Master AWS with Python: A Detailed Guide for Beginners
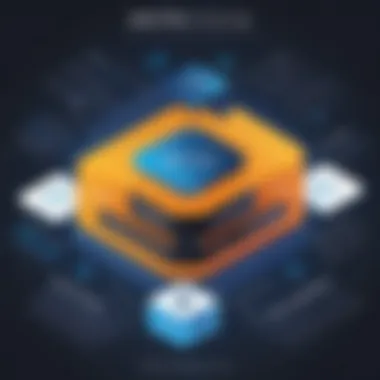
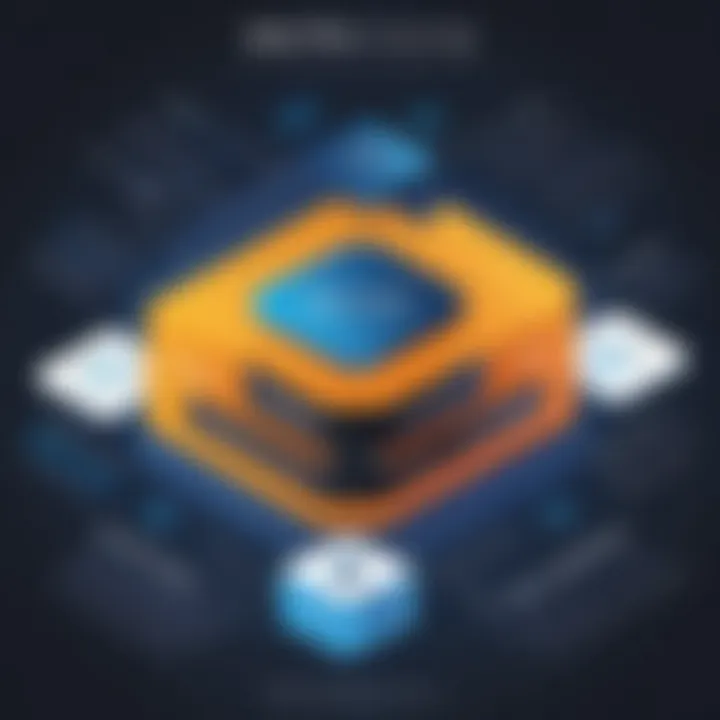
Intro
Understanding how to combine Amazon Web Services (AWS) with Python has become a valuable skill in today’s tech landscape. As businesses continue to shift towards cloud-based solutions, Python’s versatility emerges as a key player in harnessing the full potential of AWS. This guide aims to help you navigate this integration by covering actionable insights and essential skills.
Prelude to Programming Language
History and Background
Python was created in the late 1980s by Guido van Rossum. It was designed as a successor to the ABC programming language, prioritizing code readability and simplicity. Over the years, Python has grown from a small, experimental language into one of the most widely used programming languages in the world. Its ability to adapt to different paradigms—such as procedural, functional, and object-oriented programming—has bolstered its popularity.
Features and Uses
Python is often praised for its clean and straightforward syntax, which allows newcomers to grasp programming concepts more easily. It comes with a vast standard library, making various tasks manageable without the need to write extraneous code. Python is used in various fields, including web development, data analysis, artificial intelligence, and cloud computing, making it a jack of all trades, really.
Some noteworthy features of Python include:
- Dynamic Typing: Variables can change type, providing flexibility.
- Interpreted Language: No compilation needed, which speeds up development.
- Rich Ecosystem of Libraries: Libraries like make AWS integration straightforward.
Popularity and Scope
Recent surveys indicate that Python ranks as one of the most popular languages, favored by both beginners and experienced developers. Its strong community support and extensive resources contribute to its continued growth. From small startups to fortune 500 companies, Python’s applications within AWS—like serverless computing and machine learning—offer enormous capabilities.
Basic Syntax and Concepts
Variables and Data Types
To get started with programming in Python, it’s essential to understand its basic syntax. Variables act as containers for storing data values.
For example:
Here, is a string, and is an integer. Basic data types include integers, floats, strings, and booleans.
Operators and Expressions
Python supports various operators, from arithmetic to comparison. For instance, to add two numbers:
This will yield a result of . Operators are essential for creating more complex expressions and functions.
Control Structures
Control structures like conditionals and loops shape the flow of programs. Using if-statements, you can execute specific code based on conditions:
For repeated actions, loops such as and serve useful purposes.
Advanced Topics
Functions and Methods
Functions in Python are blocks of code designed to carry out a specific task. A simple example is:
With functions, code becomes reusable and organized. You might find them particularly useful while interacting with AWS services, such as automating deployment processes.
Object-Oriented Programming
Python supports OOP principles like inheritance and polymorphism, which allow developers to create complex software. When working with AWS, applying OOP can lead to more maintainable and scalable code. For instance, classes could be defined to represent AWS resources.
Exception Handling
Errors are an inevitable part of programming. Using try-except blocks, Python offers a way to manage exceptions gracefully. Here’s how it looks:
This ensures that your application doesn’t crash unexpectedly, which is always a plus.
Hands-On Examples
Simple Programs
To get your feet wet, you can start with a simple AWS interaction, such as listing S3 buckets:
This code snippet showcases the ease of communication between Python and AWS.
Intermediate Projects
As you grow more confident, consider working on a project that utilizes AWS Lambda to automate tasks or deploy a serverless application. Doing so can immensely deepen your understanding of how AWS works.
Code Snippets
Building a library of Python code snippets tailored to AWS services will provide you with quick solutions for frequent tasks. Consider maintaining a repository on GitHub or similar platforms for easy access.
Resources and Further Learning
Recommended Books and Tutorials
- Automate the Boring Stuff with Python by Al Sweigart - A great book for beginners.
