Master Java Programming: A One-Day Learning Guide
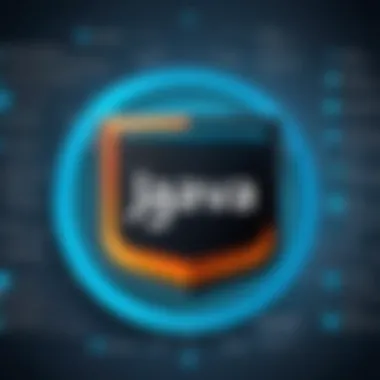
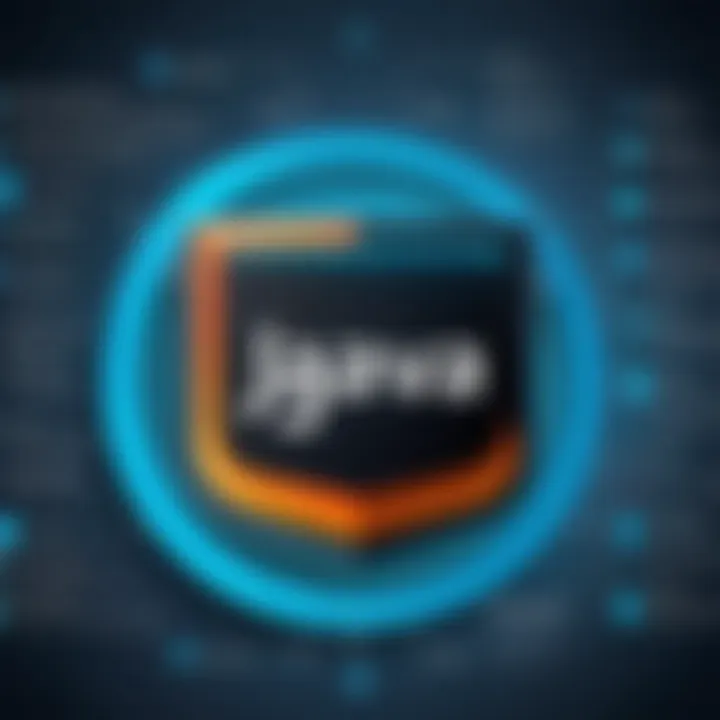
Preface to Programming Language
Programming languages are pivotal tools in the realm of technology, enabling developers to communicate with computers to create software, websites, and applications. This guide on Java outlines various aspects and sets the groundwork for meaningful understanding.
History and Background
Java was introduced to the public in 1995 by Sun Microsystems, led by James Gosling, as a response to the emerging need for a versatile programming language that could run on various devices. Its slogan, "Write Once, Run Anywhere" highlights its cross-platform capabilities, powered by the Java Virtual Machine. Developers embraced its reliability, enforceability, and scalability, making Java an attractive choice for both personal and business applications over time.
Features and Uses
Java speaks with its own loud silence as a language characterized by several defining attributes.
- Object-oriented: Java promotes clear modularity in programming. This aspect enhances code reuse and organization.
- Platform-independent: You can run Java on different hardware or OS, owing to the JVM.
- Strongly typed: This prevents certain types of errors that can occur at runtime by enforcing strict data type usage.
Java finds application across a diverse array of fields:
- Web development through Java Server Pages.
- Android app development with Google’s Android SDK.
- Enterprise applications using Jakarta EE for backend services.
Popularity and Scope
Java maintains a formidable position in programming languages popularity rankings. This proximity to mainstays like Python and C++ can be attributed to its slightly vintage yet continually evolving nature. Driven by a strong community and extensive libraries, this language presents scope from initial personalized learning projects to enterprise-level implementations. Its robustness ensures ongoing demand in the job market, marking Java as a relevant and strategic choice for learning.
Java’s blend of longevity and modern evolution positions it as a cornerstone within software development frameworks for beginners and experienced programmers alike.
Finale
In summary, understanding the historical context and foundational features of Java is crucial. Knowledge allows for better application in developing software solutions, thus making a solid groundwork to navigate deeper Java topics efficiently.
