Master JavaScript: Essential Strategies for Success
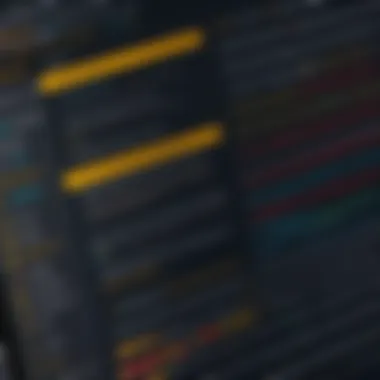
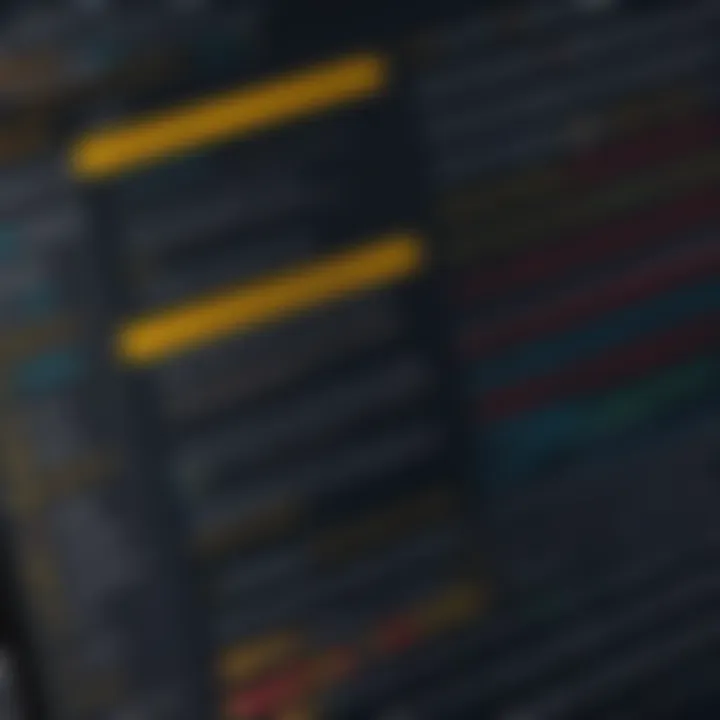
Prolusion to Programming Language
JavaScript, often called JS, is a programming language that has shaped the landscape of modern web development. Developed by Brendan Eich in the mid-1990s, it quickly became integral to web browsers, allowing developers to create interactive and dynamic user experiences. This section will give a broader understanding of JavaScriptās history and its significant role in todayās technology.
History and Background
JavaScript was introduced in 1995 and initially named Mocha, later known as LiveScript. It made its debut in Netscape Navigator as a means to enhance web pages; a far cry from the static HTML of the time. Over the years, it evolved, with the most pivotal moment occurring in 1996 when it was standardized under the ECMAScript name. This formal acceptance provided a framework that many developers followed, catalyzing its spread across different browsers.
Features and Uses
JavaScript is versatile and allows for a variety of uses:
- Client-side Scripting: Running scripts directly in the userās browser, reducing server load.
- Server-side Development: With environments like Node.js, JavaScript stretches beyond browsers and into server management.
- Mobile Application Development: Frameworks like React Native enable developers to build native apps using JavaScript.
- Game Development: It powers many web games, showing its adaptability even in graphics-heavy environments.
The language also supports asynchronous programming, meaning operations can run in the background, enhancing user experience without waiting for tasks to finish.
Popularity and Scope
The popularity of JavaScript really speaks for itself. According to Stack Overflow, it is often listed as the most commonly used programming language. Many frameworks and librariesālike React, Angular, and Vue.jsāhave surfaced to simplify complex tasks and enhance functionality. JavaScript is everywhere; from simple website animations to complex single-page applications, the scope and reach of this language are vast.
"JavaScript made web development a sandbox where creativity meets functionality."
The language has become essential for anyone delving into web technologies. Understanding its core principles not only gives one an edge in programming but also opens doors to various career opportunities in tech.
In summary, gaining a solid grounding in JavaScript is vital, both for beginners and seasoned programmers. Knowing its history and significance provides context as we delve deeper into the craft of mastering this pivotal programming language.
Intro to JavaScript
JavaScript holds a pivotal role in web development, transcending its origins as a simple scripting language to become a cornerstone of modern interactive and dynamic websites. Its significance goes beyond mere utility; it enhances user experiences and enables developers to mold the digital landscape. For anyone embarking on a journey into programming, grasping JavaScript is not simply beneficialāit's often a necessity. This article will delve into various facets of JavaScript, from its historical evolution to its myriad applications today.
