Mastering Java Spring Boot: A Complete Learning Guide
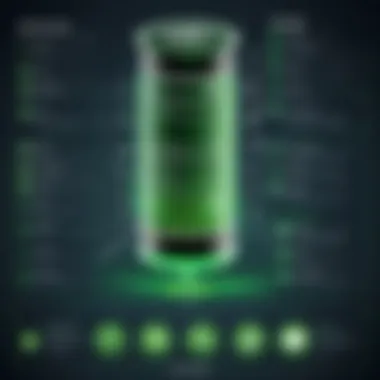
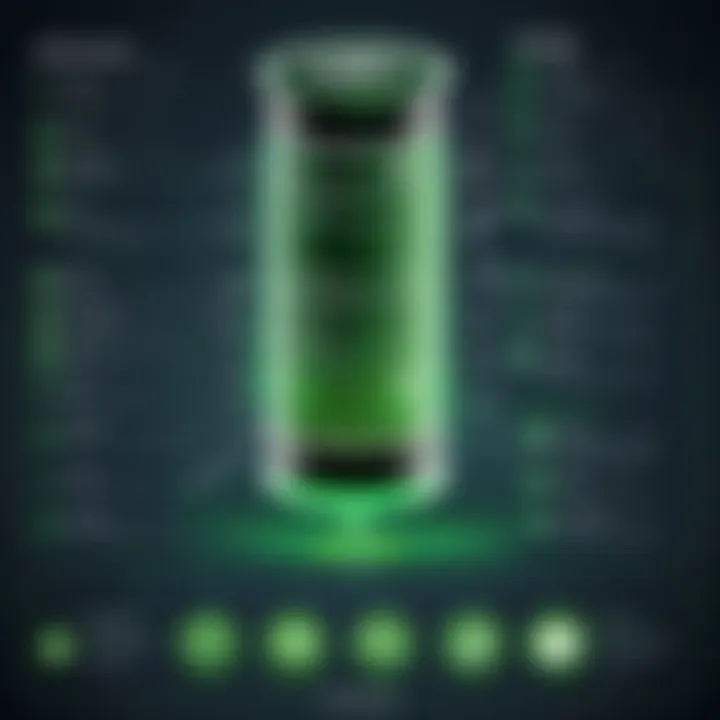
Intro
Java Spring Boot has emerged as a pivotal framework for building web applications with Java. Diving into this world opens doors to rapid application development and a variety of tools that streamline the process. This guide will unravel the essentials, practical applications, and best practices that will arm you with the know-how to harness the power of Spring Boot effectively.
Preface to Programming Language
History and Background
Understanding the roots of Java is essential for grasping Spring Boot. Java was developed by Sun Microsystems and released in 1995. Initially designed for interactive television, it evolved into a vital programming language used for a multitude of applications today.
Spring Framework, the foundation of Spring Boot, was introduced in 2003. It aimed to simplify enterprise Java development, allowing developers to create applications efficiently. The introduction of Spring Boot in 2014 made this process even more streamlined, providing a framework that requires minimal configuration.
Features and Uses
Spring Boot comes packed with features that cater to the modern developer's needs. Here are some standout features that are particularly appealing:
- Auto-Configuration: It automatically configures your application based on the dependencies you included, saving significant setup time.
- Standalone Applications: Spring Boot allows you to create stand-alone applications that run on their own, reducing overhead—no need for a traditional web server.
- Microservices Ready: Its structure lends itself well to microservices architecture, making it a popular choice for distributed systems.
- Production-Ready Features: Built-in metrics, health checks, and external configuration support make it a robust choice for production deployment.
Popularity and Scope
As developers strive for faster deployment and efficiency, Spring Boot's popularity has skyrocketed. According to various surveys, it ranks among the top frameworks for Java development. Its adaptability to varied domains—be it finance, healthcare, or e-commerce—makes it an invaluable tool in the developer's arsenal.
Basic Syntax and Concepts
Variables and Data Types
Understanding Java's syntax is your first step toward mastering Spring Boot. Variables are fundamental containers that store data and have distinct types. Here's a quick look:
- Primitive Types: These include int, double, char, and boolean, representing basic data without any added functionality.
- Reference Types: These include objects and arrays, which hold references to data, providing greater complexity.
Operators and Expressions
Operators are symbols that perform operations on variables and values. For instance, is used for addition, while checks if two values are equal. Expressions combine variables and operators to yield a result, like calculating the total price in an order processing application.
Control Structures
Control structures dictate the flow of a program. Java provides several:
- If-Else Statements: These help make decisions based on conditions.
- Loops: For/while loops allow you to perform repetitive tasks. They are essential for iterating over collections in Spring Boot.
Advanced Topics
Functions and Methods
In Java, methods define behaviors for objects. A method in a Spring Boot application could, for example, query a database or process user input. Structuring methods effectively helps maintain clean and manageable code.
