Mastering JavaScript Functions: A Comprehensive Guide
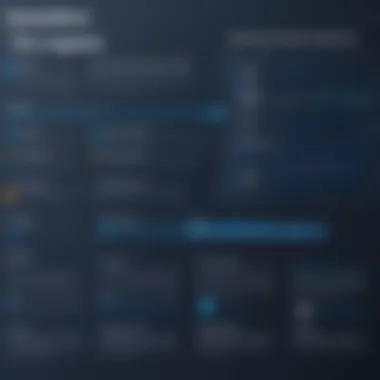
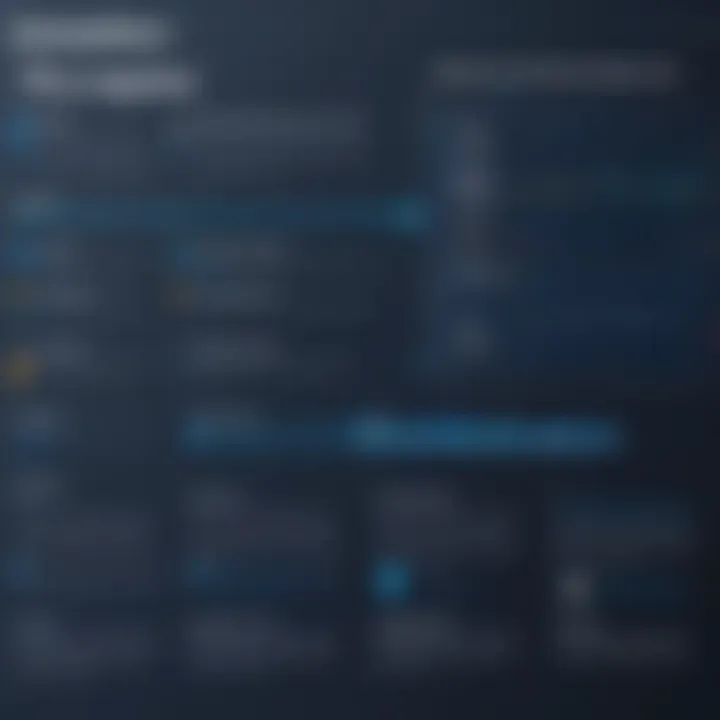
Intro
JavaScript has evolved as a crucial part of web development. Its role in creating dynamic and interactive web pages cannot be overstated. The understanding of functions in JavaScript is imperative for anyone wanting to excel in this language. Functions are fundamental building blocks, allowing developers to encapsulate code for reuse and organization.
While learning about functions, it is essential to first grasp the broader programming concepts. This article will unpack JavaScript functions comprehensively. The focus will include their definition, types, usage, and much more. Each aspect will show how important functions are in programming.
Importance of Functions in JavaScript
Functions serve numerous purposes. They allow for modular code, making it easier to maintain. They also improve clarity by breaking tasks into smaller pieces. By using functions, programmers can avoid repetition of code, leading to a more efficient coding process. One must note, however, that functions are not isolated components; they interact with variables, scopes, and closures, tying into the core essence of JavaScript.
"Understanding functions is pivotal for mastering JavaScript and its applications."
As we delve into the sections that follow, we will explore not just what functions are, but how they fit into programming as a whole. The intent is to equip you with tools that enhance your JavaScript proficiency and pave the way for advanced techniques.
Prelude to JavaScript Functions
In the landscape of programming, functions are fundamental building blocks that empower developers to write efficient and modular code. JavaScript functions, specifically, serve as a core element of the language, essential for executing tasks nimbly. Understanding how to leverage functions extends beyond mere syntax; it opens up pathways to better organization and reusability of code.
Functions enable coders to encapsulate behavior and functionality, allowing for clearer structuring of programs. They facilitate the principle of DRYβDonβt Repeat Yourselfβby enabling code reuse, thus reducing redundancy and the potential for errors.
When learning JavaScript, grasping how functions work is crucial. Functions can take inputs, perform operations, and return results. This versatility brings about significant benefits, including:
- Improved maintainability of code.
- Enhanced readability, leading to easier debugging and collaboration.
- Opportunities for encapsulation which protect data.
In essence, functions enhance a programmerβs ability to manage complexity and extend the potential of what can be achieved through coding. The subsequent sections will delve into deeper facets of functions, from their definitions to practical applications, contributing to a well-rounded understanding of this essential concept.
Understanding Functions
At its core, a function is a reusable block of code designed to perform a particular task. In JavaScript, functions serve both as a means to process operations and as a way to encapsulate behavior within programs. A simple definition elucidates that functions can be defined once and called multiple times throughout a program, extending their utility far beyond one-time use.
