Comprehensive Overview of Laravel for Web Development
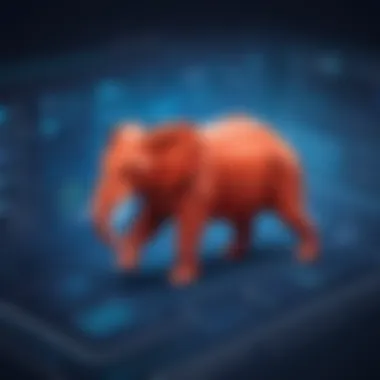
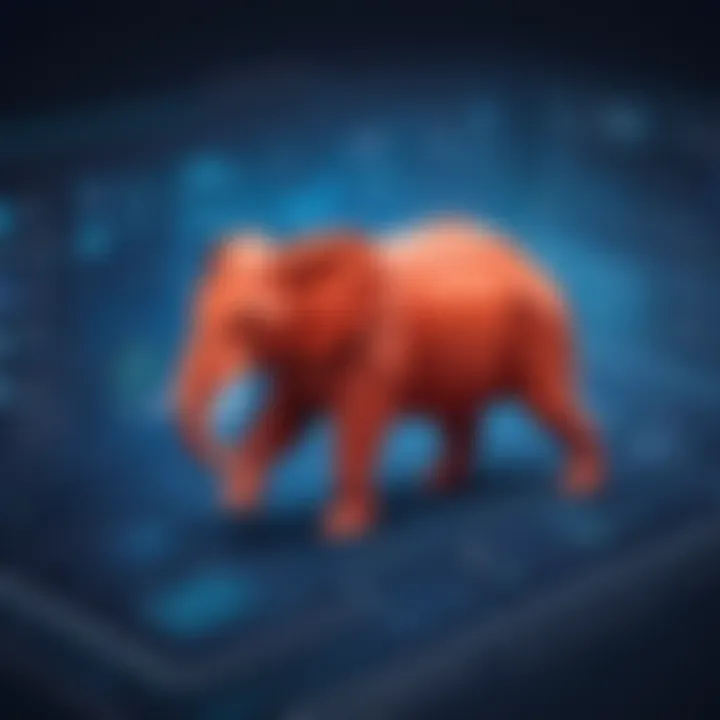
Prologue to Programming Language
Laravel is a modern PHP framework specifically designed for web development. Its robust set of features simplifies common tasks, making it a popular choice among developers.
History and Background
Laravel was first introduced by Taylor Otwell in 2011. It was created to provide a more advanced framework for web application development than the existing tools available at the time. The framework has evolved through several versions, continually incorporating feedback from the developer community. Today, Laravel is recognized for its elegant syntax and expressive tools, which streamline the development process.
Features and Uses
Laravel includes several key features that enhance the development experience:
- MVC Architecture: Laravel follows the Model-View-Controller design pattern, which organizes code efficiently.
- Eloquent ORM: This object-relational mapping feature allows developers to interact with databases using PHP syntax rather than SQL queries.
- Routing: Laravel offers a straightforward routing mechanism that allows custom URL definitions, contributing to cleaner and more manageable code.
- Blade Templating Engine: Laravel's templating engine provides a clean way to manage HTML and PHP code, making the views more readable.
- Testing: Laravel provides built-in testing support that encourages developers to create tested and reliable applications.
Popularity and Scope
Laravel has gained significant popularity in the web development community. Its ability to handle a range of projects—from small applications to large-scale enterprise software—makes it versatile. Many developers appreciate its comprehensive documentation and community support, facilitating easier problem-solving. As of now, there are numerous Laravel-based applications in production, underscoring its relevance in the field.
Basic Syntax and Concepts
Understanding Laravel begins with its basic syntax and core concepts of PHP. Although tailored for web development, familiarity with PHP is essential.
Variables and Data Types
Variables are fundamental in programming. In Laravel, PHP variables follow traditional naming conventions. The basic data types in PHP include integers, strings, arrays, objects, and more. For example:
Operators and Expressions
Laravel supports various operators like arithmetic, comparison, and logical operators. Mastering these allows for building complex expressions. For instance:
Control Structures
Control structures enable developers to dictate the flow of the application. This includes if-else statements, loops like for and foreach, and switch statements.