Evolution of JavaScript
The history of JavaScript is a tale of adaptation and innovation. It was initially born in 1995 as a side project by Brendan Eich while at Netscape. Originally called Mocha and later renamed to LiveScript, it ultimately settled on JavaScript, a name that would later be both a boon and a bane. The name association with Java, a more established programming language, spurred its rapid adoption, though the two languages serve vastly different purposes.
Through the years, JavaScript has undergone several evolutions. The introduction of the ECMAScript standard in 1997 provided structure and direction. Each version brought new features; for instance, the ES6 release in 2015 contributed significant enhancements like arrow functions and promises that embraced modern programming paradigms. Today, JavaScript is no longer stuck in web browsers; it powers backend servers using technologies like Node.js, and it extends into mobile app development through frameworks like React Native. This journey illustrates not just growth in capability but also how JavaScript has continuously reinvented itself in response to developer needs.
Significance in Web Development
In the fast-paced realms of the internet, a website's ability to engage users is crucial, and JavaScript is at the forefront of this engagement. Unlike static HTML and CSS, JavaScript enables dynamic content changes without necessitating full page reloads, sharply increasing interactivity. Simple actions such as form validation, multimedia handling, and animations spring to life with its innovative capabilities.
Key reasons outlining its significance include:
- Interactivity: JavaScript allows elements to react to user inputs, making sites not just places to read but environments to engage and navigate.
- Versatility: It can be run on both the client-side and server-side, allowing developers to use a single language across tech stacks.
- Ecosystem Development: JavaScript's thriving ecosystem, filled with libraries like jQuery and frameworks like Angular, facilitates rapid application development.
- Community Support: An active community constantly creates resources, tutorials, and tools, making it easier for newcomers to learn and grow.
"JavaScript is the language of the web and the backbone of modern web applications."
With this foundation laid, let's now venture deeper into the essentials that define JavaScript's structure and basics.
Understanding the Basics
Understanding the basics of JavaScript is akin to laying the foundation for a sturdy building; without it, whatever gets constructed on top may crumble at the first test. A solid grasp of basic concepts is crucial, as it paves the way for tackling more advanced topics.
JavaScript is not merely a language; it acts as a bridge between the developer and the user, creating interactive experiences that engage the audience. Knowing the essentials helps students and budding programmers not only write code but also understand the logic behind it. This understanding leads to more effective troubleshooting and innovation.
What is JavaScript?
JavaScript is a high-level, dynamic programming language, widely used in web development. It allows developers to create rich, interactive experiences on the web. From simple tasks like validating forms to complex operations such as creating web applications, JavaScript is essential for modern web functionality.
The language was first developed by Brendan Eich in 1995 while he was at Netscape. Originally named Mocha, it underwent several transformations before being dubbed JavaScript. It's vital to recognize that despite its name, it has no direct relation to Java; the naming was more about marketing than accuracy.
JavaScript is versatile; it runs on virtually all browsers, which makes it accessible for everyone. It has also evolved over the years, and now it supports object-oriented, imperative, and functional programming styles.
Key Syntax and Structure
The syntax of JavaScript is the set of rules that defines combinations of symbols that are considered to be correctly structured programs. Familiarity with the syntax enhances oneās ability to articulate commands effectively.
- Variables: Variables in JavaScript are declared using keywords such as , , or . Each serves its purpose and understanding their differences is essential for writing effective code.
- Operators: JavaScript uses various types of operators like arithmetic, comparison, and logical operators to manipulate variables and values.
- Control Flow: Control structures such as statements and loops help in directing the flow of execution based on conditions.
- Example:
Understanding these syntax rules not only aids in error-free coding but also empowers better problem-solving skills in coding endeavors.
Data Types in JavaScript
When dealing with any programming language, it's crucial to understand its data types, as they determine how data is stored and manipulated. JavaScript contains several different data types, which can be broadly categorized into primitive and non-primitive types.
- Primitive Types: These include String, Number, Boolean, Null, Undefined, and Symbol. They represent single values.
- Non-Primitive Types: This consists mainly of Objects, which can store collections of data and more complex entities.
For instance:
- String: Represents textual data, enclosed in quotes.
- Boolean: Represents a true or false value.