- AWS Certified Solutions Architect – Associate by Ben Piper - Preps you for AWS exams, but it also covers essential services.
Online Courses and Platforms
Online platforms like Coursera or Udemy offer specialized courses. Look for topics that specifically focus on AWS and Python integration.
Community Forums and Groups
Joining communities on platforms like Reddit or Facebook can provide tremendous support as you dive into the complexities of AWS with Python. Engaging with others who share similar interests often leads to learning opportunities and inspiration.
Remember: The journey of mastering AWS with Python is ongoing. Constant practice and exploration of new features will further your skills.
Understanding Cloud Computing
Grasping the concept of cloud computing is paramount for anyone looking to dive into the realms of AWS and Python. In the digital age, understanding how technology handles data and services via the internet is crucial. This section breaks down the fundamentals of cloud computing, highlighting its significance in both personal and professional contexts. The essence lies in knowing that cloud computing allows users to access resources remotely rather than relying solely on local hardware.
Defining Cloud Computing
Cloud computing refers to the delivery of various services through the internet, including storage, databases, servers, networking, software, and analytics. Users typically access these resources on-demand, paying only for what they use.
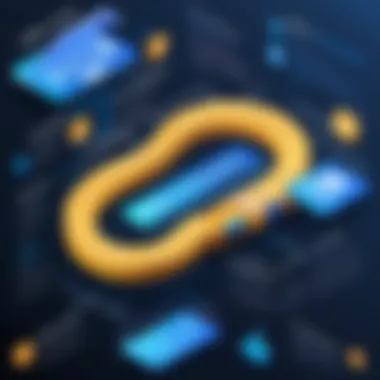
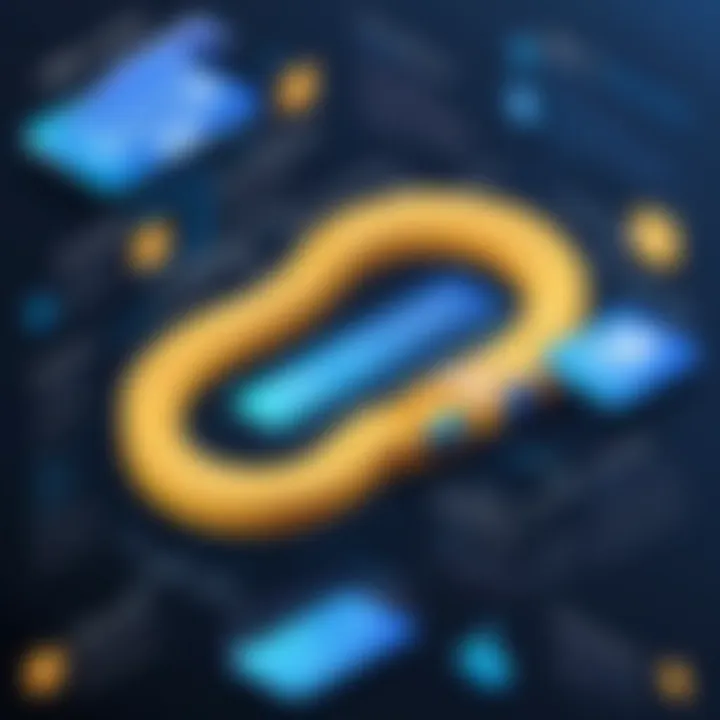
A clearer analogy is to think of cloud computing as tapping into an electric grid. Instead of producing your own electricity, you draw power from a larger supply when needed. Similarly, businesses can leverage powerful computing resources without needing to invest heavily in physical infrastructure. This flexibility and scalability are some of the critical elements that define cloud computing today.
Moreover, cloud computing generally comes in three flavors: Infrastructure as a Service (IaaS), Platform as a Service (PaaS), and Software as a Service (SaaS). These models allow users to choose their service level according to their needs, from raw computing power to fully developed applications.
Benefits of Cloud Technologies
When delving into cloud technologies, several benefits emerge that can transform how organizations operate:
- Cost Efficiency: Utilizing cloud services can significantly reduce upfront hardware costs and maintenance needs, as companies pay for only necessary resources.
- Scalability: Companies can easily scale up or down their infrastructure based on demand. For instance, during a product launch, a company can quickly provision more resources and scale back after demand decreases.
- Accessibility: Team members can access data and applications from anywhere, fostering a remote work culture and collaboration across diverse locations.
- Disaster Recovery: Data stored in the cloud is often backed up across multiple locations, which helps in ensuring data integrity and availability, even during unexpected events.
- Automatic Updates: Cloud service providers frequently update their offerings, meaning organizations can leverage the latest features without the hassle of manual installations.
"Understanding cloud computing isn’t just about technologies; it’s about recognizing the systemic change it brings to how businesses operate and innovate."
By digesting these concepts, readers will lay a solid foundation to further explore AWS services alongside Python, enabling them to exploit the full potential of cloud computing. This understanding will not just inform practice, but also guide strategic decision-making in adopting cloud technologies.
Prelude to AWS
In today's fast-paced tech landscape, wrapping your head around Amazon Web Services is not just a good idea; it’s becoming essential for anyone looking to make their mark in cloud computing. AWS stands as a powerhouse, offering a smorgasbord of cloud services tailored to any scale—from budding startups to established enterprises. By introducing readers to AWS in this article, we're laying the groundwork that enhances their understanding and empowers them to effectively utilize Python as a tool in this vast ecosystem.
AWS's scalability means that resources can expand or contract based on demand, which is a lifesaver for developers. Furthermore, its global infrastructure allows applications to be deployed quickly across different regions. Thus, when you integrate this with Python, you're opening doors to a world of possibilities that can help develop robust applications that cater to varied business needs.
