A Comprehensive Guide to Learning React Effectively
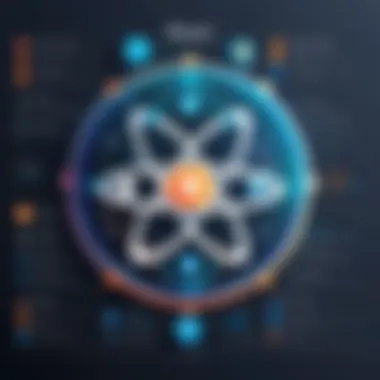
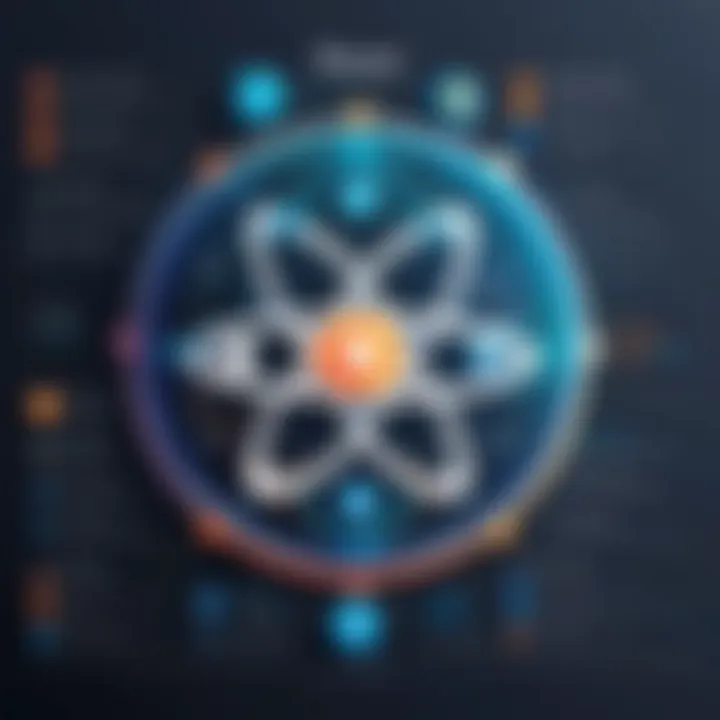
Preface to Programming Language
When discussing React, it's crucial to step back and understand the broader context of programming languages, especially JavaScript. JavaScript, initially developed in 1995 by Brendan Eich, has evolved dramatically from its early days as a simple scripting language for enhancing web pages. Back then, many saw it merely as a tool for minor tweaks to websites, but today, it reigns supreme in web development.
History and Background
The journey of JavaScript began with the desire to create an interactive web environment. In its infancy, it was used primarily for tasks like form validation and simple animations. However, as websites became more complex, so did JavaScript. In 2009, the introduction of Node.js took JavaScript beyond the browser, allowing developers to use it for server-side programming as well. This paved the way for frameworks and libraries like React, which we now turn our focus on.
Features and Uses
React is a JavaScript library that serves a specific purpose: building user interfaces, predominantly for single-page applications. It provides a component-based approach, allowing developers to encapsulate UI elements efficiently. Notable features of React include:
- Virtual DOM: React utilizes a virtual representation of the real DOM, which increases performance by minimizing direct updates to the UI.
- Reusability: Components allow for code reuse, making development faster and more manageable.
- Unidirectional Data Flow: Data in React flows in one direction, simplifying the debugging process and ensuring predictability in how components interact.
- Rich Ecosystem: With tools like Redux for state management and React Router for navigation, React's ecosystem is vast and supportive, catering to a variety of development needs.
Popularity and Scope
As of today, React has become one of the most popular libraries in the web development world, arguably rivaling the likes of Angular and Vue.js. Its adoption by major companies such as Facebook, Instagram, and Airbnb highlights its robustness and versatility. The library's growing community also fosters an environment where developers can share knowledge and best practices, ensuring that new learners have access to ample resources.
Understanding the foundational principles of JavaScript is essential before diving into React. With a strong grasp of this programming language, students and aspiring developers can fully appreciate React's capabilities and innovations, laying a solid groundwork for advanced programming concepts to come.
"Programming isn't about what you know; it's about what you can figure out."
ā Chris Pine
Moving Forward
As we transition towards the fundamentals of React itself, itās vital to cover the basic syntax and core concepts that both newcomers and seasoned developers must navigate. Letās delve deeper into the building blocks of this powerful library.
Preamble to React
In the rapidly evolving landscape of web development, the ability to create dynamic and interactive user interfaces is crucial. This is where React enters the picture. As a library focused on building UI components, React allows developers to construct applications that are not only efficient but also easy to maintain. By handling the complexities of UI design, React enables developers to focus on how their applications function and interact with users.
What is React?
React is an open-source JavaScript library maintained by Facebook and a community of individual developers. It serves as a tool for building user interfaces, allowing the creation of single-page applications with a component-based architecture. What sets React apart is its virtual DOM, which optimizes rendering and improves performance. In simpler terms, you can think of React as a toolkit that helps in breaking down the UI into manageable, reusable pieces known as components.
More than just a tool, React has become a cornerstone for developers aiming to create responsive, efficient UIs. With roots tracing back to 2011, it has evolved into a popular choice for both startups and established tech giants.
The Importance of Learning React
Understanding React opens numerous doors in todayās job market. Developers with knowledge of this library are in high demand due to its widespread use in modern web applications. By learning React, you not only equip yourself with a powerful development tool but also learn fundamental concepts that apply to various JavaScript frameworks.