- Example:
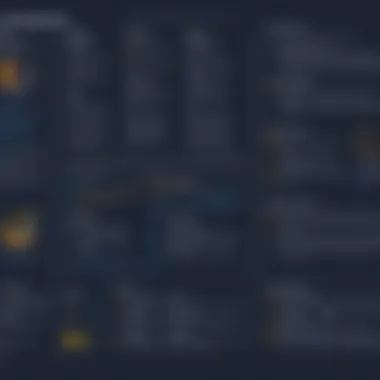
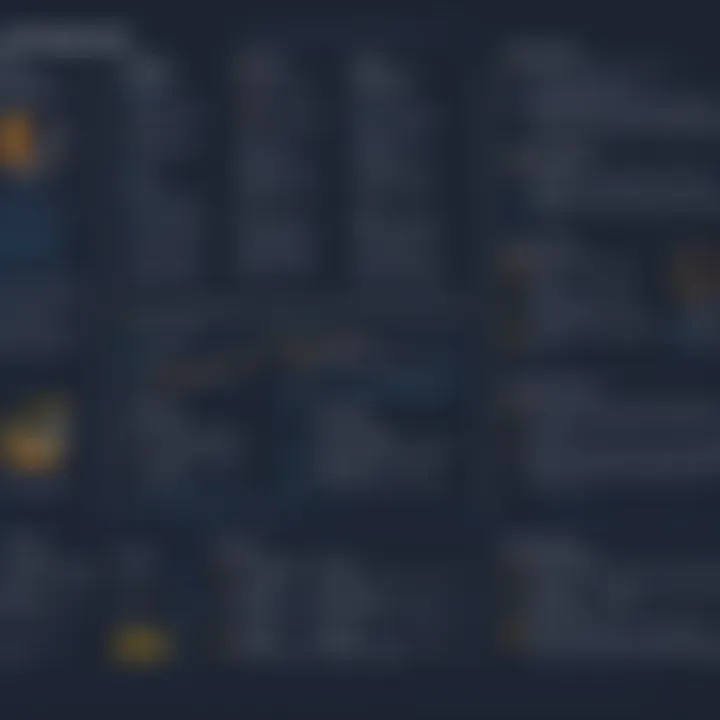
- Example:
Understanding these types is crucial because they influence how operations are performed and dictate behavior in applications. Knowing how to manipulate these data types efficiently can significantly enhance a programmerās capabilities and the overall quality of their code.
Remember, mastering the basics of JavaScript is the first step towards becoming a proficient developer. It establishes a framework from which more complex concepts can be learned.
By diving into these fundamental aspects of JavaScript, you arm yourself with the knowledge essential for any programming journey. As you delve deeper into this language, always revisit these basics to ensure a strong grasp on your learning path.
Practical JavaScript: Setting Up Your Environment
A solid foundation in programming starts with the right environment. Setting up your JavaScript environment isn't just a technical necessity; it's a strategic move that enhances your productivity and shapes your learning experience. The importance of a well-configured environment cannot be overstated, especially for those diving into JavaScript. This step not only paves the way for effective coding but also minimizes frustration while you learn the intricacies of the language. A well-organized workspace can make all the difference in maintaining focus and motivation.
Letās break down the two pivotal components of your JavaScript setup: choosing an Integrated Development Environment (IDE) or a text editor, and establishing a local development environment. Each has its nuances and benefits that cater to varying learning styles and project requirements.
Choosing an IDE or Text Editor
Choosing the right IDE or text editor is like picking the right tool for a trade; it fundamentally influences your workflow. There are myriad options available, each tailored to specific preferences and workflows. On one hand, you have full-fledged IDEs like Visual Studio Code, WebStorm, and Atom which offer rich features such as syntax highlighting, debugging capabilities, and integrated terminal support. These features can save time and provide a more guided experience for beginners.
On the other hand, simpler text editors like Sublime Text or Notepad++ can be appealing for their speed and simplicity. They allow you to quickly jot down code snippets without the overhead of additional features. The choice really comes down to personal preference, but here are some factors to consider:
- Personal Comfort: Stick with what feels intuitive. If a tool feels cumbersome, it might hinder your learning.
- Project Requirements: Some projects may demand the advanced functionalities that only an IDE can offer.
- Community Support: Opt for tools that have a robust support community. This can be invaluable for troubleshooting.
"A good programmer is not someone who knows every detail of a language but one who knows how to solve problems effectively."
Setting Up Local Development Environment
Once youāve chosen your preferred IDE or text editor, setting up your local development environment becomes the next crucial step. This environment simulates a live server on your machine, allowing you to run and test your JavaScript code without the need for an actual web server hosting. It provides a safe playground to experiment, make changes, and discover new functionalities without any risk.
Here are essential steps in setting up your local environment:
- Install Node.js: This is a JavaScript runtime that enables you to run JavaScript on your machine, outside of a web browser. It comes packaged with npm (Node Package Manager), which is great for managing libraries and dependencies.
For windows users, download the installer from https://nodejs.org/
If you're using macOS or Linux, run:
sudo apt-get install nodejs
Setting up your JavaScript environment requires thought and care, but getting it right at this stage can set the tone for your entire programming journey. With a well-structured setup, youāll have the freedom to fully explore the complexities of JavaScript and hone your skills in an engaging and effective manner.