Object-Oriented Programming
Java is an object-oriented language, underpinning most of the Spring framework. Concepts like inheritance, encapsulation, and polymorphism are central to structuring code logically.
Exception Handling
Like any other robust programming language, Java provides a mechanism for managing errors. Exception handling through try-catch blocks ensures your application remains stable and user-friendly, even when things go awry.
Hands-On Examples
Simple Programs
Creating a basic Spring Boot application can be an eye-opener. A simple “Hello, World!” application will get you started on understanding how components fit together.
Intermediate Projects
Developing a RESTful service to manage a library's inventory is an excellent project. You'll work with controllers, services, and repositories—key components of a Spring Boot application.
Code Snippets
Utilizing code snippets can accelerate your learning. Reusing proven pieces of code saves time and ensures efficient implementation of common tasks.
Resources and Further Learning
Recommended Books and Tutorials
Several resources can guide you through learning Spring Boot:
- Spring in Action by Craig Walls is a highly-regarded book for understanding Spring.
- Online tutorials from platforms like Codecademy and freeCodeCamp can provide practical exercises.
Online Courses and Platforms
Consider enrolling in courses offered on platforms like Coursera and Udemy, which often have specialist instructors walking you through complex topics in a digestible format.
Community Forums and Groups
Joining communities on platforms like Reddit or Facebook can be valuable. Engaging in discussions about challenges and solutions can enhance your understanding.
If you run into issues while coding, remember that both Stack Overflow and specialized forums can be lifesavers when the code refuses to cooperate.
With this foundational understanding, you're well on your way to mastering Java Spring Boot, a vital skill in today’s tech landscape. Keep practicing and don't hesitate to dive into projects and challenges. The best way to learn is by doing.
Preamble to Java Spring Boot
Java Spring Boot represents a major step forward in simplifying the world of Java application development. This chapter sets the stage for understanding not just what Spring Boot is, but why it has become a linchpin for programmers and businesses alike. As you navigate through this guide, keep in mind that the topics we will explore form a solid foundation for building robust applications, making your development process smoother and more efficient.
What is Java Spring Boot?
At its core, Java Spring Boot is an extension of the Spring framework aimed at simplifying the process of application setup and development. Rather than starting from scratch and dealing with the typical configuration headaches, Spring Boot allows developers to jump right into writing code. Think of it as the pre-assembled furniture of software development—the hard work of putting the pieces together is already done for you.
Spring Boot provides a range of defaults and auto-configuration options, making it particularly suited for creating stand-alone, production-grade Spring-based applications. Developers can rely on convention over configuration, which dramatically reduces the amount of boilerplate code. In practical terms, it means you can start a project with minimal fuss and focus on building functionalities that matter.
Benefits of Using Spring Boot
Using Spring Boot comes with a multitude of benefits that can enhance productivity and project outcomes:
- Rapid Development: Developers can spin up applications much faster, focusing on writing business logic rather than dealing with configuration.
- Microservice Ready: It integrates seamlessly with microservice architectures, allowing for distributed systems that can be easily scaled.
- Built-in Features: Spring Boot comes equipped with various tools and features like security, data access, and health checks, enabling developers to build comprehensive applications efficiently.
- Community Support: Given its popularity, there is a vast community and resource network for troubleshooting and implementing best practices.
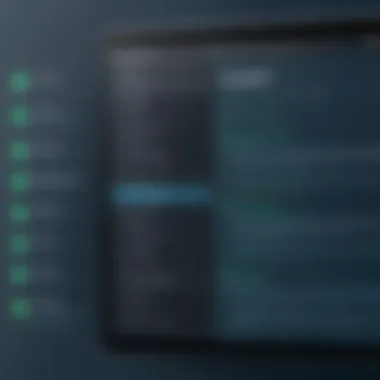
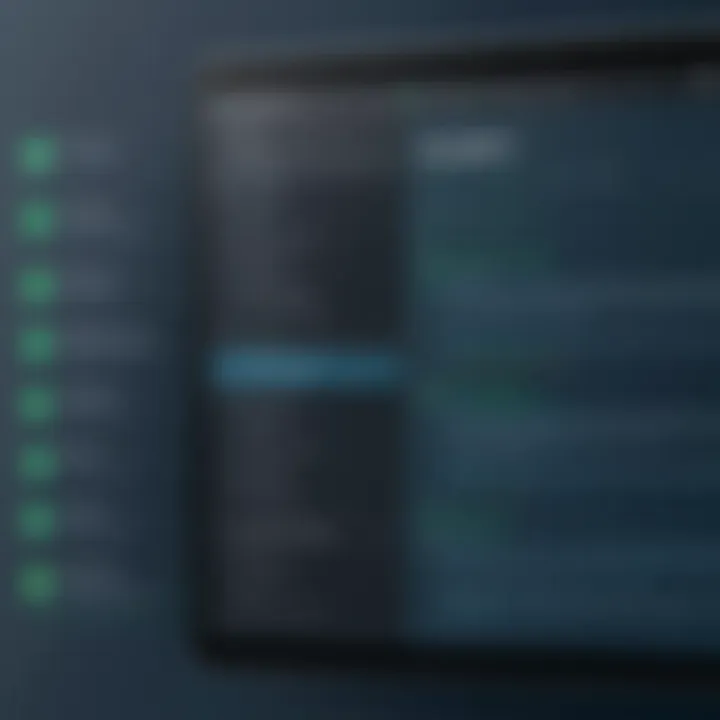
As a result, Spring Boot isn't just a tool—it's a methodology that shapes how you think about building applications.
