Mastering JavaScript with W3Schools: A Comprehensive Guide
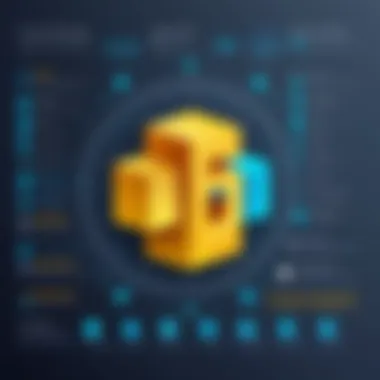
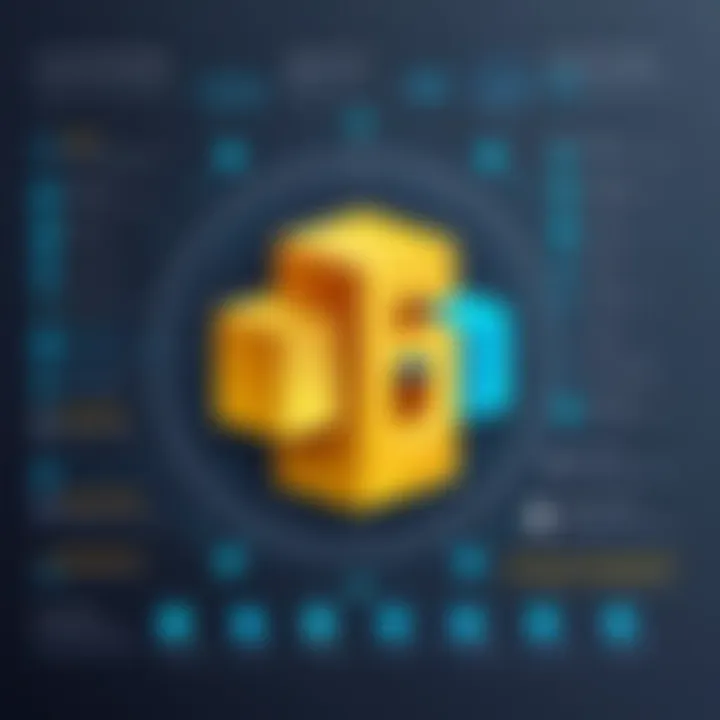
Prelude to Programming Language
Understanding JavaScript is akin to mastering a craft. The language, born in the mid-1990s, was originally designed to enable simple interactive elements on websites. Over the years, it has evolved into a powerhouse for web development, intertwining itself with nearly every aspect of modern web applications.
History and Background
JavaScript was created by Brendan Eich while working at Netscape. It's interesting to note that the language was developed in just ten days, a feat that seems almost unbelievable given its complexity today. Initially named Mocha, it quickly transformed into LiveScript before finally landing on JavaScript, capitalizing on the popularity of Java at the time despite their fundamental differences.
Features and Uses
JavaScriptâs versatility is one of its most appealing features. It
- Enables dynamic content manipulation
- Supports event handling for user interactions
- Allows asynchronous programming through callbacks and promises
- Integrates seamlessly with HTML and CSS
These features make it a favored choice for developers working on interactive web applications, single-page applications, and even server-side programming with Node.js.
Popularity and Scope
In the realm of programming languages, JavaScript stands tall. According to numerous surveys, it has consistently ranked as one of the most used programming languages. The widespread adoption of frameworks like React, Angular, and Vue.js only amplifies this. Learning JavaScript not only opens a door to web development but also offers opportunities in mobile app development and game creation.
"Mastering JavaScript is no longer just nice to have. It's a necessity for anyone aiming to excel in tech today."
Basic Syntax and Concepts
To start your journey in JavaScript, having a solid grasp of its basic syntax and core concepts is essential.
Variables and Data Types
Variables in JavaScript can be declared using , , or . Each serves a distinct purpose. For instance, allows you to declare variables with block scope. Common data types include:
- String: Used for text (e.g., )
- Number: Represents numerical values (both integers and floats)
- Boolean: Either or
- Object: A collection of related data and functions
Operators and Expressions
Operators help manipulate data values. For example:
- Arithmetic Operators: , , ,
- Comparison Operators: , , , ``
- Logical Operators: , ,
Control Structures
Control structures guide the flow of the program. The statement is used for decision-making, while loops (, ) help repeat blocks of code based on conditions. Understanding how these structures work together lays the groundwork for more advanced programming.
Advanced Topics
Once you've mastered the basics, you can dive into more intricate areas of JavaScript.
Functions and Methods
Functions are blocks of reusable code designed to perform a specific task, which help in minimizing repetitive code. Here's a quick example:
Object-Oriented Programming
JavaScript supports object-oriented programming paradigms, which allow developers to structure their code in a more understandable way. Objects can hold both data and functions, encapsulating behavior and state together.
Exception Handling
Error handling is crucial for robust applications. JavaScript provides syntax to gracefully handle exceptions, ensuring that your program can continue executing despite encountering an error.
Hands-On Examples
Practical application cements knowledge. Consider these examples:
Simple Programs
A quick JavaScript snippet that calculates the area of a rectangle might look like this:
Intermediate Projects
Building a simple to-do list application can enhance your understanding of how JavaScript interacts with the Document Object Model (DOM). This project encourages working with inputs, lists, and event listeners.
Code Snippets
Hereâs a simple code snippet for fetching data from an API:
Resources and Further Learning
To further hone your JavaScript skills, consider these resources:
Recommended Books and Tutorials
- Eloquent JavaScript by Marijn Haverbeke
- You Donât Know JS by Kyle Simpson
Online Courses and Platforms
Community Forums and Groups
Joining forums such as Reddit or Facebook groups dedicated to JavaScript can provide valuable community support and insights.
Mastering JavaScript is a journey that combines theory with practical implementation. As you navigate the resources available, from W3Schools to hands-on projects, remember that each line of code you write contributes to your proficiency in this powerful language.
Prelude to JavaScript
JavaScript stands as a significant pillar in the world of web development, granting life and interactive features to otherwise static websites. In this article, we will navigate the intricate landscape of JavaScript, starting from its foundational principles and extending to its advanced functionalities. This guide is tailored to help individuals grasp the essentials and complexities of JavaScript using the comprehensive resources provided by W3Schools.