Core Concepts of JavaScript
In any programming language, mastering the core concepts forms the foundation for success. For JavaScript, this is no different. Understanding these core concepts enables learners to build upon their knowledge effectively and tackle more complex challenges down the line. It involves grasping how JavaScript behaves and interacts within a browser, leading to smoother application development and better debugging strategies.
These core concepts include variables and scopes, control structures, and functions. Each element plays a significant role in how you write code and understand its flow. They provide the tools to manage data, control the logic in your applications, and encapsulate functionality in a way that is both efficient and maintainable.
Variables and Scopes
Variables are essentially containers for storing data values. In JavaScript, you can create variables using the keywords , , or . Each of these has its own implications regarding scope and mutability. For instance, declares a variable that is function-scoped or globally-scoped, depending on its location. On the other hand, and are block-scoped, which means they are limited to the block in which they are defined.
The idea of scope addresses where a variable is accessible within the code. This becomes particularly vital in larger applications or when working within functions. Misunderstanding scope can lead to unexpected behavior, complicating debugging processes. In fact, not knowing whether a variable is defined within the same scope can trip up a lot of novice programmers. Understanding these subtleties will go a long way in helping you manage your code effectively. Hereās a simple example:
Control Structures
Control structures in JavaScript dictate the flow of execution in your programs. They allow for decision-making capabilities that can respond differently based on differing conditions. Primary structures include conditional statements (, , ) and loops (, , ).
Using control structures effectively helps avoid redundancy in code and can lead to cleaner solutions. For example, using can streamline the decision-making process when you have multiple conditions to check. This may save you from writing many statements that would clutter your code. Hereās an illustration:
Functions in JavaScript
Functions represent one of the most powerful aspects of JavaScript. They allow you to encapsulate code into reusable blocks, making your scripts more organized. A function can take parameters, making it flexible, and return a value. You can write anonymous functions, arrow functions, or standard function declarations.
Understanding how to create and utilize functions is a monumental milestone for any programmer. It enhances code readability and maintainability. Additionally, functions enable the concept of higher-order functions, which can accept other functions as arguments or return them. This adds layers of potential for your JavaScript code.
Hereās a brief look at defining a function:
Advanced JavaScript Techniques
Advanced JavaScript techniques deepen your understanding of the language, allowing you to leverage its full potential. These concepts are crucial for anyone looking to push beyond the basics and build more sophisticated applications. By mastering these skills, you not only enhance your programming repertoire but also understand how JavaScript fits into the larger web ecosystem. Let's dive into three advanced areas: asynchronous programming, prototypes, inheritance, and working with the Document Object Model (DOM).
Understanding Asynchronous JavaScript
Asynchronous programming is a fundamental aspect of modern JavaScript, especially in web development. At its core, it allows the execution of tasks without blocking the main thread. This is crucial for maintaining a smooth user interface while handling operations like data fetching or performing calculations.
One of the primary tools for managing asynchronous operations in JavaScript is the object. A promise represents the eventual completion (or failure) of an asynchronous operation and its resulting value. By using promises, you can write cleaner and more readable code, avoiding what developers often refer to as "callback hell," where nested callback functions can lead to confusing code structures.
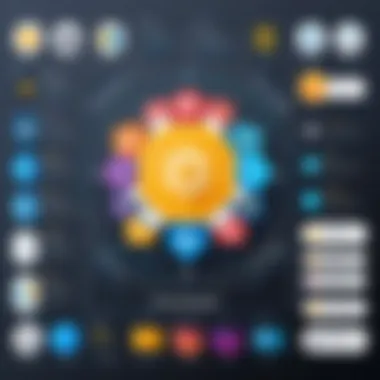
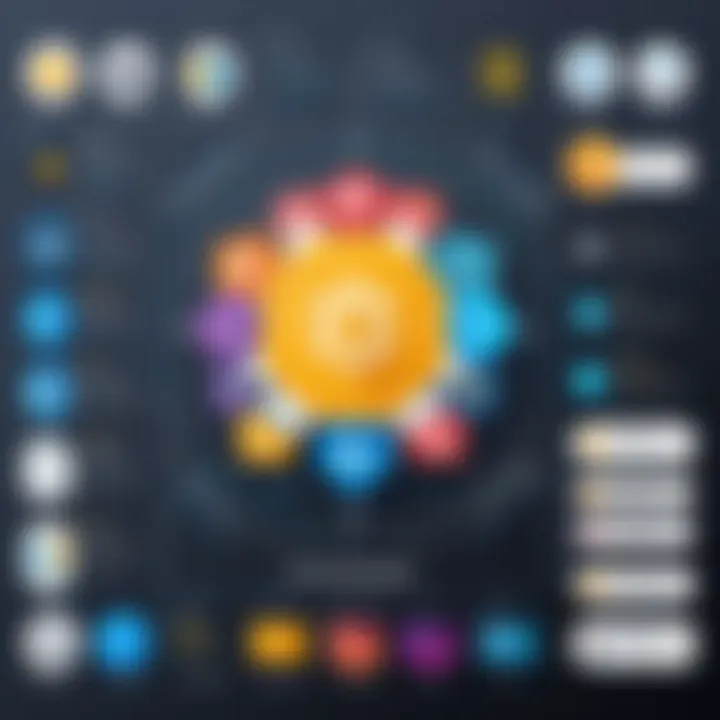
Additionally, the introduction of async/await in ES2017 further simplified working with asynchronous code. This allows you to write asynchronous code that looks and behaves like synchronous code:
Asynchronous JavaScript gives you the ability to handle multiple operations simultaneously, improving the efficiency and performance of web applications. With a solid grasp of these concepts, you'll be ready to tackle real-world programming challenges with ease.
