Utilizing Jupyter Notebook for Programming Language Tutorials
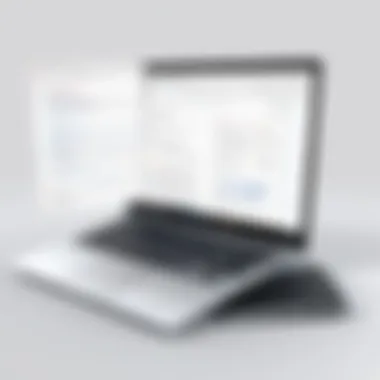
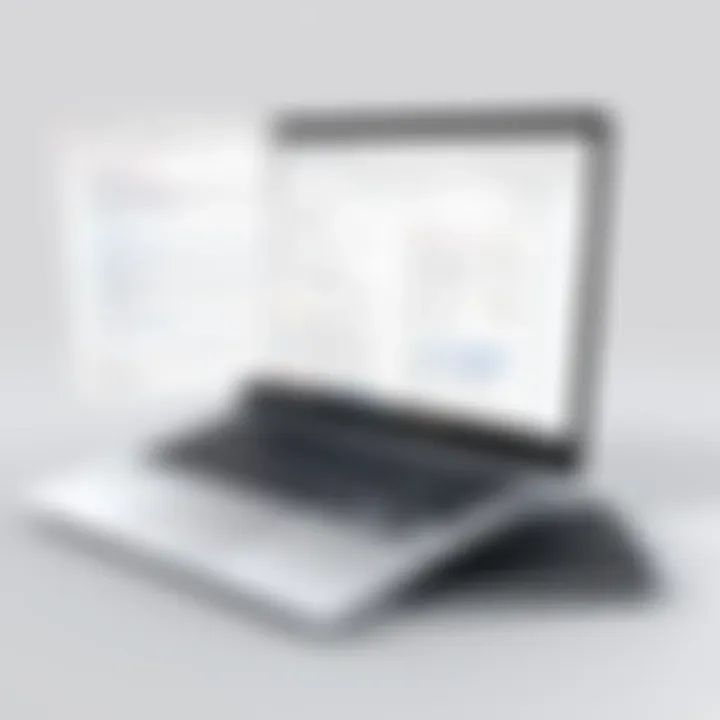
Prolusion to Programming Language
Every program begins with a programming language. These languages are the foundation for constructing applications, automating tasks, and managing data. Languages like Python, Java, and C++ underpin many modern technologies. Their development reflects a need for efficiency and precision in solving complex problems.
History and Background
The journey of programming languages started in the 1950s. Early programming languages such as Fortran and COBOL entered the landscape due to the need for systematic data processing. Over the decades, the evolution continued with languages like C and its derivatives, forming the basis for many subsequent languages. Today, programming languages are crucial in shaping our digital world.
Features and Uses
Programming languages possess unique features. These include explicit syntax rules, various data structures, and built-in libraries to simplify coding tasks. They serve numerous functions. Developers use them to create websites, applications, and systems that enhance user experience. Furthermore, languages support artificial intelligence, data analysis, and scientific computing, broadening their impact.
Popularity and Scope
The popularity of programming languages fluctuates. According to various industry reports, Python has grown significantly due to its simplicity and powerful libraries for data analysis and machine learning. Java remains strong in enterprise applications. C++ continues to be preferred for performance-critical systems. Each language has a specific scope, which influences its adoption in various fields.
Basic Syntax and Concepts
Understanding basic syntax and concepts is crucial before diving deeper into programming tasks. Each programming language has foundational concepts that guide coding practices.
Variables and Data Types
Variables serve as storage for data. They hold various data types such as integers, floats, strings, and booleans. In Python, for example:
This simple example shows how to store a string and an integer in variables.
Operators and Expressions
Operators perform operations on variables and values. Common operators include arithmetic, comparison, and logical operators. For instance, using the addition operator (+) allows summation of two numbers:
Control Structures
Control structures guide the flow of a program. Conditional statements like if-else and looping constructs such as for and while enable dynamic control in programs. For example, in Python:
This code checks if the variable 'age' qualifies as an adult.
Advanced Topics
After mastering the basics, diving into advanced topics becomes essential for developing robust applications.
Functions and Methods
Functions encapsulate code for reusability. They take inputs, perform operations, and return outputs. In Python, a function can be defined as follows:
This function greets a user by their name.
Object-Oriented Programming
Object-oriented programming (OOP) emphasizes objects that encompass both data and functionality. It is central to languages like Java and C++. OOP principles include encapsulation, inheritance, and polymorphism, which enhance code organization and reusability.
Exception Handling
Exception handling is crucial for managing errors. It allows programs to continue running despite unexpected issues. For example, in Python, a try-except block can handle errors gracefully:
This block catches the error and prevents the program from crashing.
Hands-On Examples
Practical coding experience reinforces learned concepts. Hands-on examples build confidence in programming.
Simple Programs
Creating a simple program that prints "Hello, World!" is a classic exercise for beginners. In Python:
This program serves as an introduction to the language's syntax.