Understanding the Role of JavaScript in Web Development
JavaScript functions as the primary scripting language for web development. Unlike HTML and CSS, which manage the structure and style of web pages, JavaScript injects interactivity and enables dynamic content manipulation. For instance, think about the last time you filled out a form on a website. The smooth transitions, real-time validation of your input, and the auto-saving feature? All powered by JavaScript.
Here, it is essential to recognize that JavaScript is often combined with various libraries such as jQuery and front-end frameworks like React and Angular. This integration enhances its capabilities further, allowing developers to create rich user experiences. Developers gain substantial benefits from an understanding of JavaScript, as it opens doors to numerous opportunitiesâbe it crafting engaging user interfaces or developing complex web applications.
A Brief History of JavaScript
JavaScriptâs inception traces back to 1995, birthed by Brendan Eich while he was at Netscape. It was originally designed to make web pages more interactive. The language, initially branded as Mocha, later became known as LiveScript before finally settling with the name JavaScript. This peculiar naming stems from a marketing strategy to leverage the growing popularity of Sun Microsystems' Java programming language.
The release of JavaScript was a seminal moment for web developers. It rapidly adhered to the standards supported by the World Wide Web Consortium (W3C) and ECMA International. The evolution of JavaScript has been phenomenal, leading to various iterations and updates, such as the significant ECMAScript 6 (ES6) release in 2015 which introduced important features like classes, modules, and arrow functions.
As we delve deeper into JavaScript through resources from W3Schools, it becomes clear that understanding both its history and its present functionality is crucial for mastering web development. This knowledge paves the way for approaching modern frameworks and libraries, as the foundational principles strongly influence their design.
Getting Started with W3Schools JavaScript Tutorial
Beginning your journey into JavaScript is a pivotal moment, and the W3Schools platform offers a solid foundation for learning this essential web technology. JavaScript is not just an add-on; itâs the beating heart of interactive web pages, allowing developers to create dynamic experiences that engage users. By diving into W3Schools, learners have the opportunity to grasp both the fundamental and advanced concepts of JavaScript at their own pace.
Navigating the W3Schools Platform
W3Schools serves as a comprehensive resource for budding programmers. Initially, it may appear as just another website, but itâs equipped with useful features catering specifically to learners who are new to web development. The interface is friendly and straightforward. Here, you can easily find various tutorials, references, and examples that make learning as smooth as butter.
Importantly, the layout consists of well-structured content, allowing users to navigate through topics seamlessly. Users can quickly jump between basic tutorials and advanced sections without losing their place. Here's what you can expect:
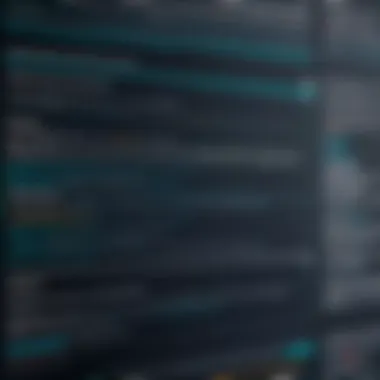
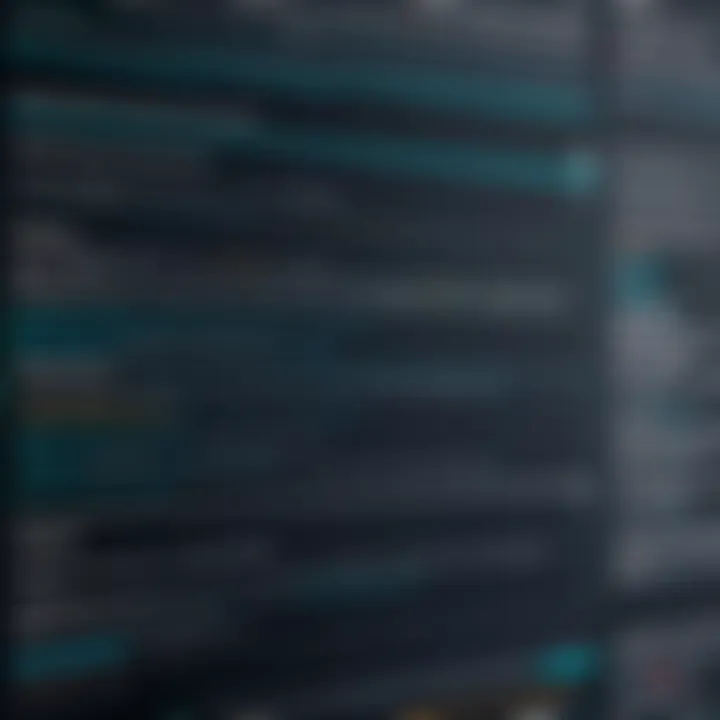
- Interactive Examples: One of the standout features of W3Schools is the ability to play with code snippets directly within the browser. This hands-on approach reinforces learning by allowing immediate application of concepts.
- Comprehensive References: The reference materials cover a broad range of JavaScript functions and methods, making it easy for users to find the specifics they need while coding.
- Quizzes and Exercises: Regular quizzes test your understanding and ensure you're keeping up with the material. This adds an engaging aspect to learning, much like how a sport requires practice.
In short, whether youâre a novice or someone looking to brush up on skills, the W3Schools platform is your go-to guide through the often overwhelming landscape of JavaScript.
Setting Up Your Development Environment
Setting up your development environment is akin to laying down the tracks before a train rolls out. Itâs all about the foundation. Luckily, accomplishing this within W3Schools is straightforward.
- Choosing a Code Editor: First, select a suitable code editor. Popular options include Visual Studio Code, Sublime Text, or even the basic Notepad. Each has its own set of features; Visual Studio Code, for example, offers extensions that make working with JavaScript a breeze.
- Installing Node.js (optional): For those interested in server-side JavaScript, installing Node.js is essential. It allows you to run JavaScript code outside a browser, giving you a taste of backend development. Installing it is a cinch, just download it from the Node.js website and run the installer.