Advanced Topics
As developers become more comfortable with Laravel, delving into advanced topics becomes essential. This helps in harnessing the framework's full potential.
Functions and Methods
Functions promote code reusability in Laravel. Defining a function encapsulates logic which can be executed multiple times without rewriting code. Methods are similar but exist within classes, following the principles of object-oriented programming.
Object-Oriented Programming
Laravel leverages object-oriented programming principles effectively. Utilizing classes and objects ensures clearer and more logical code organization. In Laravel, models often represent objects that interact with database records.
Exception Handling
Exception handling is crucial in any application. Laravel's built-in exception handling helps maintain application stability by managing errors systematically. Using try-catch blocks, developers can prevent application crashes while providing user-friendly error messages.
Hands-On Examples
Learning through practical examples aids comprehension and skill development.
Simple Programs
For beginners, start by creating simple applications to familiarize oneself with Laravel's syntax and features. A basic "Hello, World!" can be a good introduction.
Intermediate Projects
As skills advance, consider building more complex applications. A small blog application can help learn how to implement features like CRUD (Create, Read, Update, Delete).
Code Snippets
Here’s a quick code snippet demonstrating how to define a route in Laravel:
Resources and Further Learning
To deepen understanding and skills in Laravel and web development:
- Recommended Books and Tutorials: "Laravel: Up & Running" by Matt Stauffer provides a solid foundation.
- Online Courses and Platforms: Websites like Laracasts offer excellent video tutorials on various Laravel topics.
- Community Forums and Groups: Engaging with communities on Reddit or Laravel-specific forums can enhance learning and foster connections with other developers.
"Laravel is a framework that enables developers to build web applications in a streamlined, efficient manner."
This comprehensive overview reflects essential insights into Laravel as a powerful tool for modern web development.
Prelims to Laravel
Laravel is a prominent framework in the realm of web development, making it crucial for developers to familiarize themselves with its capabilities and features. Understanding Laravel provides insight into how modern web applications are built efficiently and effectively. This section will explore its historical context and core principles, illuminating its significance in contemporary development practices.
History and Development
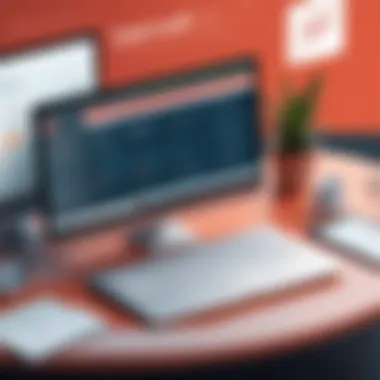
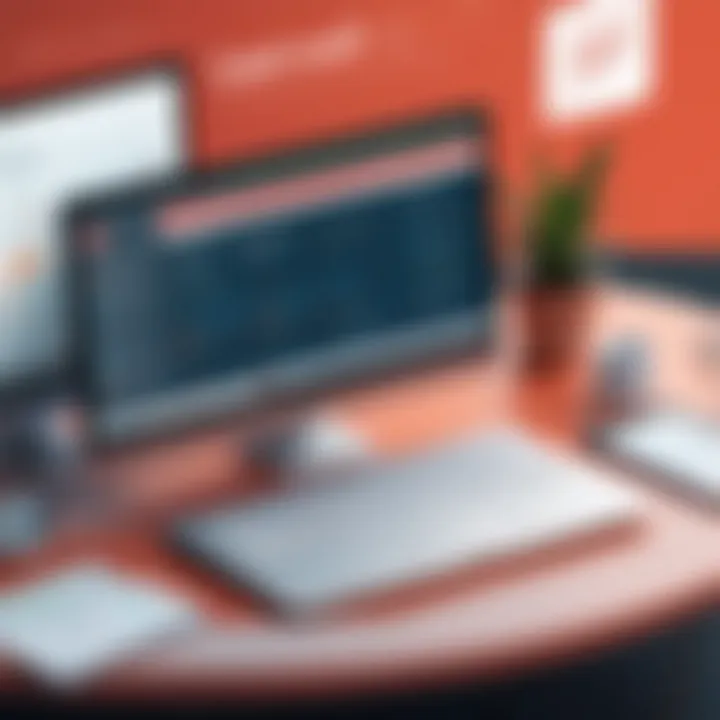
Laravel was created by Taylor Otwell and first released in June 2011. Its inception was motivated by the desire to provide a more advanced alternative to the CodeIgniter framework. Over the years, Laravel has evolved, gaining popularity rapidly among developers. Each new version introduces substantial enhancements, ranging from improved routing capabilities to built-in support for tasks like authentication and caching.
