Mastering Hadoop Code: Key Insights and Techniques
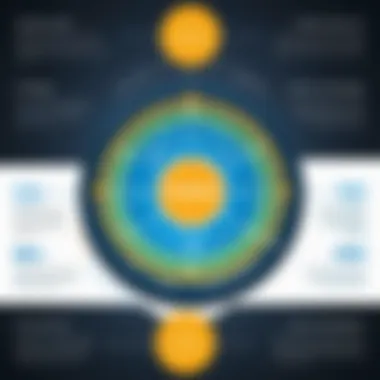
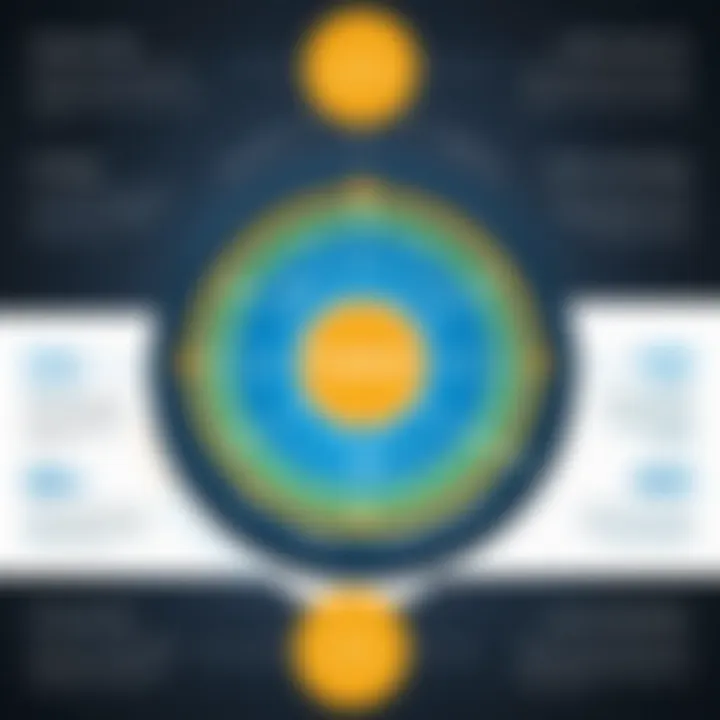
Intro
In the realm of big data, Hadoop has emerged as a titanic player, revolutionizing how we process and manage vast amounts of information. This guide aims to shed light on Hadoop code, especially for those just entering the field or those who have some experience but want to sharpen their skills.
Understanding Hadoopâs code structure and syntax is essential for making the most out of its robust architecture. As we navigate the intricate labyrinth of components and functionalities, keep in mind that every layer adds depth to how we interact with data. This journey will not just skim the surfaceâit's about diving deep into the nuts and bolts of Hadoop, including tools and practical coding techniques that can be pivotal in real-world applications.
By the end of this exploration, you will find yourself with substantial insights into Hadoop's capabilities, ready to tackle big data projects with confidence.
Prelims to Programming Language
Hadoop primarily leverages Java, one of the most enduring programming languages out there. Itâs important to understand both the language itself and its implication in Hadoopâs context.
History and Background
Java was conceived in the early 1990s, designed to be platform-independent and adaptable for various applications. As the years rolled by, it became the backbone for enterprise solutions, including those needed for extensive data processing. Hadoop, crafted by Doug Cutting and Mike Cafarella, adopted Java for its versatility and robust performance in handling complex computations and data sets.
Features and Uses
Javaâs strengths come from its object-oriented nature and a rich ecosystem of libraries. Some key features that make Java suitable for Hadoop include:
- Platform Independence: Write once, run anywhereâa very appealing feature for big data applications.
- Object-Oriented Principles: Facilitates modular design, allowing programmers to build reusable components.
- Rich API: The extensive set of built-in libraries simplifies many programming tasks.
Given its properties, Java serves as a crucial tool within the Hadoop ecosystem, impacting areas like data storage, processing, and integration with various applications.
Popularity and Scope
Java's immense popularity is due to its longevity and adaptability. In many organizations, hiring Java experts is common, leading to vibrant communities and abundant resources for learning. When it comes to Hadoop, the language plays a pivotal role, driving job opportunities and creating technical pathways for aspiring programmers. The scope stretches beyond Hadoop, reaching into AI, web development, and beyond, making it a versatile choice for anyone in the tech field.
Basic Syntax and Concepts
Before delving into how Hadoop works with Java, a solid foundation in the basic syntax and concepts of the language is essential.
