Mastering Java: A Beginner's Comprehensive Guide
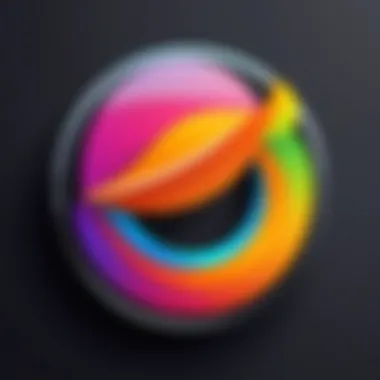
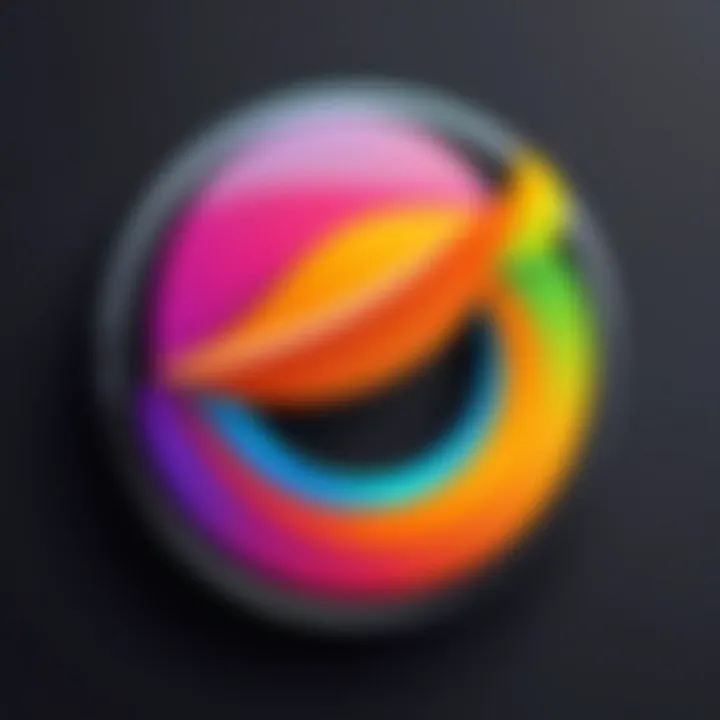
Intro
Prelude to Programming Language
Learning a programming language like Java can seem overwhelming for beginners, but understanding the fundamentals makes it manageable. Java has a rich history and has become a staple in the programming community.
History and Background
Java was created by Sun Microsystems, with its first release in 1995. The goal was to develop a language that could run on various platforms without needing specific adaptations. This led to the development of the Java Virtual Machine, which executes Java bytecodes.
Features and Uses
Java is known for its portability, which means code can run on any system with a Java Virtual Machine. Its features include ease of use, robustness, and security. Common uses of Java include web applications, mobile applications, and large-scale systems in enterprises.
Popularity and Scope
According to various surveys, Java consistently ranks among the most popular programming languages worldwide. It is widely taught in schools, used in Android app development, and implemented in server-side technologies. Its framework ecosystem including Spring and Hibernate adds to its versatility, making it applicable for various domains.
Basic Syntax and Concepts
Grasping the basic syntax and core concepts of Java is essential for any beginner. Understanding variables, data types, operations, and control flow allows learners to write simple and effective programs.
Variables and Data Types
In Java, a variable stores information that can change during execution. Common data types include:
- int for integers
- double for floating-point numbers
- char for characters
- boolean for true/false values
Here's an example of declaring variables:
Operators and Expressions
Java supports various operators that perform operations on variables and values. Basic operators are:
- Arithmetic operators like +, -, *, /
- Relational operators like ==, !=, >,
- Logical operators like && (AND), || (OR)
Expressions are combinations of variables and operators that produce a value.
Control Structures
Control structures dictate the flow of execution in programs, and are crucial for decision-making and looping operations. Some basic control structures include:
- if-else statements for conditional branching.
- for loops, used for iterating over a range of values.
- while loops, which execute a block while a condition remains true.
