Master TensorFlow with Python: A Beginner's Guide
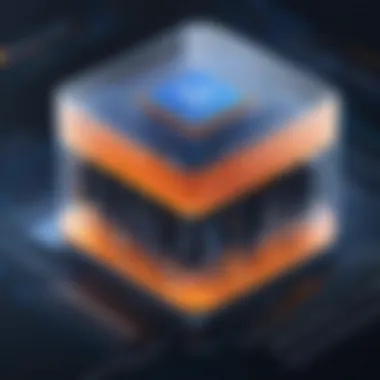
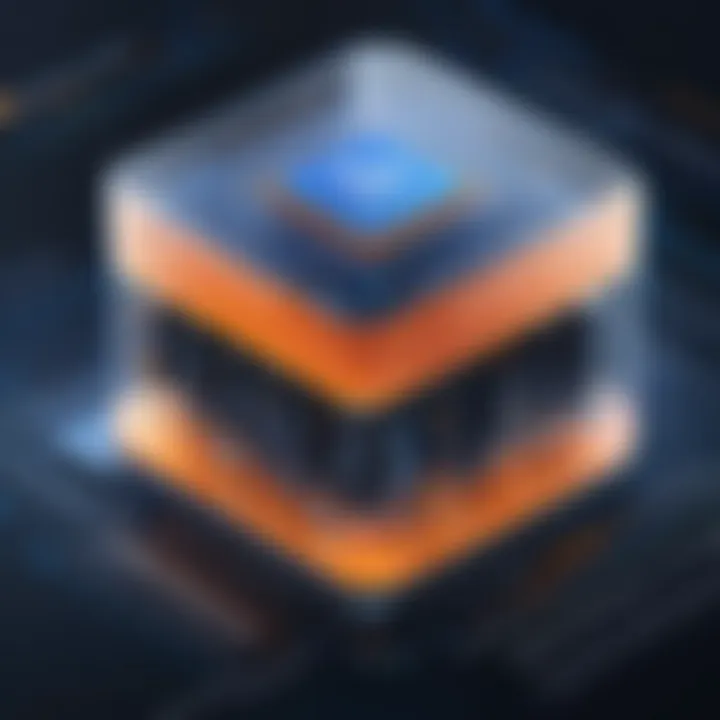
Intro
TensorFlow is an open-source library widely used for machine learning and deep learning applications. Developed by the Google Brain team, it provides a framework that lets developers create complex neural networks for tasks such as image recognition, natural language processing, and predictive analytics. This comprehensive guide seeks to illuminate TensorFlow's core features, installation, and wide-ranging applications in Python programming.
As machine learning continues to evolve, understanding TensorFlow becomes vital for anyone looking to harness its capabilities, whether for academic purposes or industry applications. This guide is structured to cater to both beginners and those with some programming experience, enabling the exploration of TensorFlow’s architecture and functionalities in a straightforward manner.
Preface to TensorFlow
When exploring TensorFlow, it is essential to grasp its foundational concepts and how it fits within the wider realm of Python programming.
History and Background
TensorFlow was introduced in November 2015 and has since been adopted by industries for its flexibility and scalability. Initially designed for internal Google use, it has grown into a robust library, capable of supporting large-scale machine learning workloads.
Features and Uses
TensorFlow offers several important features that enhance its usability:
- Flexibility: Users can build complex models through high-level APIs, such as Keras, or engage with low-level APIs for fine-tuning.
- Scalability: It can efficiently run on a single CPU or scale up to thousands of GPUs.
- Ecosystem: It has a vast ecosystem, which includes TensorBoard for visualization and TensorFlow Lite for mobile platforms.
Popularity and Scope
The demand for TensorFlow has surged as machine learning becomes a staple in various sectors like healthcare, finance, and entertainment. The community surrounding TensorFlow is also very active, offering numerous forums, tutorials, and user-friendly documentation.
Basic Syntax and Concepts
Delving into TensorFlow involves not only understanding the library but also having a firm grasp of Python language basics. Here, we cover essential programming concepts that aid in using TensorFlow effectively.