Intermediate Projects
As learners progress, they can attempt intermediate projects like a to-do list manager. This project demonstrates data storage, user interaction, and iterative logic.
Code Snippets
Code snippets serve as mini-examples that illustrate specific concepts or problem-solving techniques. They can be reused and adapted for various applications.
Resources and Further Learning
Enhancing knowledge requires access to additional resources. Several options exist for further exploration of programming.
Recommended Books and Tutorials
Books such as "Automate the Boring Stuff with Python" offer practical insights. Tutorials found at sites like W3Schools provide step-by-step guide.
Online Courses and Platforms
Platforms like Coursera and Udacity deliver online courses designed for beginners and advanced learners. These can be fit into personal schedules.
Community Forums and Groups
Joining communities helps learners connect with others sharing interests. Websites like Reddit have programming subreddits where individuals can ask questions and share solutions.
Engaging with the programming community can significantly enhance understanding and retention of programming concepts.
Preamble to Jupyter Notebook
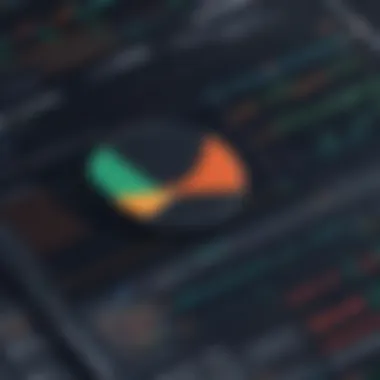
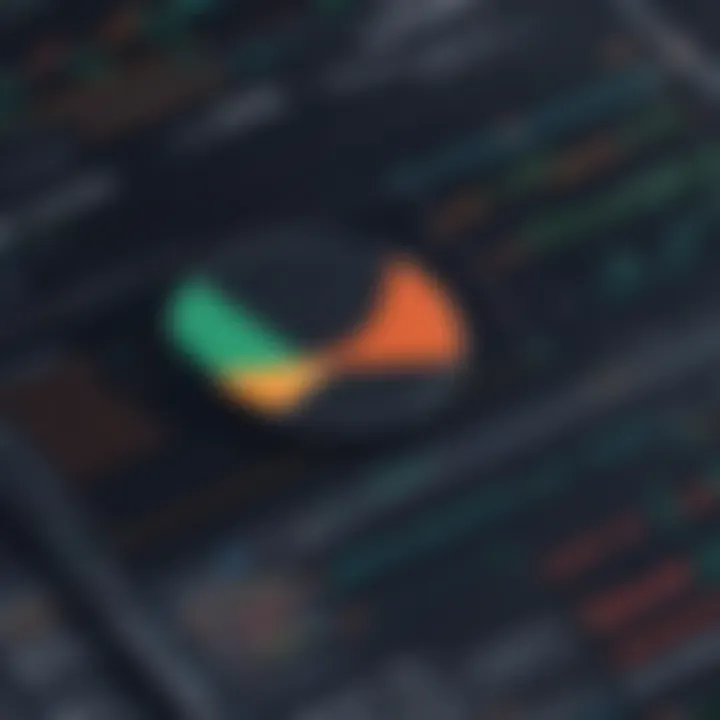
Jupyter Notebook plays a crucial role in the world of programming language education. This tool has become increasingly popular for its intuitive interface and the ability to combine code execution with text, graphics, and other media. It serves as a platform that enhances the learning experience by making programming more accessible and interactive. Students can execute code in real-time, gain immediate feedback, and visualize results seamlessly.
Using Jupyter Notebook, learners get the ability to document their coding process alongside their code. This combination of code and narrative helps in reinforcing concepts and aids in better retention. Moreover, the diversity in functionality enables instructors to create engaging tutorials that cater to various learning styles. As an open-source project, it continuously evolves, which makes it pertinent for current and future educational practices.
Furthermore, Jupyter Notebook supports many programming languages, including Python, Java, and C++. This versatility opens doors for students aiming to explore different languages without needing to switch between multiple tools. The following sections will dissect the essence of Jupyter Notebook and its historical context, key use cases, and various features tailored for programming education.
What is Jupyter Notebook?
Jupyter Notebook is an open-source web application that allows users to create and share documents containing live code, equations, visualizations, and narrative text. It was originally developed for Python, yet it now supports more than 40 programming languages. This tool primarily utilizes the reiteration of code and visual feedback, making it a powerful aid for learners and instructors alike.
History and Evolution of Jupyter
This transition from IPython to Jupyter reflects the broader demand for open and accessible educational tools. Initially targeting researchers and data scientists, the application soon found its place in the teaching space as educators recognized its potential for engaging students.
Key Use Cases in Programming Education
In programming education, Jupyter Notebook presents a multitude of practical applications. Here are some significant use cases:
- Interactive Learning: Students can learn by doing, executing code blocks and observing the outcomes immediately. This feature promotes active engagement with programming concepts.
- Data Visualization: Jupyter supports various libraries like Matplotlib and Seaborn that allow students to visualize data patterns graphically. Such visualization aids in comprehending complex datasets.