When defining a function, itβs common to specify parameters, which are like placeholders for the values that will be passed to it when invoked. This allows functions to be dynamic and adaptable, responding to various inputs accordingly. For example:
In this example, the function takes two parameters: and , and returns their sum. It showcases the straightforward nature of functions and illustrates how they can perform operations based on provided arguments.
Importance of Functions in JavaScript
Functions play a pivotal role in JavaScript due to their multi-faceted nature. They promote better organization of code and foster logical thinking by breaking down problems into smaller, manageable units. This is particularly advantageous in larger projects where individual components must communicate and work together seamlessly.
Additionally, functions support various programming paradigms, including functional programming. They allow developers to use functions as first-class citizens, meaning functions can be passed as arguments, returned from other functions, and assigned to variables, fostering a more flexible coding approach. The importance of functions can be summarized as follows:
- Modularity: Functions encourage modular design, making the codebase easier to navigate.
- Reusability: Functions can be reused across different parts of the application, which saves time.
- Clarity: With well-named functions, the code becomes self-documenting, improving comprehension.
As one embarks on this journey through JavaScript functions, it is essential to keep in mind their transformative potential. By understanding the various aspects of functions, learners can enhance their proficiency in JavaScript significantly.
Defining a Function
Understanding how to define functions is crucial in JavaScript programming. Functions are the building blocks of any JavaScript application. They allow developers to encapsulate code for reuse and provide a structured way to perform tasks. Defining a function establishes a routine that can accept input, perform operations, and return output, making code more organized and maintainable.
Syntax Overview
The syntax to create a function in JavaScript is straightforward. At its essence, the function is defined using the keyword. This is followed by a name, parentheses for parameters, and a block of code enclosed in curly braces. Here is the basic structure:
For example, a simple function to add two numbers may look as follows:
This syntax sets the foundation for how functions can be defined and invoked in JavaScript.
Function Declarations vs. Function Expressions
Understanding the difference between function declarations and function expressions is essential. A function declaration is a named function defined in the standard way shown above. It is hoisted, meaning it can be called before its definition appears in the code. For instance:
On the other hand, a function expression can be either named or anonymous and is not hoisted. It is often assigned to a variable:
In this case, calling before the assignment results in an error.
Immediate Function Invocation
Another useful concept is the immediate function invocation. This allows you to define and execute a function instantly. It is done by wrapping the function definition in parentheses and adding an additional pair of parentheses at the end. Hereβs an example:
This technique can be valuable for creating isolated scopes and avoiding polluting the global namespace. It is particularly helpful for initializing modules or defining configuration parameters without exposing them.
Understanding how to define functions correctly is key to writing effective JavaScript code. Knowing when to choose function declarations, expressions, or immediate invocations can lead to cleaner and more efficient programming.
Types of Functions in JavaScript
Understanding the types of functions in JavaScript is crucial for effective programming. Each type serves different purposes and caters to specific scenarios. By grasping these distinctions, you enhance your coding skills and can write cleaner, more efficient code. JavaScript supports various function types, including named functions, anonymous functions, and arrow functions. These allow for different styles of function definition and usage.
Named Functions
Named functions are the traditional way of defining functions. They come with a name, which provides clarity and makes it easier to call them later in the code. A named function can be invoked throughout its scope. For instance:
In this example, is a named function. The name serves as an identifier, allowing you to call it anywhere in the function's scope. Named functions also offer benefits in terms of readability and maintainability. By using descriptive names, you help others understand the purpose of the function at a glance, which is essential in larger codebases.
Anonymous Functions
Anonymous functions lack a name and are often used in situations where a function is required as an argument. Their most common use is in callbacks, such as when handling events or asynchronous actions. For example:
In this example, the callback function is anonymous. It doesnβt have a name, as it is used only once within the context of the call. This can lead to cleaner code since you do not need to create a separate function definition if you are only using a function for a short period. However, the lack of names can make debugging difficult, as stack traces may show only anonymous instead of specific names if errors occur.
Arrow Functions
Arrow functions were introduced in ECMAScript 6 and offer a more concise syntax for writing functions. They are particularly useful for writing short functions. Here's how an arrow function looks:
Arrow functions have benefits over traditional named functions. They inherit the context from their enclosing scope, making them ideal for certain programming patterns, especially when dealing with methods in classes or callbacks. This is in contrast to regular functions that often have their own , which may lead to confusion in the code.