Overview of Amazon Web Services
AWS can be envisioned as a toolbox where each tool serves a different purpose, making tasks easier and more efficient. Launched in 2006, AWS has consistently added to its suite of services which now covers everything from fundamental computing resources and storage options to more complex solutions like artificial intelligence. This flexibility makes it a popular choice among developers who seek to innovate and experiment without heavy initial investments.
A noteworthy mention here is that AWS operates on a pay-as-you-go pricing model, minimizing upfront costs and allowing businesses to only pay for what they use. This way, even small companies can leverage world-class infrastructure without breaking the bank.
Key Services Offered by AWS
AWS's offerings are myriad, but let's take a closer look at three vital areas that are particularly relevant to this guide: Compute Services, Storage Solutions, and Networking Services.
Compute Services
When you think of Compute Services, picture the engines that power your applications. AWS provides a range of options, such as EC2 (Elastic Compute Cloud), which allows users to launch virtual servers in the cloud. This flexibility is a game-changer—whether you need a single virtual server or a fleet of thousands, AWS can accommodate you. The key characteristic of Compute Services is their scalability.
A unique trait of EC2 is its auto-scaling capabilities, enabling developers to automatically increase or decrease the number of instances based on demand. This elasticity not only optimizes performance but also aids in cost management—a crucial aspect for any operation. With such an approach, organizations can focus on optimizing their applications without worrying about their underlying infrastructure.
Storage Solutions
Cloud storage is where AWS shines with options like Amazon S3 (Simple Storage Service). This service is akin to a digital storage locker where data can be both stored and retrieved with ease. With S3, users benefit from its durability and availability features, ensuring that data is never at risk of loss. The standout characteristic here is its straightforward scalability; as your requirements grow, S3 adjusts accordingly.
One of S3's unique features is its lifecycle policies, which allow you to automatically transition data between different storage classes based on specified criteria. This helps in managing costs and ensures that your data management strategy aligns well with your operational needs, be it for backups, archives, or active datasets.
Networking Services
Networking Services in AWS can often feel like the unsung heroes of cloud architecture. Services like Amazon VPC (Virtual Private Cloud) provide a segregated space within the AWS cloud where you can define your private network. Here, you have complete control over your virtual networking environment, including things like IP address ranges and subnets. The key characteristic of VPC is its flexibility, enabling you to define how your resources communicate with each other and the outside world.
A defining feature is its support for hybrid cloud architectures, allowing organizations to link their on-premises network to AWS seamlessly. This integration not only promotes enhanced security but also fosters the ability to scale without compromising control over IT resources. Ultimately, the diverse offerings in Networking Services cater to an encompassing range of business needs, providing frameworks that are both powerful and adaptable.
Incorporating these AWS services into Python applications sets a strong foundation for efficient development and management of web-based applications, allowing developers to focus more on coding and less on infrastructure management. Through this exploration, you’ll become more proficient in utilizing AWS alongside Python, bridging both worlds for a successful cloud computing journey.
Python: A Versatile Language for Cloud Applications
In the realm of cloud computing, Python stands out as a robust and adaptable programming language. Its simplicity and readability make it a go-to choice for many developers. When applied within the AWS environment, Python brings a myriad of benefits that help streamline application development and enhance productivity. Its vast library ecosystem caters to various aspects of cloud applications, making it not just a tool, but rather a comprehensive framework for building scalable, efficient systems.
Why Choose Python for AWS Development?
There are several compelling reasons to consider Python for AWS development. Firstly, the language's ease of use allows even beginners to quickly grasp the essentials of programming. As a result, Python often shortens the learning curve for those new to cloud computing. Furthermore, Python boasts a robust community that continually contributes to its libraries and tools, ensuring developers have access to the latest advancements.
Additionally, AWS offers extensive support for Python through the availability of the Boto3 library, which simplifies interactions with various services. This support means that developers can implement complex AWS functionalities with minimal code, saving time and reducing error potential. Moreover, Python’s versatility enables developers to seamlessly integrate with other technologies, such as JSON, APIs, and various data formats, enhancing the capability of their applications.
Essential Python Libraries for AWS
Python has several libraries that significantly ease the development process when working with AWS. Understanding these libraries can lead to better efficiency and effective use of AWS resources.
Boto3
Boto3 is an essential library for any developer working with AWS. This library directly interfaces with AWS services and allows Python developers to create, configure, and manage AWS resources. One of its key characteristics is the fact that it wraps around the AWS API, transforming complex requests into simple commands. This user-friendly nature makes it quite a favorite among developers.
The unique feature of Boto3 is its resource abstraction which provides a higher-level interface to AWS services, making operations less cumbersome. For instance, instead of dealing with intricate request structures, developers can simply call methods that represent higher-level abstractions of AWS resources. However, it's worth noting that Boto3 might require a fair amount of initial setup for authentication, which can be tricky for novice users.
Requests
The Requests library is vital for making HTTP requests in Python, serving as the backbone for any interaction over the web. When considering working with AWS, Requests comes in handy for making RESTful API calls. This library's primary appeal lies in its straightforward syntax, making it uncomplicated to pull in data or send requests to AWS APIs.
A particular strength of Requests is its simplicity when managing sessions and handling cookies; this is crucial when developing applications that rely on user data or need continuous interaction with various AWS services. However, some might find that advanced functionality—like asynchronous requests—may not be as robust in Requests compared to other libraries.
Flask
Flask emerges as a lightweight framework for building web applications. It offers flexibility while allowing developers to implement their projects without much overhead. By integrating Flask with AWS, one can create serverless applications using AWS Lambda, making deployments a breeze.
What sets Flask apart is its modularity and ease of use, which is particularly advantageous for developers looking to test and prototype rapidly. It also allows for intricate integrations with other AWS services during development. On the flip side, as projects grow in complexity, Flask applications can become cumbersome to manage without adopting additional structuring approaches.