Variables and Data Types
Variables in Python are used to store information, and they can take various forms such as integers, floats, lists, and dictionaries. Understanding data types is crucial in TensorFlow, as different types can impact model performance. For instance:
Operators and Expressions
Python supports several operators including arithmetic, comparison, and logical operators. Mastering these is necessary for crafting computational expressions that leverage TensorFlow's capabilities.
Control Structures
Control structures include if statements and loops that allow for decision-making and iteration. These are essential when defining model behaviors and processing data dynamically.
Advanced Topics
Once foundational skills are established, learners can progress to more advanced aspects of TensorFlow and programming.
Functions and Methods
Functions play a central role in structuring code efficiently. TensorFlow provides numerous built-in functions for operations like mathematical computations and data manipulation. Custom functions can also be defined.
Object-Oriented Programming
Object-oriented programming (OOP) can be beneficial when building complex applications. In TensorFlow, OOP is used to create reusable model classes and components.
Exception Handling
Exception handling in Python is vital for managing errors gracefully. This ensures the robustness of Tensorflow applications against unexpected inputs or situations.
Hands-On Examples
Practical engagement is key to mastering TensorFlow. Here, we will explore simple and intermediate projects to solidify understanding.
Simple Programs
Starting with basic functionality, beginners should try creating straightforward programs, such as implementing a basic linear regression model using TensorFlow.
Intermediate Projects
More complex projects could involve creating different neural network architectures for classification problems, allowing users to gain deeper practical insights.
Code Snippets
Utilize code snippets to illustrate practical implementations easily. Sample codes facilitate learning by allowing users to see immediate applications of the concepts covered above.
Resources and Further Learning
To continue the learning journey, it's important to utilize various resources available for TensorFlow users.
Recommended Books and Tutorials
Books such as "Hands-On Machine Learning with Scikit-Learn, Keras, and TensorFlow" provide in-depth exploration of machine learning practices with TensorFlow.
Online Courses and Platforms
Numerous courses are available online, including those from Coursera and edX, offering structured learning paths.
Community Forums and Groups
Joining forums like Reddit’s Machine Learning community or TensorFlow's own groups on Facebook allows learners to connect with other enthusiasts, share ideas, and seek guidance.
"TensorFlow is not just a library; it is a community that continues to influence the direction of machine learning research and trends."
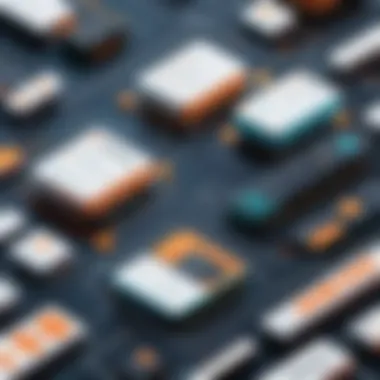
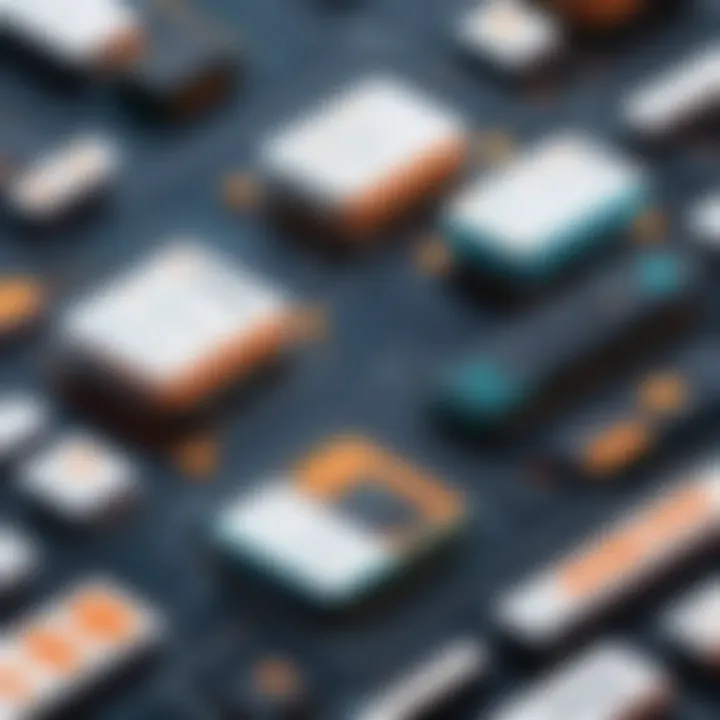
Preamble to TensorFlow
In the realm of machine learning and deep learning, TensorFlow occupies a pivotal position. This open-source library, developed by Google, provides an extensive framework that simplifies the complexities of building and deploying machine learning models. The importance of TensorFlow lies not only in its versatility but also in its capability to cater to a diverse audience, ranging from novices to experts in the field. This section aims to shed light on vital aspects of TensorFlow, including its definitions, key features, and various applications.
