JavaScript Interview Practice: Essential Guide for Developers
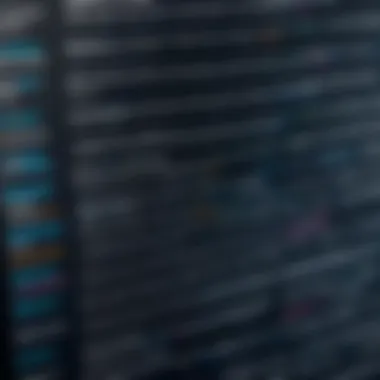
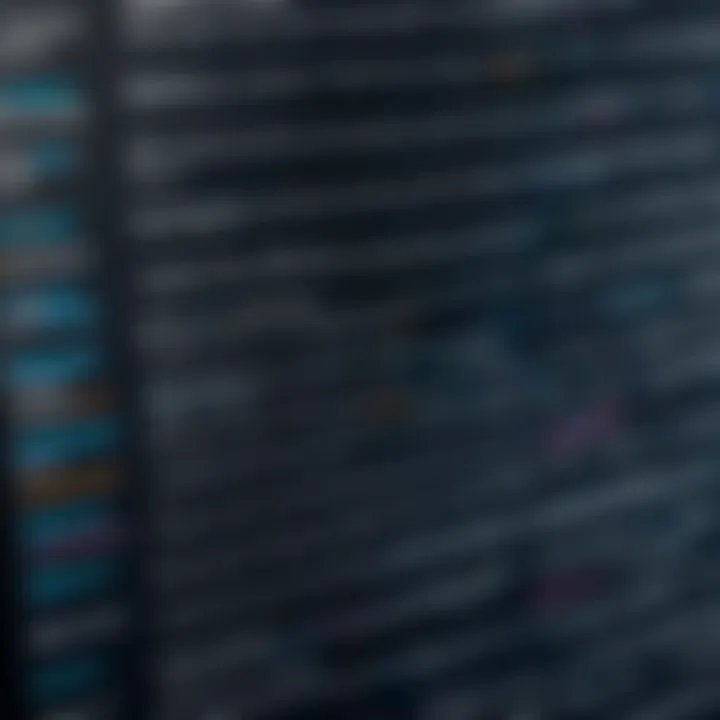
Prelude to Programming Language
JavaScript has evolved into one of the most significant programming languages in the world. It is widely used for both front-end and back-end development, powering everything from simple websites to complex web applications. This section will provide a historical context of JavaScript, discuss its defining attributes, and highlight its extensive scope in today’s tech landscape.
History and Background
Created in 1995 by Brendan Eich, JavaScript was initially designed to add interactivity to web pages. Growth in the internet market necessitated a language that could facilitate dynamic content. What began as a tool to enhance basic HTML has transformed into a robust, multi-paradigm language. Its introduction of features like closures and first-class functions has fundamentally changed how developers approach programming.
In 1997, JavaScript became standardized under the name ECMAScript, allowing for consistency across browsers. Though it faced initial skepticism, JavaScript’s capability grew along with its ecosystem, resulting in frameworks such as Angular, React, and Node.js gaining tremendous popularity.
Features and Uses
JavaScript boasts several features that contribute to its widespread use:
- High-Level Language: It abstracts the complexities of the computer's hardware.
- Dynamic Typing: Developers do not need to declare variable types explicitly, allowing for flexibility.
- Prototype-Based Object Orientation: This allows developers to build complex functionalities easily.
- Asynchronous Programming: JavaScript supports asynchronous programming, which helps in improving performance by running multiple tasks concurrently.
These features enable a wide range of applications, from creating interactive user interfaces to developing server-side applications.
Popularity and Scope
JavaScript consistently ranks as one of the top languages among developers. According to the Stack Overflow Developer Survey, a significant portion of developers use JavaScript regularly, indicating its prevalence in projects across the globe. The high demand for JavaScript developers reflects the thriving environment of web and application development.
The language's breadth of use extends to various sectors, including:
- Web Development: Core underpinnings of modern websites.
- Game Development: Interactive gaming experiences online.
- Mobile App Development: Using frameworks like React Native.
- API Development: Backend services integration.
This multifarious nature assures aspiring developers that mastering JavaScript can open a multitude of career paths.
"JavaScript is a versatile language that adapts to the ever-evolving demands of technology."