"Choosing the right tools and libraries can make or break your cloud application experience. Python, along with its powerful libraries like Boto3, Requests, and Flask, provides a solid foundation to build on!"
By leveraging Python alongside AWS, developers can undertake various projects, from data processing tasks to web applications. Each library comes with its characteristics and usability considerations that should align with the project goals and developer experience. With this understanding, one can effectively implement and manage applications in the cloud.
Setting Up Your Environment
Setting up your environment is a crucial first step when it comes to developing applications on AWS using Python. It ensures that you have all necessary tools and libraries ready for your coding journey. A properly configured environment not only streamlines development but also minimizes errors, allowing you to focus on solving problems rather than troubleshooting setup issues.
Installing Python
Before anything else, you’ll need to have Python installed on your system. Python is the backbone of the development process, providing the language's flexibility and power. To get started, go to the official Python website and download the latest version. If you're on Windows, make sure to select the version that matches your operating system – 32-bit or 64-bit. For macOS or Linux users, Python usually comes pre-installed, but it’s a good idea to check for the latest version.
After downloading, run the installer. When installing on Windows, ensure that the box for "Add Python to PATH" is checked. This setting will enable you to run Python commands from your command line without needing to specify the whole path to the Python executable. For macOS and Linux, installation can often be simplified by using a package manager. Just a quick command in your terminal:
Once installed, you can verify it by running a quick check:
If successful, you should see the version number displayed in your terminal.
Configuring Boto3 for AWS Access
Now that you have Python ready, it's time to set up Boto3, the AWS SDK for Python. Boto3 allows you to interact with various AWS services seamlessly. To install it, simply use the pip command in your command line:
After installation, you'll need to configure your AWS credentials to connect Boto3 with your AWS account. The credentials consist of an Access Key ID and a Secret Access Key, which you can generate through the AWS Management Console. Be sure to keep these secure and never share them publicly.
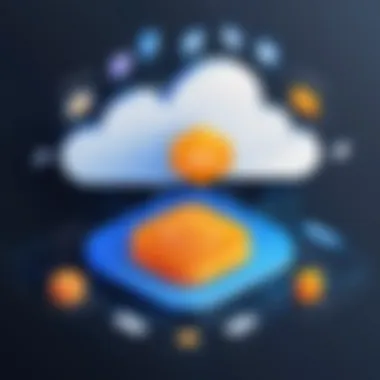
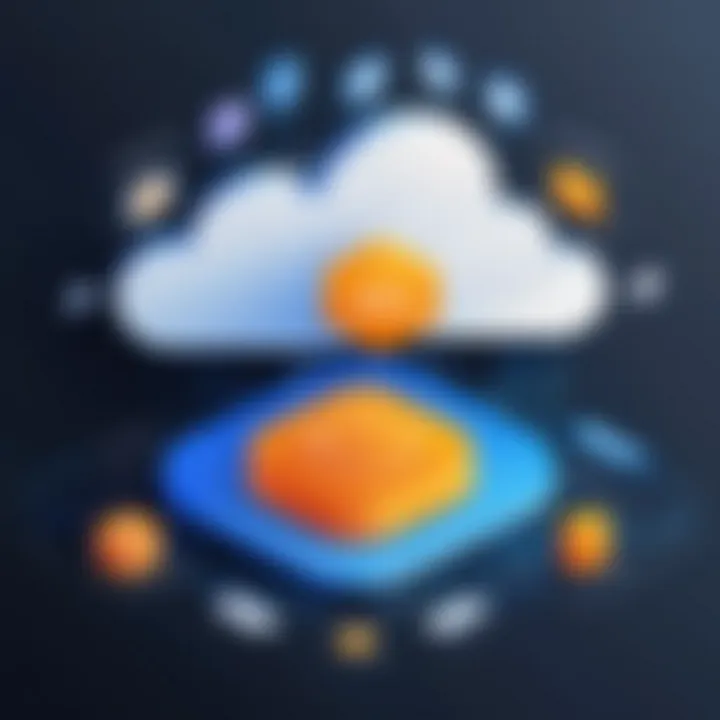
To configure Boto3, you can create a configuration file in your home directory. Typically, this file is located at for Linux and macOS or for Windows.
Here is an example of how the credentials file should be structured:
Make sure to replace , , and with your actual credentials. With this set, Boto3 will now be able to communicate with AWS services using your credentials, facilitating a smoother development experience.
"A well-set environment can save you countless hours of head-scratching and debugging later on, so it pays to get it right from the start."
Finale
Setting up your environment with Python and Boto3 is an essential foundation for building robust applications on AWS. With the right tools and configurations in place, you position yourself for success as you navigate through various AWS services. Take the time to ensure each step is performed accurately; it will benefit you tremendously as you advance in your Python and AWS journey.
Basic Operations on AWS with Python
When discussing cloud computing, one cannot overstate the importance of mastering basic operations on AWS using Python. These foundational skills empower individuals to effectively interact with various AWS services, laying the groundwork for more complex tasks later on. Moreover, a solid understanding of these operations enhances one’s ability to streamline processes, optimize resource use, and generally improve workflows in cloud environments.
Incorporating Python into AWS operations comes with several benefits. Firstly, Python’s simplicity and readability make it a popular choice among developers and data scientists alike. It allows users to quickly script tasks that would otherwise be cumbersome, especially when it comes to repetitive operations. Thus, knowing how to perform basic tasks can be the difference between a clunky, time-consuming process and a streamlined, efficient workflow.
Creating an AWS S3 Bucket
Creating an Amazon Simple Storage Service (S3) bucket is often one of the first steps taken when working with AWS. An S3 bucket acts as a storage container where users can store and manage data. Its significance cannot be stressed enough, as it serves as the foundation for file storage within AWS. Having a solid grasp of how to create and manage S3 buckets is crucial for any developer looking to utilize AWS effectively.