Advanced Topics
After mastering the basics, one can explore advanced functionalities of Java, such as methods, objects, and handling exceptions.
Functions and Methods
Solidifying your understanding of functions allows you to structure programs better. In Java, methods can take parameters and return values. Example:
Object-Oriented Programming
Java is an object-oriented language, promoting code reusability through its class-based structure. Key principles of OOP include:
- Encapsulation
- Inheritance
- Polymorphism
Exception Handling
Understanding exceptions helps create robust Java programs. Using , , and can manage runtime errors gracefully.
Hands-On Examples
Once you've read about concepts, practicing through examples builds understanding and confidence.
Simple Programs
Creating simple Java programs, like a calculator or Hello World, is a good starting point for practice.
Intermediate Projects
As your skills improve, tackle projects such as a todo list application or a simple game. Projects yield practical insight into the language’s features and capabilities.
Code Snippets
Here is a simple Hello World program:
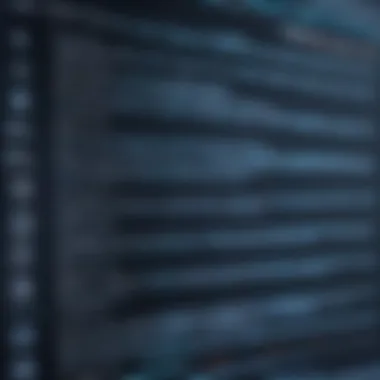
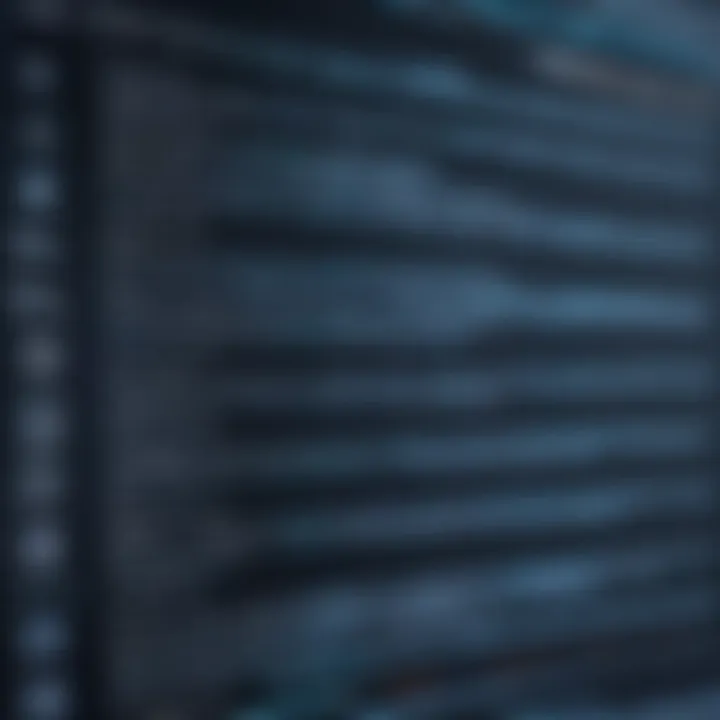
Resources and Further Learning
To truly master Java, utilizing quality resources is vital. Here are some suggestions:
Recommended Books and Tutorials
- Head First Java by Kathy Sierra and Bert Bates.
- Effective Java by Joshua Bloch.
Online Courses and Platforms
Look into udemy.com and Coursera, where multiple courses offer structured content from expert instructors.
Community Forums and Groups
Joining forums such as reddit.com or local programming meetups can enhance your learning through real-world discussions and mentorship.
Prologue to Java
Java is a programming language that has stood the test of time. Its versatility and reliability make it pivotal in modern software development. This introduction serves not only to familiarize you with the core principles of Java but also to highlight its enduring significance across various sectors. As you ponder the prospect of learning Java, consider the vast expanse of opportunities it can unlock.
