Mastering Python Function Documentation Techniques
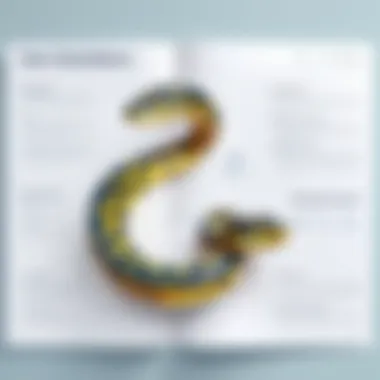
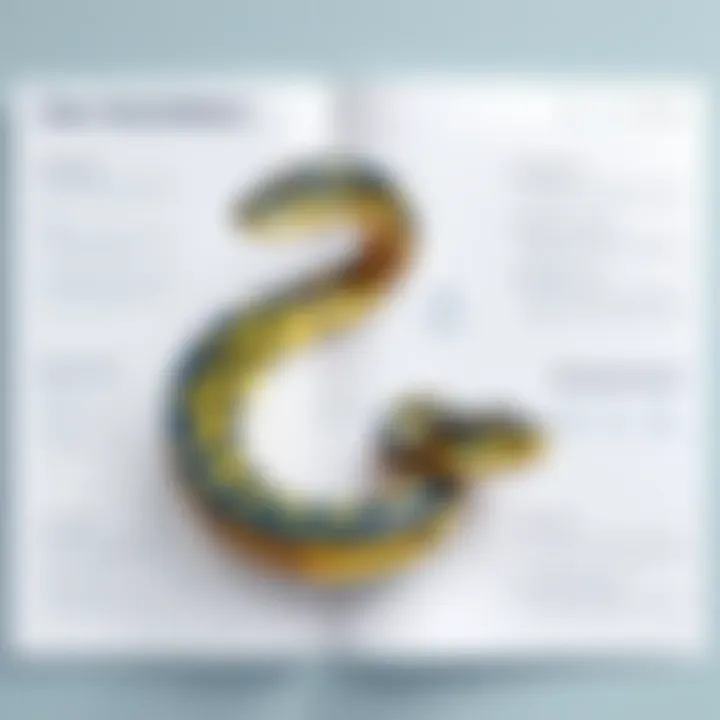
Intro
When we talk about Python programming, we are discussing much more than just writing lines of code. Effective documentation serves as a critical backbone in any codebase, guiding current and future developers through the logic and function of the code itself. This art of documenting functions in Python can often lead to smoother projects, increased productivity, and, importantly, better collaboration among team members.
In the coming sections, we will explore various angles of Python function documentation. We will dive into best practices, common pitfalls, and even tools that can help elevate your documentation game. Have you ever stared at someone else's code with the same expression as a deer caught in headlights? Documentation can prevent that discomfort and confusion. Whether you are a novice just starting out or someone with a bit more experience looking to refine your skills, understanding the nuances of documenting your Python functions is vitally important.
Letâs unfold the discussion by understanding a bit about this versatile programming language itself.
Prolusion to Python Function Documentation
When it comes to programming, clear communication is just as crucial as writing effective code. Documentation, especially in Python functions, serves as a bridge between the code creator and the user. Failing to recognize this can lead to situations where code is misunderstood or misused. In the convoluted world of software development, effective documentation is essentially an unsung hero, facilitating smoother collaboration and long-term maintenance.
Importance of Documentation
It's easy to overlook documentation when you're knee-deep in code, but don't underestimate its significance. Here are a few reasons why documentation is paramount:
- Clarity: Well-documented code makes it easier for others (or even yourself at a later date) to grasp the functionality without deciphering the inner workings.
- Legacy Maintenance: Projects can often linger for years. Proper documentation can save considerable time when revisiting old code.
- Onboarding: New team members can ramp up faster when they have access to comprehensive documentation, removing the learning curve and confusion.
"Documentation isnât just a formality; itâs a lifeline for anyone interacting with the code."
