Mastering Kafka in Java: A Comprehensive Guide
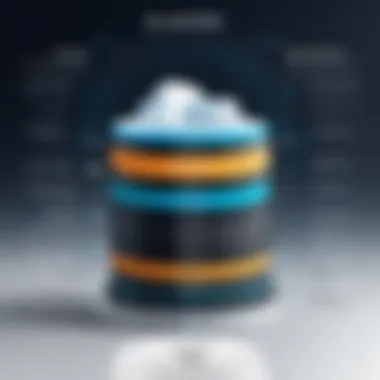
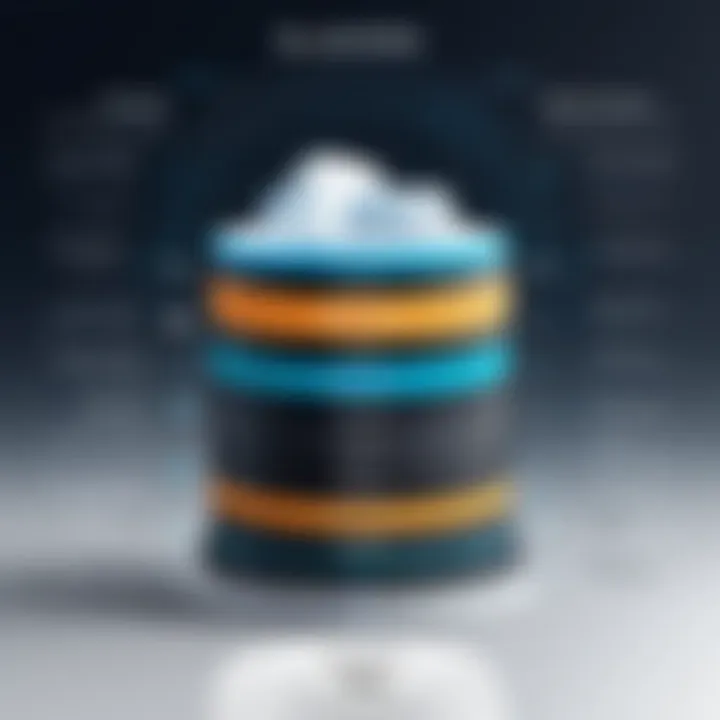
Intro
Apache Kafka serves as a backbone for modern data streaming, allowing organizations to process vast streams of data in real-time. It has grown in popularity for good reason. This article intends to peel back the layers of Kafka within the Java ecosystem, providing insights that developers can grasp and apply.
Kafka began its journey at LinkedIn, designed to handle massive amounts of log data efficiently. It's a distributed streaming platform that helps in building real-time data pipelines and streaming applications. As applications grow complex and the demand for real-time processing rises, understanding Kafka is crucial for developers.
Preface to Apache Kafka
History and Background
Kafka was initially developed in 2010. The name itself comes from the author Franz Kafka, reflecting the tool's ability to handle various data flows. When it was released to the public as open-source software in 2011, it quickly gained traction due to its high throughput, scalability, and fault tolerance. With the backing of the Apache Software Foundation, Kafka has become a key player in the data streaming landscape.
Features and Uses
Kafka stands out for several reasons:
- High Throughput: Kafka can handle hundreds of thousands of messages per second without breaking a sweat.
- Scalability: Whether you're managing a few servers or thousands, Kafka can scale horizontally to meet demands.
- Fault Tolerance: With data replication across multiple brokers, there's no single point of failure. If one broker goes down, others can pick up the slack.
These features make Kafka suited for various use cases, from processing user activity data in real-time to managing log aggregation. It's widely used in industries like finance, e-commerce, and transportation, among others.
Popularity and Scope
The community around Kafka has exploded since its inception. Organizations like Spotify, Netflix, and Uber are leveraging it for their data streaming needs. The powerful combination of Kafka and Java is particularly appealing. Java’s strong type system, performance capabilities, and vast libraries create an ideal environment for leveraging Kafka's potential. Developers familiar with Java can dive right into Kafka, making it an attractive option for companies already invested in this programming language.
Basic Concepts
To effectively harness the power of Kafka in Java, it’s essential to understand its basic components and how they interact.
Core Components of Kafka
- Producers: Applications that send data to Kafka topics.
- Consumers: Applications that read data from topics.
- Topics: Categories into which records are organized; think of these as channels for data flow.
- Brokers: Kafka servers that store data and manage requests.
Configurations
Setting up Kafka might seem daunting at first, but it’s straightforward once you get the hang of it. You will need to install Kafka along with a Zookeeper instance, which is required for maintaining its configuration and managing brokers. The basic commands that you will be using frequently include starting the server and creating topics.
Understanding the configurations is vital for optimizing your data pipelines.
Advanced Topics
Once you have the basics down, delving into advanced topics will set you up for successful implementation.
Streaming APIs
Kafka provides a set of APIs for processing streams of data. The Kafka Streaming API allows you to process data in real-time by composing applications that react to events as they happen.
Security
In today’s digital realm, ensuring data security is paramount. Kafka offers several layers of security, including SSL encryption, SASL authentication, and fine-grained access control to ensure that only authorized clients can publish or consume messages.
Hands-On Examples
The best way to solidify your understanding of Kafka in Java is through hands-on examples. Here are a few projects you could try:
Simple Program
A basic Hello World Kafka producer can help you get your feet wet. You can write a simple Java application that sends messages to a Kafka topic.