Variables and Data Types
In Java, every piece of data has a type, and declaring these variables is straightforward. Examples include:
- int for integers
- String for strings of text
- boolean for true/false values
Declaring a variable is done like this:
Operators and Expressions
Java offers a variety of operators for calculations and comparisons. These include arithmetical (+, -, *, /) and relational (==, !=, >, ). Understanding how these work is key to manipulating data in Hadoop effectively.
Control Structures
Control structures are the backbone of logic in coding. In Java, you can use:
- if-else statements for conditional logic
- for loops for iteration
- switch cases for multiple conditions
Example of an if-else statement:
Advanced Topics
As you delved into the basics, itâs crucial to explore more advanced topics to grasp Hadoop fully.
Functions and Methods
Functions, or methods as they're called in Java, allow for code reuse, making programs easier to manage. For example:
Object-Oriented Programming
Hadoopâs design thrives on object-oriented programming. Concepts such as encapsulation, inheritance, and polymorphism help in building scalable solutions. It allows for complex data processing scenarios especially when developing custom data types.
Exception Handling
In the world of data processing, things donât always go as planned. Javaâs exception handling helps manage runtime errors with ease, ensuring your Hadoop applications run smoothly. Example:
Hands-On Examples
Practical coding examples can solidify understanding and instill confidence.
Simple Programs
Start by writing a basic program that prints "Hello, Hadoop!" on screen:
Intermediate Projects
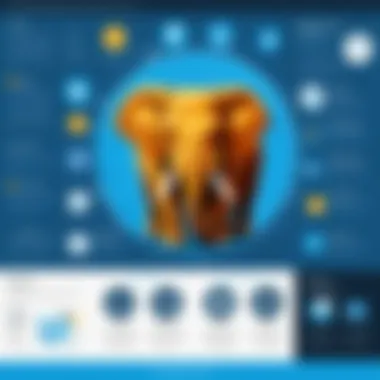
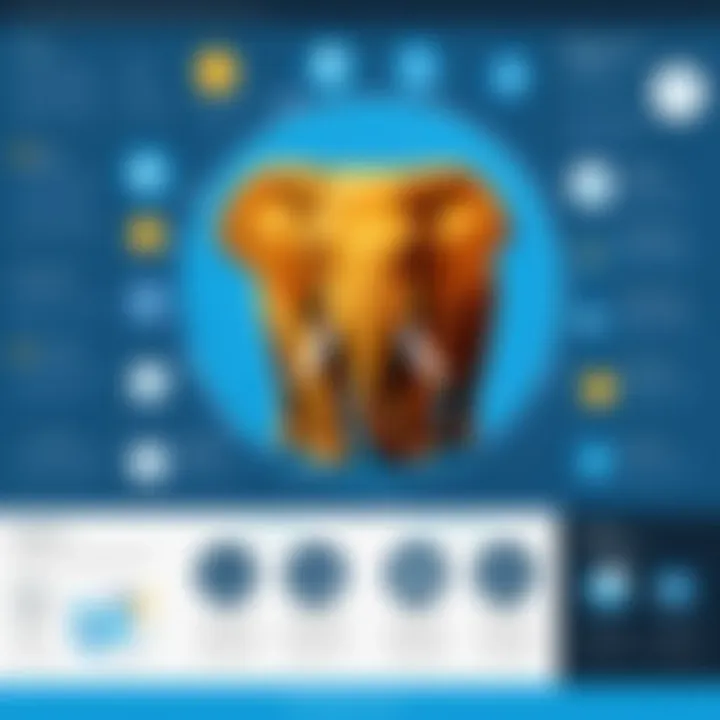
Consider a project that reads a text file, processes its content and counts the number of words. Such practical projects can familiarize you with file handling and data processing techniques in Hadoop.
Code Snippets
Sharing snippets within community forums like reddit.com can help you troubleshoot and learn from peers.
Resources and Further Learning
Learning is a never-ending journey. Here are some valuable resources to expand your knowledge.
Recommended Books and Tutorials
- Hadoop: The Definitive Guide by Tom White
- Java: The Complete Reference by Herbert Schildt
Online Courses and Platforms
Websites like Coursera and Udacity offer courses that delve into Hadoop and Java programming.
Community Forums and Groups
Engaging in forums such as reddit.com or checking out groups on facebook.com provide opportunities to learn and collaborate with others.
Ultimately, understanding Hadoop code is not merely an academic exercise; it's a key to unlocking the potential of big data in various domains. Armed with the right knowledge and skills, anyone can harness the power of Hadoop to address complex data challenges.
