Mastering JavaScript Promises for Asynchronous Programming
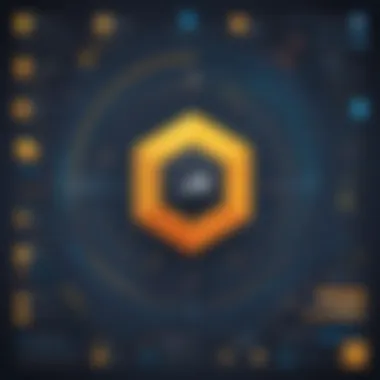
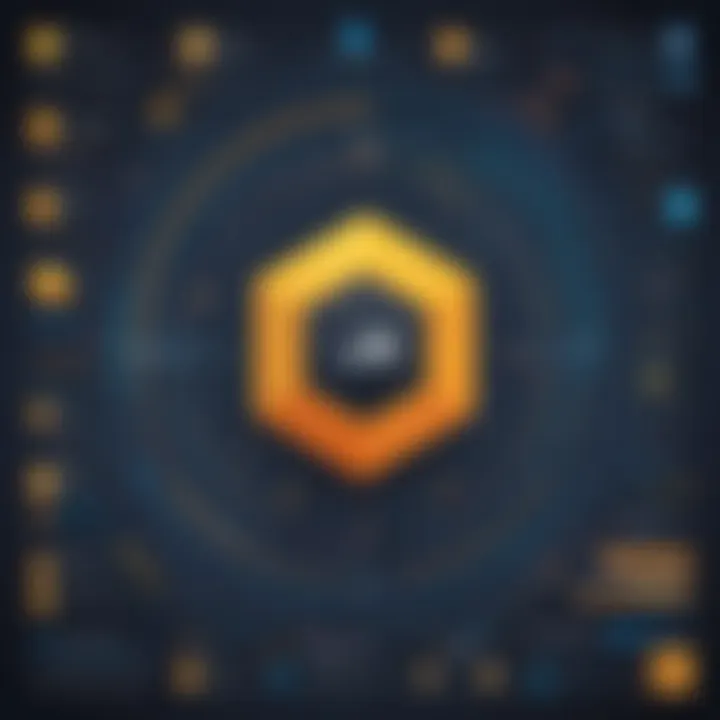
Intro
JavaScript has transformed the way developers construct applications, especially with the rise of asynchronous programming. Asynchronous code is essential when building modern web applications due to the necessity of handling multiple actions without blocking the execution of code. In this context, promises emerged as a powerful mechanism to manage asynchronous operations.
Promises can be seen as placeholders for future values that might eventually be available. The crux of their utility lies in their ability to simplify error handling and enhance the readability of code, which can often become tangled in callback hell.
In this guide, we will explore the anatomy of promises in JavaScript including their structure, functionality, and real-world applications. Furthermore, we will dive deep into error handling, pinpointing common pitfalls to avoid when working with promises. Our goal is to ensure that both budding developers and more experienced coders grasp a nuanced understanding of these powerful programming constructs.
"The promise is not just a code construct; it's a new way to think about writing asynchronous JavaScript!"
Key Points Addressed in This Guide
- What is a Promise?
- Advantages of Using Promises
- Error Handling in Promises
- Practical Examples
- Understanding the basic concept
- Lifecycle of a promise
- Improving code readability
- Managing asynchronous operations
- Common mistakes and best practices
- Using effectively
- Real-world scenarios and simple implementations
This article will serve as a foundational stone for those delving into modern JavaScript, facilitating smoother development journeys in the realm of asynchronous programming.
Intro to Asynchronous Programming in JavaScript
In the landscape of modern web development, asynchronous programming has emerged as a pivotal concept, redefining how developers approach tasks that, on the surface, seem simple. Whether it's fetching data from a server or managing complex user interactions, the need for an effective, non-blocking approach is undeniable. At first glance, one might wonder why introducing asynchronous methods even matters. Well, it boils down to enhancing user experience, improving performance, and making our code cleaner.