Prelude to Java Programming
Java programming continues to be a fundamental skill in the technology landscape. Understanding its core concepts is vital for anyone looking to enter fields like software development, web application creation, or even big data environments. Java provides versatility, platform independence, and strong community support, making it an excellent starting point for both beginners and experienced programmers. Learning Java facilitates quicker entry into sophisticated programming paradigms and enhances problem-solving abilities. This section highlights the historical development and ongoing relevance of Java in today’s tech world.
Historical Context and Development
Java was first released by Sun Microsystems in 1995. Its creation was spearheaded by James Gosling and team. The primary vision was to develop a programming language that was simple yet powerful enough to cater to various applications, foreshadowing concepts of portability and ease of use. The write once, run anywhere philosophy emerged from its design, making it remarkably attractive for developers targeting different platforms. Over the years, many versions of Java have been released, refining its capabilities and expanding its libraries to adapt to new programming demands.
Key Milestones in Java Evolution:
- Early Days (1995): Initial release focused on applets.
- Java 2 (1998): Introduction of concept Swing for GUI development.
- Java 5 (2004): Major updates like generics and enhanced for-loops.
- Java 8 (2014): Brought lambdas and streams that impacted functional programming.
Java's longevity over decades contrasts sharply with newer programming languages, despite its popularity prevalent throughout industries. Continuous updates ensure its adaptability and ensure a vast relevance in coding practice.
Relevance in Today’s Tech Landscape
In today’s market, Java signifies reliability, resulting in various enterprise applications reliant on it. Companies like Google, Amazon, Twitter, and eBay utilize Java for backend development given the framework's scalability and efficiency. Moreover, Java forms a backbone for Android applications, highlighting importance for a growing coder’s landscape.
Several key benefits emerge for learning Java at this juncture in technological evolution:
- **Robust Framework: **Using Spring or Hibernate enhances its backend capabilities in web services.
- **Extensive Libraries: **Rich libraries exist for almost any functionality desired.
- **Community Support: **A global developer community means assistance is a website or forum post away.
Java does not just dominate in programming environments but embodies itself in educational systems worldwide, nurturing the next generation of programming talent.
With a fundamental understanding of Java, one possesses a reliable tool to enter wondrous activities in software engineering and understaning complex systems.
Setting Up Your Development Environment
Setting up your development environment is a crucial step when learning Java. It lays the groundwork for your coding experience and speaks directly to the efficiency of your learning curve. A proper environment ensures you can write, compile, and run your code smoothly, providing an essential feedback loop.
Creating an effective development environment requires installing the Java Development Kit (JDK) and selecting the right Integrated Development Environment (IDE). Each serves a unique function but collectively aids in making the learning process intuitive and efficient. A poorly set up environment might lead to frustration and deter interest in coding.
Installing Java Development Kit (JDK)
The Java Development Kit, or JDK, is fundamental for anyone aspiring to write and test Java programs. It is a comprehensive tool, including the Java Runtime Environment (JRE) and development tools necessary for developing Java applications.
To begin, you need to download the JDK from the official Oracle or OpenJDK websites. The installation process varies slightly based on your operating system.
- For Windows:
- For macOS:
- For Linux:
- Download the JDK installer from oracle.com.
- Run the installer.
- Follow the on-screen instructions.
- Set the system's environment variables to recognize the Java commands.
- Use Homebrew to install by running: .
- Follow additional steps, like setting up a PATH if necessary.
- Use the package manager, such as or , to get JDK easily. Example: .
Establishing the JDK allows you to compile your code, interpret errors, and run Java applications competently.
Choosing an Integrated Development Environment (IDE)
Selecting the right IDE can highly impact your learning efficiency and coding experience.