Use Cases for Spring Boot
The versatility of Spring Boot makes it applicable in various scenarios. Here are a few common use cases:
- Web Applications: Quickly developing web applications or back-end services that require interaction with a front-end UI.
- RESTful APIs: Building robust API services to interact with other applications or services seamlessly.
- Microservices: Designing independent, modular components of larger systems with the ability to communicate effectively.
- Batch Processing: Running scheduled tasks or batch jobs that need to process data regularly or on-demand.
In essence, Spring Boot is the Swiss Army knife in a programmer's toolkit, ready to tackle various challenges with ease.
In summary, understanding the fundamentals of Java Spring Boot sets the groundwork for a successful venture into building applications. By embracing its core principles, you give yourself the tools to create scalable, flexible, and efficient applications.
Setting Up the Development Environment
Setting up the development environment is a foundational step in learning and utilizing Java Spring Boot effectively. A well-configured environment enhances productivity and allows for smoother development, debugging, and deployment processes. Without the right tools and configurations, coding can become frustrating and inefficient. Therefore, taking the time to set up your environment is key to a successful experience in Spring Boot.
Installing Java Development Kit
The Java Development Kit (JDK) is the backbone of any Java development process. To run Spring Boot applications, one must have an appropriate version of the JDK installed on their machine. Generally, developers choose either Oracle JDK or OpenJDK as their implementation.
- Download: First, navigate to the Oracle website or OpenJDK website, where you can find the latest version to download.
- Installation: Follow the installation steps relevant to your operating system. For Windows users, it's usually a matter of running the installer and following the prompts. For Linux, you might use package management commands.
- Check installation: After installation, open your command line interface and type:You should see the version details of the installed JDK, confirming it’s ready for use.
Setting Up an Integrated Development Environment
Integrated Development Environments (IDEs) are vital for enhancing the coding experience. They provide tools for editing, compiling, and debugging code, which can simplify the development process significantly. Two popular choices are Eclipse and IntelliJ IDEA.
Eclipse
Eclipse is a free and open-source IDE that has been around for years, making it a familiar choice for many developers. One of Eclipse's key characteristics is its extensive plugin ecosystem, which allows users to tailor their environments to fit their specific needs.
- Contribution: Eclipse’s rich set of features supports a wide variety of programming languages, and with the Spring Tools Suite (STS), it provides excellent support for Spring Boot development.
- Best for: Eclipse appeals to those preferring a more customizable interface, and its tight-knit community ensures there are plenty of resources available.
- Unique Features: The IDE's support for plugins is a unique feature, facilitating the addition of tools or frameworks as needed.
- Advantages/Disadvantages: While it’s customizable, some users find Eclipse to be a bit clunky compared to other IDEs, sometimes lagging in performance.
IntelliJ IDEA
IntelliJ IDEA, developed by JetBrains, is another powerful choice, known for its intelligent code assistance and productivity features. A key strength is its built-in support for frameworks like Spring.
- Contribution: This IDE excels at simplifying complex tasks, like auto-completion and instant navigation, which can save valuable time during development.
- Best for: Developers looking for a user-friendly interface will find IntelliJ intuitive. Many modern Java developers gravitate towards it due to its sleek design and advanced features.
- Unique Features: One standout feature is its integration with version control systems directly within the IDE, making teamwork much easier.
- Advantages/Disadvantages: Though it offers a free community version, the ultimate functionalities reside in the paid version, which might not suit everyone’s budget.
Configuring Maven or Gradle
To manage dependencies and build processes in Spring Boot applications, configuring a build tool is essential. Two of the most commonly used tools are Maven and Gradle.
- Maven: Known for its simplicity, Maven uses a particular XML file (pom.xml) to define the project structure and dependencies. It is easy to learn for beginners, allowing for straightforward dependency management.
- Gradle: On the other hand, Gradle uses a powerful Groovy-based DSL, offering a flexible scripting option for building projects. Its advantage lies in faster build times due to incremental builds, as it only reprocesses changes in a project.
To configure these tools
- If you opt for Maven, create a file at the project root with necessary dependencies.
