Mastering JavaScript and React for Web Development
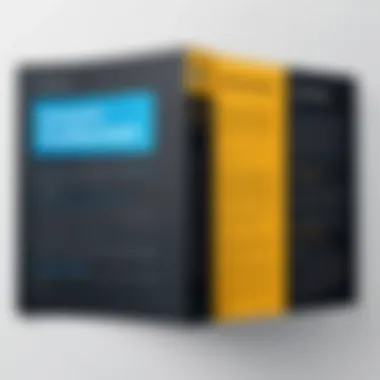
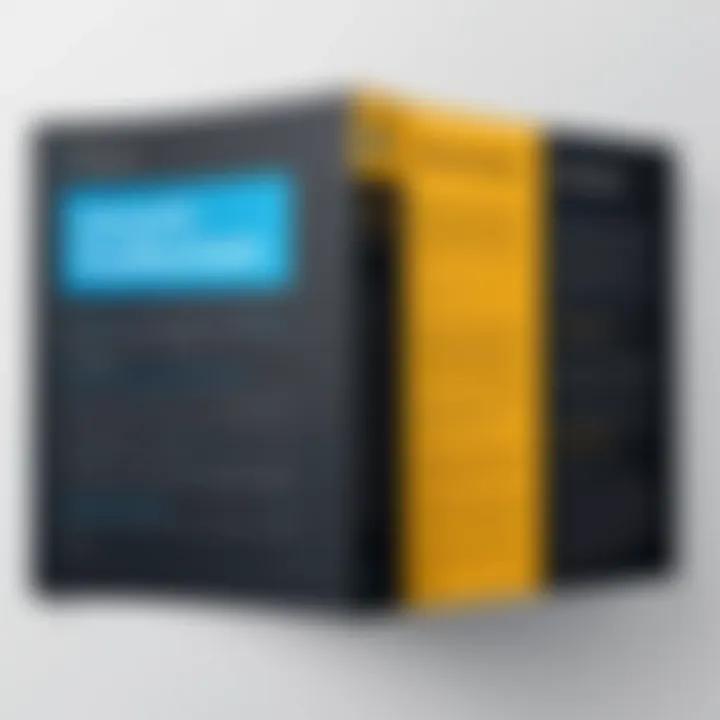
Prolusion to Programming Language
Programming languages have become the backbone of modern technology, allowing us to communicate with machines. Among these languages, JavaScript stands out, particularly when paired with the React library. This section aims to provide an understanding of JavaScript, setting the stage for its integration with React.
History and Background
JavaScript, developed by Brendan Eich in 1995, was initially conceived as a simple scripting language for web browsers. Since then, it has matured into a robust language powering much of the modern web. Over the years, its capabilities have drastically expanded, enabling developers to build complex, interactive web applications. In 2015, ECMAScript 6 brought significant enhancements, further solidifying JavaScript's place in the programming world. The emergence of Node.js in 2009 allowed for server-side scripting, making JavaScript even more versatile.
Features and Uses
JavaScript is a multi-paradigm language, meaning it supports different styles of programming, including procedural, object-oriented, and functional programming. Its asynchronous nature lets developers handle operations like data loading without freezing the user interface. Common uses of JavaScript include:
- Making web pages interactive
- Validating forms before submission
- Creating server-side applications using Node.js
- Building mobile and desktop applications using frameworks like React Native and Electron
Popularity and Scope
JavaScript is not just a fad; it's a fundamental technology of the internet. According to the Stack Overflow Developer Survey, JavaScript has consistently held the title of the most popular programming language. Its ecosystem, enriched by frameworks and libraries like React, Angular, and Vue, plays a key role in web development. The scope of JavaScript extends beyond just the webāit is also employed in game development, Internet of Things (IoT) applications, and even artificial intelligence.
"For every complex problem, there is an answer that is clear, simple, and wrong."
As such, both beginners and seasoned developers find JavaScript to be a valuable skill for their careers.
Basic Syntax and Concepts
Understanding the basic syntax of JavaScript is crucial for any aspiring developer. This section will delve into important elements that form the foundation of the language.
Variables and Data Types
JavaScript provides several ways to define variables. You can use , , or to create variables which can hold different types of data:
- Primitive types: include strings, numbers, Booleans, null, undefined, and symbols.
- Reference types: include objects, arrays, and functions.
Example of variable declarations:
Operators and Expressions
Operators are vital for performing operations on variables and values. JavaScript offers a variety of operators:
- Arithmetic operators: for calculations (+, -, *, /)
- Comparison operators: for comparing values (==, ===, !=, !==)
- Logical operators: for logical conjunctions (&&, ||, !)
Control Structures
Control structures dictate the flow of code execution in JavaScript. The most common include:
- If statements: to branch logic based on conditions
- Loops: such as , , and to repeat actions
Example of an if statement:
These features set the groundwork for more complex programming concepts and will integrate seamlessly with React.
Advanced Topics
Once the basics are understood, diving into advanced topics allows programmers to leverage JavaScript's full power in web development.