Importance of Java in Modern Development
Java's impact in today's technology landscape cannot be overstated. As a platform-independent language, it is widely used to build applications on a variety of devices. From servers to mobile devices to embedded systems, Java provides a seamless way to develop across multiple platforms. This flexibility caters specifically to the growing demand for mobile applications and enterprise-level solutions. Furthermore, Java's object-oriented nature simplifies complex problem-solving by allowing developers to build on existing frameworks.
The community backing and extensive libraries in Java add to its appeal. Developers can depend on robust security features inherent in the language, which is crucial in safeguarding sensitive data. A survey conducted by Stack Overflow in 2023 highlighted that Java consistently ranks as one of the top languages among professionals, leading to high demand among employers.
Investing your time in learning Java today positions you favorably among peers while opening doors toward lucrative job offers tomorrow.
Overview of Java Language Features
Java encompasses several key features that set it apart from other programming languages. Understanding these attributes is essential as you embark on your journey.
- Platform Independence: Java applications can run on any device equipped with a Java Virtual Machine (JVM). This eliminates the hassle of worrying about the underlying hardware.
- Object-Oriented Approach: It fosters better organization and management of software concerns, promoting reusable and modular code.
- Memory Management: Java's garbage collector automatically manages memory allocation which helps eliminate many common programming errors.
- Rich Standard Library: Java offers a comprehensive set of libraries and tools that facilitate application development without the need for third-party solutions.
- Strongly Typed: With strict type checking, Java helps minimize runtime errors which can lead to more stable applications.
By grasping these features, learners can appreciate why Java remains a powerful tool for developers aiming to create robust applications in diverse domains.
Understanding Java's features allows you to adapt your programming strategies as you encounter various challenges while coding.
Setting Up Your Environment
Setting up your environment is fundamental for learning Java effectively. This step cannot be overlooked as it lays a solid groundwork for your programming endeavors. A well-structured environment fosters a seamless workflow and enhances productivity. You need the right tools to write and test your code without interruption. This contributes significantly to learning speed and comprehension.
Installing Java Development Kit (JDK)
The Java Development Kit, often abbreviated as JDK, is the primary tool you should install first. It includes all the necessary tools for writing, debugging, and running Java programs. This kit contains the Java Runtime Environment (JRE), the compiler, and other vital components. To commence your Java learning journey, start by downloading the appropriate version of the JDK from Oracle's official website. Following the installation prompts, the kit should be installed on your system effortlessly.
When you have JDK installed, set up the Java Environment Variables. This allows your operating system to locate the JDK installation, letting you run Java programs from the command line. Open Settings, search for 'Environment Variables', and properly configure them based on your installation directory. This step ensures that you can compile and execute Java programming from any terminal or command prompt with ease.
Choosing an Integrated Development Environment (IDE)
An Integrated Development Environment is another essential component in the setup process. An IDE provides a user-friendly interface that facilitates coding, offering features such as code highlighting, auto-completion, and debugging tools. These features can save you time and help you avoid many beginner pitfalls. As a student or someone new to Java, an IDE will make your learning curve less steep and more enjoyable.
Popular IDEs for Java
Among the most widely used IDEs for Java, Eclipse and IntelliJ IDEA stand out. Eclipse is known for its robustness and is available as a free and open-source option. It offers extensive functionalities, making it a good choice for novice programmers. IntelliJ IDEA, though a paid option, has a free community version that is highly favored for its user-friendly design and intelligent code completion.
Both of these IDEs have unique features. For example, IntelliJ provides live templates and a well-integrated graphical debugger. On the contrary, Eclipse's flexibility with plugins allows for extensive customization. Each of these qualities plays a significant role in shaping the coding experience.
Features to Look for in an IDE
When selecting an IDE, several features are crucial for a productive programming environment. First, strong code navigation is essential. This feature simplifies tracing and exploring your code efficiently. Additionally, look for robust debugging tools. Integrated debugging can streamline finding issues in your code, allowing for learning through inspection.
Version control integration is also a noteworthy feature. It enables collaboration and code history management. Tools such as Git can be easily integrated into the IDEs listed. Familiarizing yourself with these tools is beneficial as you progress deeper into your Java learning.
