Master JavaScript Programming: A Comprehensive Step-by-Step Guide
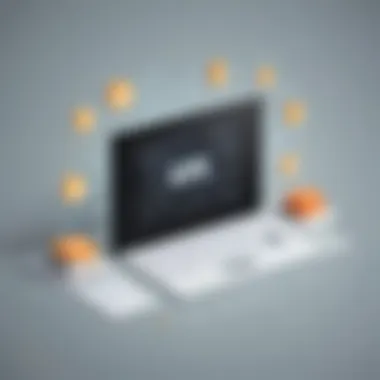
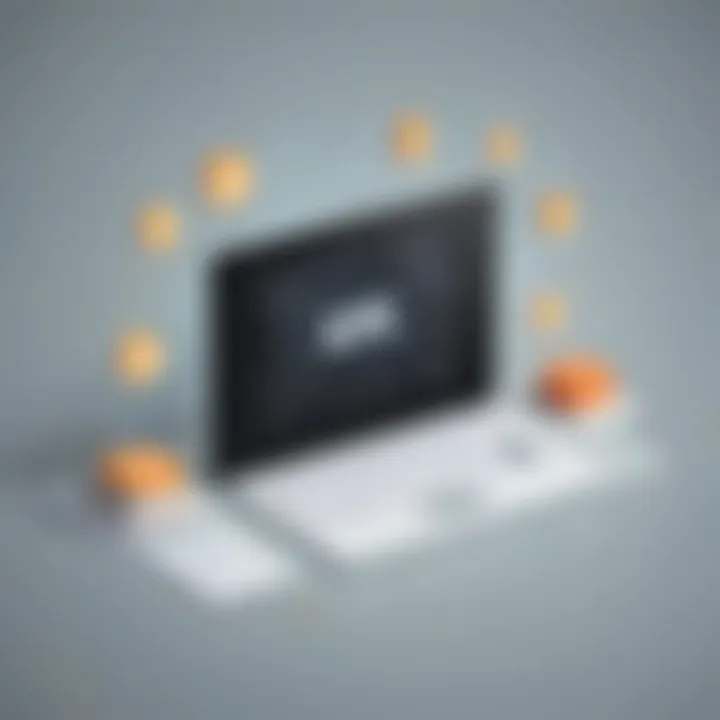
Introduction to Programming Language
JavaScript is a prominent programming language used extensively for web development. Understanding the history and background of JavaScript can provide valuable insights into its evolution and relevance in today's tech landscape. Its features and uses encompass a wide range of applications, from interactive websites to server-side development. The popularity of JavaScript is undeniable, with a vast scope in the realm of front-end and back-end development, making it an integral skill for modern programmers.
Basic Syntax and Concepts
Delving into JavaScript basics involves exploring variables and data types, essential building blocks for programming. Operators and expressions play a crucial role in manipulating data and executing operations within scripts. Control structures, such as loops and conditional statements, govern the flow of program execution, allowing for logical decision-making in code.
Advanced Topics
Advancing in JavaScript proficiency entails mastering functions and methods to encapsulate logic efficiently. Object-oriented programming principles empower developers to organize code into reusable objects, enhancing scalability and maintainability. Exception handling refines error management, enabling graceful recovery from unexpected scenarios in applications.
Hands-On Examples
Practical application of JavaScript concepts can be demonstrated through simple programs that showcase fundamental concepts in action. Intermediate projects provide opportunities to work on more complex problems, honing problem-solving skills and promoting creativity in code solutions. Code snippets offer concise illustrations of specific techniques or functionality, serving as valuable learning aids for aspiring JavaScript developers.
Resources and Further Learning
For aspiring programmers seeking to deepen their JavaScript knowledge, recommended books and tutorials offer structured guidance for self-paced learning. Online courses and platforms provide interactive learning experiences, allowing individuals to engage with dynamic content and professional instructors. Community forums and groups serve as valuable resources for networking, collaboration, and staying updated on industry trends and best practices.