- Collaborative Projects: Instructors and students can share notebooks, allowing for easy collaboration on projects or code review. This fosters a community learning environment.
- Documentation and Sharing: Instructors can create comprehensive tutorials that are easy to share with students, ensuring that they can review lessons outside of the classroom setting.
Through these features, Jupyter Notebook stands out as an essential tool for both teaching and learning programming languages. Its impact on programming education is significant, and as we explore further in this article, its setup, interface, and best practices will provide deeper insights into effective usage.
Setting Up Jupyter Notebook
Setting up Jupyter Notebook is a crucial step for anyone interested in using this tool for programming language tutorials. The importance of this topic lies in its foundational role. A proper setup is vital to ensure that users can fully leverage the capabilities of Jupyter Notebook. By configuring it correctly, learners avoid common pitfalls and maximize their coding productivity.
One of the main considerations when setting up Jupyter is the installation method. There are several ways to install Jupyter Notebook, each with its own advantages and disadvantages. Users must choose the method that suits their skill level and project needs. Ultimately, the objective is to reach a state where Jupyter Notebook runs smoothly on the userβs system.
Installation Process
The installation process for Jupyter Notebook involves several key methods. Each has unique aspects that make it suitable for different scenarios. Here is a closer look at the most common ways to install Jupyter Notebook.
Installing Anaconda
Installing Anaconda is one of the most popular ways to get Jupyter Notebook up and running. Anaconda is a distribution of Python and R programming languages, designed specifically for data science and machine learning. Its installation comes with Jupyter and many useful libraries pre-installed. This characteristic significantly eases the setup process.
The unique feature of Anaconda is its user-friendly interface. It includes Anaconda Navigator, which provides a graphical interface to manage packages and environments easily. This makes it a beneficial choice for beginners who may struggle with command line options. However, some experienced developers might find Anaconda's size to be excessive since it installs many packages they may not need.
Direct Installation with pip
Direct installation with pip is another method to use Jupyter Notebook. This approach uses Pythonβs package manager to install Jupyter individually. It's a straightforward process and suitable for those who prefer to have more control over packages.
A key characteristic of this method is its flexibility. Users can install other packages as needed after Jupyter is set up. The downside is that new users may find this method confusing without prior experience. Additionally, potential dependency conflicts can arise if care is not taken during installation.
Verifying Installation
Verifying installation is a crucial step that often gets overlooked. This process ensures that Jupyter Notebook is properly installed and functions as expected. Checking the installation helps in identifying any issues that may have occurred during setup.
A key advantage of verifying installation is it saves time and effort later on, preventing misconfigurations that could disrupt workflow. Users can run a simple command in the terminal or command prompt to confirm that Jupyter Notebook is working. Although it might seem redundant, this step can provide peace of mind when initiating projects.
Launching Jupyter Notebook
Launching Jupyter Notebook is the next step after successful installation. How users choose to launch it can influence their experience. There are two main methods to do this, and each has specific attributes worth noting.
Using Command Line
Using the command line is the first method for launching Jupyter Notebook. This approach requires entering commands directly into the terminal. For those comfortable with text interfaces, this method is efficient and quick.
The key characteristic of using the command line is its directness. Users type to launch the application quickly. However, this method might be intimidating for beginners who are not familiar with command-line interfaces. Nonetheless, mastering it can lead to enhanced productivity.
Using Anaconda Navigator
Using Anaconda Navigator provides a more visual method to launch Jupyter Notebook. Once installed via the Anaconda distribution, users can open Anaconda Navigator and click on the Jupyter Notebook icon. This user-friendly interface gives a visual representation of all environments and tools.
The main advantage of Anaconda Navigator is accessibility. It allows users who are less comfortable with coding to interact with Jupyter confidently. However, it is worth noting that this method may have slower startup times compared to the command line, which could frustrate some users.
In summary, setting up Jupyter Notebook through various methods allows users to tailor the process based on their preferences and familiarity with tools. A well-structured setup will enable learners to engage with programming languages effectively and enhance educational outcomes.
Interface Overview
The interface of Jupyter Notebook is crucial to its effectiveness as a tool for programming tutorials. It provides a user-friendly environment where learners can interact with code and visualize outcomes instantly. Understanding how the interface functions can significantly improve the learning experience. The layout includes cells, toolbars, and menus that serve specific purposes, helping users to navigate easily and implement their programming skills efficiently.
Understanding Notebook Cells
Notebook cells are the building blocks of a Jupyter Notebook. They allow users to organize content in a structured manner, making it easier to present coding lessons.
Code Cells
Code cells allow users to write and execute code directly within the notebook. This feature is integral to programming tutorials, as it enables immediate feedback on the written code. A key characteristic of code cells is their execution flexibility, which means code can be rerun as often as necessary without leaving the notebook context. The ability to visualize errors and outputs instantly makes code cells a popular choice among learners. One advantage of code cells is the ease with which they support interactive coding sessions, enhancing the learning process. However, they might be less effective for long, complex code snippets without proper structuring.