In summary, setting up a conducive environment includes installing the Java Development Kit and selecting a suitable Integrated Development Environment. These efforts equip you for an effective and enjoyable Java learning experience, allowing you to quickly grasp and apply critical concepts in your projects.
Learning the Basics of Java
Learning the basics of Java is an essential stepping stone for anyone beginning their journey in programming. This foundation goes beyond mere syntax; it serves as the architecture for understanding more complex concepts in Java. By grasping these core elements, learners can build confidence and proficiency, enabling them to tackle more advanced topics subsequently.
As Java is a widely-used programming language in various domains—from web development to mobile applications—understanding the basics opens up various career opportunities. The clarity in Java’s structure supports newcomers without becoming overly complex, streamlining the learning process for beginners.
Java Syntax and Structure
Java’s syntax is one of its most fundamental components. Understanding syntax involves knowing how to accurately write commands and code blocks. This knowledge acts as the language's backbone, allowing developers to create functional programs. Clear syntax also promotes easier debugging and better readability.
The structure of Java code follows a straightforward pattern. For beginners, using clear conventions like indentation and comments to organize code ensures readability. Learning these elements empowers developers to write better code and collaborate with others efficiently.
Data Types and Variables
Data types in Java represent different kinds of information. They define the values your variables can hold. This includes primitive types like int, char, and double, as well as reference types. Understanding data types is crucial since it influences memory management and performance.
Declaring variables allows Programmers to store and manipulate data. Mastering the concept of variables prepares beginners to handle inputs and outputs while boosting logical thinking. Given the straightforward syntax, beginners find it relatively easy to learn how to declare variables in Java, fostering a strong programming practice early on.
Control Flow Statements
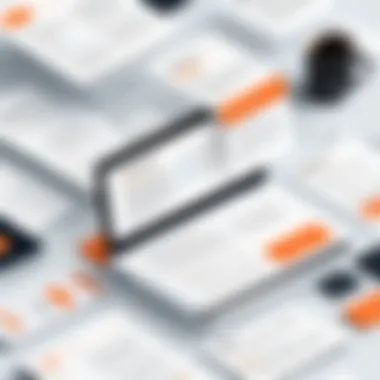
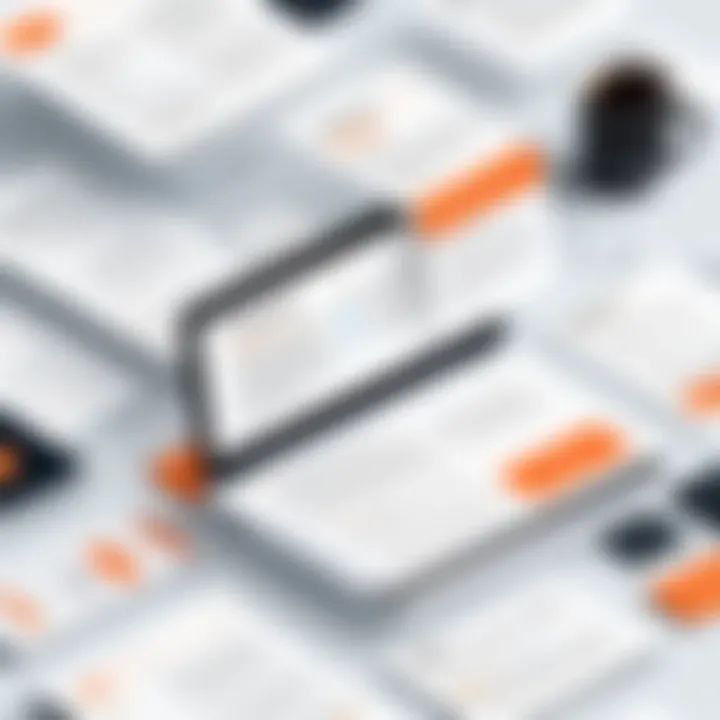
Control flow statements enable programmers to control the execution of code based on specified conditions, effectively guiding their program's logical path. Mastering these elements is critical since they form the basis of decision making in programming.