"Programming isn't about what you know; it's about what you can figure out."
Prelims to Hadoop
Understanding Hadoop lies at the heart of contemporary big data processing. Itâs like being handed a map of a vast, intricate city; without it, you'd be wandering aimlessly among towering data structures, struggling to make sense of your surroundings. This section serves as the gateway to exploring not only what Hadoop is but why itâs become a vital tool in todayâs data-driven world. It shapes the very landscape of big data applications, making it imperative for both budding programmers and seasoned data enthusiasts to grasp, at least, the foundational aspects.
What is Hadoop?
Hadoop is an open-source framework that enables the distributed processing of large data sets across clusters of computers using simple programming models. Think of it as a giant toolbox that helps you deal with mountains of data efficiently.
Hadoop consists of several components:
- Hadoop Distributed File System (HDFS): This is the storage system that allows data to be divided into blocks and spread across multiple machines.
- YARN (Yet Another Resource Negotiator): This manages and schedules resources across the cluster, ensuring that everything runs smoothly.
- MapReduce: This is the programming model for processing data. It divides tasks into smaller sub-tasks, processing them in parallel.
The beauty of Hadoop is its ability to scale up from a single server to thousands of machines. It's built to handle massive amounts of data, whether structured or unstructured. Organizations like Facebook, Yahoo, and Netflix leverage Hadoop to process petabytes of data daily.
History and Evolution
Hadoop's journey began in 2005 through the work of Doug Cutting and Mike Cafarella, evolving from its roots in the Google File System and MapReduce papers. Early adopters found it a breath of fresh air, especially in an era where traditional databases struggled to manage escalating data sizes efficiently.
The project became really blossomed after being contributed to the Apache Software Foundation in 2006. From then on, it underwent continuous enhancements and contributed to the flourishing of the Hadoop ecosystem, expanding into tools like Hive, Pig, and HBase.
Over the years, several versions were released, each improving upon the last, leading to a robust framework that underpins countless big data applications today. It stands as an emblem of technological progress in data management.
Importance in Big Data
In the world where data flows like an endless river, Hadoop is the dam allowing us to harness that flow effectively. Its ability to process vast amounts of data at high speeds makes it an invaluable asset in numerous industries.
Organizations leverage Hadoop for various compelling reasons:
- Cost Efficiency: Unlike traditional data warehousing solutions, Hadoop runs on commodity hardware, making it funding-friendly.
- Scalability: As data grows, so can the Hadoop environment; you can start with a single server and scale to thousands of machines seamlessly.
- Flexibility: It offers the ability to process all types of data, from structured to semi-structured to unstructured.
In an era where data is often deemed the new oil, understanding Hadoop is akin to learning how to refine that oil into something usable.
"Without data, you're just another person with an opinion." â W. Edwards Deming
Grasping the fundamentals of Hadoop puts you on the right path to navigating the complexities of data manipulation and analysis. As we proceed, keep your sights set on the specifics, nuances, and applications of Hadoop that shape today's big data landscape.
Hadoop Architecture
Hadoop architecture is a cornerstone of its ability to process huge amounts of data effectively. It provides the foundational framework that supports its key functions like storage and processing. Understanding this architecture is vital for anyone looking to harness the power of Hadoop for big data projects. The architecture not only outlines how components interact but also highlights the overall efficiency and scalability it offers. A well-structured architecture means better resource utilization and fault tolerance, which are crucial for managing vast datasets.
Core Components of Hadoop
Hadoopâs architecture consists of several core components that work together to handle data processing and storage. The primary components include the Hadoop Distributed File System (HDFS), yet another crucial element is the Yet Another Resource Negotiator (YARN), and MapReduce. The following highlights these components:
- HDFS: This is the storage layer responsible for saving files across a distributed network. It splits large files into smaller blocks to ensure they are easily managed and accessible across various nodes.
- YARN: It acts as the resource manager, ensuring all data processing jobs have the resources they need and operates with other computational frameworks.
- MapReduce: This is where the magic happens. It's the processing engine that takes data, transforms it, and analyzes it efficiently through a map and reduce process. Each component plays a critical role in Hadoopâs overall functionality.
With these components working in harmony, Hadoop offers robustness and flexibility that traditional systems struggle to match. Each one provides unique capabilities, contributing to the strength of the ecosystem as a whole.
