A Comprehensive Guide to Learning Java in Eclipse
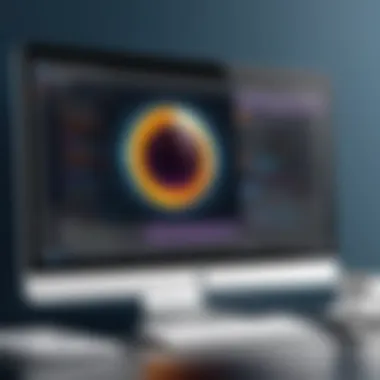
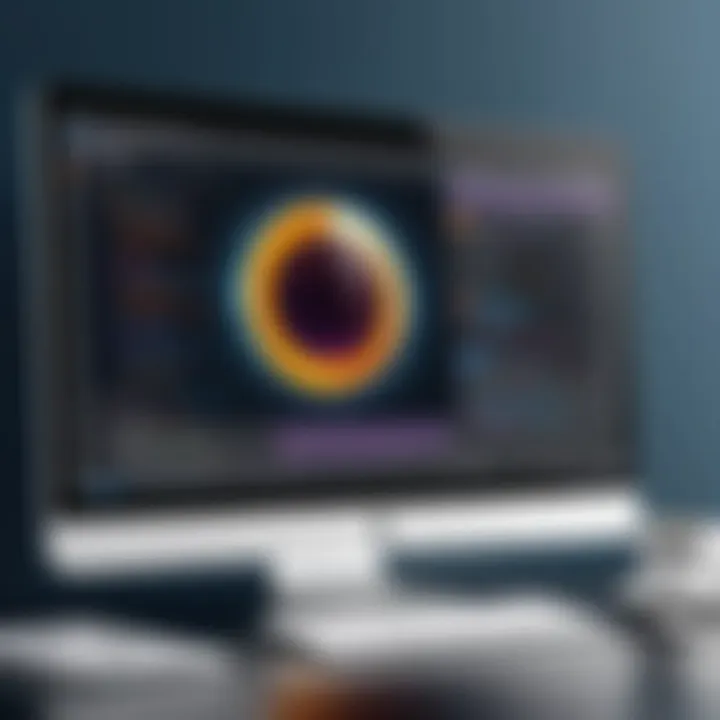
Intro
In recent years, learning Java has become a popular pursuit among aspiring programmers. This language, known for its versatility, is often used in web development, mobile applications, and large-scale enterprise solutions. Eclipse, as an integrated development environment (IDE), offers powerful tools to simplify the learning process and enhance productivity.
This guide serves not only to ease the transition into programming but also to highlight the features of Eclipse that cater specifically to Java. Whether you are a complete novice or aiming to deepen your existing understanding of Java, this resource will provide valuable insights into the essentials of the language and the IDE.
Prelude to Programming Language
History and Background
Java was developed by Sun Microsystems and released in the mid-1990s. Initially designed for interactive television, it soon found its way into other applications due to its flexibility and ease of use. Over time, Java has maintained its relevance through continuous updates and enhancements. The language promotes a principle known as "write once, run anywhere," which means that code compiled on one platform can run on any other platform that supports Java, making it exceptionally versatile.
Features and Uses
Java is characterized by several prominent features:
- Object-oriented: Supports concepts like inheritance, encapsulation, and polymorphism.
- Platform-independent: Code can run on any device with the Java Virtual Machine (JVM).
- Robustness: Strong memory management and error handling mechanisms.
- Security: Built-in security features make it a good choice for networked applications.
These characteristics have made Java a preferred language for developing web applications, mobile applications (especially Android), and server-side technologies.
Popularity and Scope
As of today, Java continues to be one of the most popular programming languages. Its extensive use in enterprise solutions and Android development showcases its widespread appeal. The community surrounding Java is large and active, ensuring continuous learning and support for both new and experienced developers. Sites like reddit.com are great platforms to engage with other learners and professionals.
Basic Syntax and Concepts
Variables and Data Types
Understanding variables is fundamental to any programming language. In Java, variables are containers for storing data values. The types of data you can store in Java include:
- int: For integers
- double: For decimal numbers
- boolean: For true/false values
- String: For text
Example of declaring variables:
Operators and Expressions
Operators in Java allow you to perform operations on variables and values. Common operators include:
- Arithmetic Operators: +, -, *, /, %
- Relational Operators: ==, !=, >, , >=, =
- Logical Operators: &&, ||, !
Expressions combine these operators with variables and constants to produce new values.
Control Structures
Control structures dictate the flow of the program. Key control structures in Java are:
- If-else Statements: For conditional branching.
- Switch Statements: For multi-way branching.
- For Loops: To iterate over a sequence.
- While Loops: To execute a block of code as long as a condition is true.
Advanced Topics
Functions and Methods
Functions, or methods in Java, allow the programmer to encapsulate logic for reuse. A method typically includes parameters and a return type, helping to organize complex programs.
Object-Oriented Programming
Java fully supports Object-Oriented Programming (OOP) principles. You can create classes and objects to represent real-world entities. It allows for code reuse and better organization.
Exception Handling
Java provides robust mechanisms for handling errors and exceptions. Using try-catch blocks allows developers to manage exceptions gracefully, ensuring the program can recover or provide meaningful error messages to the user.
Hands-On Examples
Simple Programs
Starting with simple "Hello, World!" programs is a common practice. This type of program is often the first one written when learning a new language. For the purpose of learning, understanding the structure of a simple program sets a solid foundation.
Intermediate Projects
As learners become comfortable with Java, they can move to projects that involve more complex logic, such as a basic calculator or a simple banking system.