Introduction
In the realm of programming languages, JavaScript stands out as a powerhouse that fuels the interactivity and dynamic nature of websites and web applications. Its importance cannot be overstated, as JavaScript plays a pivotal role in modern web development, driving user experiences and enhancing functionality. Understanding the nuances of JavaScript is essential for aspiring developers looking to build dynamic and responsive web solutions. In this section, we will delve into the significance of JavaScript within the context of this comprehensive learning guide.
Understanding the Importance of JavaScript
JavaScript in Web Development
JavaScript in Web Development serves as the cornerstone for creating interactive and engaging web experiences. Its versatility in manipulating the Document Object Model (DOM) allows developers to dynamically update content, handle user events, and communicate with servers asynchronously. The asynchronous nature of JavaScript enables seamless functionality without blocking the user interface, making it a preferred choice for client-side scripting. Despite some performance considerations, JavaScript's adaptability and robust ecosystem make it a powerful tool for crafting modern web applications.
JavaScript vs. Other Programming Languages
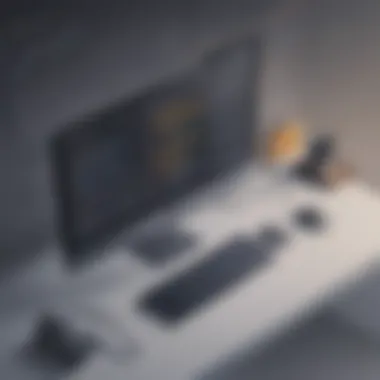
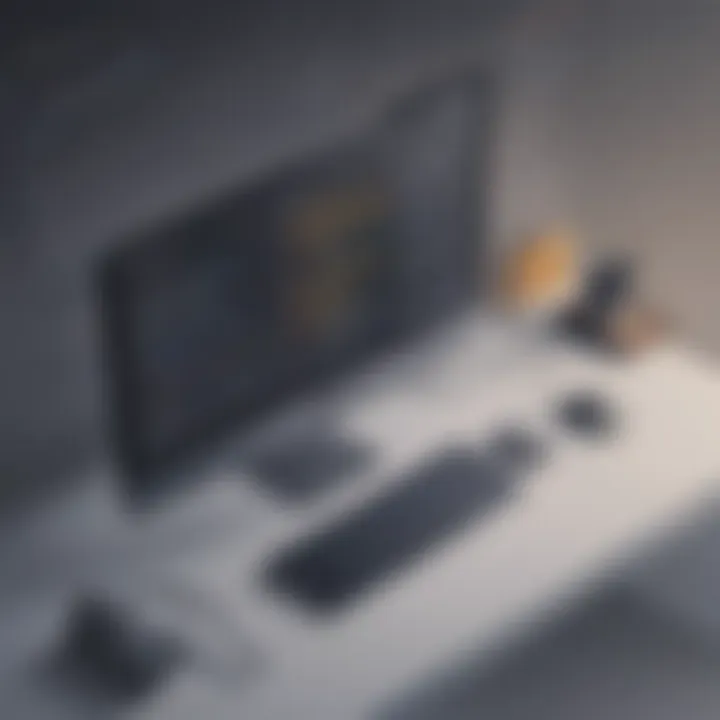
When comparing JavaScript to other programming languages, its unique characteristics shine through. JavaScript's client-side execution differentiates it from server-side languages, offering real-time interactivity without page reloads. Its prototypical inheritance and functional programming features provide flexibility in coding practices. However, JavaScript may face challenges in performance optimization compared to compiled languages. Understanding these nuances is crucial for developers to harness JavaScript's strengths effectively in their projects.