The Need for Asynchronous Models
Asynchronous models help us address tasks that might take a bit of time to complete, like loading images or making HTTP requests. Imagine encountering a website that freezes because it’s waiting for an image to load—that’s the bane of synchronous execution. Asynchronous programming allows the code to proceed without waiting for these time-consuming operations to finish.
For example, think about a dining experience at a restaurant. When you order food, the waiter doesn’t just stand there and wait for your meal to be cooked; they’ll take other orders, refill drinks, and attend to other customers. This parallel processing translates well into how JavaScript handles tasks. It can manage multiple operations simultaneously, which means better load times and an overall smoother user interaction.
Synchronous vs Asynchronous Execution
The crux of the issue often lies in distinguishing between synchronous and asynchronous execution. Synchronous methods follow a linear flow where each operation must complete before the next begins. Using the aforementioned restaurant analogy, consider synchronous execution similar to a single waiter handling one table at a time. While they’re taking your order, nobody else is being attended to. In contrast, asynchronous execution allows that waiter to juggle multiple tables, enhancing efficiency.
To illustrate:
- Synchronous:Here, waits for to complete before proceeding.
- Asynchronous:In this case, can continue processing while waiting on to finish.
In essence, understanding the difference between these two execution methods is crucial. The asynchronous approach powers dynamic web applications and serves as the foundation for utilizing promises effectively. By adopting asynchronous programming, developers can significantly elevate performance and user satisfaction, particularly in today’s fast-paced digital world.
What Are Promises?
When we talk about promises in JavaScript, we're dealing with a vital piece of the asynchronous programming puzzle. In a nutshell, a promise is like a placeholder for a value that will be available in the future. It simplifies the process of working with asynchronous operations. This is crucial in JavaScript, where operations like network requests can take an undefined amount of time. By managing these operations effectively, promises help us write clearer, more maintainable code.
Defining a Promise
A promise can be thought of as an object that may eventually produce a single value. This value can either represent the successful completion of an asynchronous operation or an error encountered during that process. When creating a promise, you typically define its behavior through an executor function. This function takes two parameters: and . If the asynchronous task is successful, you call with the result. If there's an error, is called with an error object.
Here’s a concise example:
States of a Promise
Promises have three distinct states that manage how they react to changes in their environment.
Pending
When a promise is in the Pending state, it means that the operation is still ongoing and hasn’t been completed yet. This state is crucial because it signifies that the operation hasn’t resolved or rejected. One of the key characteristics of Pending is its flexibility; it can transition to either Fulfilled or Rejected depending on the outcome of the asynchronous operation.
Being in this state is beneficial, as it keeps the promise open for resolution, allowing additional processes to occur as they await completion of the promise. The unique feature here is that it gives room for handling tasks without blocking the rest of the code from executing—an essential aspect of JavaScript's non-blocking nature.
Fulfilled
Once a promise succeeds, it enters the Fulfilled state. This signifies that the operation has completed successfully, and thus results are available for use. The key characteristic of a fulfilled promise is its inherent trustworthiness; once a promise is fulfilled, it cannot be reverted back to any other state. Hence, it's a sign that the desired outcome has been attained.
The unique feature of fulfillment speaks volumes regarding positive resolutions in programming. For instance, once a promise is fulfilled, you can proceed seamlessly to the next steps of your application without worrying about the previous operation's outcome, making this state a desirable goal in any asynchronous operation.
Rejected
On the flip side, when an operation fails, the promise enters the Rejected state. This indicates that something went wrong, and the promise provides error details to facilitate troubleshooting. A crucial aspect of rejection is how it communicates failure; the error callback can be utilized to catch and handle these failures gracefully in the flow of your application.
Just as with fulfillment, a rejected promise is definitive—it cannot transition back to pending or fulfilled. The advantages here are significant as it allows for robust error-handling mechanisms. Understanding how to deal with rejected promises can prevent your application from facing unforeseen crashes, ensuring a smoother user experience overall.
"Promises are a cornerstone of how JavaScript handles asynchronous operations, serving as an elegant solution to manage complexity and enhance code clarity."