Prolusion to JavaScript Interview Preparation
In the realm of software development, JavaScript has become a cornerstone language. Preparing for a JavaScript interview requires more than just a cursory understanding of the language. This section introduces key aspects of preparing for interviews specific to JavaScript, showcasing their importance in equipping developers to excel in technical evaluations and job opportunities.
Understanding the Basics
Definition of JavaScript
JavaScript is a high-level, interpreted programming language primarily used to create interactive effects within web browsers. It is essential for developing dynamic web applications. Its syntax is relatively simple, allowing developers to build features swiftly. This accessibility contributes to its wide adoption. A unique feature of JavaScript is its event-driven nature, allowing operations to be executed in response to user actions, which enhances user engagement. However, developers must also be mindful of potential performance issues in larger applications, which can arise from improper coding practices.
Importance in Web Development
JavaScript plays a critical role in web development by enabling fluid, interactive user interfaces. It is often accompanied by frameworks such as React and Angular, which streamline efficient application development. The combination of JavaScript with HTML and CSS allows developers to produce rich web experiences. One key characteristic is its versatility; it can be used for both frontend and backend (via Node.js). However, the need for constant learning and adapting to new frameworks can feel overwhelming for some developers.
Role in Frontend and Backend
JavaScript's role extends from simple client-side scripting to server-side programming. On the frontend, it manipulates HTML and CSS to create dynamic pages. Conversely, on the backend, it runs on servers to handle requests, manage databases, and serve content. This duality makes JavaScript a highly sought-after skill. It connects user experience design with data management seamlessly. Challenges may arise when transitioning between its frontend and backend applications, as the developer must adjust their mindset to different programming paradigms.
Purpose of Interview Preparation
Familiarity with Common Questions
Being familiar with common JavaScript interview questions is vital for successful preparation. It enables candidates to anticipate inquiries and formulate confident responses. The ability to articulate concepts clearly can set candidates apart in competitive job markets. Practicing typical patterns not only reinforces knowledge but also helps with articulation under pressure. However, rote memorization without true understanding may hinder performance during technical evaluations.
Bridging Theory and Practice
Interview preparation serves to bridge the gap between theoretical knowledge and practical application. By reviewing both concepts and real-world coding scenarios, developers can gain a holistic understanding of how JavaScript operates in professional environments. Using coding challenges to solidify theory cultivates readiness for unexpected questions during interviews. The challenge lies in the balance, as too much focus on theory can lead to neglecting the practical skills required in the workplace.
Building Confidence
Confidence is an essential component of a successful interview. Practicing interview questions and coding challenges enhances a candidate's assurance in their skills and ability to problem solve. Facing interviews can be daunting, and adequate preparation empowers candidates to present their expertise effectively. The repetition of practice can reduce anxiety, ensuring that candidates approach interviews with a growth mindset. However, a risk exists when over-preparation creates a sense of rigidity, which may detract from a candidate's ability to adapt and think on their feet during real-time problem-solving.
Core JavaScript Concepts
Understanding core JavaScript concepts is foundational for any developer looking to excel in interviews and practical applications of the language. These core elements form the basis for understanding more complex functionalities and best practices in JavaScript development. They not only shape how one writes code but also affect performance, maintainability, and collaboration.
Data Types and Variables
Understanding Primitive Types
Primitive types in JavaScript, such as strings, numbers, booleans, null, undefined, and symbols, are essential to grasping the language's behavior. Each type defines how values are stored and manipulated. Key characteristics include their immutability and how they are compared in operations. A benefit of using primitives is their straightforward nature, allowing developers to easily debug and manage them. However, primitives can sometimes lead to unexpected behavior due to JavaScript's type coercion, which is essential to understand during interviews.
Variable Declarations
Variable declarations using , , and determine the scope and mutability of variables. Each declaration has its unique properties. For instance, let and const support block scope, which is beneficial for maintaining variable states in specific contexts. A common conundrum is deciding when to use versus . Utilizing communicates the intention that a variable should not be reassigned, enhancing code clarity. A disadvantage of is its function scope, which can lead to confusion and errors in larger codebases.