Laravel's growth can be attributed to its strong community support and its commitment to continuous improvement. This has led to numerous resources, including extensive documentation, forums, and online courses that help both beginners and advanced users navigate its functionalities. As Laravel continues to develop, it remains responsive to the changing needs of web developers, making it an essential tool in the industry.
Core Principles of Laravel
Laravel is built upon several core principles that guide its design and functionality:
- Elegant Syntax: Laravel emphasizes a clean, expressive syntax that enhances developer productivity. This simplicity allows developers to focus on building applications without getting bogged down by complex code.
- Convention Over Configuration: This principle minimizes the time spent on configuration and instead relies on sensible defaults. This approach allows developers to follow best practices without excessive overhead.
- Dependency Injection: Laravel promotes the use of dependency injection to facilitate better testing and maintenance. It allows for more flexible code that can adapt to change with ease.
- Ecosystem and Community: The Laravel ecosystem includes a wide range of packages, tools, and resources that enhance its functionality. The community actively participates in the framework’s evolution and provides support for users of all skill levels.
"Laravel’s core principles ensure that developers can create robust applications while minimizing the complexity of the codebase."
In summary, Laravel stands out as a versatile framework, offering unique features and principles that set it apart. By embracing these elements, developers can build sophisticated web applications efficiently.
Setting Up a Laravel Environment
Setting up a Laravel environment is a crucial step that forms the foundation of any web development project using this framework. Proper setup ensures that developers can fully leverage Laravel’s capabilities. A well-configured environment aids in efficient development, reduces the likelihood of issues, and facilitates smooth deployment. Understanding the requirements and processes involved is essential for both beginners and seasoned developers.
System Requirements
Before diving into the installation process, it is important to consider the system requirements for Laravel. Laravel is designed to function optimally on specific software configurations. Generally, the key requirements include:
- PHP Version: Laravel requires PHP version 8.0 or greater. This ensures all features run smoothly and that the latest security updates are utilized.
- Composer: It is crucial to have Composer, a dependency manager for PHP, installed. Composer manages libraries and dependencies required for a Laravel project.
- Database: While Laravel supports several databases, using MySQL, SQLite, or PostgreSQL is recommended. Developers should ensure the version of the database is compatible with Laravel.
- Web Server: A compatible web server is needed, e.g., Apache or Nginx. These servers support routing and the handling of requests efficiently.
Additional software such as Node.js and npm may be beneficial for managing front-end assets, especially in projects that utilize a lot of JavaScript.
Installation Process
After meeting the system requirements, the next step is the installation of Laravel itself. The installation process typically follows these steps:
- Install Composer: Ensure Composer is installed on your system. This can be done by downloading it from its official website.
- Create a New Laravel Project: To initiate a new Laravel project, you can execute the following command in your terminal:Here, can be replaced with the desired name for your application.
- File Permissions: Adjust the permissions for the and directories. This is important for the framework to write files properly. You can set permissions using:
- Environment Configuration: Laravel uses an file to manage configuration settings. Configurations such as database connection, application key, and other environment variables can be edited here.
This straightforward installation process allows developers to get started quickly with Laravel, ensuring a solid base for their projects.
Configuring Your First Project
Once Laravel is installed, configuring the first project is the next step. This configuration phase gives developers control over various aspects of their application:
- Setting the APP_URL: In the file, ensure you set the to the correct URL where the application will be accessed.
