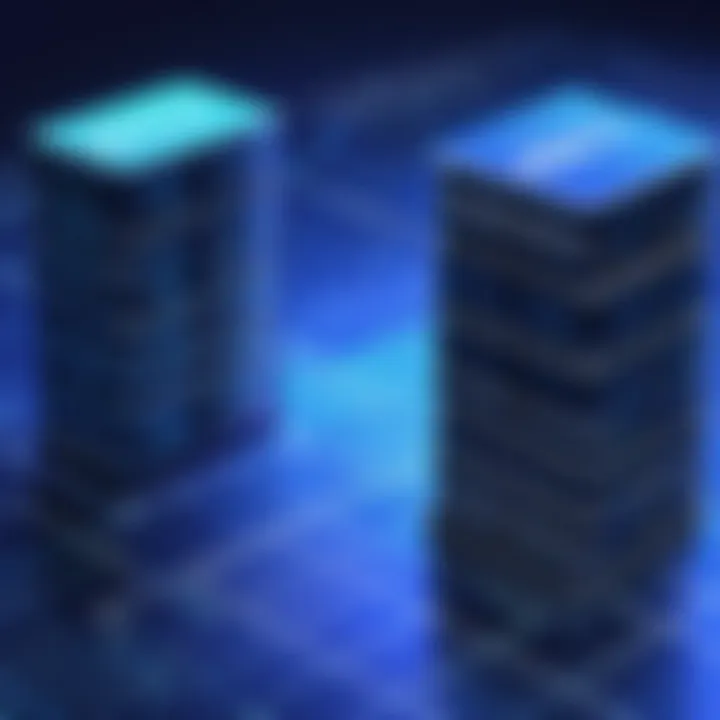
Best Practices for Array Usage
When working with arrays in C#, it’s critical to follow best practices to ensure optimal efficiency and maintainability. This section delves into some of those practices, highlighting their significance in real-world programming. It is essential to manage arrays wisely, as poor choices can lead to issues such as memory leaks, performance bottlenecks, and code that is difficult to read and maintain.
Avoiding Common Pitfalls
Many programmers encounter pitfalls when utilizing arrays. One common mistake is not initializing arrays properly. In C#, an array must be declared before it can be used. Failing to initialize an array can lead to runtime exceptions, causing programs to crash unexpectedly. Always declare an array's size during initialization to prevent this.
Another common pitfall is confused indexing. C# arrays are zero-based, meaning the first element is at index zero. This can lead to off-by-one errors if mismanaged. It’s important to validate indices when accessing elements to avoid exceptions that may lead to application crashes.
Moreover, be cautious when resizing arrays. Arrays in C# have a fixed size. If you need a dynamically-sized collection, consider using the class instead. Lists allow for automatic resizing and simpler management of data collections.
Additionally, avoid unnecessary copying of arrays. When passing arrays to methods, use references instead of creating duplicates. This practice improves performance as it saves memory and resources. When an array is passed to a method, any modifications will reflect in the original array, so make sure this behavior is desirable.
"The efficiency of array manipulation is heavily influenced by one’s approach to their usage. Small oversights can lead to larger problems down the line."
Leveraging Arrays in Real-World Applications
Arrays can serve various practical purposes in real-world applications. Their structured nature makes them ideal for numerous scenarios such as data storage and manipulation. For instance, consider an application that processes a series of monthly sales figures. An array can be used to store these values, allowing developers to perform calculations such as finding totals, averages, or trends over time quickly.
In environments like game development, arrays are often employed to manage collections of game entities, such as scores, player inventories, or even graphical elements. Utilizing arrays effectively can lead to enhanced performance and fluid gameplay experience.
Moreover, arrays can simplify sorting and searching operations. Using C# built-in methods like allows developers to organize data efficiently, which is crucial when handling an extensive data set.
Beyond simple storage, integrating arrays with powerful language features, such as LINQ, can lead to streamlined queries and manipulations of data, thus empowering developers to write cleaner, more efficient code.