Some benefits of learning React include:
- Efficiency: Reactās component-based structure allows for reusability, which cuts down on development time.
- High Performance: With its optimized rendering through the virtual DOM, apps built with React tend to be faster than traditional JavaScript.
- Strong Community Support: An active community means access to a wealth of resources, from tutorials to forums where problems can be solved.
"Learning React equips you with skills that transcend just one libraryāit's about mastering the art of building modular, efficient applications."
When to Use React
React is suitable for various applications, particularly those that require dynamic content updates and real-time data changes. Here are some scenarios where using React shines:
- Single-Page Applications (SPAs): React is a go-to for SPAs, where user experience relies on swift interactions without reloading the entire page.
- Complex User Interfaces: For applications that have numerous interactive elements, like dashboards or data-heavy applications, React offers clarity and efficiency.
- When Speed Matters: If performance is a key concern, Reactās ability to manage DOM manipulation smartly can greatly enhance loading times.
In summary, React is not just another library; it is a powerful ally for developers. Understanding its core principles can fundamentally enhance how one approaches building web applications.
Setting Up the Development Environment
Setting up the development environment is like laying the ground work before building a house. If you don't have a solid foundation, everything you build might come crashing down. In the world of React, this environment involves installing the right tools needed to create and manage your applications efficiently. A well-configured environment can save you countless hours of frustration and confusion.
To make your React development journey smooth, you'll need to install certain key elements that enable the smooth functioning of your apps. It's essential to understand that these installations are not just forms of busywork; they are fundamental steps that ensure you have everything you need at your fingertips.
Installing Node.js and npm
Node.js is like the secret sauce that makes your React application run on the server side. It allows you to build and interact with your applications comfortably. npm, which stands for Node Package Manager, is bundled with Node.js and helps manage all the third-party libraries you will inevitably pull into your project. Hereās how to set it up:
- Download Node.js: Head over to Node.js's official website to download the installer appropriate for your operating system.
- Install the Software: Follow the prompts to install Node.js on your computer. This step usually is a no-brainer; just click next until it's done.
- Verify Installation: To check if youāve installed Node.js correctly, open your terminal or command prompt and run:You should see version numbers pop up. If no errors arise, you've got a successful installation!
Creating a New React Application
Once Node.js and npm are ready to roll, itās time to create a new React application. The common tool for doing this is Create React App, a boilerplate generator that sets up everything for you. Think of it as getting a brand new, shiny bikeācomplete with gears and breaksāready to ride without worrying about the nitty-gritty mechanics.
Hereās how to proceed:
- Use npx: npx (which comes with npm) enables you to run packages without having to install them globally. In your terminal, run:Replace with the name you'd like to give your application.
- Navigate: After the project setup is complete, navigate into your project by typing:
- Start the Development Server: Finally, to see your newly minted app in action, run:Your application will open in a web browser, displaying some generically pleasant textāthe default React template.
Understanding Project Structure
A well-structured project is crucial for both productivity and maintainability. When you create your React application using Create React App, youāll notice a predefined structure that helps you organize your code effectively. Understanding this layout is key to managing your project efficiently.
In a typical Create React App project, youāll find:
- : Contains all your app's dependencies. You wonāt need to touch this folder, but it's crucial for the projectās operation.
- : The static files live here, like your index.html. This is the entry point of your application.
- : Most of your code will reside in this directory. Itās where youāll build your React components and application logic.
- : This file gives you access to scripts and lists your dependencies, among other settings. Anytime you install a new library, it'll show up here.
Understanding these pieces helps you navigate effectively through your projects, allowing for smoother collaboration and easier debugging.
Remember: A strong grasp of your projectās folder structure can significantly cut down on the confusion, especially when working on larger applications.
By mastering the development environment setup and understanding the structure of your React application, you're well on your way to building impressive user interfaces with confidence.
Core Concepts of React
Understanding the core concepts of React serves as a bedrock for anyone diving into this powerful JavaScript library. The building blocks of React are designed to enhance your ability to create dynamic web applications. Knowing how components interact, manage data, and handle user inputs will not only simplify your development process but also allow for more scalable and maintainable code. Let's break down these essential ideas that make React tick.
Components and Props
At the heart of any React application are components. Think of them as building blocks, just like bricks in constructing a house. Each component is a self-contained unit that can include its logic, styles, and rendering behavior. Components can be functional or class-based, and each brings its unique advantages.
Props, short for properties, are the means through which components communicate. You pass props from parent components to child components, allowing data to flow down the hierarchy. This flow of information is fundamental for building interactive UIs.
For example, when creating a simple button component, you can pass the label text as a prop:
This can keep your components reusable and clean. The best practice here is to ensure that your components are as dumb as they can beālet them receive data and do just one job well. The key takeaway: components structure your application while props enable communication.
State and Lifecycle
While props are essential for data flow, state is the component's internal data storage. It allows components to keep track of changes and respond to user actions. For instance, a form component can maintain the input fieldsā values through the state.
Lifecycle methods give developers control over a componentās existence during the various phases of its lifeāfrom mounting to updating to unmounting. Understanding these phases is crucial for optimizing performance and managing resources.
Consider the following example, where a component's state changes when a user clicks a button:
Understanding state and lifecycle management not only helps in creating reactive and user-friendly interfaces but also contributes to application performance.
Handling Events
User interactions are at the core of web applications, and React provides a straightforward way to manage these through event handling. Unlike traditional JavaScript, React normalizes eventsāthis means that you can use React's event system instead of dealing with the browserās differences.