Setting Up Your Development Environment
Choosing a Code Editor
Selecting the right code editor is essential for an efficient development workflow. A code editor like Visual Studio Code or Sublime Text offers features such as syntax highlighting, auto-completion, and debugging tools to streamline coding tasks. Customizability and extensibility further enhance the coding experience, allowing developers to personalize their environment for maximum productivity. While each code editor has its strengths and weaknesses, finding one that aligns with your workflow and preferences is key to optimizing your coding experience.
Installing Node.js for JavaScript Development
Node.js revolutionizes JavaScript development by enabling server-side scripting beyond the browser environment. By leveraging the V8 JavaScript engine, Node.js empowers developers to build scalable and performant backend applications. The npm package manager simplifies dependency management, providing access to a vast ecosystem of libraries and tools. Integrating Node.js into your development environment opens doors to full-stack JavaScript development, enhancing your capabilities as a versatile programmer.
Getting Started with JavaScript
When embarking on the journey of learning JavaScript, the initial step is crucial to building a strong foundation. Getting started with JavaScript lays the groundwork for understanding the language's syntax, principles, and capabilities. This section serves as the gateway into exploring the functionalities and possibilities that JavaScript offers to developers. By delving into the basics, learners can grasp fundamental concepts that are essential for advanced programming practices.
Basics of JavaScript
Variables and Data Types
In the realm of JavaScript programming, variables and data types form the building blocks of code structure. Variables act as containers for storing data, allowing for manipulation and access throughout the script. Data types define the nature of values held by variables, such as strings for text, numbers for numerical values, and booleans for truefalse statements. Understanding variables and data types is pivotal for creating dynamic and responsive scripts, enabling developers to interact with and process information effectively. While variables offer flexibility in storing different kinds of data, data types ensure consistency and accuracy in data manipulation.
Operators and Expressions
Operators and expressions in JavaScript play a vital role in executing operations and evaluations within scripts. Operators are symbols that represent actions like addition, subtraction, comparison, and logical operations, while expressions combine variables, values, and operators to produce specific outcomes. The utilization of operators and expressions enhances the functionality and efficiency of scripts, providing developers with the means to perform calculations, make decisions, and control the flow of execution. Mastery of operators and expressions empowers programmers to write concise and logical code, facilitating the implementation of complex algorithms and functionalities.
Control Flow
Conditional Statements
Conditional statements form the cornerstone of decision-making in JavaScript programs, enabling developers to execute code based on specified conditions. By using if, else if, and else statements, programmers can create branching pathways within their scripts, allowing for different outcomes based on varying situations. Conditional statements enhance the flexibility and adaptability of scripts, ensuring that code responds dynamically to changing circumstances. The judicious application of conditional statements promotes the development of robust and interactive scripts, enabling developers to address diverse scenarios and user interactions effectively.
Loops in JavaScript
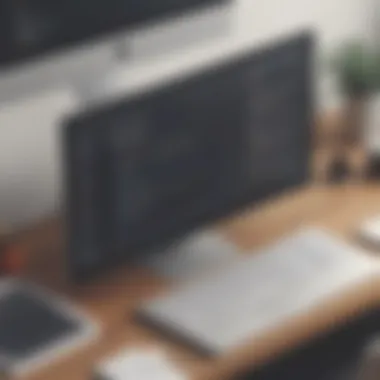
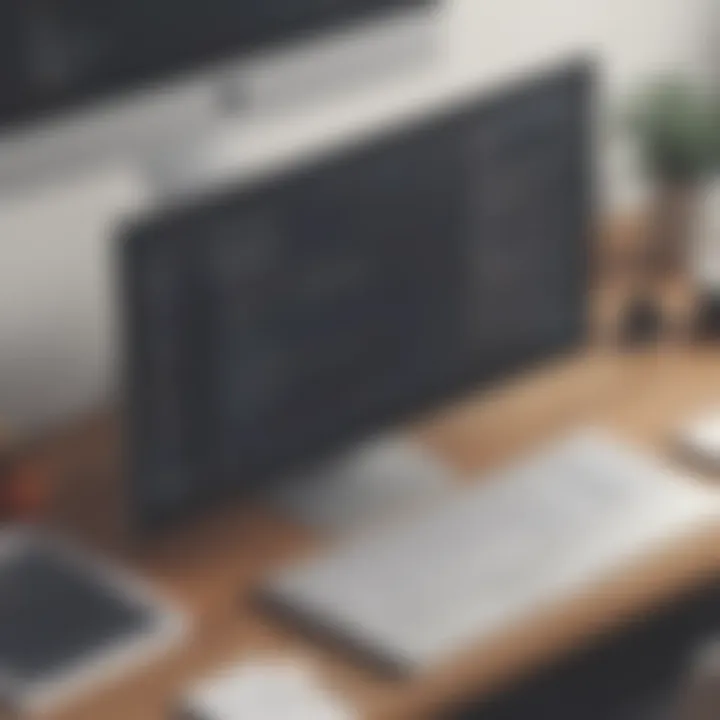