JavaScript Prototypes and Inheritance
Prototypes and inheritance are among the core concepts that set JavaScript apart from many other programming languages. Understanding how these work allows you to utilize JavaScript's flexibility and create robust software applications.
In JavaScript, every object has a prototype. When you try to access a property of an object, JavaScript will first look for that property within the object itself. If it does not find it, the search continues up the prototype chain until it either finds the property or reaches the end of the chain.
This feature enables prototype inheritance, a powerful means of creating object-oriented code. For instance, consider the following code snippet:
In this example, inherits properties and methods from through the prototype chain. Grasping how prototypes work is indispensable for any JavaScript developer looking to level up their skill set.
Working with the Document Object Model
The Document Object Model (DOM) represents the structure of a document as a tree of objects. JavaScript has a powerful ability to interact with the DOM, allowing you to manipulate HTML and CSS dynamically. This is critical when developing responsive and interactive web applications.
Interacting with the DOM is as simple as selecting elements and calling methods on them. Hereās a quick overview of how you'd update an element:
In this example, the text content of an HTML element with the ID gets updated. Additionally, you can listen for events on DOM elements, enabling dynamic interactions:
Understanding the DOM is indispensable for web developers, enabling you to create dynamic user experiences that keep visitors engaged. Mastering these advanced JavaScript techniques not only amplifies your coding skills but also enriches your ability to build complex web applications that stand out.
Remember, advancing in JavaScript is a journey. Take your time to practice these concepts, and you'll find that the language's intricacies will become second nature.
Learning Resources and Tools
Understanding JavaScript isn't just about diving into coding; itās about having the right tools and resources at your fingertips. Mastering this language requires a structured approach and a variety of learning mediums to cater to different learning styles. Whether it's visual, auditory, or kinesthetic, each learner can benefit from a tailored approach.
A well-rounded selection of learning resources can significantly enhance the learning journey. Here are a few key aspects to consider when navigating these tools:
- Adaptability: Resources should allow for a flexible learning pace. Some may breeze through topics, while others might need more time.
- Accessibility: The easier it is to access materials, the more likely they are to be utilized effectively.
- Variety: Different types of resources keep learners engaged and provide multiple perspectives on complex topics.
Providing clear paths toward resources can help learners avoid frustration and maximize retention of concepts. Here are three crucial categories:
Online Courses and Tutorials
Online courses are fantastic for structured learning. Platforms like Udemy, Coursera, and freeCodeCamp offer courses that guide students from the basics to advanced concepts. Many courses include video lectures, quizzes, and even community support. Hereās what to look for in a course:
- Interactive Components: Look for courses that offer hands-on exercises and projects. They often help solidify theoretical knowledge by putting it into practice.
- Instructor Feedback: Being able to ask questions and receive feedback is invaluable in the realm of programming. A responsive instructor can make a big difference.
- Updated Content: JavaScript evolves continuously. Opt for courses that are up-to-date and relevant to current technology trends.
"The best way to learn JavaScript is by doing it. The more you practice, the more you understand."
Books and E-books on JavaScript
For many learners, books provide an essential resource for deeper understanding. Books can dissect complex topics at a level of detail that online formats sometimes gloss over. Here are a few notable mentions:
- Eloquent JavaScript by Marijn Haverbeke - A dive into JavaScript's mechanics with practical examples.
- You Donāt Know JS (book series) by Kyle Simpson - This series breaks down various concepts with great clarity.
- JavaScript: The Good Parts by Douglas Crockford - A classic that isolates the most useful features of JavaScript.
Considerations when choosing a book include:
- Clear Explanations: The text should provide clear, comprehensible explanations.