Raw Cells
Raw cells offer a space for unprocessed text and do not allow for code execution or formatting. Their primary contribution is that they can store information that does not need to be executed or rendered. A key characteristic of raw cells is their versatility in accepting plain text, which can include code snippets or configuration details. This makes raw cells a good choice for tutorials that require context or explanatory notes separate from the code itself. One unique aspect of raw cells is their ability to serve as a transport medium for content between different notebook formats. However, their lack of formatting options and execution capabilities can limit their effectiveness in conveying educational material compared to the other cell types.
Toolbar Functions
The toolbar of Jupyter Notebook provides essential functions that streamline the user experience. It contains tools for file management, editing options, and kernel management, all of which contribute to an efficient workflow during programming tutorials.
File Operations
File operations in the toolbar allow users to manage their notebooks easily. Users can create new notebooks, save existing ones, and export their work in various formats. A key characteristic of file operations is their simple interface that facilitates quick access to essential functionalities. This makes it a valuable feature during programming tutorials where saving progress is crucial. The export feature distinguishes it by allowing learners to share their work seamlessly. However, the downside is that some users may overlook the need for regular saving, risking the loss of unsaved changes.
Edit Options
Edit options in the toolbar are designed to enhance the coding experience. They allow for easy manipulation of notebook content, such as undoing changes, cutting, copying, and pasting cells. The main advantage of these edit options is the increased efficiency they bring to working with multiple cells and refining code. Their comprehensive nature makes them beneficial in a learning context, especially when trying different approaches to a coding problem. Nevertheless, beginners may find the range of options overwhelming, which can slow down the learning process initially.
Kernel Management
Kernel management is a critical feature of Jupyter Notebook, as it controls the execution environment for the code. It allows users to start, stop, and restart the kernel as necessary. A key characteristic of kernel management is the seamless switching between different programming languages and environments, which enhances flexibility in tutorials. This feature is beneficial as it allows users to work with various programming languages within one interface. However, improper management can lead to crashes, disrupting the user experience and delaying learning.
It's essential to understand the features of the Jupyter Notebook interface. This knowledge aids in maximizing the learning potential and promotes a smoother programming journey.
Programming Languages in Jupyter Notebook
Jupyter Notebook serves as a versatile platform for learning various programming languages. This is crucial as it allows learners to interactively engage with code, which significantly enhances the educational experience. By facilitating this hands-on approach, Jupyter Notebook makes it possible for learners to experiment, visualize outcomes, and troubleshoot errors in real-time. Besides, the notebook's feature of combining code with rich text allows for integrated documentation and explanations, making it easier to grasp complex concepts.
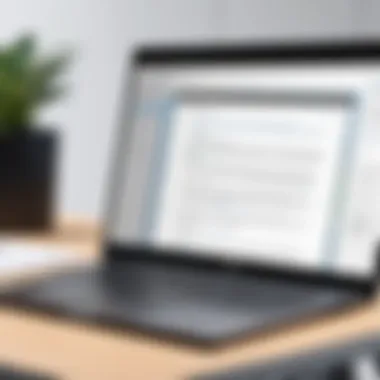
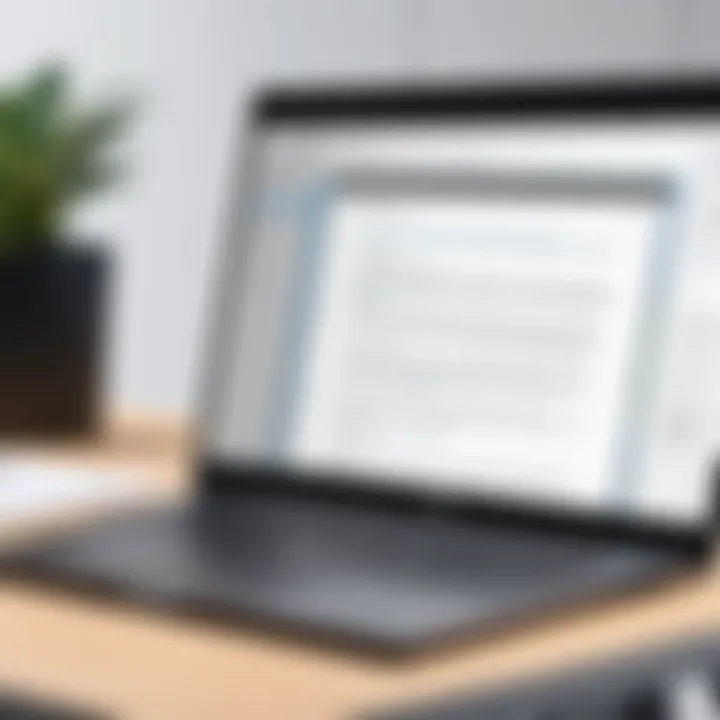
The integration of multiple programming languages further broadens the scope of Jupyter Notebook. While it is inherently designed for Python, it can support other languages like Java and C through appropriate kernels. The resulting flexibility gives learners opportunities to explore different environments without the need to switch tools or interfaces. Each language comes with its characteristics and applications, thus allowing for a comprehensive learning experience.