Functions and Methods
Functions are first-class citizens in JavaScript. They can be assigned to variables, passed as arguments, and returned from other functions.
Example of a function:
Object-Oriented Programming
JavaScript supports object-oriented programming through its unique prototype-based inheritance model. Objects can encapsulate data and functionality together.
Exception Handling
Robust applications need proper error handling. JavaScript provides , , and blocks to manage exceptions gracefully.
Example:
Hands-On Examples
To cement knowledge, hands-on examples are crucial. Let's look at a few:
Simple Programs
Creating a basic calculator helps solidify the understanding of operators and functions. By taking input from users, you can perform arithmetic operations.
Intermediate Projects
Building a to-do list application incorporates critical concepts of functions and objects, paving the way for React integration. This involves creating, updating, and deleting tasks.
Code Snippets
Short code snippets can be useful for quick reference or demonstration of JavaScript features:
Resources and Further Learning
Engaging with the wider programming community and accessing further resources can greatly enhance learning.
Recommended Books and Tutorials
- Eloquent JavaScript by Marijn Haverbeke
- You Donāt Know JS series by Kyle Simpson
Online Courses and Platforms
- freeCodeCamp
- Codecademy
Community Forums and Groups
- Reddit's r/learnprogramming
- Stack Overflow
By leveraging these resources, students will find themselves well-equipped to master JavaScript and React, paving the way towards a fulfilling career in programming.
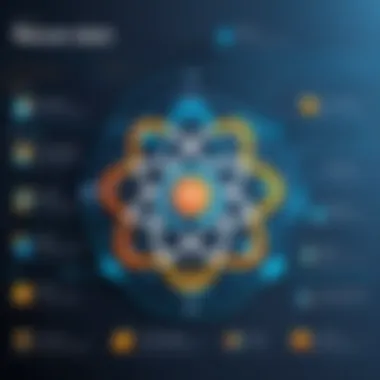
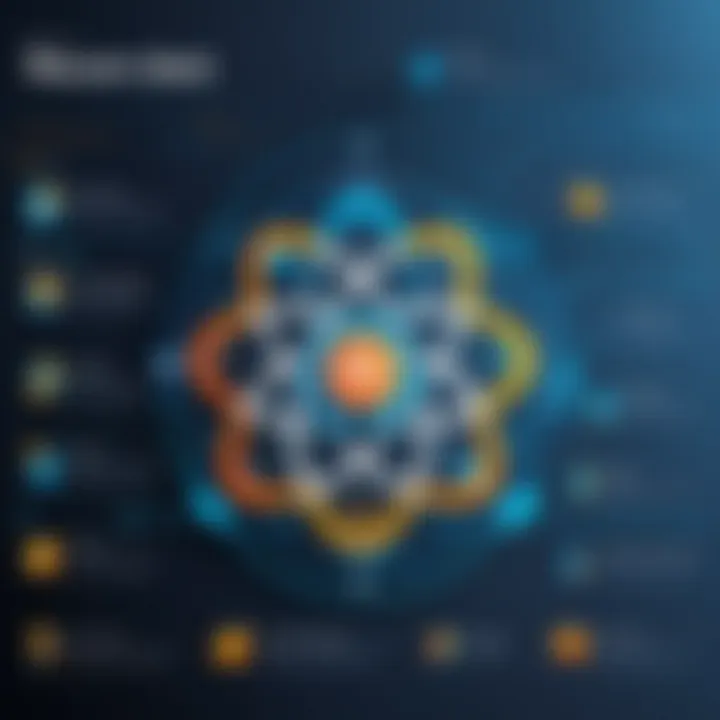
Prolusion to JavaScript
JavaScript stands as the backbone of modern web development, making it an essential component of the educational journey in mastering both JavaScript and React. This language has undergone significant transformations since its inception, evolving from a simple scripting tool into a full-fledged programming powerhouse. The importance of JavaScript lies not merely in its functionality but in its impact on how users interact with web applications. Today, understanding JavaScript is not just about writing code; itās about grasping a rich tapestry of concepts that empower developers to create dynamic, responsive, and interactive experiences.
History and Evolution
JavaScript was created in 1995 by Brendan Eich during his tenure at Netscape. Originally dubbed Mocha, it was later renamed to JavaScript to capitalize on the hype around Java at the time. This early version was quite rudimentary and served primarily to add interactivity to web pages. As browsers began to support increasingly complex applications, the language itself underwent several revisions to enhance its capabilities.
By the early 2000s, JavaScript faced some challenges, notably from competing technologies. However, the release of Ajax (Asynchronous JavaScript and XML) played a pivotal role in resurrecting its status, enabling more interactive web applications without requiring full-page reloadsāa drastic improvement in user experience. Fast forward to today, JavaScript is standardized under ECMAScript, with regular updates introducing new functionalities like arrow functions and async/await syntax, each iteration further solidifying its status at the core of web development.
Core Features
JavaScript's distinct features contribute to its widespread adoption and effectiveness.