Intermediate Project
Consider building a real-time analytics dashboard that consumes data from Kafka and displays it on a web interface. This can introduce you to concepts of data persistence and retrieval.
Code Snippet
Here’s a simple producer example in Java:
Resources and Further Learning
For those eager to dive deeper into Kafka with Java, the following resources may be beneficial:
- Recommended Books:
- Online Courses:
Platforms like Coursera and Udemy offer numerous courses focusing on Kafka. - Community Forums and Groups:
The Apache Kafka community is very active on forums like Reddit. Engaging in groups can provide support and further insights.
- "Kafka: The Definitive Guide" by Neha Narkhede
- "Designing Data-Intensive Applications" by Martin Kleppmann
Exploring Kafka in Java opens numerous doorways to enhancing data processing capabilities in software applications. By mastering its concepts, features, and practical applications, developers can significantly impact the way data flows in today’s tech landscape.
Prologue to Kafka
In today's fast-paced digital world, handling streams of data efficiently is crucial. Apache Kafka stands out as a significant player in this arena. This section introduces Kafka, highlighting its role in the Java programming environment. Understanding Kafka is not just about recognizing it as a tool; it's about comprehending how it changes the game in data streaming and messaging.
Kafka serves as a backbone for various applications, making it essential for developers and engineers whose work revolves around data transfer and processing. By diving into the inner workings of Kafka, we uncover how it enables systems to be more responsive and adaptive, especially when dealing with real-time data. This article aims to clarify Kafka's architecture, its integration with Java, and provide practical insights for developers looking to leverage its capabilities.
Overview of Event Streaming
In essence, event streaming is all about processing a never-ending flow of data. Picture a river, constantly flowing and changing with new tributaries joining in. In the tech world, this river represents real-time information, such as user actions, sensor outputs, or system logs. Event streaming lets businesses and applications process and react to these streams promptly, facilitating timely decisions and enhancing user experiences.
With event streaming, we shift away from traditional request-response paradigms, allowing for a more dynamic interaction model. For instance, consider a stock trading application. Event streaming enables instant updates when stock prices fluctuate, meaning users can make quick decisions based on the most current data. In a way, event streaming enriches data-driven projects, providing immediacy that batch processing simply can't deliver.
What is Kafka?
Apache Kafka is an open-source platform designed primarily for building real-time data pipelines and streaming applications. At its core, Kafka is a distributed messaging system that efficiently manages and processes large volumes of data streams. It operates via a publish-subscribe model, where producers send messages to topics, and consumers read from those topics.
Kafka has several key attributes that contribute to its popularity:
- Durability: Messages are persisted on disk, meaning that even if a system crashes, no data is lost.
- Scalability: Kafka can handle an increase in data volume without a hitch, thanks to its distributed architecture.
- High Throughput: With low latency, Kafka supports thousands of messages per second, making it suitable for high-demand scenarios.
- Fault Tolerance: Multiple nodes provide redundancy, ensuring that even if a broker fails, the system remains operational.
In summary, Kafka is not just a data-handling tool; it is a robust platform integral to the architecture of modern distributed systems. As we proceed through this article, we’ll delve deeper into its components, configurations, and real-world applications, particularly within Java frameworks. This knowledge is crucial for developers aiming to implement effective data solutions in their projects.
Architecture of Kafka
Kafka's architecture is the backbone of its robust functionality, specifically designed to address the challenges of modern data streaming applications. Understanding how Kafka is structured is key for developers aiming to leverage its capabilities in their Java applications. The architecture not only defines Kafka’s internal workings but also impacts its performance, scalability, and reliability, which are essential elements for any large-scale system.
Components of Kafka
The architecture of Kafka can be broken down into several essential components. Each plays a distinctive role that contributes to Kafka's efficiency and effectiveness as an event streaming platform, ensuring that developers can build high-throughput, reliable systems.
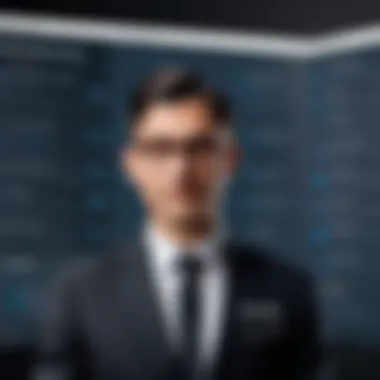
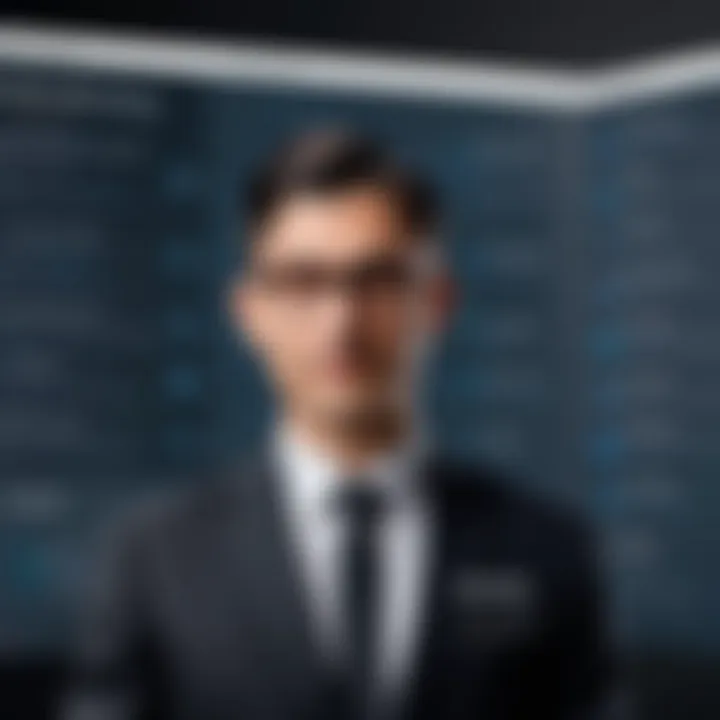
Producers
Producers are the entities responsible for sending records (or messages) to topics in Kafka. They play a pivotal role in data ingestion, pushing the information that downstream systems will later consume.