Code Snippets
Including small snippets can be helpful for quick reference and practice. For instance, a snippet to calculate the factorial of a number can reinforce concepts of loops and functions.
Resources and Further Learning
Recommended Books and Tutorials
Books such as "Effective Java" by Joshua Bloch or online tutorials on platforms like britannica.com are excellent to deepen understanding.
Online Courses and Platforms
Given their structured nature, platforms such as Coursera and Udemy offer courses tailored for Java learners.
Community Forums and Groups
Joining user groups on Facebook or technology forums can enhance the learning experience. Engaging with a community provides additional support and momentum.
Foreword to Java and Eclipse
Understanding the importance of Java and Eclipse as tools for programming is essential for any aspiring developer. Java, being one of the most popular programming languages, has a global reach that is unmatched. From web applications to mobile apps, Java's versatility makes it a preferred choice across industries. It serves not only as a foundation for learning programming concepts but also as a gateway to various advanced technologies.
Eclipse, on the other hand, is a powerful integrated development environment (IDE) that enhances the Java programming experience. It combines robust features with an intuitive interface, which is crucial when learning to code. Eclipse simplifies tasks like code writing, debugging, and project management, making it easier for beginners to grasp fundamental concepts swiftly.
Overview of Java Programming Language
Java is an object-oriented programming language that emphasizes code readability and maintainability. It was first released by Sun Microsystems in 1995 and has since grown to be foundational in various software development processes. Its syntax, derived from C and C++, is structured enough to allow for clear understanding while being flexible enough for advanced programmers to innovate.
The primary characteristics of Java include platform independence, which means code can run anywhere, anytime, and on any device due to the Java Virtual Machine. Moreover, it boasts a robust memory management system that helps prevent resource leaks and errors. Java supports multithreading, allowing simultaneous execution of tasks, which enhances application performance. Java also has a rich set of libraries and frameworks, providing a wealth of tools to streamline development.
Why Choose Eclipse as Your IDE
Choosing the right IDE can profoundly affect the learning experience of Java. Eclipse stands out for many reasons.
- User-friendly Interface: Eclipse has an appealing user interface that allows users to focus on coding without being overwhelmed. Menus and panels are intuitively organized.
- Plugin Support: The availability of a plethora of plugins makes extending Eclipse’s functionalities straightforward. This adaptability allows for customization based on project requirements.
- Integrated Debugger: Debugging is a crucial part of programming, and Eclipse’s integrated debugging tools enable users to identify and rectify issues more effectively.
- Version Control Integration: Eclipse makes it easier to work on larger projects by allowing integration with version control systems like Git. This enhances collaboration among team members.
- Comprehensive Community Support: The Eclipse community is vast. Users can find resources, tutorials, and forums which provide assistance and foster a collaborative learning environment.
"Eclipse empowers developers to focus on the code while managing the underlying complexity seamlessly."
By understanding Java's capabilities along with the advantages of using Eclipse, learners can effectively start their programming journey with a solid foundation.
Installing Eclipse for Java Development
The process of installing Eclipse for Java development is a critical step in the learning curve for any aspiring programmer. Eclipse, being a popular integrated development environment (IDE), provides a powerful platform for writing, debugging, and managing Java projects efficiently. This section will explore the vital considerations, benefits, and necessary steps for successfully installing Eclipse.
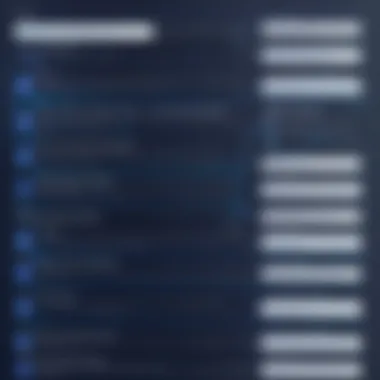
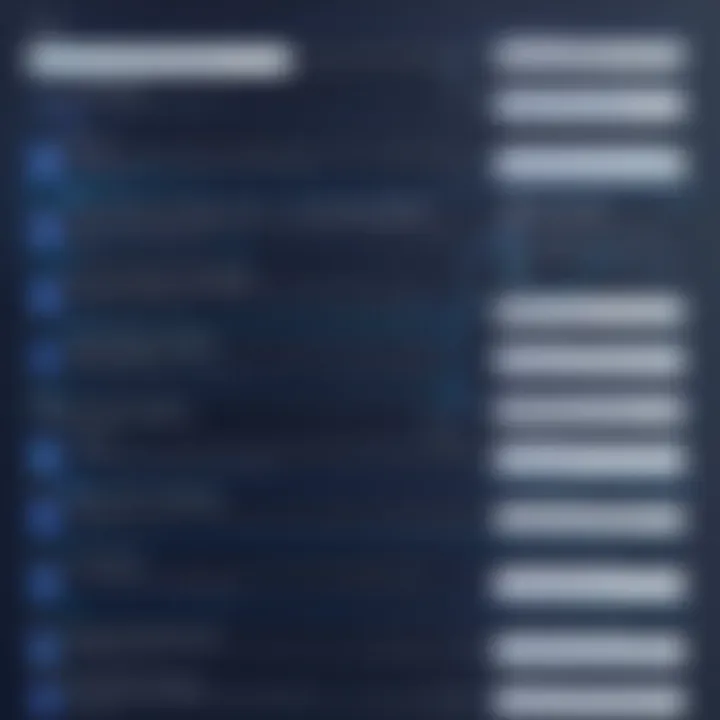
System Requirements for Eclipse
Before diving into installation, it is essential to know the system requirements to ensure that Eclipse runs smoothly. Here are the key requirements:
- Operating System: Eclipse is compatible with Windows, macOS, and Linux. Ensure you have a supported version of these operating systems.