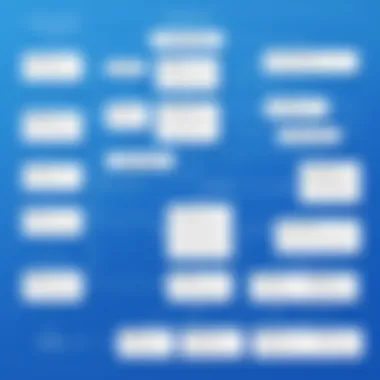
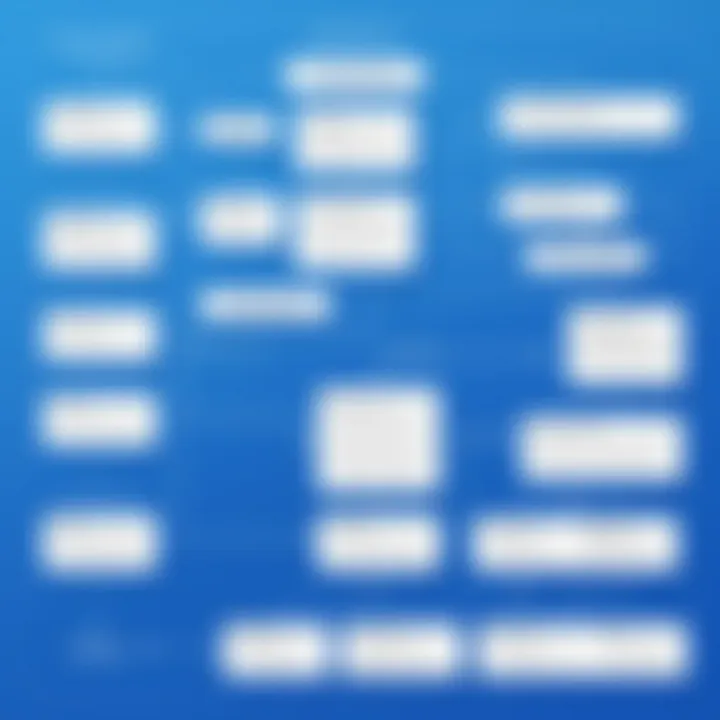
By understanding these foundational concepts of promises, developers can navigate the complexities of JavaScript's asynchronous nature, set realistic expectations for operation outcomes, and leverage these tools effectively in their programming practices.
Creating Promises
Creating promises is a foundational aspect of managing asynchronous operations in JavaScript. Understanding how to create promises not only empowers developers to handle tasks more efficiently but also forms the backbone of modern JavaScript programming. When you create a promise, you're essentially setting up a mechanism for handling eventual completion (or failure) of a task, which is crucial in scenarios involving web requests, file operations, or any activity that may take time to resolve. The benefits are clear: it allows for cleaner, more readable code and smoother error handling.
There are specific elements to consider when creating promises. First, the promise constructor itself takes a function as an argument. This function receives two parameters: and . Essentially these are callbacks that will indicate whether the operation completed successfully or failed, allowing you to define the desired behavior based on the outcome.
One of the notable considerations is ensuring that you manage state changes effectively. Failing to use the and callbacks correctly can lead to unexpected behavior in your application, often resulting in silent failures or complex debugging scenarios.
Using the Promise Constructor
To create a promise, you'll typically use the Promise constructor. Here's the basic syntax followed by an explanation:
This code initializes a new promise that runs the function provided. While performing the asynchronous operation, you will either call if the operation is successful or if it fails.
For clarity, consider this example:
In this case, after a two-second delay, the promise resolves with a message indicating data was fetched, simulating an API call that takes time. This kind of abstraction is helpful in creating non-blocking operations, allowing other code to execute while waiting for the promise to settle.
Immediate Resolution and Rejection
Often, you need scenarios where a promise resolves or rejects immediately. This can be done simply by calling or right away. Imagine you're validating some input; you might want to return a rejected promise if the data doesn't meet certain criteria:
Here, as soon as you call , it will either resolve with a success message or reject with an error message based on the input. This way, you can handle each outcome cleanly right after the promise resolves or rejects.