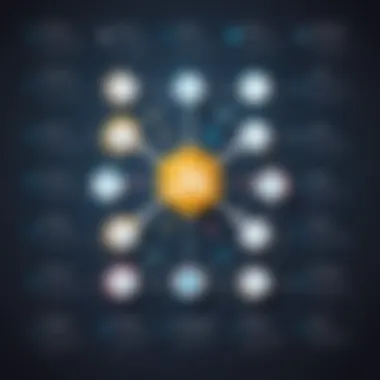
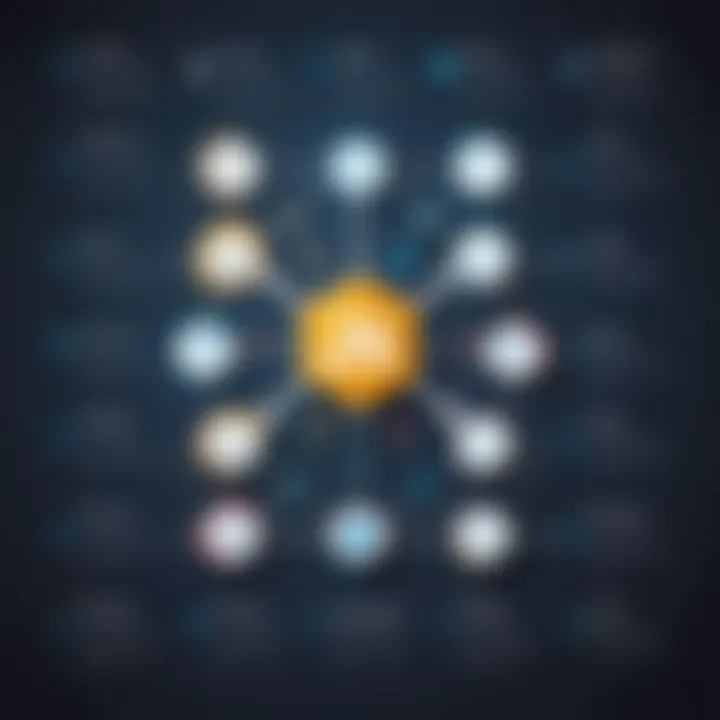
In summary, each type of function in JavaScript has its unique attributes and use cases. By understanding these differences, you can make informed decisions about when to use each function type, ultimately leading to better performance and clearer code.
Parameters and Arguments
Parameters and arguments are foundational concepts in JavaScript functions. They dictate how data is passed and managed within functions, which is crucial for achieving desired functionality and flexibility in code. By understanding parameters and arguments, developers can write more reusable and efficient functions. This section dives into the specifics of passing parameters, using default parameters, rest parameters, and the arguments object.
Passing Parameters
When defining a function, the parameters act as placeholders for the values that will be passed to it. A function can accept multiple parameters. The syntax is straightforward:
Here, and are parameters. When invoking this function, you provide actual values, known as arguments:
The values and are the arguments corresponding to the parameters in the function.
What is vital to note is that JavaScript allows users to pass any data type as an argument, from simple primitives like numbers and strings to complex objects. However, care must be taken to ensure that the function can handle the types of arguments being passed.
Default Parameters
JavaScript also offers default parameters, a feature that allows developers to set default values for parameters if no argument is provided during function invocation. This feature simplifies function usage and improves code readability. Here is how it looks:
In this case, if a caller does not provide an argument for or , the function will use and as values, respectively. This reduces the need for additional checks inside the function body to verify if parameters were supplied, making the code cleaner.
Rest Parameters
Rest parameters provide a way to represent an indefinite number of arguments as an array. This is especially useful when the number of parameters can vary. Using the rest operator, represented by three dots (``), enables functions to accept multiple arguments and organize them accordingly:
In this example, will be an array containing all passed arguments. This feature is particularly valuable for functions requiring flexibility, as it allows for easy management of a varying number of inputs. For instance, developers might use rest parameters in functions designed to handle lists or collections of items.
The Arguments Object
In addition to the above methods, there exists the object. This is an array-like object available within all non-arrow functions that represents the values of parameters passed into the function, even if they are not explicitly defined in the function's parameters. For example:
Calling this function will output an object containing three values, , , and , accessible by index. However, it is important to note that is not available in arrow functions, which is a consideration when choosing the appropriate function type.
The ability to use parameters and arguments effectively shapes the behavior of functions, making them indispensable tools in JavaScript programming.
Understanding these concepts is essential for anyone looking to deepen their knowledge of JavaScript and its capabilities.
Return Values in Functions
Understanding return values in JavaScript functions is crucial for effective programming. Functions often serve the purpose of performing actions and delivering outcomes based on input parameters. The return value of a function allows it to provide information back to the caller, making it an essential feature in programming. This enhances modularity and reuse of code. Knowing how to effectively use return values contributes significantly to writing efficient programs.
Using the return Statement
The statement is fundamental in defining what a function sends back. When executing a function, the code runs until it hits the keyword. When this happens, the function exits, and whatever follows the keyword becomes the return value. If there is no statement, the function returns by default.
For example, consider the following function:
In this case, the function takes two parameters and returns their sum. This allows the calling context to utilize the result directly. Without a return statement, the benefit of executing the function is lost. A well-structured function utilizes return values to pass outcomes back to the calling code or other functions.