What is TensorFlow?
TensorFlow is an open-source library designed for numerical computation. It leverages data flow graphs where nodes represent mathematical operations, and edges represent the data connections between them, often referred to as tensors. Tensors are the fundamental units of TensorFlow, and they serve to encapsulate data in a variety of forms. Initially launched in 2015, TensorFlow has evolved significantly, becoming a prominent tool for implementing machine learning algorithms. Its flexibility allows developers to create sophisticated models seamlessly, while the robust community support contributes to its continual advancement.
Key Features of TensorFlow
Several notable features set TensorFlow apart from other machine learning libraries:
- Flexibility: It supports production deployment across various platforms such as mobile, web, and cloud environments.
- Scalability: TensorFlow can operate on multiple CPUs and GPUs, facilitating large scale machine learning.
- Ecosystem: With tools like TensorBoard for visualization, TensorFlow Lite for mobile and embedded devices, and TensorFlow Serving for deploying models in production, users have a comprehensive suite of resources at their disposal.
- High-Level APIs: TensorFlow provides high-level APIs such as Keras, which simplify model building and training processes, making it more accessible for beginners.
These features underscore TensorFlow's capability to address a wide spectrum of machine learning needs, empowering users to build complex networks with relative ease.
Applications of TensorFlow
TensorFlow's applications are vast and varied, impacting many fields:
- Image Recognition: TensorFlow is widely used in computer vision tasks, enabling applications such as facial recognition, object detection, and more.
- Natural Language Processing: The library facilitates tasks related to language understanding and generation, such as translation and sentiment analysis.
- Healthcare: TensorFlow assists in analyzing medical data, predicting diseases, and enhancing medical imaging techniques.
- Finance: In finance, it enables fraud detection, stock price prediction, and risk assessment through various machine learning models.
In summary, the introduction to TensorFlow contextualizes its role in modern machine learning. Understanding its foundational concepts, core features, and practical applications enables users to leverage TensorFlow effectively, an essential step for their journey in the field of artificial intelligence.
Setting Up Your Environment
Establishing the right environment to work with TensorFlow is fundamental for success. Without proper setup, you may face installation errors, performance issues, or even challenges when training models. This section guides you through the essential requirements, installation processes on various operating systems, and helps ensure a smooth experience with TensorFlow.
System Requirements
Before installing TensorFlow, it’s crucial to understand the required system specifications. The minimum requirements will vary based on your intended use of TensorFlow. However, here are the general requirements:
- Operating System: Windows, macOS, or Linux.
- Python Version: Ensure you have Python 3.7 to 3.10. TensorFlow does not support versions outside this range.
- Memory: A minimum of 4GB RAM is advised; however, 8GB or more is optimal for larger models.
- Disk Space: At least 1GB free space is necessary for installation.
Moreover, for GPU support, you will need a CUDA-capable NVIDIA GPU with the appropriate setup.
Installing TensorFlow on Windows
For Windows users, installing TensorFlow can be done via the pip package manager, which simplifies the process. Follow these steps:
- Install Python: If it is not already installed, download and install Python from the Python website.
- Open Command Prompt: Search for "cmd" to open the command prompt.
- Upgrade pip: It’s good practice to ensure you have the latest version. Run the following command:
- Install TensorFlow: Execute this command in the command prompt:
- Verify Installation: After installation, check that TensorFlow is correctly installed by running:
Following these steps will get TensorFlow up and running on your Windows machine.
Installing TensorFlow on macOS
The installation process on macOS is similarly straightforward. Perform the following steps:
- Install Python: If you do not have Python, download it from the Python website.
- Open Terminal: Use the Spotlight Search to find and open the Terminal application.