Remember: Crafting promises with clarity leads to better code maintainability and reduces the chances of running into bugs later on.
In essence, understanding how to create promises is vital for anyone working in JavaScript. It paves the way for robust error handling and keeps your codebase clean and understandable.
Working with Promises
Working with promises is a cornerstone of effective asynchronous programming in JavaScript. Promises offer a structured way to handle operations that might complete in the future—like fetching data from an API or reading a file. Understanding how to use promises properly can save developers from numerous headaches, like dealing with intricate callback structures. The rise of promises has simplified the management of asynchronous code and made it accessible to developers, easing transitions for many who might have wrestled with earlier methodologies.
The then() Method
The method is where promise magic often begins. This method allows you to define what should happen when a promise is either fulfilled or rejected, hence opening a door to a clearer flow of logic. To put it simply, if a promise resolves, the function inside kicks into gear.
For example:
In this snippet, returns a promise. The first processes the response into JSON format, and the next does something with that data. Notably, the promise chain continues on success but will gracefully pass to the method if anything goes awry. This brings both clarity and organization.
Chaining Promises
Promise chaining takes the use of to another level, allowing for a clean handling of sequential operations. When one promise resolves, it can pass its results to the next linked promise. Think of it as a relay race—once one runner completes their lap, they hand off the baton to the next.
Consider this example:
Here, resolves and yields a user object. That object is then used in the next promise to grab user posts, showing how flexibly promises can adapt to complex workflows, maintaining readable code along the way.
Handling Errors with catch()
Error handling is paramount in any programming landscape, and promises equip developers with the method to tackle errors cleanly. Instead of scattering error handling code across various callbacks, the catch method centralizes error management to a specific point.
In instances like network failures or unsuccessful operations, the organized structure of promises becomes evident. For instance:
This layout ensures if any part of the promise chain fails, control jumps to the block, preventing potential confusion and allowing for more robust logging and debugging solutions.
Key takeaway: Effective use of promises transforms chaotic callback-based code into a logical flow that is easier to read and maintain.
Adopting these promise methods not only streamlines your asynchronous code but enhances its reliability. JavaScript promises establish an engaging framework for developers to build upon, ultimately leading to smoother and more efficient coding experiences.
Advanced Promise Techniques
In the ever-evolving landscape of JavaScript, mastering advanced promise techniques is pivotal for crafting efficient asynchronous code. These techniques not only streamline handling multiple promises but also improve performance and reduce the complexity of your code. Understanding advanced promise strategies like , , and can significantly enhance your ability to manage operations that need to run concurrently or require the completion of multiple tasks.
Promise.all() Method
When you have multiple asynchronous operations that need to be executed, can be a real lifesaver. It allows you to run multiple promises in parallel and returns a single promise that resolves when all the promises in the iterable have resolved. If any of the promises in the iterable reject, the entire promise is rejected with the reason of the first promise that rejected.