- Database Connection: Update the file with the appropriate database connection details:
- Generating Application Key: Laravel projects require an application key, which can be generated using:This key is used for various encryption tasks within the application.
Successful configuration allows developers to start building their first web application smoothly. Overall, the setup phase is vital as it lays the groundwork for future development, making this knowledge indispensable for any Laravel developer.
Architecture and Structure of Laravel
The architecture and structure of Laravel is fundamental to understanding its functionality as a robust framework. Laravel adopts the Model-View-Controller (MVC) design pattern, which is pivotal in separating application logic from the user interface. This separation enhances code organization and maintenance, making development more intuitive. Additionally, this structured approach brings clarity to the development process, allowing developers to focus on distinct components without confusion.
Framework Explained
The MVC framework is at the heart of Laravel. It divides the application into three interconnected parts.
- Model: Represents the data and business logic. Models interact directly with the database and provide a way to retrieve data, save data, and perform complex business rules.
- View: This is what the user interacts with. Views present the data to the user in a structured format. It ensures that the user interface remains separate from the application logic.
- Controller: Acts as an intermediary between Model and View. It retrieves data from Models and passes it to Views to display. Controllers handle user requests and input, making them essential in directing traffic within the application.
So, when developers work in Laravel, they can easily pinpoint where to make changes and understand how components interact. In summary, the MVC architecture simplifies the management of complexity in software development.
Routing and Controllers
Routing in Laravel is straightforward and powerful. It allows developers to define the URL patterns their application will respond to. Each route is linked to a controller that contains the logic for handling requests.
For example:
In this example, the URL points to the method in the . This approach enables clear and organized code, making it easy to follow the flow of requests throughout the application.
Moreover, Laravel supports RESTful routes, which align well with modern web application development. This ensures that developers can build APIs that comply with standard practices, further increasing the framework's versatility.
Views and Blade Templating
The views in Laravel are rendered using a powerful templating engine called Blade. Blade allows developers to write clean and efficient templates without compromising performance. It supports features such as template inheritance and sections, making it easy to create consistently styled views.
With Blade, you can write syntax that integrates PHP seamlessly. For instance, the following code demonstrates how to display data passed from the controller:
This simple yet effective syntax helps maintain the clarity of the code. In addition, Blade offers control structures like loops and conditionals directly in the template, reducing the need for excessive PHP script blocks.
Database Management in Laravel
Database management is a cornerstone of any web application. In Laravel, this topic aligns with the framework’s robust architecture, allowing developers to efficiently manage and interact with various database systems. Understanding Laravel's database management tools can significantly streamline development processes, enhance performance, and improve data reliability.
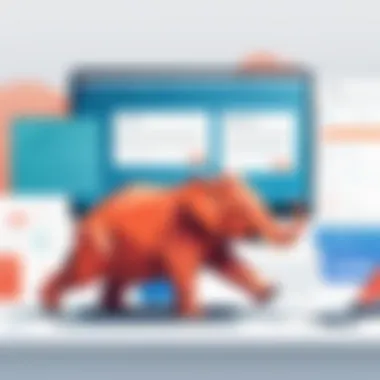
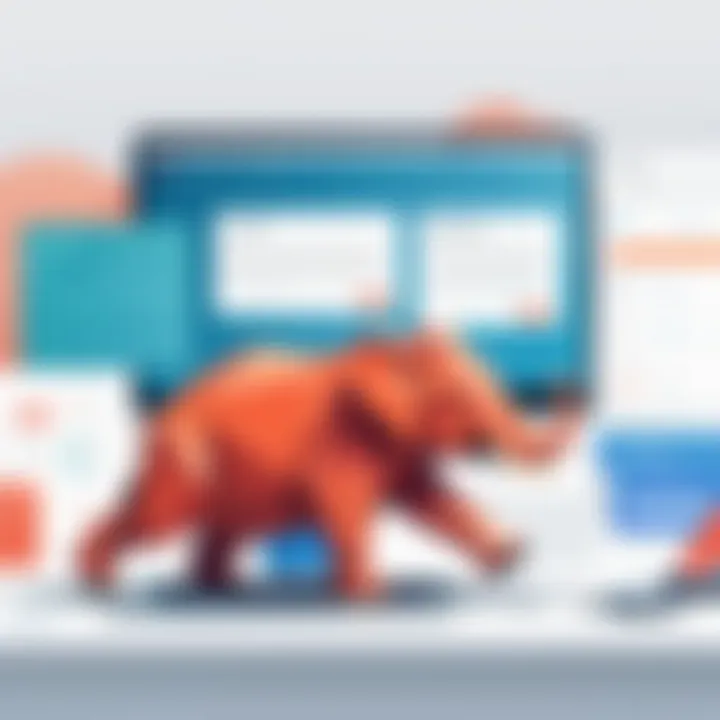
Using Eloquent ORM
Eloquent ORM is Laravel's built-in object-relational mapping tool. It simplifies the process of interacting with databases by providing an elegant syntax. With Eloquent, developers can perform queries and manage relationships between tables more intuitively, using models that represent database tables as PHP classes.