- Upgrade pip: Make sure pip is up to date by running:
- Install TensorFlow: Enter the following command:
- Verify Installation: Use this command to verify:
Once these steps are completed, TensorFlow should be installed correctly on your macOS.
Installing TensorFlow on Linux
Linux users also have a straightforward path to install TensorFlow. Here’s how to do it:
- Install Python: You can either install from your package manager or download it from the Python website.
- Open Terminal: Launch the terminal application.
- Upgrade pip: Update pip as follows:
- Install TensorFlow: Input the installation command:
- Verify Installation: Finally, run the command:
Upon finishing these instructions, you will have TensorFlow ready for use on your Linux system.
Important: Always refer to the official TensorFlow installation guide for the most accurate and updated instructions.
By carefully following these steps, you ensure your environment is ready for effective machine learning development using TensorFlow.
Basic TensorFlow Concepts
Understanding the basic concepts of TensorFlow is crucial for anyone looking to leverage this powerful library for machine learning and deep learning projects. These foundational elements not only facilitate the use of TensorFlow but also enhance comprehension of its more advanced features. By grasping these concepts, users are better equipped to troubleshoot issues, optimize models, and implement robust machine learning solutions.
Tensors Explained
Tensors are the fundamental building blocks in TensorFlow. A tensor is simply a multi-dimensional array. It can take various forms, such as scalars (0-dimensional), vectors (1-dimensional), matrices (2-dimensional), or higher-dimensional structures.
- Scalars: A single number, for example, 5.
- Vectors: An array of numbers, like .
- Matrices: A 2D arrangement, such as .
TensorFlow allows the manipulation of these structures efficiently. The ability to handle tensors enables developers to perform complex mathematical operations across large datasets. A clear understanding of tensors paves the way for effective machine learning model building.
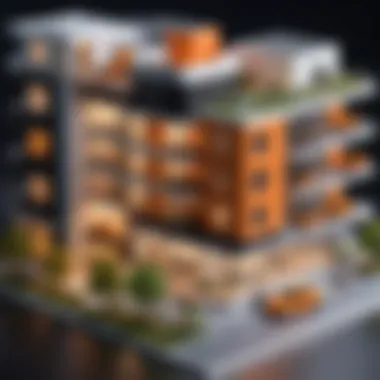
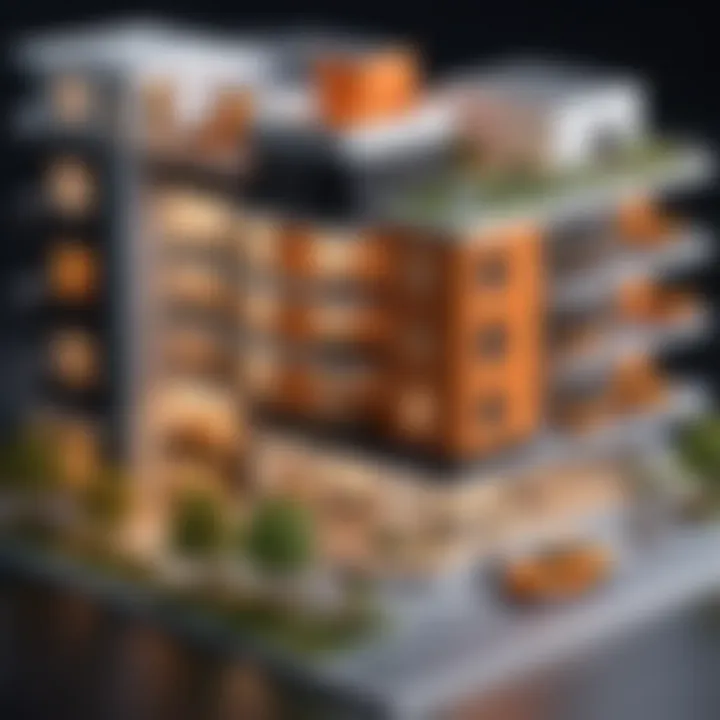
Graph Computation in TensorFlow
Graph computation is a unique aspect of TensorFlow, allowing users to visualize and organize the flow of data. In a computation graph, nodes represent operations, while edges represent the tensors that flow between these operations. This structure facilitates optimization, as TensorFlow can rearrange operations to maximize performance.