- Using Browser Developer Tools: Modern browsers come with built-in developer tools that enable you to inspect, debug, and experiment with your JavaScript code live. By right-clicking on the page and selecting âInspect,â you can access a treasure trove of functionality.
- Organizing Your Projects: Keep your files organized by creating separate folders for each project. It helps you maintain clarity as your coding endeavors grow.
By correctly setting up your environment, you position yourself for efficient learning and growth as a JavaScript developer. Such preparations ensure that when you encounter the complexities of programming, your tools are equipped to support your learning journey.
Remember, the way you set up your environment often influences your coding experience. A well-structured setup fosters productivity.
With these fundamentals in place, the journey through JavaScript will become increasingly manageable and enjoyable, serving as a strong base for mastering the language.
Core JavaScript Concepts
Understanding core JavaScript concepts is vital for anyone looking to navigate the landscape of web development. These fundamental building blocks shape how we interact with the JavaScript language and ultimately influence our coding practices. By mastering these elements, learners can develop a robust foundation that allows them to tackle both simple scripts and complex applications with confidence.
Among the essential elements are variables, data types, operators, expressions, and control structures. Each of these plays a crucial role, often interlinked, forming the crux of logic and functionality in JavaScript programming. While some may view these as mere technicalities, they harbor significant benefits. For example, having a good grasp of data types enhances efficiency and minimizes potential bugs in the code. Each concept serves as a critical puzzle piece in building dynamic web applications.
Variables and Data Types
A variable in JavaScript is like a container that holds data values. Understanding how to declare variables is the first step in programming. Itâs crucial to familiarize oneself with three types of variable declarations: , , and . While is function-scoped and has a hoisting behavior, and are block-scoped, which now leads to better management of variable scope.
Moreover, JavaScript has several data typesâString, Number, Boolean, Object, and Array, to name a few. This variety permits developers to utilize data in multiple forms, which is essential for building interactive applications. Hereâs a quick comparison:
- String: Used for text. E.g.,
- Number: For numerical values. E.g.,
- Boolean: Represents or values. E.g.,
- Object: A collection of key-value pairs. E.g.,
- Array: A special type of object used for storing sequences of data. E.g.,
Proper use of these variable types impacts memory efficiency and code clarity, allowing for easier debugging and development.
Operators and Expressions
Next, we delve into operators and expressionsâthe tools that allow us to manipulate variables and data. Operators perform operations on variables and values. They can be divided into several categories:
- Arithmetic Operators: For mathematical calculations, such as addition (), subtraction (), multiplication (), and division ().
- Assignment Operators: Used to assign values, e.g., , , .
- Comparison Operators: To compare values, including , , , , , ``, and their corresponding compound versions.
Control Structures
Control structures are the backbone of decision-making in JavaScript code. They determine the flow of execution, allowing the program to take different paths based on conditions. Examples include:
- If-Else Statements: To execute code based on specific conditions.
- Switch Statements: A cleaner alternative to multiple if-else statements when dealing with various conditions.
- Loops: Such as , , and , which facilitate repetitive tasks without the need for redundant code.
Learning how to leverage control structures effectively can drastically reduce complexity, providing clarity and enhancing both maintenance and extension of the code in the long run.
Functions in JavaScript
Functions form the backbone of JavaScript programming. They are essential for every kind of operation in your code. When you break down complex tasks into smaller, manageable pieces, functions come into play. This allows for a clearer structure, enabling you to write cleaner, more efficient code. Understanding functions not only makes you a better programmer but also makes your code reusable and easy to maintain.
Defining Functions
Defining functions in JavaScript is straightforward but holds significant importance. Functions can be declared in various ways: function declarations, function expressions, and arrow functions. Each type has its own characteristics, benefits, and constraints.
A basic function declaration looks like this:
In this example, the function takes a parameter and returns a greeting string. The ability to pass parameters makes functions flexible. You can supply different arguments to achieve different outcomes. Moreover, functions can return values, so you can perform tasks and get results that can be used later in your code.
Another way to define functions in JavaScript is through expressions, which come in two flavors: named and anonymous.
Named Function Expression
Anonymous Function Expression
Arrow functions were introduced in ES6 and provide a more concise syntax. Hereâs an example:
Using arrow functions can vastly simplify your code, but keep in mind they do not have their own context, which might change how you handle object methods.
Function Scope and Closures
Understanding function scope and closures is critical for mastering JavaScript. Scope determines where your variables are accessible in your code. In simple terms, if you declare a variable inside a function, it won't be available outside of it. There are two primary types of scope: global and local (function scope).
Global variables can be accessed from anywhere in your code, while local variables are accessible only within the function they are defined in. This encapsulation is crucial, as it helps prevent variables from interfering with one another and keeps your code running smoothly.
Closures are another fascinating aspect of functions. They allow a function to have access to its outer scope, even when the function is executed outside that scope. Hereâs how it looks in practice:
In this example, retains access to even after has completed execution. This behavior is powerful and allows for more dynamic coding techniques, such as creating private variables or functions.
"Mastering functions and their intricacies is like learning to play a musical instrument. The more time you invest in understanding it, the better you become at creating beautiful melodies in your code."
As you progress in your JavaScript journey, grasping the nuances of functions, their scope, and closures will set you apart as a proficient developer.
Working with Objects
Objects are at the heart of JavaScript, shaping how developers manage data and organize code. Understanding the intricacies of objects is crucial for anyone aspiring to master JavaScript. In essence, objects serve as containers that hold related data and functionality. They add structure to code and enable the creation of complex data models in a clear way.
When working with objects, one quickly realizes the benefits they offer:
- Encapsulation: Objects bundle together related data and functions. This means that data can be easily managed without exposing it to the whole program.
- Inheritance: JavaScript uses prototypes, allowing one object to inherit properties and methods from another. This promotes code reuse and adheres to the DRY (Don't Repeat Yourself) principle, which is a core concept in programming.
- Dynamic: Unlike static types in some other languages, JavaScript objects can be modified at runtime, offering flexibility for developers to change properties on the fly. This makes them particularly useful in a responsive web environment.