- Java Development Kit (JDK): A suitable version of JDK must be installed on your system. Eclipse generally requires JDK version 8 or higher. The installation of JRE alone may not be sufficient for development purposes.
- Memory and Disk Space: A minimum of 4 GB RAM is advised for optimal performance. Also, ensure that you have at least 1 GB of free disk space available to accommodate the IDE and additional plugins.
Having compatible hardware and software ensures that the development experience is stable and efficient.
Steps to Download and Install Eclipse
Downloading and installing Eclipse isn't overly complicated but requires precision in following the right steps to avoid issues. Below are the detailed steps:
- Visit the Official Website: Navigate to the official Eclipse website at eclipse.org.
- Choose the Right Package: Look for the "Eclipse IDE for Java Developers" package. This version includes essential tools specifically for Java development.
- Download the Installer: Select the installer that corresponds with your operating system. This will typically be a compressed file.
- Run the Installer: Once downloaded, extract the files and run the installer. Follow the installation wizard prompts to complete the process.
- Start Eclipse: After installation, open Eclipse. You will be prompted to select a workspace, which is the directory where your projects will be saved.
By following these steps, you will ensure a proper installation of Eclipse, setting a solid foundation for your programming endeavors.
Configuring Eclipse for Java Projects
Once Eclipse is installed, configuration is the next step to tailor it for Java development. Proper configuration enhances the efficiency of your coding environment. Here’s how to configure Eclipse for Java projects:
- Install Java Development Kit: If not already done, install the Java Development Kit and ensure that Eclipse recognizes it by navigating to .
- Setting Up User Libraries: Create user libraries for any external JAR files you may need for your projects by going to . This makes such libraries readily available for import into your projects.
- Customizing the IDE: Adjust themes, font sizes, and other UI elements in for a better visual experience.
- Configuring Project Settings: Create a new Java project by going to . Specify your project details and choose the correct Java version.
Starting with the right configurations can spare you frustrations down the line, allowing you to focus on building your programming skills effectively.
Overall, the installation and configuration of Eclipse are not just logistical steps; they form the bedrock of your Java programming journey. Appropriately setting up your development environment will streamline your coding, enhance productivity, and provide you with a robust platform to learn and grow as a programmer.
Exploring Eclipse Interface
Understanding the Eclipse interface is crucial for anyone who seeks to master Java programming. The design and layout of an Integrated Development Environment (IDE) like Eclipse can significantly impact productivity. It allows developers to focus more on coding and less on navigating through tools and functionalities. By getting familiar with the various components within Eclipse, learners can streamline their workflow and enhance their coding efficiency.
Understanding the Workspace
The workspace is the primary environment in which developers will conduct their activities. In Eclipse, the workspace contains all your projects and their configurations. Understanding how to effectively manage your workspace is a vital skill. You can set it up to suit your workflow, and this will pay off in terms of organization and productivity.
A workspace is similar to a file cabinet. In this case, each project is a drawer, containing files such as Java class files, images, or configuration settings. When you create a new Java project in Eclipse, it will associate that project with your selected workspace.
- Create and Switch Workspaces: You can easily create multiple workspaces and switch between them. This feature is useful if you're working on various projects or need different setups.
- Managing Resources: The workspace lets you manage resources like libraries, JAR files, and configuration settings. This management is crucial when dealing with large projects.
Navigating the Project Explorer
The Project Explorer is an essential part of the Eclipse interface. It provides a hierarchical view of your projects, making it easier to navigate through files and directories. Familiarity with Project Explorer enhances efficiency, as it allows for quick access to project elements.
You can view various components related to Java projects here, such as:
- Source Code: Access your Java source files quickly. You can easily open, edit, or delete them.
- Resources: Images, config files, and libraries can also be visualized and managed from this panel.
- Build Path Information: You can manage the build path for your project, ensuring that all necessary libraries are linked correctly.
It is beneficial to keep this panel organized. You can right-click on items for options such as creating new folders or files, renaming, or refactoring existing elements.
Using the Editor and Console
Eclipse comes with a powerful code editor that supports Java development and provides various features. The editor facilitates various tasks such as code writing, debugging, and testing Java applications.
- Code Completion: One notable feature is content assist, which offers suggestions while coding. It can significantly speed up the development process by reducing typographical errors and suggesting methods or classes.
- Syntax Highlighting: The editor also highlights syntax. This makes it easier to read and understand code, helping to spot errors early in coding.
The console is where you can view output and error messages generated by your Java programs. Understanding the console is vital for troubleshooting code. It provides immediate feedback about how your code is running and points out any errors or exceptions that may arise during execution.
"The Eclipse console will help you debug your Java applications by showing real-time error messages and outputs."
Using these tools effectively will significantly enhance your coding experience in Eclipse. By mastering the Eclipse interface, you create a conducive environment for learning and efficiency in Java programming.
Creating Your First Java Project
Creating your first Java project is a significant step in your programming journey with Eclipse. It lays the groundwork for understanding how to structure a Java application, manage files, and utilize the Eclipse environment effectively. A well-organized project enhances your coding experience and makes coding more efficient. This section will explore the crucial elements of setting up a project, writing your first program, and how to compile and run it. Following these steps will boost your confidence and solidify your skills.
Setting Up a New Project
To get started with your new Java project in Eclipse, you will first need to set up the project. Here’s how you can do it:
- Open Eclipse IDE: Launch the Eclipse application you installed earlier.