Returning Multiple Values
JavaScript does not support returning multiple distinct values from a function directly. However, there are strategies to achieve similar results. A common method is to return an object or an array containing the values. This approach maintains clarity and control over the returned information.
Here is an example that illustrates this concept:
Here, returns an object with three properties, each representing a different calculation. The caller can access these values conveniently:
This method of returning multiple values provides clarity and enhances data management. Consequently, it is important to understand how to structure return values efficiently.
Using clear return values can lead to more understandable and maintainable code.
In summary, effectively managing return values in functions can enhance programming efficiency. This area should not be overlooked as it directly contributes to the overall structure and organization of your code.
Function Scope
Understanding the concept of function scope is paramount for mastering JavaScript. Scope defines the accessibility of variables, functions, and objects in some particular area of your code. Its importance cannot be overstated, as it directly influences how variables are created, accessed, and manipulated across different functions. A solid grasp of function scope ensures that code remains clean, avoids variable conflicts, and enhances overall readability.Β
Understanding Scope
Scope in JavaScript can be simply defined as the context in which a variable is declared. The concept is divided into two primary types: global scope and local scope. Global scope allows variables to be accessible everywhere in the code, while local scope restricts access to within a specific function. Understanding this difference is crucial for anyone learning programming languages, as it eliminates confusion when handling variables in more complex scenarios.
Furthermore, scope affects how functions interact with each other. When variables are defined outside any function, they reside in the global scope. If they are declared inside a function, they are local to that function. The lexical scoping model used by JavaScript means that a functionβs scope is determined by its location defined in the code. This allows for efficient memory management and aids in preventing errors caused by unintended variable overriding.
Global vs. Local Scope
The distinction between global and local scope is critical for writing effective JavaScript. Global variables are accessible from any part of the code, which can lead to issues, especially in larger applications. If two functions use the same variable name, one might inadvertently overwrite the other. Here are some considerations:
- Global Variables: Accessible throughout the code, can be changed by any part of the program.
- Local Variables: Exist only within the function where they are declared, preventing external interference.
In practice, avoid the excessive use of global variables to maintain control and clarity over your functions. Keeping most variables local can reduce potential conflicts and keep your code modular.
Block Scope with let and const
With the introduction of ES6, JavaScript gained the capability of block scope through the use of and . These keywords allow variables to be confined to blocks, such as loops or conditionals. Unlike , which defines variables within a function scope, and create a narrower scope. This prevents variable access outside of the block, leading to safer and more predictable code.
For example:
Closures in JavaScript
Closures are a crucial topic in JavaScript that every learner must grasp to become proficient in the language. Understanding closures allows developers to leverage the benefits of function scope in their code. They play a vital role in variable scoping and data encapsulation. By mastering closures, programmers can create functions that maintain access to their lexical scope even after the outer function has executed. This feature is not just a theoretical concept; closures are frequently used in practical applications to manage data privacy, handle asynchronous behavior, and create more modular code.
Defining Closures
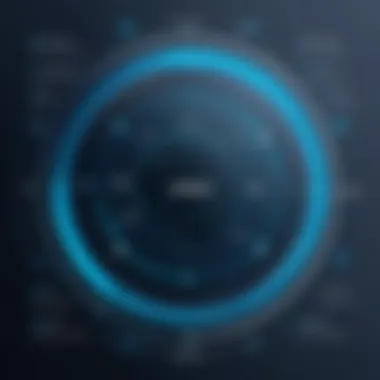
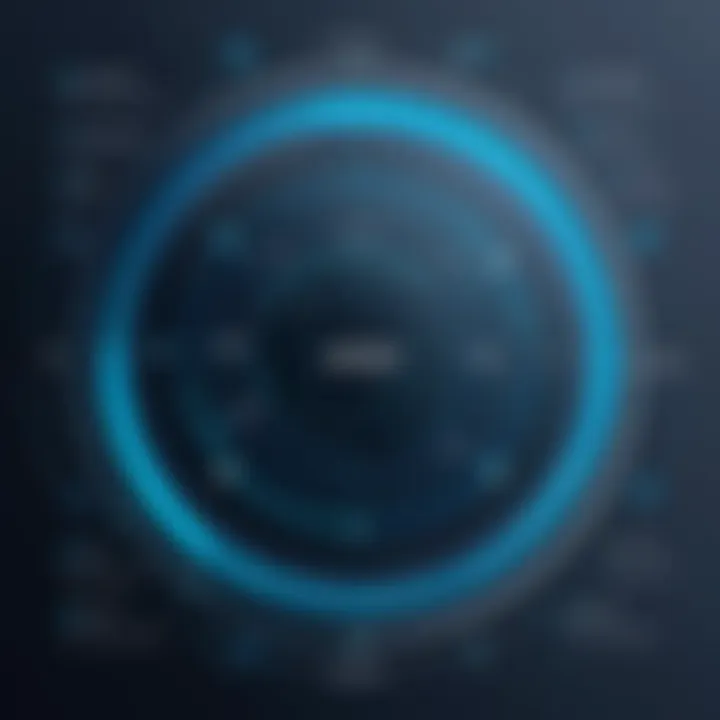
A closure is created when a function is defined inside another function. This allows the inner function to have access to the outer function's variables, even after the outer function has returned. In simpler terms, closures allow a function to "remember" its environment. Hereβs a basic example:
In this code, retains access to even after has finished executing. This is the essence of a closure β it allows for data from the outer function to be preserved in a way that is not accessible from the global scope.