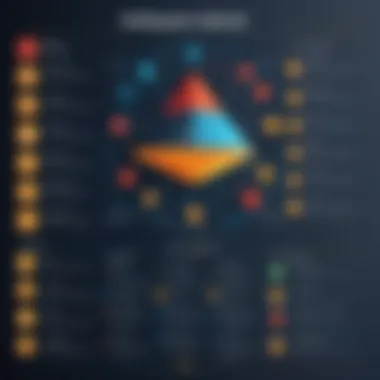
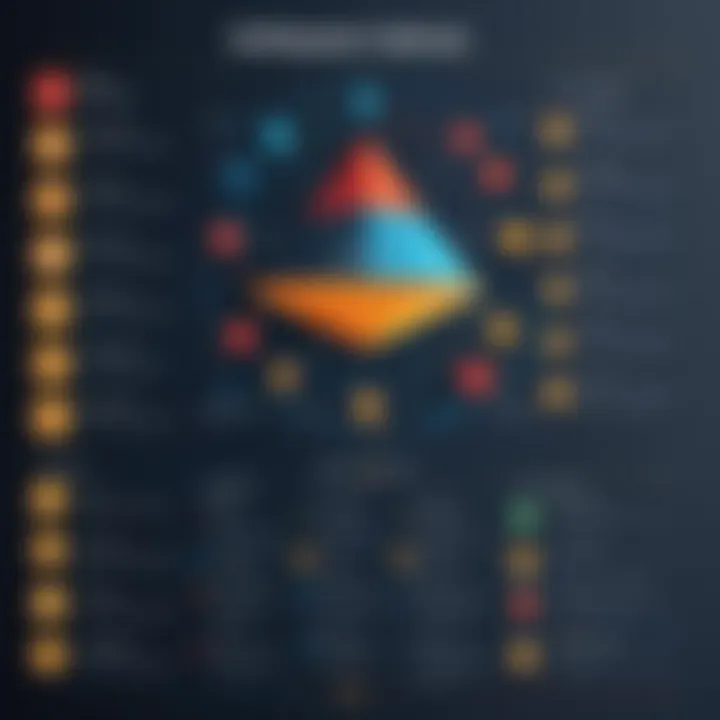
Overview of Popular IDEs
When it involves establishing a solid coding foundation, several IDEs stand out: Eclipse, IntelliJ IDEA, and NetBeans. Each provides features tailored for Java development and boasts a rich set of plugins to enhance your capabilities.
- Eclipse: Renowned for its powerful debugging capabilities and support for multiple programming languages.
- IntelliJ IDEA: Valued for its smart code completion and in-built developer tools that enhance productivity.
- NetBeans: Has a user-friendly interface. It's an excellent choice for beginners due to its simplicity and integration of tools.
Choosing any of these IDEs is beneficial as they cater to a wide range of developers, from novice to advanced.
Setting Up Your IDE for Java
After selecting your IDE, the setup process contributes significantly to a seamless Java programming experience. Each has unique installation requirements, but the process is generally straightforward. For instance, a Java-specific IDE like IntelliJ IDEA straightforwardly guides new users through workspace and project configuration.
In almost every IDE, you would typically need to:
- Install the required plugins for Java, if necessary.
- Configure the JDK path to make sure the IDE recognizes Java installations.
- Adjust preferences and settings that fit your workflow.
However, a built-in feature, such as instant project templates, can ease the exploration of Java programming even if you are a novice.
Always remember, a well-set environment fosters creativity in programming, eliminates setup complications, and allows for distraction-free learning. Your leanring curve will begin to smooth out quickly with these tools in place.
A good development setup reflects the comfort zone to experiment and learn Java more effectively.
Understanding Java Syntax and Structure
Understanding Java syntax and structure is crucial for learners aiming to master the language quickly. A grasp of syntax allows programmers to write code that Java can understand. Structured programming leads to organized and maintainable code, essential for collaboration and future modifications. In this section, we will demystify the basic elements of Java syntax and how they operate.
Basic Syntax Rules
Java's syntax incorporates specific rules that govern how programs are written. These rules dictate where code statements start and end, giving clarity to the structure of the program. Here are some fundamental syntax rules:
- Statements: Java statements end with a semicolon (). This clearly marks the end of one instruction and, thus, aids processing.
- Case Sensitivity: Java is case-sensitive. and would be considered different identifiers. Hence, maintain consistent casing throughout your code.
- Comments: Comments enhance clarity but do not affect code execution. You can include single-line comments using and multi-line comments with .
Familiarizing yourself with these rules early increases coding competency, avoiding common pitfalls.