If you think of your code as a beautifully designed house, documentation is like the instruction manual that guides a new owner through its features. Without it, one might accidentally break the front door trying to understand its function. So, donât simply write code that works; write code that speaks.
Common Misconceptions
There are a few pitfalls that many fall into when it comes to function documentation:
- Documentation is Redundant: Some assume that if the code is complex, it should in itself be self-explanatory. This is a slippery slope. Complexity can lead to misinterpretation, and without documentation, users can get lost in the details.
- Only for Public Functions: Often, there's the misconception that documentation is only necessary for public APIs or libraries. On the contrary, private functions also deserve adequate explanations, especially in collaborative environments.
- One-Time Effort: Some believe that writing documentation is a one-and-done task. Yet documentation should evolve alongside the code. Changes in functionality or optimizations demand corresponding updates in documentation.
In addressing these misconceptions, itâs crucial to shift the perspective from viewing documentation as an optional afterthought to considering it an integral element of programming. Striving for clarity and precision should always come hand-in-hand with coding efforts.
Understanding Docstrings
In the realm of Python programming, docstrings hold a crucial position. They are not merely a formality; rather, they serve as a wellspring of clarity for both the original developers and those who come across the code later. This section will delve into what docstrings are, their structure, and the diferentes types available, all essential for anyone keen on writing impeccable function documentation.
Definition and Purpose
Docstrings, or documentation strings, are special comments contained within Python functions. They explain what a function does, its parameters, the returned values, and any exceptions it might raise. Think of a docstring as a name tag for your function - it provides necessary context for those who encounter it.
Function documentation enhances code readability, fosters collaboration, and simplifies debugging. Clear documentation aids teams in comprehending the intent behind each function, significantly reducing the learning curve for new cohort members. In short, effectively written docstrings can save hours, if not days, when it comes to understanding complex codebases.
Structure of Docstrings
To craft docstrings that are both informative and easy to digest, adhering to a specific structure is paramount. Typically, a docstring starts with a brief description of the function's purpose. Then, it outlines the parameters, what the function returns, and any exceptions that may arise.
The best practice suggests starting with a one-line summary, followed by more detailed explanations. This offers a quick glance at what to expect, while still providing depth for those who need it. Proper indentation and consistent formatting make docstrings even more efficient.
Types of Docstrings
Now, let's see the types of docstrings available and how they each serve their purpose in documentation.
Single-line Docstrings
Single-line docstrings are just that: concise, typically only one line long. This type is often used for simple functions that donât require extensive descriptions. For instance:
The charm of single-line docstrings lies in their simplicity. Since they're easy to read, they work particularly well when the function's purpose is straightforward. However, they may lack the depth needed for more complex functions. When used correctly, they can effectively communicate the intent without overwhelming the reader.
Multi-line Docstrings
On the other hand, multi-line docstrings are ideal for functions that need more elaborate explanations. They allow for a detailed overview of the function, parameters, return values, and possible exceptions in a structured manner. For example:
The standout feature of multi-line docstrings is their versatility. They can provide enough context, making them an excellent option for intricate functions. The downside could be that if not formatted well, they may deter the reader. Clarity is the name of the game here.
Docstring Formats
There are various formats to structure docstrings, each with its merits. Common formats include the reStructuredText format and Google Style. Each has a unique style that caters to different needs, whether you prefer a more minimalistic approach or extensive documentation.
Choosing a docstring format primarily depends on personal preference and project requirements. It's essential to pick one and stick with it throughout the project to ensure consistency. The right format can dramatically enhance the readability of your documentation, leading to improved collaboration and understanding.