One of the key characteristics of producers is their capability to operate asynchronously, meaning they can send messages without waiting for a response from Kafka. This non-blocking behavior is a hallmark of Kafka, allowing systems to achieve high throughput—a fundamental need in many data pipeline architectures.
A unique feature of producers is their ability to batch messages. When configured correctly, batching messages can significantly reduce the network overhead and improve performance, as it minimizes the number of requests sent. However, this can complicate implementations since dealing with larger batches might lead to increased memory usage. The trade-off must be considered based on the specific requirements of the application.
Consumers
Consumers, on the other hand, are responsible for reading data from Kafka topics. They offer crucial functionality for subscribing to streams of records and processing them further.
A defining characteristic of consumers is their flexibility— they can work in groups to balance the load of consuming records from a topic. This consumer group feature is vital for ensuring that multiple consumers can share the workload, making it easier to scale applications as data volume grows.
The potential downside to using consumers is the complexity they introduce, especially when dealing with stateful operations. Managing offsets and ensuring that each record is processed exactly once without duplicates can be challenging. Developers must carefully design their systems to handle these intricacies effectively.
Brokers
Brokers represent the servers that form the Kafka cluster itself. Each broker is responsible for handling requests from producers and consumers, managing data storage, and providing distribution and replication for fault tolerance.
The key characteristic of brokers is their ability to manage large volumes of data and maintain high availability. Kafka's replication mechanism, where messages are duplicated across multiple brokers, ensures that even if one broker fails, data remains accessible. This aspect of brokers greatly influences the reliability of systems built on Kafka.
However, with scalability and reliability come risks. If a cluster grows too quickly or if brokers are not correctly monitored, there can be challenges in performance. Therefore, understanding the broker's resource utilization is essential to maintaining an efficient Kafka environment.
Topics and Partitions
Topics and partitions are central concepts within Kafka that define how messages are categorized and distributed. A topic can be understood as a stream of records, while partitions allow that stream to be divided into chunks, enabling scalability and parallel processing.
One notable feature of topics and partitions is that they provide a mechanism for load balancing. By distributing messages across multiple partitions, Kafka can parallelize the processing. Each consumer in a consumer group can consume records from different partitions, enhancing throughput significantly.
Nevertheless, this setup introduces considerations regarding message ordering. Within a partition, messages retain their order, but across them, there's no guarantee. This can pose challenges depending on the application’s requirements and must be taken into account when designing the data architecture.
Kafka's Storage Mechanism
Kafka organizes its messages into a fault-tolerant, distributed storage architecture, allowing for efficient storage and retrieval. Each topic, divided into partitions, gets stored in a compacted format with immutable logs. This structure dramatically speeds up access while providing a smooth experience for both producers and consumers.
In summary, a grasp of Kafka's architecture enables developers to leverage its full potential, making it an indispensable tool for building resilient data-driven applications. By understanding the interplay between producers, consumers, brokers, topics, and partitions, one can navigate the complexities of Kafka with greater ease and efficacy.
Setting Up Kafka in a Java Environment
Setting up Kafka in a Java environment is a crucial step for developers who want to harness the power of event streaming in their applications. Kafka acts as a central hub for real-time data flows, which can greatly enhance the performance and responsiveness of applications. To fully utilize Kafka, it’s essential to have a solid grasp on the installation and configuration processes, along with effective integration with Java. By setting up Kafka properly, developers lay the groundwork for building scalable and reliable event-driven applications. In this section, we will explore the essential steps involved in getting Kafka up and running alongside Java, as well as how to effectively connect the two.
Installation and Configuration
Installing and configuring Kafka may seem like a daunting task, but when broken down into manageable steps, it becomes an achievable goal. The installation processes may vary depending on the operating system being used, but essentially, developers need to download the Kafka binaries, set up a Kafka broker, and start the necessary services.
First, it’s important to have Java installed, as Kafka runs on the JVM. Developers can check their Java version via the command line. Once Java is confirmed, obtaining Kafka involves downloading it from the official Apache site and unzipping the files. This setup provides a robust framework on which to build streaming applications in Java.
Configuration is another piece of the puzzle. Kafka has a configuration file wherein developers can set various broker settings, like the log directories and network ports. A sample line to modify might look something like this:
This simple line indicates where Kafka should store its logs. It’s notable because poor log management can lead to severe performance issues, hence it's critical to set this right from the get-go.
Integrating Kafka with Java
After getting Kafka installed and properly configured, the next step is the integration with Java. This process usually revolves around using tools that facilitate the inclusion of Kafka libraries and handling Java's intricate classpath requirements.
Using Maven for Dependencies
Maven serves as a handy dependency management tool for Java. When used for including Kafka libraries, it simplifies the process of adding and updating dependencies. By merely specifying the Kafka library in the file, developers ensure they are always using the latest and most stable version available.
For example, developers can add the following XML snippet to their :
This makes Maven fetch the Kafka client library necessary for communicating with the Kafka environment.