Ultimately, understanding how to leverage arrays effectively in C# can significantly impact the usability and performance of applications, enhancing both the developer's and user's experience.
Advanced Array Techniques
Advanced array techniques are crucial for proficient programmers who wish to enhance their skills when working with arrays in C#. These techniques offer more efficient ways to manipulate and process data stored in arrays. It allows programmers to write cleaner, more maintainable code, especially when handling complex data structures. The use of advanced techniques can improve performance and simplify tasks that would otherwise require more time and effort. In this section, we will discuss two important aspects: using LINQ with arrays and combining arrays in C#. Both techniques are not only practical but also empower programmers to solve problems more effectively.
Using LINQ with Arrays
LINQ, or Language Integrated Query, is a powerful feature in C# that simplifies data manipulation. It provides a query syntax that can be used with different data sources, including arrays. By using LINQ with arrays, a programmer can perform complex queries in a succinct manner.
With LINQ, you can filter, project, and transform data easily. The syntax is both readable and intuitive, making array handling straightforward. For example, filtering an array to get only even numbers can be done elegantly with LINQ:
This code fetches even numbers from the array, showcasing how LINQ can make array manipulation smoother and more efficient. Additionally, LINQ methods can chain together, allowing for complex queries with minimal lines of code. Using LINQ not only optimizes array operations but also enhances code clarity, which is a priority for any programmer.
Combining Arrays in
Combining arrays is another advanced technique that can be immensely beneficial in various programming scenarios. When dealing with multiple arrays, merging them into a single array can simplify data management and retrieval. In C#, this can be achieved using several methods. The most common approach is to use the method provided by LINQ.
Here is a basic example of combining two arrays:
In this case, the method joins and , returning a new array containing all elements from both arrays. It saves time and reduces the complexity of managing individual arrays throughout your code. Moreover, using the method converts the resultant IEnumerable back to an array easily.
Combining arrays can streamline data processing tasks and make your code more manageable.
By mastering these advanced array techniques, programmers can greatly improve their coding abilities and develop more efficient algorithms, resulting in faster and more responsive applications.
Culmination
The conclusion occupies a vital role in any comprehensive exploration of the Array class in C#. It serves as the final opportunity to reflect on the critical aspects covered throughout the article. In this case, it synthesizes the essential points discussed, reinforcing the understanding of arrays and their manipulation in C#. The Array class is foundational for efficient data storage and retrieval, making it an indispensable topic for students and aspiring programmers.
Recap of Key Points
In summary, we have traversed various dimensions of the Array class. Key points include:
- Definition and purpose of arrays, which indicate their fundamental role in organizing data.
- An introduction to basic properties of the Array class, including length and indexing features that facilitate easy data access.
- Discussion of multi-dimensional arrays and jagged arrays, highlighting their structural differences and respective use cases.
- A hands-on approach to creating arrays, with particular focus on declaring, initializing, and dynamic arrays.
- An overview of common methods available in the Array class, like Sort and Copy, that ease data management further.
- Insight into performance considerations, including memory management and the efficiency of operations, which is crucial for optimizing application performance.
- Best practices to avoid common pitfalls when working with arrays, which can drastically improve coding outcomes.
- Advanced techniques, like using LINQ with arrays and combining them, which enrich the programmer’s toolkit.
Future of Array Management in
Looking ahead, the management of arrays in C# is poised for continued evolution. As programming paradigms shift towards more dynamic and flexible approaches, arrays will adapt, possibly integrating more seamlessly with newer data structures and functionalities. The advancement of technology indicates a growing need for more complex data manipulation capabilities.
Several trends could shape the future of array management:
- Increased integration with LINQ: As LINQ continues to gain popularity, we may see deeper connections that further simplify array querying and manipulation.
- Performance improvements: Ongoing enhancements in the underlying runtime may yield faster operations and better memory allocation strategies for arrays.
- Hybrid data structures: The evolution might also lead to hybrid structures that combine the efficiency of arrays with the flexibility of other collection types, optimizing both performance and usability.