- Practical Exercises: Books that contain exercises can encourage application of concepts.
- Reputable Authors: Research the authors and their backgrounds to ensure they have authority in the field.
JavaScript Libraries and Frameworks
JavaScript stands out for its rich ecosystem of libraries and frameworks that serve to streamline development processes. Understanding common libraries like React, Angular, and jQuery can give learners an edge in building robust applications. Here are important points regarding libraries and frameworks:
- Purpose: Each library has a specific use case. For instance, React is fantastic for building user interfaces, while Node.js is great for server-side development.
- Community Support: A large community behind a framework often means better documentation and quicker problem-solving.
- Learning Curve: Some libraries have steeper learning curves than others. It's wise to choose one that aligns with your existing knowledge and project goals.
Incorporating these resources into your learning path can equip you with a solid understanding of JavaScript. Remember, it's not just about learning the syntax or functions; it's about applying knowledge consistently to solve real-world problems and creating unique applications.
Building Projects to Enhance Skills
Gaining proficiency in JavaScript isn't just about reading books or taking online courses. It's in the act of building real projects where the magic happens. Engaging in practical application solidifies knowledge and offers insights that theory simply can't provide. When you roll up your sleeves and get coding, you transform abstract concepts into tangible accomplishments.
Why is project-building vital? Well, for starters, it provides a unique opportunity to apply what you've learned, reinforcing your skills while allowing you to understand the intricacies of JavaScript more deeply. As you encounter challenges, you'll also learn to problem-solve, a critical skill for any programmer.
Furthermore, having projects to showcase is a massive boost to your portfolio. Whether you're seeking a job or just trying to find clients, demonstrating tangible outcomes where you've implemented JavaScript can set you apart from the crowd. Plus, projects often lead to further learning; thereās always something new to discover, be it a method you've yet to use or a library that could speed up development.
In addition, the feedback you get from building something in the real worldābe it from peers or usersācan direct your learning towards more practical areas. Feedback is valuable; it allows growth, ensuring you arenāt just coding in a vacuum. Finally, managing projects instills a sense of discipline and organizationātraits every developer needs to thrive.
Choosing the Right Project
When it comes to selecting a project to work on, the choices can be as vast as the ocean. However, not all projects yield the same educational benefits. Hereās how to choose wisely:
- Align with Your Interests: Pick something you're passionate about. If you care about the project, motivation will come easy.
- Consider Skill Level: Don't set yourself up for failure. Ensure the project isnāt overwhelmingly advanced. Start with simple ideas, like building a to-do list app or a weather forecast app.
- Scope: Keep it manageable. Itās better to finish a small project than to get bogged down in an overly ambitious venture that never sees the light of day.
- Functionality: Aim for a project that includes various JavaScript features: working with DOM events, API calls, etc. This diversification will ensure that you practice as many skills as possible.
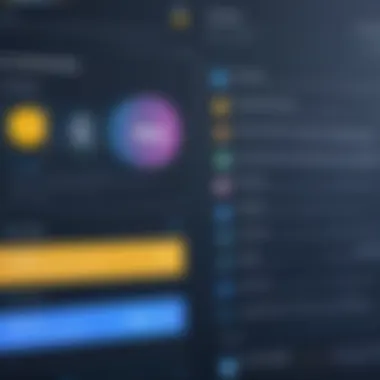
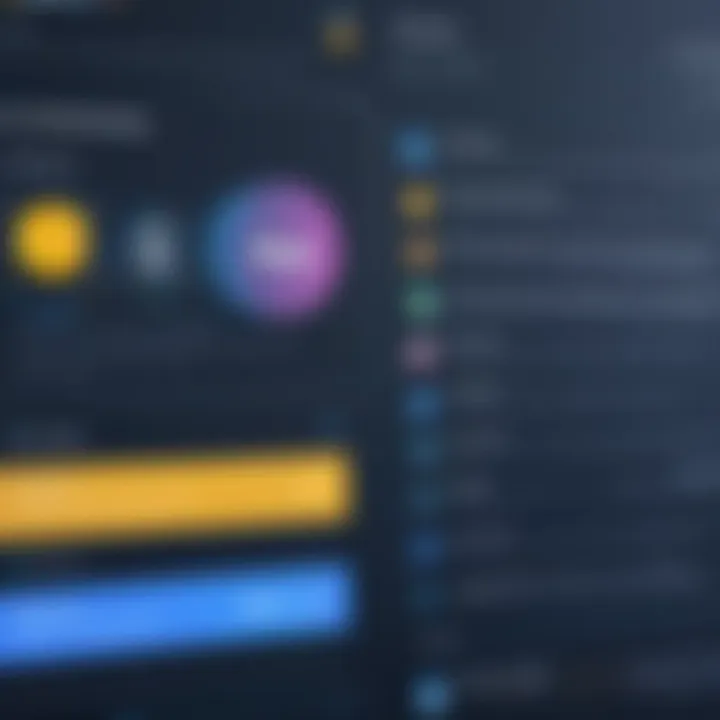
Choosing the right project can transform your learning journey. A thoughtful selection leads to successful completion and ownership of the knowledge youāve gainedāwhich ultimately yields confidence in your capabilities.