While the advantages are manifold, a good grasp of objects also means being aware of specific considerations:
- Ensuring proper use of prototypes is key to avoiding common pitfalls that can lead to unexpected behaviors.
- Understanding how context works with keyword is paramount when invoking methods on objects.
This section focuses on two essential subtopics: Understanding Objects and Prototypes, and Object-Oriented Programming Principles.
Understanding Objects and Prototypes
In JavaScript, objects are an amalgamation of key-value pairs. Each key is a string, and the corresponding value can be of any type. This flexibility allows for the creation of more complex structures like arrays or even other objects. For instance, consider the following code snippet:
Here, is an object containing properties like , , and , along with a method that uses the keyword to reference the object itself.
Prototypes create a chain of objects and help in achieving inheritance. If a property or method is not found in the object itself, JavaScript looks for it in the prototype, allowing objects to share methods and properties, thus, reducing memory usage. The following example extends our object:
Here, inherits from , showcasing how prototypes enable code reuse. However, one needs to wield this power carefully to avoid bugs that come from unintended prototype pollution.
Object-Oriented Programming Principles
With JavaScript's capabilities surrounding objects, it's essential to understand Object-Oriented Programming (OOP) principles. These principles organize code into cohesive modules that capture real-world concepts, facilitating better understanding and maintenance. Here are the key OOP principles relevant to JavaScript:
- Encapsulation: As mentioned earlier, encapsulation combines data and methods into a single unit or class, shielding direct access to some components.
- Abstraction: This principle is about hiding complex reality while exposing only the necessary parts. JavaScript allows creating objects that can simplify interactions with complex systems.
- Inheritance: This has already been discussed with prototypes. It enables new objects to adopt properties and behaviors of existing objects.
- Polymorphism: Objects can be treated as instances of their parent class, even if they are instances of a derived class. This allows for designs that can interact with various objects interchangeably.
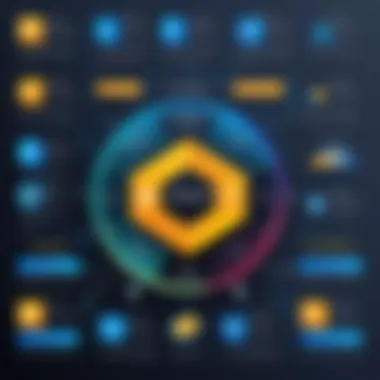
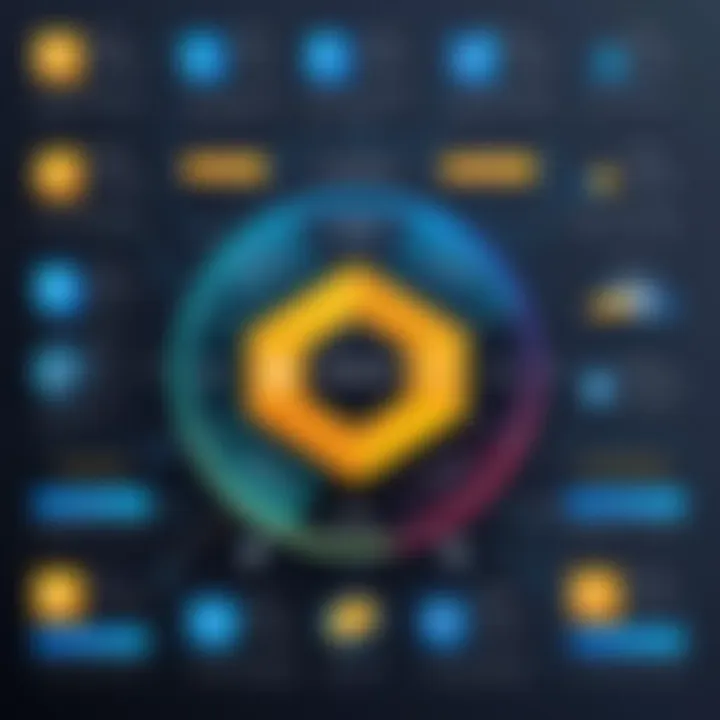
When diving into object-oriented concepts, a solid understanding of how objects and classes work in JavaScript sets the stage for mastering this paradigm. Ideally, grasping these OOP principles not only enhances coding efficiency but also amplifies one's capabilities in developing robust applications.
In summary, working with objects is not just a task but a craft that every aspiring JavaScript developer should hone. Whether it's understanding the core properties of objects or leveraging prototypes for inheritance, these concepts serve as stepping stones toward more advanced programming techniques, paving the way for creating maintainable and scalable JavaScript applications.
"To progress in JavaScript programming, learning about objects and the principles of OOP is essential; they form the foundation of complex applications."
In the next section, we will explore the nuanced aspects of manipulating these objects and how they integrate within the vast landscape of web development.
DOM Manipulation and Events
When it comes to web development, understanding the relationship between JavaScript and the Document Object Model (DOM) is key. The DOM essentially serves as a bridge between the web page you're designing and the programming language that manipulates it. This dynamic interaction is crucial because it breathes life into static HTML, making your website engaging and interactive.
Prolusion to the Document Object Model
The Document Object Model is a representation of a web page that browser can read and manipulate. Think of it as a tree structure, with nodes that represent HTML and its attributes. Each element on the pageâbe it a heading, a paragraph, or a buttonâhas its own node.
To understand it better, let's consider a simple example:
- HTML StructureIn this example, the and . When you work with JavaScript, you can access these nodes using methods like or . Understanding this structure is not just academic. It empowers you to update content, styles, and even apply effects on-the-fly.
Interacting with HTML Elements
Once youâve grasped the DOMâs structure, itâs time to delve into how JavaScript can interact with these elements. Here are critical aspects to consider:
- Selecting Elements: Using various methods such as or , you can retrieve elements you want to manipulate. These functions help pinpoint the specific parts of the DOM you want to change.
- Manipulating Attributes: Each HTML element may have attributes that can be changed. For instance, you can modify the attribute of an image or the of a link dynamically with JavaScript. This flexibility is a huge advantage when developing responsive web pages.
- Adding and Removing Elements: Need to add a new list item or an image? JavaScript lets you create new elements using and then append them to existing nodes. You can also remove elements with .