Variables and Data Types
The fundamental element of JavaScript is its handling of variables, which are containers for storing data values. Variables can hold different types of information including numbers, strings, and objects. One of the unique aspects of JavaScript is its weak typing, allowing for flexible and dynamic assignment without rigid type control. This characteristic enhances its usability, particularly for beginners who may find stricter languages daunting.
The data types include:
- Primitive types: strings, numbers, booleans, null, undefined, and Symbols.
- Reference types: objects, arrays, and functions.
Such diversity not only fosters simplicity but also offers a clear pathway for managing complex data structures in applications. However, this flexibility might lead to issues if not handled with care, particularly in larger projects where data types can easily lead to unforeseen bugs.
Functions and Scope
In JavaScript, functions are first-class citizens, meaning they can be stored in variables, passed as arguments, and returned from other functions, allowing for a high degree of flexibility in coding. Functions define the behavior of JavaScript programs, and understanding scopeāwhether global or localāis crucial in managing how variables are accessible throughout the code.
One can leverage closures to maintain state in asynchronous environments or perform tasks like data fetching in React components. However, new developers might feel overwhelmed by the scope quirks of JavaScript, such as the difference between , , and , which can lead to unintended consequences if misunderstood.
Asynchronous Programming
Asynchronous programming in JavaScript is a standout feature that allows the execution of code without blocking the main thread. This is particularly pertinent for operations that involve waiting for resources, such as API calls, enhancing user experience by not freezing the application while waiting for responses.
JavaScript introduced Promises and eventually the async/await syntax to better handle async operations, improving readability and error handling. By grasping these concepts, developers can craft smoother applications with minimal hiccups. Still, newcomers to JavaScript frequently struggle with understanding callbacks and Promises, which may lead to what's colloquially known as "callback hell" when multiple asynchronous calls are chained together.
JavaScript in the Browser
JavaScript's interaction with web browsers is what truly sets it apart. Every modern browser integrates a JavaScript engine, allowing it to run scripts directly on the client side. This capability enables developers to create responsive interfaces that can instantly react to user actions, such as clicks or form submissions, without the need for data to be sent back and forth to a server.
Moreover, JavaScript can manipulate the Document Object Model (DOM), giving developers control over the structure and style of web pages dynamically. This means that developers can craft immersive user experiences that feel more app-like than traditional web pages. The importance of this feature cannot be overstated; it represents the bridge between raw data and delightful user interfaces, affirming JavaScript's essential role in the ecosystem of web technologies.
Understanding React
React is a critical library in the modern web development landscape. Knowing how to effectively utilize React opens up new avenues for building dynamic and responsive user interfaces. One may wonder why mastering React is necessary in conjunction with JavaScript. The answer lies in the powerful features and structured approach React brings to the table, making it a favorite among developers.
React Overview
Component-Based Architecture
Component-Based Architecture underpins React's functionality, allowing developers to break down a user interface into smaller, reusable pieces. Each of these pieces, or components, is self-contained and manages its own state, which contributes to a cleaner and more organized codebase.
This architectural style offers a significant advantage: reusability. When you build a useful component, you can reuse it throughout your application, saving time and reducing errors. Think about it as building with Lego blocks; once you have the right pieces, putting them together becomes much easier.
Moreover, each component can be independently updated without the fear of affecting the entire application. However, there is a caveat: juggling many components can lead to challenges in state management and maintaining data flow. A thorough understanding of how to structure these components is essential for any developer.
Declarative vs. Imperative Programming
In the realm of programming, the choice between declarative and imperative styles can have profound impacts on how we write and think about our code. React predominantly employs a declarative approach. This means you describe what the user interface should look like based on its current state rather than detailing the steps needed to achieve that look.
The key characteristic here is clarity. With declarative programming, your code becomes more readable, making it easier to understand for both you and others. Youāre telling React what you want, and it handles the nitty-gritty of how to do it.
In contrast, imperative programming requires you to spell out every detail. This approach can result in more complex code thatās tougher to navigate.
Advantages and Disadvantages
- Declarative: Easier to read and maintain, focuses on the 'what' instead of the 'how'.
- Imperative: Greater control over the flow of operations but at the cost of increased complexity.
Installation and Setup
Getting started with React is straightforward, making it accessible even for those who are newer to programming. Setting up a React application means youāre setting the stage for building sophisticated user interfaces with efficiency and elegance.
Creating React Applications
Creating a React application involves setting up a development environment that allows you to write, test, and run your code. This is fundamentally important because it establishes the foundation upon which you will build features and functionality.
A common method to create a React application is to use frameworks or build tools like Webpack or Parcel. This may sound a bit intimidating, but itās less daunting than it sounds. Tools like Create React App help streamline this process and can be a lifesaver for beginners.
Using Create React App
Among the simplest methods for getting started in React is using Create React App. Itās a command-line tool that sets up a new React project with just a single command. You wonāt need to configure build tools or worry about complex setup processes.
The unique feature of Create React App is its zero-config setup, which allows beginners to dive straight into writing React components without being bogged down by the intricacies of development tooling. Just run the command:
"Simplicity is the soul of efficiency."