Breaking Down Projects into Manageable Tasks
Once youāve pinned down a suitable project, itās time to dive in. However, before plunging headfirst, itās crucial to break down the project into bite-sized chunks. Working in smaller segments makes the entire process less daunting. Hereās how you can do it:
- Outline Main Features: Start by listing all the functionalities you want your project to have, such as user registration or data storage.
- Establish Timelines: Set a timeframe for each task. This keeps procrastination at bay and provides a sense of urgency.
- Prioritize Tasks: Identify which tasks are essential for the project's completion and tackle those first. Perhaps itās setting up the layout before jumping into JavaScript functionality.
- Use Version Control: With tools like Git, you can track changes and manage different versions of your project. This acts as a safety net if you need to revert to a previous state.
- Test as You Go: Make sure testing isnāt left until last. Consistent testing will help catch errors early and continuously improve the quality of your work.
Remember: The journey of a thousand lines of code begins with a single task.
Breaking down your project ensures that you stay focused and avoid the common pitfalls that often lead to frustration and abandonment of projects. Taking one step at a time allows for reflection, learning, and ultimately a more rewarding experience as you build your skill set in JavaScript.
Common Pitfalls in Learning JavaScript
When diving into JavaScript, learners often find themselves ensnared in a web of challenges that can derail their progress. Understanding these common pitfalls is crucial for navigating the learning process effectively. By being aware of these potential stumbling blocks, learners can not only save time but also gain a clearer path through the complexities of JavaScript. The importance of this section lies in empowering students to identify and overcome difficulties that could otherwise become major roadblocks.
Avoiding Overwhelming Resources
In the vast ocean of online tutorials, e-books, and forums, itās easy to feel like a deer caught in headlights. With so many resources available, learners may try to absorb everything at once, leading to confusion and frustration. The key is to curate quality resources that align with oneās current skill level.
- Focus on foundational materials: Start with the basics. It's often tempting to leap into advanced concepts, but grasping core principles is essential for cohesive learning.
- Set a learning schedule: Establish a routine. Carve out specific time slots for study, to digest small chunks of information rather than overwhelming oneself with massive amounts of content.
- Engage selectively with communities: Online platforms like Reddit can provide useful insights. However, it's vital to engage with forums that suit oneās current level, ensuring that the materials discussed are comprehensible and relevant.
Remember: The journey to mastering JavaScript is not a sprint, but a marathon. Taking one step at a time makes the process more manageable and less daunting.
Understanding Errors and Debugging
Errors are an inevitable part of programming, especially while learning a new language like JavaScript. Instead of viewing errors as failures, they should be seen as lessons in the making. Recognizing common types of errors and knowing how to debug them can make a significant difference.
- Syntax Errors: These are often the most frustrating and are typically due to typos or incorrect punctuation. A misplaced semicolon or an unclosed bracket can throw a wrench in your code.
- Reference Errors: These occur when the code references an undefined variable, which can lead to confusion when a script suddenly stops running.
- Debugging Techniques:
- Use the Console: The browser console is a powerful tool. Start developing the habit of checking the console for error messages, as it provides valuable insight into what might be going wrong.
- Break Down the Code: If a particular part of your code isnāt working, isolate it. Commenting out other parts can help identify where the issue lies.
"Mistakes are proof that you are trying."
Understanding how to debug effectively enables you to learn from your errors, turning each misstep into a stepping stone towards mastery.
In summary, navigating the landscape of JavaScript is fraught with challenges, but by staying mindful of these common pitfalls, learners can carve a clearer, more empowering path towards proficiency. The journey may have its ups and downs, yet with patience and persistence, one can rise above the obstacles.
Link to learn more about JavaScript on Britannica.
Exploring the above strategies will equip learners to tackle their education with confidence and clarity.
Community and Networking
In the world of programming, the phrase "it takes a village" can ring true, especially when mastering a language as dynamic as JavaScript. A supportive community not only fortifies your learning but also enriches your experience through shared knowledge, diverse perspectives, and collaborative problem-solving. Understanding the importance of community and networking will help you develop not just as a developer, but also as an individual who is connected to a broader ecosystem.