- For Gradle, you’ll set up a file similarly, specifying all requisite dependencies in this script.
These tools streamline the project setup, ensuring all necessary libraries are included, making life easier for any developer as they dive into building their Spring Boot applications.
Fundamentals of Spring Boot
Understanding the fundamentals of Spring Boot is essential for anyone keen to harness the power of Java for practical applications. This section provides insight into the core elements that make Spring Boot a favored framework among developers. It paves the way for appreciating its ease of use, flexibility, and the robustness it brings to application development.
Understanding Spring Framework
The Spring Framework lays the groundwork upon which Spring Boot is built. It offers a comprehensive programming model that supports a wide range of enterprise applications. With its emphasis on modular architecture, developers can easily configure and extend functionalities.
One key aspect of the Spring Framework is Inversion of Control (IoC). This principle allows developers to delegate the control of object creation to the framework, making for cleaner, more maintainable code. As you embark on your journey with Spring Boot, grasping these foundational concepts is imperative. They ensure you can effectively work within the framework and leverage its capabilities to the fullest.
Key Components of Spring Boot
Spring Boot is packed with several components designed to simplify configuration and deployment. Here are two fundamental aspects:
Application Configuration
Application configuration in Spring Boot is a seamless affair, primarily due to the use of convention over configuration. This means that the framework follows standard practices, reducing the need for extensive configuration files. A notable characteristic is the application.properties or application.yml files, allowing developers to easily define application behavior with minimal effort.
A significant benefit of this approach is its ability to streamline project setup for developers. With predefined settings in place, you can focus on building features rather than getting lost in configurations. However, it's worth noting that too much reliance on defaults might lead to challenges when you need bespoke configurations for specific scenarios.
Dependency Injection
Dependency Injection (DI) is a cornerstone of Spring Boot's architecture, allowing for the automatic resolution of dependencies within your application components. With DI, you can declare dependencies, and the framework handles the instantiation. This promotes loose coupling and enhances testability.
The primary advantage of Dependency Injection is that it simplifies your application design. You no longer need to worry about the lifecycle of your objects; Spring manages this for you. However, it does add an initial layer of complexity, as you must understand how beans are registered and managed within the Spring container.
Building a Simple Spring Boot Application
Constructing a simple Spring Boot application provides a hands-on experience that solidifies your understanding of the framework’s capabilities. Start by ensuring you have the necessary development tools configured—an IDE like IntelliJ IDEA or Eclipse will serve you well. From there, you can utilize Spring Initializr to generate a basic project setup with your desired dependencies.
Next, create a simple REST controller. This setup serves as a foundational building block upon which you can expand. By grasping this process, not only do you become familiar with Spring Boot's essentials, but you also develop the skillsets needed to create more complex and feature-rich applications.
"The best way to learn is through building. With Spring Boot, your path is straightforward, making the learning experience rewarding."
In summary, understanding the fundamentals of Spring Boot equips you with critical knowledge that enhances your development experience. It sets the stage for deeper dives into areas such as data access, security, and microservices.
Creating RESTful Web Services
Creating RESTful web services is a cornerstone in today’s web architecture, especially when working with Java Spring Boot. In this section, we will explore the intricacies of developing RESTful APIs, how they integrate into your applications, and why embracing this approach is beneficial for modern software development.
Intro to REST Architecture
REST, or Representational State Transfer, is an architectural style that outlines how resources on the web should be defined and addressed. At its heart, REST uses standard HTTP methods, such as GET, POST, PUT, and DELETE. Each of these methods corresponds to standard operations that clients interact with on a web server.
In the realm of Spring Boot, RESTful architecture is not just a necessity but a strong approach to make your applications scalable and maintainable. Understanding the concepts of statelessness and resource representation is crucial. When designing RESTful services, it’s vital that each request from a client contains all the information the server needs to fulfill that request, creating a stateless interaction.
The beauty of REST lies in its simplicity and the way it aligns seamlessly with HTTP's natural functionality, making it the go-to choice for web services today.
Building a REST Controller
Crafting a RESTful web service in Spring Boot primarily revolves around the creation of REST controllers. These special classes are annotated with . They handle incoming requests and send back responses using JSON, which is widely accepted in web applications.
This simplistic structure offers a pathway to manage your data neatly. Each method corresponds to a specific HTTP operation, ensuring the services remain organized and maintainable. This encapsulation of business logic within controllers makes the application easier to manage and test.