Loops serve as iterative structures in JavaScript, facilitating the repetition of code blocks until certain conditions are met. With for, while, and dowhile loops, developers can automate tasks, iterate through data structures, and streamline repetitive processes in scripts. Loops optimize code efficiency, reducing redundancy and enhancing the scalability of applications. By harnessing the power of loops, programmers can iterate over arrays, manipulate objects, and traverse complex data structures with ease, boosting productivity and code maintainability.
Intermediate JavaScript Concepts
Functions and Scope
Function Declarations vs. Expressions
Exploring the nuances between function declarations and expressions reveals a fundamental aspect of JavaScript development. Function declarations are hoisted to the top of the script during execution, allowing you to invoke them before they are defined. On the other hand, function expressions are treated as variables, offering more flexibility in terms of where and how they are declared. The choice between these two methods depends on the specific use case and coding style adopted in the project. Understanding when to utilize function declarations for explicit definitions or function expressions for more dynamic implementations is key to writing efficient and maintainable JavaScript code.
Closures and Lexical Scope
Grasping the concept of closures and lexical scope provides insight into how JavaScript manages variable access within functions. Closures enable functions to retain access to variables from their containing scope even after the parent function has finished executing. This behavior allows for powerful design patterns like the Module Pattern, where data encapsulation is crucial. Leveraging lexical scope ensures that functions can access variables defined in their outer scope, promoting code reusability and encapsulation. However, improper handling of closures can lead to memory leaks and unexpected behaviors, emphasizing the importance of understanding the intricacies of lexical scoping in JavaScript development.
Arrays and Objects
Working with Arrays
Mastering the manipulation of arrays is pivotal in JavaScript programming. Arrays offer a versatile and efficient means of storing multiple values, enabling operations like sorting, filtering, and iterating with ease. Understanding how to work with arrays involves leveraging built-in functions such as map, filter, and reduce to streamline data processing tasks. Additionally, exploring array methods like push, pop, shift, and unshift equips developers with the tools to modify array contents dynamically. Arrays serve as the backbone of many data structures and algorithms, making proficiency in array manipulation a valuable skill in JavaScript development.
Creating and Manipulating Objects
Objects are essential components in JavaScript for organizing and accessing structured data. By mastering object creation and manipulation, developers can model real-world entities with ease. Objects in JavaScript are dynamic and can be modified on the fly, allowing for flexible data representations. Understanding how to manipulate object properties, create prototypes, and implement inheritance enhances the scalability and maintainability of JavaScript code. Furthermore, employing object literals, constructor functions, and ES6 classes offers various paradigms for object-oriented programming in JavaScript, catering to diverse project requirements and coding styles.
Advanced JavaScript Topics
In this section of the article, we delve into the significance of Advanced JavaScript Topics within the context of this comprehensive JavaScript learning guide. Advanced JavaScript Topics play a pivotal role in expanding the readers' knowledge beyond the basics and intermediate concepts, providing a deeper understanding of complex functionalities and techniques that are crucial in modern web development practices. These topics serve as a bridge towards mastering JavaScript programming and are essential for anyone looking to elevate their coding skills to a professional level.