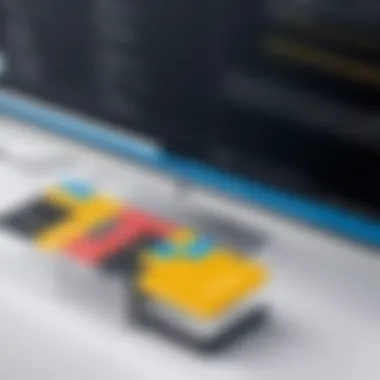
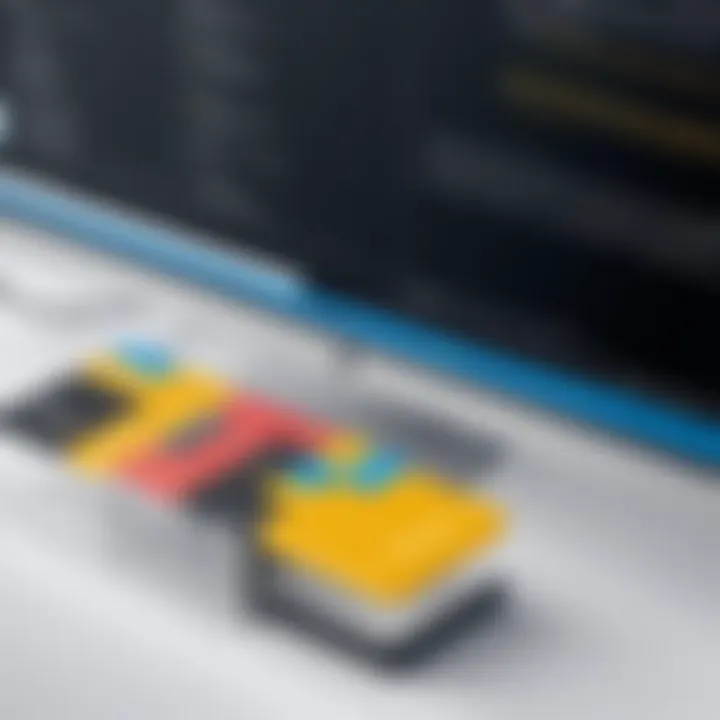
Ultimately, by grasping the types of docstrings and how to structure them, one lays the foundation for effective function documentation. Well-documented code not only serves the original programmer but also assists future developers who might need to work with the existing codebase.
Docstring Conventions in Python
When it comes to crafting effective Python function documentation, adhering to established conventions is crucial. Docstring conventions not only provide consistency across the codebase but also facilitate easier understanding among developers. Whether youâre working solo or as part of a large team, these guidelines assist in maintaining clarity and improving the quality of the documentation.
Remember, good documentation acts like a beacon; it guides users through your code more seamlessly. Clear documentation raises the overall standard of your programming practices, boosts collaboration, and allows future maintainers to grasp your work quickly.
PEP Guidelines
PEP 257 stands for Python Enhancement Proposal 257, and it is a cornerstone for documenting Python code. It lays out the primary standards for writing docstrings. Here are some key points to keep in mind:
- Docstring Placement: The docstring should be the first statement in the function, class, or module.
- Triple Quotes: Always use triple quotes for docstrings, even for one-liners.
- Capitalization and Punctuation: Start with a capital letter and end with a period.
Following these basic rules increases the readability of the documentation. More importantly, it allows for consistent formatting across projects, making it easy for developers to jump from one codebase to another without missing a beat.
Google Style Documentation
The Google style guide offers a different angle when it comes to formatting docstrings. Being slightly more elaborate, this style focuses on structuring information clearly. Here are its main features:
- Summary Line: A concise one-line summary followed by a blank line.
- Parameters Section: Clearly indicates function parameters, including their type and purpose.
- Return Type: Specifies what the function returns, which can be particularly useful for understanding its behavior at a glance.
This structure makes it easier for readers to digest the purpose and behavior of your functions quickly.
NumPy/SciPy Documentation Style
NumPy and SciPy have established their own conventions, primarily aimed at scientific computing. Known for being meticulous about detail, this style emphasizes comprehensive documentation. Some defining aspects include:
- Extended Description: Following the summary, allow room for more in-depth explanations. This can hold insightful details regarding the function's functionality or any complex algorithms used.