This method is quite useful in scenarios where you need the results of several asynchronous tasks before proceeding. For instance, imagine fetching user data and their related posts from a server:
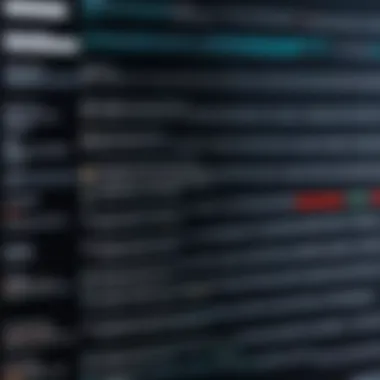
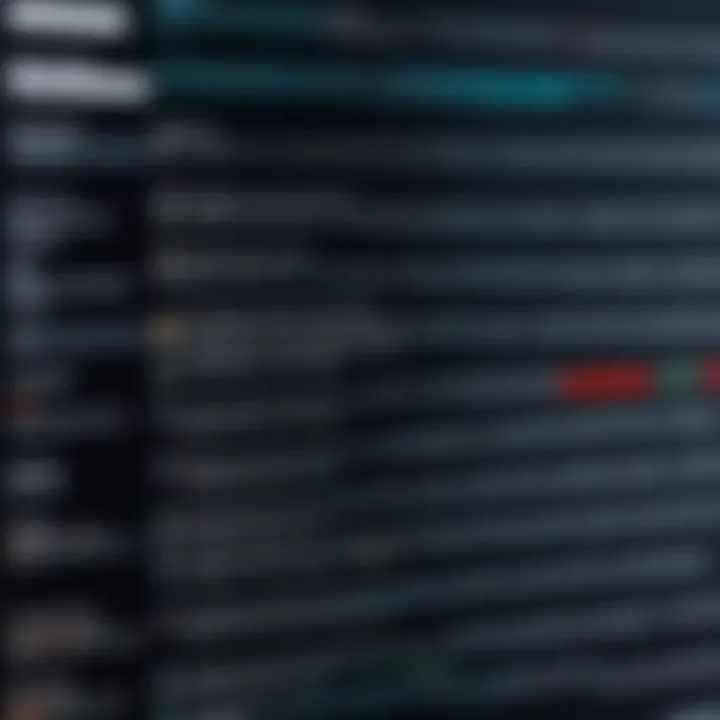
In this example, both and are called simultaneously. Only when both operations are complete does the callback get executed. This offers a clean and powerful way to manage multiple asynchronous calls.
Promise.race() Method
If you need to act based on the quickest promise resolution, can come in handy. Unlike , which resolves when all promises are resolved, resolves as soon as the first promise in the iterable resolves or rejects.
This method is particularly useful in environments where timing is crucial, such as an API call that may take longer than expected:
Here, if resolves before the timeout promise, the block executes. If not, the promise will reject, signaling either a failure in fetching the data or hitting the timeout. It helps keep your application responsive and prevents long wait times for users.
Promise.allSettled() Explained
provides another layer of flexibility when dealing with multiple promises. Unlike , which stops executing and returns the error of the first promise that fails, waits for all promises to settle (either resolved or rejected) and returns an array of objects describing the outcome of each promise.
This technique is beneficial when you need the result of each operation regardless of whether they succeed or fail:
In this code sample, you'll see how using allows handling success and failure cases separately. Whether or resolves or rejects, you'll still get valuable information about both operations, making it much easier to react accordingly.
The power of these advanced promise techniques can't be underestimated. Not only do they enhance code clarity, but they also increase error resilience and overall application performance.
By mastering these patterns, you can make your asynchronous JavaScript code much more effective and readable. With the right understanding, you can tackle complex scenarios with confidence.
Common Use Cases for Promises
Within the realm of JavaScript, promises serve as a cornerstone for efficient asynchronous programming. Understanding real-world applications can empower developers not just to write cleaner code but also to tackle complexities in data handling. Essentially, utilizing promises can make code more manageable, improve maintainability, and ultimately enhance performance in interactive applications. Now, let's delve into three common scenarios where promises shine: making HTTP requests, handling file operations, and managing timers and delays.
Making HTTP Requests
When it comes to web development, making HTTP requests is perhaps one of the most prevalent use cases for promises. Whether you're fetching data from an API or sending information to a server, the asynchronous nature of promises fits perfectly. The previous callback structure often led to nested callbacks, commonly referred to as "callback hell". Promises help to flatten this structure, allowing developers to chain requests cleanly.
For instance, fetching user data from a JSON API can be accomplished elegantly using a promise:
In the example above, the function returns a promise. Upon resolution, the response can be turned into JSON, making it easy to work with. If any step fails, the method ensures that errors are handled gracefully.
Handling File Operations
File operations, especially in a Node.js environment, are another area where promises show their might. When you read or write files, handling these operations synchronously can block your entire application. Instead, promises enable you to perform these actions without freezing the system.