Key features of Eloquent include:
- Active Record Implementation: Eloquent models utilize an Active Record pattern, allowing direct manipulation of database records.
- Simple Relationships: You can define one-to-one, one-to-many, or many-to-many relationships seamlessly within your code.
- Query Scope Functions: This feature allows for the encapsulation of common queries, making them reusable across your application.
Using Eloquent improves the clarity and maintainability of code. It abstracts away the complexities of query syntax, enabling developers to focus on business logic rather than database management intricacies.
Migrations and Seeds
Migrations and seeds are essential tools for maintaining database structure and populating data respectively.
Migrations provide a way to version control your database schema. This allows developers to easily create, modify, and share the database structure among team members in a structured manner.
When a migration is run, it updates the database schema without directly executing SQL commands. This adds an additional layer of safety, allowing for more manageable database modifications over time.
Seeds are used to populate your database with sample or initial data. This is particularly useful during development when you need data to test your application. By running a seed, developers can create multiple records in bulk through defined classes, enhancing testing efficiency.
Advantages of using migrations and seeds include:
- Collaboration: Team members can work together without conflicts in the schema.
- Rollback Capabilities: Reverting changes is straightforward if something goes wrong.
- Progressive Improvements: Changes can be gradually introduced instead of large, disruptive updates.
Query Builder Techniques
Laravel also features a powerful Query Builder that offers an alternative to Eloquent ORM. The Query Builder provides a fluent interface for constructing SQL queries using a simple, expressive syntax. This flexibility allows developers to execute complex database queries without writing raw SQL.
Main aspects of the Query Builder include:
- Fluency: Queries can be built step-by-step with clear methods, making them readable and straightforward.
- Database Agnosticism: It supports various database systems, ensuring that developers can transition between them easily.
- Prepared Statements: Built-in support for prepared statements minimizes risks like SQL injection vulnerabilities.
Using Query Builder techniques encourages better security practices. They result in cleaner code, which leads to easier debugging and updates over time. By mastering both Eloquent and Query Builder, developers can handle virtually any database interaction required in their Laravel applications.
"Understanding database management in Laravel is crucial for building scalable and maintainable web applications."
Building Web Applications with Laravel
Building web applications using Laravel framework is a crucial aspect that deserves careful consideration. Laravel provides elegant syntax, built-in support for key functionalities, and a vibrant ecosystem that allows developers to create efficient applications. It stands out for its ability to streamline various tasks, thus enhancing productivity.
Creating RESTful APIs
Creating RESTful APIs in Laravel is not just a feature; it's an essential skill for modern web development. RESTful design principles focus on stateless communication and resource handling through standard HTTP methods. The Laravel framework simplifies API creation through its routing capabilities, utilizing the powerful Eloquent ORM for database interaction.
Developers can harness Laravel’s facade to define API routes efficiently. This setup increases maintainability and scalability. Additionally, Laravel offers built-in support for handling JSON responses, which aligns perfectly with the needs of front-end frameworks like React or Vue.js. For instance, you can define a route like this:
This method provides a clear structure for API endpoints, making it easy to manage. Furthermore, utilizing Laravel’s resource controllers helps in keeping the code organized by automating the CRUD operations. This not only saves time but also reduces the potential for errors.