- Parameters and Returns: Categorically list parameters with types and descriptions, followed by return values similarly listed.
- Examples: Often, incorporating examples shows practical uses of the function, which can significantly boost comprehension.
By leveraging the NumPy/SciPy style, you can create documentation that is not merely functional but also educational.
In summary: Choosing the right docstring convention can significantly influence the clarity and usability of your documentation. Each style comes with its own sets of strengths, thereâs no one-size-fits-all; however, understanding these conventions will allow for more efficient communication in the coding world.
Elements of Effective Function Documentation
Properly documenting Python functions is not simply a matter of habit; it is a vital practice that incurs long-term benefits for developers. Not only does effective documentation serve as a guide for current users, but it also lays a solid foundation for future developers or collaborators who may work with the code later. Clear documentation can help to expedite the onboarding process for new team members, making it easier for them to understand the purpose of different functions and how they interact within the larger program. Moreover, high-quality documentation often translates into fewer misunderstandings and errors when integrating multiple components of a codebase.
Function Purpose and Description
At the heart of any function is its purpose. Clearly defining what a function does is pivotal, and it should be articulated in simple yet precise language. When addressing the function's purpose, itâs effective to provide context on why the function exists and what problem it solves. For instance, a function meant to calculate the factorial of a number should explain its mathematical relevance. A well-crafted description efficiently communicates not only the function's outcome but also any assumptions it makes.
For example:
Tip: Avoid jargon and unnecessary complexity. Aim to make your document accessible to developers at various skill levels.
Parameters and Arguments
When it comes to parameters and arguments, clarity is once again key. Each parameter should be described clearly with its expected type and purpose. Including relevant details regarding default values or valid ranges can prevent misusage. Take this example:
Itâs crucial to specify which parameters are mandatory, and it can be helpful to demonstrate examples of valid input where applicable.
Return Values
It is equally important to specify the data type of the function's return value. This information helps users anticipate the outcome of calling the function. For instance, if a function returns a list, the user should know this in advance to enable effective manipulation. Additionally, one can also mention possible return scenarios or exceptions pertaining to specific inputs. Hereâs a compelling way to document a return value:
Exceptions and Errors
Handling exceptions efficiently is vital for user experience, and documenting such behaviors is equally essential. Users should be made aware of potential errors they may encounter when executing a function. Specifying which exceptions might be raised and under what circumstances allows users to write better error-handling code. For example:
In this case, the user gets a clear understanding of what to expect, and as a result, can write their code accordingly.
In summation, effective function documentation encompasses a clear delineation of a function's purpose, precise parameter descriptions, comprehensive return values, and thorough exception management. These elements not only enhance readability for current users, but they also establish a solid framework for future contributors to the project.
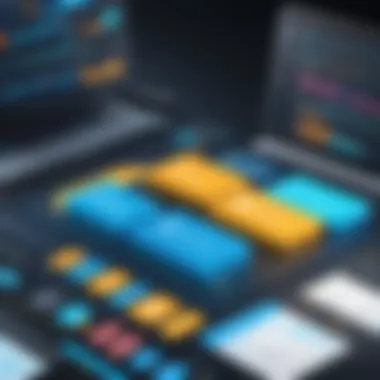
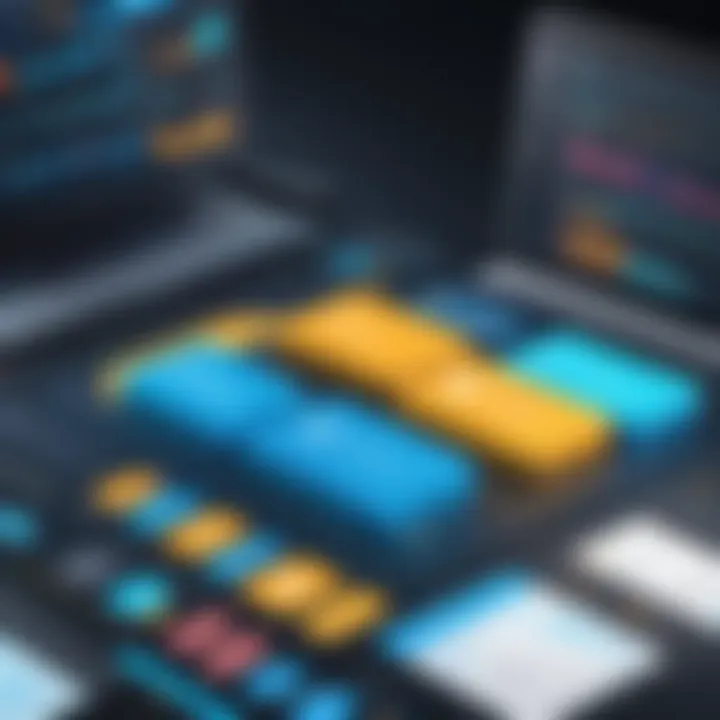
Tools for Generating Documentation