Participating in Online Forums
Participating in online forums is like throwing your hat into a vast ring filled with like-minded individuals eager to share, learn, and grow. Websites such as Reddit or specialized platforms like Stack Overflow provide immense wealth of knowledge on a variety of topics related to JavaScript. Joining these discussions allows you to gain insights into practical problems that others face, providing clarity on complex ideas that often feel daunting.
- You can ask questions and receive answers from experienced developers.
- Often, just reading through existing threads can illuminate best practices and common pitfalls, which may save you countless hours of frustration.
- Sharing your own experiences can also cement your knowledge, reinforcing what youāve learned while contributing back to the community.
To dive deeper, you might find it beneficial to subscribe to specific JavaScript-related subreddits or forums. This way, you can keep up to date on the latest trends and discussions, becoming part of ongoing dialogues that shape the landscape of JavaScript development.
Joining Coding Meetups and Workshops
Engaging in coding meetups and workshops provides a face-to-face interaction that online environments sometimes lack. These gatherings present an opportunity to hone your skills in a practical, high-energy setting. Connecting with other learners or industry veterans can lead to the kind of mentorship that pays dividends over time.
Key Benefits:
- Networking: Meeting others who share your interests can lead to job opportunities or some collaborative projects.
- Hands-On Learning: Workshops typically require you to solve problems on the spot, which can be invaluable for reinforcing your knowledge.
- Feedback: Instant feedback from peers and leaders can accelerate your learning by helping you identify areas for improvement.
In the tech community, relationships often lead to doors opening in ways that formal applications might not. Hence, participate in coding meetups in your area or even virtual ones. Platforms like Facebook or Meetup.com can help you find local gatherings where coding enthusiasts converge.
"The best way to predict your future is to create it." ā Abraham Lincoln
This sentiment resonates well within the tech community, where you are often narrating your own journey through the connections you make and the skills you build. As you navigate the world of JavaScript, remember that the friends and allies you gather can shape the trajectory of your career.
The Future of JavaScript
As the tech landscape continues to evolve, the significance of JavaScript remains undiminished. Its ubiquitous presence in web development sets the stage for a thrilling transformation in the coming years. Understanding the future of JavaScript doesn't just equip learners with knowledge; it prepares them for practical applications and emerging opportunities that are bound to arise. As JavaScript becomes more integral in various industries, grasping its trajectory allows developers to adapt and excel.
Emerging Trends in JavaScript Development
JavaScript has been consistently evolving, adopting features that enhance its capabilities. Several noteworthy trends are shaping its development landscape:
- Framework Evolution: Frameworks like React, Angular, and Vue.js are constantly being refined, leading to more efficient development processes. The future may see improved integration between these frameworks and JavaScript, streamlining the way developers build applications.
- TypeScript Adoption: More developers are picking up TypeScript, which offers a type-safe subset of JavaScript. This shift allows for better error detection and improved code maintainability. As projects grow in size and complexity, the advantages of TypeScript in large-scale applications will likely increase.
- Integration with AI and Machine Learning: JavaScript's role in AI and machine learning is potentially expanding. Tools and libraries are being developed to bring machine learning capabilities directly into JavaScript, making it accessible to more developers.
"In the world of programming, keeping an eye on trends can be the difference between leading the pack and falling behind."
- Serverless Computing: The rise of serverless architectures offers JavaScript a new playground. Developers can write JavaScript functions that run in response to events, allowing for highly scalable applications without worrying about server management.
These trends not only highlight how JavaScript is adapting but also how it is being shaped by its community of developers who continue to innovate.
The Role of JavaScript in Industry
JavaScript's impact transcends traditional web development; its role in various sectors is crucial:
- Web Development: JavaScript remains the backbone of interactive web applications. With the emergence of Progressive Web Apps (PWAs), it's becoming even more vital to create engaging user experiences that rival native apps.
- Mobile Development: With frameworks like React Native, developers can utilize their JavaScript knowledge to build mobile applications efficiently. This trend indicates a future where the line between web and mobile development continues to blur.
- Enterprise Solutions: Companies are integrating JavaScript technologies into backend systems, thanks to Node.js. This integration allows for full-stack development, where a singular language powers both client and server sides, simplifying the development process.
- Education Technology: Online learning platforms are increasingly teaching JavaScript, providing a clearer pathway for beginners. As more educational resources come online, the language will likely see broader adoption in various fields.
By embracing and understanding these shifts, programmers increase their potential to leverage JavaScript in versatile environments, solidifying their position in the workforce.
As we look ahead, it's clear that JavaScript's future is bright. The combination of emerging trends and its expansive role in multiple industries make it an essential skill for developers. Keeping abreast of these developments is no longer optional but a necessity for anyone serious about a career in programming.