What makes Maven particularly beneficial is its ability to manage transitive dependencies. If a library you depend on requires other libraries, Maven brings them in automatically, saving the developer a significant amount of hassle. However, it's worth noting that setting up Maven can have a slight learning curve for newcomers, yet its long-term advantages outweigh initial hurdles.
Setting Up Properties
Setting up properties involves defining essential configurations that dictate how an application interacts with Kafka. These configurations include critical parameters such as the Kafka broker address and serializer settings.
One of the distinct features of setting up properties is the and classes, which allow developers to solidify their setup by specifying attributes relevant to producers and consumers respectively. For example:
This snippet sets up the essential properties needed to create a Kafka producer that can send messages to a broker running on . The choice of serializers can deeply influence how data is transmitted and interpreted; thus, setting these elements with care is vital.
While setting properties can be straightforward, poorly defined properties could lead to communication breakdowns or inefficient data processing. Documentation often provides guidance on best practices, which developers should utilize to mitigate potential issues. The balance between clear-cut implementation and nuance is what makes a developer adept.
By following the steps outlined above, users can effectively set up Kafka within their Java environment, ensuring a solid foundation for building robust applications that leverage real-time data streaming.
Kafka Producer API in Java
The Kafka Producer API acts as the vital cog in the wheel of data publishing. It enables applications to send records to Kafka topics where the records can later be processed or consumed. Understanding this API is particularly beneficial for developers as they work towards building efficient and robust data pipelines. Its fundamental role helps in laying down a solid foundation for applications that thrive on real-time data streams.
The benefits of using the Kafka Producer API include its scalability, durability, and ability to handle high-throughput messaging. Moreover, it offers flexibility in message formatting, allowing developers to publish data in various forms, from text to binary. Additionally, compensating for network issues and ensuring message delivery are intrinsic features of this API that significantly enhance application performance.
Creating a Producer
Creating a producer in Kafka starts with the configuration setup, which lays the groundwork for smooth operations. The primary decision is to choose the appropriate serializer for the keys and values being sent. Here’s a basic rundown of the steps involved:
- Set up Properties: Before hitting the ground running, you need to define the properties. These may include:
- Create the Producer: With configuration at hand, it’s time to create the producer instance. It’s often done with a simple line of code:This instantiates your producer with all the configurations you specified.
- Handle Resource Management: Producers utilize resources since they typically run as long as your application. Hence, it’s crucial to close the producer gracefully at the end to avoid memory leaks:Ensuring this can save future headaches.
- Bootstrap servers: Tells the producer where Kafka brokers are located.
- Key and Value Serializers: Specify how the data should be serialized. Common examples are and .
These steps are like laying bricks for a new home; they create a sturdy foundation upon which you can build complex functionality.
Sending Messages
Once the producer is set up, you’re ready to send messages. In Kafka, messages are always sent to a specified topic. You can send data asynchronously or synchronously, depending on your requirements. Here’s how sending messages works:
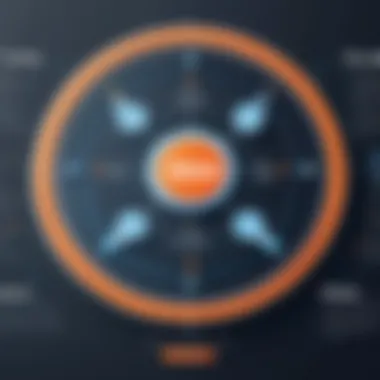
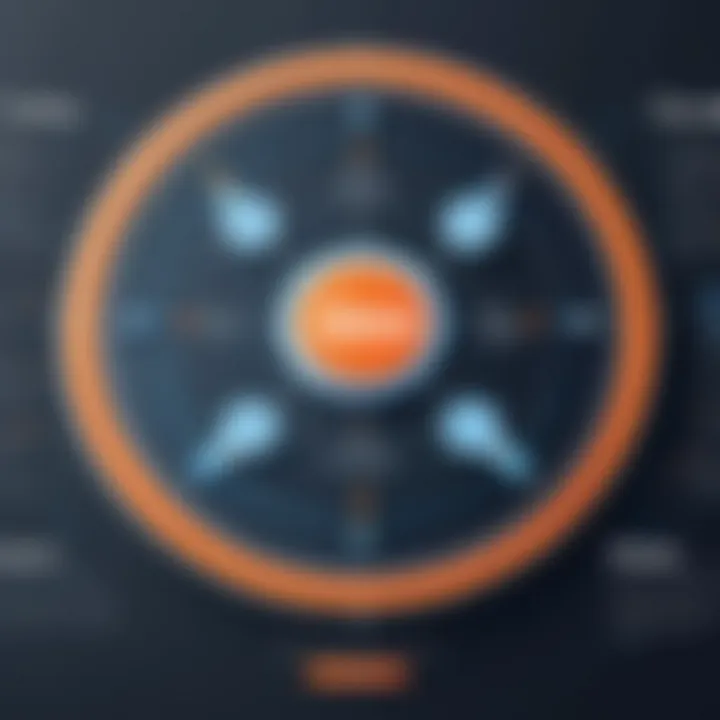
- Create a ProducerRecord: This object encapsulates the data you want to send along with the target topic. It includes:
- Send the Message: To send the record, call the method. You can choose to handle success and failure scenarios by implementing callbacks if needed:This sends the message and allows you a chance to react to its status.