Form Handling and Validation
Form handling in Laravel is designed with developer convenience in mind. The framework uses an elegant syntax for handling form submission, making it straightforward to process user inputs. Laravel's form request validation allows developers to encapsulate the validation logic within dedicated classes, improving code clarity and organization.
Laravel provides various validation rules that can be easily applied. For example:
This snippet demonstrates how clean and readable Laravel's validation can be, making it simple to ensure user inputs meet specific criteria. Additionally, error messages are automatically generated and can be customized, which enhances user experience. Developers can also leverage Laravel's session and flash messaging features to communicate validation errors back to the user effectively.
Middleware Utilization
Middleware plays a pivotal role in Laravel applications by providing a way to filter HTTP requests entering the application. This feature is essential for managing tasks such as authentication, logging, and input sanitation before reaching the application's core logic.
Using middleware, developers can easily enforce rules across different routes without repeating code. For instance, by applying the middleware, you can ensure that only authenticated users can access specific routes:
This approach not only enhances security but also improves the overall application structure. Additionally, creating custom middleware in Laravel is straightforward and allows developers to extend functionality tailored to specific needs.
"Laravel is not just a framework; it is a well-thought-out ecosystem that fosters an efficient development process, especially for web applications."
Using Laravel allows developers to concentrate on crafting solutions rather than battling with repetitive code or cumbersome setups.
Testing in Laravel
Testing plays a pivotal role in modern web development, allowing developers to ensure the quality and reliability of their applications. In the context of Laravel, a robust PHP framework, testing is not just an option but a key component of the development process. Adopting testing practices leads to fewer bugs, easier maintenance, and ultimately more efficient workflows. Developers gain confidence when pushing updates or adding features because they can rely on automated tests to catch potential issues.
Unit Testing Essentials
Unit testing, a fundamental practice in Laravel, focuses on testing individual components or modules of the application in isolation. Laravel simplifies unit testing through its built-in testing capabilities, powered by PHPUnit. Through unit tests, developers can validate that the logic within classes and methods behaves as expected.
Using unit testing brings numerous benefits. First, it fosters a culture of early bug detection, which is essential for maintaining application integrity. Second, it contributes to better code structure and design, as developers often need to write code that is isolated and testable. This leads to more modular and clean code architecture.
Testing a simple class might look like this:
This example highlights how straightforward it can be to define tests in Laravel. The use of assertions allows verification of expected outcomes, making it easier to catch failures before deployment.
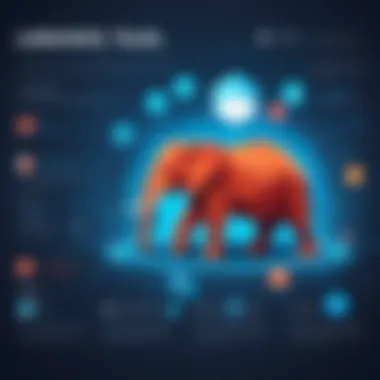
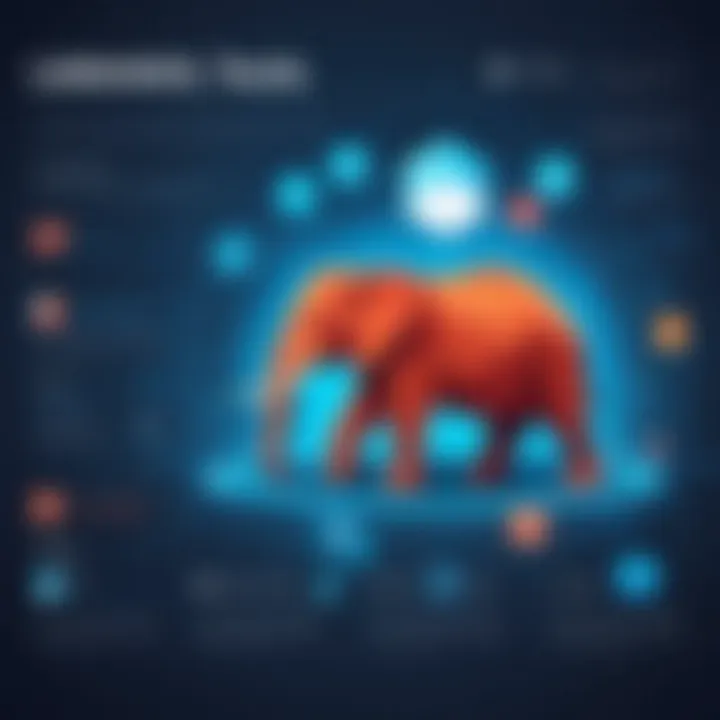
Feature Testing Approach
Feature testing extends beyond the confines of a single component, assessing the application's complete behavior from the user's perspective. In Laravel, feature tests are designed to confirm that the various parts of an application work together as intended, ensuring a seamless user experience.