Understanding HDFS and YARN
Digging deeper, HDFS and YARN are essential to grasping Hadoop's architectural backbone. HDFS is not just a typical file system; it is designed specifically for the distributed environment that Hadoop operates in. Its design emphasizes fault tolerance by replicating data blocks across various nodes, ensuring that even if some nodes fail, data remains intact and accessible. Therefore, when data is written to HDFS, it is automatically split into blocks, typically 128 MB each, and distributed across the cluster with several copies for reliability.
On the flip side, YARN manages resources effectively by taking in jobs and deciding where they will run within the cluster based on available resources. This allocation of resources is dynamic, adapting as necessary to changing workloads. In the end, both HDFS and YARN contribute significantly to making data processing in Hadoop remarkably effective. A sound grasp of both will offer insights into how data flows and is manipulated within Hadoop.
Role of MapReduce
MapReduce is often regarded as the brain behind Hadoop's processing power. It operates as a programming model that simplifies the way large datasets are processed in parallel across many machines. The process consists of two fundamental steps: the Map function, which filters and sorts data, and the Reduce function, which computes the final results based on the mapped data.
This two-step process allows for scalable data processing, ensuring jobs can be executed in distributed fashion. For example, in handling a massive data set of user activity logs, the map function would aggregate the logs by user ID, while the reduce function could summarize these logs to present total activity counts. This abstraction not only makes it easier for programmers to write scalable applications but also optimizes resource usage across Hadoop clusters.
In essence, understanding the role of MapReduce empowers developers to work efficiently with Hadoopâs capabilities, leading to faster and more impactful data analytics.
Hadoop Code Basics
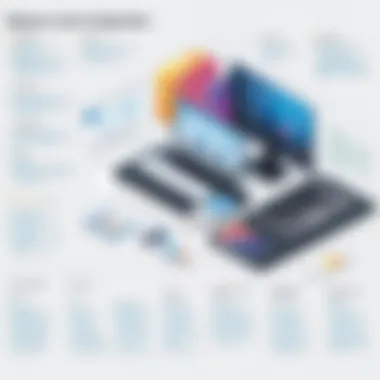
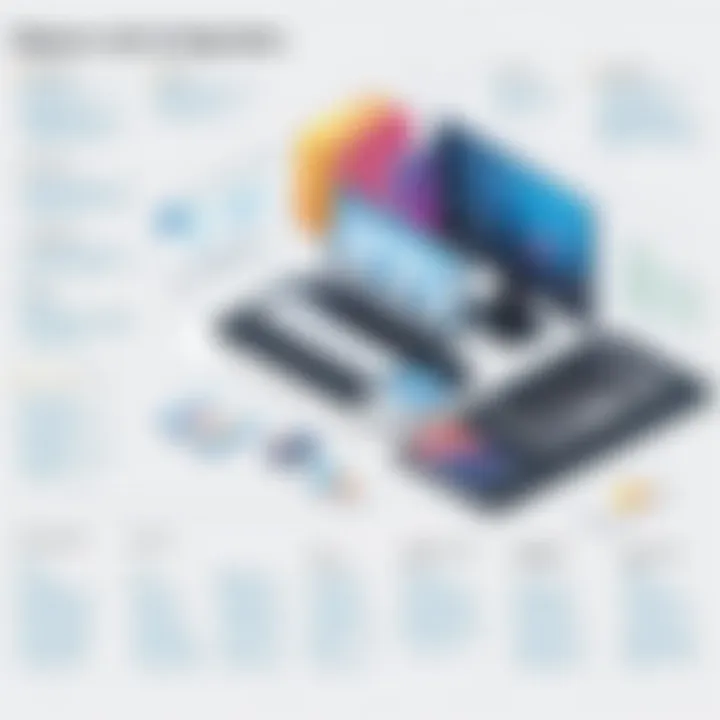
Understanding the intricacies of Hadoop code is essential for developers who wish to harness the power of big data effectively. The Hadoop code basics section lays the groundwork for engaging with Hadoop's powerful ecosystem. This section emphasizes the initial steps required to jump into writing and executing Hadoop code, catering especially to students and budding programmers keen on diving into programming with Hadoop.
Hereâs why mastering these basics holds importance:
- Foundation of Knowledge: Before diving into advanced programming techniques, having a grasp of the basic setup and coding practices is crucial. It allows for smoother transitions into more complex topics.
- Enhanced Practical Skills: Setting up your environment and understanding code structure directly impacts your ability to create efficient data processing applications.
- Error Reduction: Familiarity with common configuration files helps anticipate potential issues, leading to quicker debugging and more robust code.