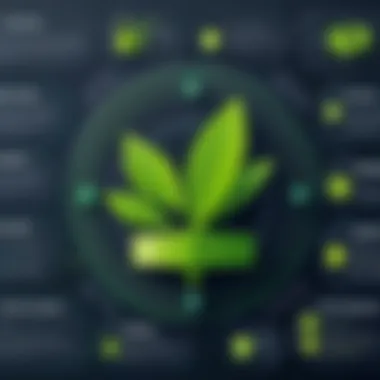
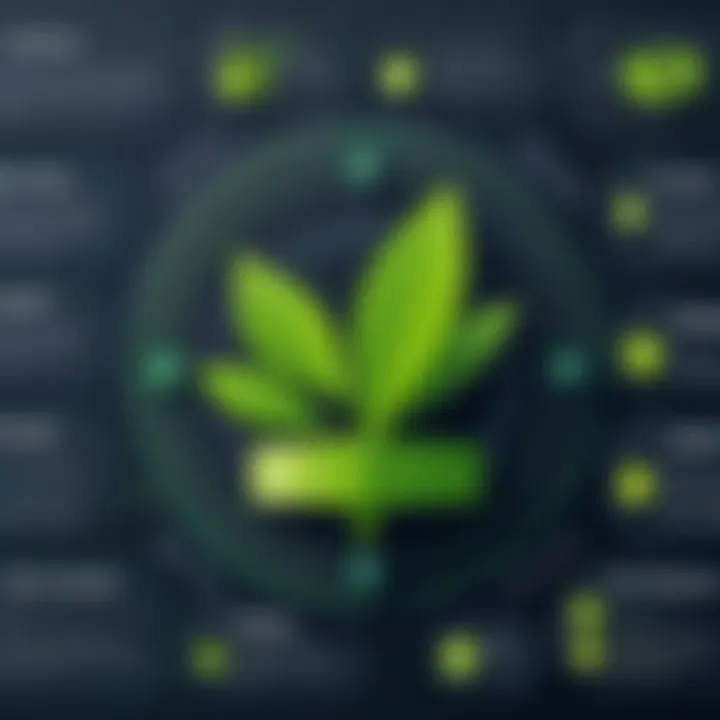
Understanding Request and Response Entities
In a RESTful service, understanding how request and response entities work is vital. When a client sends a request, it often sends a payload encapsulated as a JSON object. In Spring Boot, you can represent this payload as a Java object using to bind request data directly to a Java object. This allows for powerful data manipulation without cumbersome data handling processes.
When the server processes the request, it will return a response, usually in JSON format as well. This response can be customized to include status codes that indicate the success or failure of an operation. For instance, a successful resource retrieval may return a status, while a creation operation might yield a status. Additionally, sending back meaningful responses with the relevant data can greatly enhance client application interactions.
Data Access in Spring Boot
In the realm of application development, managing data effectively is key. Data Access in Spring Boot focuses on how applications connect to databases and manage data operations. It streamlines the process of working with data sources efficiently and allows developers to create robust applications that leverage powerful data handling capabilities. Spring Boot provides various features and frameworks that simplify database interactions. This section outlines the critical aspects of data access, including configuration, tools, and best practices necessary for successful data management in a Spring Boot application.
Configuring Database Connections
The first step in working with data in Spring Boot involves configuring the database connections. This configuration establishes how your application communicates with a database.
To set up a database connection, you typically define your database details in the or file. Here you provide essential parameters like the database URL, username, password, and driver class name. For instance:
This snippet configures a connection to a MySQL database. Keep in mind that the driver class may change depending on your database choice. Such configuration is crucial because it dictates how your Spring Boot application interacts with the underlying database.
Using Spring Data JPA
Spring Data JPA simplifies data access for Java applications. It provides easy integration with JPA (Java Persistence API) and streamlines the process of developing the data access layer. With JPA, you can focus on your application's business logic while handling the underlying database interactions without wrestling with boilerplate code.
When you use Spring Data JPA, you define repositories that extend interfaces like or . For example:
This interface allows CRUD operations on the entity and enables a custom query method based on last name. In a matter of minutes, you've created a fully functional data access layer with little effort.
Handling Database Operations
Once you've set up the database connection and data access layer, you'll need to handle various database operations. This includes tasks such as creating, reading, updating, and deleting records—often abbreviated as CRUD.
Using Spring Data JPA, these operations can typically be accomplished with a few method calls.
- Create: Use the repository's method to add a new record:
- Read: Retrieve records using methods like or specific queries:
- Update: To update an existing record, use the method again with the modified object:
- Delete: Remove a record by its ID with the method:
This process allows developers to maintain clean and efficient code while enabling comprehensive database operations, a testament to why data access is a cornerstone of effective application development with Spring Boot.
Important: Always ensure to apply appropriate error handling when dealing with database operations to manage exceptions gracefully, enhancing the application's robustness.
Spring Boot Security
In the world of application development, security isn’t just a nice-to-have; it’s a must-have. When building applications with Spring Boot, the security aspect becomes even more crucial as developers are tasked with safeguarding sensitive data, preventing unauthorized access, and ensuring the integrity of their applications. This section dives deep into Spring Boot Security, covering its essentials, implementation steps, and how to configure it effectively to keep your application safe.