Using a graph can also aid in debugging and visualizing how data transitions through different layers of a model. A simple example can illustrate this:
- Define the operations: Nodes can perform calculations, transformations, or any operation on tensors.
- Connect nodes: Edges represent data flow from one operation to another.
- Execute the graph: Once the graph is defined, TensorFlow can execute it efficiently.
Sessions and Execution
In earlier versions of TensorFlow, a session is a runtime environment necessary to execute a graph. Sessions encapsulate the control and state of the TensorFlow runtime. Within a session, a user can run calculations based on the defined operations and tensors.
Although recent updates have introduced eager execution, making operations run immediately without needing a session, understanding sessions remains essential for legacy projects and when diving into specific optimizations. In practice:
- Creating a session: You can initiate a session using in v1.x versions.
- Running an operation: Execute a graph with .
Sessions simplify the complexity of managing resources when running big computations.
Overall, mastering these basic concepts in TensorFlow lays a solid foundation for anyone searching to explore more complex applications of this library. Understanding tensors, the architecture of computation graphs, and the role of sessions in execution provides the essential toolkit for further study and experimentation in machine learning.
Building Your First TensorFlow Model
Building your first TensorFlow model is a critical step in understanding how to work with this powerful library. It serves not only as an introduction but also as a practical engagement that allows you to apply theoretical concepts in a tangible manner. The process of model building is essential because it encapsulates the principles of both creating neural networks and training them using data. In this section, you will discover how to construct models that can learn from data and make predictions.
This foundational skill equips users to tackle real-world problems through machine learning. Understanding model structure and behavior enhances your capacity to innovate solutions in various domains. The benefits of getting hands-on experience with model building are numerous, and some important considerations will be outlined as you proceed.
Creating a Simple Linear Model
A simple linear model is a great starting point for anyone learning TensorFlow. It serves to illustrate the fundamental aspects of model creation in a form that is easy to grasp. This model predicts outcomes based on a linear relationship between input features and target values.
To create a simple linear model in TensorFlow, you need to define the model architecture. Here’s a small example:
This code initializes a simple model with one layer. The layer indicates that it is a dense fully connected neural network layer that takes input of size one.
Training Your Model
Training is where the model learns from the dataset provided. The process involves feeding data through the model and adjusting the model parameters based on the error in predictions. TensorFlow simplifies this through its callable methods.
To train the model you created, you typically need to choose a loss function and an optimizer. For instance:
In this snippet, the model uses stochastic gradient descent (sgd) as an optimizer and mean squared error as the loss function. The method trains the model for a specified number of epochs, which indicates how many times the learning algorithm will work through the entire training dataset.
Evaluating Model Performance
After training, it is crucial to evaluate how well the model has performed. This step ensures that the model generalizes appropriately to unseen datasets. Model evaluation typically involves assessing various metrics depending on the nature of the problem—classification or regression.
Using TensorFlow, you can evaluate the model with:
This command outputs the loss metric, allowing you to measure the model's performance. Based on these results, fine-tuning may be necessary to improve accuracy. Key metrics to consider include accuracy, precision, recall, and the F1 score for classification tasks, or mean absolute error and R² score for regression tasks.
Remember that the effectiveness of your model can only be maximized by continued refinement and testing against different datasets.
Understanding these aspects of TensorFlow makes it easier to build more complex models as your skill level increases.
Advanced TensorFlow Techniques
In the realm of machine learning and artificial intelligence, the proper utilization of advanced techniques is crucial for creating models that not only function effectively but also optimize performance across various types of datasets. The section on advanced TensorFlow techniques explores the intricate tools and methodologies that enhance data handling and model building. By employing these techniques, practitioners can harness the full potential of TensorFlow, resulting in more accurate predictions and improved efficiency in projects.
Preface to Keras
Keras is a high-level neural networks API that runs on top of TensorFlow. It allows for the fast development of deep learning models with a user-friendly and modular approach. This framework is particularly valuable for those who are getting started with deep learning as it simplifies the process significantly. Keras supports multiple backends, making it versatile and adaptable to various hardware configurations.