Asynchronous JavaScript
Understanding Promises
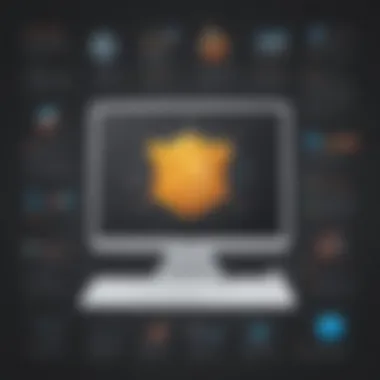
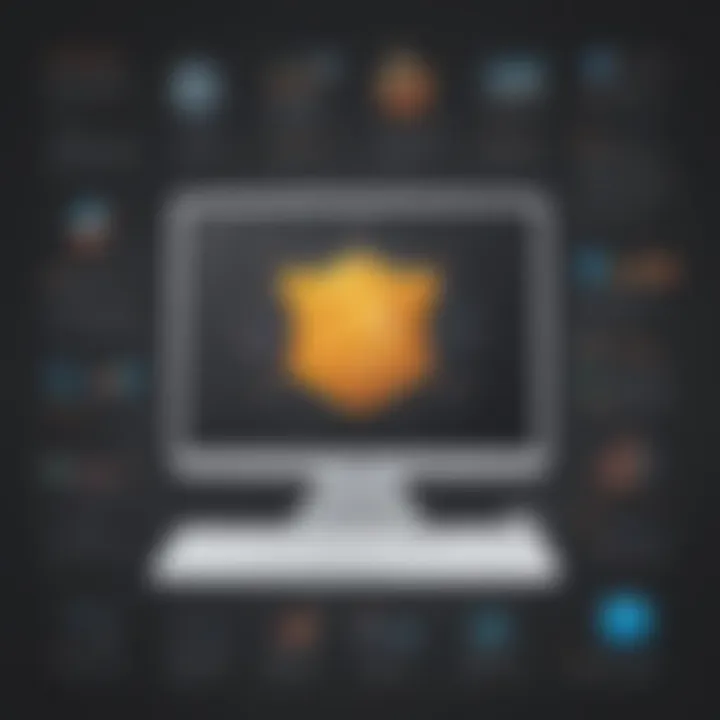
Delving into Understanding Promises, we encounter a fundamental aspect of asynchronous JavaScript programming. Promises offer a streamlined approach to handling asynchronous operations, allowing for more efficient and readable code. The key characteristic of Promises lies in their ability to manage asynchronous tasks and handle their results seamlessly, enhancing the overall functionality and performance of JavaScript applications. As an integral feature of this article, Understanding Promises provides a structured method for dealing with asynchronous tasks, reducing callback hell and promoting cleaner code architecture. While Promises bring about advantages such as improved code maintainability and error handling, they also present challenges like proper error propagation and Promise chaining complexities within the scope of this tutorial.
Working with AsyncAwait
Another aspect explored is the utilization of AsyncAwait in JavaScript programming. AsyncAwait simplifies the process of writing asynchronous code by enabling developers to write asynchronous functions in a synchronous manner, enhancing the readability and logic flow of the codebase. The primary characteristic of AsyncAwait revolves around making asynchronous code appear synchronous, making it a favored choice in this JavaScript guide for its user-friendly approach to handling asynchronous operations. By providing a cleaner syntax and reducing callback nesting, AsyncAwait aids in the creation of more organized and maintainable code. While the unique feature of AsyncAwait lies in its concise syntax and straightforward error handling mechanism, it also introduces potential drawbacks such as the need for proper error management and potential performance implications within the content of this article.