- Select Workspace: A workspace is a directory that holds your projects. Choose the desired location or use the default one.
- Create New Java Project: Go to > > .
- Name Your Project: In the dialog box that appears, enter a meaningful name for your project. This name should reflect the purpose of the project.
- Configure Project Settings: You can set up the project structure, choose the Java version, and configure libraries if necessary. Ensure that your setup matches the needs of your upcoming tasks.
- Finish Setup: Click on the button. Eclipse will create the project and display it in the Project Explorer, ready for you to begin coding.
Setting up a project is straightforward, but it’s essential to keep in mind the organization of files and packages as you progress.
Writing Your First Java Program
After successfully setting up your project, it is time to write your first Java program. This involves creating a new Java class with a method, which serves as the entry point of any Java application. To write your program:
- Create a New Class: Right-click on the folder of your project, then select > .
- Name the Class: Enter a class name, such as . It is standard practice to start class names with an uppercase letter.
- Add the Method: Check the box that says to generate the main method automatically. This method must be present for the program to run.
- Write Your Code: In the newly created class, you can now write your Java code. A simple program that prints "Hello, World!" looks like this:
- Save Your Work: Save your file by clicking on the menu and then , or simply use the shortcut Ctrl + S.
This simple program is the foundation of your first Java application, showing how to print output to the console.
Compiling and Running the Program
The final step to complete your project is to compile and run it. The Eclipse IDE streamlines this process significantly. To execute your Java program, follow these steps:
- Compile Your Program: Eclipse compiles the Java code automatically when saving. If there are no syntax errors, your program should compile successfully.
- Run the Program: To run your program, right-click on your Java class (HelloWorld.java) in the Project Explorer.
- Select Run As: Choose > .
- View Output: The program’s output will display in the console at the bottom of the Eclipse window.
By following these steps, you have successfully created, written, and run your first Java program in Eclipse.
The successful execution of this program signifies your entry into Java programming. The feeling of seeing your printed message, no matter how simple, is a small yet vital achievement on your learning journey. Embrace this moment, as it marks the beginning of your exploration into Java and programming at large.
Java Fundamentals in Eclipse
Understanding Java fundamentals is essential for anyone delving into the world of programming using Eclipse. This section emphasizes the core principles that underpin Java, making it easier to grasp more advanced concepts. With a strong foundation in fundamentals, learners can write efficient, maintainable code and develop complex applications successfully.
Data Types and Variables
In Java, data types are the building blocks for defining the nature of the variables. Each variable can store data of a specific type. Java is statically typed, which means the type of a variable must be declared before it is used. Among the common data types are:
- int: Represents integer values.
- double: Used for floating-point numbers.
- char: Stores a single character.
- boolean: Represents true or false values.
Variables act as containers for holding data. For example, to define a variable that holds an integer value, the code would look like this:
Understanding how to declare and use variables is vital for all Java programs. It allows for effective data management and manipulation, which is an integral part of programming in Eclipse.
Control Structures: Loops and Conditionals
Control structures govern the flow of execution in a program. In Java, there are primarily two types of control structures: conditionals and loops.
- Conditionals: These allow the program to make decisions based on certain conditions. Common structures include , , and statements.
- Loops: Loops enable repetitive execution of a block of code as long as a specified condition is met. The main types of loops are , , and .
For example, a simple statement would look like this:
Control structures are essential for creating dynamic behavior in Java applications. By mastering these concepts, learners can write more complex and interactive programs that respond to various inputs.
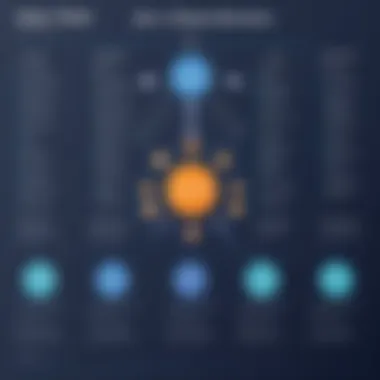
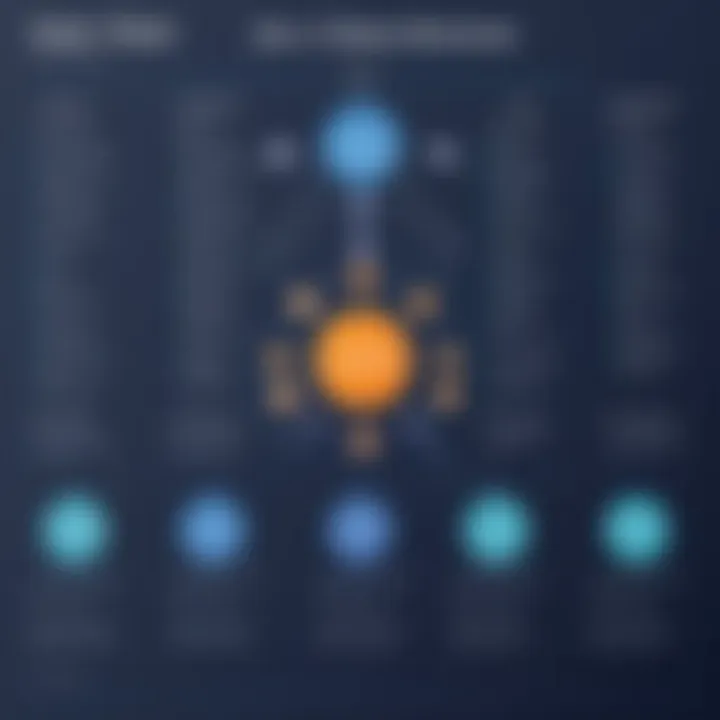
Methods and Functions
Methods are blocks of code that perform specific tasks. They are crucial for organizing code, making it modular and reusable. Each method can take parameters and return a result. Defining a method starts with the , followed by the method name and parentheses for parameters. Here’s a basic example:
This method takes two integers and returns their sum. Functions encapsulate behavior, enabling better structure and cleaner code.