If Statements
The if statement is a fundamental part of Java's control flow mechanism. It allows the code to execute certain parts based on boolean expressions. This feature empowers developers to create dynamic applications based on user input or other conditions.
A key characteristic of if statements is their simplicity. This makes them a popular choice for implementing logic in programs. A unique aspect of if statements is their flexibility, allowing developers to nest multiple conditions for complex decision making. For beginners, understanding if statements simplifies the approach to various programming tasks, paving the way for success.
Switch Statements
Switch statements provide an alternative to if statements, offering a more structured way to perform multiple path evaluations using a single variable. This syntactic approach can enhance code organization and readability.
The characteristic neatness of switch statements makes them a beneficial choice when dealing with multiple specific conditions rather than large sets of variables. Their unique feature is the case structure, which leads to concise logic. While beneficial, beginners should evaluate scenarios closely, as switch statements can be less flexible compared to if statements if conditions become more complex.
Loops (For, While)
Loops are powerful programming constructs that allow for repeated execution of block of code under certain conditions. Utilizing loops effectively helps reduce redundancy in coding and supports tasks such as iteration over collections.
Both for and while loops have unique characteristics. The for loop is typically used where the number of iterations is known. This makes it a popular tool for scenarios like array traversal. On thje other hand, while loops are structured to continue based on a boolean expression, offering greater flexibility but requiring careful attention to avoid infinite iterations.
Consideration of both types of loops fosters good coding habits. Recognizing when to use for versus while can impact program efficiency and readability, thus is invaluable for beginners.
Mastering control flow statements equips beginners with essential skills in logical reasoning; this skill tremendously benefits in future programming endeavors.
Understanding Object-Oriented Programming
Understanding Object-Oriented Programming (OOP) is critical for new learners of Java. OOP helps structure code in a way that models real-world concepts. This approach significantly enhances the reusability of code. It also improves the maintainability of software projects as they scale. Beginners must grasp OOP principles—not just to write Java code, but to write it effectively.
Furthermore, OOP principles pave the way for cleaner designs and more intuitive software architecture. Learning these concepts prepares learners for larger frameworks in Java, such as Spring or Hibernate, which thrive on OOP paradigms. Thus, comprehending OOP provides a foundational knowledge that extends beyond Java into broader programming practices.
Core Principles of OOP
The four core principles of OOP include encapsulation, inheritance, polymorphism, and abstraction. Each of these principles plays a distinctive role in how Java programs are structured.
Encapsulation
Encapsulation refers to bundling the data and methods that work on that data within a single unit or class. This principle enhances security by restricting access to certain components of an object. A key characteristic is the use of access modifiers, allowing you to control visibility. This restrictiveness is a beneficial approach because it helps protect the object's state from unintentional interference and misuse.
The unique feature of encapsulation in Java is that it allows classes to hide internal states and require all interaction to be performed through specified methods (getters and setters). This creates a tighter control over how important data is accessed and modified. While encapsulation can lead to higher upfront development complexity, the long-term benefits far outweigh these initial challenges.
Inheritance
Inheritance is the mechanism that allows one class to inherit properties and behaviors from another. Many developers favor inheritance for its ability to promote code reusability and organization. The key characteristic here is the
Advanced Java Concepts
Advanced Java Concepts are crucial for developing robust applications and enhancing your programming proficiency. This section discusses two essential elements: Exception Handling and the Java Collections Framework. Understanding these topics allows learners to manage errors effectively and utilize data structures optimized for Java applications.
Exception Handling
Exception Handling in Java is a mechanism to gracefully manage unexpected events during program execution. This practice enhances the reliability of applications. When errors occur, Java provides built-in tools to catch and deal with them instead of allowing the whole program to terminate abruptly. The following benefits underscore its importance:
- Improved Stability: Programs continue to work even if an error occurs.
- Error Tracking: Developers have tools to precisely locate problems in code.
- Clearer Code: By isolating error handling logic, code becomes easier to read and maintain.