By harnessing these capabilities, you can truly transform the user experience on your website.
"The true essence of a website lies in its interactivity. JavaScript and the DOM make this possible.â
In summary, DOM manipulation is an indispensable skill for any aspiring web developer. It allows manipulation of HTML elements dynamically, responding to user actions and making the website feel alive. The interplay between the DOM and JavaScript sets the stage for modern web applications, offering vast potential for creativity and user engagement.
Asynchronous JavaScript
Asynchronous JavaScript is one of those concepts that, once grasped, opens up a world of possibilities for web developers. Imagine you're at a cafe, sipping your favorite latte, while also checking your emails. Youâre not tied down, waiting for Gmail to load; instead, you can multitask. This is the essence of asynchronous programming. In the realm of coding, it allows functions to run in the background, freeing up the main thread and enabling a more fluid and responsive user experience.
In web development, achieving optimal performance often hinges on the ability to handle tasks that might take timeâlike fetching data from an API without stalling the complete functionality of your application. Asynchronous JavaScript is the answer to this challenge. It helps keep your web applications snappy, ensuring that users do not face any disruptive wait times.
Understanding Callbacks and Promises
Callbacks were once the go-to solution for handling asynchronous tasks in JavaScript. A callback is a function passed as an argument to another function and is executed after the first function has completed its operation. Itâs a straightforward approach, but it can lead to something known as "callback hell"âwhere multiple nested callbacks create a convoluted structure that is hard to manage and read. Here is a simple explanation:
This example showcases how a function can be executed after data is fetched. However, chaining multiple calls can lead to messy code. Enter Promisesâa more elegant solution.
Promises allow you to write cleaner and more manageable asynchronous code. They represent a value that might be available now, or in the future, or never at all. You can attach handlers with and methods to indicate what to do once a Promise is settled (either fulfilled or rejected).
Promises make it easier to avoid callback hell, as the code becomes more linear and simpler to read. One of the major advantages of Promises is their ability to handle errors more gracefully.
Working with Async/Await
Async/Await is built on top of Promises, further simplifying how you can handle asynchronous code. It brings a synchronous look to asynchronous code, which can make it much easier to reason about. This feature allows a function to pause execution until a Promise is resolved, without stopping the entire program.
By marking a function as , you allow the use of the keyword within it. This keyword tells JavaScript to wait for the specified Promise to resolve or reject before continuing.
Here is a clearer example:
Notice how the code now reads almost like a serial process, aiding in readability. This is especially beneficial when dealing with multiple asynchronous operations, such as making several API calls in order. For instance:
Utilizing simplifies complex workflows and makes your JavaScript code much easier to manage.
In summary, mastering asynchronous JavaScript is crucial for building efficient web applications that provide a seamless user experience. By understanding callbacks and Promises, as well as utilizing async/await, developers can tackle a wide array of real-world problems without getting bogged down in their code.
The asynchronous paradigm has become a cornerstone of modern web development, and as you delve deeper into JavaScript, grasping these concepts will serve you well. Not only does it enhance performance, but it paves the way for more interactive and engaging user interfaces.
JavaScript Debugging Techniques
Debugging is an essential skill in programming. For JavaScript, it serves not just as a remedy for errors but also as a mechanism to understand and refine code. Proper debugging techniques can illuminate how your JavaScript interacts with HTML and CSS as well as improve the overall efficiency of your projects. This section will delve into common debugging tools and techniques, while also examining effective strategies for testing your code, reinforcing the relevance of debugging in mastering JavaScript.
Common Debugging Tools and Techniques
When you embark on your journey through JavaScript, inevitably, youâll encounter bugsâthose pesky errors that can halt your programming progress. Fortunately, there are several tools that can ease the debugging process. Here are some you might find particularly useful:
- Browser Developer Tools: Most modern browsers come with built-in developer tools. For instance, Google Chrome and Firefox include a JavaScript console, which allows you to execute snippets of code and see real-time errors.
- Console.log(): Itâs a classic method. Adding to your code lets you see variable values and flow of execution. Itâs as straightforward as it gets, yet incredibly effective for immediate feedback.
- Linting Tools: These tools, like ESLint, help catch errors before you even run your code. They analyze code quality and provide stylistic recommendations which can prevent bugs.
- Debuggers: Tools like Visual Studio Code have debugging capabilities that allow you to step through your code, set breakpoints, and inspect variables at runtime. Utilizing a debugger often gives a clearer view of what's happening under the hood.
- Online Debugging Platforms: Websites like JSFiddle and CodePen let you write and test JavaScript directly in your browser. Utilizing these tools can often yield quicker feedback compared to your local setup.
"Debugging is like being the detective in a crime movie where you are also the murderer."
Strategies for Effective Code Testing
While debugging hones in on resolving errors, itâs critical to implement thorough testing strategies. This not only aids in catching bugs but also ensures that the code meets its intended function with reliability. Here are some effective code testing strategies:
- Unit Testing: This strategy involves testing individual components of your code. Frameworks like Jest and Mocha can automate this process, allowing you to ensure that changes in one part of your application don't inadvertently break another.
- Integration Testing: After unit testing, youâll want to ensure that all parts of your application work together as intended. This involves testing interactions between different modules or services within your application.
- End-to-End Testing: This takes it a step further by simulating real user scenarios. Tools like Cypress or Selenium allow for comprehensive testing that reflects actual usage, helping you spot user experience issues early.
- Code Reviews: Sometimes the best debugging strategy is a second pair of eyes. Code reviews encourage collaboration and knowledge sharing. They help in identifying bugs and potential optimizations that you may have overlooked.
- Automated Testing: Implementing continuous integration can greatly aid the testing process. This allows you to run tests automatically when changes are made, catching issues early in development.
With effective debugging and testing techniques, mastering JavaScript becomes a more manageable and insightful endeavor. Each approach contributes not only to solving existing issues but also to a deeper understanding of how to write cleaner, more efficient code. Remember, the road to becoming proficient in JavaScript is not just about writing code but also about knowing how to refine and enhance that code.