Data Types and Variables
In Java, data types specify the kind of value a variable can hold. This is essential for memory management and efficient operation. Data types fall broadly into two categories:
- Primitive Data Types: Easily recognized types like , , , and . For example:
- Reference Data Types: These are complex types, including objects and arrays. For example:
It's important to choose the right data type based on the program's needs. Effective use of variables simplifies code and boosts performance.
Control Structures: If, Switch, Loops
Control structures change the flow of the program based on conditions and iterations. Mastery of these constructs is vital for problem-solving and logical processing in Java.
- If Statements: This is the most fundamental control statement.
- Switch Statements: Useful when you have multiple conditions against a variable. It simplifies conditional structures, maintaining readability.
- Loops: Useful for repeating tasks. The common loops in Java include , , and loops. Here's an example of a loop:
These control structures empower coders with precise analytics and iterative capabilities. Mastering them shapes strong programming thinking effectively.
Core Object-Oriented Programming Concepts
Object-oriented programming is a paradigm that has huge influence in modern software development. Java, being fundamentally an object-oriented language, promotes clear and efficient software design. Learning core object-oriented programming concepts is vital for any programmer, as these principles provide a framework for creating robust solutions to complex problems.
Key benefits include:
- Code reusability: By using objects, developers can create code that is efficient and reusable.
- Maintenance: Object-oriented design allows changes to be made to applications without disrupting the entire system.
- Real-world modeling: This paradigm helps in structuring software to mimic real-world systems, making it easier to conceptualize and manage.
The three main concepts are: classes, inheritance, and encapsulation. Understanding these elements strengthens one's programming foundational knowledge.
Classes and Objects
In Java, a class serves as a blueprint to create objects. An object is an instance of a class that encapsulates both data and behaviors relevant to that data.
- Definition: A class groups data and methods designed to manipulate that data. Once a class is defined, multiple objects can be created from it, each retaining its own state.
- Example: For example, consider a class which can have properties like , , etc., and behaviors like and . An object could be , an instance of the class.
- Benefits: Utilizing classes simplifies data management and enhances code clarity, both jwhich are crucial as projects scale.
Inheritance and Polymorphism
Inheritance enables a new class to inherit attributes and methods from an existing class, promoting code reusability. This allows developers to create a hierarchal relationship between classes.
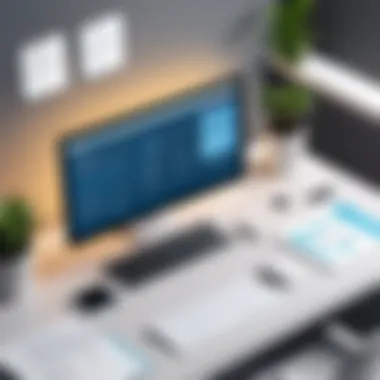
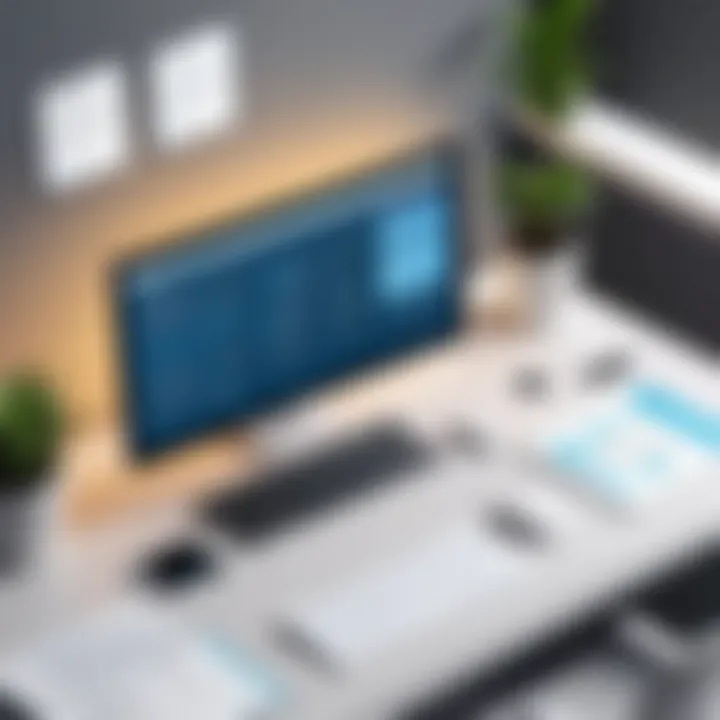
- Categories: The parent class (or super class) gives base functionality, while a child class (or sub-class) can enhance or override that functionality as needed.