Step-by-Step Guide
The step-by-step guide to creating an S3 bucket simplifies the process for newcomers. It ensures that the end-user can follow each point without getting tangled up in the details. This clarity is particularly beneficial as it demystifies the operation and allows even novices to set up their environments seamlessly.
One key characteristic of this guide is that it covers all essential settings and configurations, so nothing important is overlooked. Each step is elaborated with caution not to overcomplicate matters. Instead, it promotes a straightforward approach that highlights why S3 is a preferred choice for many developers.
A unique feature of the step-by-step guide is its focus on user permissions, which points to the importance of security in cloud operations. While creating a bucket is simple, ensuring that the right policies are attached to it is paramount, thus blending usability with safety.
Handling Exceptions
Handling exceptions is another critical aspect of operations. When creating an S3 bucket or performing any AWS operations, errors can easily pop up due to insufficient permissions or network issues, among others. A systematic approach to managing these exceptions enables users to implement quick fixes and maintain their flow of work.
One notable characteristic of handling exceptions is that it fosters a programming discipline where one anticipates issues before they become problematic. For example, capturing exceptions allows for logging error messages, which can be invaluable for debugging.
Moreover, this practice exemplifies the notion of writing robust code, something that elevates the skill level of a developer. Recognizing which errors to catch and how to deal with them can significantly reduce downtime and frustration.
Uploading Files to S3
Uploading files to S3 is yet another essential operation for any developer. This task often extends beyond merely placing files in storage; it encompasses organizing these files for ease of access and management. Mistakes in this area can lead to disorganized data, complicating retrieval later on.
Using Boto3
Boto3 is the Python SDK for AWS, which makes it much simpler to interact with AWS services, including S3. Utilizing Boto3 for uploading files not only streamlines the process but also opens up a myriad of options that might otherwise go unnoticed.
A defining characteristic of Boto3 is its comprehensive capability; one can perform various actions with an ease that is not easily matched. For instance, Boto3 allows for integration with other AWS services, enabling complex workflows that can involve multiple resources, a testimony to its versatility.
However, some might argue that Boto3 has a learning curve. While it greatly simplifies operations, understanding its full capabilities can take some time. Still, the benefits of mastering this tool greatly outweigh its initial complexity.
File Management Techniques
Understanding file management techniques is equaly crucial in effectively utilizing S3. Knowing how to rename, delete, or modify files in S3 can save considerable time and headaches in a real-world scenario. These practices ensure that data remains organized and easily accessible.
A standout feature of effective file management is its ability to automate routine tasks. Utilizing scripts to manage files not only keeps your S3 bucket neat but also minimizes the risks of human error. By creating a structured approach, you maintain a professional standard in your cloud storage solutions.
In the end, the lessons learned from basic operations on AWS with Python serve as indispensable skills for any aspiring developer. Whether creating buckets or uploading files, the knowledge acquired here paves the way for more advanced cloud-based applications.
Advanced AWS Services and Integration
When diving into cloud computing, particularly with AWS, understanding advanced services is like having a treasure map. With AWS constantly evolving, embracing its more intricate features can streamline development and create powerful applications. Advanced AWS services offer scalability, efficiency, and flexibility, which are crucial for anyone looking to harness the full potential of cloud environments.
From serverless architectures to databases designed for quick access, integrating these advanced services can drastically shape how applications function. When using Python with AWS, leveraging these services not only enhances performance but also simplifies management tasks.
Using Lambda for Serverless Applications
AWS Lambda allows developers to run code without having to provision or manage servers. This serverless setup drastically reduces overhead, letting users automate functions in a scalable manner. The beauty of Lambda lies in its event-driven architecture, which triggers code execution in response to specific events, such as uploads to S3 or database updates. This enhancement means developers can focus on writing code instead of worrying about infrastructure.
Benefits of AWS Lambda include:
- Cost-effectiveness, where you only pay when your code runs
- Simplified deployment and scaling, as AWS manages the infrastructure for you
- Continuous invocation capabilities, adapting seamlessly to application demands.
But while appealing, Lambda does have its quirks, like the cold start issue. This is when allocation and initialization times lag behind the execution of functions, affecting performance. Overall, Lambda presents a compelling choice for Python developers aiming for efficiency and innovation without the headache of server management.
AWS DynamoDB with Python
DynamoDB is a fully managed NoSQL database that provides fast and predictable performance with seamless scalability. For anyone using Python to build applications on AWS, understanding DynamoDB is key to managing large datasets efficiently. Its flexibility allows developers to model relationships and scale as needed without worrying about database maintenance.
Setting Up DynamoDB
Setting up DynamoDB is fairly straightforward. One key characteristic of DynamoDB is its table structure, where data is stored in tables but can dynamically adjust to various data types within items. This results in enhanced adaptability for application needs.
One unique feature of DynamoDB is automatic scaling, which means it can adjust capacity in response to demand automatically. This ability not only prevents high costs during slow periods but also ensures performance during traffic spikes.
However, this flexibility can have its disadvantages. Developers must understand partition keys well, as inefficient design could lead to throttling during peak loads. Nonetheless, it remains a thriving option for those integrating Python with AWS, blending functionality with user needs seamlessly.
Performing CRUD Operations
When it comes to application functionality, the ability to perform CRUD (Create, Read, Update, Delete) operations is vital. This feature of DynamoDB complements Python's versatility perfectly. Through Python's Boto3 library, executing CRUD operations becomes intuitive. For instance, inserting a new item in a DynamoDB table can be done using a simple API call.
The hallmark of performing CRUD operations here is its low-latency performance. Particularly for applications that require real-time data analysis, this characteristic makes DynamoDB a popular choice among developers.