One primary benefit of using Keras within TensorFlow is its focus on simplicity and ease-of-use. Developers can quickly prototype models and test different architectures without getting bogged down by the underlying complexities of TensorFlow itself. This rapid development ability allows researchers and practitioners to iterate faster, leading to quicker insights and outcomes.
Building Deep Neural Networks
Deep neural networks (DNNs) are essential for tackling complex problems, such as image recognition and natural language processing. Constructing DNNs in TensorFlow requires a solid understanding of the layers, activation functions, and optimization techniques.
When building a DNN, a typical structure includes multiple hidden layers, each designed to learn specific features from the input data. A common framework is to start with an input layer, followed by hidden layers, and finally a output layer. Each layer uses an activation function, like ReLU or sigmoid, to introduce non-linearity to the model.
In TensorFlow, one can leverage various tools for constructing these networks. The class simplifies the stacking of layers and allows for quick adjustments to configurations. Here is an example of how to construct a simple model:
Building a DNN requires careful consideration of the architecture to achieve a balance between complexity and performance. Too many layers can lead to overfitting, while too few may underfit the data. It is imperative to test out different configurations and assess their effectiveness using training and validation datasets.
Model Optimization Strategies
Optimization is key to enhancing the performance of machine learning models. Several strategies are employed to fine-tune models for better accuracy and efficiency.
- Learning Rate Adjustment: The learning rate acts as a control knob for the gradual improvement of the model. Dynamic learning rates, such as those using callbacks, help in converging faster.
- Regularization Techniques: Implementing regularization techniques like dropout or L2 regularization can be effective in reducing overfitting. These methods help in ensuring that the model generalizes well even on unseen data.
- Batch Normalization: This method stabilizes learning by normalizing activations. It tends to accelerate training and can lead to a better overall model.
- Early Stopping: This involves monitoring the validation loss during training and halting when the loss begins to increase. This prevents unnecessary overfitting and preserves the model’s predictive power.
Consideration of these strategies is crucial for improving model performance. Each project may call for different combinations of techniques, so practitioners must evaluate and adapt their approach accordingly.
"A well-optimized model does not just perform well on training data but maintains its predictive capabilities on new data."
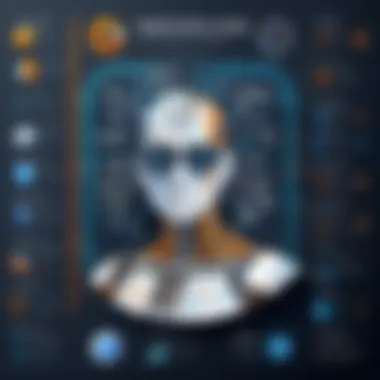
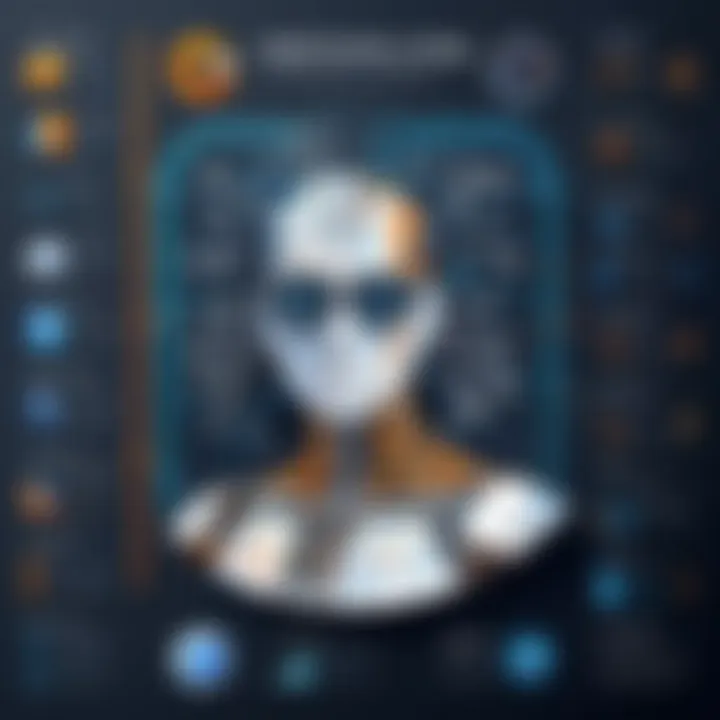
By exploring these advanced TensorFlow techniques, users can enhance their understanding and application of TensorFlow, paving the way for more sophisticated machine learning solutions.