For instance, using the module in Node:
This example demonstrates how easy it is to manage file reading asynchronously with promises. By avoiding explicit callbacks, the code becomes cleaner and its intent clearer.
Managing Timers and Delays
Another nifty use of promises is managing timers or introducing delays in code execution. Especially in scenarios where you need to wait for a specific duration before executing subsequent code, promises can cleanly wrap the functionality. Instead of relying on the older structure with callbacks, wrapping it in a promise can prevent callback nesting.
Consider the following implementation:
In this example, the function returns a promise that resolves after the specified time in milliseconds. This way, you can integrate delays without convoluting your logic.
This clever approach allows clearer readability, making promise-based code easier to reason about.
Summary
Potential Pitfalls of Promises
When delving into the world of JavaScript promises, it isn't all smooth sailing. As much as promises can slickly handle asynchronous operations, they can also lead developers into murky waters. Understanding these potential pitfalls is crucial for maintaining clean, efficient, and manageable code. Let's navigate through some of the common missteps, as well as the implications they carry. The more aware we are of these challenges, the better we can design our code to avoid them.
Callback Hell and Promise Hell
Callback hell is a term that many programmers dread. Picture this: you start off writing a simple asynchronous function with a one-level deep callback. Everything looks good, right? But as your project grows, you find yourself nesting multiple callbacks within one another, leading to a tangled mess of code that's all but impossible to read or debug.
When you transition to promises, while they do alleviate some of the callback chaining issues, they’re not a silver bullet. You can still find yourself in what’s often referred to as "promise hell." This happens when promises are nested deeply, resulting in a long chain that’s just as difficult to manage.
Instead of handling raw callbacks, you might have something like this:
When things get complicated, each chained method can turn into another layer of nesting. To sidestep this, consider using , which allows for a more straightforward, linear style of coding, making it easier to read and maintain.
Unhandled Promise Rejections
One of the significant issues with promises is unhandled rejections. You fire up a promise, and if it fails and you forget to handle the rejection, your program might just carry on like nothing happened. This can lead to silent failures, where errors occur without any clear indication. Like driving with a cracked windshield—you might not see it right away, but it's only a matter of time before it causes a major issue.
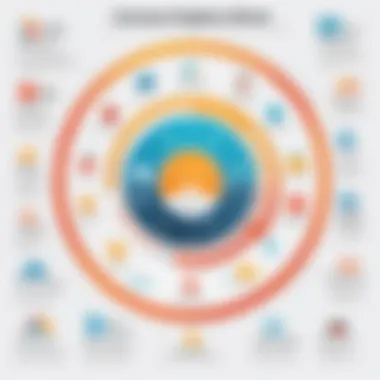
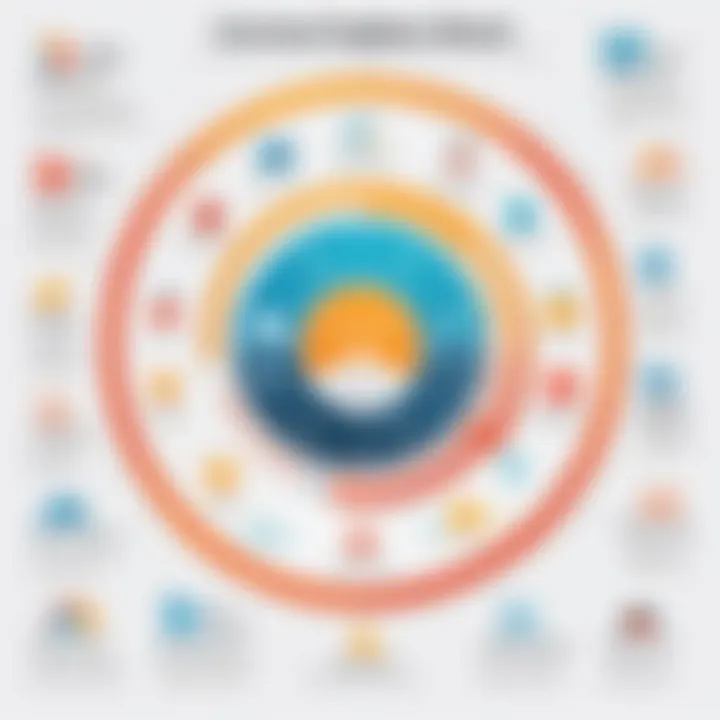
JavaScript provides the method to handle these rejections gracefully. However, when a rejection is not caught, it leads to warnings in the console. In a larger application, this could give you a headache trying to pinpoint where things went off course.