A typical way to handle exceptions involves using blocks. Here is a basic example:
Utilizing this approach enables your applications to manage uncertainties effectively, creating a better experience for end-users.
Java Collections Framework
The Java Collections Framework provides a set of classes and interfaces designed for storing and managing groups of objects. It is fundamental for any serious programming endeavor in Java, as it simplifies the handling of data structures. The primary features include:
- Efficiency: Handling data collections becomes simpler and faster.
- Flexibility: Various types of collections (like lists, sets, and maps) cater to diverse needs.
- Scalability: Applications efficiently manage high volumes of data.
Some frequently used interfaces and classes include the following:
- : Implemented by classes such as and for ordered collections.
- : Used in and for collections without duplicates.
- : Represents key-value pairs with classes like and for fast lookup.
It is essential to choose the right data structure based on your application needs, as different structures can affect performance and memory usage significantly. In summary, mastering Exception Handling and the Java Collections Framework equips beginners with the necessary skills to become adept in Java programming, enabling them to tackle real-world challenges more efficiently.
Remember, strong applications focus not just on what data is processed, but also on how errors are managed and how data is efficiently organized.
Practical Java Programming
Practical Java programming is a cornerstone of learning this versatile programming language. Understanding theoretical aspects is important, but applying that knowledge through real coding challenges cements that understanding. Students and aspiring programmers benefit immensely from this practical approach as it transforms abstract concepts into tangible skills.
In this section, we will delve into two critical elements of practical Java programming: first, writing your initial Java program, and second, gaining proficiency in debugging and testing applications. These skills are essential not only for immediate learning results but also for future development practices.
Writing Your First Java Program
Writing your first Java program is often considered a rite of passage in the learning journey. This program typically involves a simple 'Hello, World!' output, which serves as an introduction to Java syntax and execution. This straightforward program lays the foundation for more complex project creation down the line.
Key Points to Follow:
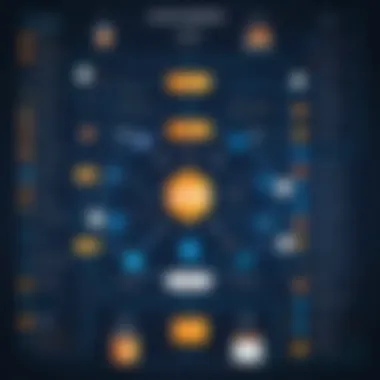
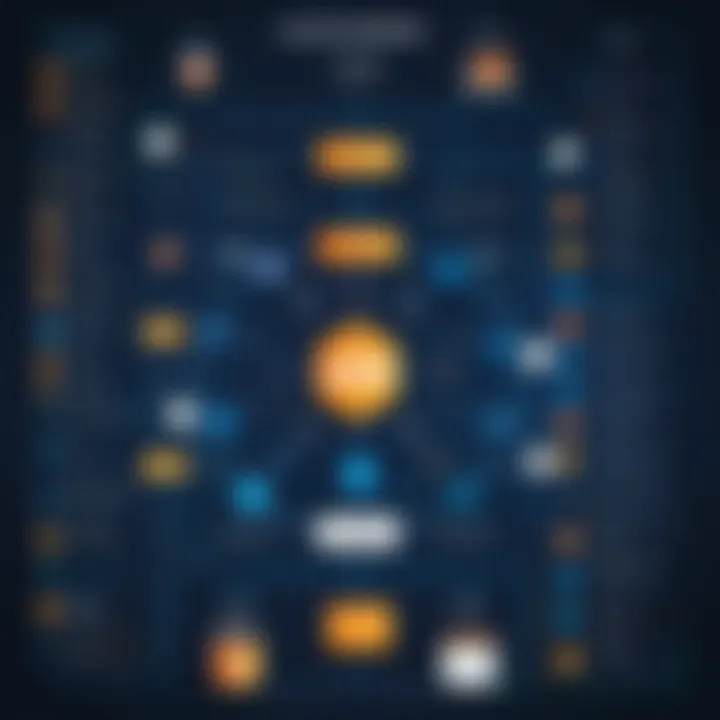
- Set up your environment: Ensure the Java Development Kit (JDK) and Integrated Development Environment (IDE) are properly installed. Choose an IDE that you find user-friendly, such as Eclipse, IntelliJ IDEA, or NetBeans.