Utilizing TensorFlow for Image Recognition
Image recognition is a crucial application of machine learning. By leveraging TensorFlow, developers can create models that can identify objects, classify images, and extract information from visual data. In today's digital landscape, the ability to process images is invaluable. Businesses utilize image recognition for various purposes, including security, retail, media, and social media monitoring.
The importance of this topic lies not only in its practical applications but also in the underlying technology that drives it. TensorFlow offers optimized libraries and tools that facilitate the development of complex algorithms for high performance. This section explores the essential steps to harness TensorFlow for image recognition, focusing on preparing datasets, building models, and deploying those models into real-world applications.
Preparing Your Dataset
Successful image recognition starts with a well-prepared dataset. The quality and diversity of your training data significantly affect your model's performance. Here are some crucial aspects to consider when preparing your dataset:
- Data Collection: Gather images that represent the categories you want to classify. Make sure to include various angles, lighting conditions, and backgrounds.
- Data Labeling: Each image should be accurately labeled. Use tools like LabelImg or RectLabel for this task. Labels should be consistent and representative of the object in the image.
- Data Augmentation: To improve your model's robustness, augment your dataset. Techniques such as rotation, flipping, and adjusting brightness can create variations without the need for additional data collection.
- Splitting the Dataset: Divide your dataset into training, validation, and testing sets. A common practice is to use 70% for training, 15% for validation, and 15% for testing.
Building the Image Classification Model
After the dataset is prepared, the next step involves constructing the image classification model. TensorFlow, in conjunction with its Keras API, makes this process more accessible. A simple architecture might look like this:
- Import Required Libraries:
- Create a Sequential Model:
- Add Layers: Start with convolutional layers followed by pooling layers. For example:
- Flatten and Output Layers: Finally, flatten the output and add dense layer for classification.
- Compile the Model: Use an appropriate optimizer, loss function, and metrics:
- Train the Model: Fit the model to your training data.
Make sure to monitor the training process using validation data to avoid overfitting.
Deploying the Model
After training the model, deployment is the final phase. You can deploy the model in various environments, depending on your application. Here are some common options:
- Web Application: Use TensorFlow.js to integrate the model into a web application. This allows real-time image recognition directly in the browser.
- Mobile Apps: Convert the model using TensorFlow Lite for mobile deployment. This is ideal for Android and iOS applications, ensuring efficient performance on mobile devices.
- Cloud Deployment: Host the model on cloud platforms like Google Cloud Platform or AWS, making it accessible via REST APIs. This allows for scalable solutions without needing to manage the infrastructure.
Troubleshooting Common Issues
In any journey through the intricacies of machine learning, particularly when using TensorFlow, users may encounter various pitfalls. Addressing trouble spots proactively can save time and frustration. This section will illuminate common issues that arise when working with TensorFlow, equipping users with the knowledge to effectively troubleshoot and resolve such challenges.
Installation Errors
Installation is a critical first step in any project. The process of installing TensorFlow may present errors that can derail progress if not properly managed. Common installation errors include incompatibility with system requirements, issues with package management such as pip, and conflicts between different versions of dependencies. It is essential to carefully adhere to installation instructions pertinent to the operating system in use.
Here are some frequent installation errors you might face:
- Version Conflicts: New versions of TensorFlow may have dependencies that conflict with existing libraries.
- CUDA/CuDNN Issues: For those leveraging GPU support, incorrect setups for CUDA and CuDNN can prevent TensorFlow from running altogether.
To mitigate these problems, verify that your environment meets the specified requirements and the installation command aligns with your configurations. Additional tips include creating a virtual environment dedicated to TensorFlow projects. This method keeps your workspace clean and devoid of conflicts with other projects or libraries.
Performance Optimization Issues
Once TensorFlow is successfully installed, users may face performance challenges during model training or data processing. Optimization is vital to ensure resources are utilized effectively. Slow training times or high memory usage are common signs of performance issues.
Some factors to consider include:
- Batch Size: Experiment with different batch sizes to find an optimal balance for your specific hardware.