Here's an example demonstrating unhandled rejection:
In the above, if you run this code, you won’t see any error handling. Instead, you'll just get a vague warning message, making debugging infinitely harder.
To avoid unhandled promise rejections, always ensure that you include a or handle your promises in a try-catch block if you're using .
To sum up, while promises offer an elegant solution to asynchronous programming in JavaScript, they come with their unique set of challenges. By understanding callback hell and managing unhandled rejections, you arm yourself with the tools needed to code more effectively.
Coding Examples and Practical Applications
When delving into the world of JavaScript promises, practical examples can truly illuminate concepts that otherwise may seem dry or vague. Coding examples and real-world applications bridge the gap between theoretical understanding and tangible results. They help programmers grasp how promises behave in various contexts, thereby instilling confidence when tackling asynchronous tasks. In this section, we'll explore specific coding scenarios where promises shine, emphasizing their structure and benefits along with some common considerations to keep in mind.
Example of an API Call Using Promises
API calls often serve as a cornerstone in modern web applications, enabling developers to retrieve or send data to remote servers. Using promises simplifies this process significantly. Here’s a straightforward example of how you can make an API call using the Fetch API, which is promise-based.
In this snippet, the function initiates a request to the specified URL. Once the request is completed, it uses the method to process the response. Here, we check if the response is okay before converting it to JSON. If anything goes awry, the method captures and logs the error. This approach highlights the clarity afforded by promises in handling the sequence of operations—no more nested callbacks leading to confusion or callback hell.
Using Promises in a Real-World Application
Promises not only ease the process of API interactions but also integrate seamlessly into larger applications. Whether you're developing a simple website or a sophisticated web app, the utility of promises becomes evident.
Consider a scenario where an application relies on user input to fetch data. Our application may need to retrieve user details and their posts from two different endpoints. Using promises can make our code not just cleaner but also more efficient. Here’s how this might unfold:
In this example, we start by defining two functions to retrieve user details and their associated posts. The beauty of promises here is seen when we chain operations so that fetching posts only happens after successfully retrieving user details. This not only saves us from excessive nesting but also provides a clear, linear path through our asynchronous operations.
"In real-world applications, promises help maintain clean, manageable code that is easier to troubleshoot and scale."
By utilizing promises effectively, developers can tackle the complexities of asynchronous programming, ensuring smoother operations in applications that depend heavily on real-time data and interactions.
As you explore coding examples and practical applications, keep in mind that practice makes perfect. Tinkering with the code, seeing what breaks, and understanding why it works will deepen your grasp of promises in JavaScript.
Migrating from Callbacks to Promises
In the realm of JavaScript, callbacks have long served as a go-to method for handling asynchronous operations. However, as applications grew in complexity, so did the code structure with callbacks, often leading to what is infamously known as callback hell. Thus, migrating to promises represents not only a necessary evolution but a beneficial one for developers aiming for cleaner and more manageable code. In this section, we delve into the significance of this transition, the advantages it brings, and the crucial considerations developers need to ponder during the migration process.
Understanding the Transition
Transitioning from callbacks to promises can feel like leaving your comfort zone. Initially, callbacks seem straightforward enough. You submit a request, and when it’s complete, a function is called to handle the result. However, this model quickly becomes unwieldy when multiple asynchronous operations are involved.
Promises introduce a structured way to address these challenges. They simplify the handling of asynchronous actions by providing a cleaner alternative that can resolve or reject outcomes. A promise acts as a placeholder, representing a value that may be available in the future. It either fulfills successfully, rejects with an error, or remains pending until resolved.