Advanced JavaScript Concepts
Advanced JavaScript concepts are crucial for anyone looking to elevate their programming abilities beyond the basics. These concepts embrace more complex and nuanced features of the language, paving the way for efficient coding practices and better structure in projects. Familiarity with these topics can significantly improve the developerâs ability to build scalable applications and to work collaboratively in a team environment.
In this article, we will delve into two key areas; modules and ES6 features, and JavaScript design patterns. Each of these topics contributes to a robust understanding of the language and equips developers with tools to design more efficient, maintainable code.
Modules and ES6 Features
The introduction of ES6, also known as ECMAScript 2015, brought transformative features to JavaScript, among which are modules. Modules allow developers to break their code into separate files, leading to a cleaner structure and improved maintainability. Essentially, they help organize code by encapsulating related functionalities.
Using modules not only enhances readability but also promotes code reusability. You can define a module in one file and import it wherever needed. This way, you avoid duplication and reduce the potential for errors, which is a hallmark of good programming practice. For instance, hereâs a simple example of how you can define and import a module:
This modular approach also streamlines the testing process, as you can test individual modules without having to run the entire application every time. Not only is this beneficial for performance, but it's also a big win for productivity.
Being well-versed in ES6's features like template literals, arrow functions, and destructuring can also streamline the way you write code. For instance, arrow functions offer a more concise syntax, while template literals let you easily create strings with dynamic content.
JavaScript Design Patterns
Design patterns are proven solutions to common programming problems. In the realm of JavaScript, they provide effective approaches to structuring your code, which is essential for both maintainability and scalability.
Some commonly used JavaScript design patterns include the Module Pattern, Factory Pattern, and Singleton Pattern. Each of these patterns serves specific purposes, which can help in organizing your code effectively.
- Module Pattern: This pattern helps manage private and public parts of your code, shielding the details that donât need to be exposed. Itâs particularly useful in cases where you want to create specific functionality without cluttering the global namespace.
- Factory Pattern: It allows you to create objects without having to specify the exact class of the object being created. This can be particularly useful when dealing with numerous similar objects.
- Singleton Pattern: Ensures that a class has only one instance and provides a global point of access to it. This is helpful in scenarios such as managing application states.
"Understanding design patterns is a stepping stone towards becoming a proficient developer who can tackle complex problems with ease and elegance."
By incorporating advanced JavaScript concepts into your programming toolkit, you position yourself to tackle real-world challenges with confidence. As you progress through these topics, youâll find that they not only enhance your coding skills but also improve your problem-solving capabilities.
In essence, these advanced concepts are essential for any developer aiming for mastery in JavaScript, especially when leveraging resources like W3Schools to further enhance your learning journey.
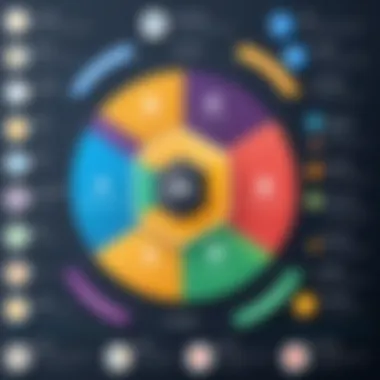
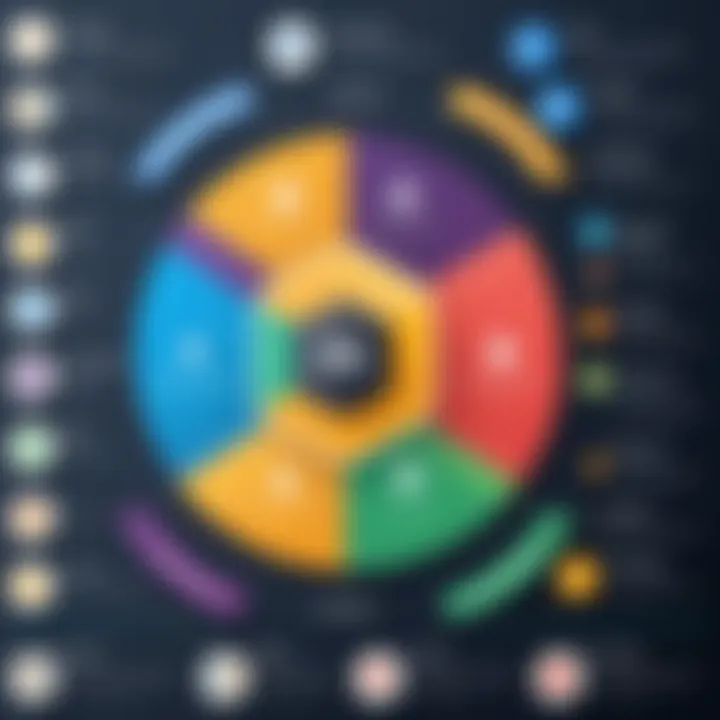
Understanding JavaScript Libraries and Frameworks
JavaScript libraries and frameworks have become indispensable tools in the ecosystem of web development. Understanding their significance is crucial for anyone aiming to master JavaScript. Libraries, like jQuery and Lodash, are designed to simplify tasks, offering pre-written code snippets that developers can utilize to save time and avoid reinventing the wheel. For instance, with a library like jQuery, you can manipulate the DOM and handle events with ease, which can be a real time-saver for both novice and seasoned developers.
Frameworks, on the other hand, offer a structured environment that manages the coding process from start to finish. They tend to enforce certain patterns and practices, which can make your code more maintainable and scalable. Popular frameworks like Angular, React, and Vue.js present a cohesive approach to developing applications, allowing developers to focus on the overall architecture while having the necessary tools at their disposal.
Using libraries and frameworks provides various benefits:
- Speed: They can drastically reduce development time by providing reusable components and functions.
- Community Support: Most libraries and frameworks have large communities. This means a wealth of resources, plugins, and tutorials are available, which can be invaluable for learning and troubleshooting.
- Best Practices: They often embody best practices in their design, promoting clean and efficient code, reducing common pitfalls.
When diving into libraries and frameworks, itâs essential to consider your project's requirements and how these tools can elevate your work.
"Choosing the right library or framework can turn a development headache into a smooth journey, where you can focus on creativity rather than getting bogged down in repetitive tasks."