- Polymorphism: This concept lets classes define methods in different ways based on their requirements. It is the ability for a method to perform different tasks based on the object calling it.
- Practical example: If you have a base class called , you could have sub-classes like and , both of which have the method , but implement it differently: Dog barks, Cat meows.
Encapsulation and Abstraction
Encapsulation is the bundling of data and methods that operate on that data within a single class. This protects object integrity by restricting unauthorized access.
- Access Modifiers: Use of private, public, and protected modifiers granulates control over data visibility.
- Abstraction: Abstraction simplifies complex reality by reducing unnecessary details, exposing only the essential aspects. In practice, this may mean implementing interfaces to define available actions without unveiling implementation details to the user.
- Real verstinisi: When utilizing encapsulation, you might implement getters and setters to control access to a property while abstracting the complexity of operations involved behind the scenes.
Understanding core object-oriented programming concepts in Java is not only essential for application development but also sets a strong foundation for adopting other programming paradigms in the future.
Essential Java Libraries and Frameworks
In the realm of Java programming, libraries and frameworks play a crucial role in enhancing productivity and simplifying complex tasks. They offer pre-written code which developers can utilize for different functions, making the process of software development more efficient. Using these tools can not only save a significant amount of time but also foster consistency and best practices in coding. In this section, we will explore the key elements of Java libraries and frameworks, particularly focusing on the Java Standard Library and some of the popular frameworks available for developers today.
Prelims to Java Standard Library
The Java Standard Library offers a diverse collection of classes and interfaces that the Java programming language provides by default. It contains essential packages and APIs that facilitate everyday tasks, including file input and output, networking, data manipulation, and graphical user interface development.
One significant aspect of the Java Standard Library is that it is designed to be easy to use, allowing even beginners to leverage its components quickly. For instance, packages like provide collections framework, which is essential for storing and manipulating groups of data, such as lists, trees, and hash tables. By utilizing these built-in libraries, programmers can avoid unnecessary reinventing of the wheel and leverage tested, reliable components, making it a smart addition to your learning journey when studying Java.
Overview of Popular Java Frameworks
Java frameworks simplify the development process by providing an environment that streamlines coding work. They allow developers to focus on the core aspects of their applications, rather than spending time on repetitive tasks. Below, we will discuss two of the most essential frameworks in the Java ecosystem, Spring Framework and Hibernate, both of which are widely used in various projects.
Spring Framework
The Spring Framework is immensely popular for its comprehensive programming and configuration model. One of the most advantageous aspects of Spring is its capability to facilitate the development of robust applications while providing extensive tools such as dependency injection, aspect-oriented programming, and transaction management. These features contribute significantly by improving module interconnectivity and overall code maintainability.
A key characteristic of the Spring Framework is its scalability. This framework adapts easily, from small applications to massive enterprise systems, accommodating developers as their needs evolve. Notably, Spring offers support for building REST API services and integrating with various databases, making it a fundamental tool for anyone looking to deepen their Java skills. However, navigating Spring's breadth of features may contribute to a steep learning curve for beginners.
Hibernate
Hibernate is a powerful Object-Relational Mapping (ORM) framework that simplifies database operations in Java. It enhances developer productivity by handling the complexities of setting up database connections and writing time-consuming SQL queries directly. With Hibernate, Java objects can be mapped to database tables seamlessly, which translates into less boilerplate code.
The main benefits of Hibernate lie in its ability to cache data to improve performance, offering query options either through SQL or Hibernate Query Language (HQL). The framework's lazy loading capability allows data to be fetched only as needed, enhancing the efficiency of resource usage. Although Hibernate requires a basic understanding of relational databases, its benefits make it an excellent choice for developing scalable and maintainable applications.
Using libraries and frameworks not only blocks the pitfall of repetitive coding but also ensures your work is aligned with industry standards.