- Batch Sending: For performance tuning, you might want to send messages in batches. This can be done by accumulating multiple objects in a loop before sending them, reducing overhead and improving throughput.
- Topic name: Where the message will go.
- Key: (optional) Helps to determine the partition.
- Value: This is the actual message content.
Kafka Consumer API in Java
In the realm of Apache Kafka, the Consumer API plays a pivotal role in how developers access and process data streams. This section dives into the mechanics of the Kafka Consumer API and explains its significance in creating robust data solutions using Java.
The Kafka Consumer API is fundamentally about reading records from Kafka topics, which encapsulate streams of data categorized by key and partition. This functionality is essential as it allows applications to subscribe to various topics and read messages efficiently. Key benefits include real-time messaging, data decoupling, and the ability to process information at scale.
Utilizing the Consumer API effectively requires an understanding of its core functionalities and best practices. As applications evolve, managing multiple consumers, handling offsets, and ensuring message order become critical considerations. With the growing demand for data-driven applications, mastering the Consumer API is essential for any developer looking to harness the power of Kafka.
Creating a Consumer
Creating a consumer in Kafka involves specific steps to ensure proper configuration and functionality. Below is a breakdown of how to establish a Kafka consumer in a Java application:
- Dependencies: Ensure you have the necessary Kafka client libraries in your project. For example, using Maven, you can add the Kafka client dependency in your :
- Configuration: Set up the consumer properties, which include parameters like the bootstrap server, deserializer, and group ID. Here's a sample configuration:
- Creating the Consumer: With the properties defined, instantiate the Kafka consumer:
- Subscribing to Topics: A critical step is to subscribe the consumer to one or more topics:
These steps provide a robust starting point for any Kafka consumer in a Java context. By configuring the consumer correctly, developers lay the groundwork for reliable data handling.
Consuming Messages
Once the consumer is set up and subscribed to a topic, it’s time to engage with the message consumption process. Consuming messages involves polling the Kafka broker for new records and processing them appropriately.
Here’s how it typically unfolds:
- Polling for Messages: The consumer continuously polls the broker for new messages. This is done using the method, which retrieves records from the subscribed topics:
- Processing Records: After polling, messages can be processed in a loop. Here’s a simplified example:
- Committing Offsets: A critical consideration in consuming messages is managing offsets. This ensures that messages are acknowledged, preventing them from being reprocessed. Committing offsets can be done manually or automatically:
- Automatic: This commits offsets automatically at intervals.
- Manual: This allows for fine control over when offsets are committed, which is useful in ensuring exactly-once processing semantics.
Consuming messages from Kafka topics is both straightforward and powerful. By leveraging the Consumer API, developers can create responsive applications that interact seamlessly with streaming data. Understanding the nuances of message consumption, including offset management, is vital for building reliable data solutions.
Advanced Kafka Features
In the realm of Apache Kafka, there exists a plethora of advanced features that not only enhance its capabilities but also play a crucial role in making it a leading choice for modern data streaming applications. Understanding these features is vital for developers looking to leverage Kafka effectively within their Java applications. The advanced functionalities—like Consumer Groups, Kafka Streams, and Kafka Connect—add layers of effectiveness and flexibility that are often indispensable in large-scale processing tasks.
Consumer Groups
Consumer groups are a core concept within Kafka that significantly improve message processing efficiency and scalability. A consumer group comprises one or more consumers that work together in unison to pull messages from topics. With this idea, each consumer in a group reads from a unique partition of the topic, ensuring that workload is evenly distributed. This mechanism provides high throughput while maintaining fault tolerance.
One notable benefit of using consumer groups is the ability to balance load. If you have high traffic and a single consumer, it may become overwhelmed with the number of messages to process. By adding more consumers to a group, you can harness parallel processing. Each consumer will pick up where others leave off, thus accelerating your application's responsiveness.
"Using consumer groups translates to increased performance and more efficient message handling."
You also gain the capability for easy scaling. For instance, if you find that your consumers are struggling to keep pace with incoming data, you can simply add another consumer to the group. They will automatically begin fetching messages without requiring intricate reconfiguration. In this way, consumer groups foster an adaptable structure, enhancing Kafka's reputation for handling enormous workloads gracefully.
Kafka Streams
Moving on to Kafka Streams, this feature enables the development of real-time applications and microservices that can process data on the fly, right as it streams in. This is quite different from traditional batch processing that can result in significant delays. Kafka Streams provides a powerful library for building applications that can read, process, and even write data back to Kafka topics.
A big plus of Kafka Streams lies in its simplicity. With its Java-based structure, developers familiar with traditional Java programming will find it quite straightforward to work with. The library allows for data manipulation and aggregations using a fluent API, enabling complex transformations without delving deep into additional systems.
Benefits of Kafka Streams include:
- Real-time processing: Respond to events as they happen, improving the timeliness of data-driven decisions.
- Built-in fault tolerance: Kafka Streams applications can automatically recover from failures, which means less downtime.
- Event-time processing: Handle events in the order they occurred, regardless of arrival time anomalies.
The integration of Kafka Streams into Java applications not only simplifies the coding process but also maximizes the impact of the streaming data.
Kafka Connect
When it comes to integrating various systems with Kafka, Kafka Connect acts as an efficient bridge. It's a tool to stream data between Kafka and other systems, be they databases or other data sources and sinks. Utilizing Kafka Connect can significantly reduce the complexity generally associated with building custom connectors.
With Kafka Connect, you can use pre-built connectors to easily integrate with popular databases such as MySQL, PostgreSQL, or even NoSQL solutions like MongoDB. This streamlines the process of getting data in and out of Kafka without requiring extensive coding effort.