Overall, understanding these foundational concepts prepares you to tackle more complex topics and dive deeper into the full potential of React.
Building Blocks of React
The concept of building blocks in React serves as the foundation for creating dynamic and efficient web applications. Understanding these components is crucial for anyone looking to master React as they encapsulate the main practices and patterns utilized in this library. These building blocks, mainly components and state management practices, allow developers to construct applications that are not only robust but also maintainable and scalable.
Components and Props
Components form the backbone of any React application. They are reusable pieces of code that can represent part of a user interface. Understanding how to effectively use components and the props they accept is critical for efficient coding in React.
Functional Components
Functional components in React are simply JavaScript functions that return JSX. They are celebrated for their simplicity and ease of use. A key characteristic of functional components is that they do not maintain their own state, making them an ideal choice for representing UI that is static or driven purely by props. This simplicity is a significant reason why developers often favor them for presentational purposes.
Another unique feature of functional components is their compatibility with React Hooks, which allow for managing state and side effects in a more expressive way. This enables developers to write cleaner code compared to the class-based components of yesteryear.
While functional components are lauded for their simplicity, itās worth noting that they may fall short in some complex scenarios where lifecycle methods are required. However, this concern has been largely mitigated with the introduction of Hooks, making functional components even more powerful and flexible.
Class Components
Class components, on the other hand, are ES6 classes that extend from . They are more complex compared to functional components and allow for local component state. This ability is beneficial for more sophisticated applications where components need to manage their own state and use lifecycle methods to have control over the rendering processes. The key characteristic here is the structured way to handle state and lifecycle through methods like , , and .
A unique feature of class components is the potential for more granular control over the component's local state and lifecycle. However, this comes at a cost ā they tend to be more verbose and can result in code that is harder to read and maintain. This complexity sometimes discourages new developers from using class components unless necessary, favoring the ease of functional components instead.
State Management
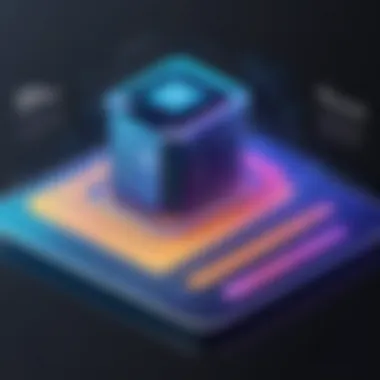
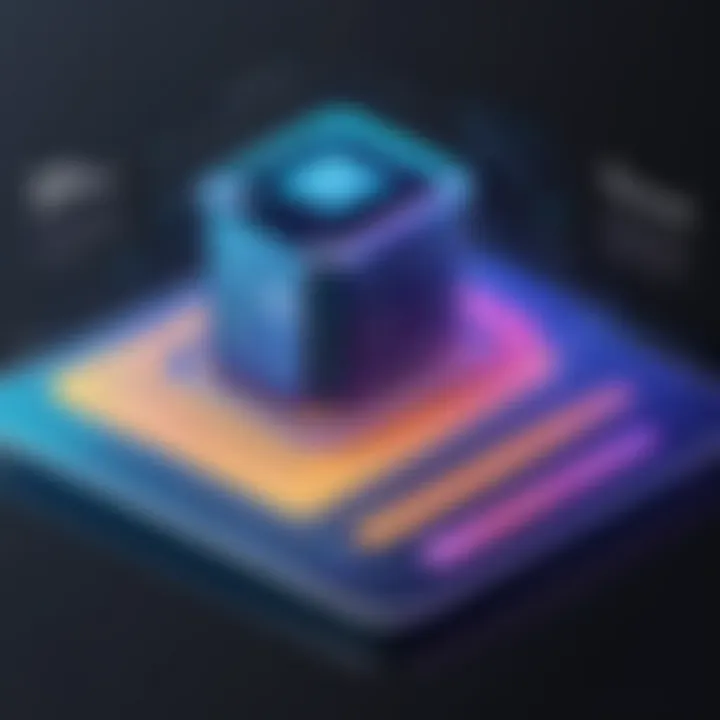
State management is another crucial building block in React as it allows components to maintain information that can change over time. Grasping the dynamics of state management can significantly enhance the interactivity of applications.
Understanding State
To understand state is to grasp one of React's core functionalitiesāit allows a component to maintain dynamic data. Each component in React can have its state that influences the rendering of the UI. This is a powerful feature because it gives developers control over how the user interacts with the application.
The key characteristic of understanding state is recognizing that state is local to the component but can be passed down through props. This makes it easy to manage application flow, but also poses considerations regarding data flow and debug responsibilities within a given state space. Its unique feature is that it encourages a clearer separation of concerns by compartmentalizing data management, easing the maintenance burden as the app grows.
Updating State
Updating state effectively is fundamental for building dynamic applications in React. The method is essential for triggering re-renders and ensuring the UI stays in sync with data changes. This characteristic allows developers to render their UI with the latest information without manually manipulating the DOM.