ES6 Features
Arrow Functions
One of the pivotal features introduced in ES6 is Arrow Functions, offering a compact and succinct method for writing functions in JavaScript. Arrow Functions contribute significantly to enhancing code conciseness and readability, making them a preferred choice in this educational material for their efficiency in writing function expressions. The key characteristic of Arrow Functions lies in their lexical this binding, which simplifies the handling of function scope and context within JavaScript applications. In this guide, Arrow Functions streamline the process of defining functions and provide a more intuitive syntax for concise function implementation. Despite their benefits in reducing verbosity and improving code clarity, Arrow Functions may present limitations such as lack of binding of this keyword and potential confusion for new developers navigating complex codebases.
Template Literals
The exploration of Template Literals in this JavaScript guide uncovers a versatile feature in ES6 for string interpolation and multiline text formatting. Template Literals revolutionize the way strings are constructed in JavaScript by allowing embedded expressions and multiline strings within a single backtick-delimited template. The key characteristic of Template Literals is their ability to enhance string manipulation efficiency and readability, offering an elegant solution for string formatting in modern JavaScript applications. By enabling the embedding of expressions directly within strings, Template Literals facilitate dynamic content generation and improve code maintainability. While the unique feature of Template Literals lies in their flexible syntax and improved string formatting capabilities, they may pose challenges in terms of compatibility with older browsers and potential code readability issues within this instructional material.
Practical Projects and Exercises
Practical projects and exercises play a crucial role in reinforcing the concepts learned in this article. By engaging in hands-on projects, readers can gain practical experience and apply theoretical knowledge to real-world scenarios. These exercises help solidify understanding, improve problem-solving skills, and boost confidence in JavaScript programming. Moreover, practical projects provide a practical avenue for learners to experiment, test ideas, and deepen their understanding of JavaScript programming concepts.
Building a To-Do List App
Task Initialization and Display
Task initialization and display are essential aspects of developing a functional to-do list app. Task initialization involves setting up the necessary structures to store tasks efficiently. Display functionality ensures that tasks are shown clearly and intuitively to the user. The key characteristic of task initialization and display is its simplicity and user-friendliness. This approach allows users to easily create, view, and manage their tasks effectively. The unique feature of task initialization and display lies in its intuitive design, making task management streamlined and accessible. However, one potential disadvantage could be the need for careful planning to ensure the smooth operation of task initialization and display within the app.
Adding and Deleting Tasks
Adding and deleting tasks are fundamental actions in a to-do list app. Adding tasks involves inserting new entries into the task list, while deleting tasks removes unwanted items from the list. The key characteristic of adding and deleting tasks is their direct impact on task management and organization. Both functions contribute significantly to the app's usability and efficiency. The unique feature of this process is the ability to swiftly modify task lists based on user input. However, potential disadvantages may include the risk of accidental deletions or data loss if not implemented with caution.
Coding Challenges
Solving Algorithmic Problems
Solving algorithmic problems is a fundamental aspect of mastering JavaScript. It involves applying logic and problem-solving skills to tackle complex coding challenges. The key characteristic of solving algorithmic problems is its focus on developing analytical thinking and algorithmic efficiency. This practice enhances a programmer's ability to break down complex problems into manageable steps. The unique feature of this process is the structured approach to problem-solving, fostering strategic thinking and enhancing coding skills. However, one potential disadvantage could be the time and effort required to master advanced algorithms and problem-solving techniques.
Implementing Data Structures in JavaScript
Implementing data structures in JavaScript is pivotal for efficient data management and manipulation. It involves creating and utilizing data structures like arrays, objects, stacks, and queues to organize and store information effectively. The key characteristic of implementing data structures is its role in optimizing data access and storage. This approach enhances the performance of JavaScript code and supports the implementation of complex algorithms. The unique feature of this practice lies in its versatility and applicability across various programming tasks. However, potential disadvantages may arise from the complexity of implementing certain data structures and selecting the most suitable structure for specific programming requirements.