However, there are caveats to consider. The eventual consistency model of DynamoDB means that immediate data retrieval isn't guaranteed after updates in certain scenarios, which can lead to unexpected results if not properly handled. This aspect necessitates an understanding of DynamoDB's consistency models, but once mastered, CRUD operations can significantly elevate the effectiveness of Python applications.
A well-designed application architecture incorporating AWS services can lead to substantial operational efficiency.
By leveraging these advanced services, developers not only enhance their applications but also ensure lasting performance and sustainability in their cloud endeavors.
In summary, navigating advanced AWS services with Python can unlock a realm of possibilities. The blend of serverless computing with Lambda and a robust database like DynamoDB can lead to efficient, scalable, and powerful applications that adapt to evolving demands in today’s fast-paced digital landscape.
Security Best Practices in AWS with Python
When venturing into the realm of cloud computing, particularly with AWS and Python, understanding security best practices cannot be overstated. The landscape of data management and application deployment is littered with threats that can jeopardize sensitive information and diminish operational integrity. In this section, we will explore critical security strategies that maximize the safety of your AWS resources when using Python.
Understanding IAM Roles
IAM, which stands for Identity and Access Management, plays a pivotal role in safeguarding AWS environments. By clearly defining permissions and roles, organizations can control who can access various resources in a granular way. In simple terms, IAM roles function as a set of instructions specifying what actions are allowable for different users, applications, or systems in the AWS ecosystem.
Consider the scenario where a developer is building an application that interacts with AWS S3 buckets to store user data. Instead of embedding AWS credentials directly into the code, which is both a security risk and difficult to manage, the developer can assign an IAM role to their application. This role grants permissions too access the necessary S3 actions, effectively separating access credentials from the application itself.
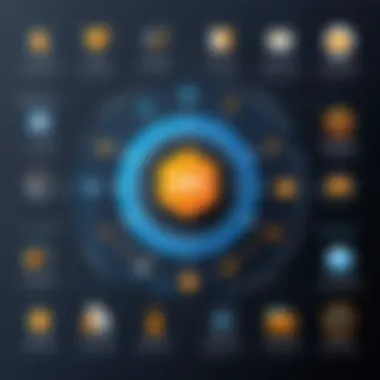
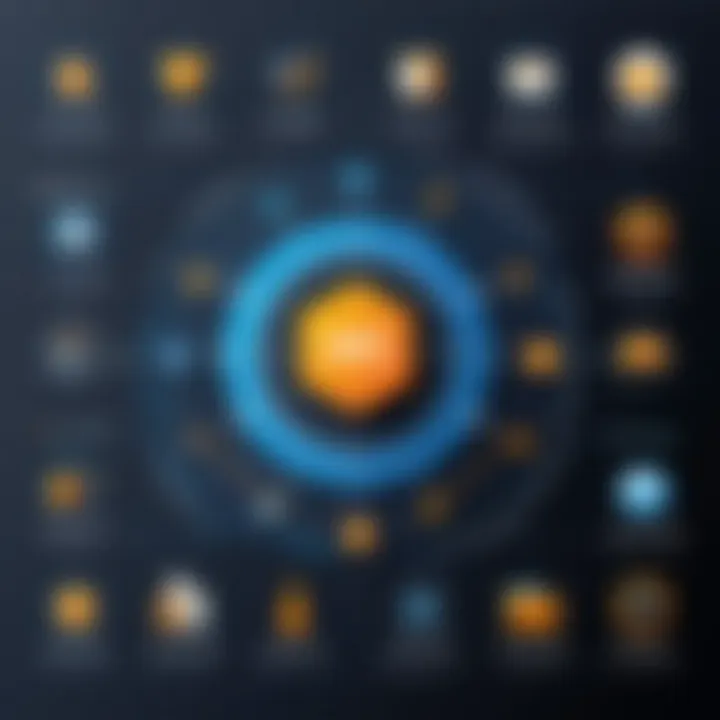
Key points about IAM roles include:
- Temporary permissions: IAM roles can provide access without the need for long-term access keys, reducing potential exposure.
- Least privilege principle: Always start with the minimum permissions needed and add more only when absolutely necessary.
- Easily revocable: If a role becomes irrelevant or overly permissive, it’s straightforward to modify or remove it, thus improving security posture.
“The right role at the right time makes all the difference.”
Managing AWS Credentials Securely
Effective management of AWS credentials is essential, especially when working with sensitive data and applications. Mismanagement of credentials can lead to unauthorized access, exposed resources, and ultimately, data breaches. Here’s how you can safeguard your AWS credentials while utilizing Python:
- **Use environment variables to manage sensitive information such as API keys, access keys, and database passwords. Hardcoding these details into your code can be a surefire way to expose them if the code is ever shared or pushed to a version control system, like Git.
- Adopt AWS Secrets Manager or AWS Systems Manager Parameter Store. Both services allow you to securely store, retrieve, and manage secrets, which can be accessed programmatically through your Python application. This way, sensitive information doesn’t leak into places it shouldn’t.
- Regularly rotate your credentials to limit the exposure time of a compromised key. Establish a routine process that fosters security maintenance and looks at credential usage logs to identify any anomalies.
- Implement multi-factor authentication (MFA) for user accounts accessing AWS. This adds an additional layer of security beyond just the username and password.
Managing AWS credentials doesn’t just involve avoiding pitfalls; it’s about being proactive and applying tools that AWS provides to help in hardening security.
By diligence and a strategic approach to IAM and credential management, you can create a resilient environment that not only defends against potential threats but actively promotes a secure approach to cloud application development.