Mastering the basics ensures a smooth learning curve when exploring advanced topics in Java programming.
Object-Oriented Programming Concepts
Object-oriented programming (OOP) is a fundamental programming paradigm that enables developers to structure software in a more intuitive and efficient manner. This section focuses on the important principles behind OOP, specifically how they apply to Java development in the Eclipse IDE. Understanding these concepts will allow you to manage complexity in your code, leading to reusable and maintainable programs.
The core concepts of OOP are classes and objects, inheritance, polymorphism, encapsulation, and abstraction. Each of these concepts provides unique benefits that contribute to a robust coding experience.
Defining Classes and Objects
In Java, a class is a blueprint for creating objects. It defines properties, often called attributes, and methods, which are the behaviors of those attributes. An object is an instance of a class. Consider the following example:
In this example, the class contains attributes like color and model, and a method that allows the car to perform an action. To create an object from the class, you would write:
This simplicity in defining and using classes and objects allows for organized code. It encourages modular programming, making it easier to manage various aspects of your application independently.
Inheritance and Polymorphism
Inheritance allows a new class to inherit the properties and methods of an existing class. This helps avoid redundancy and promotes code reusability. For example, if you have a class , you could create a class that inherits from it:
In this scenario, inherits the method from . When you create an instance of , it can call both and methods, illustrating the power of inheritance.
Polymorphism refers to the ability of a single function or method to act in different ways depending on the object type. In Java, polymorphism occurs with method overloading and overriding. In the case of overriding, a subclass can provide a specific implementation of a method that is already defined in its superclass, allowing for specific behavior tailored to the subclass.
Encapsulation and Abstraction
Encapsulation is the practice of restricting access to certain details of an object and exposing only what is necessary. This is typically achieved using access modifiers, such as , , and . By limiting access, you lay the groundwork for a more secure and manageable codebase. Consider the following example:
In this example, the attribute is private, so it cannot be changed directly from outside the class. Users interact with and methods, ensuring that balance is managed in a controlled way.
Abstraction involves simplifying complex reality by modeling classes based on the essential properties and behaviors relevant to the problem at hand, while hiding the details that are not necessary for understanding. Abstraction allows you to focus on high-level functionalities without getting bogged down by implementation complexities.
"Abstraction is an essential pillar of OOP, enabling developers to define interfaces and methods that can be used without knowledge of the underlying implementation."
In summary, mastering object-oriented programming concepts is crucial for effective Java programming in Eclipse. These principles not only aid in developing complex applications but also enhance maintainability and readability in your code.
Java Libraries and Frameworks in Eclipse
Java libraries and frameworks play a crucial role in enhancing the development experience within the Eclipse integrated development environment (IDE). Understanding how to utilize these resources can significantly elevate your programming efficiency. Libraries provide a set of pre-written code that developers can use to perform common tasks quickly, avoiding the need to write every line from scratch. Frameworks, on the other hand, offer a structure that guides the development process, ensuring best practices are followed while allowing for customization and scalability.
The use of libraries and frameworks in Eclipse can lead to improved code maintainability and reusability. By incorporating libraries, developers can leverage existing solutions for common problems, which can save time and reduce the likelihood of errors. When working with frameworks, developers can benefit from established design patterns, which can enhance the organization of code and facilitate easier collaboration among team members.
However, working with libraries and frameworks requires some consideration. Choosing the right library or framework is essential, as compatibility issues may arise. Developers need to ensure that their selected resources are up-to-date and well-supported. Additionally, it is important to understand the learning curve associated with more complex frameworks, as they may introduce additional concepts that are not found in a simpler coding environment. With these elements in mind, the benefits of using libraries and frameworks in Eclipse can far outweigh the considerations.
Using External Libraries
Incorporating external libraries into your Eclipse projects is an advantageous practice that can significantly save development time. External libraries are reusable pieces of software that simplify complex tasks in programming. For example, libraries like Apache Commons or Google Guava provide essential utilities that streamline operations, improving overall productivity.
To add an external library to your project in Eclipse, follow these steps:
- Download the library from a reliable source. Make sure it is the right version.
- In Eclipse, right-click on your project in the Project Explorer and select 'Build Path'.
- Choose 'Configure Build Path'.
- In the Libraries tab, click 'Add External JARs'. Choose the downloaded library files.
- Click 'Apply and Close'.
By integrating external libraries, you will find that common tasks, such as file handling or data manipulation, become simpler. Always test the libraries you incorporate to ensure they meet your project's needs.
Integrating Frameworks with Eclipse
Frameworks are foundational to software design, offering a robust environment that supports application development. In the context of Java, frameworks like Spring and Hibernate are commonly used to facilitate more organized and efficient coding practices.
To integrate a framework in Eclipse, you usually need a little more setup than with external libraries, as most frameworks require configuration. Generally, you can integrate a framework by:
- Adding the framework’s JAR files similarly to external libraries using the project’s build path settings.
- Using Maven or Gradle, which are widely used management tools for adding libraries and ensuring all dependencies are correctly set. These tools automatically handle versioning and allow for easier updates.