Some key features include:
- Ease of use: Connectors can be configured with simple JSON configurations, making it accessible for developers at all levels.
- Scalability: Kafka Connect can scale out horizontally. As your data intake grows, simply add more workers to handle increased load.
- Fault tolerance: Like many components of Kafka, Connect offers built-in mechanisms for handling failures, ensuring that data is not lost in transit.
In summary, the advanced features in Kafka serve as a powerful toolkit for developers aiming to build robust data processing applications. Whether leveraging consumer groups for efficient load balancing, employing Kafka Streams for real-time processing, or using Kafka Connect to simplify external data integration, these components can bring significant improvements to your application architecture.
Error Handling and Logging in Kafka
Effective error handling and logging are crucial in any system that processes data, especially in complex event-streaming environments like Apache Kafka. When integrating Kafka into Java applications, developers often encounter various issues that can impede data flow and system performance. Understanding how to address these challenges not only enhances the robustness of applications but also aids in maintaining seamless operations. This section will delve into common errors encountered while using Kafka, as well as best practices for logging that can facilitate easier debugging and monitoring of Kafka systems.
Common Errors
Errors in Kafka can arise from a variety of sources, affecting both producers and consumers. Here are some notable examples:
- Message Serialization Failure: When sending messages, the data must be converted into a byte format that Kafka can handle. If there’s an issue with the serialization process, producers might fail to send messages.
- Connection Issues: Network-related problems can thwart communication between the Kafka client and the broker. This can happen due to various factors like firewall settings or broker downtime.
- Offset Management Problems: Consumers track offsets to know which messages have been processed. If there’s a mismatch or the consumer tries to consume from an invalid offset, it can lead to message consumption failures.
- Resource Exhaustion: In high-throughput scenarios, it's possible to hit resource limits, be it memory or CPU. This can lead to delays in message processing and unexpected behavior.
Understanding these common issues can significantly ease troubleshooting. It’s advisable to implement fallback mechanisms in your application to gracefully handle these situations, rather than failing catastrophically. With the right error handling strategies, you can make sure your Kafka applications can recover without much fuss.
Best Practices for Logging
Logs are critical for monitoring and diagnosing issues in Kafka applications. To make logging effective, consider the following best practices:
- Use Log Levels Wisely: Configure logging to capture different levels (INFO, WARN, ERROR). A higher granularity allows developers to filter critical issues without losing valuable context.
- Centralized Logging: Implement a centralized logging system, such as ELK (Elasticsearch, Logstash, and Kibana) or Splunk. This makes it easier to track logs from multiple services and correlates them effectively, especially in distributed systems.
- Contextual Logging: Always log contextual information such as timestamps and transaction IDs. This provides insights during analysis, especially when tracking requests through different system components.
- Structured Logging: Instead of traditional log formats, consider using structured logging with formats like JSON. This enhances the parsing and searching capabilities of logs, streamlining the monitoring process.
By adhering to these logging practices, developers can create a more transparent environment where errors can be quickly identified and resolved. Effective logging not only aids in trouble shooting but also contributes to overall system health, so it’s worth investing time to get it right.
Remember: Good logging practices can save a developer hours of headache by providing clear insights when things go sideways.
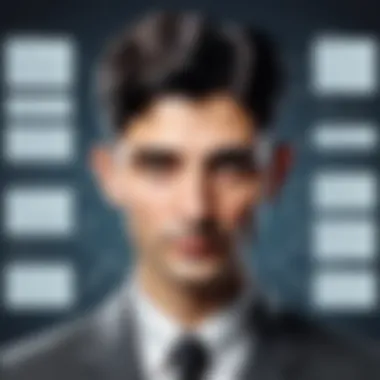
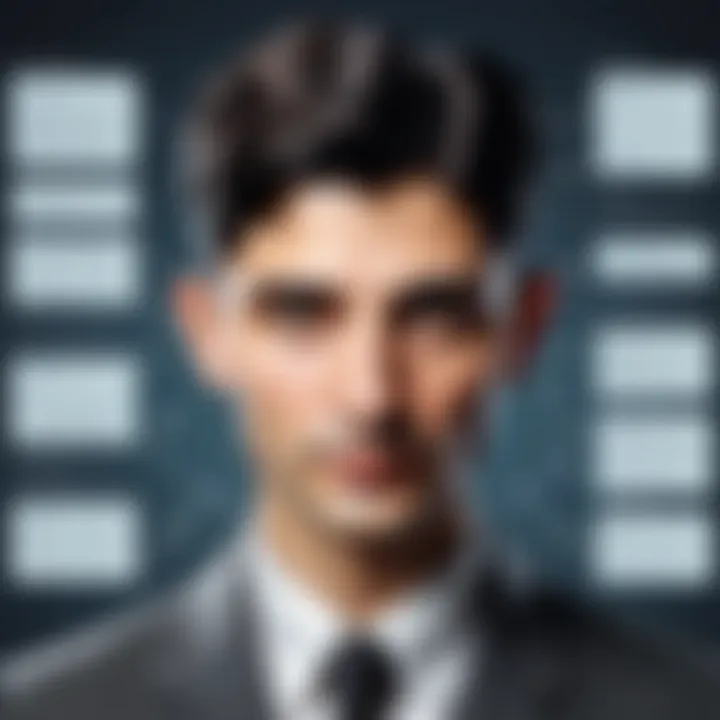
By focusing on error handling and logging effectively, developers can ensure their Kafka applications are resilient and maintainable. Whether troubleshooting errors or analyzing performance through logs, having a robust strategy in place can lead to smoother operations and a better user experience.