Documentation tools play a pivotal role in enhancing Python function documentation. They automate the process of creating comprehensive, organized, and easily navigable documentation, which can be a game-changer for both individual developers and teams. Leveraging such tools streamlines the documentation process and ensures that the documentation remains up-to-date with the codebase. This not only saves time but also promotes consistency and clarity in conveying functionality. Moreover, utilizing documentation tools fosters better collaboration among team members, as well as providing an accessible resource for users of the code.
Sphinx Documentation Generator
Sphinx is like the Swiss Army knife for documentation in the Python world. Built originally for the Python documentation, it has garnered popularity for its versatility and extensive features. One of the standout characteristics of Sphinx is its ability to output documentation in various formats, including HTML, PDF, and ePub. This adaptability allows developers to cater to a range of audiences, whether they prefer reading in a browser or in a book format.
Sphinx relies heavily on reStructuredText as its markup language, which may seem daunting at first but pays off with powerful capabilities. It supports a seamless integration with popular Python libraries and has extensions that can enhance functionalities, such as enabling automatic API documentation generation. For example, you can document your function directly in the code, and Sphinx will extract that automatically when generating documentation. This two-step process ensures that documentation is both accurate and current.
Another appealing aspect of Sphinx is its theming capabilities. Developers can customize the look and feel of their documentation, giving it a polished appearance that stands out.
pydoc Tool
On the simpler side of things, we have pydoc. This built-in tool comes ready to deploy with Python installations and is designed to generate documentation quickly and efficiently. Itâs like having a lightweight manual at your command, complete with help on modules, functions, and classes.
A defining feature of pydoc is its command-line interface, allowing for easy usage without any extra setup. Simply run in your terminal, and voilĂ ! You get an instant text version of the documentation. If you prefer something more visual, pydoc can also produce HTML outputs, which can be served on a local web server. This quick access to documentation can be invaluable for both debugging and education purposes.
While pydoc is not as feature-rich as Sphinx, it serves as an excellent tool for quick reference, particularly during the early stages of development or for smaller projects. It emphasizes efficiency and is reliable for straightforward documentation needs without the overhead.
PyDocGen Framework
PyDocGen is worth mentioning as it embodies the paradigm of generating documentation straight from Python files. It can be particularly handy for larger codebases with multiple developers. With PyDocGen, you can enjoy not just function documentation, but also detailed project-level documentation.
Using a configuration file, you can specify parameters such as output directory, file formats, and more, customizing your documentation generation process to fit your projectâs needs. PyDocGen releases you from the tedium of writing repetitive documentation; instead, it adopts a systematic approach for parsing your code and presenting it in a clear and organized manner. Another strength of PyDocGen is its support for templates, which can assist in maintaining a cohesive look for documentation across different projects.
Moreover, unlike other tools, PyDocGen can pull docstring information along with additional annotations directly. This feature offers a depth of information that's not only useful for developers but also for stakeholders who may not be programmers but still need to understand project capabilities.