Practical Applications of Closures
Closures have several practical applications in JavaScript programming. They are not merely theoretical constructs; they serve as powerful tools for solving real-world coding problems. Here are some common use cases:
- Data Privacy: Closures can be used to encapsulate variables and functions, preventing them from being accessed directly from the outside. This protects data and encapsulates functionality.
- Partial Application: Closures can create functions that are pre-configured with some parameters, enabling more flexible and reusable code.
- Memoization: This is a technique where the results of expensive function calls are cached, and closures help keep the state of these results.
- Event Handlers: When registering event handlers, closures can be used to maintain access to local variables, ensuring they remain available when the event is triggered.
- Asynchronous Programming: In callbacks, closures allow access to variables from their defining scope, making it easier to manage async function behavior.
In summary, closures are more than just a language feature. They enable advanced programming patterns, enhance code organization, and contribute to better memory management. Understanding how to use closures effectively can significantly improve your JavaScript programming.
Higher-Order Functions
Higher-order functions represent a key concept in JavaScript that significantly enhance the language's expressiveness and capability. They are functions that can accept other functions as arguments and also return functions as outputs. This makes them particularly valuable for creating powerful abstractions and enabling functional programming patterns. Understanding higher-order functions is essential for anyone looking to write efficient and maintainable JavaScript code.
What is a Higher-Order Function?
A higher-order function is a function that either takes one or more functions as arguments or returns a function as a result. This definition differentiates them from first-order functions, which do not deal with other functions directly. The flexibility offered by higher-order functions facilitates complex operations by allowing developers to define generic behavior that can operate on various conditions via callbacks.
For example, consider a function that filters an array based on a specific criterion; this can be a higher-order function because it takes another function to determine what that criterion is. This ability to pass functions around enables an entirely different level of abstraction that can simplify code and increase its readability.
Common Higher-Order Functions in JavaScript
In JavaScript, several built-in higher-order functions are widely used. Understanding these can greatly enhance programming efficiency:
- Array.prototype.map: This method creates a new array populated with the results of calling a provided function on every element in the calling array. It allows for clean, optimized transformation of data.
- Array.prototype.filter: This function creates a new array with all elements that pass the condition implemented by the provided function. It's key for filtering data based on dynamic criteria.
- Array.prototype.reduce: This method executes a reducer function on each element of the array, resulting in a single output value. It is useful for accumulating results from a series of operations.
- Array.prototype.every: This function tests whether all elements in the array pass the test implemented by the provided function, returning a Boolean value. It helps ensure that conditions are consistently met across the elements.
- Array.prototype.some: This function checks if at least one element in the array passes the test implemented by the provided function, also returning a Boolean value. It provides a convenient way to search within arrays.
The power of higher-order functions lies in their capability to be composed and combined, creating sophisticated functionality with less code and greater clarity. As you become more familiar with these concepts, the efficiency and simplicity of your code should improve, leading to better programming practices overall.
"Higher-order functions provide an elegant means of manipulating functions and abstractions, forming the core of functional programming in JavaScript."
Function Methods
Understanding function methods in JavaScript is crucial for effective programming. These methods offer a powerful way to manipulate the behavior of functions, enhancing flexibility and reusability. By grasping call, apply, and bind methods, programmers can optimize their code, making it more efficient and easier to maintain.
Function methods primarily assist in function invocation and context binding. These capabilities are essential, especially when dealing with objects and ensuring proper value of . Below, we explore each of these methods, their specific uses, and the advantages they bring to JavaScript programming.
Call, Apply, and Bind Methods
Call Method: The call method allows you to execute a function with a specified context and arguments passed individually. This can be especially useful when working with objects that require a specific context.
In this code, the function uses to access the property of the object.
Apply Method: The apply method is similar to call, but it expects arguments in an array format. This can be quite handy when you have an array of values that you want to pass as arguments to a function.
Here, takes for since does not use it, passing the array as arguments instead.
Bind Method: The bind method creates a new function that, when called, has its keyword set to the provided value, with a given sequence of arguments preceding any provided when the new function is called. This is particularly helpful when you need to fix the context of a function for future execution.
In this example, sets the context of to and initializes it with the first argument as . The resulting function can be called later with just the specified argument.