Setting Up the Hadoop Environment
To start programming with Hadoop, the first step invariably involves setting up the system where you'll run your code. The following are important aspects to consider when establishing your Hadoop environment:
- Choosing the Right OS: Hadoop is primarily built to run on Linux, making it a necessity to have a compatible operating system. However, you can also use Windows with specific configurations.
- Downloading Hadoop: You can get the distribution from Apache's official site. Select a stable version to avoid compatibility issues when learning.
- Installation Process: Follow the clear instructions on the website to install Hadoop. This often includes adding Java as Hadoop runs on the Java Virtual Machine (JVM).
After installation, ensure to configure environment variables like and , which are essential for the correct functioning of Hadoop.
Writing Your First Hadoop Program
Writing your first program may seem daunting, but once you've set up your environment, you can start creating simple MapReduce applications. Hereâs a quick guide:
- Create Input Files: Start by creating text files that hold the data you want to process. Simple CSV or plain text files work perfectly at this stage.
- Write MapReduce Code: This involves writing Java classes. Your primary class should extend and base classes. Hereâs a simplified example:
- Compile and Run: Use the Hadoop command-line interface to package your code and submit it for execution. The pattern is generally as follows: .
Understanding Configuration Files
Configuration files are key players in Hadoop's operation, dictating how the cluster behaves. Here are a few essentials:
- core-site.xml: This file configures core settings like the file system and the underlying Hadoop framework.
- hdfs-site.xml: dictates configurations related to HDFS properties, such as replication factors and data storage.
- mapred-site.xml: governs MapReduce settings, including job history and resource management.
- yarn-site.xml: essential for applications running under YARN, determining how these applications interact with the resource manager.
Understanding these files is pivotal for customizing your setup, ensuring your applications run smoothly according to your needs. Familiarizing yourself with their structure and properties facilitates the creation of well-tuned applications.
Highlight: Understanding the Hadoop environment, writing efficient programs, and mastering configuration files are foundational for effective Hadoop programming. Their significance cannot be overstatedâmemorable experiences await those who take the time to get these elements right.
Programming with Hadoop
Programming with Hadoop is a crucial element in leveraging the full power of this ecosystem. It's not just about writing code; itâs about understanding how your code interacts with vast amounts of data. Hadoop was designed to process large datasets effectively, and mastering it transforms you into a valuable asset in any data-driven field. With Hadoop, you can explore, analyze, and extract meaningful insights from gigabytes to petabytes of data. Letâs dive into some key aspects of programming with Hadoop.
MapReduce Framework
The MapReduce framework is at the heart of Hadoop programming. It splits tasks into smaller chunks, allowing for parallel processing of data. This is done in two main steps: Map and Reduce. In the Map step, unaffiliated key-value pairs are formed as data is processed. Then, during the Reduce phase, those key-value pairs are summed or aggregated based on their key. Not only does this maximize efficiency, but it also makes coding easier.
Programming in MapReduce means working primarily through two important components: the Mapper and the Reducer. The Mapper prepares data to be distributed to the Reducers, laying down the groundwork necessary for analytics.
An example of a Mapper might look like this:
This code snippet shows a typical Mapper that counts words in input text. It introduces essential concepts, like using counters and writing context, both vital in Hadoop programming.
Example of a MapReduce Job
Now, letâs look at a straightforward example of a MapReduce job that counts words in a dataset. Assume you have a collection of documents, and you want to know how often each word appears. The job is simple but illuminates the core process. Start by defining the job in your Java code:
In this snippet, you can see how the Mapper and Reducer classes are integrated. This example shows how easily you can construct a MapReduce job, providing a straightforward pathway for data analysis.
Optimizing MapReduce Code
When working with large datasets, efficiency is paramount. Optimizing MapReduce jobs allows you to save on both time and resources. Here are some practical tips to enhance your MapReduce code:
- Combining and Grouping Data: - Use combiners where appropriate to minimize network traffic.
- Tuning Configuration Parameters: Modifying settings like the number of mappers can drastically influence performance.
- Data Locality: Ensure that your computation is as close as possible to the data. This minimizes transfer time over the network.
- Using Efficient Data Formats: Data formats like Avro, Parquet, and ORC can improve the speed of read/write operations due to their optimized structure.
Consider leveraging libraries like Apache Pig or Apache Hive for easier handling of complex data transformations in Hadoop.