This form of testing allows developers to simulate user actions and evaluate how the application responds, typically through the HTTP interface. For instance, testing user registration might involve checking if the database correctly saves user data after a successful form submission.
A feature test sample could be written as follows:
Here, developers assess both the front-end interaction and the back-end functionality, verifying that a new user is stored in the database. This holistic approach to testing covers critical integration points, allowing teams to stay responsive to user needs and system requirements.
"Testing is not just about finding bugs, it's about ensuring a quality experience for users."
Thus, testing in Laravel emerges as a foundational element in creating robust web applications. By embracing unit and feature testing, developers can ensure higher quality code and more satisfied users.
Advanced Features of Laravel
In the landscape of web development frameworks, Laravel stands out not only for its elegant syntax and robustness but also for its advanced features. These features enhance the efficiency of developers and streamline the process of building modern web applications. Understanding these functionalities is critical for anyone looking to master Laravel. They not only simplify complex tasks but also boost productivity. Such capabilities contribute to creating scalable, maintainable, and high-performing applications.
Task Scheduling
Laravel's task scheduling is a remarkable feature that provides an intuitive way to manage periodic tasks in a web application. Traditionally, developers relied on Cron jobs to perform scheduled tasks, which often required a certain level of system knowledge and manual setup. Laravel abstracts that complexity through a fluent interface, making it easier to define synchronous tasks directly in the application.
With Laravel's method, common server operations like sending scheduled emails or cleaning up logs can be easily configured. A typical use case could be setting up a daily report generation task. By defining the task in the file, developers can dictate when a task should run using simple syntax.
The elegance of this approach is twofold: it minimizes server-level dependencies and centralizes task management within the application itself. This feature proves especially valuable in large projects with numerous background jobs, allowing for easier scaling without a steep learning curve.
Job Queues and Events
Job queues and events in Laravel enhances application performance, especially when it comes to handling time-consuming processes. The framework allows developers to queue jobs for processing in the background, which is essential for maintaining optimal user experience. Long-running tasks—like uploading large files or processing images—can slow down the main application response. By delegating such jobs to a queue, Laravel ensures that application users remain engaged without delay.
Setting up a job in Laravel is straightforward. You can create a job class with artisan command, and then implement it according to your requirements. This modular approach allows for better organization of code and makes testing simpler. For instance:
After creating a Job class, it can be queued easily:
In addition, Laravel uses a robust events system that enables application components to communicate without tight coupling. Events can be fired in response to actions such as user registration or order placements. Listeners can then respond to those events and perform related tasks asynchronously
To sum up, leveraging queue and event systems enables developers to optimize response times, enhance user experiences, and scale applications efficiently.
"With Laravel's task scheduling and queue job features, developers can manage complex tasks with ease, keeping their applications responsive and user-friendly."
These advanced features exemplify Laravel's commitment to improving developers' workflows while meeting the demands of modern web application development.
Community and Ecosystem
The Laravel community plays a crucial role in the framework's success and growth. This extensive network includes developers, contributors, and enthusiasts who share knowledge, tools, and best practices. Engaging with this community provides numerous benefits, helping both newcomers and seasoned developers alike. With constant support and collaboration, users have access to a wealth of information that can greatly accelerate their learning curve.