Benefits and Considerations
These methods are not just syntactic sugar; they provide clear benefits:
- Dynamic Context: You can dynamically change the context of function execution, enhancing modularity in code.
- Simplified Argument Passing: The apply method is particularly useful when working with large sets of arguments in array formats.
- Function Pre-configuration: The bind method allows creating function variations without losing access to the desired context.
However, it is essential to note that using these methods comes with a notational overhead. They require careful consideration of the function's context and its intended state.
Understanding function methods can lead to more maintainable and efficient code. Use them wisely to gain control over function invocation and behavior.
Error Handling in Functions
Error handling is a critical aspect of programming, especially in JavaScript. Functions are often the building blocks of a program, and when they encounter errors, it can lead to unexpected behavior and poor user experience. Understanding how to effectively handle errors helps ensure that your applications run smoothly.
In this section, we will discuss two main techniques for error handling: using try and catch statements, and creating custom error handling functions. These approaches provide a structured way to manage errors and maintain control over your code.
Using Try and Catch
The try and catch statement is a fundamental feature in JavaScript that allows developers to gracefully handle errors. When you encapsulate code that might throw an error in a try block, the catch block will execute if an error occurs. Hereβs a simple example:
In this code snippet, if throws an error, the catch block will catch it, and you can log or handle the error appropriately. This mechanism prevents the entire program from crashing and allows for a better user experience by providing informative feedback.
Error messages should be clear and helpful. When logging errors, include relevant details like what function failed and the input values. This can assist in debugging and improving the overall quality of your code.
Creating Custom Error Handling
While JavaScript's built-in error handling mechanisms are useful, sometimes you may need a more tailored approach. Creating custom error handling allows the programmer to define specific behaviors for different types of errors.
You can achieve this by defining your own error classes. For instance:
In this example, we define a class that extends the built-in class. When throws a , the catch block can distinguish it from standard errors using the error's name.
Custom error handling leads to clear and concise debugging. By providing specific error types, a developer can write catch blocks that handle different exceptions in unique ways. This results in a more robust and maintainable codebase.
Asynchronous Functions
Asynchronous functions are a critical part of modern JavaScript development. They allow for non-blocking operations, making it possible for code to execute while waiting for other processes to complete. This is especially important in web development, where user experience relies heavily on smooth and responsive interfaces. With the rise of complex applications, understanding asynchronous functions has become increasingly vital for developers.
Benefits of using asynchronous functions include improved performance and a better user experience. Instead of waiting for a function to finish running, the program can continue executing other tasks. This can significantly reduce load times and enhance interaction on web applications.
However, working with asynchronous functions introduces certain challenges. Properly managing their execution order is crucial. Poor handling can lead to issues like callback hell, where multiple nested callbacks make code difficult to read and maintain. To avoid this, JavaScript provides structures like Promises and Async/Await syntax, which reestructura the way asynchronous operations are performed.
Understanding these structures is essential for anyone looking to master JavaScript functions.
Understanding the Async/Await Syntax
The Async/Await syntax is a more intuitive way to work with asynchronous code. By using the keyword before a function definition, that function will always return a promise. This makes it easier to write and manage asynchronous code, making it look synchronous.
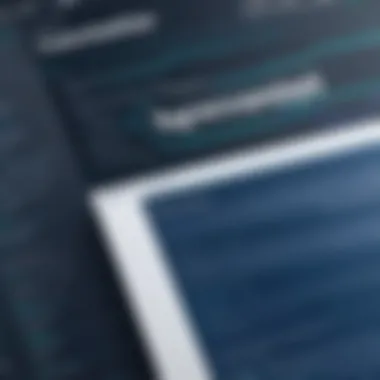
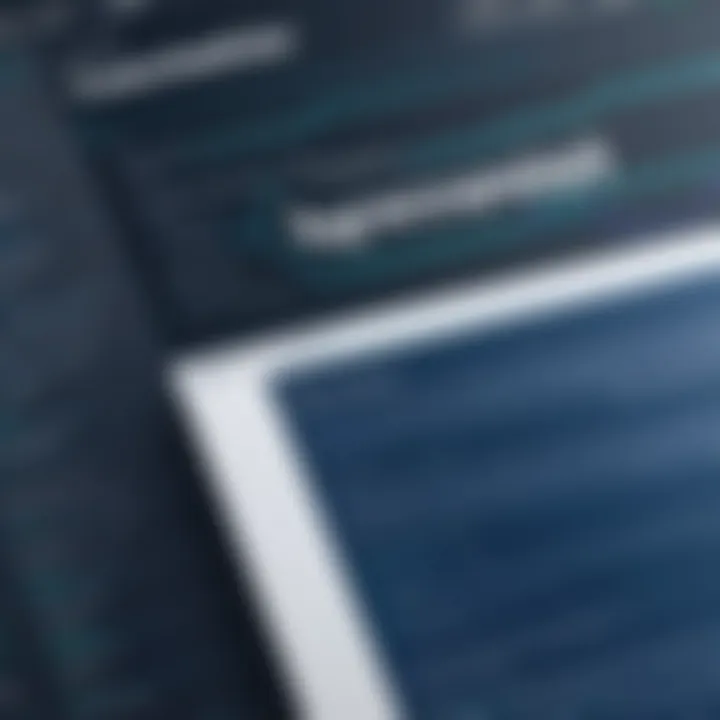
For example, consider the following code snippet:
In this example, the keyword pauses the execution of the function until the promise returned by is resolved. This ease of reading and writing makes the code cleaner and easier to debug. However, it is important to note that can only be used within an function.