Python Programming
Prelude to Python in Jupyter
Python is one of the most popular programming languages used in Jupyter Notebooks for various reasons. Its simplicity allows beginners to focus on learning programming concepts without being bogged down by complicated syntax. The immediate feedback loop provided by running Python code in Jupyter is particularly useful; users can write small snippets of code and see results instantly, which is beneficial for understanding programming flow and logic.
A notable characteristic of using Python in Jupyter is the ease of integrating libraries such as NumPy and Pandas. These libraries allow users to handle data effortlessly. However, one must note that Python is dynamically typed, which can sometimes lead to runtime errors that may confuse beginners. Nevertheless, projects employing Python in Jupyter often yield fruitful results, making it a popular choice for users.
Running Python Code
Running Python code in Jupyter Notebook can be seen as one of the essential features for learners. The process is straightforward: users can write their code in a code cell and run it to see the output immediately. This immediacy is vital for experimentation and debugging. Beginner coders can check their understanding of functions and libraries right away, promoting a more practical learning environment.
The simplicity of running code comes with a downside. Sometimes, the output can be misleading if users do not fully understand how scope or variable types work in Python. Nonetheless, having a place to quickly test ideas greatly enhances the learning curve for any user.
Visualizing Data with Matplotlib
Data visualization is crucial in today's data-driven world, and using Matplotlib in conjunction with Python in Jupyter Notebook can make this task feel more achievable. Users can produce a variety of plots to represent data graphically. This engagement often leads to deeper insights, assisting the learners in interpreting results clearly and effectively.
The accessibility of Matplotlib makes it a favored choice. Its integration with Jupyter Notebooks simplifies the process of visualizing complex data sets. However, it is important to consider that initial setup may require users to learn some basic syntax, which could be daunting for absolute beginners. Yet, once learned, the ability to visualize data is an invaluable asset in programming education.
Using Jupyter for Java Code
Setting Up Java Kernel
Jupyter Notebook's capability to support Java illustrates its versatility. To handle Java code within Jupyter, users generally need to set up a specialized kernel, such as IJava. This setup allows Java programmers to benefit from the interactive features of Jupyter while working in Java, facilitating a unique combination of coding and documentation.
A key advantage of setting up the Java kernel is the ability to execute Java code in an environment familiar to those who may have previously used Jupyter for Python. It offers a comprehensive platform for users familiar with Java syntax and enables quick debugging processes. On the flip side, users must navigate the initial setup process, which can feel complicated for some.
Executing Java Code Samples
The execution of Java code samples in Jupyter Notebook provides another significant benefit. Users can run chunks of Java code and receive immediate feedback, similar to what they experience in Python. This feature supports learning through trial and error, which is effective when grasping Java's object-oriented principles.
The flexibility to combine text and code facilitates better understanding. Yet, considering the differences in error handling between Java and Python, beginners might experience frustration when applying familiar debugging techniques that work in Python.
Integrating Jython
Integrating Jython into the Jupyter environment adds another layer of adaptability for users inclined towards Python and Java. Jython allows users to execute Python code in a Java Virtual Machine. This integration bridges the two languages, enabling the use of Java libraries directly within Python code in Jupyter.
The notable feature of integrating Jython boosts the learning curve for users looking to transition between the two programming languages. However, the performance can vary, and users may encounter compatibility issues that might require adjustments or a learning curve to resolve.
Exploring and ++ Integration
Installing IRkernel for R
Integrating C and C++ within Jupyter requires installation of the IRkernel, which provides an interface for R, aiding users in execution and code visualization. This addition allows for the interplay between R and C, expanding a user's ability to handle computational tasks effectively.
The installation process is relatively straightforward, enhancing Jupyter's multifunctionality. However, as C and C++ are lower-level languages, learners often face a steeper learning curve in understanding memory management and debugging compared to higher-level languages like Python.
Writing and ++ Code
Writing C and C++ code in Jupyter Notebook enables programmers to experience both languages in an interactive, user-friendly format. Highlighting key syntactical differences and understanding their implications in real-time helps learners digest complex concepts.
The compelling aspect of using Jupyter for C and C++ programming is the immediate response to code execution. Despite this, beginners will likely struggle with aspects like pointer usage or memory allocation without proper guidance.
Compiling and Running Code
Compiling and running C code within Jupyter adds significant value to the user's learning experience. It offers insights into compiler behavior and code optimization techniques, crucial for understanding how C works under the hood.
While benefits exist, the requirement to manage compilations can introduce an additional layer of complexity for users. This may lead to frustration without familiarity with compilation processes. Nonetheless, the practical application fosters a comprehensive understanding the language, enriching the overall learning experience.
Best Practices for Using Jupyter Notebook
Using Jupyter Notebook effectively involves more than just running code. It requires thoughtful organization and clear documentation. This section outlines some best practices to enhance the learning experience. These best practices not only streamline coding but also improve understanding for those who are teaching or learning programming languages.
Organizing Notebooks
Naming Conventions
Naming conventions are crucial in managing Jupyter Notebooks. They provide clarity regarding the content and purpose of each file. A well-chosen name can instantly convey information about the notebook's focus. For instance, name your notebook . This makes it easy to identify and locate specific notebooks in a large collection.