Events in React are camelCase, and you can pass functions as event handlers. For example, to respond to a click event, simply attach an onClick handler:
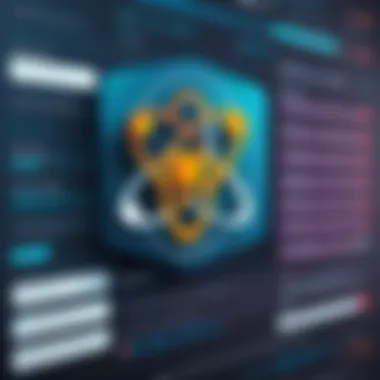
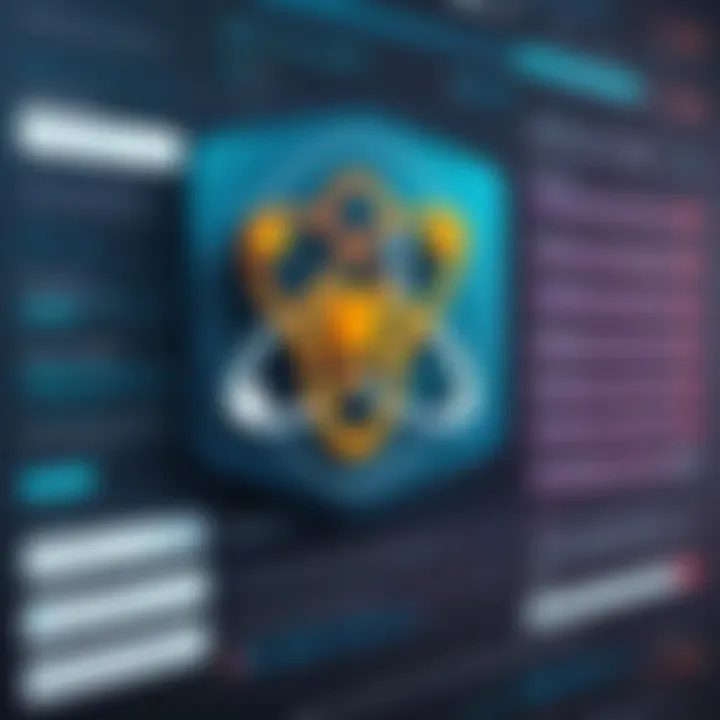
Another important point is that event handlers in React do not automatically bind the current , so you often have to use either arrow functions or binding in the constructor.
Using events effectively contributes significantly to interactivity in your applications. It effectively transforms static components into responsive components that users can engage with.
In summary, grasping the core concepts of Reactācomponents and props, state and lifecycle, and event handlingāis crucial for building robust applications.
These fundamental ideas form the backbone of React development and pave the way for more advanced techniques later on. Keep practicing these concepts to become adept at leveraging React to its full potential.
Building Your First React Application
Embarking on the journey of building your first React application is akin to taking a deep dive into a pool of immense potential. The ability to conceptualize and construct a tangible project is not just a simple task; it encapsulates the heart of learning in any programming discipline. In the context of React, this initial step opens up a world of opportunities for budding developers, enhancing their skills and boosting their confidence.
Developing your first app serves several crucial functions. Firstly, it consolidates understanding of theoretical concepts youāve learned thus far. You might find the principles behind components, props, and state dancing in your head, but until you actually implement them, they remain abstract ideas. Furthermore, the hands-on experience provides a practical insight into Reactās ecosystem ā a pathway to learning how everything clicks together. Learning by doing is genuinely the key takeaway.
Another noteworthy aspect is problem-solving. Building an application isnāt just about writing code; it's about encountering challenges, debugging them, and emerging with solutions. This problem-solving mindset will become an invaluable asset as you delve deeper into React and programming in general.
Additionally, once you step into the real-world application of your skills, you'll realize how interconnected knowledge becomes. You may start with just understanding simple components, yet as you progress, the complexity intertwines beautifully and creates intricate applications capable of offering users intuitive experiences.
Setting Up Your Component
When initiating your React project, the first order of business is setting up your component. This is where you define the building blocks of your application. Think of components as the Lego bricks that form the foundation of a house. Each brick serves its purpose, yet together they create something functional and beautiful.
In practical terms, a component can be created using either a class or a functional component. Newer React developers are encouraged to start with functional components, largely due to their simplicity and the rise of hooks. To kick off the creation of a component, you would typically write:
This snippet demonstrates how to create a basic functional component. Once you've crafted your component, it's essential to import it into your main application file so it can be rendered.
Rendering Elements
Rendering elements is the next logical step in your React journey. Once you've established your components, itās time for them to shine on the screen. Rendering is essentially how your components output HTML. React takes care of this process more elegantly than traditional JavaScript methods by allowing you to use JSX ā a syntax extension that resembles HTML.
Hereās a quick look at how you can render your component within the app:
This structure takes your and inserts it into the DOM element with the of . Itās remarkable how succinctly React bridges the gap between your code and the visual representation on the web.
Styling Components
Every piece of art needs a touch of flair. Similarly, styling your components is where aesthetics and functionality meet in your React app. There are a handful of methods to apply styles in React: inline styles, CSS stylesheets, and CSS-in-JS solutions.
Inline styling might appeal to you for its simplicity. You can style right within your JSX as follows:
External stylesheets are equally straightforward. Simply link a CSS file within your project. This keeps your styles separate from your logic, which might be useful for larger applications. You can have a file called and import it into your component:
Lastly, CSS-in-JS approaches, such as styled-components, are an elegant way to scope your styles to the component itself, avoiding any style bleed.