Promises in Asynchronous Functions
Promises are a fundamental aspect of working with asynchronous functions. They represent a value that may be available now, or in the future, or never. Promises have three states: pending, fulfilled, or rejected.
Here is a brief breakdown of how promises work in JavaScript:
- Pending: Initial state, neither fulfilled nor rejected.
- Fulfilled: The operation completed successfully.
- Rejected: The operation failed, returning an error.
Using promises makes it easier to handle asynchronous operations. They allows chaining, meaning that multiple asynchronous calls can happen in a sequence. Consider this example:
In this case, if fetching the data fails, the error is caught in the block, allowing for better error management compared to traditional callback methods. This capability is essential for writing robust applications that can handle real-world user interaction.
Function Best Practices
In the realm of JavaScript programming, best practices for functions are crucial. They not only enhance the quality of your code but also facilitate easier debugging and maintenance. Implementing these practices can lead to improved performance and a more efficient code base, ultimately making your programming experience more gratifying.
Writing Clean Code
Clean code is a conversation among developers. It emphasizes clarity and simplicity in your functions, making them easier to read and understand. Here are some key elements for writing clean code:
- Descriptive Naming: Utilize clear and descriptive names for your functions. This aids in understanding their purpose at a glance. For example, instead of naming a function , consider .
- Function Length: Strive for brevity. A function should generally perform one task. Breaking complex functions into smaller ones increases readability and reusability.
- Consistent Style: Adhere to a consistent coding style. This includes indentation, bracket placement, and spacing.
By following these principles, your code not only becomes cleaner but also more maintainable. As a reminder, consider this guidance:
"Clean code communicates intention."
Optimizing Function Performance
Performance optimization in functions can significantly impact the efficiency of your applications. Here are some practices to consider:
- Avoid Unnecessary Computation: Always aim to minimize computations within a function. If a value does not change, compute it once and store it for future use.
- Use Looping Wisely: If a function involves looping through a collection, ensure that it is necessary and efficient. Consider methods like or that can provide cleaner and faster alternatives.
- Limit Scope: Keep variables and functions scoped as tightly as possible. This not only enhances performance but also minimizes the risk of naming collisions in larger applications.
Testing JavaScript Functions
Testing JavaScript functions is a significant aspect of programming that enhances software reliability and ensures the code performs as intended. Reliable functions minimize the risk of errors and improve overall project quality. They also simplify the maintenance process. When functions are tested rigorously, it becomes easier to isolate issues, understand code behavior, and confirm that changes do not break existing functionality.
There are various methods to test JavaScript functions. The two primary methods are unit testing and debugging. Each has its unique advantages and best practices. Unit testing enables developers to verify individual components, while effective debugging techniques help identify the root causes of any malfunctions in the code.
Unit Testing Functions
Unit testing represents a crucial practice in software development. It focuses on testing individual units of code, typically functions, to ensure they operate correctly. The importance of unit testing can't be overstated; it increases code reliability, facilitates changes, and serves as documentation that demonstrates intended function behavior.
To unit test a function, you'll generally use a testing framework like Jest or Mocha. These frameworks provide tools and structure to write and execute test cases efficiently. A unit test typically checks the outputs of a function for given inputs, verifying that the function behaves as expected.
Benefits of Unit Testing:
- Improves Code Quality: Ensures that each function works as intended before integration.
- Facilitates Refactoring: Safeguards changes, as tests indicate if existing functionality remains intact.
- Serves as Documentation: Offers clear examples of how functions should perform under various conditions.
To demonstrate a simple unit test, consider a function that adds two numbers:
A corresponding unit test could look like this:
In this example, the test checks that the function correctly calculates the sum of and .
Debugging Techniques
Debugging is another critical component in the testing process, focusing not just on identifying problems but also on resolving them. Effective debugging techniques can save considerable time and effort. By understanding the flow of code and how different functions interact, a programmer can address issues that unit tests may have missed.
Common debugging techniques include:
- Using Console Logs: This simple method involves outputting variables or messages at specific code points to observe behavior.