Performance Tuning in Kafka Applications
In the landscape of data-driven applications, the significance of performance tuning cannot be overstated. For applications utilizing Apache Kafka, a robust performance is essential, as it directly impacts throughput, latency, and ultimately, user experience. Therefore, understanding the nuances of performance tuning is vital for developers aiming for efficiency and reliability in their systems.
Performance tuning refers to the adjustments and optimizations made to enhance the responsiveness and stability of an application. When it comes to Kafka, this process isn't merely about squeezing out every drop of performance but rather ensuring that your producers and consumers operate harmoniously while effectively managing the load.
Why Performance Tuning Matters
When you consider the volume of data streaming through Kafka, achieving optimal performance can mean the difference between a sluggish system and a high-speed pipeline. Key benefits of performance tuning in Kafka applications include:
- Increased Throughput: Efficiently configured producers and consumers can handle higher volumes of messages per second.
- Reduced Latency: Optimizing message delivery leads to quicker data processing, enhancing real-time application responses.
- Scalability: As the demand for data increases, a well-tuned Kafka setup can seamlessly scale to accommodate additional load without breaking a sweat.
Ultimately, performance tuning aligns your Kafka setup with the specific requirements and workloads of your application, ensuring you are prepared for both peak loads and quiet times.
Optimizing Producer Performance
To get the best performance from producers, a few strategies can be adopted. Here's a practical guide to fine-tuning producer performance:
- Batching: By configuring the producer to send records in batches rather than individually, you can significantly reduce the number of requests made to the Kafka cluster. This not only speeds up the message sending process but also lessens network overhead.
- Compression: Utilizing compression algorithms like Snappy or Gzip can reduce the size of the messages transmitted, leading to faster send times and efficient use of bandwidth. Just keep in mind the trade-off in CPU usage when compressing data.
- Acknowledge Settings: Adjusting the acknowledgment settings (acks) lets you control how many broker acknowledgments a producer needs before considering a request complete. Setting this to zero (0) can increase throughput, but you risk losing messages in transit. A value of one (1) balances performance and durability, while 'all' ensures every replica acknowledges the message, providing robustness at the cost of speed.
Producer Configuration Example
This example configures the producer to batch records for 10 milliseconds and utilize Gzip for compression, which can lead to better performance metrics.
Optimizing Consumer Performance
On the flip side, ensuring that consumers are running at peak performance is equally important. Here are some strategies to consider:
- Parallelism: Kafka allows you to create multiple consumer instances within a consumer group, enabling parallel processing of messages. This is particularly beneficial in high-load scenarios where one consumer might become a bottleneck.
- Fetch Size Tuning: Adjust the setting to alter how much data each fetch request returns. If this value is too low, consumers may make unnecessary requests. Conversely, if it’s too high, it might lead to increased memory consumption and delays in processing.
- Auto Offset Commit: By default, consumers can automatically commit offsets of messages they’ve processed. While convenient, this may lead to lost messages in cases of failure. It can be beneficial to disable this and commit offsets manually after processing messages.
"Performance in Kafka isn't about how much data you handle at a time, but how fast and reliably you can manage that data."
These techniques help in creating a responsive and effective consumer, capable of handling the demands of real-time applications.
In closing, performance tuning for both producers and consumers is an essential aspect of Kafka application development. By focusing on optimizing these components, developers can enhance the efficiency and reliability of their real-time data pipelines.
Real-World Use Cases of Kafka in Java
Kafka stands as a pillar in modern data solutions, serving numerous enterprises with its event streaming capabilities. The importance of this section is to elucidate how Kafka's architecture and functionalities translate into real-world applications, especially in Java environments. Understanding these use cases provides concrete examples of how developers leverage Kafka to build robust, scalable systems. Consequently, the information presented here might enlighten those striving to harness Kafka’s potential in their projects, leading to better decision-making and strategic implementations.
Data Pipeline Implementations
One of the most prevalent use cases for Kafka in Java is data pipeline implementation. Companies generate vast amounts of data daily—through user interactions, system logs, and transactions. Here, Kafka acts as a conduit that efficiently channels this data into centralized processing systems.
For instance, consider a retail company that collects customer data from multiple touchpoints: mobile apps, online purchases, and in-store transactions. By utilizing Kafka, the company can stream this data in real-time into various analytics tools or databases. This not only promotes timely insights but also helps to maintain the integrity and consistency of the data being processed.
Key Benefits of Using Kafka in Data Pipelines:
- High Throughput: Kafka can handle massive data loads without breaking a sweat. Most commonly used in scenarios where data arrives in large volumes, its architecture allows it to scale effortlessly.
- Fault Tolerance: With its distributed design, it ensures that even if part of the system fails, data can still be processed without loss. The replicated log feature means fault tolerance is second nature to Kafka.
- Data Replay: Notably, Kafka’s unique approach allows consumers to go back and replay events. This is particularly beneficial for debugging and data recovery scenarios.
In Java, implementing such a data pipeline using Kafka is made convenient with the Kafka Producer API, where developers can easily create producers to send messages to a Kafka topic, often wrapped in a more extensive processing framework.
Here’s a simple example of a data producer in Java:
This exemplifies the simplicity and elegance of sending data through Kafka in a Java environment.