An effective way to summarize the contributions of libraries and frameworks in Java is that they guide beginners and help them develop their skills more swiftly. Knowing where to focus your study effort is essential. Following your day of learning, ensure to note those libraries and frameworks that might align with your goals.
Building Your First Java Application
Creating your first Java application marks a significant milestone in your programming journey. It serves as a practical avenue to apply the theoretical concepts you have learned throughout your study. Beyond mere syntax, building an application consolidates various Java features while inspiring confidence in your coding abilities. As you work through the following sections, discerning how to structure and develop a simple program becomes clearer, leading to a more profound understanding of Java.
Creating a Simple Java Program
To embark on this journey, start small. A simple Java program typically includes defining a class, writing a method, and using the method to execute your program. Here’s how you can structure your first program:
In this example:
- Class Definition: declares the class named HelloWorld. All Java programs need a class definition.
- Main Method: is the entry point. It's where the Java Virtual Machine starts executing the code.
- Output Statement: prints text to the console.
When you modify sections of this basic framework—by changing text between the parentheses, for example—you immediately see results, reinforcing the concept of interaction through code.
Compiling and Running Your Java Application
After creating your simple Java program, the next crucial step entails compiling and executing your application. This process bridges the gap between writing code and seeing it come to life on your console. To compile and run the earlier example, follow these steps:
- Open Command Line Interface: Depending on your system, this would be Command Prompt on Windows or Terminal on macOS.
- Navigate to Your Java File: Use the command to change directories to where your file is kept.
- Compile the Program: Execute the following command:If all is well, there will be no output. You will see a new file , which is the compiled bytecode that the Java Virtual Machine understands.
- Run the Program: To see your program in action, input this command:Upon execution, you should see printed to your console. This straightforward cycle of writing, compiling, and executing demonstrates the core workflow when working with Java.
Learning to create and run a Java application is integral, as it cements your understanding of Java’s syntax and operational mechanics.
Make sure to practice by modifying your program—change the printed message or introduce basic conditional statements. Each alteration cements your knowledge deeper, preparing you for more complex tasks ahead.
Debugging and Error Handling in Java
Understanding debugging and error handling in Java is crucial for anyone starting their programming journey. Even a small typo can lead to errors that halt a program's execution. Java provides tools and techniques to help developers find and correct bugs efficiently.
Debugging is the process of identifying and fixing bugs to make sure the code runs as intended. It enhances the learning experience and builds problem-solving skills.
Common Errors and Exceptions
In Java, errors and exceptions can arise from various issues. Here are the some common ones you may encounter:
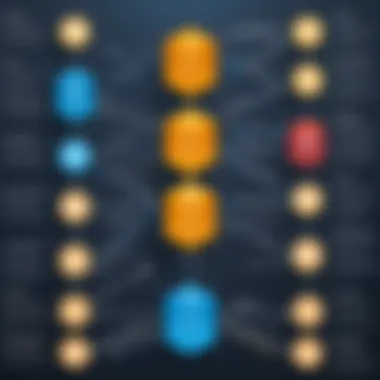
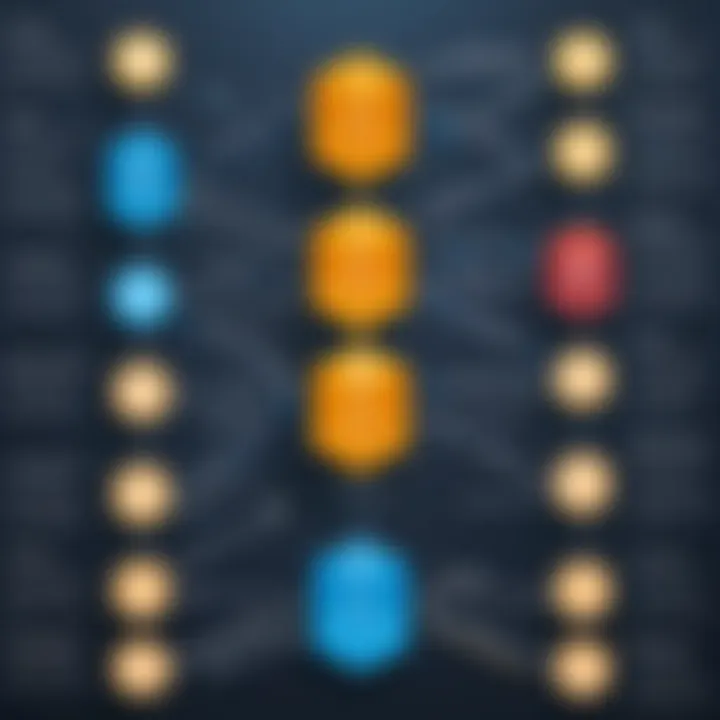
- Syntax Errors: These occur when the code does not follow Java's language rules. For example, a missing semicolon can lead to a syntax error.