- Using Breakpoints: Many code editors allow breakpoints to be set, pausing execution to inspect variables and function calls in real-time.
- Step-Through Debugging: Involves executing code line-by-line to observe the sequence of execution and catch any deviations from expected behavior.
Effective debugging leads not only to clearer code but also to a deeper understanding of how functions operate within a larger system.
Debugging is not merely about fixing errors; it's also about understanding and improving code performance.
Through thoughtful unit testing and debugging, developers can ensure their JavaScript functions are robust, predictable, and ready for integration with larger applications.
Common Pitfalls in Function Usage
Understanding common pitfalls in function usage is crucial for successful programming in JavaScript. These mistakes can lead to bugs, unexpected behaviors, and a harder coding experience. Acknowledging potential issues allows developers to avoid them, ensuring code runs efficiently and effectively. Recognizing these pitfalls also aids in creating better practices for writing functions. This section discusses key mistakes and how to navigate through them for optimal function performance.
Mistakes to Avoid
- Not Understanding 'this' Context:
The value of 'this' can be quite confusing. Developers often have a hard time with what 'this' refers to in function scopes. This can lead to unexpected results, especially in callback functions. - Not Returning Values:
Functions that do not return a value when expected can result in undefined behavior. This oversight can complicate further operations in the code that depend on those values. - Overusing Global Variables:
Relying heavily on global variables can create namespace pollution. This increases the risk of accidental overwriting and bugs, resulting in less maintainable code. - Ignoring Function Dependencies:
A common mistake is not considering how functions depend on one another. This may lead to functions that fail when called out of the intended order. - Failing to Handle Errors:
Not including error handling in functions can make debugging difficult. Ignoring potential issues leads to larger problems down the road.
How to Overcome These Issues
To address the common pitfalls mentioned, specific strategies can help improve function usage.
- Be Clear About 'this':
Use Arrow Functions to ensure that 'this' is preserved in the context. Alternatively, use to explicitly define the value of 'this'. - Always Provide Return Statements:
If a function needs to return a value, ensure that the return statement is included. This practice avoids confusion later in the codebase. - Limit Global Variables:
Instead of using global variables, encapsulate variables within local function scopes. This decreases the risk of accidental overwriting. - Understand Function Dependencies:
Keep track of function dependencies. Use comments and documentation to clarify which functions rely on which ones. This practice prevents execution issues. - Implement Error Handling:
Use and blocks to handle potential errors in functions. This approach improves code stability and can help diagnose problems quickly.
"Mistakes are a part of the learning process in programming. By recognizing and addressing them, you grow as a developer."
By recognizing common pitfalls and implementing strategies to overcome them, programmers can enhance their function usage. Developing a strong understanding of these issues allows for more robust and maintainable code.
Closure
In this article, we have explored the multifaceted world of JavaScript functions. Understanding functions is crucial as they form the backbone of JavaScript programming. Functions enable code reuse, maintainability, and logical organization within your projects. The knowledge gained throughout this guide empowers you to create clean, efficient, and effective JavaScript code.
Recap of Key Concepts
To summarize, we covered several critical elements regarding functions in JavaScript. Key points include:
- Defining Functions: The syntax and differences between declarations, expressions, and immediate invokes were outlined.
- Types of Functions: Each function type, from named to arrow functions, was discussed with examples.
- Parameters and Arguments: We examined how to pass data to functions, including default values and the use of rest parameters.
- Return Values: The process of returning values and returning multiple values through arrays or objects was explained.
- Scope and Closures: Understanding the significance of variable scopes, global and local contexts, and the utility of closures enhances your ability to manage state.
- Higher-Order Functions: These functions that take other functions as inputs help create powerful and flexible code patterns.
- Error Handling: We looked at techniques like try-catch to manage exceptions and ensure robust code execution.
- Asynchronous Functions: The async/await syntax and promises were highlighted as essential tools for handling asynchronous operations.
- Best Practices: Emphasis was on writing clean code and optimizing function performance throughout all examples.
- Testing and Debugging: Techniques for testing and debugging functions were integral to ensure reliability.
This recap encapsulates the core concepts crucial for mastering JavaScript functions. By integrating these ideas into your programming practices, you can enhance the quality of your code.
Continuing Your JavaScript Journey
As you move forward in your programming career, integrating these concepts into your workflow is essential. Consider the following actions to further your skills:
- Experiment: Write various functions, practice different types, and understand how they interact.
- Build Projects: Apply what you have learned in real projects. This is a practical way to reinforce your knowledge.
- Join Communities: Engage with platforms like Reddit or Facebook to connect with other learners and share your insights and questions.
- Seek Resources: Keep exploring advanced topics and resources, such as the MDN web documentation and other educational platforms, to enrich your understanding.
Embrace the journey of learning JavaScript functions. The skills you develop will be fundamental in your evolution as a programmer.