Using the right tools can elevate your documentation process, ensuring that it is efficient, effective, and user-friendly.
When choosing among documentation tools, consider your projectâs scale, your team's familiarity with the tools, and the audience that will consume the documentation. Taking the time to set up proper documentation tools ultimately leads to cleaner code and an easier understanding of your functions.
Integrating Documentation with Code
Integrating documentation with your code serves as the backbone of any meaningful programming project. It ensures that your intent is transparent, both for yourself and future collaborators. When you make it a habit to keep documentation alongside your code, youâre not just throwing words at a wall; you're crafting a narrative that explains the hows and whys behind your programming decisions. The clarity achieved through well-structured documentation can make a world of difference in terms of collaboration and maintenance.
The benefits of embedding documentation into your codebase are manifold. For one, it assists in the onboarding process for new team members or even for the original author revisiting the code after some time. A seamless connection between code and documentation can help circumvent misunderstandings that might arise from ambiguity in your code's functionality.
Documentation Strings in IDEs
Using documentation strings within integrated development environments (IDEs) is where the magic often begins. Most IDEs today can recognize docstrings and will provide hints, tooltips, or even auto-completions based on those annotations. This ease of access enhances productivity by reducing the time spent searching through files to recall what a function does.
- Visibility: Having docstrings readily available allows developers to see the purpose of functions without needing to dive into the implementation details.
- Error Prevention: Developers are less likely to misuse functions they're not familiar with, as they can quickly reference what each function requires and what it returns.
- Code Review: During code peer review sessions, clear documentation helps reviewers understand your logic better and provide more meaningful feedback.
It's important to remember that docstrings in IDEs are not just formalities; they should be treated like an essential part of your coding habits. You want usersâwhether itâs you in a few months or someone in a completely different timezoneâto pick up your code and hit the ground running.
Best Practices for Inline Documentation
Inline documentation offers a unique twist on how we think about coding practices. Itâs the friendly face of your code, explaining complex logic right where it occurs. Here are some best practices to consider for writing effective inline documentation:
- Clear and Concise: Your comments should be easy to digest. Avoid using jargon unless absolutely necessary.
- Descriptive Naming: Sometimes, a function name can say a lot. Pair names that convey intent with comments that clarify any intricate logic.
- Consistent Style: Stick to a style guide for inline documentation to minimize confusion. Whether you follow PEP 8 or adopt another style guide, consistency is key.
- Keep it Relevant: If you add a comment, it should directly relate to the line or block of code. Avoid commenting on something thatâs self-explanatory.
"Code is like humor. When you have to explain it, itâs bad." - Cory House
In summary, successfully integrating documentation with code enriches both the development experience and the end product. Clear documentation, both within IDEs and inline, fosters a collaborative environment that bolsters productivity and comprehension.
Tutorial: Writing Your First Function Documentation
Writing effective function documentation is critical for any programmer looking to improve their code's usability and maintainability. This tutorial focuses on three essential aspects: identifying the function's purpose, structuring the docstring, and testing documentation. It serves as a practical guide to help you develop the skills needed for creating clear and informative documentation that benefits both you and others who may use your code.
Identifying the Function's Purpose
Understanding what a function does is foundational in crafting its documentation. Before you even begin to write, take a step back and ask yourself: What problem does this function solve? Knowing the primary purpose will guide your writing process and ensure that readers grasp the function's use at first glance.
When pondering the functionâs purpose, consider these questions:
- What inputs does the function require?
- What outputs will it provide?
- Are there any side effectsâdoes it modify global state or alter data passed in?
By laying out these elements, you can more effectively convey the importance of the function within the greater scope of your program. Itâs much like highlighting the main attractions when giving a tour; if you donât set the stage, people might miss the best parts altogether.