Adopting consistent naming conventions aids collaboration. When multiple users work on projects, uniform names minimize confusion and enhance productivity. Clearly defined conventions may include using underscores or dashes to separate words and being concise yet descriptive.
However, overly complex names may hinder quick recognition. An balance should be sought between name length and informativeness, as too many details can clutter the workspace.
Directory Structure
A well-thought-out directory structure is important for organizing Jupyter Notebooks. It affects accessibility and the overall user experience. Group related notebooks into folders based on categories like course subject, project type, or programming language. This practice makes navigating easier and fosters efficient workflows.
Maintain a hierarchy that reflects the project backend. For instance, you can have a folder containing subfolders for various projects. Clear structures reduce the time spent searching for files and improve focus on tasks at hand.
Although this structure provides benefits, it requires regular maintenance. If not managed well, folders can become cluttered, which defeats their purpose. Regular reviews and adjustments are necessary to maintain clarity and organization.
Commenting and Documentation
Documenting Code Changes
Documenting code changes is an essential habit for programmers. It fosters transparency and understanding in collaborative environments. Consistently noting adjustments made to the code helps others understand the evolution of the project and the rationale behind revisions.
This practice can range from commenting within the code to maintaining a detailed changelog. Each entry should summarize what was changed, why, and any implications of the change.
Adequate documentation can mitigate confusion and streamline onboarding processes for new team members or students. While it may seem tedious, thorough documentation pays off in the long run by enhancing code readability. Moreover, it reduces the cognitive load required to comprehend modifications.
"Good documentation is crucial. It not only assists others but also helps you remember your own thought process."
To conclude, the best practices mentioned above create a strong foundation for utilizing Jupyter Notebooks. They contribute to efficient organization and clear communication among users, essential for the learning and teaching of programming languages.
Troubleshooting Common Issues
In the journey of mastering programming languages through Jupyter Notebook, encountering problems is not uncommon. This section aims to address common issues that users might face after launching Jupyter Notebook. Understanding these issues can drastically improve your learning experience. Resolving kernel errors, rendering issues, and package installation conflicts are critical for ensuring smooth operation of Jupyter Notebook and effective code execution.
Kernel Errors
Kernel errors often arise when Jupyter Notebook cannot start the kernel for a specific programming language. This can be attributed to several reasons, such as an incomplete installation or a corrupted environment. Kernel errors are significant as they impede your ability to execute code, which is counterproductive to learning.
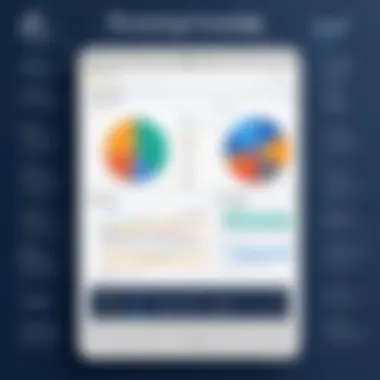
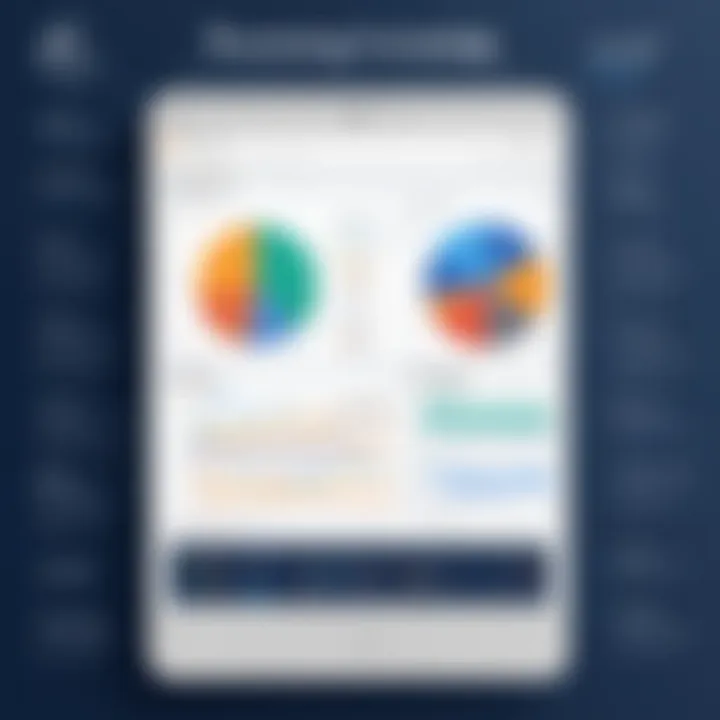
To troubleshoot kernel errors:
- Check your installation: Ensure that Jupyter Notebook and the respective kernels for your programming languages are correctly installed.
- Restart the kernel: Sometimes, simply restarting the kernel can resolve temporary glitches.
- Update packages: Ensure that all libraries and dependencies are up to date, as outdated packages can lead to compatibility issues.
If the problem persists, consulting forums such as Reddit can provide insights into similar experiences from other users.