Advanced Hadoop Coding Techniques
In the realm of big data, Hadoop stands as a pivotal framework that many developers choose to work with. However, venturing beyond the basics into advanced coding techniques can set certain developers apart from the rest. These techniques not only bolster performance but also enhance the efficiency of data processing tasks. In this section, weâll peel back the layers of advanced Hadoop coding techniques and shine a light on their significance for developers.
Using Hadoop Streaming
Hadoop Streaming serves as a gateway for non-Java programmers, allowing them to utilize any language of choice for writing their MapReduce jobs. This means a Python enthusiast can craft scripts in Python while still exploiting Hadoopâs powerful infrastructure.
By leveraging Hadoop Streaming, you can:
- Write scripts in various languages: Whether it's Python, Ruby, or Perl, this flexibility can drastically reduce the learning curve, making Hadoop more accessible.
- Rapidly prototype: Since you can work in your preferred language, experimenting with algorithms becomes quicker and more intuitive.
- Integrate existing code: Thereâs no need to rewrite functions; existing code can be readily adapted to Hadoop jobs.
This snippet showcases a simple setup for a MapReduce job using Python scripts for both mapper and reducer components. Thus, Hadoop Streaming can greatly enhance the development process, benefitting a broader pool of developers.
Integrating Hadoop with Other Tools
The true power of Hadoop unfolds when it interacts seamlessly with other tools. Integration allows developers to harness various technologies for added functionality. For instance:
- Apache Spark: Spark can complement Hadoopâs batch processing with real-time stream processing capabilities, creating a more versatile environment for data analytics.
- Apache Hive: Hive introduces an SQL-like query interface to Hadoop, catering to users familiar with relational databases, effectively demystifying data manipulation in Hadoop.
- Apache Pig: Pigâs scripting language offers another layer of simplification and abstraction over Java, making it easier for developers to handle complex data flows.
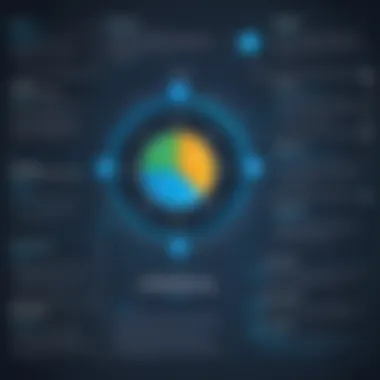
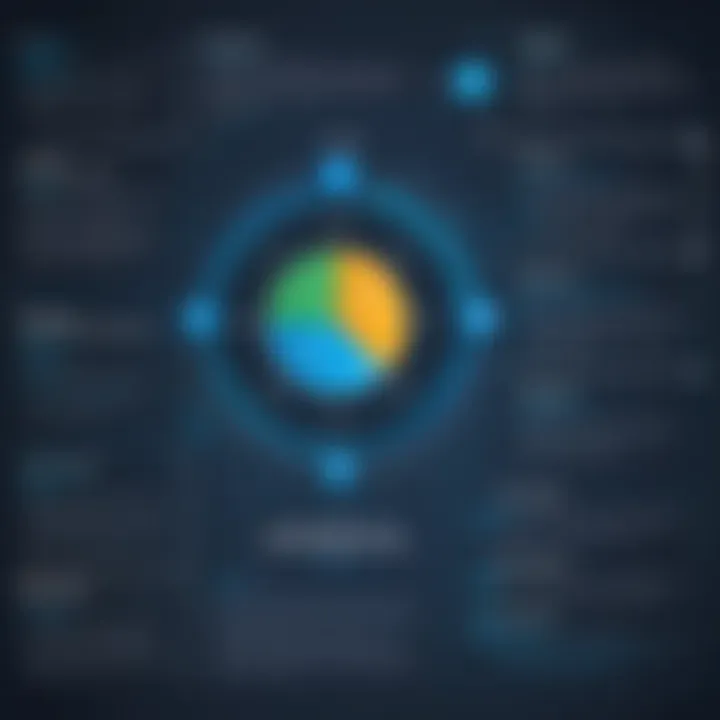
Moreover, these integrations enable a fluid workflow that aligns with business needs. This adaptability is crucial because as new requirements emerge, the ability to smoothly integrate additional capabilities without starting from scratch makes life easier for developers.
Debugging Hadoop Jobs
Debugging in a distributed environment like Hadoop can be quite the challenge. Errors that arise may not just be a result of coding mistakes but can stem from the complexities involved in a distributed platform. Here are some strategies that can guide you:
- Utilize logs: Each Hadoop job generates logs which can be accessed in the Hadoop interface. Understanding these logs can provide insights into failures and performance issues.