- Data Pipeline: Utilizing TensorFlow's data API can significantly enhance data input speeds, ensuring your model is not waiting on data.
- Hardware Utilization: Check if your CPU or GPU is being fully utilized. Performance profiling tools can help identify bottlenecks in processing.
To optimize performance, it is advisable to monitor resources actively and adjust parameters dynamically. Fine-tuning these elements will lead to improved training times and overall efficiency in model performance.
Debugging Model Training Problems
In the realm of machine learning, debugging is often an inevitable part of the development lifecycle. Common issues during model training may include overfitting, underfitting, or convergence failures. Identifying the root causes of these problems requires a methodical approach.
Here are key strategies for effectively debugging model training:
- Review Model Architecture: Ensure the neural network’s design is suitable for the task at hand. Complex models may require adjustments based on data size and diversity.
- Loss Function Monitoring: Keep an eye on loss values throughout the training process. Sudden spikes or stagnation can indicate hooking issues.
- Learning Rate Fine-tuning: Adjust the learning rate as it is crucial for proper convergence. Too high a rate can cause overshooting, while too low can slow down learning significantly.
"Debugging is like being the detective in a crime movie where you are also the murderer." Unpacking these errors leads to more effective corrections and refined models.
By establishing a keen awareness of common pitfalls in installation, performance, and training, users can cultivate a robust foundation for their TensorFlow projects. Each challenge presents an opportunity for growth and learning, fostering skills that extend beyond TensorFlow itself.
Further Resources for Learning TensorFlow
In the complex landscape of machine learning, having accessible resources can significantly enhance your understanding and proficiency in TensorFlow. This section discusses various avenues for further learning, including books, online courses, community forums, and official documentation. These resources serve not only to bridge gaps in knowledge but also to provide continual learning opportunities as TensorFlow evolves.
Books and Online Courses
Books and online courses are pivotal in structured learning. They often present concepts in a coherent and progressive manner, making them essential for both beginners and those at a more advanced level. Recommended books include "Deep Learning with Python" by Francois Chollet, which connects theory with practical code examples using Keras and TensorFlow. Further, "Hands-On Machine Learning with Scikit-Learn, Keras, and TensorFlow" by Aurélien Géron offers a comprehensive overview of building effective machine learning systems. These texts provide a solid theoretical foundation along with practical exercises.
Online learning platforms, such as Coursera and Udacity, offer tailored courses on TensorFlow. Many of these courses feature hands-on projects and quizzes, allowing students to apply what they learn in real-time. For instance, Coursera's "TensorFlow in Practice" specialization includes courses by Andrew Ng, a distinguished leader in the field. Such courses can accelerate learning by providing direct feedback from instructors.
Community and Forums
Communities and forums play a crucial role in fostering discussions and clarifying doubts. Platforms like Reddit and Stack Overflow create vibrant spaces where learners can exchange ideas, share experiences, and seek guidance. Engaging in discussions or even reading through the questions can provide insights that documents sometimes overlook. Joining TensorFlow-specific groups on platforms like Facebook can also connect individuals with shared interests and experiences.
In addition, TensorFlow's own community forum serves as an excellent resource for troubleshooting and collaboration. Users can ask questions, provide answers, and contribute to discussions, making it an important part of the learning ecosystem. Connecting with peers can also encourage accountability and motivation throughout the learning journey.
Official TensorFlow Documentation
The official TensorFlow documentation is an indispensable resource. It provides in-depth information regarding APIs, modules, and functionalities available in TensorFlow. Navigating through comprehensive guides and tutorials can deepen understanding of the intricacies involved in TensorFlow. Keeping abreast of the latest updates through the documentation ensures users make use of the most current features and best practices.
It's crucial to regularly refer to this documentation, particularly when implementing complex models or troubleshooting issues. The guides provided not only demonstrate how to use various TensorFlow components but also offer examples that highlight how these components interact.
"Documentation is not just for beginners. It should be a part of every user's toolkit, regardless of experience level."
By leveraging these resources, users can cultivate a robust understanding of TensorFlow. Continuous learning in this field is necessary due to the rapid evolution of technology. Making use of these provided materials will empower students and programmers to stay informed and skilled.