After integration, you can benefit from the structured environments that frameworks provide. Creating a project using Spring, for instance, can ease dependency management and make code cleaner.
It is significant to note that while frameworks can greatly aid development, they can also introduce complexity. Understanding the framework's architecture and lifecycle is essential for leveraging its full potential.
Utilizing Java libraries and frameworks in Eclipse not only aids in speeding up development but also leads towards a more organized, maintainable code. As you progress in your Java programming journey, mastering these aspects will surely reflect in your code quality and overall efficiency.
Debugging and Error Handling
Debugging and error handling are critical skills for any programmer, particularly for those working with Java in Eclipse. These processes help identify issues in code and rectify them before they become problematic during execution. Debugging enhances the overall reliability and efficiency of the code. In Java, many errors can arise, some of which are more challenging to spot than others. Understanding how to effectively manage these errors is invaluable in the learning process. The aim here is to illuminate the constants of detecting and resolving problems that may arise when writing Java applications within Eclipse.
Identifying and Fixing Errors
When writing any program, especially in Java, encountering errors is a common experience. Errors can be categorized into three main types: syntax errors, runtime errors, and logical errors.
- Syntax Errors: These are mistakes in the code that violate the grammar rules of Java. They are the easiest to identify, as the Eclipse IDE usually highlights them. For instance, forgetting a semicolon at the end of a statement can result in a syntax error. The IDE will provide an error message indicating where the problem is, allowing for quick fixes.
- Runtime Errors: These occur during program execution and can lead to the program crashing. For example, trying to access an array element that does not exist will trigger an ArrayIndexOutOfBoundsException. To resolve these, debugging is essential.
- Logical Errors: These errors are the hardest to detect since the code may run without any errors, but produce incorrect results. Identifying these errors involves careful analysis of the logic used in the program. Writing tests can help in detecting such errors.
To fix errors in Java, one must read the error messages carefully, pinpoint the issue, and correct it. Using the debugging tools of Eclipse can significantly facilitate this process, allowing for step-by-step execution of code to inspect variable states and flow control.
Using Eclipse Debugger Tools
Eclipse provides a robust set of debugging tools that make error handling more manageable. Knowing how to use these tools is essential for identifying and fixing errors efficiently.
- Setting Breakpoints: A breakpoint halts execution of the program at a specific line of code. This allows the programmer to inspect variables and program flow leading up to that point. To set a breakpoint, simply double-click in the left margin of the code editor next to the line number.
- Step Execution: Once a breakpoint is hit, you can use step execution features. These include:
- Variable Inspection: While in debugging mode, Eclipse allows you to inspect and modify variable values dynamically. This is crucial for understanding how data changes throughout the execution flow.
- Console Output: The console provides real-time feedback on program execution. It can display error messages and output generated by the program, assisting in identifying areas of concern.
- Step Into: This moves into the method being executed, allowing you to track the control flow.
- Step Over: This allows you to execute the current line and move to the next one without entering methods.
- Step Return: This finishes the execution of the current method and returns to the calling method, useful for breaking out of complex method calls.
Debugging is not a one-time task; it requires continual practice and engagement with code to master.
Utilizing these features effectively will greatly reduce the time spent on troubleshooting and enhance the quality of your Java applications. As you advance in your Java programming skills, mastering debugging techniques will provide you with the confidence to tackle more complex coding challenges.
Advanced Java Programming Techniques
In the realm of Java programming, mastering advanced techniques is crucial for developing high-performance applications. This section introduces two core topics: generics and collections, as well as multithreading and concurrency. Understanding these concepts significantly enhances the ability to write efficient, clean, and robust Java code.
Generics and Collections
Generics is a feature that allows developers to implement classes, interfaces, and methods with a placeholder for the type of data they will work with. This leads to stronger type checks at compile time and reduces the risk of runtime errors. Essentially, generics provide a way to define algorithms that can operate on different types of objects, which promotes code reusability and clarity.
Collections, on the other hand, refer to various data structures that hold groups of objects. Java provides a powerful Collections Framework that includes lists, sets, maps, and queues. Mastering these data structures is essential for efficient data handling and manipulation.
Benefits of using generics and collections include:
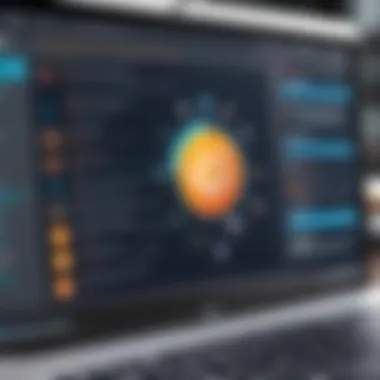
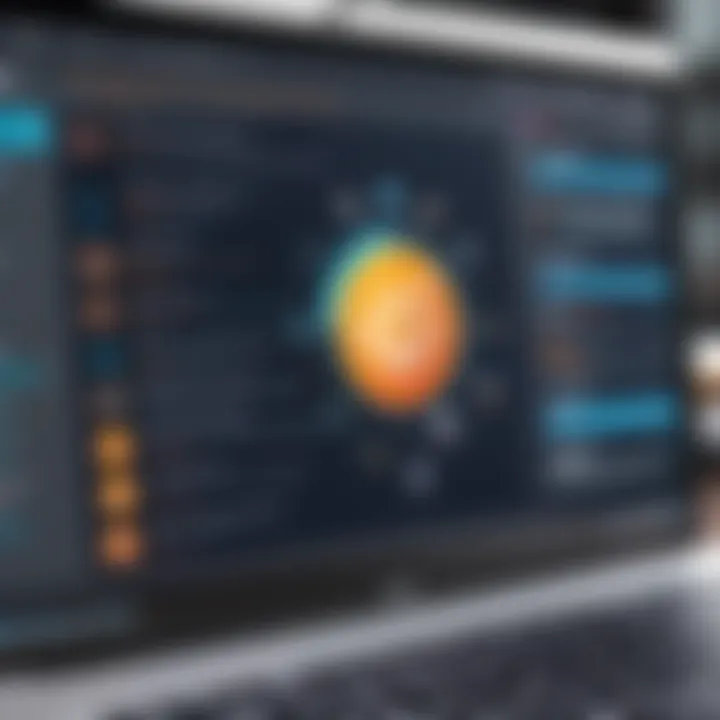
- Type Safety: Generics enable stronger type checks during compilation, reducing the potential for ClassCastException at runtime.