- Job History Server: This tool allows you to review past jobs which can illuminate why something didnât perform as expected. You can check the success or failure state of jobs and analyze the respective logs for hints.
- Test in smaller clusters: Before deploying on larger datasets, running jobs on small test clusters can help surface issues early without excessive resource costs.
"Proper debugging can illuminate hidden patterns within your code, sometimes revealing bugs that wonât appear until interacting with specific datasets."
Through these techniques, the often arduous process of debugging can be streamlined. Sticking to a methodical approach towards identifying and resolving issues not only saves time but also bolsters the reliability of the applications you develop.
Common Challenges in Hadoop Development
Hadoop has significantly transformed how we process and analyze vast amounts of data. However, like any powerful tool, it comes with its own set of challenges that can trip up even the most seasoned developers. In this section, we will examine these common challenges and how understanding them can be beneficial not only for troubleshooting but also for optimizing your use of Hadoop.
Handling Large Datasets
When it comes to big data, size matters. Hadoop is designed to handle large datasets, but the sheer volume can pose management obstacles. For instance, if datasets are poorly organized, it can lead to inefficiencies that slow down processing times. Developers often find themselves wrestling with data distribution and replication strategies.
One effective approach to mitigate these issues is to leverage the Hadoop Distributed File System (HDFS) to ensure load balancing across nodes. Proper sharding and data locality can drastically improve performance. Also, understanding the ingress and egress patterns is crucial for efficient data handling in Hadoop.
"The best way to predict the future is to create it." â Peter Drucker
Performance Tuning
Performance tuning in Hadoop is an ongoing battle, reminiscent of tuning a fine instrument. A poorly tuned Hadoop job can lead to skyrocketing costs and extended processing times, which isnât ideal in a competitive environment. Various factors affect performance, such as resource allocation and job configurations.
For instance, adjusting parameters like or can have immediate impacts on how jobs perform. Additionally, using combiners to minimize data transfer or optimizing data formats for storage can lead to significant enhancements in speed and resource efficiency. Regularly monitoring your clusters' performance metrics also helps identify bottlenecks and areas needing adjustment.
Error Management and Troubleshooting
Errors in Hadoop jobs occur with alarming frequency, often at the most inconvenient times. Having a structured approach to error management is not just wise but essential for any developer. Understanding common errors, whether they stem from configuration issues or resource shortages, is crucial for resolving them swiftly.
Using tools like Apache Ambari can aid in monitoring your Hadoop ecosystem and provide real-time insights into the health of your clusters. Regularly reviewing logs can also help in pinpointing recurring problems, making it easier to troubleshoot before they escalate into larger issues.
Additionally, employing a systematic approach to debuggingâsuch as isolating the segments of code responsible for the errorsâcan prove invaluable. With adequate documentation and version control, the process of troubleshooting becomes less daunting.
End
As we wind down this discussion of common challenges in Hadoop development, it becomes evident that each hurdle presents an opportunity for optimization. By familiarizing oneself with large dataset handling, performance tuning, and rigorous error management, developers set themselves up for success in leveraging Hadoopâs capabilities. Addressing these challenges head-on lays the groundwork for a more efficient, robust, and scalable data processing environment.
Best Practices for Writing Hadoop Code
Writing Hadoop code that is efficient, maintainable, and scalable is crucial in ensuring the success of big data projects. Following best practices helps minimize errors, enhances collaboration among team members, and streamlines the development process. By focusing on specific elements and the considerations surrounding them, you can optimize your Hadoop coding ventures.
Adopting Coding Standards
Coding standards are the backbone of any strong development environment, especially within the Hadoop ecosystem. They provide a common foundation for all programmers involved in the project. When everyone adheres to the same standards, it becomes easier to understand and maintain code collaboratively.
- Consistency: Consistent naming conventions, indentation styles, and overall formatting lead to a cleaner codebase. For instance, you might use camelCase for method names and snake_case for variable names to differentiate purposes clearly.
- Readability: Itâs paramount for code to be readable. Avoid overly complex expressions. Instead, strive to write code that another developer would find intuitive. The easier it is to pick up and grasp your logic, the better.
- Comments and Documentation: Code should not just run efficiently, it should also tell a story. Brief but informative comments explaining the purpose of different sections can save time in the long run when others (or even yourself) revisit the code.
Adopting solid coding standards is an investment that pays dividends in project integrity and team productivity.