Advanced React Concepts
Understanding advanced concepts in React is pivotal for any developer aiming to master the library. These concepts go beyond the basic building blocks of React, allowing developers to create more efficient, maintainable, and scalable applications. With a firm grasp of these concepts, youāll not only enhance your coding skills but also streamline your development process, making it less cumbersome.
Context API
One of the standout features of React is its Context API. This comes into play when dealing with state management across various component levels. Rather than prop-drilling, or passing props through every intermediary component, the Context API enables a more streamlined approach.
Imagine a scenario where you have a theme or user authentication state that multiple components need access to. Instead of juggling props and potentially introducing bugs, you can create a context provider that sits at a higher level in your component tree. This way, any component within that provider can easily access the data without unnecessary duplication or confusion.
"The Context API can be a developer's best friend when managing global state efficiently."
To set up the Context, you would typically create a context object using . This object provides a component to encapsulate the state and a to access it. Hereās a snippet to illustrate:
This pattern provides a clean way to manage shared state across your application.
Hooks in React
React Hooks, introduced in version 16.8, marked a significant shift in how developers handle state and side effects in functional components. Before hooks, managing state was often reserved for class components. Hooks, however, empower developers to utilize state and lifecycle features without the complexity of classes.
The most commonly used hooks are , , and . The hook is simple yet powerful, enabling state management within functional components. Hereās how you might use it:
handles side effects, such as data fetching or subscribing to an event. It consolidates lifecycle methods like and into a single API, making it more intuitive:
By incorporating hooks, developers can write more concise and readable components.
Higher-Order Components
Higher-Order Components (HOCs) are another advanced React technique, acting as functions that take a component and return a new component. This abstraction is particularly useful for code reuse. A common use case for HOCs is in handling functionality like authentication, data fetching, or logging.
A simple HOC could be one that adds a loading spinner to a component while fetching data:
This allows you to wrap any component with loading functionality without modifying its original implementation:
Through HOCs, components can remain focused on their core functions while simply extending their behavior. This manipulation provides a cleaner separation of concerns within your application.
Equipped with these advanced concepts, developers can function more effectively within the React ecosystem, creating applications that are robust and responsive to change. Mastery over the Context API, Hooks, and Higher-Order Components will not only enhance your skill set but also your application's performance and maintainability.
State Management in React
State management plays a pivotal role in React applications. At its core, managing state refers to the way an application keeps track of, modifies, and reacts to changes in data. When applications grow in complexity, effective state management becomes crucial; it ensures that user interfaces respond seamlessly to user input and that data flows predictably throughout the app. If youāre building anything more than a trivial user interface, diving deep into state management can save you a world of hassle down the line.
Prologue to Redux
Redux is a predictable state container for JavaScript applications. Itās often used with React to manage the global state of the application. What sets Redux apart is its emphasis on immutability and the unidirectional data flow.
To take a closer look, consider the following key points:
- Single Source of Truth: Redux stores the whole state of your application in a single, immutable state tree. This means that any component can access shared data, making it easier to manage.
- Actions and Reducers: It uses a concept of actions, which are payloads of information that send data from your application to the Redux store. Reducers, on the other hand, are pure functions that take the previous state and an action, returning the next state. This separation of concerns simplifies debugging and testing.
- Enhanced Debugging: Thanks to the strict structure of Redux, tracking changes and debugging become simpler. Developers can implement features like time-travel debugging, making it possible to rewind to earlier states of the application.
Integrating Redux with React
Integrating Redux with React has never been more straightforward, especially with the official library, React-Redux. This library provides bindings to work seamlessly with Redux, allowing React components to access the global state.
To get started, follow these steps:
- Install Necessary Packages: You can install Redux and React-Redux using npm or yarn:
- Create Redux Store: Youāll need to set up a store to hold your applicationās state. This can be done with the function:
- Provide Store to App: Wrap your outermost React component with the component from React-Redux. This way, any component nested inside it can access the Redux store.
By following these steps, you connect Redux state management to your React application efficiently.
Understanding the Redux Flow
Understanding the flow of Redux can profoundly influence how you architect your React applications. Hereās how it works in a nutshell:
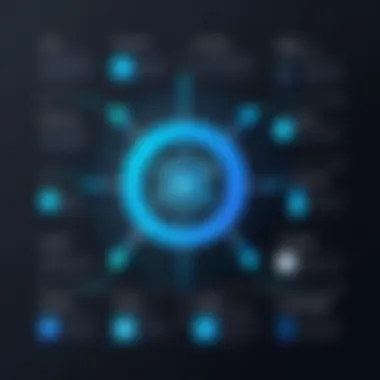
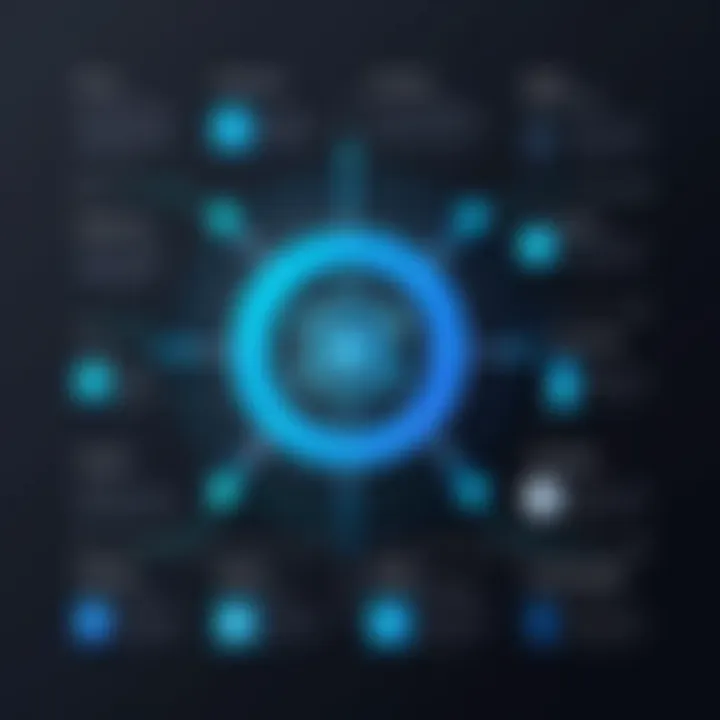
- Dispatching Actions: When a user interacts with the application, it often triggers an action. This action is dispatched to notify the store that something has occurred.