Overview of Popular JavaScript Libraries
Several libraries have gained traction among developers for their functionality and ease of use. Some noteworthy ones include:
- jQuery: Perhaps the most well-known library, jQuery simplifies HTML document traversing, event handling, and Ajax interactions. It is widely respected for its simplicity and cross-browser compatibility.
- Lodash: This utility library helps simplify common programming tasks. With functions for manipulating arrays and objects, it makes performing operations like filtering, cloning, and deep-comparing a breeze.
- D3.js: If data visualization is your goal, D3.js is indispensable. It offers powerful tools to bind data to the DOM and apply data-driven transformations.
Libraries can save time and boost productivity, especially for developers looking to produce effective solutions rapidly. They are akin to a Swiss-army knife; a small investment in learning can pay off handsomely.
Choosing the Right Framework for Your Needs
When it comes to selecting a framework, a one-size-fits-all solution doesn't cut it. The "right" choice depends on various aspects of your project, including:
- Project Scope: If youâre building a small application, a lightweight framework like Vue.js might be sufficient. For more substantial projects needing robust structure and maintainability, Angular may be the preferred choice.
- Learning Curve: Some frameworks, like React, are simpler to grasp and can help you hit the ground running. In contrast, Angular sometimes requires a steeper learning curve because of its comprehensive nature.
- Community and Ecosystem: A framework with an active community can provide immense benefits. Check for available plugins, integration capabilities, and relevant resources that can enhance your workflow.
Here are some key pointers to keep in mind:
- Prioritize your teamâs existing skill set and familiarity with the framework.
- Assess performance needs and scalability as your application grows.
- Donât rush into a decision; explore the documentation and user feedback to understand what suits you best.
Ultimately, the choice of framework can shape not just your coding practices but also your overall application architecture and user experience.
Real-World Applications of JavaScript
JavaScript isn't just about making webpages flashy or interactive. Its significance stretches far beyond those initial impressions, solidifying its position as a backbone of modern web development. This section focuses on how JavaScript permeates various aspects of technology and places an emphasis on its versatile applications, offering both budding developers and seasoned gurus insights into its day-to-day implementation.
JavaScript in Web Development
In the realm of web development, JavaScript reigns supreme. The moment you load a webpage, JavaScript plays a role behind the scenes. It allows developers to create dynamic and engaging content, enhancing user experience dramatically. Here are some critical contributions JavaScript makes to web development:
- Interactive Features: It powers interactive elements like sliders, tabs, and forms. This interactivity keeps users engaged and encourages them to explore.
- Single-Page Applications: Frameworks such as React.js and Angular utilize JavaScript to create single-page applications (SPAs). This means that users can interact with a page without full reloads, streamlining navigation and improving performance.
- API Integration: JavaScript can connect to various APIs, facilitating tasks like data fetching, sending data, and handling user authentication seamlessly. For instance, using the Fetch API or Axios allows developers to easily communicate with backend servers.
"To code is to communicate with the computer, the learning process is akin to mingling with keys and doors to open limitless possibilities."
The adjustment of JavaScript's capabilities through innovative libraries and frameworks signifies that web developers can achieve more with less code. The availability of libraries such as jQuery simplifies DOM manipulation, while Bootstrap integrates easy styling, benefiting both novice and experienced developers.
JavaScript in Mobile and Server Development
JavaScript isnât confined to the web or front-end applications; it also finds substantial use in mobile and server development. Thanks to its versatility, developers can write both client-side and server-side code using the same language, promoting efficiency and ease of learning. Hereâs how it manifests in these spaces:
- Mobile App Development: With frameworks like React Native, developers can build native mobile applications using JavaScript. This approach saves time and effort since one set of skills can cater to both web and mobile environments.
- Server-Side Applications: With Node.js, JavaScript empowers developers to run code outside the browser, on servers. This capability enables the establishment of entire applications with JavaScript, enhancing the speed of development while allowing real-time capabilities.
Express.js, a popular framework for Node.js, helps create robust back-end systems. This synergy between client and server simplifies data exchanges and creates more cohesive applications.
Resources for Further Learning
Learning JavaScript can be an overwhelming venture, much like trying to navigate a vast ocean without a compass. The resources you choose to dive into can either anchor you firmly in knowledge or leave you drifting amid uncertainty. As you progress on your journey from novice to mastery, the right learning materials will be your lifeboat, guiding you through the complexity of coding.
Recommended Books and Online Courses
When it comes to finding the right books and courses, the choices can be as varied as night and day. Books provide depth and allow for a more comprehensive understanding, while online courses typically offer practical, hands-on experiences. Here are a few recommendations that have resonated well with learners:
- Eloquent JavaScript by Marijn Haverbeke: This book is often heralded as a cornerstone for beginners. It covers a multitude of topics from basic syntax to more advanced concepts, with exercises designed to solidify your understanding.
- You Donât Know JS (Book Series) by Kyle Simpson: This series digs deep into JavaScript and challenges many common misconceptions. Itâs a favorite among developers who want a solid theoretical foundation combined with valuable insights.
- Codecademyâs JavaScript Course: An interactive platform where all the learning takes place in real-time. This makes it perfect for those who prefer a hands-on approach right from the get-go.
- Udemyâs JavaScript Bootcamp: With many updated courses focusing on the latest JavaScript versions and frameworks, Udemy offers a plethora of options that suit various learning styles and needs.
Each of these resources can be uniquely beneficial, depending on your personal style of learning and the specific skills you wish to develop. As you sift through these options, consider your goals: Are you looking to grasp the basics, or do you want to explore more advanced concepts?
Communities for JavaScript Developers
After you've amassed some knowledge, finding a supportive community can make a world of difference. Diving into the depths of JavaScript alongside others who share the same passion allows for exchange of ideas, solutions to coding challenges, and even networking opportunities. Here are a few notable platforms:
- Redditâs r/javascript: A lively community where developers share news, tutorials, and tips. Engaging in discussions can keep you updated on the latest trends and tools.
- Stack Overflow: While primarily a Q&A platform, the community here offers insights into real-world problems and solutions. It's a great resource for when you're stuck.