Reactive Streaming Applications
Another innovative use of Kafka in Java lies within reactive streaming applications. As industries evolve, the demand for real-time data processing increases. Companies want systems that not only respond to events but also react in real-time to changes in data flow. Here, Kafka shines by enabling developers to build applications that can process streams of data as they arrive.
In this scenario, the Reactive Streams API complements Kafka's architecture beautifully. By integrating this API in Java, developers can create systems that effectively manage asynchronous data streams, optimizing resource usage while ensuring a responsive design.
A classic application of this concept can be seen in fraud detection systems in banking. By utilizing Kafka, banks can stream transaction data in real-time, allowing for immediate analysis against various fraud detection algorithms. Any suspicious activity can trigger alerts or auto-reject actions within seconds, greatly minimizing potential losses.
Benefits of Reactive Streaming with Kafka Include:
- Real-Time Processing: As data arrives, it can be processed immediately rather than stored and processed later.
- Event-Driven Architecture: Applications become highly interactive and adaptable; they can adjust to incoming data flows dynamically.
- Scalability: As transaction volumes shift, reactive systems built around Kafka can scale horizontally, making them a future-proof investment.
To wrap it up, employing Kafka in real-world applications demonstrates its versatility and proficiency in handling varied data processing needs. From data pipelines that keep business insights flowing to reactive systems that respond instantly to events, Kafka proves to be an essential tool that Java developers should keep in their arsenal.
Kafka Security Considerations
Security is a pivotal aspect to bear in mind when implementing Kafka in real-world applications. As data flows through Kafka, it not only moves from one service to another but also often contains sensitive information. Failing to secure this data could lead to significant ramifications, including data leaks or unauthorized access to critical systems. In this context, understanding and implementing security measures is no longer just an optional add-on; it’s a necessity for any organization looking to harness the power of Kafka.
Authentication Mechanisms
Authentication in Kafka revolves around verifying the identity of clients attempting to connect to the Kafka cluster. There are several authentication mechanisms supported by Kafka that you can utilize, ensuring that only authorized users have access. Here are the primary methods:
- SASL (Simple Authentication and Security Layer): SASL allows various authentication protocols, such as PLAIN, SCRAM-SHA-256, and GSSAPI, to be implemented. Each has its unique characteristics and can be tailored to fit specific security requirements.
- SSL/TLS: By employing SSL/TLS, data in transit can be encrypted, which not only aids in securing the communication between clients and brokers but also helps in authentication. SSL certificates play a critical role here, enabling client verification.
To implement these authentication methods effectively, clear policies must be set regarding client certificates and credentials. Failure to adhere to these can open doors for attackers, undermining your security postures.
Authorization Practices
Once authentication has been established, the next step is authorization, which controls what authenticated users can actually do in Kafka. Strong authorization measures are essential for ensuring that users can only access the resources they are permitted to. Kafka utilizes a combination of Access Control Lists (ACLs) and role-based access control to enforce these practices.
- Access Control Lists (ACLs): ACLs define who can do what with your Kafka resources, such as topics and consumer groups. By setting up appropriate ACLs, you can allow or deny permissions to produce, consume, or manage data.
- Role-Based Access Control (RBAC): This method can streamline the process by assigning roles to users or services instead of handling permissions individually. It simplifies managing permissions as roles can encompass multiple privileges.
The End
Concluding an article of this magnitude isn’t simply about summarizing facts. It’s about truly weighing the intricacies of managing Kafka in Java and what these insights mean for the connected ecosystem of data-driven applications.
First and foremost, we’ve examined how Kafka functions as a pivotal player in the event streaming landscape. Understanding its architecture and features gives developers a solid grounding to harness its capabilities effectively. This knowledge is not just academic; it can profoundly influence the performance and scalability of real-world applications.
Moreover, we’ve discussed various components and tools that make up the Kafka framework, from producers to consumers, and how they operate in tandem within Java environments. Having clarity on these elements ensures developers can more adeptly troubleshoot any issues that may arise and implement best practices from the get-go.
"In the world of data streaming, knowledge is not just power; it’s a necessity."
Summary of Key Points
- Kafka’s Architecture: We explored how Kafka is built to handle large volumes of data streams while maintaining delivery guarantees and durability.
- Kafka APIs: The in-depth discussions on both Producer and Consumer APIs provided necessary steps for creating and managing streams of data. Whether sending or receiving messages, these APIs are crucial.
- Performance Tuning: We covered techniques to enhance the performance of both producers and consumers, ensuring that applications run seamlessly.
- Security Considerations: Addressing security helped emphasize the importance of authentication and authorization mechanisms within Kafka implementations.
Future of Kafka and Java Integration
As we look ahead, the integration of Kafka with Java will only become more sophisticated. With the continuous evolution of data principles, coding practices, and application requirements, developers will likely see:
- Increased Demand for Real-Time Processing: Businesses are striving for quicker insights and more responsive applications. Kafka's ability to handle real-time data streams ensures it will remain a staple in the developers' toolkit.
- Enhanced Tooling Support: As the ecosystem of tools and libraries continues to grow, startups and larger corporations alike will develop more resources to simplify Kafka's integration with Java.
- Community Engagement: The open-source nature of Kafka invites a vibrant community that contributes to its improvement. Engaging with this community can lead to discovering new patterns and solutions to common challenges.
- Focus on Security and Compliance: As data regulations tighten, future iterations of Kafka will likely place a greater emphasis on security features, ensuring users can manage data safely.
In sum, the journey of integrating Kafka into Java applications has just begun. Keeping an eye on future trends is essential for any developer who aims to stay ahead in the tech game.