- Code Reusability: Generic algorithms can work with different data types without loss of type information.
- Reduced Boilerplate Code: With generics, less code is needed to achieve similar functionality, making code easier to read and maintain.
Here’s a simple example of generics in practice:
Multithreading and Concurrency
Multithreading is a programming concept that allows multiple threads to execute concurrently, thereby improving the efficiency of applications. Understanding how to manage threads is crucial in Java, as it enables developers to create applications that can perform several tasks simultaneously without hampering the responsiveness or performance.
Concurrency is a broader concept that addresses the execution of multiple threads and how they interact. When effectively managed, concurrency can lead to significant performance improvements in applications, particularly those that involve extensive processing tasks or I/O operations.
Important considerations for multithreading and concurrency include:
- Thread Safety: It is vital to ensure that shared resources are accessed consistently by multiple threads to prevent data inconsistencies.
- Synchronization: Java offers mechanisms such as methods and blocks to control thread access to resources.
- Executor Framework: This provides a higher-level API that simplifies thread management and allows for a cleaner separation of tasks from the implementation details.
By utilizing multithreading and concurrency, Java developers can create efficient applications that fully exploit modern multi-core processors.
In summary, mastering advanced programming techniques like generics and collections, as well as multithreading and concurrency, is vital for an effective Java developer. These concepts not only improve code quality and maintainability but also enhance application performance. Understanding these techniques is essential for anyone who aims to become proficient in Java programming.
Version Control and Collaboration
In software development, version control is a critical element that allows developers to manage changes to their code over time. It is especially vital when working in teams, where multiple people are modifying the same files. Effective collaboration among team members is essential for project success, and understanding how to integrate version control into your workflow can enhance productivity and maintain code integrity.
Integrating Git with Eclipse
Git is one of the most popular version control systems available. When integrated with Eclipse, it enables developers to track changes, create branches, and merge code seamlessly. Setting up Git within Eclipse can be achieved through the EGit plugin, which offers a visual interface for Git operations.
To integrate Git with Eclipse:
- Install EGit: Go to the Eclipse Marketplace and search for EGit to install it if it is not already included in your version of Eclipse.
- Clone a Repository: Use the version control perspective in Eclipse to clone an existing Git repository. This allows you to work on the latest version of a project.
- Commit Changes: After making code changes, right-click your project, select "Team," then "Commit" to save your modifications to the local repository.
- Push Changes: To share your changes with others, you can push them to a remote repository. This keeps everyone's code up to date and minimizes conflicts.
"Version control is not just about saving code; it’s about preserving history and promoting teamwork."
When you embrace version control, you minimize the chances of losing important work, which is a common issue in team settings. Also, using branches allows developers to experiment with new features without affecting the main codebase.
Collaborating with Team Members
Collaboration is more than just sharing code; it involves communication, coordination, and collective problem-solving. With tools like Git and Eclipse, collaboration becomes smoother. Here are key aspects to keep in mind when working with a team:
- Clear Communication: Always update team members on your progress and any challenges you face. This transparency helps in resolving problems quickly and enhances team dynamics.
- Regular Meetings: Schedule regular catch-ups to discuss project status. This practice can keep everyone aligned and ensure any discrepancies are addressed promptly.
- Code Reviews: Implement a system for peer reviews. Code reviews can improve code quality and share knowledge among team members.
- Issue Tracking: Utilize issue tracking tools integrated into Eclipse or used externally. Properly tracking bugs and features ensures everyone is aware of what needs addressing.
When you work effectively as a team using version control, you not only improve your workflow but also develop better coding practices. The combination of Git and Eclipse provides an efficient framework for version control, making it easier to manage mutual work toward a common goal.
In summary, mastering version control and collaboration techniques will not only enhance your coding skills but also position you to work more effectively within a team, which is a crucial aspect of modern software development.
Explore more about version control systems on Wikipedia.
Best Practices for Coding in Java
When learning Java, adhering to best practices is crucial for writing clean, efficient, and maintainable code. These principles not only streamline the development process but also enhance collaboration among teams, making code easier to read and modify in the future. Emphasizing best practices can significantly reduce debugging time and increase the overall quality of software projects. This section details the importance of code readability and maintenance, as well as the value of commenting and documentation.
Code Readability and Maintenance
Code readability refers to how easily other developers (or even your future self) can understand your code. The importance of this aspect cannot be overstated. Clear, readable code improves communication among team members and aids in identifying issues during code reviews. Specific strategies to enhance readability include:
- Consistent Naming Conventions: Use meaningful, descriptive names for variables, methods, and classes.
- Proper Indentation: Ensure nested structures are indented correctly to visually delineate code blocks.