- State Changes in Reducers: The dispatched action is processed by a reducer, which updates the state accordingly. Reducers must not mutate the existing state, instead creating a new instance of the state.
- State Updates in Components: Once the state changes are made, the components subscribed to the Redux store automatically re-render, reflecting the new state in the UI.
This unidirectional flowāactions leading to state changes, which then lead to UI updatesāhelps keep your application predictable and manageable.
"In Redux, if you want to keep track of app state in a clear, consistent way, follow the data flow from actions, to reducers, to the UI."
React Router for Navigation
Navigating between different views or pages in a React application is a critical aspect of providing a smooth user experience. This is where React Router comes into play. It enables developers to implement dynamic routing in a single-page application seamlessly, allowing users to navigate effectively without full page reloads. Understanding how to set up and utilize React Router equips developers with the tools to create more responsive and engaging applications.
Setting Up React Router
Getting started with React Router is straightforward. First, you need to install the library. You can do this using npm by running the following command in your terminal:
Once it's installed, you can start incorporating it into your React app. The basic setup involves wrapping your application's component tree with a . This will keep your application's UI in sync with the URL. Hereās a quick example of how to set up React Router:
In this example, renders the first that matches the current location. By setting up your routes this way, you allow users to visit different parts of your application without reloading the entire page, ensuring a better user experience.
Routing Basics
Understanding the fundamentals of routing in React is essential for making a well-structured application. Hereās a breakdown of some core concepts:
- Route: Defines a mapping between a URL path and a specific component that should be rendered. For instance, a user visiting will see the component.
- Link: This component is used to create links that will navigate between different routes without causing a page refresh. Instead of using a traditional tag, use for internal navigation.
- Nested Routes: Sometimes, you may have routes that contain other routes. This allows for organized and modular applications. For example, you can have a main profile route and nested routes for settings or activity history.
This is how to create a link:
Navigating Programmatically
While controlling navigation through user interactions is common, there are scenarios where you need to navigate programmatically, such as after form submissions or API responses. React Router provides several methods to achieve this, primarily through the hook.
Hereās how you can do it:
- Import : This hook gives you access to the history instance used by React Router.
- Navigate Using : After an event, like submitting a form, you can push a new path to the history stack.
Example:
Navigating programmatically gives you more control over the user experience, allowing your application to remain dynamic.
In summary, React Router is a powerful tool that enhances navigation capabilities in React applications. It allows developers to efficiently manage views and ensure transitions between them are smooth, enhancing the overall user experience. Proper understanding and implementation of React Router is essential for creating effective single-page applications.
Testing React Applications
Testing applications is a cornerstone of ensuring that the code behaves as intended, especially in the fast-paced environment of web development. In the React ecosystem, testing is not just a supplementary task; itās an essential practice that safeguards against unexpected behaviors and bugs that can plague user interfaces. This section delves into the significance of testing within a React context, examining its advantages and considerations that developers must weigh when implementing this practice.
Preface to Testing in React
When developers build applications, they face numerous challenges. Bugs can emerge from even minor changes in code, causing unexpected results that can sour the user experience. Testing in React helps to identify and fix these issues early in the development process. Itās like having a safety net; it ensures that when you add a new feature or improvement, existing functionality remains intact.
Benefits of Testing
- Increased Confidence: Developers can push changes without fear of breaking existing features.
- Early Problem Detection: Catching bugs before they reach production reduces the cost and effort of fixing issues later.
- Documentation: Well-written tests serve as living documentation for your code, illustrating intended behavior.
Careful consideration must go into what and how to test. Unit tests, integration tests, and end-to-end tests each hold unique benefits. By understanding these facets, a developer can tailor their approach based on the application's requirements.
Using Jest for Testing
Jest is a popular testing framework that integrates seamlessly with React applications. Developed by Facebook, it comes with a rich set of features that facilitate effective testing, making it a go-to choice for many developers.
Key Features of Jest
- Zero Configuration: Most React projects using Create React App come pre-configured with Jest.
- Snapshots: Jest can take a snapshot of your components and alert you if they change unexpectedly.
- Mocking Functions: It allows you to mock functions and modules, isolating the components you want to test.
Utilizing Jest is straightforward. Hereās a quick example:
This snippet tests whether the renders a link with the text "learn react". It exemplifies how tests can be expressive and maintain a clear relationship with the actual code, ensuring every piece is working as expected.
Enzyme for Component Testing
While Jest offers extensive capabilities, Enzyme is another tool that deserves mention, especially when it comes to testing React components. Developed by Airbnb, Enzyme provides utilities that make it easier to assert, manipulate, and traverse your React component's output.