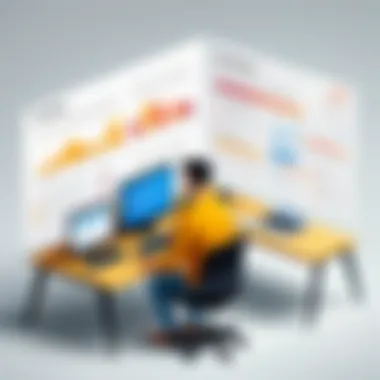
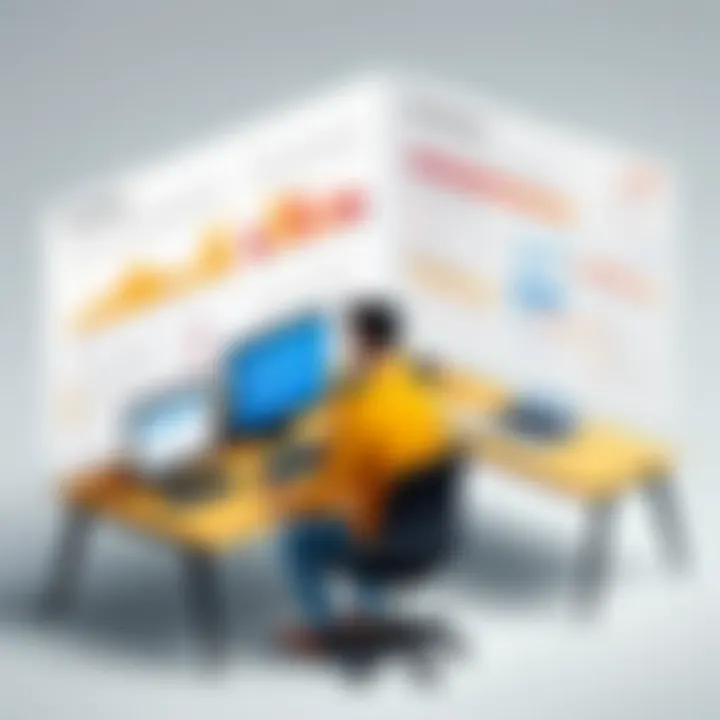
Scope and Hoisting
Scope defines where variables are accessible in the code. Hoisting is JavaScript's behavior of moving declarations to the top of their containing scope. Understanding scope helps in preventing unintended access to variables. Hoisting allows variables to be used before their declaration. This can be beneficial for organizing code but can also introduce errors if not properly managed. Developers must be aware of how hoisting impacts their code structure and variable access to avoid pitfalls during interviews.
Functions and Scope
Function Expressions vs Declarations
The distinction between function expressions and function declarations is crucial for understanding JavaScript execution flow. Function declarations are hoisted, meaning they can be called before their definition. This is a beneficial feature, as it allows functions to be organized flexibly in code. On the other hand, function expressions are not hoisted, which can lead to errors if one tries to call them prematurely. This uniqueness provides developers with control over their functions and influences how they structure their code.
Arrow Functions
Arrow functions present a concise syntax for writing function expressions. They differ from traditional functions mainly in their lexical scoping of , making them a popular choice for maintaining context in callback functions. A unique feature of arrow functions is that they cannot be used as constructors and do not have their own . While they simplify syntax, it’s key to understand when not to use them, particularly in scenarios where a traditional function’s features are required.
Call, Apply, and Bind
, , and are methods that allow developers to control the context () of a function. Call and apply are used to invoke a function immediately with specified arguments, while creates a new function that, when called, has its set to a specific value. This is beneficial for maintaining context in object-oriented programming. Understanding how and when to use these methods can massively improve the flexibility and reliability of code, especially in event handling.
Objects and Arrays
Object Creation and Manipulation
Objects are collections of key-value pairs and are a pillar in JavaScript’s structure. Understanding how to create and manipulate objects allows developers to model real-world entities. A beneficial feature of objects is their ability to contain methods, making them excellent for encapsulating behavior alongside data. The limitations arise when managing complex or deeply nested objects, which can lead to performance issues or errors.
Array Methods and Iteration
JavaScript arrays come equipped with various methods for manipulating data. Familiarity with built-in methods such as , , and enhances coding efficiency. Being able to iterate over arrays effectively is a core skill for developers. An advantage of these methods is their readability and reduction of boilerplate code, but understanding the performance implications of different methods remains important for real-world applications.
Destructuring and Spread Operator
Destructuring provides a succinct way to unpack values from arrays or objects into distinct variables, enhancing code clarity and reducing redundancy. The spread operator allows for an easy way to merge arrays or copy objects. While these features promote clean code, they can introduce complexity for those unfamiliar. Striking a balance between readability and sophisticated data handling is essential when implementing these techniques.