- Limit Line Length: Keep lines short enough to avoid horizontal scrolling, typically around 80-120 characters.
When readability is prioritized, coding becomes intuitive. This supports flipping between different parts of the application without losing context. Moreover, readable code is easier to maintain. When bugs arise or enhancements are needed, developers can adjust existing code more swiftly, leading to more effective software evolution.
Commenting and Documentation
While well-structured code may communicate a lot alone, comments and documentation play a vital role in the coding process. They provide context about why certain decisions were made and explain complex logic in simpler terms. The importance of commenting includes:
- Clarifying Code Purpose: Describing what a section of code does helps others understand its intent without deciphering every line.
- Outlining Algorithms: Comments can explain the reasoning behind specific algorithms or complex logic that may not be immediately apparent.
Furthermore, maintaining comprehensive documentation is crucial for onboarding new team members and keeping project goals aligned. This includes:
- API Documentation: Clear descriptions of what each public method does and how it should be used.
- User Manuals: Guides for using the software can save time during troubleshooting and enhance user experience.
"Good code is in itself a form of documentation that other people can understand."
In closing, by embracing best practices in your Java coding endeavors, you set the stage for effective collaboration, smoother project transitions, and ultimately better software quality.
Resources for Continuous Learning
In the realm of programming, continuous learning is pivotal. While the basics of Java and Eclipse provide a solid foundation, the rapid evolution of technology necessitates ongoing education. Utilizing various resources allows learners to remain current with trends, tools, and best practices. The importance of having reliable sources for continuous learning cannot be overstated.
Investing time in quality resources can enhance one’s understanding of not just Java, but of programming as a whole. These resources can range from books and online courses to forums and community groups. Each element plays a significant role in solidifying knowledge and fostering practical application. Knowing where to look for information, updates, and expert opinions is valuable for programmers at all levels.
Recommended Books and Online Courses
Books are a traditional yet effective medium for learning. A comprehensive text can offer structure that self-paced learning paths might lack. Some recommended books for mastering Java include:
- "Effective Java" by Joshua Bloch: This book is highly regarded for its insight into best practices in Java programming.
- "Java: The Complete Reference" by Herbert Schildt: This serves as an excellent comprehensive guide for beginners.
On the other hand, online courses provide flexibility and often interactive elements, which can greatly enhance the learning experience. Platforms such as Coursera, Udemy, and edX offer high-quality Java courses. A few notable courses are:
- "Java Programming and Software Engineering Fundamentals" Specialization on Coursera: A series of courses designed to build a solid foundation.
- "Java Complete Masterclass" on Udemy: This course covers topics from basics to advanced techniques.
Participating in Programming Communities
Engaging with programming communities is another crucial aspect of continuous learning. These spaces offer networking opportunities, knowledge sharing, and real-world problem-solving techniques. Notable communities include:
- Reddit: Subreddits like r/java or r/learnprogramming allow users to ask questions, share resources, and discuss recent trends.
- Stack Overflow: This platform is essential for troubleshooting and getting answers from experienced programmers.
- Facebook Groups: There are dedicated groups for Java developers, which provide insights and community support.
Participating in community events, forums, or local meetups can help learners stay motivated and feel connected. This collaboration often brings new perspectives and ideas, ultimately enriching one’s programming journey.
"Continuous learning is the minimum requirement for success in any field."
By leveraging these resources, learners can significantly improve their skills and knowledge. Embracing a culture of continuous learning will not only contribute to personal growth but will also prepare individuals for the dynamic nature of the tech industry.
End and Next Steps
As this guide comes to its close, it is crucial to reflect on the journey of learning Java with Eclipse. The significance of this concluding section lies in helping learners solidify their comprehension and strategize their ongoing development. This article has laid a foundational structure for understanding both Java programming and the capabilities of Eclipse as an IDE. Now, readers must consider how to apply what they have learned in practical settings.
Reviewing What You've Learned
In this section, reviewing the key points covered in the earlier parts of the guide is essential. Reflecting assists in reinforcing knowledge and identifying areas requiring more focus. Here are some considerations for reviewing:
- Identify Core Concepts: Revisit the main topics like data types, object-oriented programming, and debugging techniques. Understand how these elements interconnect within Java development.
- Practical Application: Recall the projects you created and the programming challenges you faced. Consider how those experiences contribute to your skill growth.
- Engagement with Resources: Reflect on the additional resources mentioned throughout this guide. Whether it’s books or online communities, think about how they can further enhance your understanding.
By engaging in a critical review, learners can create a more cohesive knowledge base. This step is not just about recalling facts; it’s about making connections that deepen understanding.
Setting Goals for Further Development
Goal setting is a powerful motivator in the learning process. For those who have progressed through this guide, consider the following strategies for setting effective goals:
- Short-term Goals: These can include completing specific exercises or projects using Java in Eclipse. Setting deadlines can help maintain focus and commitment.
- Long-term Goals: Think about where you want to be in your programming journey in a year or two. Whether it's mastering a specific framework, contributing to open-source projects, or even transitioning careers, having clear long-term objectives can provide direction.
- Engaging with the Community: Participate in forums such as Reddit or join study groups on platforms like Facebook. These interactions can expose you to different problem-solving approaches and collaborative techniques.
In summary, setting goals is about creating a roadmap. By defining what you want to achieve and breaking it into manageable steps, you increase your chances of sustained motivation and eventual success.
"Learning is not the end, but only the beginning of a continuous journey in knowledge acquisition."
This conclusion and the strategies presented are instrumental for learners. They help bridge the gap between understanding theoretical concepts and applying them effectively in real-world scenarios.