- Create a new project: Launch your IDE, and start a new Java project. This can often be done via a menu option or toolbar icon.
- Write the code: In the new file, input the following code:This code defines a new class 'HelloWorld' with a main method that performs actions. The function prints text to the console.
- Compile and run: After writing the code, compile it within your IDE. You may encounter errors if there are any syntactical issues. Once the code successfully compiles, run the program.
- Observe the output: In the console, you should see 'Hello, World!' This affirmation demonstrates that you correctly executed your first program, marking an important milestone in your programming journey.
Writing this first program is crucial. It not only familiarizes you with the fundamental syntax of Java but also introduces you to the developmental process. Practicing further modifications, like changing the displayed text or introducing variables, can enhance your comprehension and confidence as a programmer.
Debugging and Testing Java Applications
Debugging is a vital skill in programming, as it helps identify and rectify errors in your code, ensuring that your application runs smoothly. Additionally, testing allows you to validate that the program operates as intended before deployment.
Important Strategies:
- Incremental Development: Write your code in small increments rather than all at once. This method allows you to catch errors quicker.
- Use a Debugger: Most IDEs offer integrated debugging tools. These tools allow you to set breakpoints in your code, inspect variables, and navigate through execution flows step-by-step.
- Common Print Statements: Often, simply printing out values at various parts during execution offers insights into where things may be going wrong. This approach is less formal than using a debugger but can be very effective.
- Understanding Exception Handling: Learning to use try-catch statements will allow your program to continue running in the event of an error, as well as let you respond to those errors gracefully. Users should not encounter abrupt program terminations.
Debugging is not just about fixing errors. It is about understanding why the error happened in the first place, which enhances our problem-solving skills.
In sum, debugging and testing your Java applications refine your coding abilities. You will gain experience in problem identification, gain familiarity with your tools, and as you progress, you will approach errors with confidence and clarity.
By incorporating practical programming activities into your learning routine, you set the stage for continued growth and mastery in Java.
Utilizing Resources for Learning
Utilizing various resources for learning is paramount for beginners venturing into Java programming. Learning a language such as Java demands a multilayered approach. Students must tap into different materials and platforms tailored to their learning styles and needs. Consequently, leveraging diverse resources not only accelerates comprehension but ensures comprehensive mastery of programming concepts.
Moreover, each medium provides unique advantages that enhance the overall educational experience. Ensuring an understanding of the requirements and functionalities involved in each type of resource is essential to optimize one’s knowledge gain.
Online Tutorials and Courses
Online tutorials and courses present a structured way to learn Java. These platforms offer interactive learning experiences that guide you from the fundamentals to more advanced topics, promoting a more engaging learning process.
Popular online platforms such as Coursera, Udacity, and Codecademy provide a wealth of resources. Here are some benefits:
- Self-Paced Learning: Modules are generally available on-demand, giving students freedom over their learning speed.
- Visual Aids: Videos and visuals can represent code snippets, making code logic clearer compared to traditional text.
- Practical Assignments: Many courses include projects as assignments, allowing hands-on experiences to reinforce learning.
When selecting a course, it is wise to look for curated content. Tutorials like “Java Programming Masterclass” on Udemy prioritize quality, teaching essential concepts that every Java learner must grasp.
Books and Documentation
Books and formal documentation present foundations in Java programming. They provide in-depth knowledge with considerable nuances usually overlooked in other formats. A few well-regarded books include
Building Real-World Projects
Building real-world projects is a critical aspect of learning Java because it transforms theoretical knowledge into practical skills. Engaging in project development encourages problem-solving and deepens your understanding of core programming principles. Moreover, working on tangible applications showcases your abilities to potential employers and can strengthen your portfolio.
Key benefits of building projects include:
- Hands-on experience: Applying what you've learned in real scenarios solidifies concepts.