Asynchronous JavaScript
Understanding Callbacks
Callbacks are functions passed as arguments to other functions, facilitating asynchronous programming. They are fundamental for executing code after asynchronous tasks, such as API calls. Key characteristics include their flexibility in handling responses and maintaining control over execution flow. However, overusing callbacks can lead to what is known as "callback hell," which makes code unwieldy and difficult to understand. Balancing their use with promises or async/await can mitigate this issue.
Promises and Async/Await
Promises represent the eventual completion or failure of an asynchronous operation and its resulting value. The async/await syntax simplifies working with promises by allowing developers to write asynchronous code in a synchronous manner. This feature significantly improves code readability and organization. Understanding how to implement promises effectively is crucial. However, one must also be cautious about proper error handling, as unhandled promise rejections can lead to hard-to-trace bugs.
Error Handling in Asynchronous Code
Error handling is a vital aspect of writing robust asynchronous JavaScript. Implementing try/catch with async/await and using with promises protects the application from unexpected errors. Understanding how to effectively handle these errors is a beneficial skill that ensures resilience in applications. Failing to manage errors properly can lead to a poor user experience and complicate debugging efforts. This knowledge is critical when preparing for technical interviews that focus on practical coding scenarios.
Common JavaScript Interview Questions
Common JavaScript interview questions play a crucial role in the hiring process for developers. They serve as the foundation upon which technical conversations are built during interviews. Understanding these questions can help candidates demonstrate their knowledge and problem-solving abilities.
Candidates who prepare for these questions not only familiarize themselves with essential concepts but also enhance their ability to think critically on their feet. These common questions often address basic and intermediate concepts and provide a clear insight into a candidate's grasp of JavaScript fundamentals, making it easier for interviewers to assess competence efficiently.
Basic Level Questions
Variable Declaration Differences
The variable declaration differences in JavaScript primarily involve , , and . Each of these keywords has distinct behaviors and scopes. Understanding this contributes significantly to code quality and bug prevention.
declares a variable that can be re-assigned, but it has function scope or global scope, depending on where it is declared. In contrast, and are block-scoped. allows for re-assignment, while creates a constant reference. These distinctions help prevent accidental overwrites and scoping errors, which is beneficial for debugging and maintaining code.
By knowing when and which declaration to use, developers can write cleaner and more predictable code. This knowledge aids in avoiding common pitfalls in JavaScript programming and is thus a focal point of many interviews.
Array vs Object
When discussing arrays and objects in JavaScript, the fundamental difference lies in their structure and intended use. Arrays are specialized for ordered collections, while objects are ideal for key-value pair storage. This distinction is significant because it dictates how data should be stored and accessed.
Arrays allow direct access to elements via their indices, making them suitable for lists or sequences. Objects, however, organize data in a way that is more readable and easier to manipulate when dealing with named properties. Observing these properties helps candidates to articulate their design choices during interviews.
Understanding when to choose an array over an object and vice versa can greatly enhance code efficiency. This knowledge is essential, as many interviewers may pose practical scenarios that involve both structures.
Understanding 'this'
Understanding the keyword in JavaScript is one of the more challenging concepts for many developers. It does not refer to the variable that contains it; rather, its value is determined by the context in which it is used. This flexibility can be beneficial for creating dynamic functions, but it also complicates the understanding of code flow.
In global context, refers to the global object. In a function, refers to the caller of the function. In the context of an object, it refers to the object the method is called on. Understanding this behavior is essential for avoiding common mistakes, particularly with event handlers and asynchronous functions. This knowledge is critical in interviews, as many programming problems hinge on correctly managing .