Comparative Advantages
- Shallow Rendering: Test components in isolation without worrying about their children, simplifying the test structure.
- Lifecycle Method Simulation: Enzyme allows invoking React lifecycle methods, which is paramount for testing component behaviors over time.
As with Jest, using Enzyme can simplify the testing of complex components. For example:
In this example, using shallow renders only the component without its descendants, which allows for straightforward assertions and decreases the risk of introducing defects from child components.
In summary, testing in React is not merely an option, but a necessity. By utilizing tools like Jest and Enzyme, developers can establish a testing suite that enhances the reliability of their applications, ensuring robust performance under diverse conditions. Proper testing practices enable quicker debugging processes and provide a safety net against future code modifications.
Performance Optimization in React
In the world of web development, performance can be the make-or-break factor. In the case of React, optimizing performance is essential for delivering a smooth and responsive user experience. A sluggish application can frustrate users, lead to high bounce rates, and ultimately cost businesses revenue. Therefore, knowing how to optimize performance in React applications is not merely beneficial; it is a crucial skill that developers must acquire.
Understanding performance optimization in React revolves around a few key elements:
- Efficiency in rendering.
- Minimizing re-renders.
- Enhancing load times.
With these elements in mind, developers can benefit from a smoother application operation and improved overall user satisfaction. Letās look closer at some specific techniques to achieve these goals.
Code Splitting
Code splitting is a clever method that allows developers to load only the necessary code for the current page, rather than the entire application upfront. This results in faster load times and allows users to access the parts of the application they need without delay.
Hereās how code splitting works in React:
- Dynamic Imports: Using the function within your React components allows for dynamic loading of components. This way, when a user navigates to a specific route, related code can be fetched at that moment rather than being included in the main bundle.
- React's Built-in Support: React includes and which help manage code splitting seamlessly. By using these features together, developers can easily load components only as they are needed.
Adopting code splitting will help keep your application lightweight and increase loading speed greatly, especially for larger applications.
Memoization Techniques
Memoization is a powerful optimization technique that ensures functions do not run unnecessarily. This is particularly useful in React, where re-rendering components can lead to performance drainage.
What memoization does is cache the result of expensive function calls and returns the cached result when the same inputs occur again. In React, the most common tools for memoization are:
- React.memo: This higher-order component allows React to skip rendering if the props have not changed. Hereās a quick example:
- useMemo Hook: This hook allows you to memoize expensive calculations. It returns a memoized value and only recomputes it when the dependencies change:
Employing memoization techniques can drastically reduce unnecessary rendering and improve the responsiveness of your React applications.
Lazy Loading Components
Lazy loading components in React is a way to delay the loading of components until they are actually needed. This effectively helps in reducing the initial load time of the applicationāonly loading parts of the UI when they are needed.
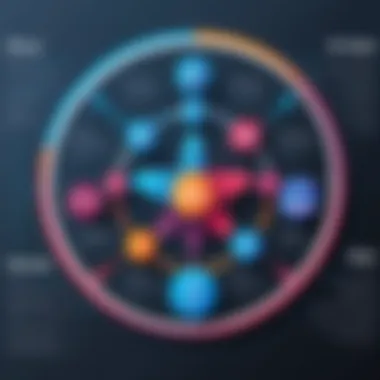
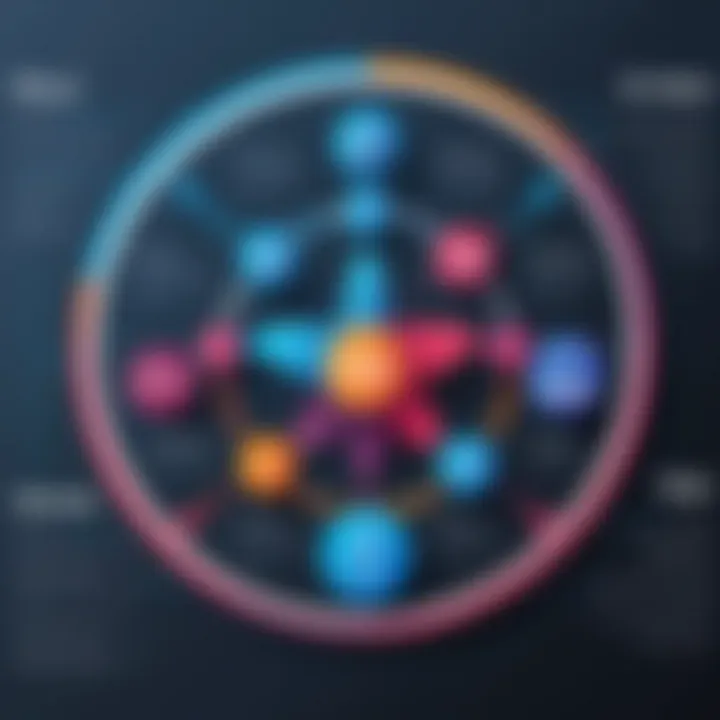
To implement lazy loading in a React application, you can use the following steps:
- Dynamic Import: Similar to code splitting, dynamic import allows you to import components asynchronously:
- Suspense for Fallback: To ensure that the application provides a fallback UI while the component loads, you can wrap the component in :
Lazy loading components ensures that users donāt have to wait long before interacting with your application. This method prioritizes the loading of essential content first while deferring the download of other components until they are required.