- Problem-solving skills: Projects will inevitably introduce challenges, enhancing your ability to tackle technical issues.
- Creativity: Developing your unique projects encourages innovative thinking.
- Time management: Completing projects requires planning and execution within deadlines, teaching important professional skills.
When taking on projects, beginners should carefully consider the complexity and scope to avoid being overwhelmed. Properly balancing challenge with your current skill level promotes sustained interest and learning.
Identifying Project Ideas for Beginners
Selecting the right project is crucial for effective learning. Beginners should choose ideas that are manageable yet educational. Here are some suggestions:
- Basic calculator: A simple console application to reinforce basic syntax and understanding of data types and control flows.
- Todo list application: An interactive program that introduces Java Collections and data handling.
- Guess the number game: A fun way to practice decision-making control structures.
- Simple personal diary: A more complex structure to manage user input, file handling, and more intricate data management.
A project need not be complex to provide value. Always prioritize projects that ignite your passion. Such enthusiasm will sustain your learning journey.
Project Structure and Organization
Organizing a project is essential for smooth development and maintenance. Good structure can make the difference between a functional product and one that becomes unwieldy. Below are essential considerations for structuring your Java project:
- Use packages: Group related classes into packages to enhance clarity and maintainability.
- Follow naming conventions: Clear, descriptive names for classes and methods improve readability and debugging.
- Implement version control: Utilize tools like Git to track changes and collaborate easily.
- Include documentation: Add in-line comments and external documentation to explain your decisions for future reference and for other developers.
Structured projects not only benefit you but also others reviewing your code.
Attention to detail and a well-defined folder structure reflect professionalism. This trait is attractive to future employers.
Building real-world projects allows beginners to confront practical challenges while gaining confidence. Through this process, they will navigate the nuances of Java and emerge more competent in their programming journey.
Culmination and Future Learning
In concluding this guide on learning Java, it's vital to recognize that this programming language transcends mere syntax and structures. Mastering Java opens a pathway to understanding software development principles and better prepares you for challenges ahead. Learning should not be viewed as a fixed goal but rather as a lifelong journey, especially in a field that evolves constantly. Knowledge of Java can enhance your employment opportunities and boost your confidence in tackling more complex coding challenges.
Continuing Your Java Education
Continuing your education in Java involves deepening your grasp on topics you have previously encountered. Engage with advanced concepts such as Java Streams, Lambda Expressions, and Multi-threading. Here are several effective methods:
- Practice Regularly: Everyday coding practice is crucial. Build new projects or contribute to open-source initiatives. This offers real-world experience and enhances your problem-solving skills.
- Join Online Courses: Utilize platforms like Coursera or Udemy. They offer tailored courses that allow you to learn at a comfortable pace.
- Read Advanced Books: Picking up books such as "Effective Java" by Joshua Bloch can give you great insights into best practices.
- Participate in Coding Challenges: Websites like LeetCode and HackerRank provide problems that test your skills rigorously. It forces you to think creatively and improve.
By incorporating these aspects into your routine, you solidify the foundations of your Java knowledge and set the stage for advancing your career.
Exploring Related Technologies
As you grow more comfortable with Java, consider exploring other technologies that complement your skills. The programming landscape is diverse, and familiarity with different tools can enhance your productivity and broaden your employment opportunities. Here are a few you might want to investigate:
- Spring Framework: Specifically for Java developers, Spring reduces the complexity in managing server-side applications and empowers you to build enterprise-level applications with ease.
- JavaScript: Since most web applications utilize JavaScript for front-end work, knowing it will make you a full-stack developer.
- Database Management: Understanding SQL or NoSQL databases allows you to grasp how Java applications interact with data effectively.
- Cloud Technologies: Familiarity with AWS or Azure opens your paths as cloud deployments become critical in application development.
By delving into these technologies, you enhance your marketability and keep your skills relevant in an ever-changing industry.
Reflecting on your learning journey is important. Take note of your growth, your successful projects, and areas where you need improvement. This not only measures progress but also motivates further learning.