Intermediate Level Questions
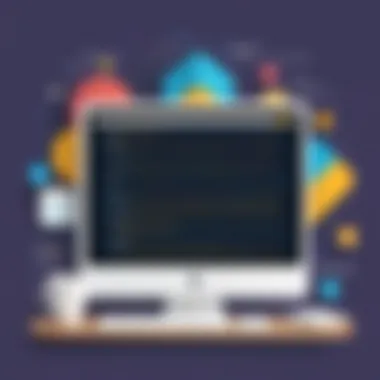
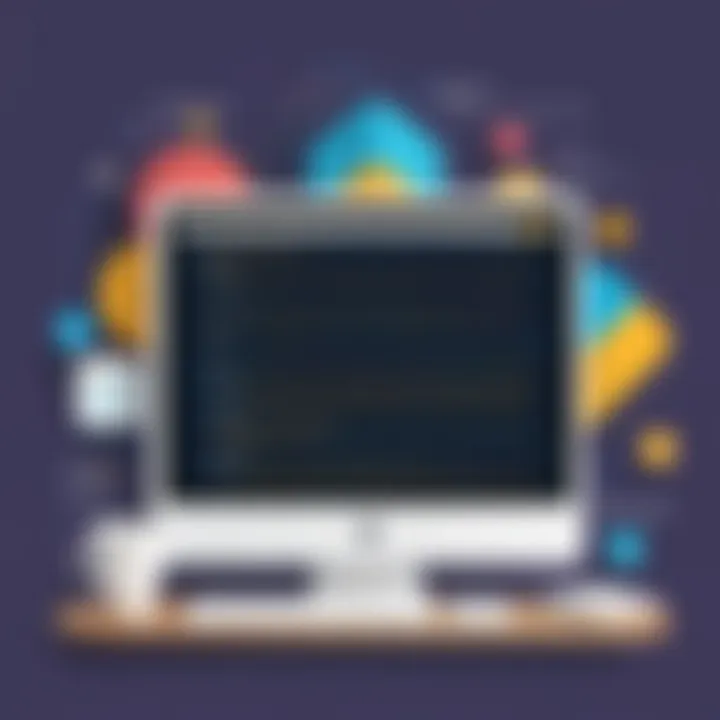
Closure Explained
Closures are a powerful feature in JavaScript that allow a function to access variables from its outer scope even after the outer function has finished executing. This concept contributes to data privacy and encapsulation, which are both important for creating robust applications.
In interviews, understanding closures is often tested through coding exercises that require candidates to maintain state or create factory functions. The interplay between inner and outer functions can be particularly beneficial in maintaining clean code.
Clear communication of how closures help manage state in JavaScript applications is advantageous during interviews, showcasing a candidate's depth of thought.
Event Loop Mechanism
The event loop mechanism is fundamental to JavaScript's non-blocking architecture, enabling asynchronous programming. It allows the execution of code, collecting and processing events, and executing queued sub-tasks all while maintaining a single-threaded event management process.
Understanding the event loop is crucial when dealing with asynchronous functions such as , , or even Ajax calls. This understanding can immensely improve task management and efficiency in applications, characteristics highly regarded during technical evaluations.
Candidates who can explain how the event loop processes tasks and its impact on performance demonstrate a solid understanding of JavaScript fundamentals.
Prototypal Inheritance
Prototypal inheritance is a distinct feature of JavaScript, which facilitates object behavior sharing without the use of classical inheritance patterns found in other programming languages. This characteristic allows objects to inherit properties and methods from other objects directly, creating a simpler object-oriented model.
This model can be less complex to understand than class-based inheritance, particularly for new developers. Interviewers often focus on this aspect, testing the ability to create flexible object structures that leverage prototypal inheritance effectively.
Being able to discuss benefits, such as dynamic object creation and modification, as well as potential challenges like debugging, is key when addressing this topic during an interview.
Practical Coding Challenges
Practical coding challenges are vital in the preparation for JavaScript interviews. They focus on applying theoretical knowledge to solve real-world problems. By engaging with these challenges, candidates enhance their problem-solving skills. They also learn to write efficient and clean code. This practical approach helps to bridge the gap between understanding concepts and actually using them in a working environment.
Moreover, these challenges reflect the scenarios one may encounter in a job position. Employers favor candidates who can think critically and translate abstract ideas into functional code. Therefore, practicing coding challenges is not just beneficial; it is essential for anyone looking to excel in a JavaScript interview.
Array Manipulation Challenges
Filtering Unique Values
Filtering unique values from an array is a common challenge within JavaScript. This task requires a clear understanding of how arrays function in JS. The process typically involves eliminating duplicate values while retaining the original order of the elements. This exercise is particularly insightful as it teaches developers about the methods available for array manipulation, such as and .
The key characteristic of filtering unique values is its practical application. It is a beneficial choice for an interview because it tests a candidate's ability to manipulate data effectively. The unique feature here lies in the array's mutability and the ability to transform it in a non-intrusive manner. This skill is crucial in many real-world applications. However, candidates must be cautious about performance issues, especially with large datasets due to the time complexity of different approaches.