Key Benefits of Migrating to Promises:
- Improved Readability: Promises allow for better organized and more readable code. Instead of nesting functions, you can chain and calls.
- Error Handling: Promises provide a unified way to handle errors, allowing you to avoid repetitive error checking and streamline debugging.
- Composition: You can easily compose multiple asynchronous actions together without the architectural problems associated with nested callbacks.
This transition not only enhances readability but also boosts maintainability, which is crucial for long-term success.
Refactoring Existing Code
Refactoring existing code to utilize promises instead of callbacks can seem daunting, yet it can ultimately lead to cleaner and more efficient codebases. Here are some considerations to take into account during this process:
- Identify Callback-Heavy Areas: Start by examining your codebase for sections that involve multiple nested callbacks or complex error handling. Target these areas for refactoring.
- Start Small: Begin with one function or feature at a time. This piecemeal approach minimizes risks and helps maintain functionality while changes are being made.
- Convert Callback Functions: Replace existing callback functions with promise-based alternatives. For example, you might change a function that uses a callback to return a new Promise, resolving or rejecting it inside the function:
- Testing: Ensure that every change is thoroughly tested. This is vital to confirm that the new promise-based code behaves as expected.
- Leverage Modern Tools: Utilize libraries or frameworks that inherently use promises, like Axios for HTTP requests. They often streamline the process, allowing for efficient integration and less configuration.
Ultimately, while this migration process may seem labor-intensive, it dramatically enhances your code quality and paves the way for future scalability. As you embrace promises, the enhancement of your development experience becomes palpable.
"Converting from callbacks to promises is not just a change in syntax; it's a transformation in mindset that can lead to more resilient and elegant code.”
By taking the time to refactor and understand the nuances of promises, you’re investing in a brighter coding future.
The End on JavaScript Promises
As we wrap up our exploration of promises in JavaScript, we must ponder their transformative role in modern programming. Promises anchor the world of asynchronous operations, offering not just a promise to return results, but a structured path to handle complexities in code with grace and clarity. This topic is not merely an accessory to enhancing JavaScript; it's a foundational pillar that empowers developers to write more maintainable and less error-prone applications.
The Future of Promises in JavaScript
In looking towards the horizon, one must consider how promises will evolve alongside JavaScript itself. With the rise of concurrent programming paradigms and frameworks, such as React and Vue, the utility of promises is increasingly entwined with state management and UI rendering. As more developers embrace functional programming styles, we can expect promises to integrate seamlessly with async/await syntax, enhancing readability and making error handling less cumbersome.
Moreover, the JavaScript community continuously iterates on patterns and libraries that could reshape how we think about promises. Libraries like Axios and Fetch are already streamlining HTTP requests with promise-based architectures. As new paradigms emerge, incorporating promises into the developer workflow will likely become even more intuitive.
"Promises are not just a way to handle asynchronous operations, but a bridge to a more manageable and cleaner codebase."
Final Thoughts and Best Practices
To truly harness the power of promises in JavaScript, one must adopt best practices that resonate with clarity and robustness. First, always handle errors with care. Using effectively not only prevents silent failures but also debugs code with greater efficiency.
It's also crucial to remember the importance of understanding when to use promises appropriately. Overuse can lead to what is known as promise hell, where callbacks and promises intertwine excessively, creating convoluted structures. Ensure that your asynchronous logic is clear and maintainable.
- Keep your promise chains flat: This practice facilitates readability, reducing the complexity of how promises are constructed.
- Be cautious with unhandled rejections: Make it a habit to attach error handlers, whether at the end of a chain or by using process-wide handlers to catch unhandled rejections.
- Prioritize readability: Code is read much more often than it’s written. Clarity should be your guiding principle.
In summary, JavaScript promises are not merely tools; they are essential instruments that offer a lifeline in dealing with the asynchronicity of our code. As developers, grasping their potential leads to superior coding practices, fosters collaboration, and enhances the overall experience of programming in JavaScript.