A major advantage of updating state correctly is that it can enhance user experience by providing responsive feedback based on interaction. However, one must also be cautious; it's easy to introduce bugs if updates aren't handled properly, particularly in asynchronous contexts. Thus, understanding the conventions around state updates is vital for guiding development practices and ensuring responsive feedback loops in user interaction.
In summary, mastering these building blocksācomponents, props, and state managementāarms you with essential skills to craft effective applications in React. Each element has its own benefits and trade-offs, making them pivotal in forming a deeper understanding of this powerful library.
Integrating JavaScript with React
When it comes to merging JavaScript with React, understanding this relationship is fundamental for building effective web applications. The integration plays a vital role because React is fundamentally JavaScript; it's a library designed for crafting user interfaces using JavaScript. By understanding how to leverage JavaScript within React components, developers can create more dynamic, interactive experiences for users.
Moreover, integrating JavaScript empowers developers to utilize existing knowledge of the language while taking advantage of React's more structured approach to handling the user interface. This effortless interaction enhances productivity and brings fluidity to web application development.
Event Handling
Event handling is crucial in React as it allows developers to manage user interactions efficiently. In traditional JavaScript, attaching event listeners can be cumbersome, often leading to messy code. React simplifies this with a cleaner syntax. You can directly specify event handlers as props on your components.
For instance, consider a simple button component:
In this snippet, the event handler is passed as a prop, which is both straightforward and intuitive. This integration shows how React abstracts complexities, letting developers focus on creating functionality instead of wrestling with JavaScript events.
Benefits of Event Handling in React:
- Simplified Syntax: Event handling becomes easier to understand and manage.
- Automatic Binding: React automatically binds the context in class components, eliminating common pitfalls with traditional JavaScript.
- Component Lifecycle Awareness: Events in React can be managed efficiently in line with the component lifecycle, allowing for cleaner, more maintainable code.
Conditional Rendering
Conditional rendering serves as another critical pillar when integrating JavaScript with React. It allows components to dynamically display content based on specific conditions, making applications more responsive to user actions and state changes.
Instead of managing complex visibility toggling with JavaScript, React encourages a declarative approach. With expressions and conditional statements directly in the render function, you can easily determine what gets displayed.
Hereās a simple example:
In this example, if is true, it shows a welcome message; otherwise, it prompts for login. This makes your component much cleaner and easy to follow.
Key Considerations for Conditional Rendering:
- Efficiency: By conditionally rendering only whatās necessary, applications can be optimized to load faster.
- Readability: Code can be more readable, as it clearly shows intents without unnecessary boilerplate.
- Improved User Experience: Users receive instant feedback and tailored content based on their interaction, greatly enhancing overall engagement.
Lists and Keys
The ability to render lists efficiently is another significant aspect of integrating JavaScript within React. Lists in React must always come with a unique prop which helps the virtual DOM to identify which items have changed, are added, or removed. This optimization leads to better performance since React can re-render only the items that have changed rather than the entire list.
Here is a quick illustration of rendering a list:
In this case, each fruit is mapped to a list item with a unique . It is important to use stable unique IDs as keys when possible, instead of using the index.
Why Keys Matter in Lists:
- Performance Improvement: Helps React manage updates efficiently.
- Avoids Bugs: Unique keys prevent issues when items are reordered, added, or removed, ensuring consistent component behavior.
Advanced React Concepts
In the realm of modern web development, mastering advanced concepts of React is akin to learning to speak fluently in a new language. Advanced React Concepts not only empower developers to build highly efficient applications but also allow for better organization of complex codebases. These concepts bridge the gap between basic understanding and practical application, making them essential for anyone looking to carve a niche in React development.
Understanding hooks and the Context API enriches oneās capability to manage state and lifecycle events elegantly. As applications grow in size and complexity, efficient state management becomes paramount. Leveraging the right tools can lead to enhanced performance and improved user experience. Letās delve into the specifics of these advanced topics.
Hooks
Preamble to Hooks
Hooks fundamentally changed the way components are managed in React. They allow developers to use state and other React features without writing a class. The key characteristic of the Introduction to Hooks is their simplicity and direct integration with functional components. They provide a clean, intuitive way to encapsulate component logic. For instance, with hooks, there's no longer a need to wrangle with the complexities of bindings that are common in class components.
A unique feature of hooks is their ability to share stateful logic between components. This flexibility offers several advantages, including less boilerplate code and improved organization. However, hooks do come with their own set of rules, primarily that they must be called at the top level of a component and not nested within loops or conditions. This flexibility can lead to challenges if not managed thoughtfully.
Using useState and useEffect Hooks
When discussing Using useState and useEffect Hooks, we dive into two of the most used hooks that underpin Reactās powerful features. The hook allows functional components to maintain state. It provides a key benefit: more concise and readable code that gives developers the control to update state directly. This radically simplifies state management as compared to traditional class components.
On the other hand, the hook deals with side effects in components. It acts as a bridge that connects React components with the outside world, such as fetching data or interacting with the DOM. The beauty of is its ability to run code after render, enabling asynchronous calls without disrupting the flow of the application.