Sorting Algorithms
Sorting algorithms are foundational in programming. They help in organizing data, which is a common necessity in software development. Understanding how to sort an array improves a programmer's ability to maintain data efficiently. By practicing sorting algorithms, candidates become familiar with various methods, such as bubble sort, quick sort, and merge sort.
The key characteristic of sorting algorithms is the ability to handle datasets with diverse types of information. They are a beneficial focus for interviews since they can indicate a candidate's logical thinking and problem-solving aptitude. A unique feature of these algorithms is their efficiency, as each algorithm has its own time complexity. While bubble sort might be simpler to understand, it is less efficient compared to quick sort in practical applications. Candidates should therefore weigh advantages and disadvantages when approaching sorting tasks.
Removing Duplicates
Removing duplicates from an array is an essential challenge that tests understanding of array operations. This task involves producing a new array that contains only unique items, leaving out any repetitions. This exercise highlights the importance of data cleanliness in software applications.
The ability to efficiently remove duplicates is a beneficial skill. It is critical for developers who work with large datasets and need to ensure data integrity. A unique feature of this challenge is the potential for using different techniques, such as the approach with combined with , or utilizing a more efficient . Each method comes with its own advantages, primarily in terms of code simplicity versus execution speed.
String Manipulation Challenges
Reversing Strings
Reversing strings is a simple yet effective coding challenge. This task is valuable because it requires an understanding of string operations and their manipulations. In interviews, efficient methods to reverse a string showcase a candidate's knowledge about JavaScript features.
The main characteristic of this challenge is its straightforwardness. Reversing a string serves as a beneficial choice for beginners, allowing them to grasp the fundamental mechanics of strings in JavaScript. A unique feature is the ability to use methods like , , and to achieve the task. While it's an easy challenge, candidates must ensure they handle edge cases such as empty strings or strings that are palindromes.
Character Frequency Counter
Creating a character frequency counter is a dynamic challenge that tests candidates' understanding of object manipulation. This challenge requires writing code that counts how many times each character appears in a string.
The key characteristic of this challenge lies in its ability to merge concepts from arrays and objects. It provides a beneficial opportunity to showcase skills in data structuring and algorithm design. A unique feature is the efficiency of the approach, as using an object to store results can lead to optimal solutions. This task can, however, become more complex with larger strings.
String Compression
String compression involves reducing the size of a string without losing information. This is a practical challenge that often comes up in interviews, as it embodies understanding compression algorithms and efficient data representation.
The main characteristic of string compression is its relevance in real-world applications, especially in optimizing storage. This challenge stands as a beneficial exercise in showcasing efficiency and logic. A unique feature is the implementation of algorithms to achieve the compression, which can vary in effectiveness. Candidates should be able to explain their approach and consider trade-offs between readability and performance.
Engaging in practical coding challenges not only enhances your JavaScript skills but also prepares you for the dynamic demands of the tech industry.
Scenario-Based Questions
Scenario-based questions are crucial in any technical interview, particularly for JavaScript developers. These questions assess not only a candidate's theoretical understanding of the language but also their practical problem-solving skills. Interviewers use these scenarios to evaluate how candidates apply JavaScript concepts to real-world situations, which is vital in a development environment.
The format of scenario-based questions allows interviewees to demonstrate their thought process and coding methods. This approach highlights a candidate's ability to troubleshoot, optimize, and think critically. Moreover, it gives insights into how they collaborate in a team setting, since developers often deal with complex problems collaboratively.