Structuring the Docstring
Once you're clear on the function's purpose, the next step is structuring your docstring effectively. A well-organized docstring allows users to quickly find the information they need. It's customary to include several key components:
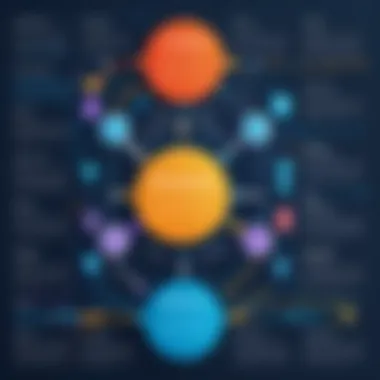
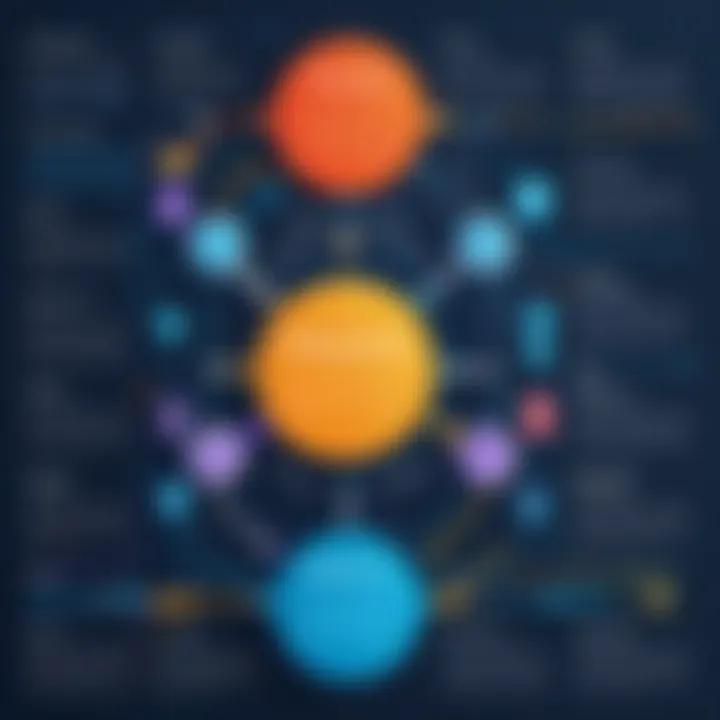
- Brief Description: Start with a concise summary that states what the function does. This is often just a sentence or two.
- Parameters: List each parameter, its expected type, and a short description of what it represents.
- Returns: Specify the return type and what the return value signifies.
- Exceptions: If applicable, mention any exceptions that might be raised during the function's execution.
Hereâs a simple example:
A well-structured docstring not only helps other programmers but also enables you to revisit your code later and comprehend it quickly. Itâs always easier to follow a clear roadmap when youâre trying to navigate; the same goes for code.
Testing Your Documentation
It's one thing to write documentation, but ensuring it works as intended is another ball game. Testing your documentation means not just checking for typos but verifying that it accurately reflects the function's behavior.
Here are some tips to effectively test it:
- Run Automated Tests: Implement unit tests that not only test the function but also use the documentation as a reference while asserting expected outcomes.
- Seek Peer Feedback: A fresh pair of eyes can spot weaknesses in your documentation that you might overlook.
- Use Documentation Generators: Running a tool like Sphinx can help identify issues in your documentation by providing a structured output.
Don't just take my word for it; testing documentation is as crucial as writing code! If your documentation is incorrect, it can lead to misunderstanding and frustration, which is a road sought to be avoided in the coding world.
To summarize, effective documentation paves the way for better code comprehension and collaboration. By focusing on the function's purpose, structuring your docstring properly, and rigorously testing what you've written, you set the stage for a coding experience that fosters clarity and cooperation.
Challenges in Function Documentation
Documenting Python functions is not merely a box-checking exercise; it plays a pivotal role in the overall development process. Yet, even seasoned developers encounter thorny issues when it comes to creating and maintaining effective documentation. Acknowledging these challenges is essential for anyone aspiring to enhance their Python function documentation skills. From consistency issues to the repercussions of code alterations, let's explore these factors in detail.