However, while powerful, these hooks require a decent understanding of their dependencies. Mismanaging dependencies can lead to unnecessary re-renders or missed updates, which can be frustrating to debug.
Context API
Moving on to the Context API, this feature addresses some of the limitations presented by React's prop-drilling concept. In applications where certain data must be accessible at various levels of the component tree, the Context API offers a streamlined way to share data across components without having to pass props down explicitly. This can simplify the flow of data immensely.
One of the main benefits of using the Context API is the reduction of props drilling. Instead of passing data through numerous layers, you can wrap a component tree with a context provider that allows child components to access shared data directly. Itās particularly advantageous in larger applications where managing state across various components can quickly become tangled.
Yet, one must be cautious with its implementation. Overusing context can lead to performance issues and make components less reusable. Therefore, understanding when and how to utilize the Context API is crucial for its effective application.
"The future of web development necessitates a thorough grasp of state management, with Reactās Hooks and Context API at the forefront."
The End
Practical Applications
Understanding the practical applications of JavaScript and React is key for anyone looking to develop real-world web applications. While theoretical knowledge lays the groundwork, practical experience sharpens your skills and deepens your understanding. In this section, we dive into two important areas: building a simple To-Do application and integrating APIs, both pivotal in day-to-day programming tasks.
Building a Simple To-Do App
Project Setup
Setting up a project might sound mundane but it plays a crucial role. Proper setup helps maintain organization and clarity throughout the development process. A well-structured project can save you a mountain of trouble down the line. For this article, we use Create React App as it provides a neat boilerplate. Its simplicity allows novices to get projects rolling without needing to configure everything from scratch.
A key characteristic of this approach is simplicity. You get predefined scripts and configurations. It is popular because it takes away the initial headache of tooling, letting you focus on learning Reactās core concepts. This feature allows new developers to jump right in without having to wade through setup documentation.
However, a downside might occur when advanced users seek heavy customizations. Yet, it remains a beneficial starting point for beginners eager to grasp React efficiently.
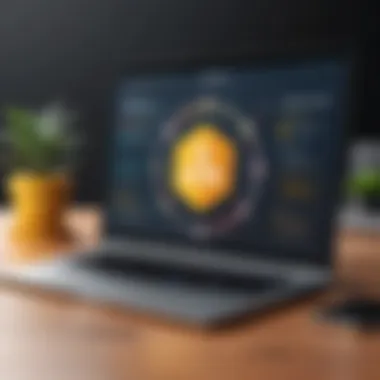
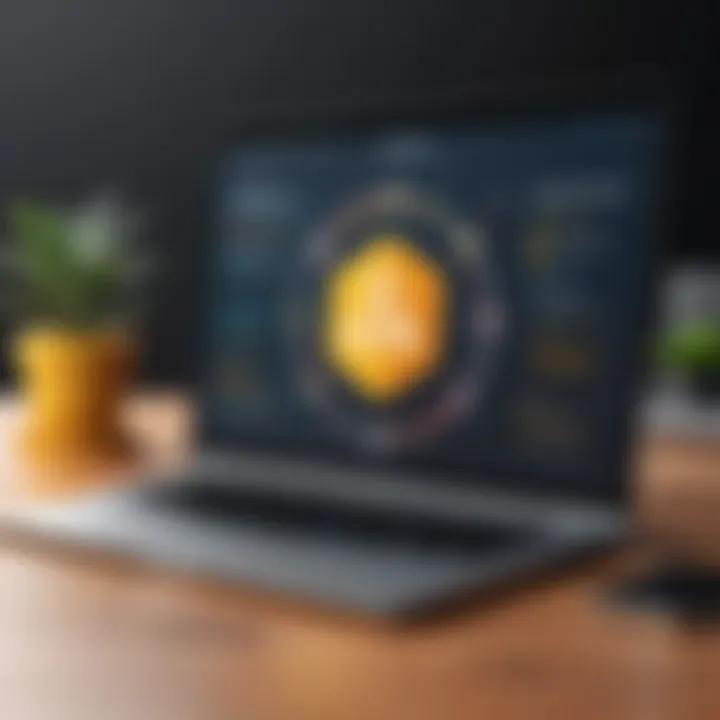
Implementing Features
Once the project is set, the real fun begins: implementing features. Here, we construct the fundamental functionalities of a To-Do app. This often includes adding, removing, and marking tasks as completed. Implementing features like this emphasizes the concept of state management, reinforcing your understanding of how React manages data.
A core benefit of focusing on features is that it is highly relatable. Almost everyone has used a To-Do app, making it a practical choice that serves to enhance learning engagement. A unique detail here is that as you implement each feature, you see instant results in your application. This immediate feedback can be incredibly rewarding, keeping motivation high.
On the flip side, as features grow complex, so does state management. You may run into challenges like prop drilling or inefficient re-renders. Still, the experience you gain while navigating these challenges is invaluable and prepares you for more complex projects in the future.