Monitoring and Management Tools
In the realm of AWS and Python integration, monitoring and management tools serve as the backbone of operational excellence. These tools not only help in keeping an eye on system performance but also provide critical insights that can forecast potential issues. By effectively managing resources, users can ensure their cloud environments are running optimally and costs are kept in check. A robust monitoring strategy can steer users away from pitfalls that could lead to downtime, data loss, or unexpected expenses.
AWS CloudWatch for Monitoring
AWS CloudWatch is a powerful monitoring service that enables users to observe their AWS resources and applications in real-time. This tool collects and tracks metrics, collects log files, and sets alarms. It's like having an ever-watchful guardian that ensures everything is running smoothly.
Setting Alarms
Setting alarms is one of the hallmark features of AWS CloudWatch. By utilizing this capacity, you can get alerts whenever a certain threshold is breached. This process is indispensable as it provides proactive notifications that can prevent minor hiccups from escalating into major outages. A key characteristic of setting alarms is its versatility. Users can configure them for various metrics—CPU usage, memory utilization, or even custom metrics unique to an application.
Among the unique features of setting alarms is the ability to trigger automatic corrective actions. For example, if your server's CPU hits an alarming number, an alarm can enact a Lambda function to scale resources automatically. This adaptability enhances operational efficiency, ensuring that resource demands are met without manual intervention. However, it's crucial to recognize that excessive alarms can lead to alert fatigue, causing users to overlook genuine issues. So, striking the right balance is vital.
Analyzing Logs
Analyzing logs is a crucial part of effective monitoring that drives insight into system behavior. With AWS CloudWatch, users can collect, monitor, and visualize log data from different sources. Understanding logs is indispensable for troubleshooting and performance tuning. By dissecting logs, developers can pinpoint not just what's happening but also why something went wrong.
A standout feature of log analysis is its ability to integrate with CloudWatch's metric filters. This enables users to generate custom metrics from log data, further refining the monitoring process. An advantage here is the deep dive into system interactions that traditional metrics might miss. However, digging through logs requires a keen understanding and sometimes specific domain knowledge; thus, it might not always be user-friendly for everyone, especially beginners.
Managing Costs with AWS Budgets
Managing costs is a pressing concern for developers and businesses alike. AWS Budgets allows users to set a budget for their AWS usage and costs. This tool is not only about monitoring; it proactively helps organizations avoid surprises at the end of each billing cycle. Users can set thresholds for spending and receive alerts when the limits are approaching.
Effective cost management is critical in optimizing resource usage and ensures investments in cloud infrastructure are not wasted. AWS Budgets' unique feature sets, like custom budget reports, allow you to tailor insights specific to your organization’s needs. By utilizing these features, users can strategize better spending habits and forecast future expenses effectively. Nevertheless, understanding the intricacies of budgeting on AWS may present a learning curve, especially for those new to cloud computing.
"Monitoring and management tools are not just optional add-ons; they are essential for sustaining a healthy and cost-effective cloud environment."
The thoughtful deployment of monitoring and management tools like AWS CloudWatch and AWS Budgets sets the stage for better operational insight and cost control. These tools form integral components of any AWS strategy, allowing developers to harness the full power of cloud computing while maintaining a vigilant eye over system performance and expenditures.
Use Cases of AWS with Python
When integrating AWS with Python, it is vital to consider the practical applications of the technology at hand. Python, known for its simplicity and flexibility, shines in its ability to leverage AWS services for varied use cases. Understanding these real-world applications can not only enlighten students and budding developers but also highlight the numerous benefits involved in adopting such a robust platform. By exploring these use cases, one can appreciate how Python, combined with the potent offerings of AWS, enables innovative solutions in the cloud space.
Data Analysis and Processing
The realm of data analysis and processing stands as one of the most compelling use cases for utilizing AWS with Python. In today’s data-driven world, organizations gather copious amounts of data daily, and the ability to process it effectively can mean the difference between success and stagnation.
1. Scalability
AWS provides scalable storage and computing solutions, which are crucial when dealing with large datasets. Services like Amazon S3 (for storage) and Amazon EC2 (for compute resources) enable users to store vast amounts of data and efficiently analyze it with Python libraries such as Pandas or NumPy.
2. Tools and Integration
Python has an extensive collection of libraries that can seamlessly integrate with AWS services to perform data analysis. For instance, the Boto3 library allows Python scripts to interact directly with AWS resources. Additionally, you can utilize AWS Glue for data transformation, making it easier to prepare datasets for analysis.
Utilizing the right tools can elevate your data analysis tasks from mere operations to impactful insights.
3. Real-Time Processing
With the increasing demand for real-time insights, tools like AWS Lambda, combined with Python scripts, can help process and analyze data on-the-fly. By ingesting data through Amazon Kinesis and executing Python code to analyze streams in real-time, businesses can make informed decisions faster than their competitors.
Real-Time Web Applications
Creating real-time web applications is another front where AWS and Python join forces to create dynamic and responsive systems. These applications can range from social media platforms to live-streaming services, where user engagement depends on instantaneous interactions.
1. Frameworks and Services
Using popular frameworks like Flask or Django, developers can build the backends of web applications in Python, while leveraging services from AWS to host and manage their resources. AWS Elastic Beanstalk simplifies deployment, scaling, and monitoring without extensive configuration.
2. WebSockets for Communication
Real-time communication is vital for many applications, especially those involving chat or collaboration features. Libraries like Socket.IO in Python can be used in conjunction with AWS services to handle WebSocket connections, ensuring a smooth and responsive user experience.
3. Data Storage and Management
Storing user data or application metadata can be done with ease using Amazon DynamoDB. Its fast and flexible NoSQL database service allows you to store structured data and retrieve it in near real-time. Python, combined with the Boto3 library, can facilitate quick reads and writes, offering low-latency access to your application data.
As various industries adapt to changing technology landscapes, utilizing AWS with Python for real-time web applications will likely become increasingly prevalent.