- Facebook Groups: Several groups cater specifically to JavaScript developers. They host discussions, challenges, and sometimes even live coding sessions.
- Discord Servers: Many coding-related Discord servers have channels dedicated to JavaScript. This allows for quick exchanges of information and getting feedback in real-time.
Engaging with a community can provide motivation. Seeing fellow learners tackle challenges can inspire you to push your own boundaries.
Building your skills in JavaScript is not a solo journey; the people you connect with can elevate your learning experience. For those looking to master JavaScript, the right communities can be equally as important as the resources you study. As you explore these neighborhoods of knowledge, remember that every question brings you one step closer to mastery.
Common Pitfalls and Best Practices
When delving into JavaScript, understanding common pitfalls and honing best practices is crucial for anyone wanting to advance in this programming language. The landscape of coding can be littered with traps for the unwary; hence, having a grasp of where these pitfalls lie can save you countless headaches down the line. Not only can avoiding mistakes keep your code cleaner, but it also enhances your ability to work effectively in teams and maintain your projects over time.
Coding isnât just about getting the job done; itâs about writing code thatâs understandable, reusable, and scalable. This section emphasizes the importance of adopting best practices in your code, which can ultimately lead to a more sustainable coding journey.
Common Mistakes to Avoid
JavaScript, while powerful, can also be tricky due to its flexible nature. Below are some common missteps that can hinder your coding efforts:
- Neglecting to declare variables: Forgetting to use keywords like , , or can lead to variables becoming global, which complicates debugging.
- Misunderstanding scoping rules: JavaScript has function scope and block scope, and misinterpreting these can cause variables to behave unexpectedly.
- Using instead of : This can cause type coercion issues, leading to bugs that are hard to trace because allows different types to be considered equal.
- Ignoring asynchronous code: Forgetting to handle callbacks properly can result in code that doesnât execute in a logical order, often seen in UI updates or data fetching scenarios.
- Copy-Pasting Code without Understanding: This can lead to bugs that are hard to diagnose, as one may misapply logic that doesn't fit the current context.
Recognizing and avoiding these pitfalls is essential. By breaking bad habits early, you ensure a smoother experience as you progress.
Writing Clean and Maintainable Code
Clean and maintainable code serves as a foundation for effective programming practices. Itâs not just a mantra; itâs a necessity. Here are some strategies to consider:
- Consistent Naming Conventions: Whether you choose camelCase, snake_case, or another naming convention, consistency is key. This makes understanding your code more intuitive for both yourself and others.
- Commenting Your Code: Not every line needs a comment, but explaining the purpose of complex code snippets helps maintain readability.
- Breaking Code into Functions: Smaller, well-defined functions can enhance modularity, enabling easier debugging and code reuse.
- Avoiding Global Variables: Whenever possible, try to keep your variables scoped within functions or blocks to prevent conflicts and enhance code organization.
- Utilizing Modern JavaScript Features: Embrace features from ES6 and beyond, like arrow functions and template literals, which can help you write cleaner and more readable code.
Remember, code is read more often than it is written.
By adhering to these practices, not only will you write better JavaScript, but youâll also create a repository of work that you can be proud of and that others can understand and maintain with ease.
Culmination
The conclusion serves as a crucial point for any learning journey, especially in a complex terrain like JavaScript. Here, itâs important to take a step back and reflect on what you've learned. This section wraps up your understanding, reinforcing key concepts that shaped your learning path. Not only does it summarize the fundamental and advanced elements of JavaScript explored in this article, but it also emphasizes the significance of continuous growth in your programming skills.
In essence, moving to mastery hasn't been a walk in the park, nor should it be viewed as a final destination. Mastery is more like a compass guiding you through the vast expanse of software development. It requires you to be proactive, to keep asking questions, and, most importantly, to keep coding.
You should think about the following benefits of introspection at this stage.
- Reinforcement of Learning: By revisiting what you previously grasped, you solidify your understanding, making it easier to recall when needed.
- Identifying Gaps: Sometimes, it takes a reflective moment to realize what areas need more attention. This stage is where you can pinpoint specific concepts that need revisiting.
- Encouragement for Future Learning: Reflection here boosts motivation and prepares you for the challenges ahead.
"Learning is not the product of teaching. Learning is the product of the activity of learners." â John Holt
As you reflect, consider these elements: Did you find a specific section particularly challenging? Were there a-ha moments that illuminated your understanding? Or did certain tutorials on W3Schools resonate more than others? These realizations will offer guidance as you think about the next steps in dev eloping your skills.
Reflecting on Your Learning Journey
Reflecting on your learning journey isnât just a post-mortem; itâs a necessary practice. Acknowledge your challenges, celebrate your victories, and learn from your missteps. In the world of code, problems pop up like weeds, yet each resolved issue contributes to your growth. Grab a notepad or open a new document, and start jotting down your experiences:
- Challenges Encountered: What bugs or errors did you struggle with? How did you navigate them?
- Resources Utilized: What did you find more useful? Was it W3Schools or perhaps sources like Wikipedia and Britannica that illuminated difficult concepts for you?
- Community Interaction: Did you engage in forums like Reddit to seek help? How did this shape your learning?
Each of these insights will serve as both a ledger of accomplishments and a roadmap for your ongoing education in programming.
Next Steps in Your JavaScript Mastery
Your journey doesnât end here. The land of JavaScript is vast, teeming with libraries, frameworks, and advanced methodologies waiting for you to explore. Ask yourself: What do you want to tackle next? Not every programmer is the same, so your path might diverge from others. Here are some options:
- Deepen Your Understanding of Frameworks: Consider diving into frameworks like React or Angular, shaping how you build interfaces.
- Explore Node.js: Understanding server-side development can broaden your skill set, making you a full-stack developer.
- Engage with Open Source Projects: Contributing to projects on platforms like GitHub can not only enhance your coding ability but also expand your network.
- Stay Ahead with Continuous Learning: Follow blogs, subscribe to YouTube channels, or engage in online courses to keep up with new developments.
Ultimately, the key takeaway is to stay curious. Build projects, break things, and immerse yourself in the JavaScript community. The more you practice, the closer you inch towards craft mastery.