Rendering Issues
To mitigate rendering issues:
- Clear your output: Periodically clear the outputs of your cells, as excessive output can slow down rendering.
- Use the latest version: Sometimes, simply using an updated version of Jupyter Notebook can fix rendering bugs.
Effective visualization is vital for understanding complex data sets, making troubleshooting rendering issues important.
Package Installation Conflicts
Working with multiple programming languages in Jupyter Notebook may lead to package installation conflicts, especially if different languages or projects require varying library versions. These conflicts can disrupt the expected functionality of your projects.
To address package installation conflicts:
- Use virtual environments: Creating separate environments for different projects helps isolate package installations and avoid conflicts.
- Check dependencies: When installing new packages, pay attention to the dependencies to ensure compatibility.
- Reinstalling packages: If a package is behaving unexpectedly, uninstalling and reinstalling it can resolve issues.
Enhancing Jupyter Notebooks with Extensions
Enhancing Jupyter Notebooks through extensions is crucial for optimizing its capability in teaching programming languages. It allows users to customize their experience and tailor the environment to meet specific needs. Extensions can provide additional functionalities such as improved code formatting, data visualization enhancements, and user interface modifications. These enhancements promote interactivity and engagement, making the learning process more effective for students.
Popular Jupyter Extensions
Nbextensions
Nbextensions is a popular package that adds a range of tools and features to Jupyter Notebooks. One specific aspect is its ability to enable additional functionalities like collapsible headings, table of contents navigation, and code folding. The key characteristic of Nbextensions is its ease of use; users can activate or deactivate the extensions directly from the notebook interface without modifying the code.-
This choice is beneficial for beginners who may not be familiar with Python programming yet want to enhance their notebooks. One unique feature of Nbextensions is the presence of a user-friendly graphical interface. This allows users to easily select the extensions they want to use, making it accessible for all skill levels. While Nbextensions offers many advantages, one consideration is that it may introduce compatibility issues with certain Jupyter configurations.
JupyterLab
JupyterLab is beneficial because it allows users to work seamlessly with different file types, including notebooks, scripts, and data files. A unique feature is the ability to create multiple tabs and panes, offering a more productive workflow. However, since it is more complex than the classic notebook interface, it may have a steeper learning curve for new users.
Interactive Widgets
Interactive Widgets is an extension that allows users to create interactive graphical elements such as sliders, buttons, and drop-down menus directly within Jupyter Notebooks. This aspect enhances the learning experience by allowing students to manipulate variables and see real-time updates in results and visualizations. The key characteristic of Interactive Widgets is that it enables more hands-on experimentation with coding concepts and data analysis techniques.
The benefit of using Interactive Widgets is that it caters to different learning styles by making the material more engaging. A unique feature is its ability to link widgets to Python code, creating dynamic visualizations that automatically refresh when user inputs change. On the downside, implementing these widgets can sometimes require a good understanding of the underlying code, which might challenge less experienced users.
Installing Extensions
Installing extensions in Jupyter Notebooks is essential for accessing their full potential and enhancing your overall experience. Here are two common methods for installing them.
Using pip
Using pip is one of the easiest ways to install Jupyter extensions. One specific aspect of using pip is that it leverages Python's package management system. The key characteristic of pip is its straightforward syntax, allowing users to install extensions with simple commands such as .
This method is beneficial because it easily integrates with other Python packages and maintains package dependencies efficiently. The unique feature of pip is its wide usability, as nearly all Python packages can be installed through it. However, users should be cautious of potential version conflicts between packages when using pip, which can cause problems in the notebook environment.
Using conda
Using conda for installing Jupyter extensions is another effective method, especially for users who manage environments and dependencies carefully. The specific aspect of conda is its ability to create isolated environments, which can help avoid conflicts between different package versions. The key characteristic is that users can control package versions effectively, leading to a more stable coding environment.
Using conda is beneficial for those who require robust management of dependencies and environments. A unique feature of conda is its channel system, which allows users to access packages from different sources. On the downside, conda can be slightly more complex than pip for users who are unfamiliar with environment management, which might lead to confusion for beginners.
Case Studies and Practical Applications
Engaging with real-world applications of Jupyter Notebook enables learners and educators to grasp its full potential in programming education. This section will delve into two main areas: how Jupyter is used in data analysis projects and its role in machine learning workflows. Practical case studies help illustrate the versatility and advantages of Jupyter, turning abstract concepts into concrete skills.
Real-world Applications of Jupyter
Data Analysis Projects
Data analysis projects highlight the significance of using Jupyter Notebook for handling large datasets and conducting exploratory data analyses. This aspect is vital since effective data manipulation periods remain a foundational skill for programmers and analysts alike. Jupyter provides an interactive environment that is indispensable for visualizing data interactively while conducting analyses on the fly. The ability to mix both descriptive text and executable code is a key characteristic that enhances understanding and clarity within the analysis.
One unique feature of these projects is the use of visualization libraries such as Matplotlib and Seaborn. These tools facilitate the creation of plots and charts directly within the notebook. As a result, this enhances the data comprehension process, allowing users to present findings visually. However, one must be cautious with the complexities of very large datasets, as performance can be an issue depending on the system's limitations.