Maintaining Consistency
When it comes to documentation, consistency is king. Inconsistent documentation can confuse users and lead to misunderstandings about how functions should be used. Consider a scenario where a function's purpose is documented in one style in one module and another style in a different module. This inconsistency can create a muddled learning experience for users.
To tackle this challenge:
- Adopt a standard format: Whether it's adhering to PEP 257 or using Google or NumPy style guidelines, having a consistent format across all functions makes a world of difference.
- Use templates: Predefined templates for documentation can guide developers in providing uniform information, making the task less cumbersome.
Maintaining consistency in function documentation fosters trust and credibility, ensuring that other programmers can rely on the documentation for accurate information about how to call and utilize the functions effectively.
Updating Documentation with Code Changes
One of the most frequent headaches in software development is synchronizing code with documentation. Developers often make changes to the functionality of a function without updating its corresponding docstring. This mismatch can lead to a predicament where users are left puzzled, trying to interpret a function based on outdated information. This can be critical, especially in environments where code is frequently updated or iterated upon.
To alleviate this issue:
- Implement a review process: Having a checklist during code reviews, ensuring that documentation gets updated along with the code, can make transitioning smoother.
- Utilize automated tools: Tools like Sphinx can help identify discrepancies between your code and the documentation, alerting you when updated information is required.
By prioritizing the updating of documentation alongside code changes, developers can create a seamless experience for those utilizing their functions.
Ensuring Clarity for Users
Clarity is the bedrock of effective documentation. If the information isn't easily digestible, users may struggle when attempting to integrate functions into their applications. It's crucial that function documentation is not only comprehensive but also clear and to the point. A complex explanation may require users to decipher the text rather than quickly grasp the function's purpose and usage.
To ensure clarity:
- Use straightforward language: Avoid jargon or overly technical terms unless absolutely necessary. This makes the documentation accessible to a broader audience, including beginners.
- Provide examples: Examples are worth their weight in gold. They can demonstrate practical uses of a function, helping users see not just how to use it, but why they might want to.
Clarity elevates the user experience, leading to fewer code errors and more productive programmers.
"Effective documentation is an investment in future productivity."
By recognizing these challenges and tackling them head-on, programmers can significantly enhance the quality of their function documentation, paving the way for improved code usability and team collaboration.
Finale
In this closing segment, itâs crucial to reiterate the value of function documentation in Python programming. Not only does adequate documentation facilitate understanding for both the documentor and the user, but it also elevates the overall quality of the code and promotes best practices within development teams. By taking the time to meticulously document functions, programmers ensure that their code is not just written, but communicated.
Recap of Key Points
To summarize the critical elements discussed earlier:
- Significance of Documentation: It is the glue that binds your code to its users, enhancing usability and maintainability.
- Types of Docstrings: Recognizing the difference between single-line and multi-line docstrings helps cater to various coding scenarios.
- Adhering to Conventions: PEP 257, Google Style, and NumPy conventions each provide robust guidelines for structuring documentation effectively.
- Key Elements: Effective documentation encompasses the function's purpose, parameters, return values, and exception handling.
- Challenges: Maintaining consistency and clarity can be a real struggle, but overcoming these hurdles is necessary for productive codebases.
With this framework in mind, the importance of clarity in documentation becomes even clearer:
"Good documentation is like a map: it guides you through the terrain of code, helping prevent wrong turns and dead ends."
Encouragement for Continued Learning
In the ever-evolving landscape of programming, continual learning is essential. For those engaged with Python or any other language, familiarity with the documentation process is not just beneficial, it's a necessity. As you write and refine your own documentation, seek resources that delve deeper into stylistic nuances and emerging practices in the field.
Engaging with community platforms like Reddit or browsing academic repositories on Wikipedia) can provide fresh insights and real-world applications.
Remember, honing your documentation skills will not only make your code more approachable but also level up your understanding and mastery of Python as a whole. Stay curious, keep experimenting, and continue to document diligently.