- Runtime Errors: These happen during execution. For instance, trying to access an element in an array outside of its bounds can throw an ArrayIndexOutOfBoundsException.
- Logic Errors: These are more complex as they don’t stop the program but yield incorrect results. Understanding your program logic profoundly can help minimize these errors.
Java has built-in exceptions such as NullPointerException and IOException that offer context when things go wrong, making the debugging process a bit more manageable.
Practice Exercises for Reinforcement
Practical experience is vital when learning any programming language, including Java. Exercises reinforce theories by allowing learners to directly apply concepts. This technique helps a solidify understanding and improve retention. When students engage in hands-on activities, the complexities of java programming become clearer.
Several aspects make practice exercises essential in the learning process:
- Application of Knowledge: By solving real-world problems, learners can see how theoretical concepts work in practice.
- Error Recognition: Encountering common errors during exercises makes learners familiar with debugging. This enhances problem-solving skills.
- Confidence Building: Completing tasks boosts learners' self-efficacy in programming. The sense of accomplishment inspires further exploration.
- Cementing Concepts: Engaging regularly in exercises leads to greater command over Java syntax and structures, aiding moving to advanced topics more smoothly.
Success in exercising boosts motivation to progress towards more complex topics, setting strong foundational skills.
Hands-On Coding Challenges
Coding challenges provide a platform to test and expand your coding skills. They allow learners to apply concepts in a dynamic environment. Websites such as Codecademy and LeetCode offer a wealth of coding challenges. These challenges are oriented towards enhancing problem-solving capabilities within the scope of Java programming.
Engaging with coding challenges has distinct benefits:
- Variety: There are diverse challenges catering to every level. You can start easy and gradually tackle harder problems.
- Peer Support: Many coding challenge platforms have active communities. Engaging with these peers can provide insights and support.
- Instant Feedback: Coding platforms usually allow for instant checking of a solution, enabling iteration and learning from mistakes immediately. This feedback loop contributes to effective learning.
Here are a few example challenges learners might encounter:
- String Manipulations: Create methods to reverse strings or check for palindromes.
- Collection Handling: Write functions using Java's Collections for sorting or filtering data.
- Basic Algorithms: Implement algorithms like sorting (Bubble sort, Selection sort) and searching (Binary search)
These challenges enhance critical thinking and spur innovative solutions, applying what has been learned.
Building Small Projects
Moving from practicing hands-on coding to creating small projects signifies an important step in skill development. Small projects demonstrate how Java components come together in practical applications. They encapsulate learning and result in tangible outcomes that showcase abilities.
When building small projects, consider the following:
- Choose Simple Ideas: Something manageable, like a to-do application or a basic calculator, is ideal. Keep the goals achievable initially.
- Learn through Failure: Projects will have bugs and issues. Each challenge faced fosters learning and introspection.
- Utilize Version Control: Start using a system like Git. It allows you to track project changes and learn versioning, which is key in programming environments.
- Expand as Skill Increases: Once basics are completed, expand functionalities to your projects. Aim to incorporate features like user input validation or data persistence.
Finished projects stand as a testament to past work. Sharing them on Github not only showcases efforts but also allows for exploring community feedback, enticing a social collaboration aspect to learning.
By engaging in hands-on challenges and building projects, you elevate your coding skill level and gain essential experiences vital for adept Java programming.
This practice section facilitates applying instructional lessons into personal experience, ensuring learners fully utilize their new skills towards real programming challenges.