Machine Learning Workflows
When discussing machine learning workflows, Jupyter Notebook stands out as a critical tool for experimentation and iterative development. The importance of machine learning in various sectors cannot be understated as it drives innovation and decision-making. The interactive nature of Jupyter allows developers and data scientists to run multiple trials with ease, tweaking parameters in real-time.
What makes machine learning workflows particularly beneficial here is Jupyter's ability to integrate various libraries like TensorFlow and Scikit-learn seamlessly. This unity allows for quick testing of models and evaluation of results. An advantage of using Jupyter is its capability to document processes comprehensively. Yet, machine learning can sometimes involve extensive data preprocessing pipeline, which might slow down the training process.
Teaching Programming with Jupyter
Course Development
Incorporating Jupyter Notebook into course development is increasingly valued in modern educational contexts. One specific aspect is the design of curricula that integrates practical assignments and examples. This contribution makes programming concepts more relatable and easily digestible for students. The key characteristic of this approach is the focus on experiential learning, where students can work through code and see immediate results.
Jupyter allows instructors to scaffold learning experiences by embedding questions and exercises within notebooks, thus guiding learners through key concepts with structured pathways. The unique feature here is the enhancement of the learning experience while bridging theoretical knowledge with application.
Student Engagement Strategies
Using Jupyter Notebook as a tool for student engagement strategies is becoming popular. The specific aspect often discussed includes how interactive tutorials maintain student interest. One essential characteristic of Jupyter in this context is the promote of active learning. Hands-on coding practices help keep learners engaged, allowing them to explore examples and edit code as they progress.
Notebooks can host quizzes and even interactive visualizations that respond to user input. The advantage here is the immediate feedback it generates, reinforcing learning in real time. A downside could be technical issues students might face if they are unfamiliar with the interface, leading to frustration.
By providing an avenue for exploration and demonstration of concepts, Jupyter Notebook is becoming a go-to resource in the realm of programming education. Its practical applications are almost limitless, making it an essential asset for both learners and educators.
Culmination
The conclusion serves as the final synthesis of the insights presented throughout the article. It is significant because it reinforces the key elements discussed and helps readers internalize the concepts. The objective of this section is to summarize critical takeaways while also allowing space for forward-looking thoughts about the future of Jupyter Notebooks in programming language tutorials.
Summary of Key Points
In the preceding sections, we covered numerous aspects essential to effectively utilizing Jupyter Notebook for programming tutorials. Here are the primary takeaways:
- Jupyter Notebook is an interactive environment, particularly useful for teaching programming concepts.
- Installation can be achieved through multiple methods, simplifying accessibility.
- Jupyter supports various programming languages like Python, Java, and C++, allowing versatility for educators and learners alike.
- Best practices in organization and documentation enhance the effectiveness of teaching.
- Troubleshooting common errors leads to a smoother learning experience.
- Extensions can augment the core functionality of Jupyter, offering additional tools and capabilities.
- Practical applications and case studies provide real-world context, making the learning process relevant.
Future Trends in Jupyter Notebooks
The future of Jupyter Notebooks appears promising, particularly as educational needs evolve. A few trends to consider include:
- Increased Integration with Online Platforms: As cloud computing becomes more prominent, we can expect better integration with online services, thus enabling remote work and collaboration in real-time.
- Enhanced Data Science Features: As data science continues to gain traction, Jupyter may evolve to include built-in features catering specifically to data analysis and machine learning workflows.
- Focus on Interactivity: With advancements in user interface technologies, future versions of Jupyter could become even more interactive, enriching the overall experience for learners.
- Broader Language Support: As the tech landscape shifts, support for emerging languages could be expanded, ensuring that Jupyter remains relevant for various programming contexts.
- Improved Resource Management: Optimizations around resource usage and execution times can make Jupyter more efficient, particularly in educational settings where multiple users access shared resources.
The landscape of programming education is changing, and tools like Jupyter Notebook are at the forefront, helping learners navigate this shift.
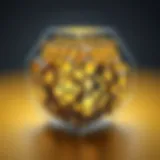
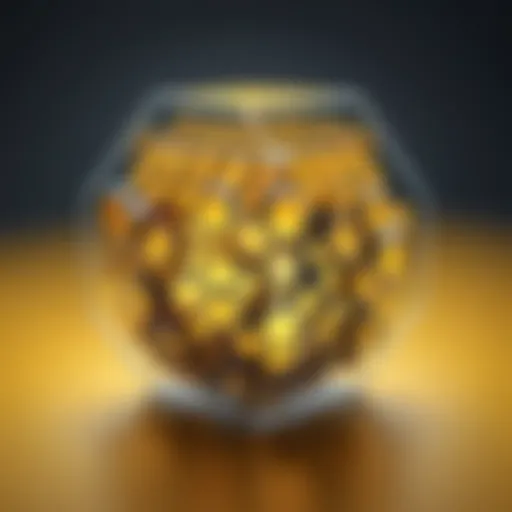