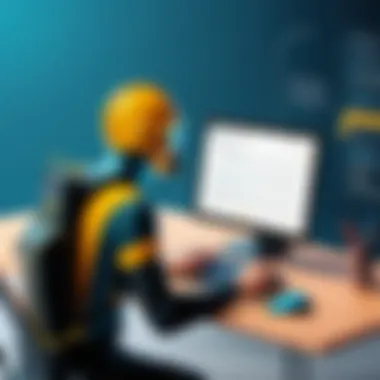
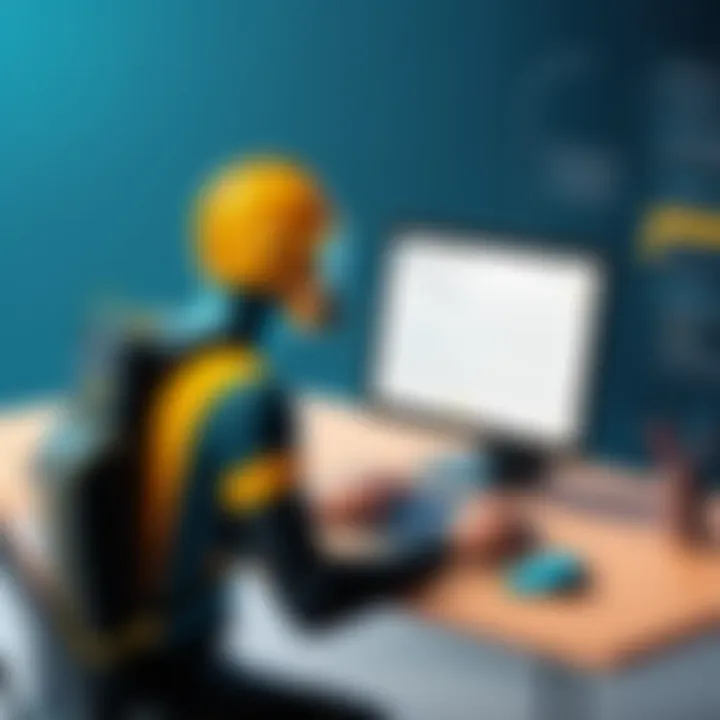
Debugging Scenarios
Identifying Common Errors
Identifying common errors is an essential skill for JavaScript developers. Errors in JavaScript can stem from various sources, such as syntax mistakes or logical errors. By sharpening this skill, candidates can quickly address issues during development instead of getting stalled by them. This not only saves time but also enhances overall productivity.
A key characteristic of identifying common errors is the focus on understanding error messages returned by the JavaScript engine. Recognizing what these messages mean can be very helpful. Because JavaScript is dynamically typed, developers often face unexpected data types, leading to common mistakes. Therefore, comprehending these fundamental errors is a beneficial choice in interview prep as it builds a strong foundation for troubleshooting.
The unique feature of this aspect lies in its practicality. Candidates are often required to demonstrate their debugging process. This process enhances their analytical abilities, presenting a distinct advantage in interviews. However, if not approached correctly, focusing solely on identifying errors without understanding their context can lead to incomplete solutions.
Using DevTools for Troubleshooting
Using DevTools for troubleshooting is an integral part of modern JavaScript development. Browsers like Chrome come equipped with built-in debugging tools that allow developers to inspect elements, view console messages, and analyze performance. This skill contributes directly to a developer's effectiveness in identifying issues and understanding application flow.
The key characteristic of using DevTools is its real-time ability to debug code. This method is popular because it allows developers to see the effects of their changes immediately. With features like breakpoints and watch expressions, candidates can explain their thought process clearly during interviews, showcasing their coding dexterity.
The unique feature of this tool is that it fosters a hands-on approach to debugging. Candidates can share their screen or describe their experience effectively, thus highlighting their efficiency. However, reliance on tools without foundational knowledge can lead to dependency, which may be a disadvantage during discussions about manual debugging techniques.
Error Handling Best Practices
Error handling best practices are pivotal for writing robust JavaScript applications. Developers need to anticipate possible errors and construct code that can handle them gracefully. This ability significantly contributes to the overall software quality, enhancing user experience and application stability.
A prominent characteristic of error handling is the use of techniques like try-catch blocks and custom error messages. Such practices are beneficial, as they help developers convey meaningful feedback. When candidates discuss these strategies in interviews, it shows they are prepared for unexpected outcomes.
The unique feature of focusing on best practices is that it illustrates a developer's commitment to quality coding standards. Good error handling enhances debugging and maintenance, but lack of proper implementation can make programs less resilient. This can reflect poorly in an interview setting, making it essential for every candidate to master this aspect.
Optimization Scenarios
Improving Performance of Functions
Improving performance of functions is vital for developers aiming to create efficient applications. Understanding how to optimize function performance is key to ensure applications run smoothly, especially during high-load scenarios. This contributes to overall application responsiveness and user satisfaction.
A key characteristic of this aspect is learning fundamental concepts like memoization or debouncing. Such techniques are popular because they can dramatically reduce unnecessary computations and enhance speed. Candidates who can discuss these strategies effectively demonstrate a deep understanding of how to optimize JavaScript code.