Integrating APIs
Fetching Data from APIs
In modern apps, data is usually sourced from APIs, making fetching data a vital skill. By integrating APIs into our To-Do app, we advance our understanding of asynchronous programming, which is essential in JavaScript. APIs allow an app to fetch resources dynamically, thus enhancing functionality.
The beauty of fetching data lies in its real-time nature. You can bring in live data from external sources, keeping your app fresh and relevant. This aspect is a huge factor in today's web applications, especially those relying on user-generated content or external databases.
However, complexities may arise such as handling errors or managing various response data formats. Understanding these nuances gives you a more comprehensive grasp of how web applications interact with the outer world.
Displaying Data in Components
Now that you can fetch data, displaying it in your components is the next step. This aspect highlights Reactās core strength: component-based architecture. With every piece of data displayed, you are showcasing your ability to manage props and state.
A standout characteristic of this step is reusability. Display components can be reused for different data types, making your code cleaner and more efficient. When components are designed to accept props, you gain the flexibility to adapt to various data scenarios, which is essential for scalable applications.
On the downside, getting the display logic right can sometimes be trickier than expected, especially when dealing with nested data structures. However, mastering this process deepens your comprehension of Reactās rendering lifecycle and prepares you for larger, more intricate projects.
Integrating practical applications bridges the gap between theory and practice, making learning effective.
In summary, practical applications not only cement your knowledge but also graft theory onto functional skills. The experience gained through building familiar applications like a To-Do app and integrating APIs allows you to step into the real world of programming with confidence.
Testing React Applications
Testing is an integral part of software development, especially in the realm of React applications. It not only ensures that your code is working as expected, but it also helps maintain code quality and enhances the reliability of your application. When developing with React, incorporating testing can save a lot of headaches later on. This section will delve into the essentials of testing in React, outlining why it matters, the benefits it brings, and key considerations when implementing tests.
Among the benefits, testing can facilitate easier debugging and help in catching bugs earlier in the development cycle, which ultimately leads to a higher-quality product. It can also serve as a form of living documentation that others can refer to when they need to understand how different parts of your application function. Moreover, a well-tested application can boost the confidence of developers since it is easier to modify without fear of breaking expected behaviors.
The inclusion of tests is based around two key elements: unit tests and integration tests. Each of these plays a distinct role in ensuring the robustness of your application. Well-conceived tests lead to a streamlined development process where new features can be implemented with less risk.
Preface to Testing
In the context of React, testing becomes essential for ensuring components behave correctly. This isnāt just about making sure they display the right information; itās about testing how they interact with one another and how they respond to user actions. Unit testing is aimed at testing the smallest possible parts of an application in isolation. This could be a single function or component.
Types of Testing in React:
- Unit Testing: Focuses on individual components. For instance, if a button is supposed to trigger a function, a unit test would check if that function indeed gets called upon interaction.
- Integration Testing: Examines how several components work together. This could involve testing a form component that combines an input field and a submit button to see if it updates state correctly.
There are several frameworks and libraries available for testing React components, but the significance of starting with a robust testing foundation cannot be overstated. Familiarity with how to write tests will not only enhance the quality of the code but also give peace of mind to developers when deploying code to production.
Using Jest with React
Jest is one of the most popular testing frameworks used in the React ecosystem. Developed by Facebook, it provides a rich feature set that makes it a preferred choice among developers. One of the key advantages of Jest is its simple and intuitive API, which enables developers to write tests quickly.
Features of Jest
- Snapshot Testing: This allows you to capture a rendered component's output and compare future outputs against the original snapshot. If thereās a change, Jest will alert you, making it easy to identify unexpected modifications in your component.
- Mock Functions: Jest brings the capability to mock functions, which is beneficial when testing components that depend on various external data or processes. This isolation helps you focus on the logic without unnecessary complexity.
Hereās a simple example that shows how you can set up a basic test using Jest for a React component:
In this example, we are testing that the renders a particular text as expected. As you expand your test suite, the confidence in your application will also increase, allowing for smoother changes and feature additions.
Remember that while the goal is to write thorough tests, it is just as important to be mindful of not over-testing or writing redundant tests. Striking a balance is key.
Through understanding these concepts of testing, React developers can significantly enhance the quality and maintainability of their applications, ensuring that users have a seamless experience.
Deployment and Hosting
Deployment and hosting are critical phases in the life cycle of any application, particularly when working with frameworks like React. After spending countless hours devising a beautiful interface and seamless user experience, the last thing you want is a clumsy deployment process to ruin all your hard work.
Proper deployment ensures that your application runs smoothly in a production environment. It allows users to access your site via a web browser, transforming your application from a local development project into a web-based product. Having a reliable hosting platform can also significantly influence performance, scalability, and security of your application. Selecting the right strategies and solutions will ultimately reflect on how users perceive your application, which can make or break your credibility like a house of cards.
Preparing for Deployment
Before initiating the deployment process, your application should be thoroughly tested to ensure that all bugs are ironed out. The final product needs to be a polished and fast app that users find easy to navigate. Some key steps to consider during preparation include:
- Minifying and optimizing your code to improve load times.
- Ensuring all components function as expected.