Challenges and Limitations
When venturing into the integration of AWS with Python, one must tread carefully through the maze of challenges and limitations that can arise. Understanding these hurdles is crucial, not only for the success of your projects but also for navigating the expansive landscape of cloud computing effectively. These challenges can shape the trajectory of your learning and development journey, guiding how you approach AWS services with Python.
Common Pitfalls in Development
In the world of cloud computing, developers often stumble upon various pitfalls that can derail their progress. Here are a few of the most common:
- Insufficient Understanding of AWS Services: Often, developers dive into coding without fully grasping how AWS operates. Failing to comprehend the specific services can lead to inefficient architecture and wasted resources. For instance, not knowing when to use an S3 bucket for storage versus a DynamoDB table for structured data can impact both performance and cost.
- Neglecting Security Protocols: When working with sensitive data, overlooking AWS security features can spell disaster. Developers might skip implementing IAM roles or fail to set proper permissions. This can open doors for vulnerabilities in your cloud setup.
- Ignoring Proper Error Handling: Writing code without considering how it might fail is a rookie mistake. If exceptions are not handled correctly, an application can crash unexpectedly, leaving users in a lurch and wasting valuable resources.
"In programming, you can experience a world of frustration when you fail to account for the unexpected. Always be prepared to handle errors gracefully."
- Underestimating Cost Implications: Poor understanding of how different services are charged can lead to runaway costs. For instance, running instances continuously or using services that scale automatically without monitoring can accumulate charges faster than expected.
Falling into these traps can hinder your projects significantly. It's advisable to take a step back, do some research, and ensure that you understand the AWS landscape before jumping into development.
Understanding AWS Costs
Navigating costs in AWS can seem like walking through a minefield. Each service comes with its pricing structure, which can be quite convoluted. Here are some key considerations:
- Service Pricing Models: Specific services, like EC2, are billed based on the instance type, region, and duration of usage, while S3 charges are tied to storage use and requests made. Understanding these models is essential to budgeting properly.
- Free Tier Limits: AWS does offer a free tier for new users, but these limits are easily exceeded. For beginners, it’s easy to assume certain actions are free when they might incur costs after going beyond specific allowances.
- Monitoring and Budgeting Options: AWS provide tools like AWS Budgets that allow you to set spending limits. Enabling billing alerts can help you monitor usage and avoid nasty surprises at the end of the month.
- Long-Term Commitments: If you foresee long-term usage of a service, consider Reserved Instances for EC2 or similar offerings. These can save you significant amounts compared to pay-as-you-go models.
Future Outlook of AWS and Python
In today's rapidly evolving technological landscape, the combination of Amazon Web Services (AWS) and Python presents a bright horizon for software development and cloud applications. As businesses increasingly shift to cloud infrastructure, the importance of understanding how Python interacts with AWS cannot be overstated. This relationship opens doors for innovation, efficiency, and leveraging the vast array of services offered by AWS. Here, we explore the upcoming trends and advancements specific to this integration.
Emerging Trends in Cloud Computing
The cloud computing scene is evolving at a breakneck speed. Emerging trends not only redefine how businesses leverage technology but also impact how developers utilize programming languages like Python within the AWS ecosystem.
- Serverless Architectures: One standout trend is the rise of serverless computing. AWS Lambda allows developers to run code without provisioning servers. Python, being lightweight and flexible, is a natural fit for such architectures. This approach offers reduced operational costs and increased agility in developing applications, allowing teams to focus on writing code while AWS handles the scaling and infrastructure.
- Artificial Intelligence (AI) and Machine Learning (ML): AWS provides several services like Amazon SageMaker for machine learning. With Python’s robust data manipulation libraries like Pandas and NumPy, developers can create powerful AI models without hitting a wall. The integration of Python with AWS tools facilitates enhancing data-driven decision-making.
- Multi-cloud Strategies: Businesses are now adopting a multi-cloud strategy to avoid vendor lock-in and enhance redundancy. This means that developers need to ensure their Python applications work seamlessly across different cloud providers, including AWS, which can lead to more versatile and adaptable coding practices.
"The future belongs to those who believe in the beauty of their dreams." — Eleanor Roosevelt
- Containerization and Microservices: Docker and Kubernetes are reshaping how applications are developed and deployed. AWS offers services like Amazon ECS and EKS to simplify running and managing containers. Python is often used in microservice architectures, allowing for smaller, manageable codebases that can evolve independently.
Advancements in Python for AWS
As Python itself continues to evolve, its integration with AWS enhances the toolkit available to developers. Key advancements are paving the way for smoother, faster, and more innovative cloud solutions.
- Improved SDKs and Libraries: Python’s Boto3 library allows for easy interfacing with AWS services. The continuous updates to Boto3, including new features and support for the latest services, make it simpler and more efficient for developers to harness AWS capabilities.
- Enhanced Community Support: The Python community is burgeoning, resulting in more resources, tutorials, and forums dedicated to Python and AWS. Platforms like Reddit and specialized forums provide a wealth of information for newcomers and experienced developers alike, creating a collaborative environment for knowledge sharing.
- Inclusivity of Data Science Libraries: Integrating libraries such as TensorFlow and scikit-learn with AWS services enables developers to build scalable machine learning applications seamlessly. This allows anyone to tap into the power of data analytics directly from Python applications running on AWS.
- Streamlined DevOps Tools: With the increasing emphasis on continuous integration and continuous delivery (CI/CD), tools like AWS CodePipeline and AWS CodeDeploy simplify the deployment of Python applications, making it easier for developers to maintain velocity without sacrificing quality.
In summary, the future of AWS and Python is characterized by an ever-expanding landscape filled with opportunities. Understanding and adapting to these trends will not only allow developers to remain competitive but also harness the full potential of cloud computing.