The unique feature lies in the ability to measure performance through various tools and methodologies. Candidates can showcase before-and-after scenarios to prove their strategies work, which serves as evidence of their analytical capabilities. However, oversimplifying performance improvements without justifying them can lead to misinformed conclusions during interviews.
Memory Leaks and Management
Memory leaks in JavaScript can degrade application performance significantly. Understanding how to manage memory effectively is essential, especially in long-running applications. This consideration contributes to overall development where smooth performance is expected.
A key characteristic of memory management is the focus on recognizing and solving memory leaks. Awareness of common pitfalls, such as closures or detached DOM elements, can prevent significant issues down the line. Learning to manage memory effectively is especially beneficial in interviews, as candidates can articulate their preventive strategies.
The unique feature of memory leak management is its relation to overall application efficiency. It's important for developers to demonstrate this knowledge through best practices. However, candidates who lack insight into identifying leaks may struggle to discuss this topic comprehensively in interviews.
Best Practices for Code Efficiency
Best practices for code efficiency encompass various strategies that enhance the maintainability and performance of code. Especially in JavaScript, where efficiency can be impacted by multiple factors, understanding these practices is essential.
A significant characteristic of this aspect is knowledge of clean coding principles along with patterns and architectures. These practices are widely known because they facilitate easier collaboration among teams. Candidates discussing these principles showcase a solid understanding of both aesthetics and functionality.
The uniqueness of this subject matter reveals how foundational skills translate into practical scenarios. When candidates can apply these practices, it indicates preparedness for real-world challenges. Nonetheless, an over-focus on best practices without practical application can leave candidates vulnerable during discussions of coding challenges.
In summary, scenario-based questions in JavaScript interviews play a vital role in assessing a candidate's problem-solving capabilities. Prospective developers must be equipped with not only theoretical knowledge but also practical skills to succeed in a competitive environment.
Epilogue and Further Resources
Summary of Key Learnings
Important Concepts Reviewed
The Important Concepts Reviewed section highlights the fundamental principles of JavaScript discussed throughout the article. Understanding the basics, such as data types, variable scope, and function contexts, is crucial for aspiring developers. These concepts form the backbone of JavaScript programming. Their significance lies in enabling developers to write clean, efficient code and adapt to various coding challenges. Moreover, reviewing these concepts reinforces key knowledge, ensuring retention and comprehension, essential for interview success.
Practice Recommendations
In the Practice Recommendations section, the focus is on consistent hands-on practice. This involvement is essential for mastering JavaScript. Engaging with coding challenges, such as those presented earlier in the article, helps to solidify understanding and improve problem-solving skills. Practical experience is arguably the most effective method to prepare for interviews, as it brings theory to life. Utilizing platforms like LeetCode or Codewars can greatly complement the learning experience and establish confidence in one’s capabilities.
Encouragement for Continuous Learning
The Encouragement for Continuous Learning section emphasizes the importance of ongoing education in technology. Given the rapidly evolving nature of programming languages and frameworks, developers must stay informed. Engaging with new materials—be it through online resources, books, or workshops—keeps skills relevant and sharp. Continuous learning fosters adaptability, which is essential in both interviews and practical work environments.
Books and Online Resources
Recommended Literature
The Recommended Literature for JavaScript is not just informative but also essential for in-depth understanding. Titles like "Eloquent JavaScript" by Marijn Haverbeke offer structured insights into both basic and advanced concepts. Such resources provide readers with comprehensive explanations and practical examples that enhance clarity. These texts are valuable because they blend theory with practical application, making them a solid choice for anyone serious about mastering JavaScript.
Online Courses and Tutorials
Participation in Online Courses and Tutorials can provide structured learning experiences tailored to various skill levels. Platforms such as Udemy, Coursera, or freeCodeCamp offer courses that cover everything from the basics to advanced topics. The unique aspect of these platforms is their interactive nature, enabling learners to immediately apply what they learn. This approach integrates theory and practice in ways that books alone cannot, giving learners valuable reassurance as they prepare for assessments.
Community Forums for Support
The final element, Community Forums for Support, plays a crucial role in fostering a supportive learning atmosphere. Platforms like Reddit and Stack Overflow allow learners to ask questions, share experiences, and connect with peers. The key characteristic of these forums is the wealth of knowledge shared by community members. Assistance from experienced developers offers nuanced insights that textbooks may overlook. Thus, leveraging community support can greatly enhance your learning journey and provide encouragement during challenging times.