- Setting environment variables, ensuring that your application has everything it needs to communicate with back-end services.
- Implementing error handling to manage any potential issues that arise during the deployment process.
After performing these checks, youāll be seated on a stronger foundation, better equipped for whatever deployment might throw your way.
Hosting Platforms
Netlify
Netlify has made waves in the web development community, and for good reason. It simplifies the continuous deployment process through its user-friendly interface and hassle-free setup. The key feature that sets Netlify apart is its ability to integrate seamlessly with Git; every time you push your code, it automatically rebuilds your site. This characteristic allows developers to focus on coding instead of worrying about deployment.
Netlify provides exceptional performance, including features such as instant cache invalidation and optimized image handling, which significantly improves loading times. However, itās not all sunshine and rainbows. Developers have pointed out that certain advanced features might require digging deep into the documentation, which could be a bit overwhelming.
"Netlify redefines the deployment game, allowing developers to deploy with just a push of the button!"
Vercel
Vercel, formerly known as Zeit, has gained a reputation for its speed and simplicity. Primarily targeted towards Next.js apps, Vercel offers a smooth deployment experience. One of its notable characteristics is the support for serverless functions, which allows developers to execute backend logic without the need for a dedicated server. With Vercel, your applications can scale automatically based on demand, making it a popular choice among developers who want peace of mind regarding performance.
One unique feature of Vercel is the support for preview deployments, meaning you can review changes in a live environment before merging to your main branch. This capability significantly reduces the risk of introducing bugs into your production code. However, like any tool, Vercel has its downsides; the speed it offers can sometimes mask underlying issues in your app that require fixing.
In summary, choosing the right hosting platform will drastically affect deployment and runtime performance, influencing how well your application serves its users. With the options available like Netlify and Vercel, identifying which one suits your specific needs is imperative.
The deployment phase, paired with effective hosting solutions, not only enhances a userās experience but also plays a crucial role in shaping the journey of your application from inception to end-user interaction.
Culmination
Wrapping up our exploration of JavaScript and React, itās crucial to recognize the significance of this conclusion. This isn't merely an ending; it encapsulates everything we have discussed throughout the guide. Understanding the role of JavaScript in web development while grasping how React enhances user interfaces is foundational.
Summary of Key Concepts
When we think about the key concepts, several points stand out:
- JavaScript Fundamentals: We delved into variables, data types, functions, and even asynchronous programming. Understanding these concepts is critical as they form the backbone of React development.
- React Architecture: The component-based approach in React allows developers to build encapsulated components that manage their own state, promoting reusability and maintainability.
- State Management: Recognizing how state works in React, and how it can be updated effectively, helps create dynamic web applications.
- Advanced Patterns: We brushed upon hooks and the context API, which foster a more functional approach to managing state and side effects in React, making the code cleaner and more efficient.
- Testing and Deployment: Testing applications ensures quality before deployment, and knowing about various hosting platforms assures smooth transitions from development to production.
These core ideas are not just compartmentalized but interconnected. They weave together to create a fortifying framework that prepares you for real-world applications.
Next Steps in Learning
As you look ahead, consider these actionable steps to continue your learning experience:
- Practical Projects: Start by building small applications. Whether itās a to-do list or a personal blog, hands-on practice is invaluable.
- Explore Advanced Topics: Dive deeper into React's ecosystem. Look into libraries like Redux for state management or Next.js for server-side rendering.
- Participate in Communities: Join forums on platforms like reddit.com or Facebook groups focused on JavaScript and React. Engaging with the community can offer insights and support.
- Online Courses & Resources: Seek out courses on platforms like Codecademy or freeCodeCamp that focus on JavaScript and React. Having structured learning paths can enhance your comprehension and proficiency.
- Stay Updated: The tech landscape evolves rapidly. Follow blogs, podcasts, or newsletters to keep pace with changes and emerging best practices in JavaScript and React.
By following these steps, you craft a solid foundation and pave the way for continuous learning. Embrace this journey, and youāll find the world of JavaScript and React vast and rewarding.
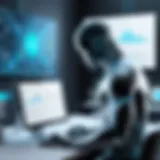
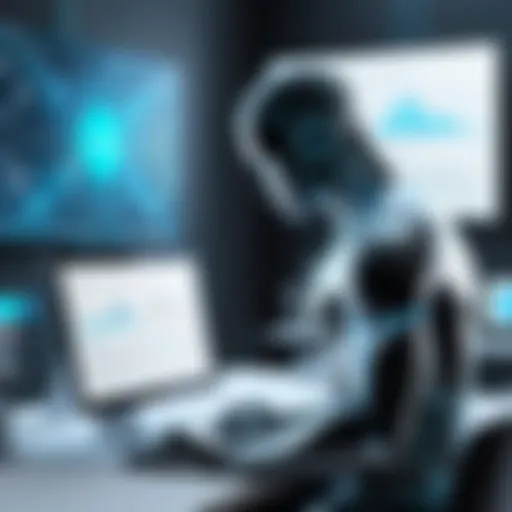