In summary, performance optimization in React is all about understanding and implementing strategies like code splitting, memoization, and lazy loading. These techniques will not only enhance the performance of your applications but also provide a better experience for your users, keeping them engaged and satisfied. By weaving these optimization strategies into your development workflow, you're setting yourself up for success in the fast-paced world of web development.
"The key to long-term performance is to optimize the process, not just the result."
Deployment Strategies for React Applications
In the landscape of modern web development, deploying applications efficiently is just as crucial as building them. A solid deployment strategy for React applications can make or break user experience, application performance, and even your project's success in meeting user demands. Think of it as a meticulous game plan; ensure all pieces are in their right place for the big reveal to users.
Preparing for Deployment
Before you even think about hitting that deploy button, itās wise to take a hard look at a few preparatory steps. The first thing to do is to finalize your applicationās build. Use the React script that brings together the entire project into an optimized bundle. This process involves minimizing files and making everything production-ready. It ain't rocket science, but it certainly helps to understand a few key concepts:
- Environment Variables ā Switch from development to production modes, which might affect API endpoints and feature flags.
- Static Assets ā Any media or assets should be included in the build to avoid 404 errors later.
- Testing ā Conduct thorough tests to ensure that each feature behaves as intended. Remember, better safe than sorry!
Having clarity on these elements before deployment can save headaches down the line.
Deploying on Various Platforms
React has built a reputation for flexibility. Various platforms can host your application, each with their own pros and cons. Letās break them down:
- Vercel ā A top choice for front-end developers. You get instant deployments and serverless functions. Perfect for applications that need to scale seamlessly.
- Netlify ā Another favorite. It's straightforward to set up. You can also utilize its continuous deployment system directly from Git.
- Heroku ā Best for those looking to deploy full-stack applications. It supports Node.js environments with a straightforward deployment process via Git.
While each platform serves a different purpose, itās best to pick one that aligns with your project's needs. Check out the documentation on each platform to tailor the deployment according to your specifications.
Continuous Integration and Deployment
Once youāve darted successfully into deployment, the next step is to implement a continuous integration and deployment (CI/CD) strategy. This is a game-changer if you're keen on maintaining quality and speed.
CI/CD automates the steps you previously took manually, thus reducing the chances of human error.
- Continuous Integration (CI) involves automatically testing and merging code changes. Think of it as a safety net that ensures every change is reviewed and validated before being deployed.
- Continuous Deployment (CD) goes hand in hand with CI. It takes the code and deploys it to production automatically after passing through tests. Thereās no manual intervention, making it faster and more efficient.
A typical pipeline could look like this:
- Code Submission - Developer pushes code to the repository.
- Build Triggers - Automated systems run tests on the new code.
- Testing Phase - Code undergoes extensive testing, including unit and integration tests.
- Deployment - Successful builds are deployed to production without manual handling.
Setting up CI/CD may require some initial effort, but once itās in place, it becomes a powerful ally in managing projects effectively.
By weaving together these strategies for deployment, nodding to best practices, and continuously improving, you set yourself up for future success in React projects. Remember, effective deployment isn't just a one-time event; itās an ongoing journey that requires attention to detail and adaptability!
Best Practices in React Development
When delving into React development, itās crucial to grasp best practices that streamline not only your workflow but also the maintainability and performance of your applications. Effective strategies can lead to smoother collaboration, fewer bugs, and a much cleaner codebase. In an ecosystem where large-scale applications are common, adopting these practices isn't merely advisable; it's essential.
Writing Clean and Maintainable Code
Maintaining a codebase in React can sometimes feel like a juggling act. To avoid chaos, striving for clean and maintainable code should sit at the forefront of your development process. Start by organizing your code effectivelyāgroup related components and separate concerns logically. Functions should do one thing, and do it well. For instance, a component designed for fetching data should not handle rendering. Keeping components focused not only aids in debugging but also improves the readability of your code.
Alongside organization, consistent naming conventions are key. You don't want othersāor future youāto scramble trying to discern what a function does based purely on its name. Therefore, descriptive naming fosters understanding. Adopting tools like ESLint can help you enforce these style guidelines and catch any slip-ups along the way.
"Good code is its own best documentation."
ā Anne Hunstiger
Component Reusability
In React, the notion of component reusability saves time and effort while enhancing your overall architecture. When components are designed to be reusable, they not only reduce redundancy but also create a more cohesive system. Think of components like building blocks. Each unique block should be designed with an understanding that it may be used multiple times across the application.
To achieve this, design components to accept props wisely. This ensures flexibility without compromising the integrity of the component. A button component, for instance, should cater to various styles and functionalities without needing to rewrite its internal logic. By fostering reusability, you cut down on future development time and make your project easier to maintain.
Start by identifying common patterns within your UI. If you find yourself repeatedly writing similar components, consider refactoring them into reusable versions. Not only does this approach make your code cleaner, but it also simplifies updates since changes can be made in one central location.
Documentation and Comments
While writing code thatās self-explanatory is a noble goal, reality often begs the need for good documentation and comments. Clear documentation can serve as a roadmap for other developersāor even yourself, when you revisit the project months down the line. Initial investment in documenting how components function and interact can pay off significantly in reducing onboarding time for new team members.
Effective comments go a long way as well. Comments should clarify why certain actions are taken rather than what the code does; the latter should ideally be deduced from the code itself. For instance, instead of commenting "This checks if the user is authenticated," a comment like "Redirect users who are not authenticated to the login page" provides better context.