Resources for Further Learning
Learning Java extends beyond the basic syntax and programming models. The journey involves continuously updating one’s knowledge and adapting to new developments in the programming landscape. In this regard, utilizing various resources for further learning is critical. Resources come in multiple formats and address different facets of Java learning. Accessibility, depth of information, and interactivity are key considerations in this section.
Online Courses and Documentation
Online courses serve as an excellent starting point for many learners. They offer structured content designed to guide students through the intricacies of Java programming step by step. Websites like Coursera and edX provide comprehensive Java courses subdivided into manageable modules. Often, a mix of video lectures, quizzes, and assignments forms an effective mosaic for effective learning.
Furthermore, Oracle offers extensive documentation detailing programming fundamentals and advanced concepts in Java. This documentation is the authoritative resource written by those who create and maintain Java itself. Issues relevant to version updates are documented here, enabling effective learning aligned with the latest industry standards.
A few notable online course platforms include:
- Coursera - Offers a variety of Java courses from well-known universities.
- Udacity - Provides nano degrees focused on Java development.
- Pluralsight - Features expert-created quests for a detailed learning experience.
Community documentation on websites like Stack Overflow is invaluable too. By accessing questions and solving issues related to programming tasks, learners can see practical applications of Java concepts. This way of learning cultivates problem-solving skills necessary in real-world scenarios.
Books and Community Forums
Books are a time-tested resource for structured and focused learning. They often cover the subject matter in sequential logic, which can greatly benefit a learner hoping not to miss key concepts. Authors such as Cay S. Horstmann and Herbert Schildt have written approachable guides like Core Java Volume I and Java: A Beginner’s Guide, respectively.
Books not only encompass theoretical knowledge but also include practical exercises. Exercises in these books provide hands-on experiences which reinforce learning outcomes. Readers should choose books that align with their learning stages and specific interests in public-facing Java applications, back-end software development, or mobile app programming.
Pursuing community forums such as Reddit, where substantial discourse takes place, can greatly enhance learning. Subreddits like r/java offer community insights, new methodologies, and practical advice from those currently practicing in the field. Engaging with professionals here opens doors for networking and further insights into what tools or best practices might be beneficial.
Collaboration fosters a learning environment. Whether through credits of online environments or mutual learning with peers, community forums and educational literature pacify the vain thirst for understanding and enhancing one’s Java journey.
Closure and Next Steps
Learning Java in a single day presents both challenges and opportunities for aspiring programmers. As you conclude this guide, it's important to understand that this journey does not end here. The steps you have taken laid a strong foundation, yet mastery will demand further exploration and practice. Assessing your learning effectively ensures that you can leverage acquired skills for future endeavors in technology.
Reflecting on Your Learning Journey
When you step back to reflect on your learning experiences, consider what concepts resonated most with you. Did certain subjects provoke deeper interest? Reflective practice can deepen understanding. Consider keeping a journal to note questions that arise as you engage with Java. Document insights and challenges you face during programming tasks. This will serve as a roadmap for your learning.
Assessment of your initial programming capabilities helps in identifying areas requiring further improvement. Reflection also highlights your strengths, enabling you to build upon them effectively. Exploring online forums, such as Reddit, can provide valuable community insights.
Setting Goals for Advanced Learning
With the basics of Java under your belt, it is crucial to set defined goals for advancing your skills. Craft specific and achievable targets that challenge you. Perhaps you wish to master object-oriented programming, data structures, or even game development using Java.
Some useful approaches include:
- Following structured online courses: These can supplement your knowledge with in-depth material. Websites like Coursera, Udacity, or even free resources contribute to a well-rounded learning structure.
- Engaging in projects: Building applications allows practical growth. Start with simple projects, like a calculator or task list, and gradually move to more complex applications.
- Joining community coding contests: Platforms like CodeWars and LeetCode provide engaging ways to practice and apply your knowledge.
Setting timelines for each goal can strengthen your discipline. Embrace the learning journey with persistence and curiosity. Each effort will sharpen your programming ability, ideally positioning you for success in various fields. Remember that learning is an ongoing voyage, and with Java, the possibilities are extensive.