Intro to Spring Security
Spring Security is a powerful and customizable authentication and access control framework for Java applications. It's not just about ensuring your application is secure; it's about providing developers with the flexibility and tools they need to authenticate users and authorize actions based on their roles.
The importance of Spring Security lies in its integration with the Spring ecosystem. It supports various authentication mechanisms such as basic authentication, form-based login, and OAut. With it, you can implement security best practices seamlessly, preserving your application’s integrity and protecting user data.
Spring Security facilitates the creation of robust security architectures, enabling developers to address authentication challenges without delving into complex, custom solutions.
Implementing Authentication
When it comes to implementing authentication in a Spring Boot application, the framework provides a straightforward way to secure your endpoints. The primary goal here is to verify user identities before allowing access to specific resources.
- Basic Authentication: This method encodes the user's credentials in base64. It's simple but not recommended for production systems unless used over HTTPS.
- Form-Based Login: This is the go-to for many applications. Users enter their credentials into a form, and Spring Security handles the authentication process. Configure it in your by overriding the method.Here's a sample snippet for form-based login:
- Token-Based Authentication: When building APIs, you might lean towards token-based methods. With JSON Web Tokens (JWTs), after a user logs in, they receive a token that should be sent with every subsequent request, allowing users to remain authenticated and reducing repetitive login prompts.
Implementing authentication is just the first step. Once users are authenticated, it's crucial to define what they can access.
Configuring Authorization Rules
Authorization in Spring Security deals with what authenticated users can do with their access. It's about setting the rules that dictate who can access specific parts of your application.
- Role-Based Access Control (RBAC): Assign roles to users and configure what each role can or cannot do. For instance, you could define admin users who can access everything while regular users have limited access.
- Method Security: In addition to URL-based security, Spring Security allows you to secure methods at a finer level using annotations:
Consider this for method security example in a service class:
By configuring authorization rules through Spring Security, you ensure that only users with sufficient privileges can access sensitive operations, effectively reducing the risk of unauthorized actions within your application.
In summary, understanding and implementing security in your Spring Boot applications is vital. By leveraging Spring Security, you can authenticate users and enforce strict authorization rules, making your applications robust against potential threats.
Advanced Spring Boot Features
Advanced Spring Boot features can significantly enhance the development process, making it a vital topic in understanding how to maximize the capabilities of this framework. These features allow developers to create applications that not only function well but can also adapt to changing needs and environments. Knowing how to leverage these advanced functionalities provides an edge in today’s fast-paced development world.
Using Spring Boot Actuator
Spring Boot Actuator is often regarded as a hidden gem in the Spring Boot ecosystem. It offers a suite of production-ready features that help in monitoring and managing applications. To put it simply, it’s like having a health check-up tool for your application.
With Actuator, you can expose various endpoints that provide essential information about your application’s status, metrics, and configuration. You can check how many requests your application is handling or even what the uptime looks like. This functionality is imperative for maintaining performance and ensuring that the application remains robust and reliable.
- Key Endpoints: Some key endpoints included are , which checks the health of your application, and , providing detailed metrics about your application.
To enable Actuator, simply add the dependency in your :
Wouldn't you agree that having the right tools at your fingertips makes all the difference?
Integrating Spring Boot with Messaging Services
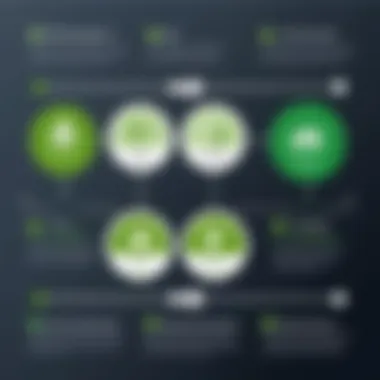
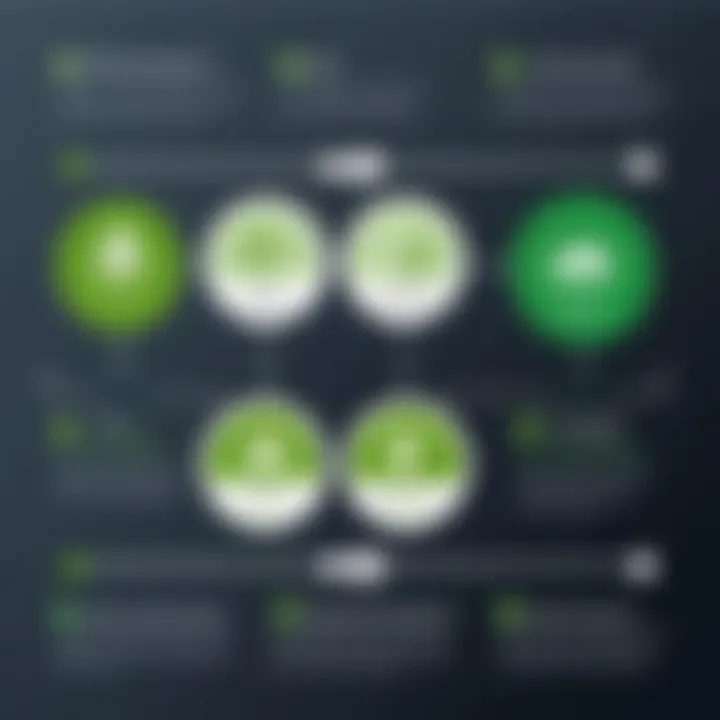
Messaging services are an integral part of modern applications, enabling communication between different parts of an application or between entirely separate systems. Integrating Spring Boot with messaging systems like RabbitMQ or Apache Kafka can open avenues for scalability and resilience, allowing applications to operate in a more asynchronous and event-driven manner.