In summary, implementing these best practices in your React development can significantly enhance your efficiency and ensure a robust, maintainable codebase. As you move forward, always remember that clean code, reusability, and clear documentation are not just advanced practices; they're fundamental aspects that will define your success in the realm of React.
Common Challenges and Solutions
When embarking on the journey of learning React, students often find themselves hitting a few roadblocks. Understanding these common challenges is crucial because it helps tailor learning strategies and solidifies a more profound comprehension of how to effectively use React. Addressing potential pitfalls not only aids in improving coding skills but also builds resilience and resourcefulness. Letās dive into some of these typical challenges, along with solutions that can serve to demystify the more complex aspects of React.
Debugging React Applications
Debugging is like hunting for buried treasure; it's often laborious but immensely rewarding once you find what you're looking for. In React, errors can manifest in various shapes and sizes. For instance, you might encounter āInvalid Hook Callā errors, where components struggle to adhere to the rules of hooks. These errors are akin to a puzzle and often require a keen eye to dissect the components to identify the offending code.
Here are practical tips for debugging:
- Use React Developer Tools: This browser extension is invaluable. It helps you inspect your React tree and trace component hierarchies.
- Console Logging: Sometimes, the simplest solution is the most effective. By using , you can monitor state changes and pinpoint where your application might be going awry.
- Error Boundaries: Implementing error boundaries can prevent the entire application from crashing due to one componentās failure, allowing you to catch errors at a certain point.
Handling Performance Issues
Performance issues in React can feel like trying to drive a car with the brakes onāfrustrating and counterproductive. As your application grows, it can become sluggish, which can deter users and affect overall experience. Recognizing common performance bottlenecks is key.
- Avoid Unnecessary Re-renders: Know when components need to update and use or to avoid redundant rendering.
- Optimize Context Usage: When working with context for state management, be cautious as it can cause excessive re-renders if not managed well. Only change the value of context when absolutely necessary.
- Use the and : These features allow you to split code effectively so users only download the parts they need when they need them, improving load time.
Managing State Complexity
State management can feel like a tangled ball of yarn; as it grows more complex, it risks becoming a disaster. Knowing how to manage state efficiently is paramount when building scalable applications.
- Lift State Up: When multiple components need access to the same state, lifting the state to their common ancestor is beneficial. This practice keeps the data flow tidy and predictable.
- Use Custom Hooks: Custom hooks can encapsulate related logic and state, allowing you to reuse functionality across components. This keeps your code DRY and easier to manage.
- State Management Libraries: For larger applications, consider using libraries like Redux or Zustand which provide a more structured way to manage global state, although they come with their own learning curve.
Debugging effectively, optimizing performance, and managing state complexity can make or break your React experience. Each of these challenges not only tests your problem-solving skills but also enhances your proficiency as a developer.
By anticipating these hurdles and knowing how to address them, you can refine your journey into React development, transforming challenges into stepping stones for growth. Don't forget, learning to navigate these problems isn't a sign of weakness but a hallmark of a thoughtful developer.
Ending and Further Learning Resources
The Conclusion and Further Learning Resources section serves as the culmination of your deep dive into React. It offers a reflective pause, summing up the arduous journey through the complexities of learning this robust JavaScript library. This part is crucial because it doesnāt just present a summary; it nudges learners toward continuing their education, ensuring they keep their skills sharp in a rapidly evolving tech landscape.
In todayās fast-paced digital world, keeping up with the latest tools and methodologies in web development is not merely beneficial but essential. Obviously, React is a significant player in this game. But to truly master it, one must go beyond the basics and seek out additional resources. This section aims to guide readers in finding valuable materials and communities that can further enhance their understanding and application of React.
Summarizing Key Takeaways
Here are the most relevant points captured throughout our exploration:
- Understanding Components: Reactās core feature is its component-based architecture, enabling modular and reusable code.
- State Management: Successfully managing state is fundamental to building dynamic applications, making tools like Redux essential for complex projects.
- Learning Curve: While React has a relative learning curve, consistent practice and proper resource allocation can ease the process.
- Testing and Optimization: Rigorous testing and performance optimization ensure applications run smoothly and efficiently.
- Community and Resources: Active participation in community forums facilitates continuous learning and improvement.
"A problem shared is a problem halved." Active engagement in communities can lead to faster problem-solving and exposure to diverse ideas.
Recommended Books and Courses
For those seeking to deepen their React knowledge, consider these well-regarded resources:
- Books:
- Online Courses:
- Learning React by Alex Banks and Eve Porcello ā A practical guide perfect for beginners.
- React Up & Running by Stoyan Stefanov ā This book provides a solid foundation and real-world examples.
- Fullstack React by Accomazzo, Murray, and Lerner ā A comprehensive guide that covers the full ecosystem around React.
- Udacityās React Nanodegree ā An excellent course that takes a project-based approach, ensuring practical experience.
- Courseraās Frontend Web Development with React ā This course offers a solid curriculum tailored for those starting their React journey.
Community Resources and Forums
Thriving in the React environment necessitates interaction with the developer community. Here are valuable platforms for engagement:
- Reddit: Subreddits like r/reactjs offer a space for sharing projects, troubleshooting, and learning from othersā experiences.
- Stack Overflow: A go-to site for developers facing specific coding issues, ensuring access to a wealth of knowledge.
- Facebook Groups: Various groups devoted to React development can provide tips, projects ideas, and collaboration opportunities.
- GitHub: Explore repositories to see how others are implementing React, which can inspire your future projects.
By continually engaging with these resources and communities, you position yourself to not only learn but excel in the realm of React, navigating its currents with confidence as you build robust applications.