Documentation and Version Control
Documentation is often the unsung hero of programming efforts, especially in something as complex as Hadoop. It helps ensure everyone is on the same page regarding project goals and technical decisions. Moreover, version control is equally significant. It allows for pausing and rolling back changes, which is crucial when troubleshooting or adapting code.
- Use Documentation Tools: Consider utilizing platforms like GitHub or Bitbucket. They not only support version control but also provide spaces for documentation, ensuring everything is housed in one place.
- Writing Clear Commit Messages: When using version control, each commit should represent a logical change grouped under clear messages. This practice is bread and butter for anyone maintaining or reviewing the project.
- Code Reviews: Facilitate discussions around the documentation and changes during reviews. Itâs a great opportunity to add collective wisdom to the code and its documentation.
Proper documentation coupled with diligent version control not only safeguards your work but enhances team communication, making sure that no one is left in the dark.
Testing and Validation Techniques
Testing is where code transformation really shines. It assures you that your Hadoop jobs run as expected under various conditions, and it brings additional confidence to the deployment phase.
- Unit Testing: For every component you build within Hadoop, unit tests should be a priority. Writing tests that confirm each piece functions as intended can be a lifesaver when you start stacking components together.
- Integration Testing: This step comes in when multiple units need to work together. Validating their collaborative behavior helps to establish that the system as a whole performs according to requirement.
- Load Testing: Given the nature of Big Data, understanding how your application behaves under stress is vital. Simulate high loads to uncover potential bottlenecks before actual deployment, ensuring hard-won data isn't compromised in usage.
Many leverage frameworks like Apache JUnit which pairs well with Hadoop programming to streamline and automate testing processes.
In summary, applying best practices such as adopting coding standards, diligent documentation, and rigorous testing are foundational to successful Hadoop coding. Not only do they improve your project's integrity and ease of use, they also establish a trustworthy environment for development. Embracing these practices creates a smoother path for teamwork and innovation in the Hadoop ecosystem.
Future of Hadoop Development
As we look ahead, the importance of understanding the future of Hadoop development can't be understated. With continuous advancements in technology, the landscape for big data is ever-changing, and so too is Hadoop's role within it. The discussions around the future often revolve around several key elements that are vital for programmers and developers alike. These include emerging trends, the integration of cloud computing, and the ongoing evolution of the Hadoop ecosystem itself.
Emerging Trends in Big Data Technologies
One cannot deny that technologies around data management are constantly evolving. Emerging trends that are shaping the future of Hadoop development include:
- Artificial Intelligence and Machine Learning: Businesses are increasingly relying on AI and machine learning to extract valuable insights from data. Hadoop is becoming the backbone of these technologies, enabling the storage and processing of vast amounts of data needed to train models.
- Data Lakes vs. Data Warehousing: With the growing popularity of data lakes, the way organizations handle unstructured data is changing. Unlike traditional data warehousing, Hadoopâs flexibility in managing varied data types is making it an ideal choice for creating data lakes.
- Real-time Data Processing: As the demand for real-time analytics increases, the integration of tools like Apache Kafka with Hadoop is becoming a norm. This allows organizations to react instantaneously to data as it flows in, which is critical in sectors like finance and healthcare.
"The rapid growth of big data technologies has positioned Hadoop as an essential player, but where it goes next remains to be seen."
The Role of Hadoop in Cloud Computing
Hadoop's compatibility with cloud computing frameworks is proving to be a game-changer. With the rise of services like Amazon EMR and Google Cloud Dataproc, businesses can leverage the power of Hadoop without the need for heavy on-premise infrastructure. The benefits are numerous:
- Cost-Effective Scalability: Companies can scale their usage of Hadoop based on their immediate needs, significantly lowering costs compared to maintaining an on-premises setup.
- Access to Additional Tools: By integrating Hadoop into the cloud, developers gain seamless access to a suite of additional tools and services, from machine learning to advanced analytics.
- Enhanced Collaboration: The cloud encourages more collaboration among teams spread across different locations, allowing for real-time sharing and processing of data.
Continued Evolution of the Hadoop Ecosystem
As the Hadoop ecosystem grows, its components continue to be refined and improved. Keep an eye out for:
- Increased Focus on Security and Compliance: With the heightened scrutiny on data privacy, future Hadoop distributions are likely to place more emphasis on security features.
- Tool Integration: Collaborations with more data processing tools, such as Apache Spark and Apache Flink, are paving the way for a more versatile data handling environment.
- Community Growth: The vibrant community around Hadoop fuels continual enhancements and innovations, ensuring it stays relevant amid fast-paced technological advances.