This integration allows for applications to communicate seamlessly, thus removing the tight coupling between services.
- RabbitMQ can enhance your app's capabilities by handling various messaging needs, while Apache Kafka is excellent for high-throughput requirements.
Here's a basic example of a configuration for RabbitMQ:
When you adopt these messaging services, you're essentially future-proofing your application. Applications that communicate effectively are the ones that perform better in the long run.
Creating Microservices with Spring Boot
The migration towards microservices architecture is one of the defining trends in software development. Creating microservices with Spring Boot tremendously streamlines the process of breaking down applications into smaller, manageable services that can be developed, deployed, and maintained independently.
Spring Boot provides diverse features to facilitate microservices architecture. From simplified configuration to built-in support for RESTful services and security, it has your back from the ground up. Each microservice is self-contained yet can work harmoniously with the others through APIs. This modular approach can lead to enhanced agility and faster time-to-market.
Some notable characteristics of microservices in Spring Boot include:
- Independent Deployment: Each microservice can be deployed independently, helping with version control.
- Fault Isolation: If one microservice fails, it doesn’t bring down the entire system, unlike monolithic applications.
Taking the leap into microservices opens up your application to utilize resources more efficiently, allowing you to better respond to user demands.
Testing in Spring Boot
Testing is a cornerstone of any robust software development process, and Spring Boot is no exception. In the realm of Java Spring Boot, implementing effective testing strategies ensures the stability and reliability of applications. As we navigate through this section, the focus will center on understanding different testing frameworks, writing unit tests, and performing integration testing. Each of these components plays a vital role in safeguarding the code's functionality and enhancing the overall quality of software products.
Preamble to Testing Frameworks
Testing frameworks provide the necessary tools and structure to effectively write and manage tests. In the Spring Boot ecosystem, several frameworks stand out, each catering to different testing needs:
- JUnit: This is often the first framework that comes to mind when considering unit testing in Java. JUnit boasts a simple annotation-based structure which makes the writing of tests more intuitive.
- Mockito: This framework allows for mocking objects, which can be extremely useful when you want to isolate the class under test. It helps simulate the behavior of complex objects without needing their implementation.
Understanding how to integrate these frameworks into Spring Boot is essential. They enable developers to ensure that their application works as expected, both in isolation and in the broader context of the application.
Writing Unit Tests
Unit tests target the smallest testable parts of an application’s code, typically single methods or classes. The primary aim of unit testing is to validate each piece of the codebase independently. Here's how to approach writing unit tests in Spring Boot:
- Setting Up: First, make sure you have JUnit and Mockito dependencies included in your or file. Without these, you’ll find yourself at a dead end.
- Writing the Test Class: Create a separate test class for each class being tested. This will keep your tests organized and straightforward.
- Example of a Unit Test: Here’s a simple code snippet illustrating a basic unit test using JUnit and Mockito:
Writing concise, clear unit tests like these helps catch bugs early in the lifecycle, reducing the time and effort required for debugging later.
Integration Testing
Integration testing goes a step further, assessing how different modules or services work together. While unit tests examine the smallest parts of an application, integration tests ensure that these parts operate seamlessly in conjunction with each other. Here’s how to approach integration testing in Spring Boot:
- Spring Boot Test Annotation: Utilize which will bootstrap the entire context for the tests. This provides a great environment mimicking production settings.
- Testing with Real Data: Unlike unit tests which use mocks, integration tests often use real data. For instance, you might hit the actual database or call real services.
- Example of an Integration Test: Here's a simple example of how an integration test can be structured:
Integration tests are essential because they verify the connections and interactions between modules, thus ensuring that the application behaves as expected in a more holistic manner.
"Testing is not just a phase, it’s an integral part of the development. Leaving out testing is like building a house on sand."
The effort put into writing unit and integration tests can save time and resources down the line and contribute significantly to a successful project outcome.