Laravel Packages
The ecosystem of Laravel is enriched by a variety of packages. Packages are as modular pieces of code that allow developers to extend the framework's functionality. Commonly used packages, such as Spatie's Laravel Media Library or Laravel Debugbar, help streamline development tasks.
Benefits of using packages include:
- Flexibility: Packages enable developers to customize their applications according to specific project needs.
- Time-Saving: Instead of writing repetitive code, developers can utilize these packaged solutions.
- Maintained Quality: Most packages are maintained by the community, which encourages following best practices.
Using Composer, Laravel's dependency manager, developers can easily integrate and update packages. This feature allows for efficient management of project dependencies, thus maintaining the project's health and stability. Additionally, exploring existing packages can inspire developers to create their own, contributing further to the Laravel ecosystem.
Resources and Best Practices
Accessing quality resources can significantly enhance a developer's experience with Laravel. Online forums, tutorials, and documentation serve as fundamental tools for learning. Some excellent resources include:
- Laravel Official Documentation (https://laravel.com/docs): Comprehensive and regularly updated, it serves as the primary source of knowledge.
- Laracasts (https://laracasts.com): This platform offers video tutorials tailored to different skill levels, making complex topics more accessible.
- GitHub (https://github.com/laravel/laravel): The official repository contains a wealth of examples and community-contributed enhancements.
Adopting best practices is essential for maintaining code quality and project efficiency.
Consider the following suggestions:
- Use Dependency Injection: It encourages code reusability and makes testing easier.
- Follow Naming Conventions: Consistent naming improves code readability.
- Write Tests: Comprehensive testing ensures that code behaves as expected and helps prevent regressions.
By utilizing available resources and adhering to best practices, developers can navigate the Laravel landscape more effectively. Engaging with the community and staying informed can transform the development process, ensuring a more enjoyable and productive experience.
Closure and Future of Laravel
The conclusion of any examination of a framework like Laravel is significant for understanding its potential impact and growth trajectory in web development. Understanding the future directions of Laravel can empower developers to make informed choices about their projects, whether they are just starting out or have been working with the framework for some time. This section focuses on how Laravel has solidified its place in the software development landscape, what the key takeaways are, and the latest trends shaping its future.
Summary of Key Takeaways
Summarizing the main insights from this article is crucial for solidifying knowledge among readers. Laravel stands out for its elegance and simplicity, offering a plethora of features that enhance productivity. Some key points to remember include:
- Modularity through Packages: Laravel's ecosystem thrives due to its expansive packages. These tools not only promote code reusability but are essential for building complex applications more efficiently.
- Robust Community Support: Laravel is backed by a strong community of developers who continuously contribute to its improvement. Forums, Github, and documentation are rich resources for troubleshooting and learning.
- Ease of Learning: The framework's clean syntax and comprehensive documentation make it accessible for learners, reducing the barrier to entry for those new to programming.
- Adoption of Modern Practices: From RESTful routing to the MVC architecture, Laravel embraces modern web development trends, making it relevant in today's fast-evolving tech landscape.
Emerging Trends and Developments
The future of Laravel is continuously evolving. Emerging trends indicate the framework's adaptability and the community's embrace of modern technologies. Some key trends include:
- Microservices Architecture: There is a growing interest in breaking down applications into smaller, manageable services. This approach aligns well with Laravel's capabilities, making it easier for developers to scale and maintain applications.
- Increased Use of AI and Machine Learning: As AI becomes more prevalent, Laravel is starting to incorporate tools and packages that facilitate the integration of machine learning functionalities within web applications.
- Focus on Performance Optimization: The Laravel community is constantly seeking ways to optimize applications for faster performance, enhancing user experiences and system efficiency.
- Cloud Integration: With the rise of cloud computing, Laravel's compatibility with various cloud platforms is gaining attention, making deployment and scaling more manageable for developers.
"Laravel is not just a framework; it’s a community and an ecosystem that evolves with the demands of modern web development."