Deployment Strategies
In the realm of application development, mastering deployment strategies is crucial for ensuring your Java Spring Boot applications run smoothly and efficiently in a production environment. Deployment is more than just moving code from one place to another; it's about preparing your application for its intended users and ensuring reliability, scalability, and security. Making informed decisions regarding your deployment can save you from a whole lot of headaches down the road.
Preparing Application for Production
Before you can release your Spring Boot application into the wild, you'll need to make sure it's set for production. This involves several steps to fine-tune performance and fortify security. Start by configuring the application properties for production settings, which could include setting the server port, enabling the appropriate logging, and adjusting database connections to point towards your production database. Don't forget to remove any test configurations and data that might expose sensitive information.
Performance tuning is also paramount in this phase. You might want to look into optimizing your database queries, refining your caching strategies, or even adjusting the JVM settings that can influence how your application performs. Just like writing the perfect script, every little detail counts when it comes to ensuring the end-user experience is top-notch.
Deployment Options in the Cloud
When it comes to deploying your Spring Boot applications, you have quite a buffet of options at your disposal. Cloud environments are particularly well-suited for Java applications due to their flexibility and scalability. Let’s break down two of the heavy hitters in the cloud space: AWS and Azure.
AWS
Amazon Web Services (AWS) has carved out a significant niche in the world of cloud computing. One of the many characteristics that make AWS a go-to choice is its comprehensive suite of services that cater to different needs. From Elastic Beanstalk, which simplifies deployment, to EC2 instances where you can have full control, AWS is versatile.
A unique feature of AWS is its ability to integrate seamlessly with other AWS services such as Amazon RDS for database solutions or S3 for storage. This ecosystem can significantly enhance the functionality of your Spring Boot applications. However, it’s worth noting that while AWS offers immense power, the learning curve can be steep, and cost management requires careful consideration.
Azure
On the flip side, Microsoft Azure also brings a robust set of tools and services tailored for deploying applications. One key characteristic of Azure is its tight integration with Microsoft products, making it a favorable choice, especially if you're already using tools like Visual Studio or Microsoft SQL Server.
Azure simplifies the deployment of Spring Boot applications through services like Azure App Service, which can automatically manage scaling based on user demand. This is particularly beneficial when you expect variable workloads. Yet, similar to AWS, navigating Azure’s pricing can be tricky if you aren't well-versed in its pricing structure.
Managing Applications with Docker
Containerization with Docker has become one of the preferred methods for deploying applications in recent years. Docker allows you to package your Spring Boot application along with its environment into containers, ensuring consistency across different environments.
This is especially handy when it comes to development and production parity. With the right Docker setup, what runs on your local machine will seamlessly run in production. Furthermore, managing application deployments with Docker enables easy scaling and resource allocation. The key here is to create Docker images that contain everything your application needs to function; this way, you are setting the stage for a smoother deployment process.
In summary, the world of deployment strategies for Java Spring Boot applications is rich with options and considerations. Each environment, tool, and technique offers unique advantages and potential pitfalls. By preparing for production, exploring cloud options like AWS and Azure, and leveraging Docker, you're paving the way for a successful and robust application rollout. Remember, getting it right upfront saves time, effort, and resources down the track.
Finale
As we wrap up this guide, it’s essential to reflect on the importance of mastering Java Spring Boot. This framework simplifies the development of robust applications, streamlining processes and reducing the time it takes to bring projects to life. With its extensive ecosystem, developers can achieve a remarkable amount with minimal effort, allowing for increased productivity. Understanding Spring Boot can act as a launchpad for advancing your programming skills, making you a valuable asset in the tech landscape.
Summarizing the Learning Journey
Throughout this comprehensive journey, we have explored various aspects of Java Spring Boot—from initial setup to deployment strategies. Key elements such as dependency injection, the intricacies of RESTful services, and security implementations have been covered. By delving into these crucial areas, learners have been equipped not just with theories but with practical skills as well.
In the modern tech environment, knowledge is power. Having a solid grasp on Spring Boot means you can adopt a versatile approach to software development. Whether you're aiming to build microservices or secure applications, the insights gained here will serve you well.
Next Steps for Aspiring Developers
After familiarizing yourself with the tenets of Spring Boot, what comes next? Here are a few potential paths you might consider:
- Hands-on Projects: Create your own projects to implement what you’ve learned. This could range from a simple REST API to a full-fledged application.
- Joining Communities: Engage with forums on platforms such as Reddit or Facebook. Sharing challenges and solutions will deepen your understanding and expose you to varied perspectives.
- Further Learning: Dive deeper into advanced topics, such as performance tuning, Spring Cloud, and integrating Spring Boot with other frameworks.
- Contributing to Open Source: Find Spring Boot projects on GitHub that interest you. Contributing can enhance not just your skills, but also your professional network.
In closing, embarking on the Spring Boot journey can be both rewarding and intellectually stimulating. Embrace the challenges and be willing to learn as you continue on your path toward becoming a proficient developer.